Python Read File Into String
How to Read a File into a String in Python:
Reading a file in Python is fairly straightforward, thanks to the built-in `open()` method. Here’s how you can do it:
1. Using the open() method:
The `open()` method is used to open a file and returns a file object that can be used to access its contents. It takes two parameters: the path to the file and the mode in which you want to open it. Let’s take a look at the different modes in file opening:
– Read mode: `open(‘file.txt’, ‘r’)`
This opens the file in read-only mode, allowing you to access its contents. However, you cannot modify the contents of the file.
– Write mode: `open(‘file.txt’, ‘w’)`
This opens the file in write mode, allowing you to modify its contents. Be careful, as this will overwrite the existing contents of the file.
– Append mode: `open(‘file.txt’, ‘a’)`
This opens the file in append mode, allowing you to add content to the end of the file. Existing contents will not be overwritten.
2. Specifying the path to the file:
Before you can read a file, you need to specify its path. This can be an absolute or relative path. An absolute path specifies the file’s location from the root directory, while a relative path specifies the file’s location relative to the current working directory.
Accessing the contents of the file:
Now that you have opened the file, there are two primary methods you can use to access its contents:
1. Using the read() method:
The `read()` method is used to read the entire contents of a file as a single string. It returns the file’s contents as a string which can be assigned to a variable or used further in your program. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’)
contents = file.read()
print(contents)
file.close()
“`
2. Handling reading errors:
When reading a file, there are a couple of common errors you may encounter:
– FileNotFoundError:
This error occurs when the specified file does not exist or the path is incorrect. To handle this error, you can use a try-except block as shown below:
“`python
try:
file = open(‘file.txt’, ‘r’)
contents = file.read()
print(contents)
file.close()
except FileNotFoundError:
print(“File not found.”)
“`
– PermissionError:
This error occurs when you do not have permission to access the file. To handle this error, you can use a try-except block as shown below:
“`python
try:
file = open(‘file.txt’, ‘r’)
contents = file.read()
print(contents)
file.close()
except PermissionError:
print(“Permission denied.”)
“`
Closing the file:
Once you have finished reading the file, it is essential to close it using the `close()` method. This releases any system resources associated with the file and ensures the file is closed properly. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’)
contents = file.read()
print(contents)
file.close()
“`
Why closing the file is important:
Closing the file is crucial, as it prevents memory leaks and ensures that any changes made to the file are properly saved. If you forget to close the file, it may lead to unexpected behavior or errors in your program.
Working with different file types:
Python provides specialized modules and libraries to handle different file types effectively. Here are a few common file types and the corresponding modules you can use:
– Text files: For reading and writing plain text files, the built-in functions and methods we’ve discussed earlier are sufficient.
– CSV files: To work with CSV files, you can use the `csv` module, which provides methods to read and write data in CSV format.
– JSON files: For reading and writing JSON files, the `json` module can be used. It provides functions to encode and decode JSON data.
– XML files: Python provides the `xml.etree.ElementTree` module to work with XML files. It allows you to parse, manipulate, and generate XML data.
Handling large files efficiently:
When dealing with large files, reading the entire file into memory as a single string may not be feasible or efficient. To overcome this, Python provides a couple of alternatives:
– Using the read() method with a specified size:
The `read()` method can accept an optional parameter specifying the number of characters to read. By reading the file in smaller chunks, you can conserve memory and process the file efficiently. Here’s an example:
“`python
file = open(‘large_file.txt’, ‘r’)
chunk = file.read(1024) # Read 1024 characters at a time
while chunk:
# Process the chunk
chunk = file.read(1024) # Read the next chunk
file.close()
“`
– Using a for loop to read the file line by line:
If you only need to process the file line by line, you can use a for loop with the file object. This avoids reading the entire file into memory, making it suitable for large files. Here’s an example:
“`python
file = open(‘large_file.txt’, ‘r’)
for line in file:
# Process each line
file.close()
“`
Error handling and exception handling:
When reading files, it is essential to handle any potential errors or exceptions that may arise. Python provides try-except blocks to catch and handle exceptions. Here’s an example of handling specific exceptions:
– Using try-except blocks:
“`python
try:
file = open(‘file.txt’, ‘r’)
contents = file.read()
print(contents)
file.close()
except Exception as e:
print(“An error occurred:”, str(e))
“`
– Handling specific exceptions:
Apart from the generic `Exception` class, you can also handle specific exceptions that may occur during file reading. For example:
– IOError: This exception is raised when an input/output operation fails.
– OSError: This exception is raised when a system-related operation fails.
Reading a file from a URL or web page:
Python allows you to read files from URLs or web pages using the `urllib` library, which provides the necessary modules. Here’s how you can do it:
1. Using the urllib.request module:
The `urllib.request` module provides functions to open URLs and read their contents. Here’s an example:
“`python
import urllib.request
url = ‘http://example.com/file.txt’
response = urllib.request.urlopen(url)
contents = response.read().decode(‘utf-8’)
print(contents)
“`
2. Using the urllib.error module:
The `urllib.error` module provides classes for handling URL-related errors. Here’s an example:
“`python
import urllib.request
import urllib.error
try:
url = ‘http://example.com/file.txt’
response = urllib.request.urlopen(url)
contents = response.read().decode(‘utf-8’)
print(contents)
except urllib.error.HTTPError as e:
print(“HTTPError:”, str(e))
except urllib.error.URLError as e:
print(“URLError:”, str(e))
“`
Assigning the file contents to a string variable:
To assign the contents of a file to a string variable, you can use the `read()` method as discussed earlier. However, it is essential to strip any whitespace or newline characters if you want to manipulate the string further. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’)
contents = file.read().strip()
print(contents)
file.close()
“`
In conclusion, Python provides several methods and modules for reading files into strings. By using the `open()` method and appropriate file modes, you can access the file’s contents. It is crucial to handle any errors or exceptions that may occur during file reading. Additionally, Python offers specialized modules for working with different file types, efficient techniques for handling large files, and the ability to read files from URLs or web pages. Remember to close the file properly after reading and consider stripping whitespace or newline characters when assigning the file contents to a string variable.
FAQs:
Q1. How can I read a text file line by line in Python?
To read a text file line by line, you can use a for loop with the file object. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’)
for line in file:
# Process each line
file.close()
“`
Q2. What is the difference between read() and readline() in Python file handling?
The `read()` method reads the entire contents of a file as a single string, while the `readline()` method reads a single line from the file. If you want to iterate through the lines of a file one by one, you can use a while loop with the `readline()` method.
Q3. How do I know if I have reached the end of a file while reading in Python?
The `readline()` method returns an empty string when it reaches the end of a file. By checking for an empty string, you can determine if you have reached the end of the file.
Q4. How can I read a file as a list of lines in Python?
To read a file into a list of lines, you can use the `readlines()` method. It reads all the lines of a file and returns them as a list of strings. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’)
lines = file.readlines()
file.close()
“`
Q5. How can I read a file encoded in UTF-8 in Python?
When reading a file encoded in UTF-8, you need to specify the encoding in the `open()` method. Here’s an example:
“`python
file = open(‘file.txt’, ‘r’, encoding=’utf-8′)
contents = file.read()
file.close()
“`
Q6. How can I write a string to a file in Python?
To write a string to a file, you can open the file in write mode (`’w’`) or append mode (`’a’`) using the `open()` method. Here’s an example:
“`python
file = open(‘file.txt’, ‘w’)
file.write(‘Hello, World!’)
file.close()
“`
Q7. What if I forget to close the file after reading in Python?
Forgetting to close the file can lead to memory leaks and unexpected behavior in your program. It is essential to close the file using the `close()` method to release system resources and ensure the file is closed properly.
Q8. How can I handle file reading errors in Python?
You can use try-except blocks to handle file reading errors in Python. By catching specific exceptions such as `FileNotFoundError` or `PermissionError`, you can handle the errors gracefully and display appropriate messages to the user.
How To Read A Text File Into A String Variable And Strip Newlines?
Keywords searched by users: python read file into string Read file txt Python, Python read file as string, Python read file line by line, Readline Python end of file, Python file read, Read text file to list Python, Read file UTF-8 Python, Write string to file Python
Categories: Top 45 Python Read File Into String
See more here: nhanvietluanvan.com
Read File Txt Python
Python is known for its simplicity and power when it comes to handling file operations. Whether you need to read a text file, a CSV file, or even a JSON file, Python provides several built-in functions and libraries to facilitate this process. In this article, we will focus specifically on reading a text file in Python.
I. Reading a Text File: The Basics
To read a text file in Python, we can use the built-in function “open”. This function takes two parameters: the file path and the mode in which we want to open the file. For reading, the mode parameter should be set to “r” or “rt”. Here’s an example:
“`python
file_path = “example.txt”
file = open(file_path, “r”)
“`
Once the file is open, we can read its contents using various methods. The most common approach is to use the “read()” method, which reads the entire file content and returns it as a string. Here’s how we can use it:
“`python
file_content = file.read()
print(file_content)
“`
It’s important to note that once we have finished reading the file, it is good practice to close it using the “close()” method:
“`python
file.close()
“`
II. Reading Files Line by Line
Reading an entire file content might not always be necessary or efficient. In some cases, we might only need to process individual lines of the file. Python provides a convenient way to iterate over the lines of a file without loading the whole file into memory at once. We can achieve this by using a “for” loop directly on the file object:
“`python
with open(file_path, “r”) as file:
for line in file:
print(line)
“`
In this example, the “with” statement ensures that the file will be closed automatically after we finish reading it. By iterating over the file object, Python reads and processes one line at a time, making it memory-efficient and suitable for handling large files.
III. File Encoding and Handling Special Characters
While reading text files, it’s crucial to consider their encoding. Different encodings might result in different interpretations of special characters, leading to incorrect readings. To specify the encoding explicitly, we can include it as an argument in the “open” function:
“`python
with open(file_path, “r”, encoding=”utf-8″) as file:
# File processing operations
“`
By specifying the correct encoding, we ensure that we read the file correctly and handle special characters appropriately.
IV. Frequently Asked Questions (FAQs)
Q1. Can we read a file located in a different directory?
Yes, we can read a file from a different directory by providing the complete or relative path to the file. For example, to read a file in a subdirectory named “data,” we can use the following code:
“`python
file_path = “data/example.txt”
with open(file_path, “r”) as file:
# File processing operations
“`
Q2. How can we handle exceptions while reading files?
When reading files, it’s essential to handle potential exceptions that might occur, such as FileNotFoundError or PermissionError. We can use the “try-except” block to catch and handle these exceptions effectively. Here’s an example:
“`python
try:
with open(file_path, “r”) as file:
# File processing operations
except FileNotFoundError:
print(“File not found.”)
except PermissionError:
print(“Permission denied to access the file.”)
“`
By using exceptions, we can gracefully handle errors and ensure that our program doesn’t crash unexpectedly.
Q3. Can we read only a specific portion of a file?
Yes, Python allows us to read a specific portion of a file by using the “read(size)” method. The “size” parameter indicates the number of characters or bytes to be read. Here’s an example:
“`python
with open(file_path, “r”) as file:
portion = file.read(100) # Read the first 100 characters
print(portion)
“`
By specifying the desired size, we can limit the amount of content we read from the file.
Q4. Is it possible to read files in binary mode?
Yes, Python supports reading files in binary mode. To do this, we can open the file in binary mode by setting the mode parameter to “rb.” Here’s an example:
“`python
with open(file_path, “rb”) as file:
# Binary file processing operations
“`
In binary mode, the file content is processed in binary format, which is useful for handling non-text file types, such as images or audio files.
In conclusion, reading a text file in Python is a straightforward task thanks to the built-in functions and libraries provided by the language. By mastering the fundamentals and understanding additional functionalities, such as iterating over lines, handling encodings, and dealing with exceptions, you can confidently read and process various text files efficiently in Python.
Python Read File As String
There are multiple approaches to read a file as a string in Python, and the choice depends on the specific requirements of your project. Let’s dive into some of the popular methods:
1. Using the `read()` method:
The most straightforward method to read a file as a string in Python is by using the `read()` method. This method reads the entire contents of the file and returns them as a single string. Here is an example:
“`python
file_path = ‘path/to/file.txt’
with open(file_path, ‘r’) as file:
contents = file.read()
print(contents)
“`
In this code snippet, we open the file in read mode using the `open()` function and store it as a file object `file`. Then, we use the `read()` method on the file object to read its contents as a string and assign it to the variable `contents`. Finally, we print the string.
2. Using the `readlines()` method:
If you want to read a file as a list of strings, where each line becomes an element of the list, you can use the `readlines()` method. This method reads the file line by line and returns a list of strings. Here is an example:
“`python
file_path = ‘path/to/file.txt’
with open(file_path, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line)
“`
In this code snippet, we open the file in read mode, similar to the previous example. Then, we use the `readlines()` method to read the file line by line and store the lines as a list of strings in the variable `lines`. Finally, we iterate over the `lines` list and print each line.
3. Using the `for` loop:
In Python, you can directly iterate over a file object using a `for` loop, which reads the file line by line without explicitly calling any methods. Here is an example:
“`python
file_path = ‘path/to/file.txt’
with open(file_path, ‘r’) as file:
for line in file:
print(line)
“`
In this code snippet, we open the file using the `open()` function as before. Then, we iterate over the `file` object directly in the `for` loop, which reads each line of the file and stores it in the variable `line`. Finally, we print each line.
4. Using the `read()` method with a specified size:
If you want to read the file in chunks of a specific size rather than the entire contents at once, you can pass a size parameter to the `read()` method. This is useful when reading large files to avoid loading everything into memory. Here is an example:
“`python
file_path = ‘path/to/file.txt’
chunk_size = 1024 # read 1024 characters at a time
with open(file_path, ‘r’) as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
print(chunk)
“`
In this code snippet, we define a `chunk_size` variable to specify the number of characters to read at a time. We then use a `while` loop to read the file in chunks until the end is reached. The `read()` method returns an empty string when there is nothing left to read, which we check for using the `if not chunk` condition. Finally, we print each chunk.
Now, let’s address some frequently asked questions about reading a file as a string in Python:
Q1. Can I read a binary file as a string using these methods?
A1. Yes, you can read a binary file by specifying the appropriate mode when opening the file. For example, if you want to read a binary file as a string, open the file with the `’rb’` mode instead of the `’r’` mode.
Q2. How can I handle encoding issues while reading files?
A2. When reading files, it is important to consider the encoding of the file. By default, Python uses the system’s default encoding. However, you can specify the encoding explicitly by providing the `encoding` parameter to the `open()` function. For example: `with open(file_path, ‘r’, encoding=’utf-8′) as file:`
Q3. Is there a limit to the file size I can read as a string?
A3. The size of the file you can read as a string depends on the available memory on your system. If you try to read a large file that exceeds your system’s memory capacity, it may cause an out-of-memory error. In such cases, it’s advisable to use approaches that read files in chunks, as mentioned above.
Reading a file as a string is a common task in Python, and understanding the various methods available will help you handle file input effectively in your projects. Whether you need to process small text files or handle large log files, Python provides flexible options to suit your needs.
Python Read File Line By Line
Reading a file line by line is a fundamental task in many programming scenarios, such as processing large datasets or analyzing log files. Python offers multiple approaches to accomplish this task, each with its own advantages and use cases. Let’s delve into these methods and explore their functionalities.
Method 1: Using the `readline()` Function
The simplest method to read a file line by line is by using the `readline()` function. This function reads a single line from a file each time it is called. By calling `readline()` iteratively, we can read the entire file line by line. Here’s an example:
“`python
with open(‘filename.txt’, ‘r’) as file:
line = file.readline()
while line:
# Process the line
print(line)
line = file.readline()
“`
In the example above, we open the file specified by `’filename.txt’` in the read mode `’r’`. We use a `with` statement to ensure the file is properly closed after reading is finished. We then use a `while` loop to iterate through the file until a line becomes empty (which indicates the end of the file). Within the loop, we can perform any desired operations on each line, such as printing or processing the data.
Method 2: Using a `for` Loop
Another approach is to use a `for` loop to directly iterate over the lines of a file without calling a specific function. This is a more concise and Pythonic way of reading files line by line. Here’s an example:
“`python
with open(‘filename.txt’, ‘r’) as file:
for line in file:
# Process the line
print(line)
“`
In the above code, the file is opened using the same `with` statement. Each iteration of the `for` loop assigns the next line from the file to the variable `line`, allowing us to process it as desired. This method eliminates the need to call `readline()` repeatedly, making the code more readable and concise.
Method 3: Using the `readlines()` Function
The `readlines()` function reads all the lines of a file into a list and returns it. By storing all the lines in memory, this method allows us to access any line at any time without re-reading the file. Here’s an example:
“`python
with open(‘filename.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
# Process the line
print(line)
“`
In this method, the `readlines()` function reads all lines from the file and returns a list where each element represents a line. We can then iterate over this list using a `for` loop or access individual lines using indexing. However, it is worth noting that this method may not be suitable for very large files as it can consume a significant amount of memory.
FAQs:
Q1. Can I read a file located in a different directory?
Yes, you can read a file located in a different directory by providing the full or relative path to the file when opening it. For example, to read a file named `’data.txt’` located in a subdirectory called `’files’`, you can use `’files/data.txt’` as the file path argument in the `open()` function.
Q2. How can I check if the file exists before reading it?
To check if a file exists before reading, you can use the `os.path.exists()` function from the built-in `os` module. This function returns `True` if the file exists and `False` otherwise. Here’s an example:
“`python
import os
file_path = ‘filename.txt’
if os.path.exists(file_path):
# Read the file
with open(file_path, ‘r’) as file:
# Read file line by line
else:
print(“File does not exist.”)
“`
Q3. How can I skip empty lines while reading a file?
To skip empty lines while reading a file, you can add a condition to filter out lines that contain only whitespace characters. Here’s an example of how you can modify the `for` loop:
“`python
with open(‘filename.txt’, ‘r’) as file:
for line in file:
if line.strip():
# Process non-empty lines
print(line)
“`
In the code above, the `strip()` function is called on each line, removing any leading or trailing whitespace characters. The condition `if line.strip()` evaluates to `True` only if the line is not empty, allowing us to process only the non-empty lines.
In conclusion, reading files line by line is a common task in Python. We discussed three different methods to accomplish this: using the `readline()` function in a `while` loop, iterating directly with a `for` loop, or using the `readlines()` function. Each method offers its own advantages and is suitable for different scenarios. By understanding these techniques and addressing frequently asked questions, you are now equipped to read files line by line in Python confidently.
Images related to the topic python read file into string
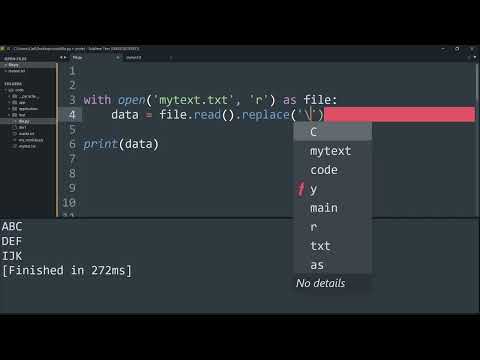
Found 15 images related to python read file into string theme
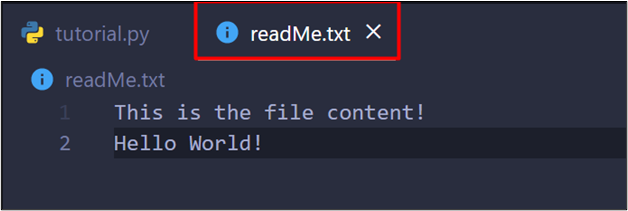
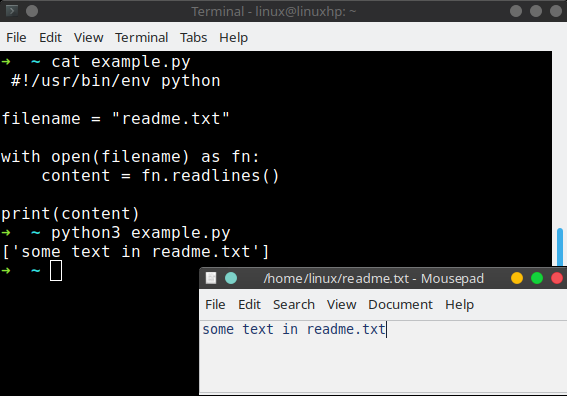
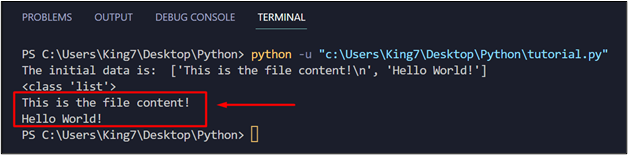
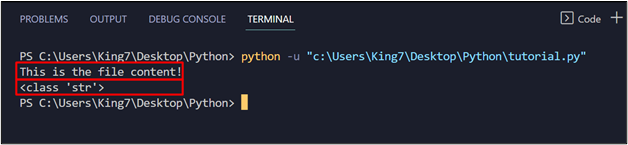

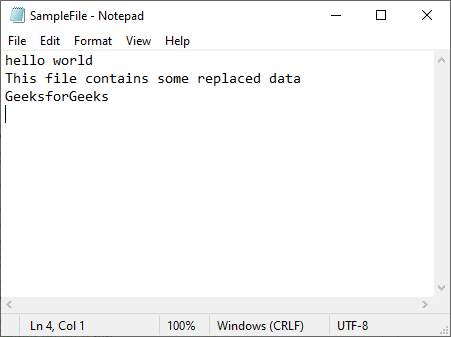
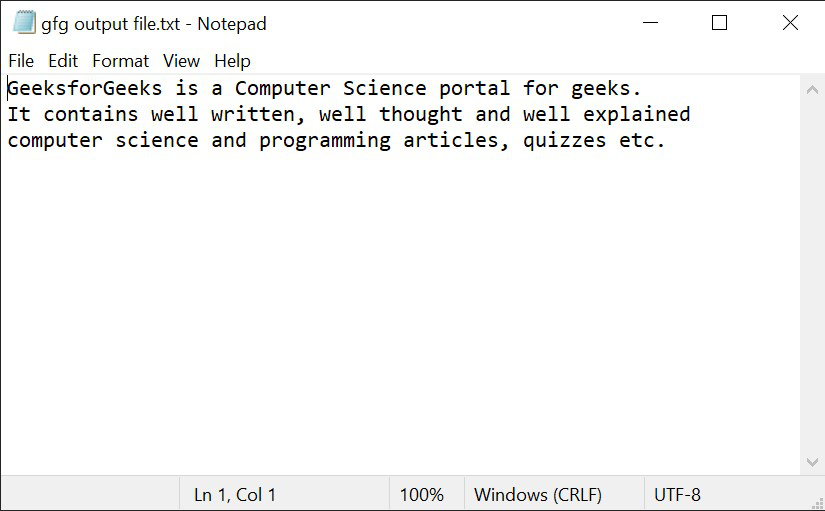

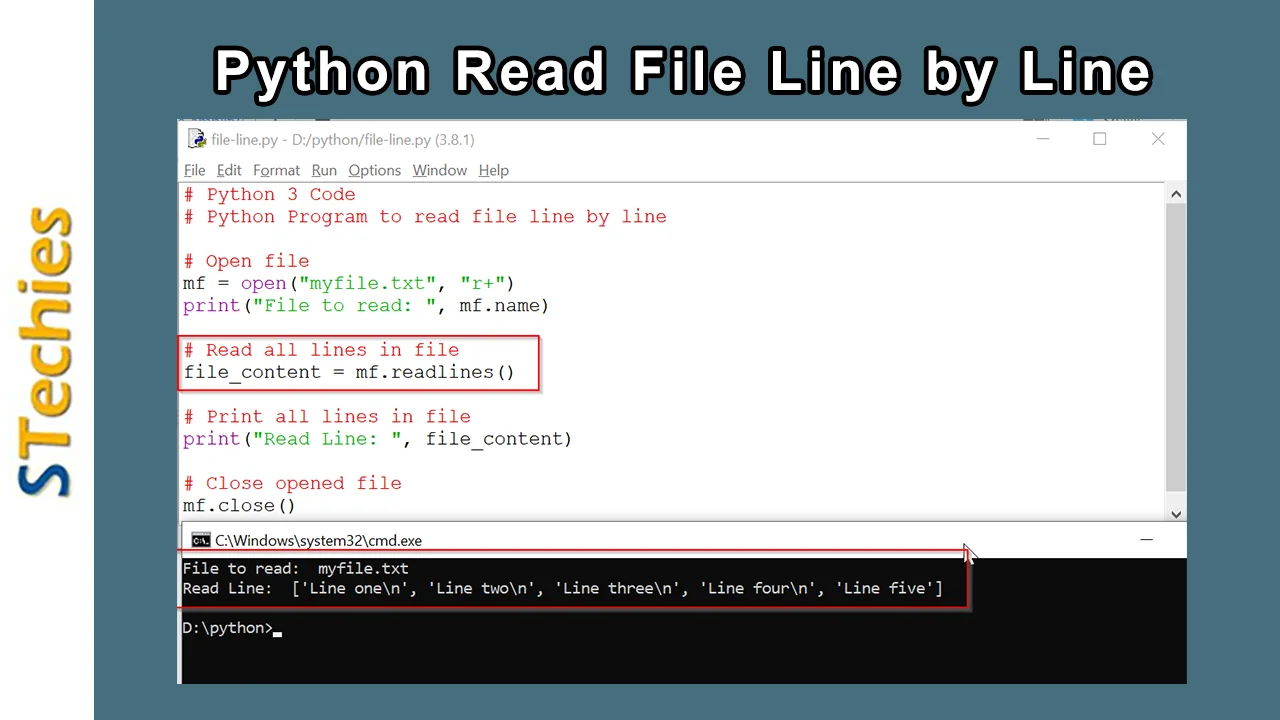
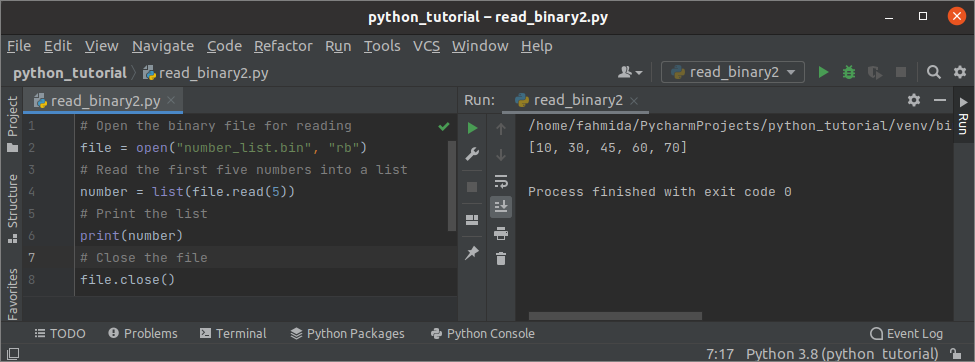
![Python Search for a String in Text Files [4 Ways] – PYnative Python Search For A String In Text Files [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2022/02/sales_text_file.jpg)
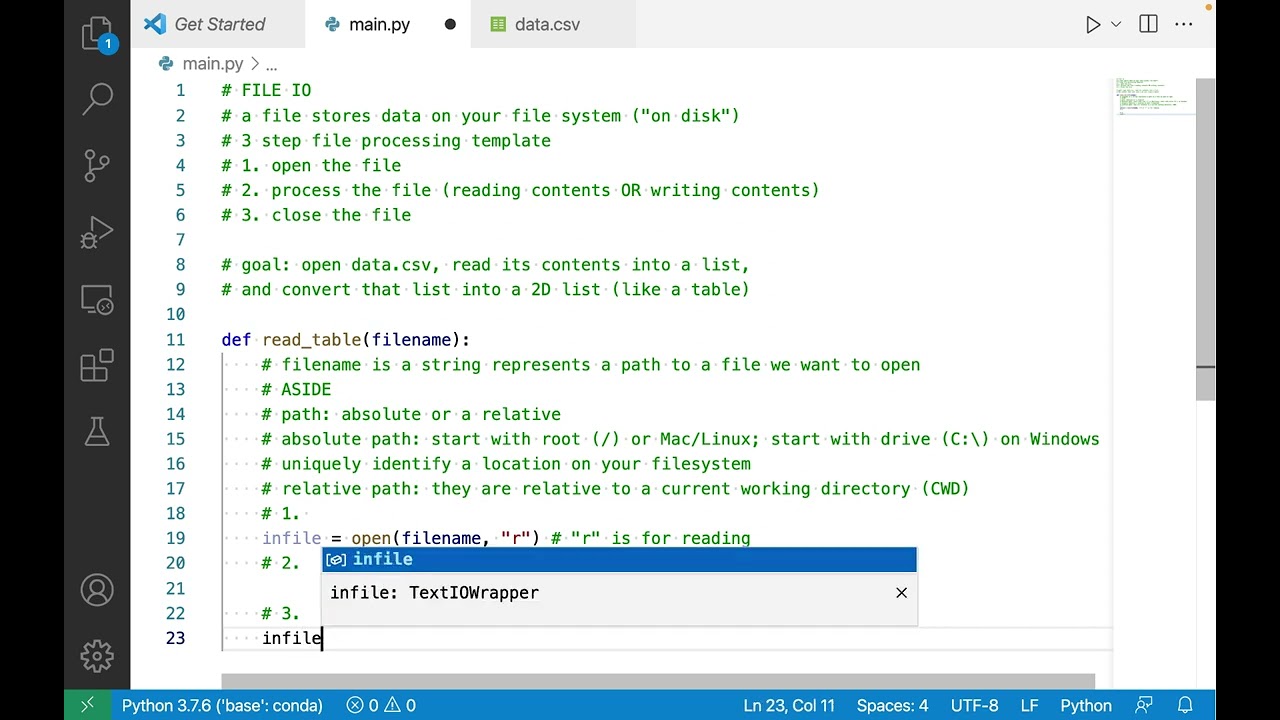


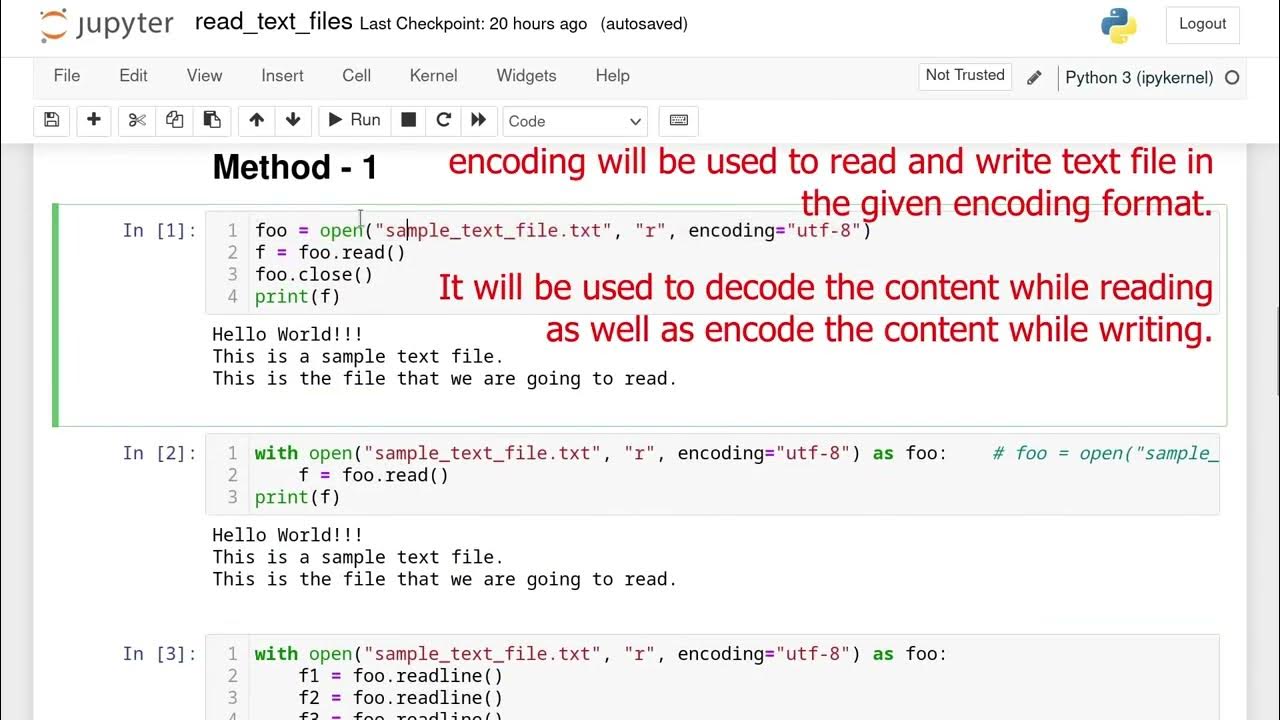
![Python Writing List to a File [5 Ways] – PYnative Python Writing List To A File [5 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/12/file_after_writing_python_list_into_it.png)
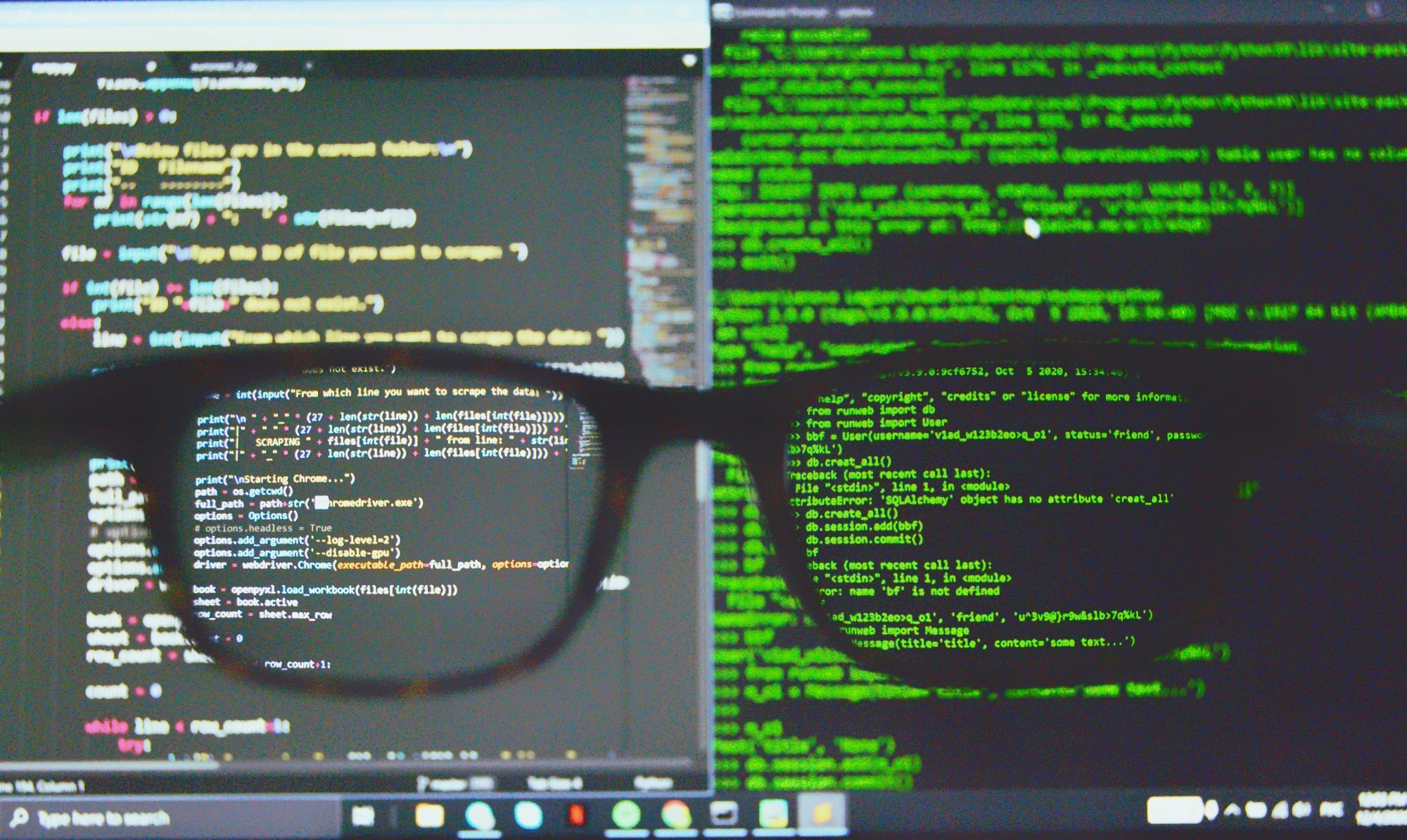
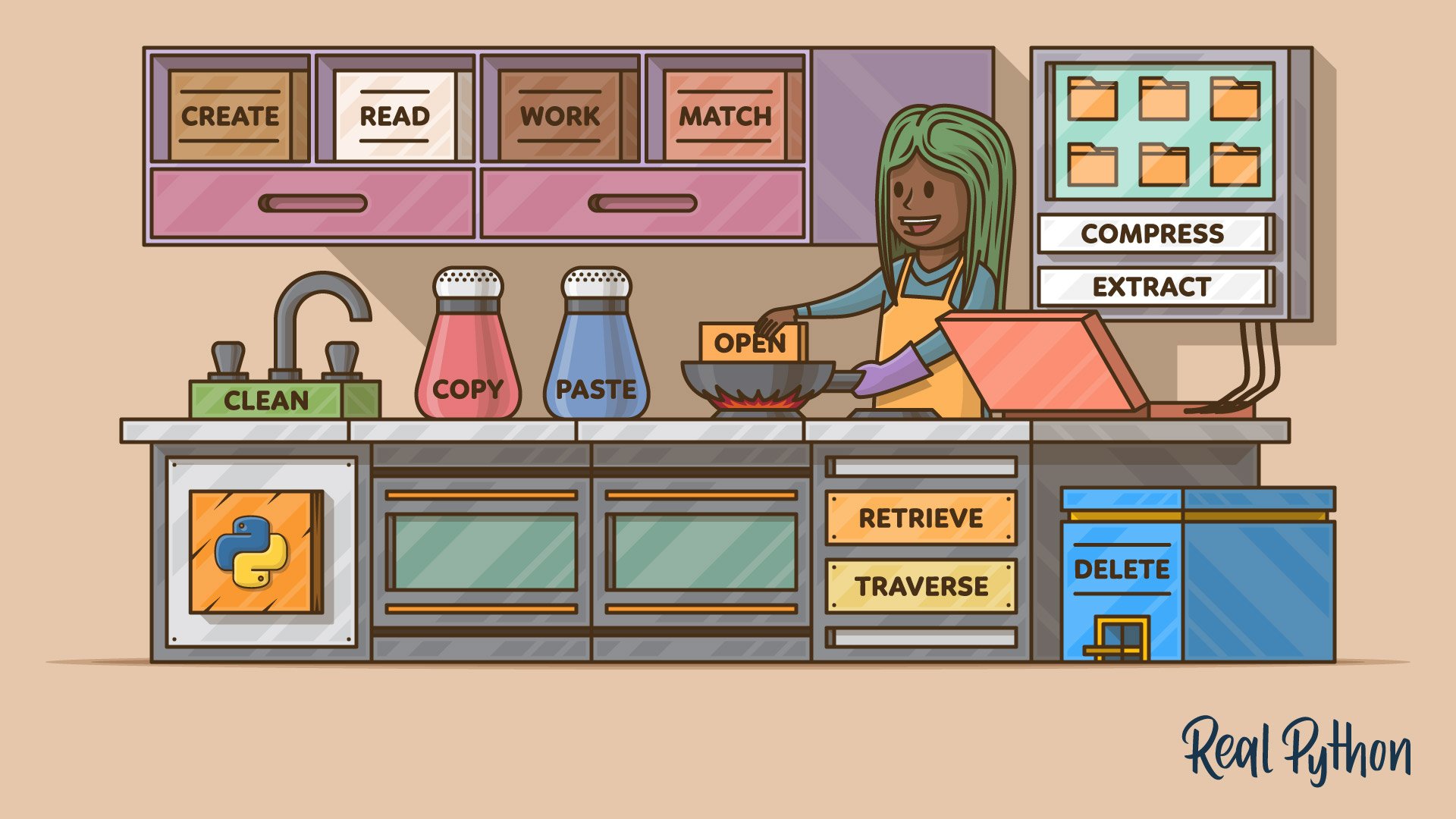
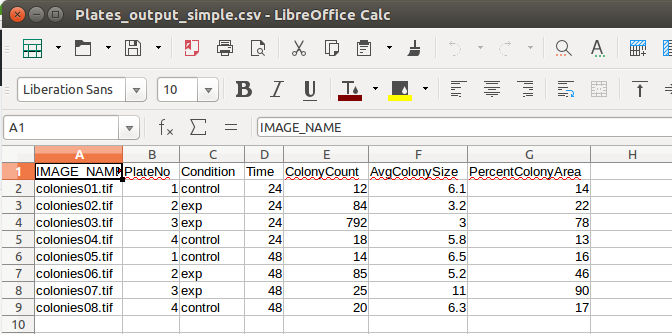

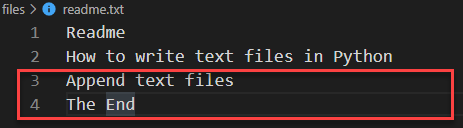
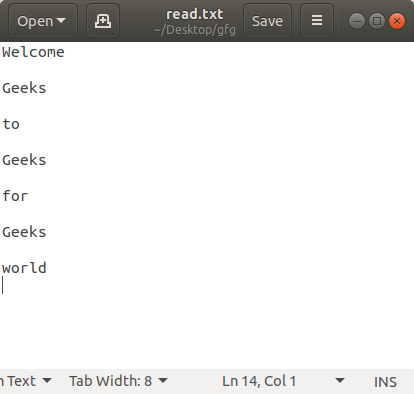
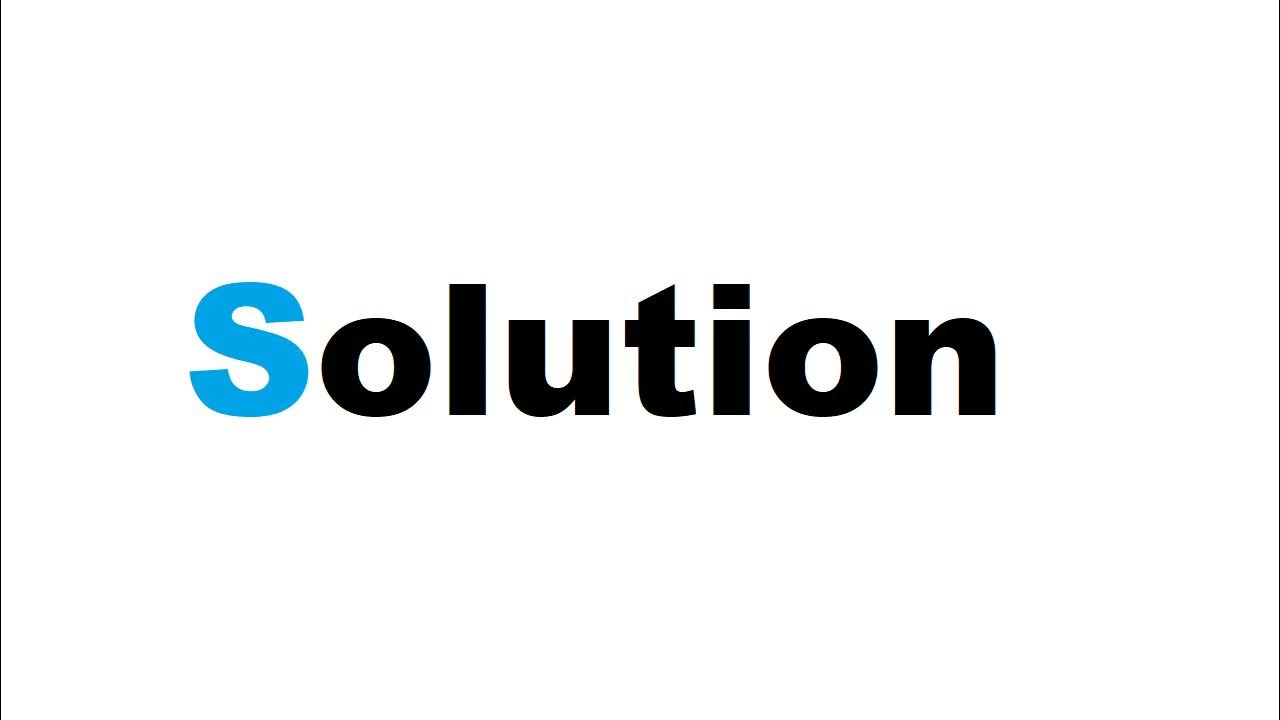

![Python Delete Lines From a File [4 Ways] – PYnative Python Delete Lines From A File [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/python_delete_lines_from_file.png)
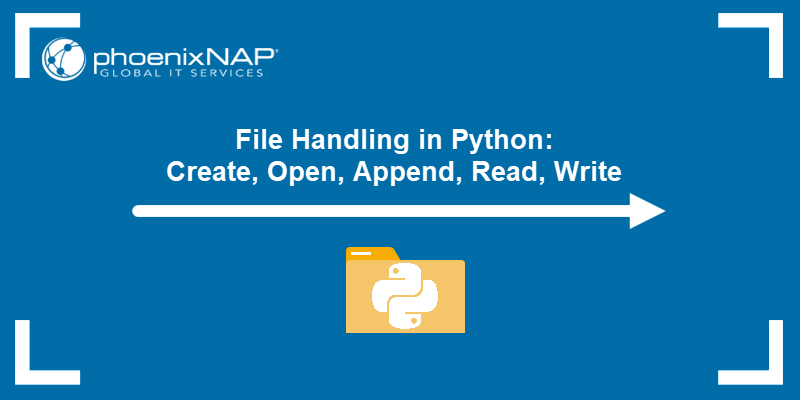

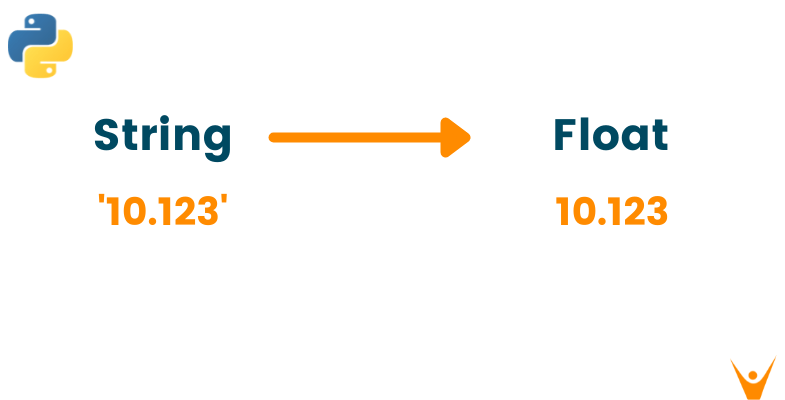
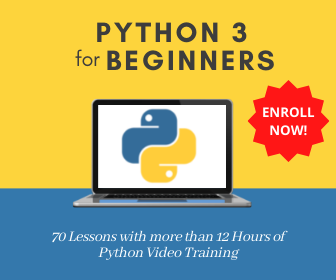
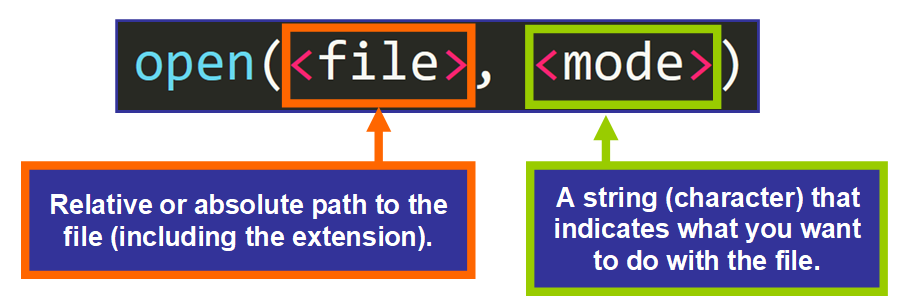
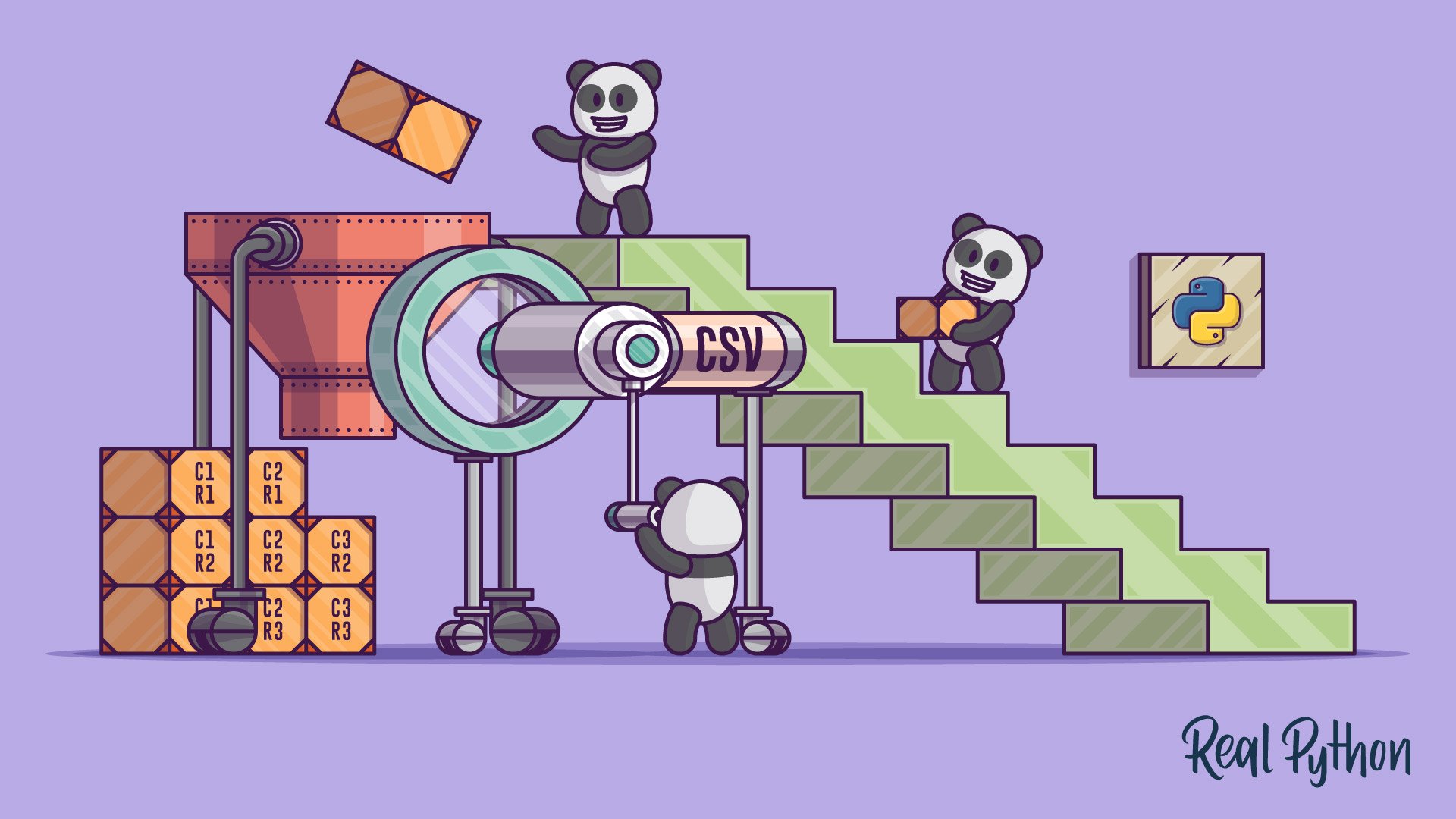
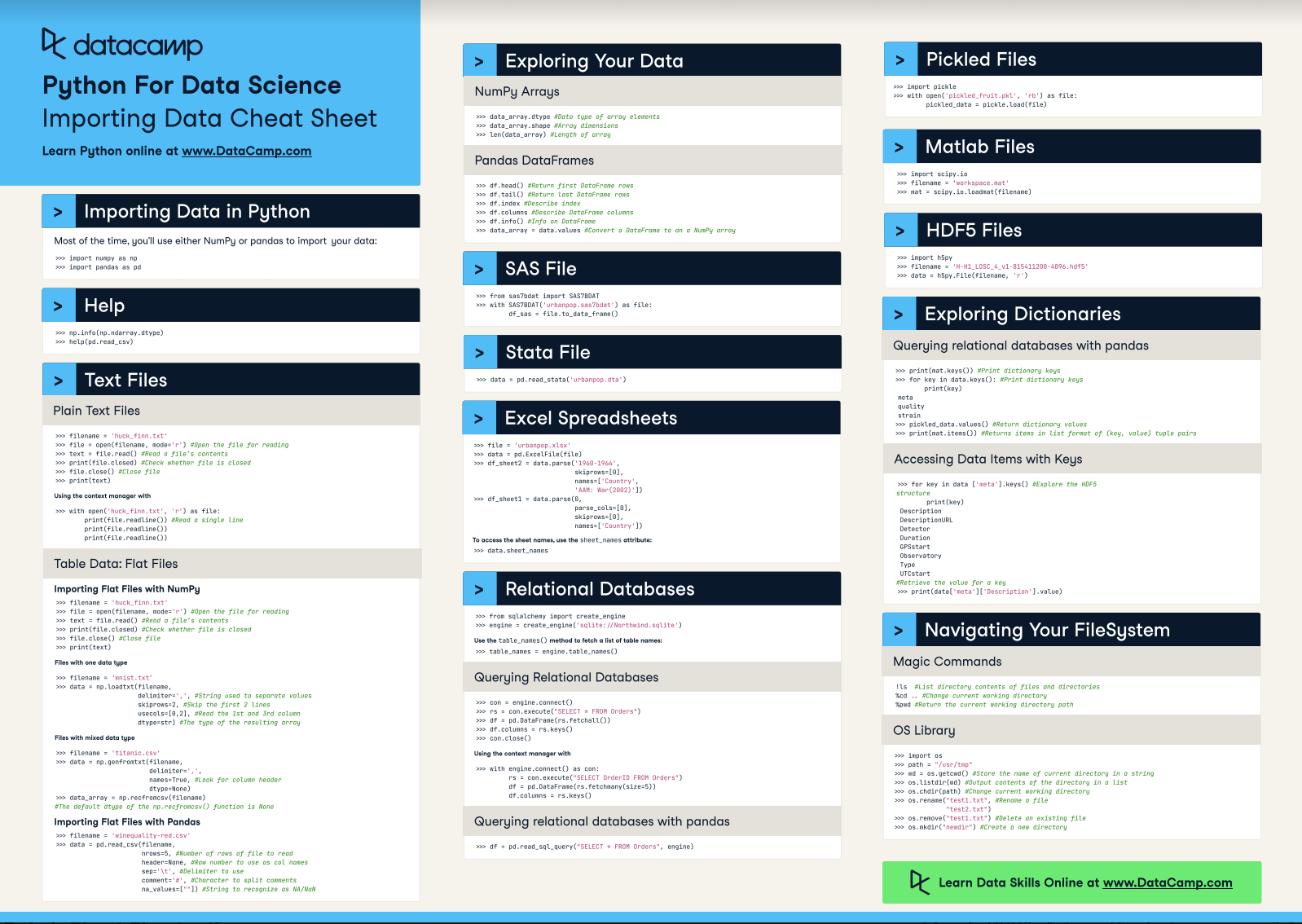
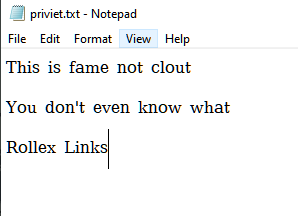
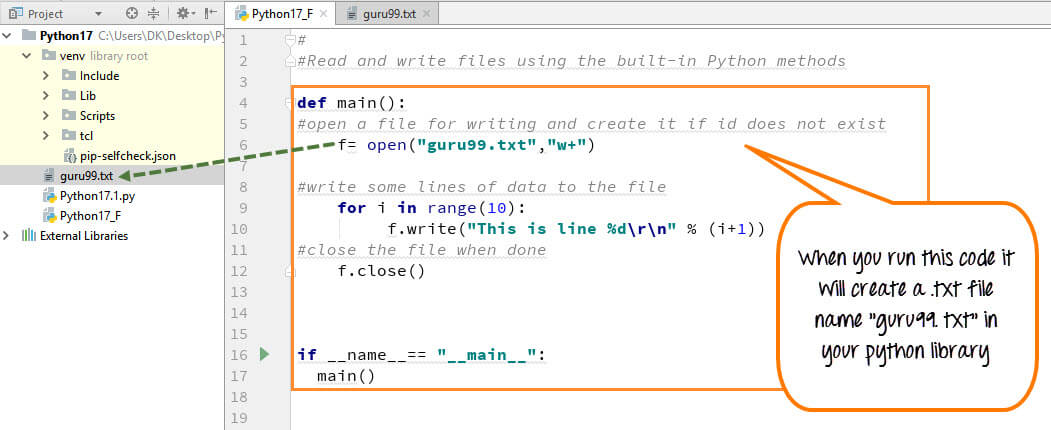
![Python Write Variable To File [4 Methods] - Python Guides Python Write Variable To File [4 Methods] - Python Guides](https://i0.wp.com/pythonguides.com/wp-content/uploads/2023/01/Python-write-variable-to-file-using-str-function.jpg)



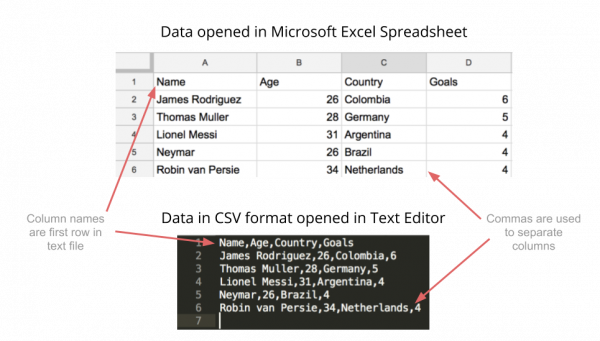
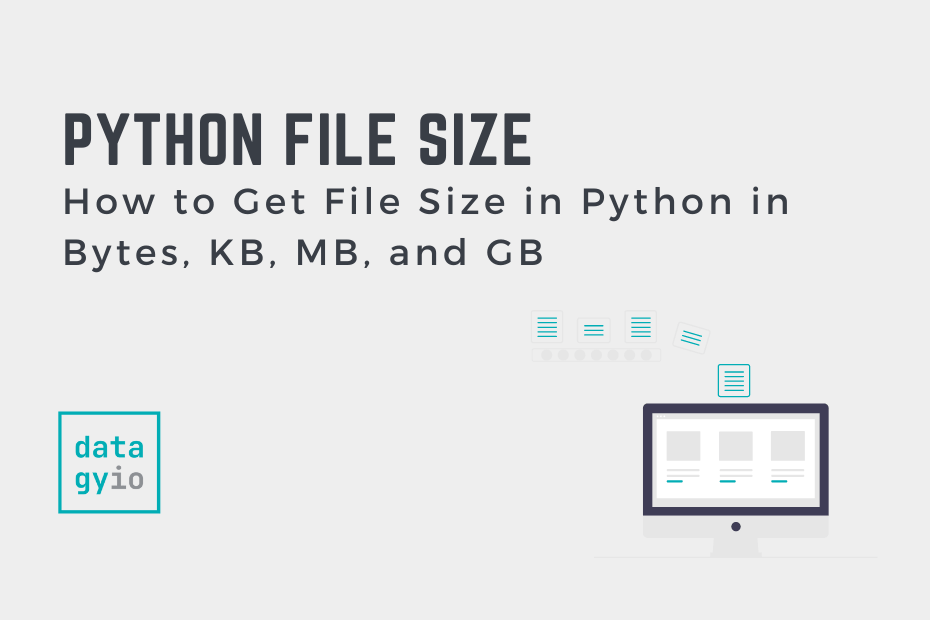
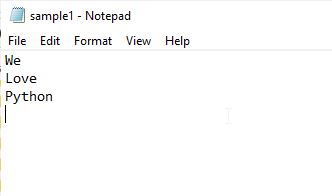
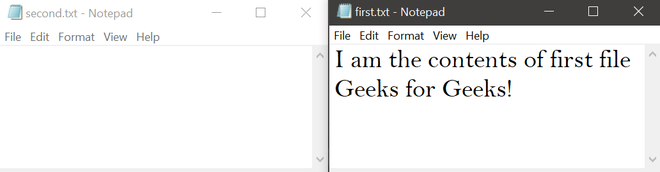
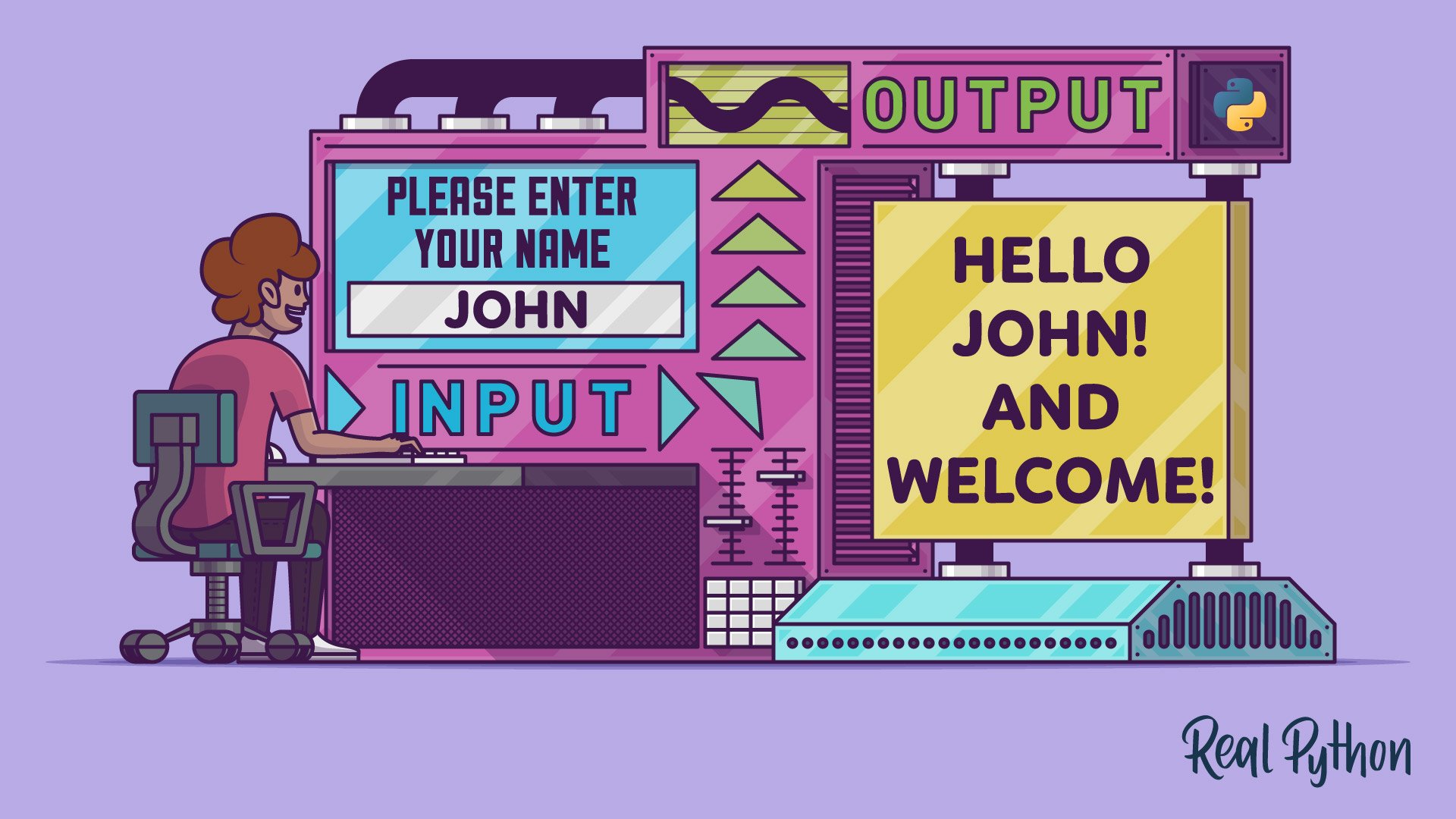
![File Handling in Python [Complete Series] – PYnative File Handling In Python [Complete Series] – Pynative](https://pynative.com/wp-content/uploads/2021/07/file_handling_in_python.png)

Article link: python read file into string.
Learn more about the topic python read file into string.
- How to read a text file into a string variable and strip newlines?
- How to read File as String in Python – TutorialKart
- Read File as String in Python – AskPython
- Read a text file into a string variable and strip newlines in Python
- How to Read a Text file In Python Effectively
- Python Read File Into String – Linux Hint
- Python read file into string – Java2Blog
- Read a text File into a String and Strip Newlines
- How to read a text file in Python – Tutorialspoint
- How to read files into a string in Python 3? – EasyTweaks.com
See more: https://nhanvietluanvan.com/luat-hoc