Plot Time Series Python
Time series data refers to a collection of data points that are recorded over regular intervals of time. In this type of data, each observation is associated with a specific timestamp, allowing analysts to discover patterns and trends that evolve over time. Time series data is widely used in various fields, including finance, economics, weather forecasting, and sales forecasting.
Characteristics of Time Series Data:
1. Time Dependence: Time series data exhibits a temporal ordering, where the order of the observations matters. The values recorded at one time point can influence those recorded at subsequent time points.
2. Trend: Trends represent the long-term variation in the data over time. They can be upward (indicating growth) or downward (indicating decline).
3. Seasonality: Seasonality refers to patterns that repeat at fixed intervals within a time series. For example, sales of sunscreen tend to increase during the summer months.
4. Cyclicity: Cyclicity represents patterns that occur over an extended period but lack fixed intervals. These patterns are different from seasonality as they do not follow a specific time frame.
5. Irregularity: Irregular or random fluctuations occur due to unpredictable factors and noise. These fluctuations can be caused by unexpected events, errors, or random variation.
Importance of Analyzing Time Series Data in Python:
Analyzing time series data using Python provides several advantages:
1. Powerful Libraries: Python offers various powerful libraries, such as Pandas and NumPy, which provide numerous tools and functions specifically designed for time series analysis. These libraries simplify data manipulation, visualization, and modeling tasks.
2. Data Preprocessing: Python libraries provide robust features for data preprocessing, including cleaning the data, handling missing values, and transforming the data into a suitable format for analysis. These techniques are essential for ensuring accurate and reliable results.
3. Data Visualization: Python libraries like Matplotlib, Seaborn, and Pandas offer extensive visualization capabilities to plot and explore time series data. Visualizing data allows analysts to identify trends, seasonal patterns, and anomalies, aiding in the understanding and interpretation of the data.
4. Forecasting and Predictive Modeling: Time series analysis in Python enables the development of accurate forecasting models, enabling businesses to predict future trends, make informed decisions, and optimize their operations. Python libraries like StatsModels, scikit-learn, and TensorFlow provide a wide range of forecasting algorithms and techniques.
Basic Terminologies Related to Time Series Analysis:
1. Timestamp: A timestamp represents a specific point in time. It can be in the form of a calendar date, time of day, or a combination of both.
2. Time Interval: The time interval refers to the duration between two consecutive timestamps in a time series. It can be constant or irregular depending on the data.
3. Frequency: Frequency determines the number of observations that occur within a specific time interval. It can be hourly, daily, monthly, or any other defined interval.
4. Lag: Lag represents the time period between an observation and its preceding or succeeding observation. Lagging a time series allows for the identification of patterns and relationships.
5. Autocorrelation: Autocorrelation measures the correlation between a time series and a lagged version of itself. It helps identify dependency and patterns within the time series data.
6. Seasonality: Seasonality refers to patterns that repeat at fixed intervals within a time series, such as daily, weekly, or yearly.
7. Exogenous Variables: Exogenous variables are external factors that influence the behavior of a time series but are not dependent on it. They can be included in forecasting models to improve accuracy.
Loading and Exploring Time Series Data in Python
Python provides powerful libraries like Pandas and NumPy to load and analyze time series data efficiently. Here are the steps to load and explore time series data in Python:
1. Importing Libraries:
To start, import the required libraries:
import pandas as pd
import numpy as np
2. Loading Time Series Data:
Use the Pandas library to load time series data:
data = pd.read_csv(‘time_series_data.csv’)
The ‘read_csv’ function reads the data from a CSV file and stores it in a Pandas DataFrame.
3. Data Preprocessing:
Perform data preprocessing techniques to ensure data quality:
– Data Cleansing: Remove irrelevant or inconsistent data, such as duplicates or outliers, to ensure data accuracy.
– Handling Missing Values: Address missing values in the data by either removing the respective rows or filling them with appropriate values using techniques like forward or backward filling or interpolation.
4. Visualizing Time Series Data:
Visualize the loaded time series data using various plots:
– Line Plots: Create a line plot to visualize the overall trend and patterns in the data over time.
– Scatter Plots: Use scatter plots to identify the relationships and correlations between different variables in the time series.
– Histograms: Create histograms to analyze the distribution of values in the time series.
Time Series Decomposition and Trend Analysis
Time series decomposition involves breaking down a time series into its key components, including trend, seasonality, and residual (randomness). Trend analysis focuses on identifying and characterizing the long-term change patterns in the time series. Here are the steps to perform time series decomposition and trend analysis in Python:
1. Decomposing Time Series:
Decompose the time series into its components using library functions like seasonal_decompose from statsmodels library:
from statsmodels.tsa.seasonal import seasonal_decompose
result = seasonal_decompose(data, model=’additive’)
trend = result.trend
seasonal = result.seasonal
residual = result.resid
The seasonal_decompose function breaks down the time series into trend, seasonal, and residual components.
2. Trend Analysis:
Perform trend analysis using techniques like moving averages and exponential smoothing:
– Moving Averages: Calculate the moving averages to smoothen out the fluctuations and estimate the underlying trend. Use functions like rolling_mean from Pandas:
rolling_mean = data[‘value’].rolling(window=3).mean()
– Exponential Smoothing: Apply exponential smoothing techniques to assign different weights to the observations and highlight the underlying trend:
from statsmodels.tsa.api import ExponentialSmoothing
model = ExponentialSmoothing(data[‘value’], trend=’add’, seasonal=None)
fitted_model = model.fit()
trend = fitted_model.fittedvalues
The ExponentialSmoothing class from the statsmodels library provides methods to perform exponential smoothing operations.
3. Detecting and Handling Outliers:
Detect and handle outliers in the time series data using statistical techniques like Z-score or by visual inspection. Remove or replace the outliers to avoid distortion of the data and maintain the accuracy of the analysis.
Time Series Forecasting Techniques
Time series forecasting refers to predicting future values based on historical data. Python provides various techniques and libraries for time series forecasting. Here are the steps to perform time series forecasting in Python:
1. Overview of Forecasting Methods:
Understand different forecasting methods, including ARIMA (Autoregressive Integrated Moving Average), exponential smoothing, and LSTM (Long Short-Term Memory) neural networks. Each method has its strengths and limitations, making it suitable for specific time series data patterns.
2. Building and Training Forecasting Models:
Utilize Python libraries like StatsModels, scikit-learn, or TensorFlow to build and train forecasting models:
– ARIMA: Build an ARIMA model using the ARIMA class from the statsmodels library:
from statsmodels.tsa.arima_model import ARIMA
model = ARIMA(data[‘value’], order=(1, 1, 1))
fitted_model = model.fit()
– Exponential Smoothing: Train an exponential smoothing model using the ExponentialSmoothing class from the statsmodels library (as shown in the trend analysis section).
– LSTM: Implement an LSTM forecasting model using libraries like TensorFlow or Keras:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
model = Sequential()
model.add(LSTM(32, activation=’relu’, return_sequences=True, input_shape=(n_steps, n_features)))
model.add(LSTM(32, activation=’relu’))
model.add(Dense(1))
model.compile(optimizer=’adam’, loss=’mse’)
model.fit(X_train, y_train, epochs=10, batch_size=32)
Replace “n_steps” and “n_features” with appropriate values based on the input and output shapes of the LSTM model.
3. Evaluating Model Performance:
Evaluate the performance of the forecasting models using metrics like Root Mean Square Error (RMSE), Mean Absolute Error (MAE), and Mean Absolute Percentage Error (MAPE). Comparing these metrics helps select the best model for accurate predictions.
Advanced Time Series Analysis
Advanced time series analysis techniques are useful for handling more complex time series data. Here are some important concepts for advanced time series analysis in Python:
1. Stationarity:
Ensure that the time series data is stationary, meaning its statistical properties like mean, variance, and autocorrelation remain constant over time. Stationarity is crucial for accurate forecasting and modeling. Techniques like differencing, detrending, and logarithmic transformations can be used to make the data stationary.
2. Autocorrelation and Partial Autocorrelation:
Perform autocorrelation and partial autocorrelation analysis to identify the lagged dependencies and relationships between observations in the time series. This analysis helps determine the orders of the AR (autoregressive) and MA (moving average) components in ARIMA modeling.
3. ARIMA and SARIMA Models:
ARIMA (Autoregressive Integrated Moving Average) and SARIMA (Seasonal ARIMA) models are powerful for analyzing and forecasting complex time series data. They consider the autocorrelation and seasonal patterns within the data. Python libraries like statsmodels provide functions to build and analyze ARIMA and SARIMA models.
Beyond Basics: Seasonality and Exogenous Variables
To further enhance time series analysis and forecasting, consider seasonality and exogenous variables. Here are some techniques:
1. Identifying and Handling Seasonality:
Identify and handle seasonality in time series data using techniques like:
– Seasonal Decomposition of Time Series: Decompose the time series into its seasonal component to identify and analyze the periodic patterns.
– Fourier Transformation: Apply Fourier transformation to convert the time series data into its frequency domain. This helps identify the dominant frequencies and their corresponding seasonal components.
2. Incorporating Exogenous Variables:
Include exogenous variables in time series models to improve forecasting accuracy. Exogenous variables are external factors that influence the behavior of the time series. For example, incorporating weather data in sales forecasting can account for the impact of weather conditions on consumer behavior.
3. ARIMAX Models:
ARIMAX (Autoregressive Integrated Moving Average with Exogenous Variables) models combine the ARIMA model with exogenous variables. They account for both the endogenous time-dependent behavior and the exogenous influences to make accurate forecasts. The statsmodels library in Python provides functionality for building ARIMAX models.
FAQs:
Q: How can I plot time series data using Pandas?
A: To plot time series data using Pandas, you can utilize the plot() function on a Pandas DataFrame or Series. For example, if you have a DataFrame named “data” with a “date” column and a “value” column, you can plot it using: data.plot(x=’date’, y=’value’).
Q: How can I plot a time series by date in Python?
A: To plot a time series by date in Python, you can convert the “date” column to the datetime format using Pandas’ to_datetime function. Then, set the “date” column as the index of the DataFrame or Series before plotting. For example: data[‘date’] = pd.to_datetime(data[‘date’]) data.set_index(‘date’).plot().
Q: Are there any specific libraries in Python for time series plotting?
A: Yes, there are several libraries in Python for time series plotting. Some commonly used ones include Matplotlib, Seaborn, and Pandas. These libraries provide numerous functionalities to visualize time series data, including line plots, scatter plots, histograms, and more.
Q: How can I create real-time time series plots that update automatically in Python?
A: To create real-time time series plots that update automatically, you can use libraries like Matplotlib or Plotly. They provide functionalities to continuously update the plot as new data becomes available. You can use techniques like animation or dynamic plotting to achieve real-time updates.
Q: How can I plot a time series with datetime values on the x-axis?
A: To plot a time series with datetime values on the x-axis, ensure that the “datetime” column is in the datetime format. Then, set the “datetime” column as the x-axis while plotting using the specific plotting function of the chosen library. For example, with Matplotlib, use: plt.plot(data[‘datetime’], data[‘value’])
#14 Time Series Data Visualization In Python | Analyze Financial Data | Matplotlib Tutorial 2021
Keywords searched by users: plot time series python Plot time series pandas, Plot by date python, Time series plot, Seaborn time series Plot, Matplotlib realtime plot update, Plot datetime, Pandas time series, Line plot Python
Categories: Top 18 Plot Time Series Python
See more here: nhanvietluanvan.com
Plot Time Series Pandas
Time series data refers to a sequence of observations collected at regular intervals over time. These observations could represent stock prices, weather measurements, population growth, or any other data that is recorded over a specific time period. Plotting time series data allows us to gain insights into trends, patterns, and seasonality, making it a crucial step in analyzing and interpreting temporal data.
To get started with plotting time series data in pandas, we first need to ensure that our data is properly formatted. Pandas provides a specialized data structure called a DataFrame, which is ideal for handling time series data. A DataFrame consists of labeled columns and rows, where each column represents a variable, and each row represents an observation at a specific time.
To load time series data into pandas, we typically start with a CSV (comma-separated values) file, which contains our data in tabular format. We can use the `read_csv()` function to read the CSV file into a DataFrame. Once the data is loaded, we can convert the appropriate column into a datetime type using the `to_datetime()` function. This step is crucial for pandas to recognize the column as a time series index.
Now that we have our time series data stored in a DataFrame, we can begin plotting. Pandas offers various plotting functions to visualize time series data, including `plot()`, `plot.scatter()`, `plot.bar()`, `plot.pie()`, and more.
One of the most common plots is a line plot, which displays the values of a variable over time. We can use the `plot()` function by specifying the x-axis and y-axis variables. For example, to plot a time series of stock prices, we can use the following code:
“`python
import pandas as pd
df = pd.read_csv(‘stock_prices.csv’)
df[‘Date’] = pd.to_datetime(df[‘Date’])
df = df.set_index(‘Date’)
df[‘Close’].plot()
“`
This code snippet reads the stock price data into a DataFrame, converts the `’Date’` column to a datetime index, and then plots the `’Close’` variable against the time series index using `plot()`. The resulting plot will show the trend of the closing prices over time.
Apart from line plots, pandas also provides other plot types, such as scatter plots and bar plots, which can be useful for visualizing relationships and distributions within time series data. For instance, we can use the `plot.scatter()` function to create a scatter plot of two variables, given a time series index:
“`python
df.plot.scatter(x=’Temperature’, y=’Energy Consumption’)
“`
This code snippet plots the `’Temperature’` variable against the `’Energy Consumption’` variable using a scatter plot. By examining the plot, we can observe any potential relationship or correlation between temperature and energy consumption over time.
Customization is another important aspect when plotting time series data. Pandas provides an extensive set of parameters to modify the appearance, style, and labeling of plots. These parameters include linewidth, linestyle, color, marker, title, axis labels, and much more. Additionally, pandas integrates seamlessly with other visualization libraries like Matplotlib, allowing further customization by leveraging its advanced functionalities.
Now, let’s address some frequently asked questions when working with time series plotting in pandas:
**Q: Can I plot multiple time series on the same plot?**
A: Yes, pandas allows you to plot multiple time series on the same plot by specifying additional variables. For example, to plot the closing prices of multiple stocks:
“`python
df[[‘Stock1’, ‘Stock2’, ‘Stock3’]].plot()
“`
**Q: How can I visualize seasonality in time series data?**
A: Pandas offers various techniques to visualize seasonality, such as by using the `seasonal_decompose()` function from the `statsmodels` library. This function decomposes a time series into its trend, seasonality, and residual components, allowing you to examine each component separately.
**Q: Can I plot aggregated values over a specific time period, like monthly averages?**
A: Yes, pandas provides the `resample()` function to compute aggregated values over different time intervals. You can combine `resample()` with aggregation functions like `mean()`, `sum()`, `min()`, etc., to calculate aggregated values and then plot them.
**Q: How can I add labels and annotations to my time series plot?**
A: Pandas allows you to add labels and annotations using the `plt` module from Matplotlib. You can access the underlying Matplotlib axes object using the `axes()` function and then use methods like `set_xlabel()`, `set_ylabel()`, `annotate()`, etc., to add labels and annotations to your plot.
In conclusion, plotting time series data in pandas offers a versatile and powerful way to analyze and visualize temporal patterns. By utilizing the various plotting functions and customization options, we can gain valuable insights into trends, relationships, and seasonality within our time series data. Whether you are an analyst, data scientist, or researcher, pandas provides the tools necessary to explore, interpret, and communicate your findings effectively.
Plot By Date Python
Before we dive into the code, let’s briefly discuss the importance of plotting data by date. Time-series data often exhibits patterns and trends that are dependent on the time component. By visualizing the data over time, you can easily identify regularities, patterns, and anomalies that might not be apparent from examining the raw numbers alone. This visual representation can help in making predictions, detecting seasonality, or identifying any unusual behavior in the data.
Python provides several libraries to help you plot data by date, such as Matplotlib, Seaborn, and Plotly. In this article, we will focus on using Matplotlib, one of the most commonly used plotting libraries in Python.
To get started, let’s assume that you have a dataset with date and corresponding values. The date can be in various formats, such as YYYY-MM-DD, DD/MM/YYYY, or even as a timestamp. We will use the datetime module in Python to handle the dates and Matplotlib to plot the data.
First, we need to import the necessary libraries:
“`
import matplotlib.pyplot as plt
from datetime import datetime
“`
Next, let’s define some sample data to plot. For the sake of simplicity, let’s consider a dataset with monthly temperature readings for a particular location. We will assume that the dataset is in the format YYYY-MM, where the year and month are separated by a hyphen. Here’s an example of how the data might look:
“`
dates = [‘2019-01’, ‘2019-02’, ‘2019-03’, ‘2019-04’, ‘2019-05’]
temperatures = [10, 12, 15, 18, 20]
“`
Now, we need to convert the date strings into datetime objects:
“`
converted_dates = [datetime.strptime(date, ‘%Y-%m’) for date in dates]
“`
The `strptime` function from the datetime module allows us to parse the date string into a datetime object using the specified format. In this case, `%Y-%m` corresponds to the year and month format.
Once we have the converted dates, we can proceed to plot the data using Matplotlib:
“`
plt.plot(converted_dates, temperatures)
plt.xlabel(‘Date’)
plt.ylabel(‘Temperature (°C)’)
plt.title(‘Monthly Temperature’)
plt.show()
“`
The `plot` function from the `plt` module creates a line plot with the converted dates on the x-axis and the corresponding temperatures on the y-axis. The `xlabel`, `ylabel`, and `title` functions are used to label the axes and title the plot. Finally, the `show` function displays the plot.
This is a basic example of how to plot data by date in Python using Matplotlib. However, there are many customization options available to enhance the visuals and make the plots more informative.
**Frequently Asked Questions (FAQs)**
**Q1: Can I plot data with different time intervals, such as hourly or weekly data?**
A1: Absolutely! The same principles can be applied to plot data with different time intervals. You would need to adjust the datetime format accordingly. For example, for hourly data with the format YYYY-MM-DD HH:MM, you would use `%Y-%m-%d %H:%M`.
**Q2: Can I plot multiple time-series data on the same plot?**
A2: Yes, you can plot multiple time-series data on the same plot by calling the `plot` function multiple times for each dataset and customizing the color or style of each line.
**Q3: How can I add markers or change the line style in the plot?**
A3: You can pass additional arguments to the `plot` function to customize the line style, markers, or colors. For example, `plt.plot(converted_dates, temperatures, marker=’o’, linestyle=’–‘, color=’r’)` will plot the data with red dashed lines and circular markers.
**Q4: Is it possible to add legends to the plot?**
A4: Yes, you can add legends to the plot using the `legend` function. You need to provide a label for each dataset and call the `legend` function after plotting the data. Here’s an example: `plt.legend([‘Dataset 1’, ‘Dataset 2’])`.
**Q5: Are there any other libraries apart from Matplotlib for plotting data by date?**
A5: Yes, Python offers several other libraries for plotting time-series data, such as Seaborn, Plotly, and Bokeh. Each library has its own strengths and can be used depending on your specific requirements.
Plotting data by date in Python can be an invaluable tool for analyzing and visualizing time-series data. It allows us to uncover patterns, trends, and anomalies that may not be apparent from the raw data. With the help of libraries like Matplotlib, you have the flexibility to customize the plots and make them more informative. By harnessing the power of Python and these libraries, you can gain deep insights into your time-series data and make better-informed decisions.
Time Series Plot
Introduction:
In the world of data analysis, time series plot stands as a powerful tool for visualizing data that evolves over time. From financial markets to climate patterns, time series data holds countless insights waiting to be uncovered. In this article, we will explore the intricacies of time series plot, understand its importance, and discover how it can be used to uncover trends, patterns, and seasonalities in data.
Understanding Time Series Plot:
A time series plot is a graphical representation of data collected at regular intervals over a defined period. It allows us to visualize how a variable changes over time, providing a valuable means of discovering underlying patterns, trends, and seasonal variations. By plotting data points along the y-axis against corresponding time intervals on the x-axis, a time series plot provides a clear picture of how a certain phenomenon has evolved over time.
The Importance of Time Series Plot:
Time series plots have several valuable applications across various domains. Economists, for instance, rely on time series analysis to study the behavior of financial markets and forecast future trends. Climate scientists use time series plots to analyze temperature fluctuations and identify long-term patterns. In healthcare, time series plots can be used to monitor patients’ vitals and track the progress of medical treatments. Moreover, businesses employ time series plots to analyze sales patterns, optimize inventory management, and plan marketing strategies. Thus, it becomes evident that time series plots play a vital role in understanding and predicting real-world phenomena.
Identifying Trends:
One of the key benefits of time series plots is their ability to uncover trends in data. A trend refers to the long-term movement of a variable over time. By observing the slope of the line connecting the data points on a time series plot, it becomes possible to determine whether the variable is experiencing an upward trend, a downward trend, or remains relatively constant. Trends can shed light on underlying phenomena and help forecast future outcomes.
Detecting Seasonalities:
Another important aspect of time series plots is their ability to detect seasonal patterns. Many variables exhibit periodic fluctuations influenced by seasonal factors. For example, sales of sunscreen tend to increase during the summer months, while sales of winter clothing rise during the colder seasons. By observing recurring patterns in a time series plot, analysts can identify seasonalities and take appropriate actions accordingly. Seasonal adjustments are often made to strip out the seasonal effects and better understand the underlying long-term trends.
Uncovering Patterns:
Time series plots can also reveal complex patterns that might be hidden in raw data. Irregularly spaced data points can be connected in a smooth curve, allowing the identification of recurrent cycles, oscillations, and irregularities. Patterns may indicate periodic behaviors, abrupt shifts, or the presence of outliers. Uncovering such patterns is crucial for making accurate predictions and informed decisions.
FAQs:
Q1. How should I select the time interval for plotting a time series?
A1. The time interval depends on the nature of the data and the insights you aim to gain. For instance, for daily stock market data, plotting each day’s closing price could be useful. However, for macroeconomic variables, you may consider monthly or quarterly intervals for a broader perspective.
Q2. What software/tools can I use to create time series plots?
A2. There are numerous software options available for creating time series plots, including popular data analysis tools like Python’s Matplotlib, R’s ggplot2, and Microsoft Excel. These tools offer various customization options to enhance the visual representation of time series data.
Q3. Is it possible to forecast future values using time series plots alone?
A3. Time series plots are helpful in identifying trends, patterns, and seasonalities, but they do not provide direct forecasting abilities. To make accurate predictions, time series analysis techniques such as autoregressive integrated moving average (ARIMA) or exponential smoothing (ETS) models can be employed.
Q4. How can I handle missing data in time series analysis?
A4. Missing data is a common challenge in time series analysis. If missing values are sporadic, interpolation techniques like linear interpolation or using rolling averages can be used to estimate the missing points. However, if there are large intervals of missing data, more advanced techniques like multiple imputation or state-space models may be necessary.
Q5. Are there any limitations to using time series plots?
A5. While time series plots are powerful tools, they do have limitations. Time series plots assume that the observations are taken at regular intervals and that past patterns will continue to hold in the future. Additionally, they may not adequately capture abrupt changes caused by external events, and hence, analysts need to complement them with other techniques for a comprehensive analysis.
Conclusion:
Time series plots are invaluable tools for analyzing and interpreting data that evolves over time. By visualizing trends, patterns, and seasonalities, these plots assist in understanding the past, present, and future behavior of various variables. Whether used for economic forecasts, climate analysis, or business optimization, time series plots offer unique insights that drive informed decision-making. So, harness the power of time series plots, and unlock the hidden secrets within your data.
Images related to the topic plot time series python
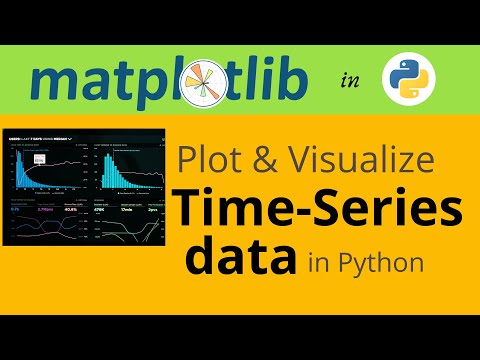
Found 50 images related to plot time series python theme
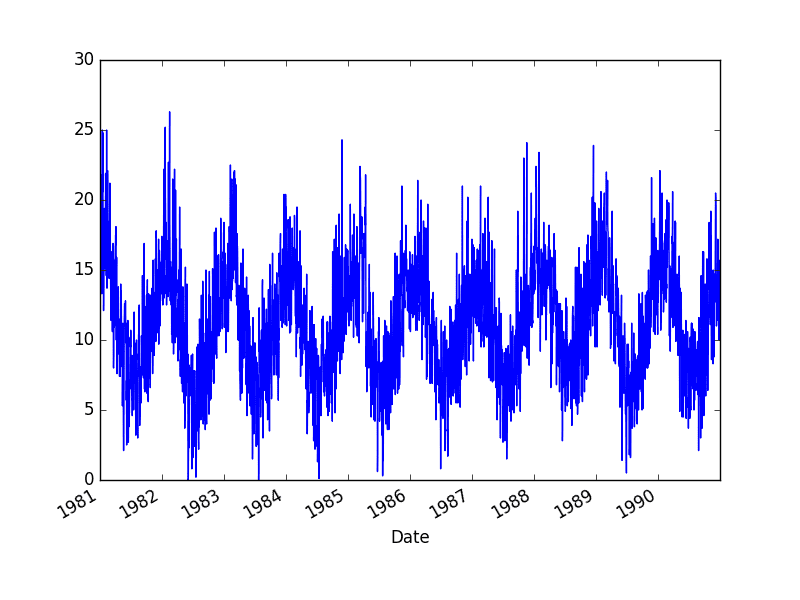
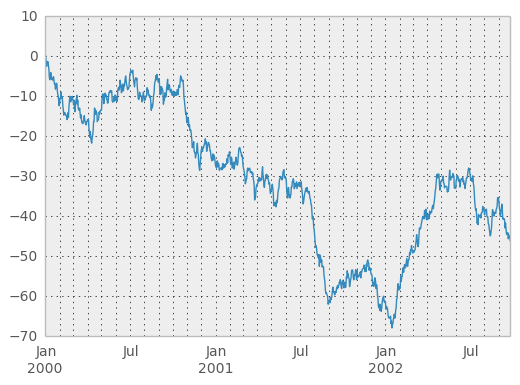
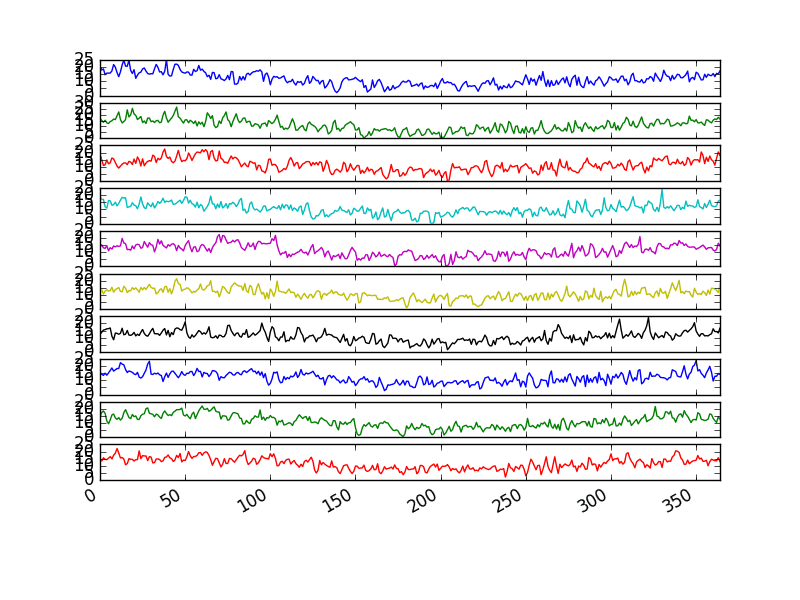
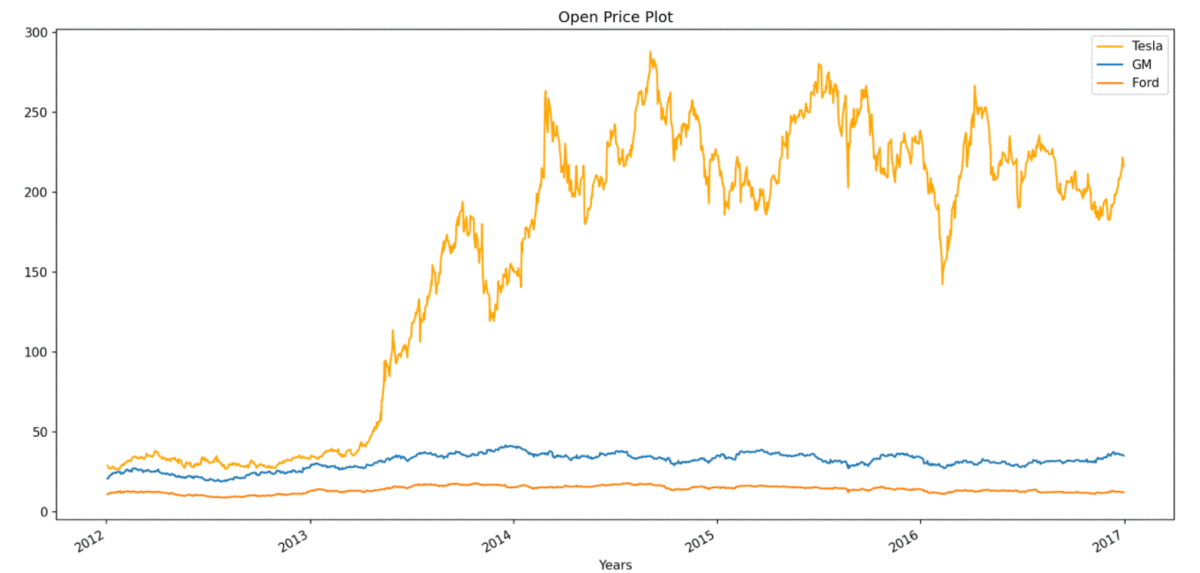


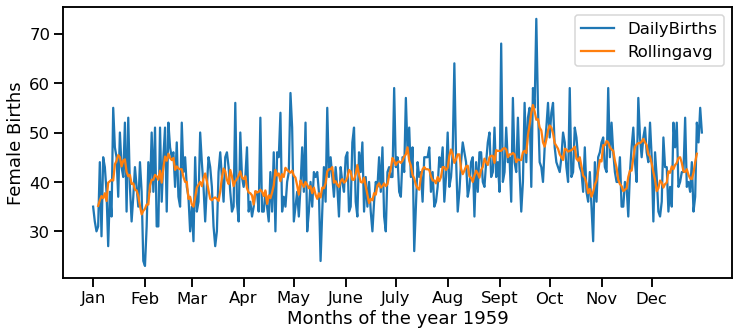


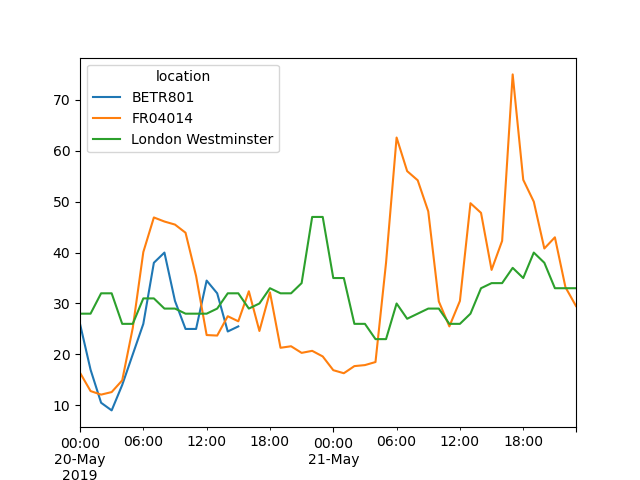

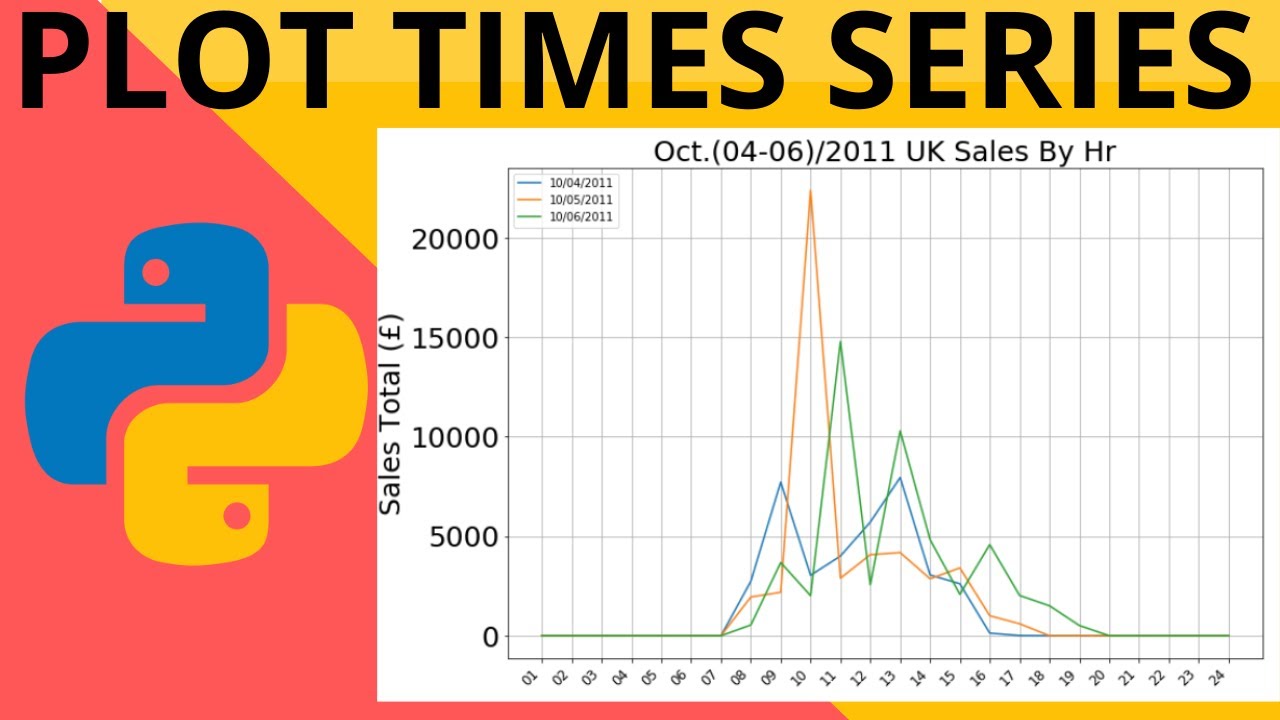


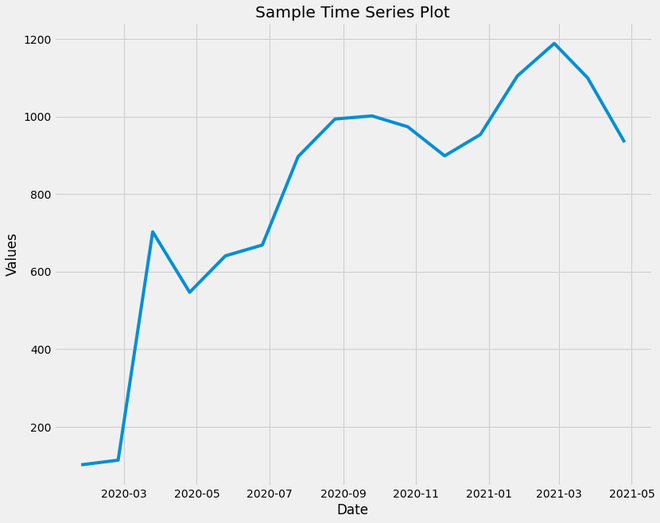

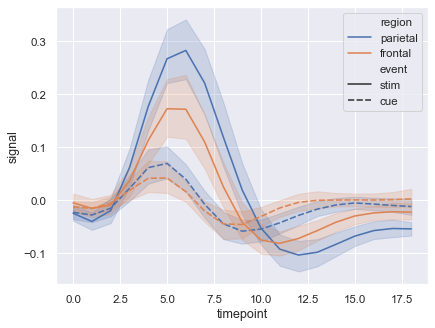
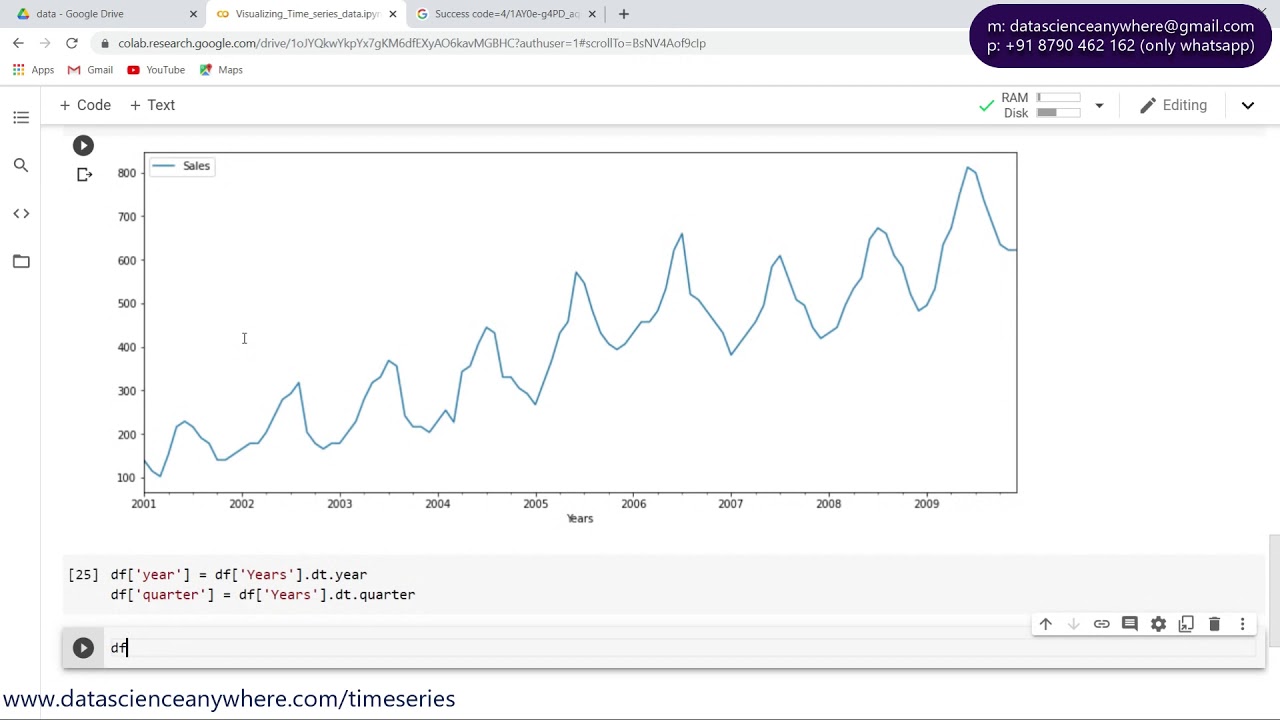

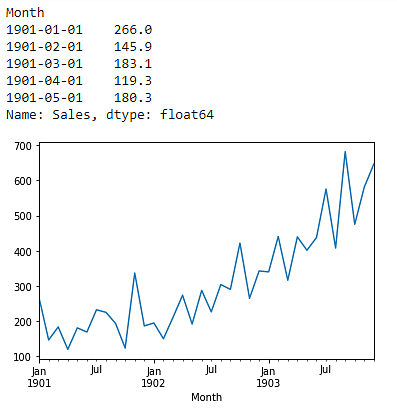


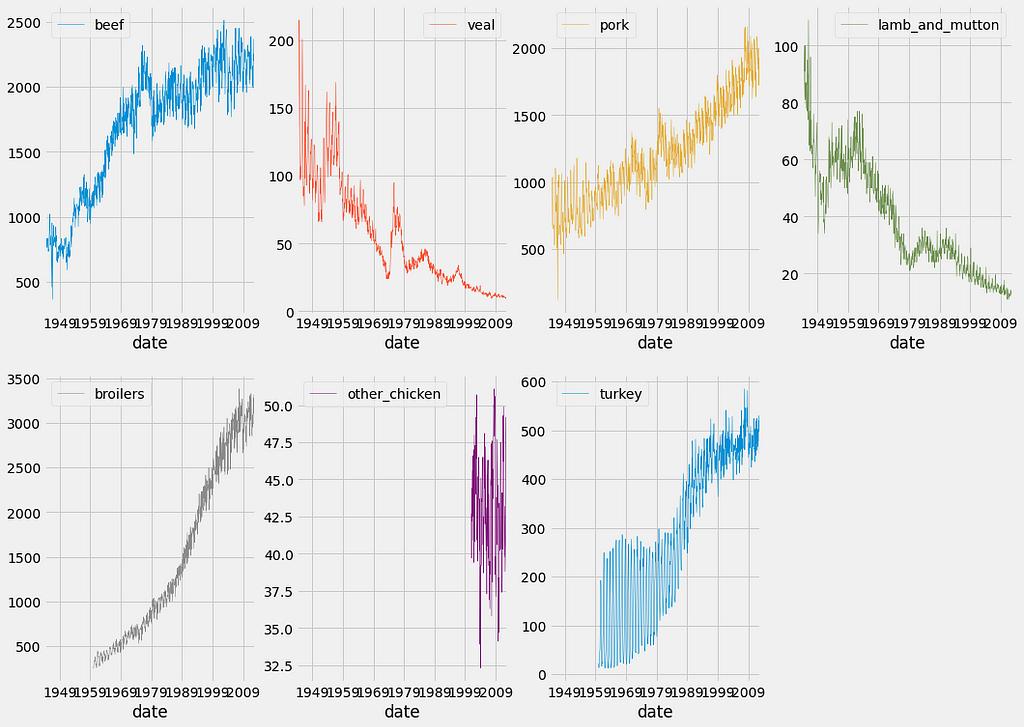
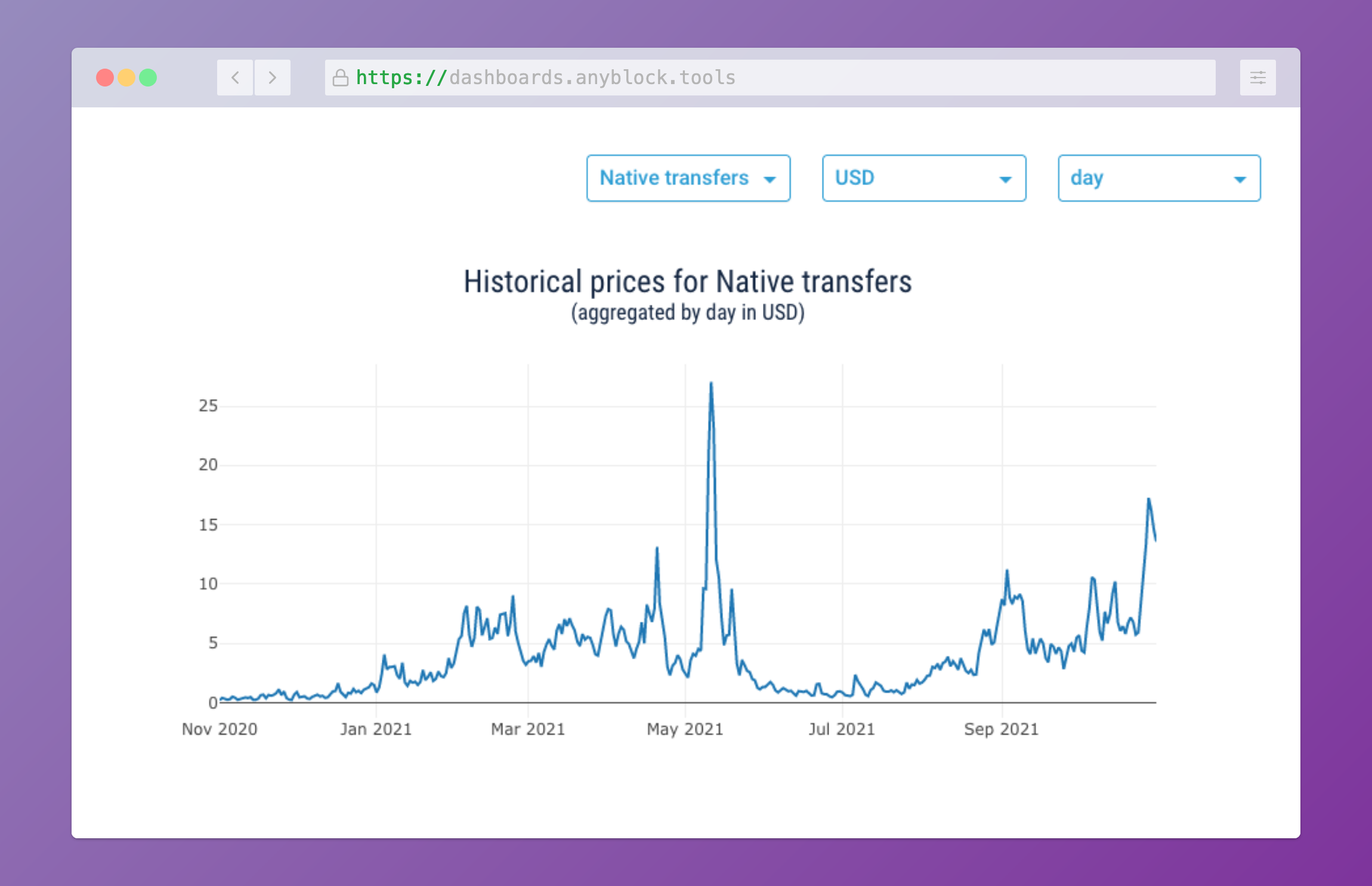

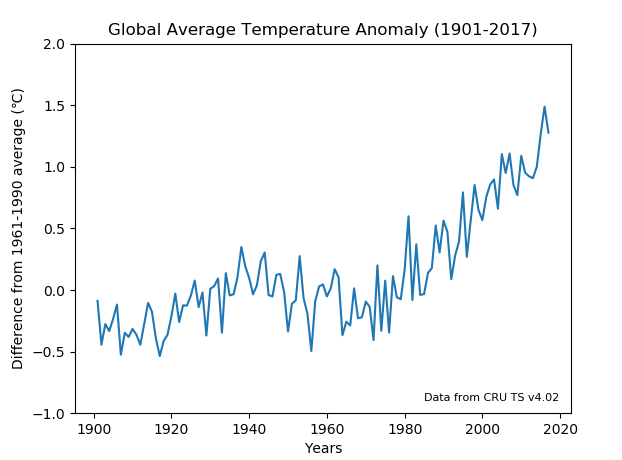


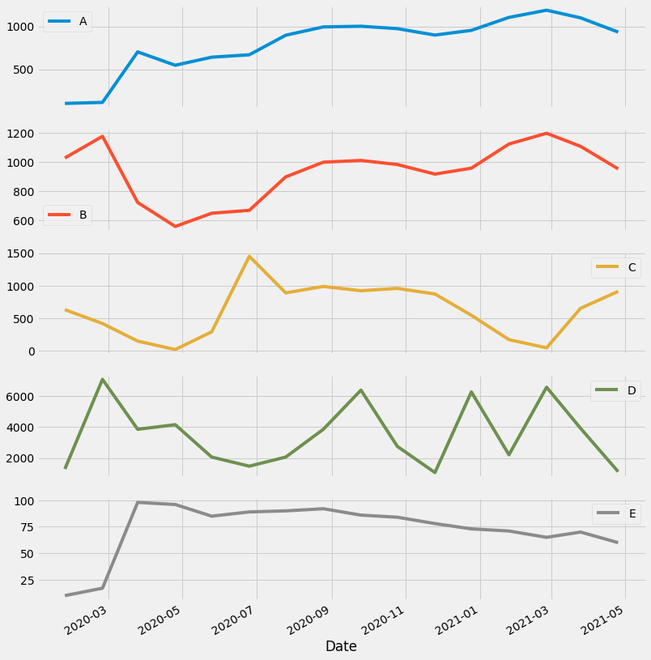
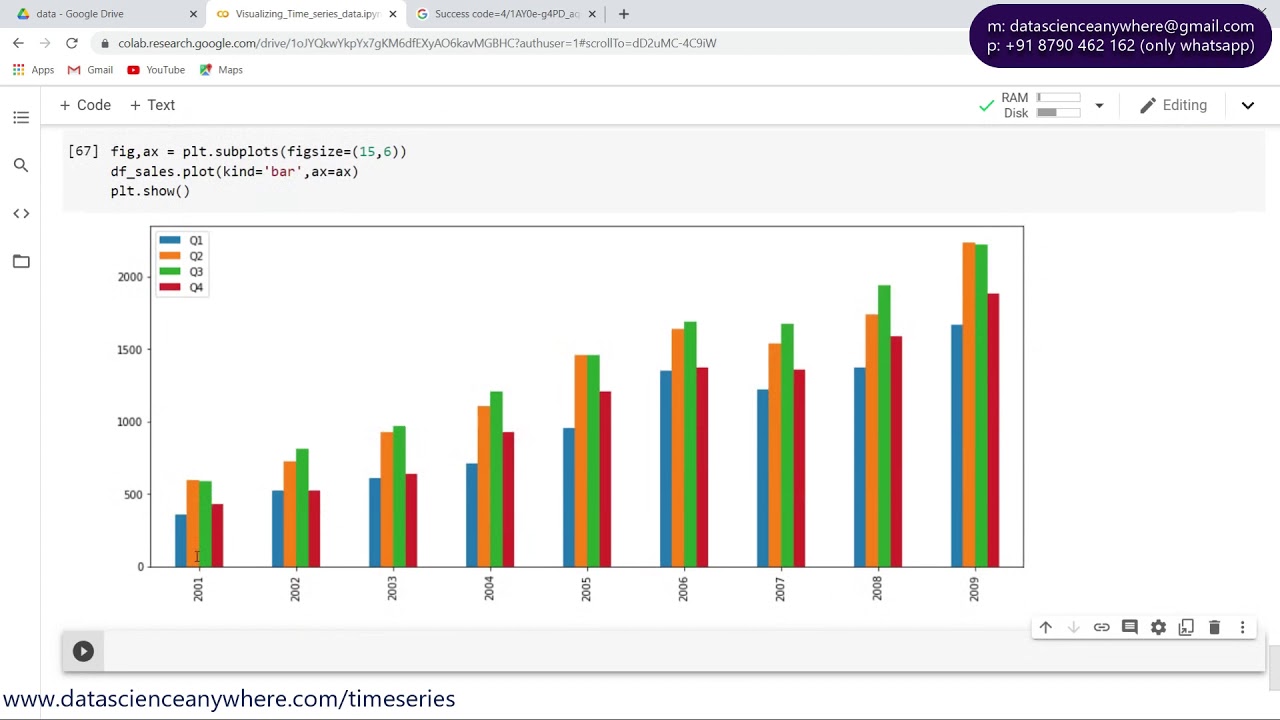
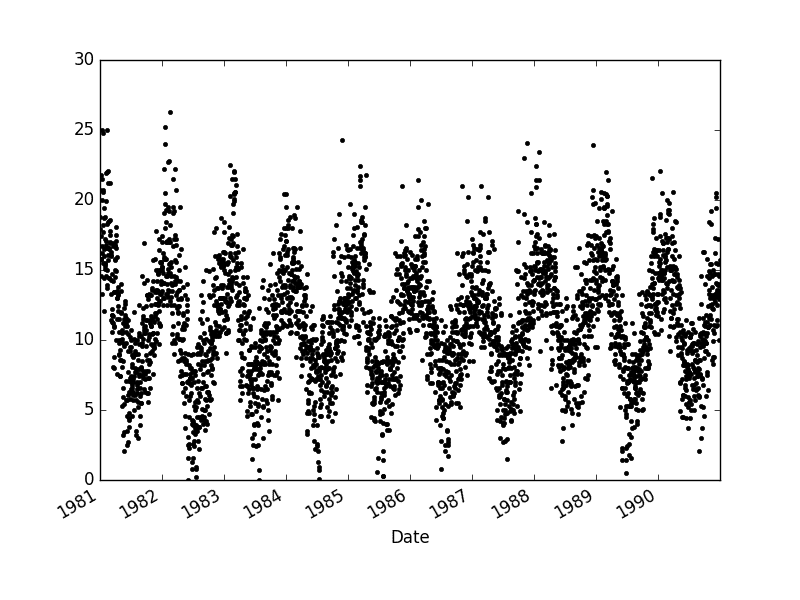
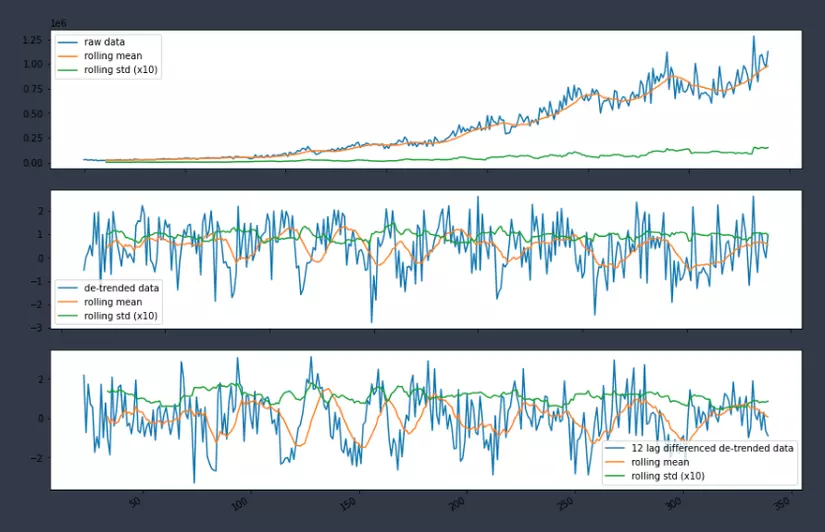
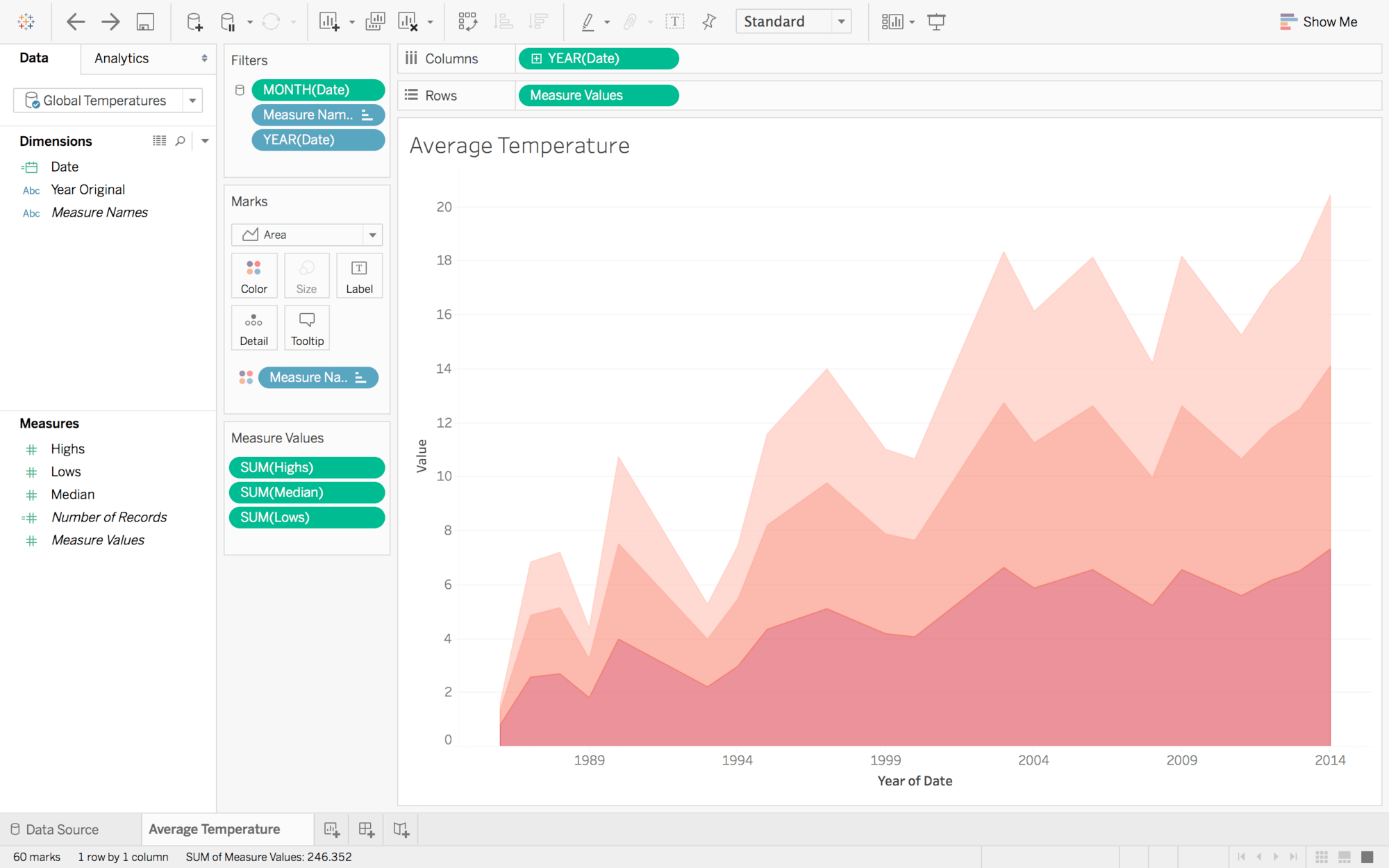
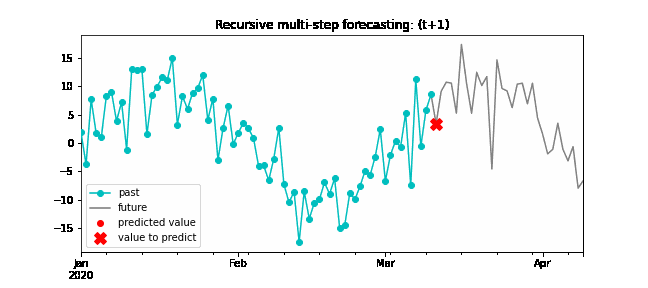
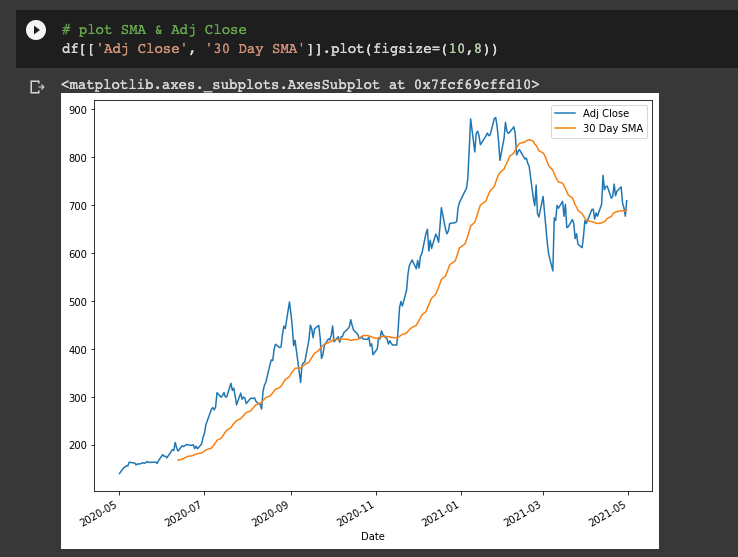
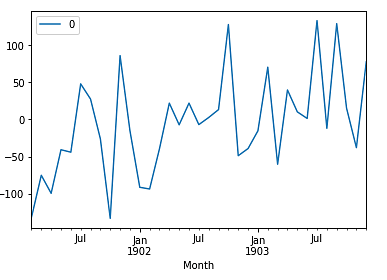
Article link: plot time series python.
Learn more about the topic plot time series python.
- How to Plot a Time Series in Matplotlib? – GeeksforGeeks
- Matplotlib Time Series Plot – Python Guides
- Matplotlib time series line plot – DataCamp
- Time Series Data Visualization with Python
- Time series plot with Matplotlib – Python Graph Gallery
- How to Plot a Time Series in Matplotlib? – GeeksforGeeks
- Python – Time Series – Tutorialspoint
- How to Generate Time Series Plot in Pandas – Spark By {Examples}
- How to visualize time series data – InfluxData
- matplotlib – How to plot time series in python – Stack Overflow
- How to plot a time series in Python – Tutorialspoint
- Time series and date axes in Python – Plotly
- How to handle time series data with ease – Pandas
See more: nhanvietluanvan.com/luat-hoc