Python Remove First Character From String
1. Using Slicing to Remove the First Character from a String:
One of the simplest ways to remove the first character from a string in Python is by using slicing. Slicing allows us to extract a portion of a string by specifying the start and end indices. To remove the first character, we can simply specify the slice starting from index 1.
Here’s an example to remove the first character using slicing:
“`python
string = “Hello World”
new_string = string[1:]
print(new_string) # Output: “ello World”
“`
2. Removing the First Character Using the str.lstrip() Method:
The `str.lstrip()` method in Python removes leading whitespace characters or a specified set of characters from the left side of a string. By using this method, we can remove the first character from a string by specifying it as a character set.
Here’s an example to remove the first character using the `str.lstrip()` method:
“`python
string = “Hello World”
new_string = string.lstrip(string[0])
print(new_string) # Output: “ello World”
“`
3. Removing the First Character Using the str.join() Method:
The `str.join()` method concatenates each item in an iterable (e.g., a list) into a string, using the specified string as the separator. We can leverage this method to remove the first character by joining the characters after the first character.
Here’s an example to remove the first character using the `str.join()` method:
“`python
string = “Hello World”
new_string = ”.join(string[1:])
print(new_string) # Output: “ello World”
“`
4. Removing the First Character Using the str.replace() Method:
The `str.replace()` method in Python replaces all occurrences of a specified substring with another substring. We can make use of this method to replace the first character with an empty string.
Here’s an example to remove the first character using the `str.replace()` method:
“`python
string = “Hello World”
new_string = string.replace(string[0], ”, 1)
print(new_string) # Output: “ello World”
“`
5. Removing the First Character Using the re.sub() Method:
The `re.sub()` method from the `re` module in Python is used to replace all occurrences of a pattern in a string with another string. We can apply this method to substitute the first character with an empty string using a regular expression pattern.
Here’s an example to remove the first character using the `re.sub()` method:
“`python
import re
string = “Hello World”
new_string = re.sub(‘^.’, ”, string)
print(new_string) # Output: “ello World”
“`
6. Removing the First Character Using the str.partition() Method:
The `str.partition()` method divides a string into three parts based on a separator and returns a tuple containing these parts. We can utilize this method to partition the string based on the first character and concatenate the last two parts.
Here’s an example to remove the first character using the `str.partition()` method:
“`python
string = “Hello World”
new_string = string.partition(string[0])[2]
print(new_string) # Output: “ello World”
“`
With these methods, you can easily remove the first character from a string in Python. However, it’s important to note that these methods assume the string is not empty. To prevent errors, you may need to add additional checks before applying these methods.
FAQs:
Q1. How can I get the first character of a string in Python?
A: To get the first character of a string in Python, you can use indexing. By accessing the character at position 0, you can obtain the first character.
Example:
“`python
string = “Hello”
first_char = string[0]
print(first_char) # Output: “H”
“`
Q2. How can I remove the first and last characters from a string in Python?
A: To remove the first and last characters from a string in Python, you can use slicing. By specifying the slice starting from index 1 and ending at the second-last index, you can ignore both the first and last characters.
Example:
“`python
string = “Hello World”
new_string = string[1:-1]
print(new_string) # Output: “ello Worl”
“`
Q3. How can I remove the last character from a string in Python?
A: To remove the last character from a string in Python, you can again use slicing. By specifying the slice starting from index 0 and ending at the second-last index, you can exclude the last character.
Example:
“`python
string = “Hello World”
new_string = string[:-1]
print(new_string) # Output: “Hello Worl”
“`
Q4. How can I remove a specific character from a string in Python?
A: To remove a specific character from a string in Python, you can use the `str.replace()` method. By replacing the character with an empty string, you can effectively remove it from the string.
Example:
“`python
string = “Hello World”
char_to_remove = ‘o’
new_string = string.replace(char_to_remove, ”)
print(new_string) # Output: “Hell Wrld”
“`
Q5. How can I remove a substring from a string in Python?
A: To remove a substring from a string in Python, you can use the `str.replace()` method. By replacing the substring with an empty string, you can eliminate it from the string.
Example:
“`python
string = “Hello World”
substring_to_remove = “o ”
new_string = string.replace(substring_to_remove, ”)
print(new_string) # Output: “HellWrld”
“`
In conclusion, Python provides multiple methods to remove the first character from a string. Depending on your specific requirements, you can choose the method that best suits your needs. Additionally, we have explored other string manipulation techniques such as getting the first character, removing the last character, removing a specific character, and removing substrings from a string. Understanding these methods will empower you to efficiently manipulate strings in Python.
How To Remove The First Character From A String In Python
Keywords searched by users: python remove first character from string Get first character of string Python, Pandas remove first character from string, Remove first and last character from string Python, Remove last character from string Python, Remove character in string Python, Get character from string Python, Remove in string in Python, Python remove substring
Categories: Top 66 Python Remove First Character From String
See more here: nhanvietluanvan.com
Get First Character Of String Python
Python is a versatile programming language that offers various methods to manipulate and extract data from strings. In this article, we will focus on one essential task: extracting the first character of a string in Python. Whether you are a beginner or an experienced developer, this guide will provide you with everything you need to know.
Getting Started: Understanding Strings in Python
Before diving into extracting the first character of a string, let’s first understand what strings are in Python. A string is a sequence of characters enclosed in single, double, or triple quotes. Python treats strings as immutable objects, meaning that once created, they cannot be changed.
To declare a string variable, you can use any of the following methods:
“`python
str1 = ‘Hello’
str2 = “World”
str3 = “””Python”””
“`
Extracting the First Character: The Syntax
To extract the first character of a string, Python provides a simple and straightforward method. You need to use square brackets with the index `0`, as shown below:
“`python
str = “Python”
first_char = str[0]
print(first_char) # Output: P
“`
In this example, we declared a string `str` with the value “Python”. By accessing the element at index 0 (`str[0]`), we extract the first character ‘P’ and assign it to the variable `first_char`. Finally, we print the value of `first_char` and obtain ‘P’ as the output.
It is worth noting that Python uses zero-based indexing, meaning that the first element of a string has an index of 0. Therefore, accessing `str[0]` provides the first character, `str[1]` the second character, and so on.
Handling Empty Strings and Strings with No Valid First Character
When dealing with strings, it is important to consider scenarios where the string might be empty or contain no valid first character. To handle such cases, you can add a conditional statement to check if the string is empty or the first character exists using the `len()` function, as shown in the following code snippet:
“`python
str1 = “”
str2 = “123”
if len(str1) > 0:
first_char1 = str1[0]
else:
print(“Empty string!”)
if len(str2) > 0:
first_char2 = str2[0]
else:
print(“No valid first character in string!”)
“`
In this example, we declare two strings, `str1` as an empty string and `str2` as “123”. Using the conditional statement, we check whether the length of `str1` and `str2` is greater than zero. If it is, we can safely extract the first character; otherwise, we handle the respective cases.
Frequently Asked Questions (FAQs):
Q1: What happens if I try to extract the first character from an empty string?
Attempting to access the first character of an empty string will result in an `IndexError` since there are no valid characters to extract. It is crucial to handle this scenario to avoid potential program crashes.
Q2: Can I directly access the first character of a string without assigning it to a variable?
Yes, it is possible to access the first character of a string directly without assigning it to a variable. For example:
“`python
str = “Python”
print(str[0]) # Output: P
“`
In this case, we directly print the first character ‘P’ without using any additional variables. However, assigning it to a variable can be useful if you need to perform further operations or manipulate the first character later in your code.
Q3: How can I extract the first character of multiple strings in one go?
If you have multiple strings and want to extract their first characters simultaneously, you can use a loop or list comprehension. Here’s an example using a loop:
“`python
strings = [“Python”, “Java”, “C++”]
first_chars = []
for string in strings:
first_chars.append(string[0])
print(first_chars) # Output: [‘P’, ‘J’, ‘C’]
“`
In this code snippet, we have a list of strings `strings` and an empty list `first_chars`. We loop through each string in `strings`, extract the first character, and append it to `first_chars`. Finally, we print the list `first_chars` containing the first characters of all the strings.
Q4: Can I modify the first character of a string using the same method?
No, strings in Python are immutable, meaning you cannot modify them directly. If you want to change the first character of a string, you will need to create a new string with the desired modification.
In conclusion, extracting the first character of a string in Python is quite straightforward using square brackets with the index `0`. By understanding the syntax and potential scenarios, you can confidently handle empty strings and strings without valid first characters. Python’s flexibility allows you to use this method in diverse ways, such as accessing multiple strings’ first characters simultaneously. Remember to handle exceptions and embrace the immutability of strings to effectively manipulate your data.
Pandas Remove First Character From String
### Removing the First Character
To remove the first character from a string in Pandas, we can make use of the `str` accessor, which provides numerous string methods for data manipulation. One such method is `str.replace()`, which can be used to replace a specific character or substring with another value. By replacing the first character with an empty string, we effectively remove it from the original string.
Let’s dive into some examples to illustrate how to remove the first character from a string using Pandas.
“` python
import pandas as pd
# Create a DataFrame
data = {‘Name’: [‘John’, ‘Emma’, ‘Jacob’, ‘Olivia’],
‘Age’: [25, 32, 28, 31]}
df = pd.DataFrame(data)
# Remove the first character from the ‘Name’ column
df[‘Name’] = df[‘Name’].str[1:]
“`
In the code snippet above, we start by importing the Pandas library and creating a DataFrame called `df` with two columns: ‘Name’ and ‘Age’. We then use the `str` accessor to access the ‘Name’ column and remove the first character from each entry. By assigning the modified values back to the ‘Name’ column, we successfully remove the first character from the original strings.
### Other Methods to Remove the First Character
While using `str.replace()` is one way to remove the first character from a string in Pandas, there are a few alternative methods that achieve the same result. Let’s take a look at them:
1. Using `str.lstrip()`:
The `str.lstrip()` method removes leading characters from the left side of the string. By specifying a substring with only the first character, we can remove it from the string.
“` python
df[‘Name’] = df[‘Name’].str.lstrip(df[‘Name’].str[0])
“`
2. Using `str.slice()`:
The `str.slice()` method allows us to slice a string from a given starting position. By slicing from the second character onwards, we effectively remove the first character.
“` python
df[‘Name’] = df[‘Name’].str.slice(1)
“`
Both methods produce the same output, removing the first character from the strings in the ‘Name’ column of the DataFrame.
### FAQs
#### Q: Can I remove multiple characters from the start of a string?
Yes, you can remove multiple characters from the start of a string using any of the aforementioned methods. Simply adjust the position and length of the slice or substring accordingly. For instance, if you want to remove the first three characters, you can modify the code snippet using `str.slice()` as follows:
“` python
df[‘Name’] = df[‘Name’].str.slice(3)
“`
This would discard the first three characters from the ‘Name’ column.
#### Q: Will removing the first character modify the original DataFrame?
Yes, removing the first character will modify the original DataFrame. When you use any of the methods mentioned above, the changes are made directly to the ‘Name’ column of the DataFrame, and the modifications will persist unless assigned back to the column.
#### Q: How can I remove the first character only from specific rows?
If you need to remove the first character from the strings in specific rows, you can utilize boolean indexing to select those rows and apply any of the methods discussed.
“` python
df.loc[df[‘Age’] > 30, ‘Name’] = df.loc[df[‘Age’] > 30, ‘Name’].str[1:]
“`
In the example above, we use boolean indexing to select only the rows where the ‘Age’ is greater than 30. Then, we apply the `str` accessor to the ‘Name’ column of those rows and remove the first character.
#### Q: Can I remove the first character from multiple columns simultaneously?
Yes, you can remove the first character from multiple columns simultaneously by applying the desired string manipulation methods to each column individually.
“` python
df[[‘Name’, ‘Age’]] = df[[‘Name’, ‘Age’]].apply(lambda x: x.str[1:])
“`
In this case, we use the `apply()` function along with a lambda function to apply the `str[1:]` operation to both the ‘Name’ and ‘Age’ columns.
### Conclusion
In this article, we explored different ways to remove the first character from a string in Pandas. Using methods like `str.replace()`, `str.lstrip()`, and `str.slice()`, we can easily achieve this task. Additionally, the FAQ section addressed some common queries regarding multiple character removal, modification of the original DataFrame, selective removal, and simultaneous removal from multiple columns. With this knowledge, you can now confidently manipulate and clean your string data using Pandas.
Images related to the topic python remove first character from string
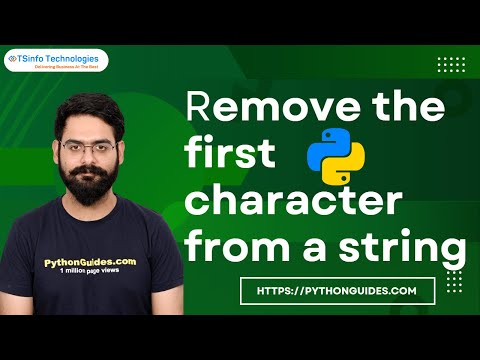
Found 24 images related to python remove first character from string theme
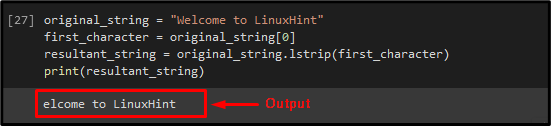

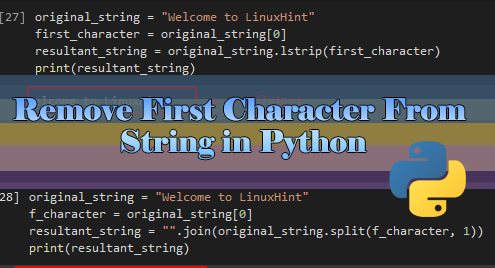
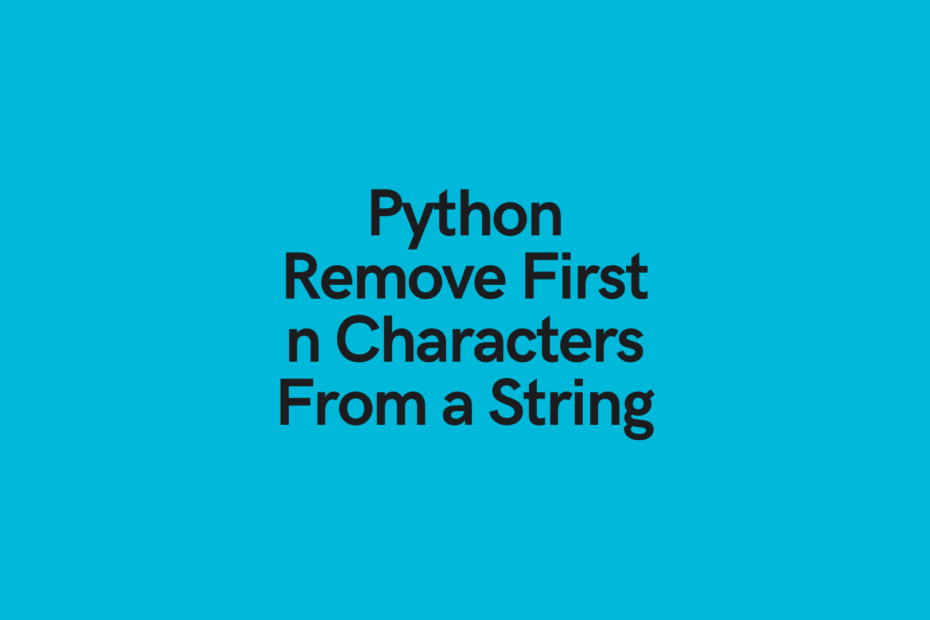
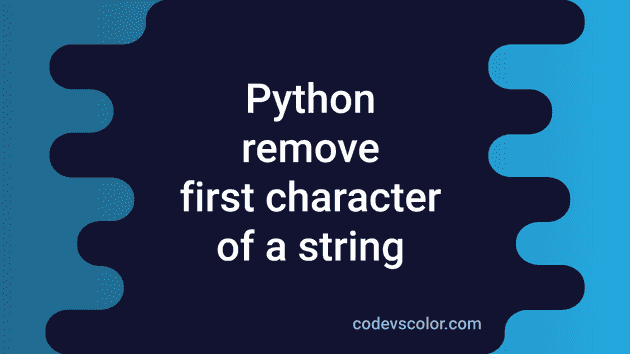

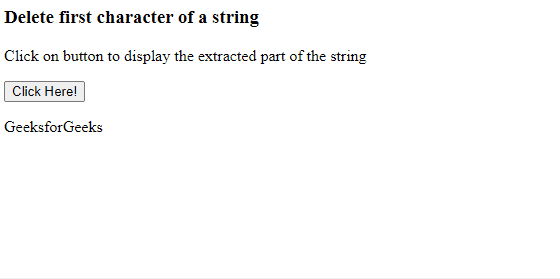
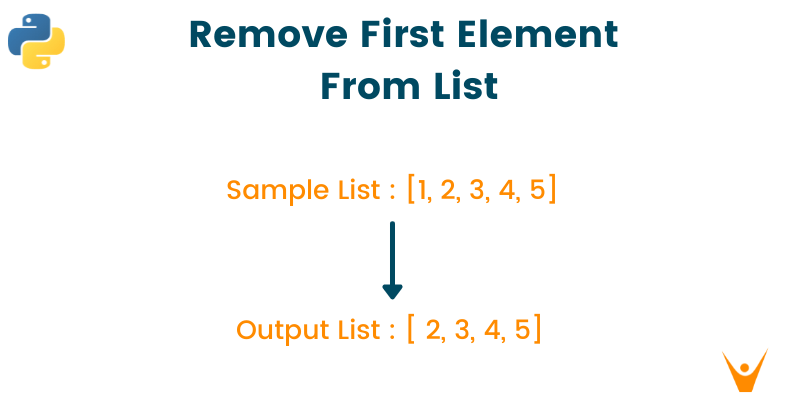
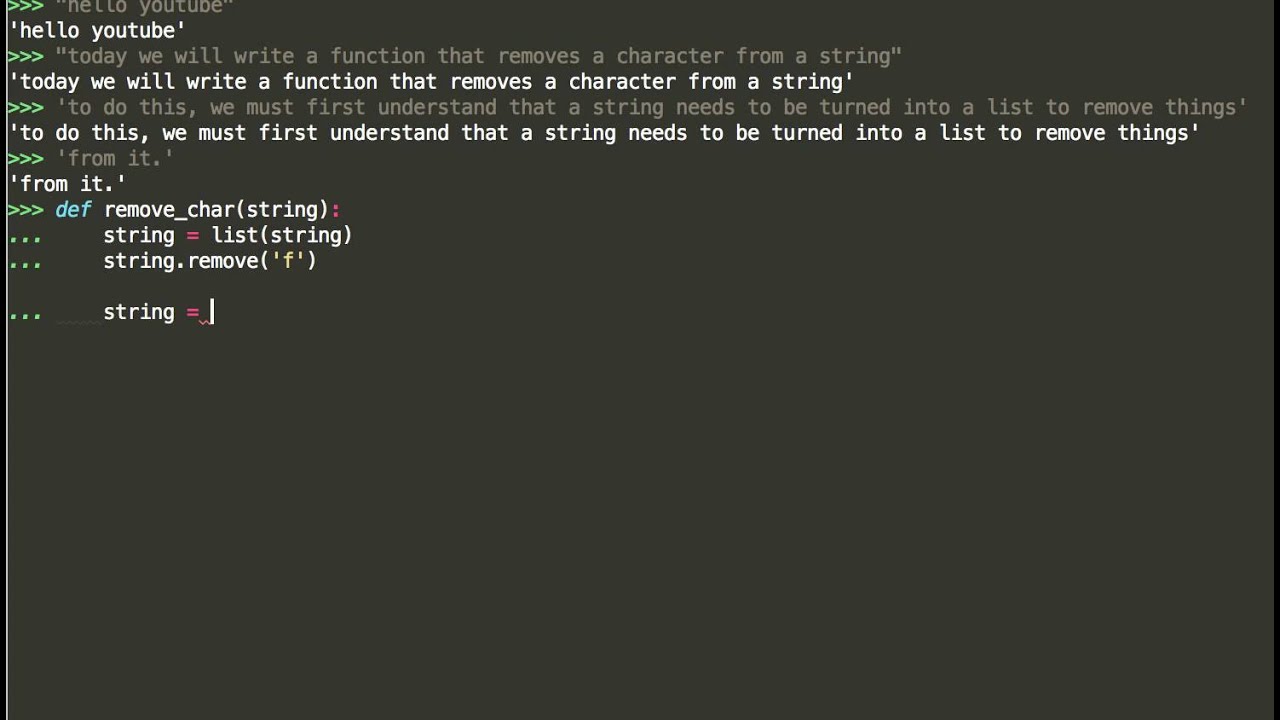
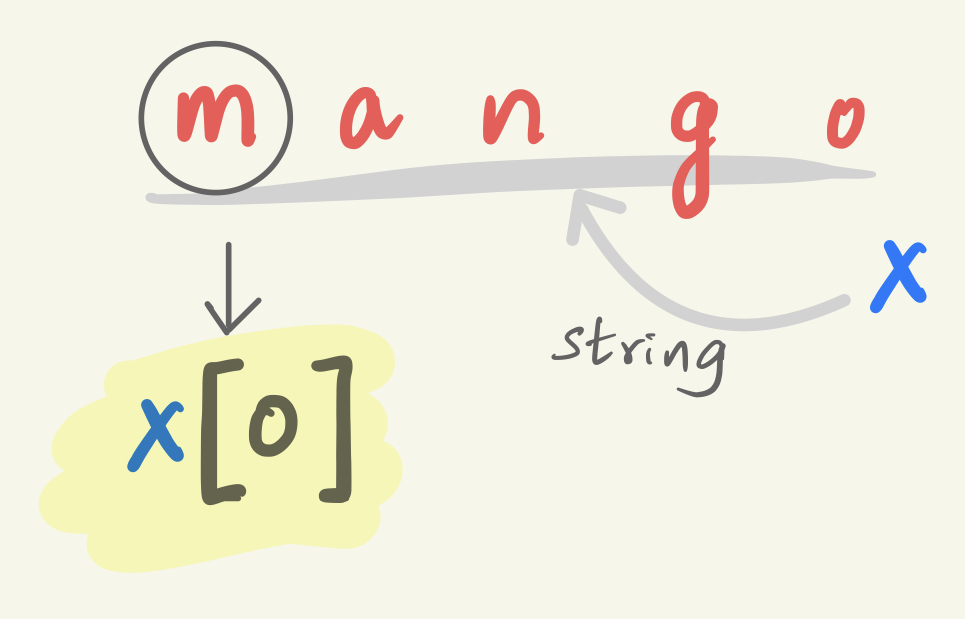

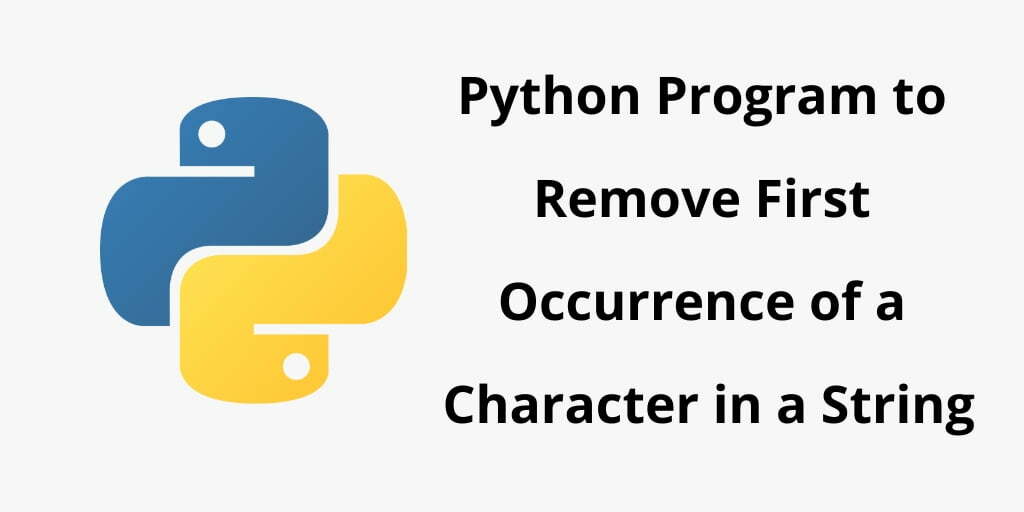
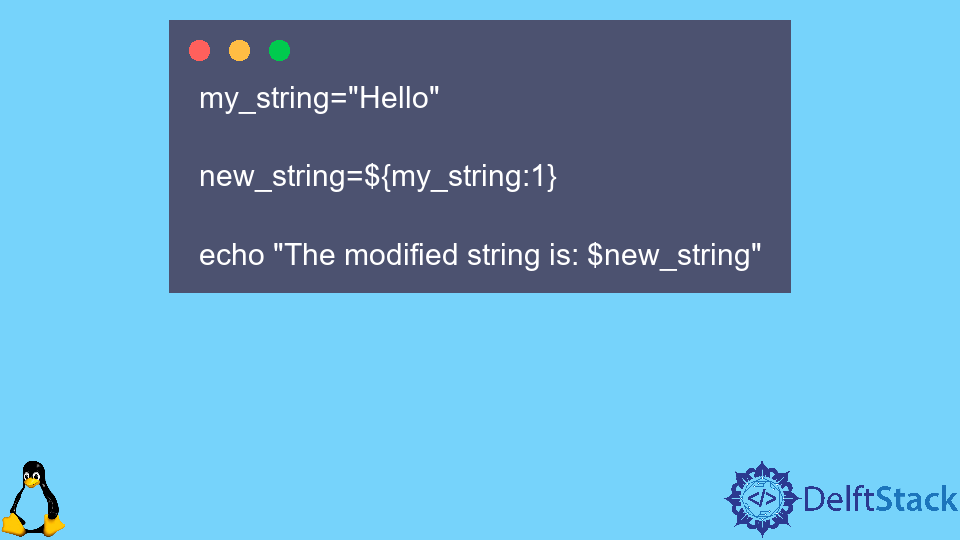
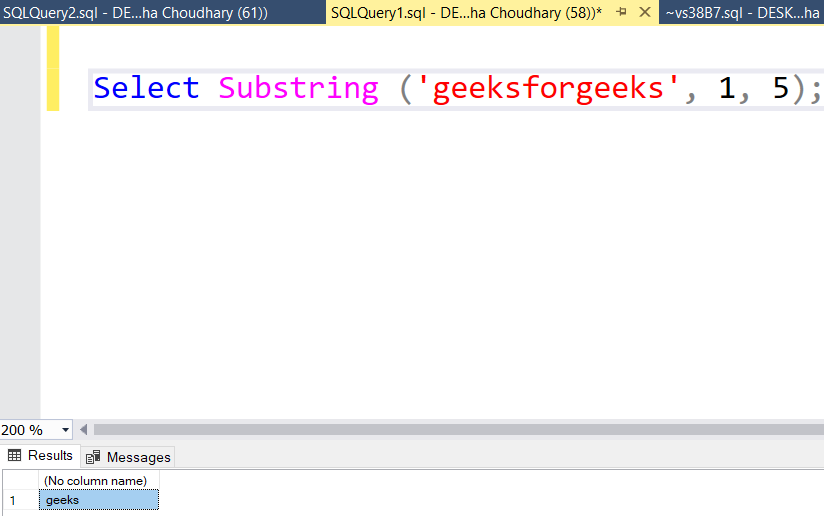

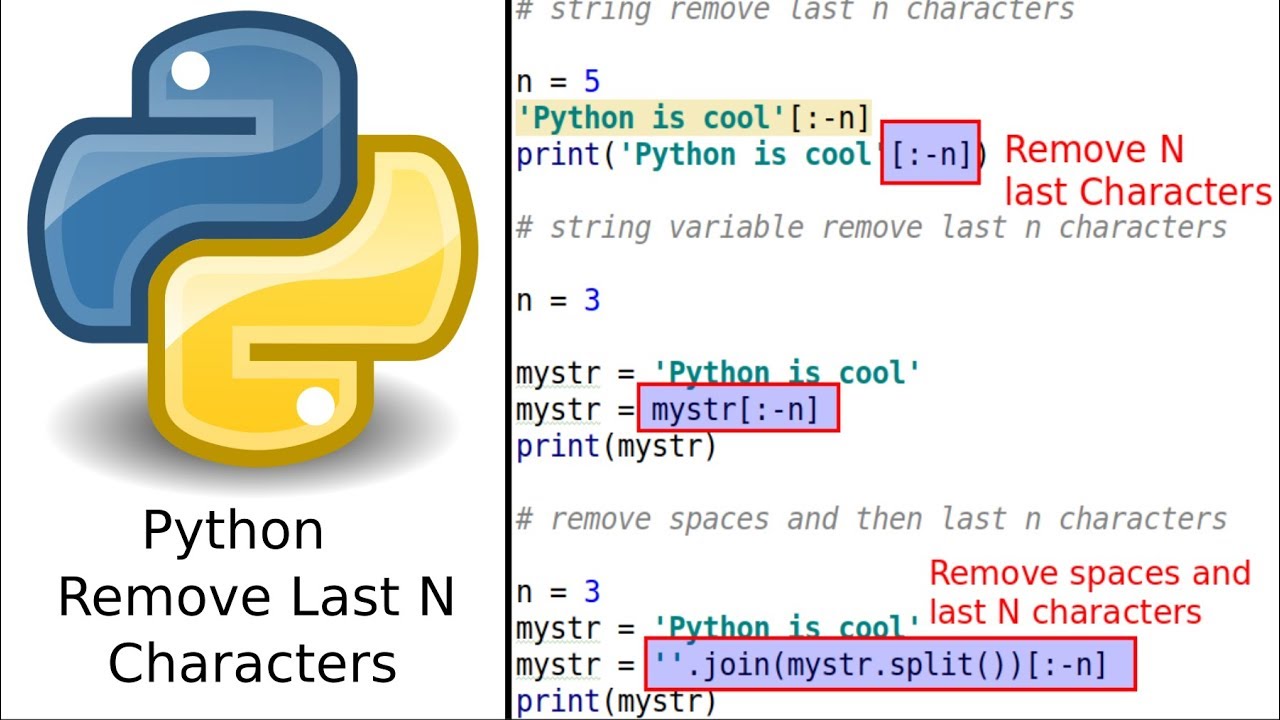
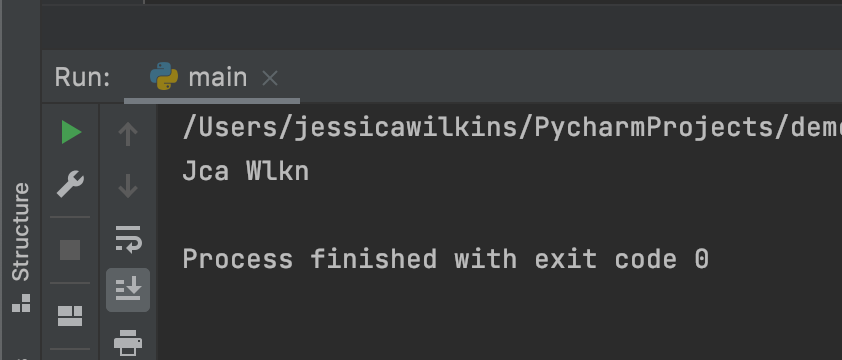
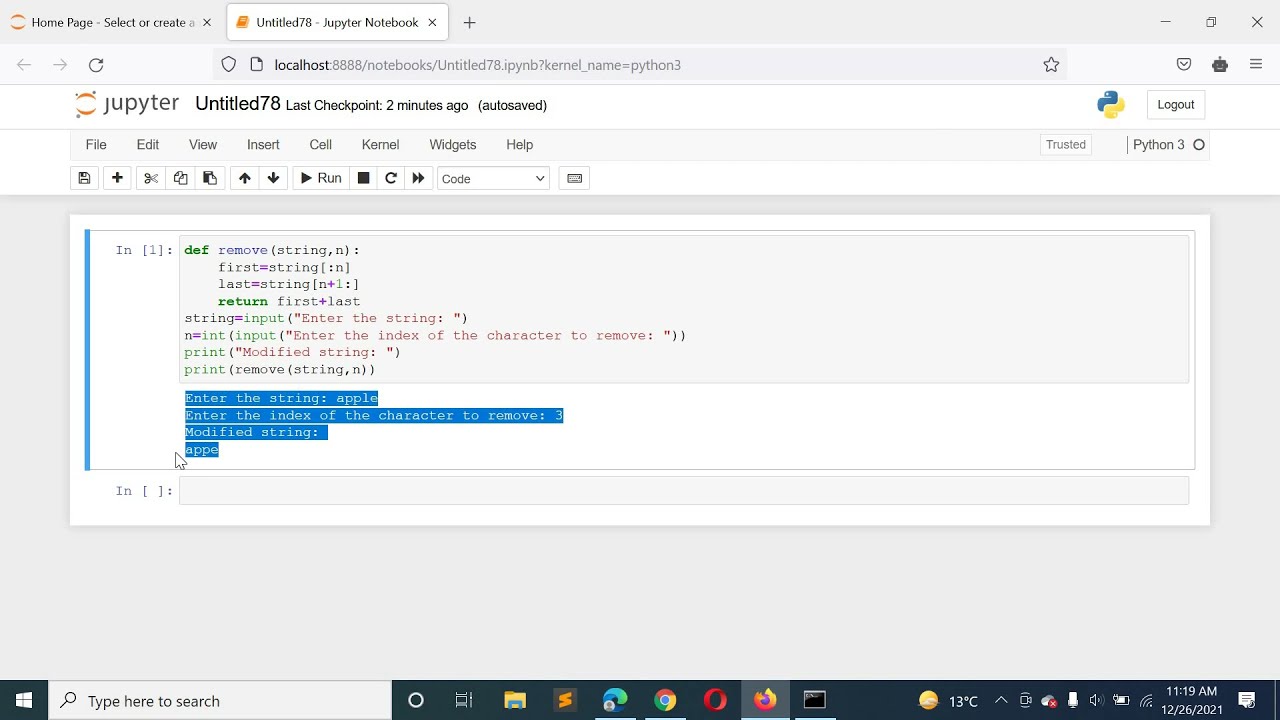

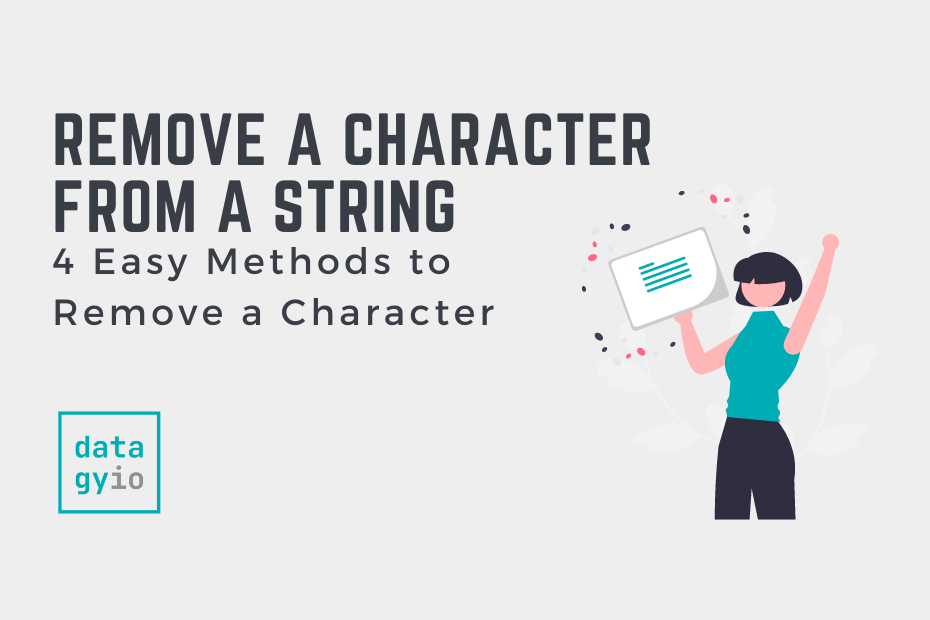
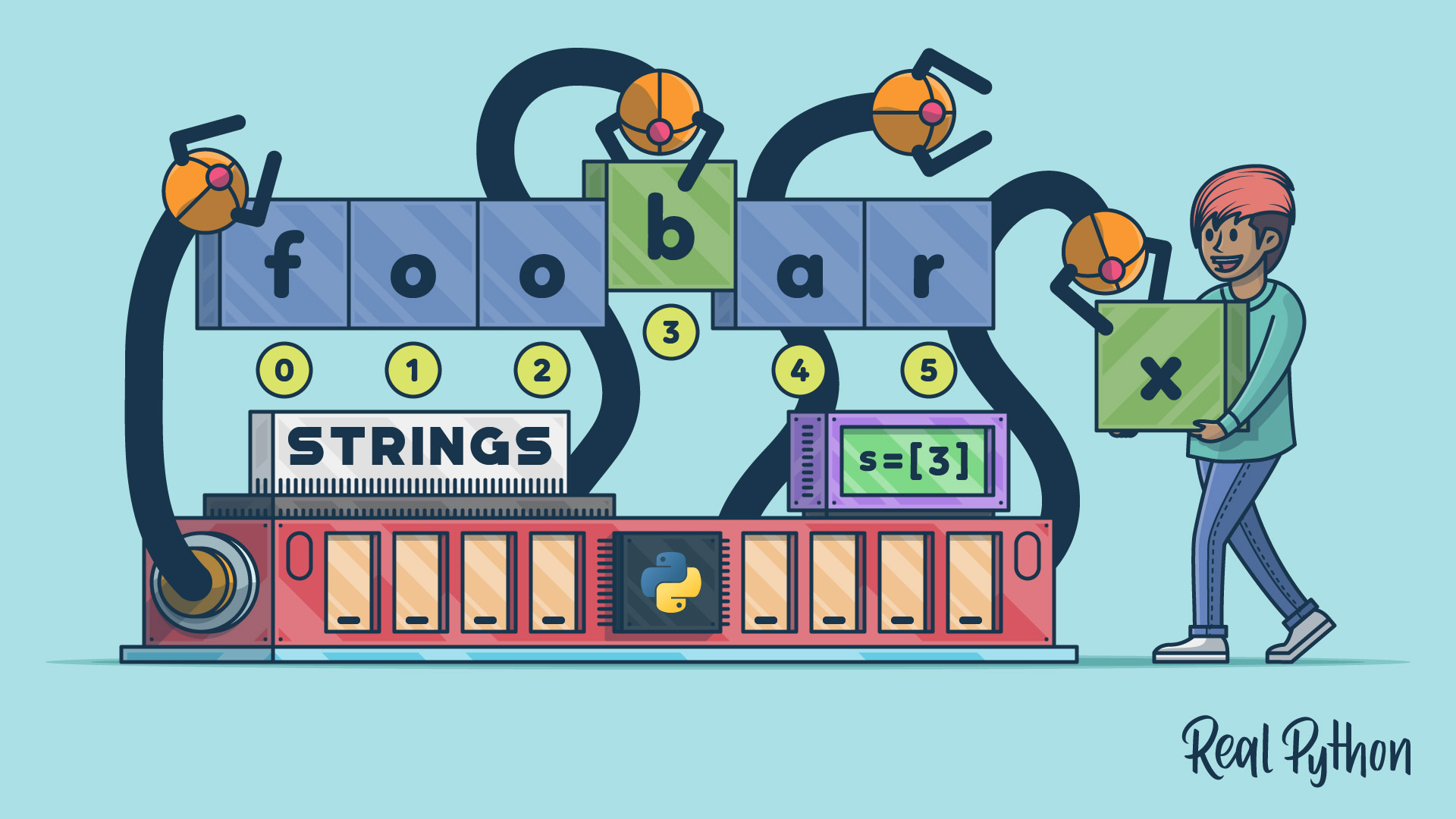
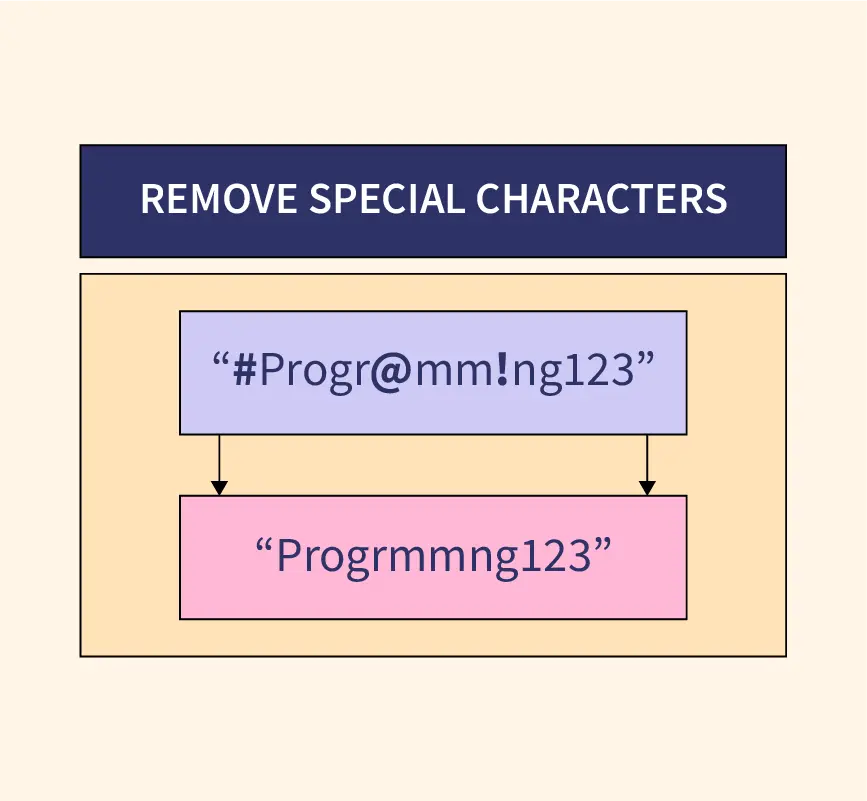

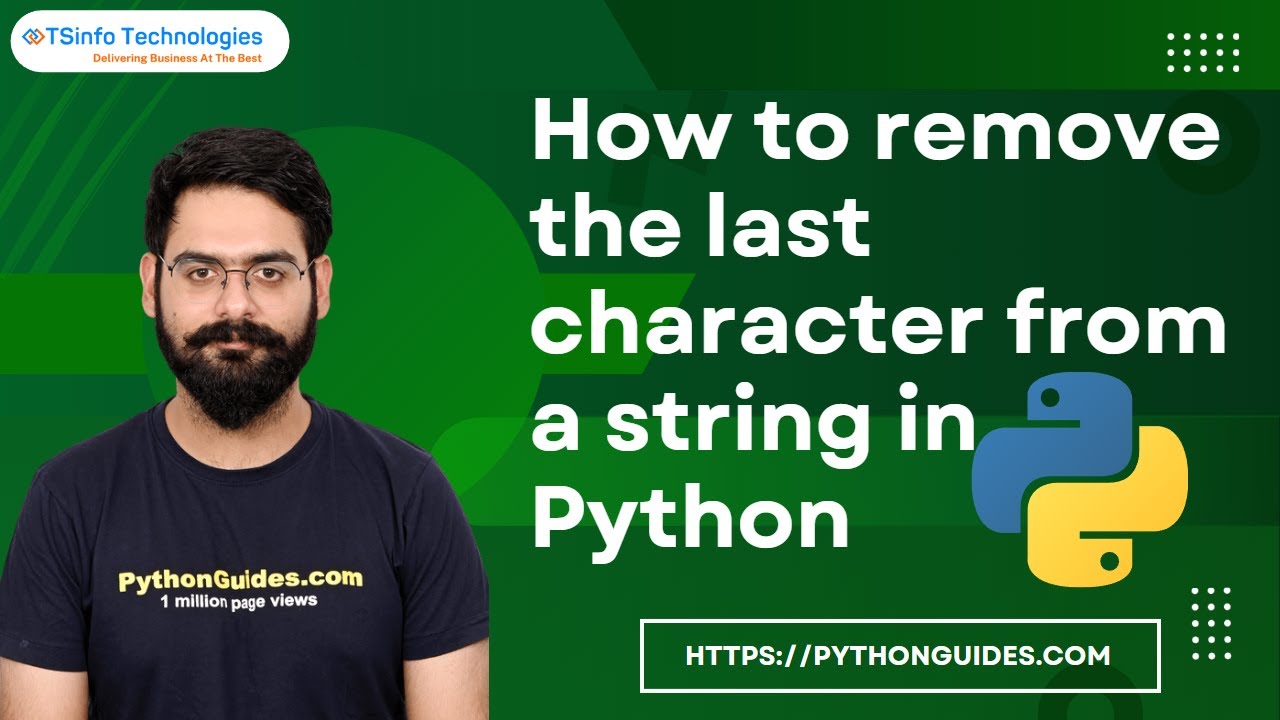
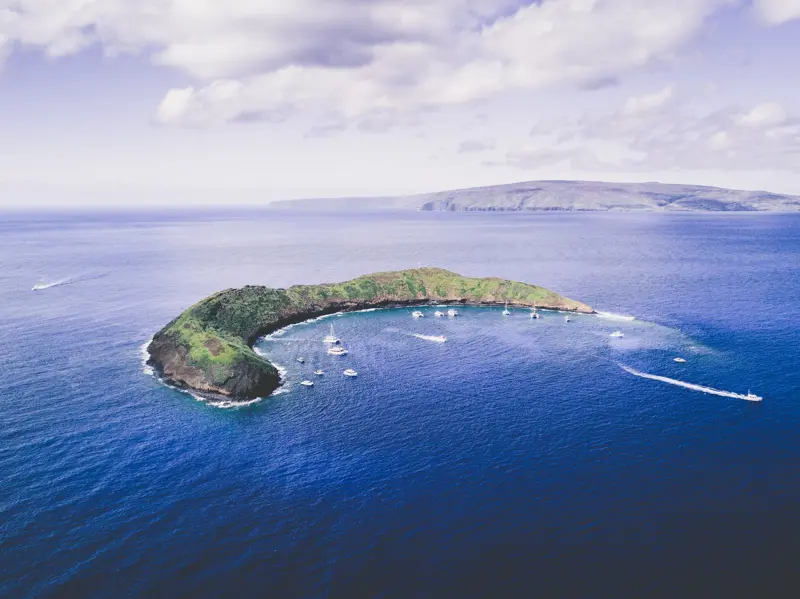
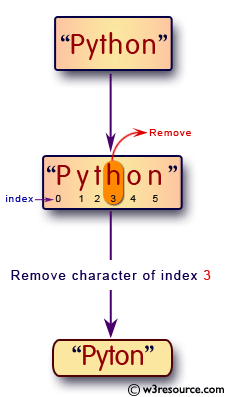
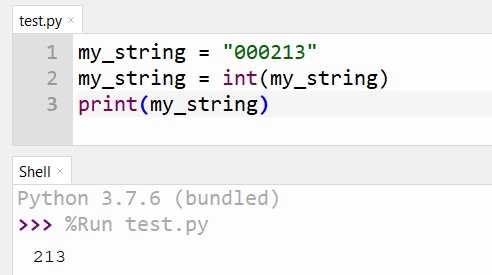
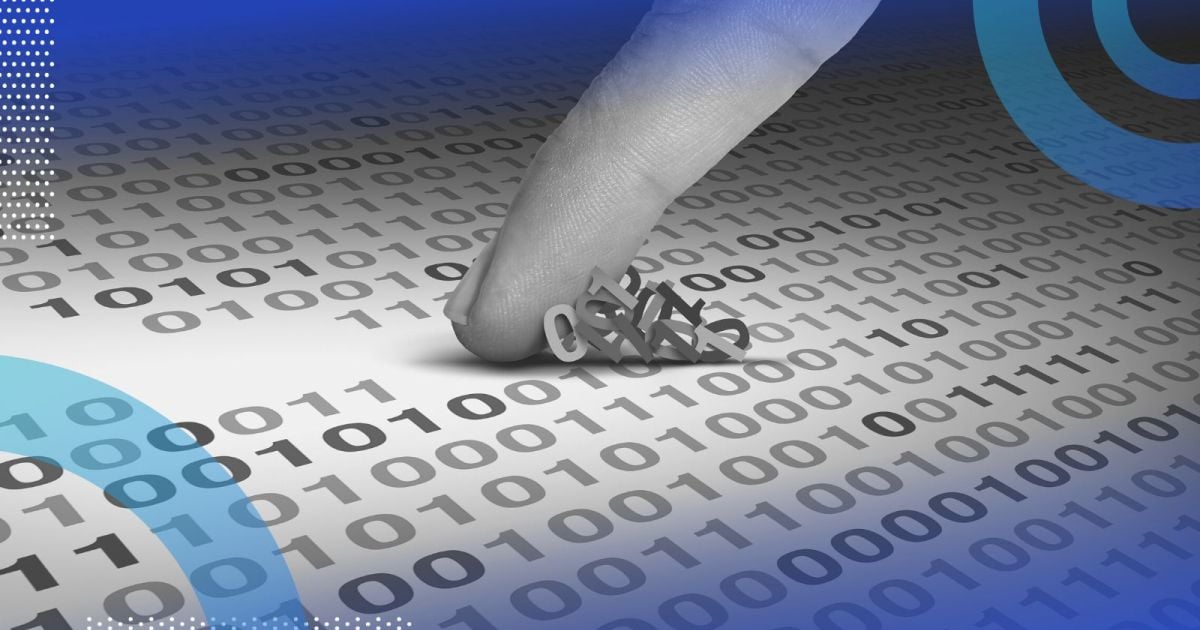
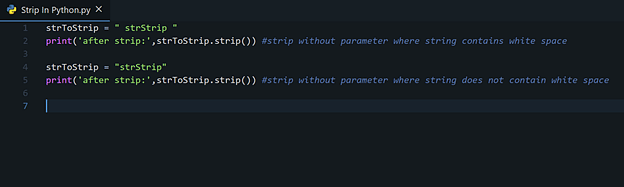
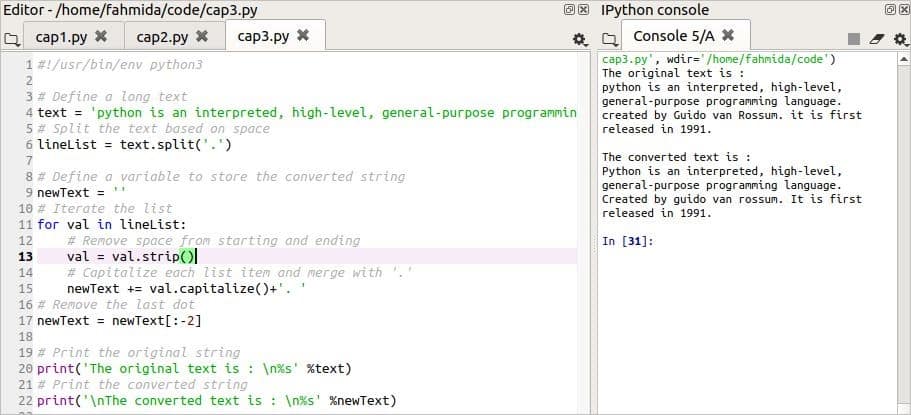
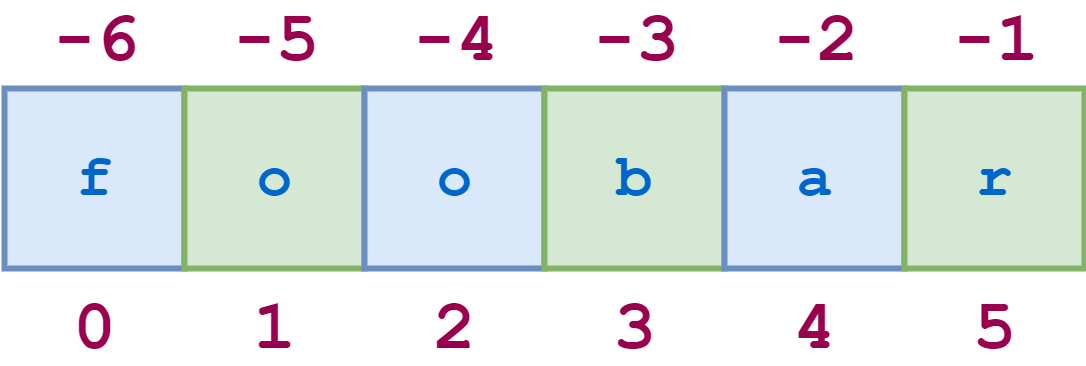
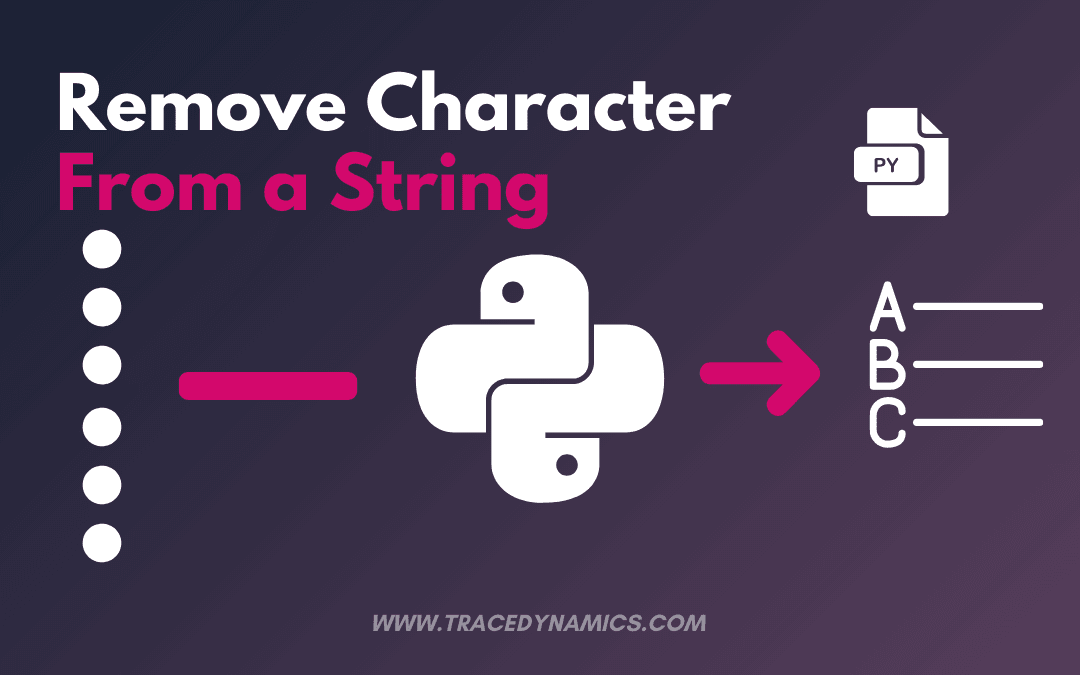
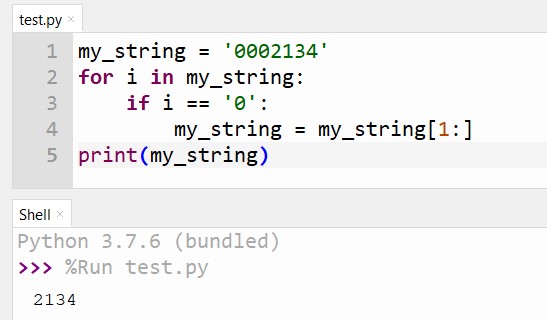
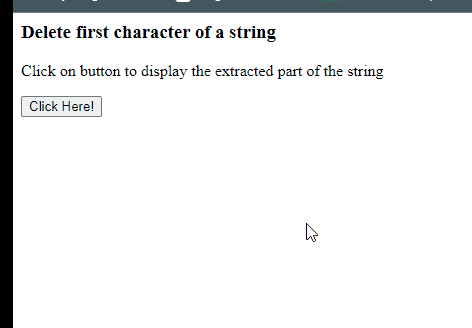


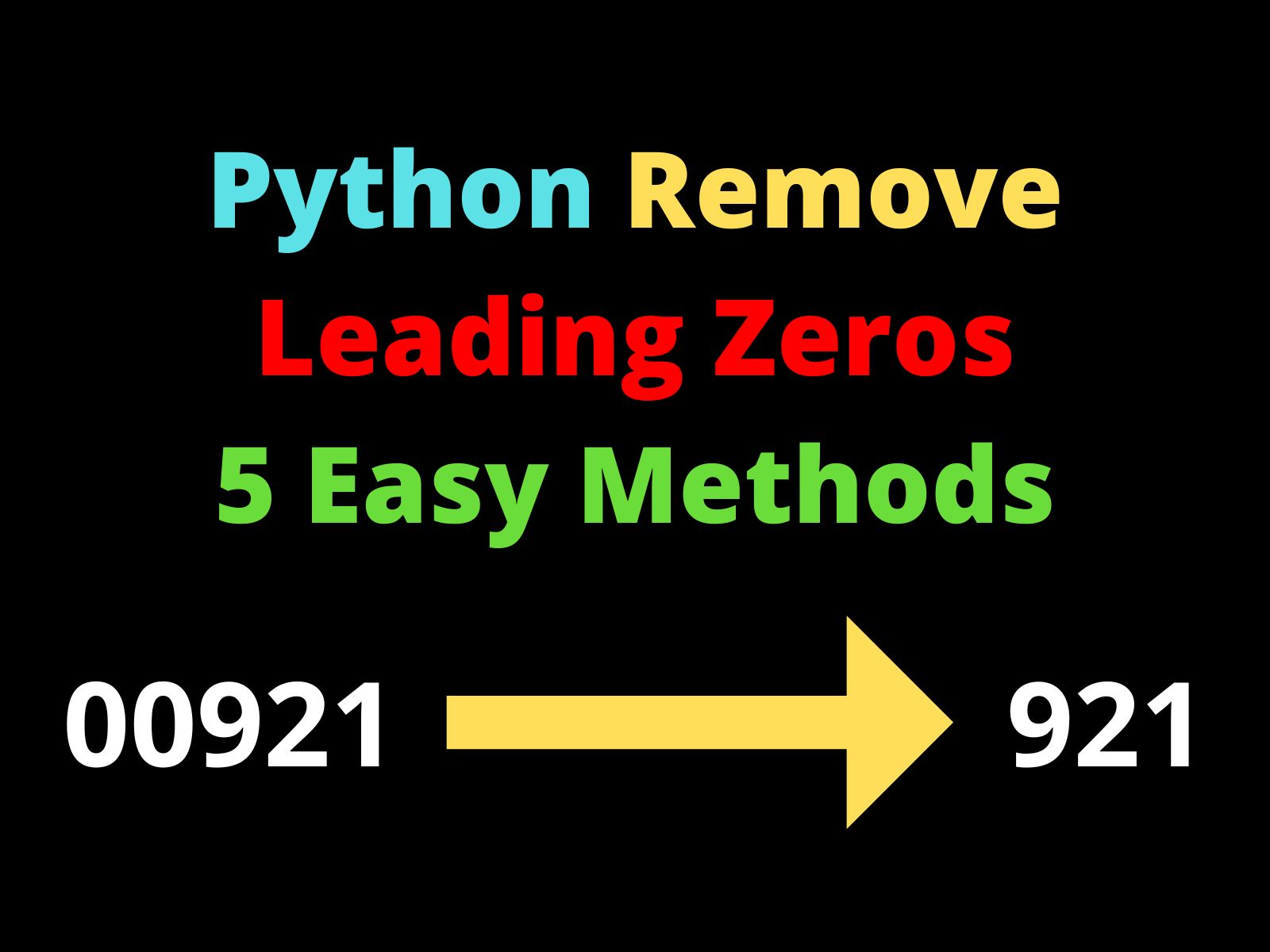
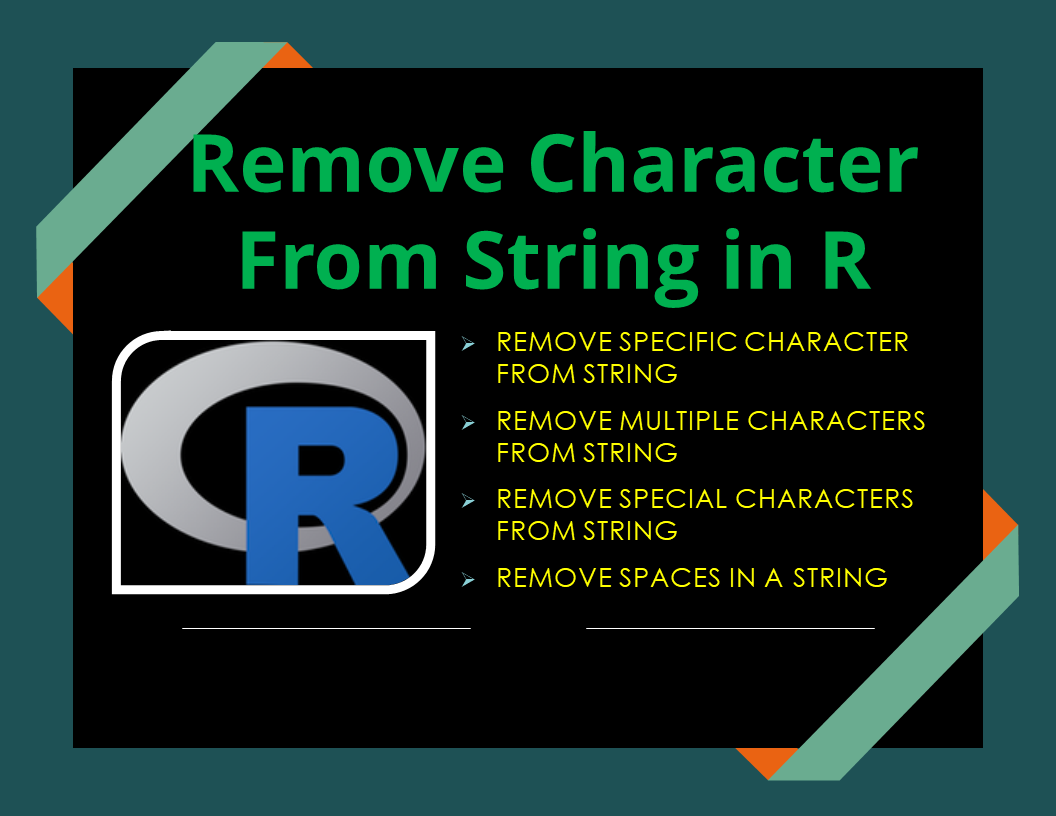
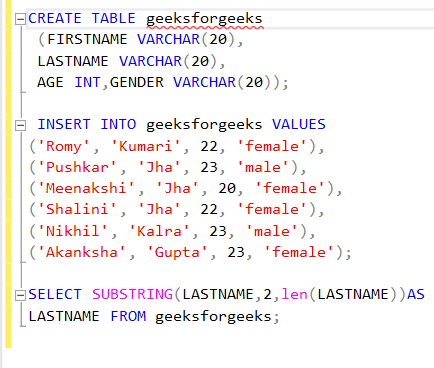
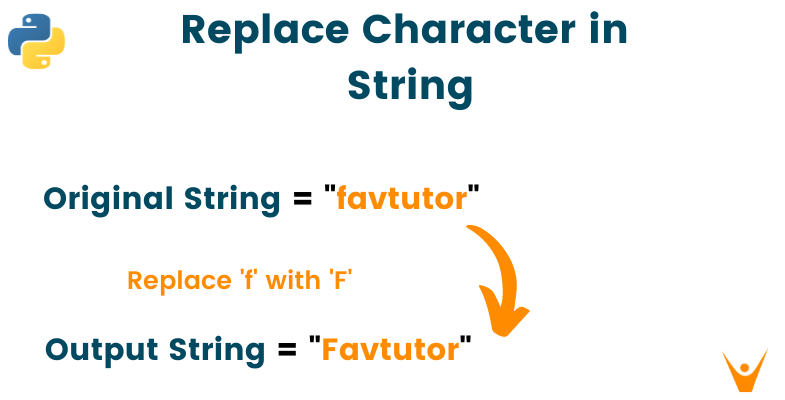
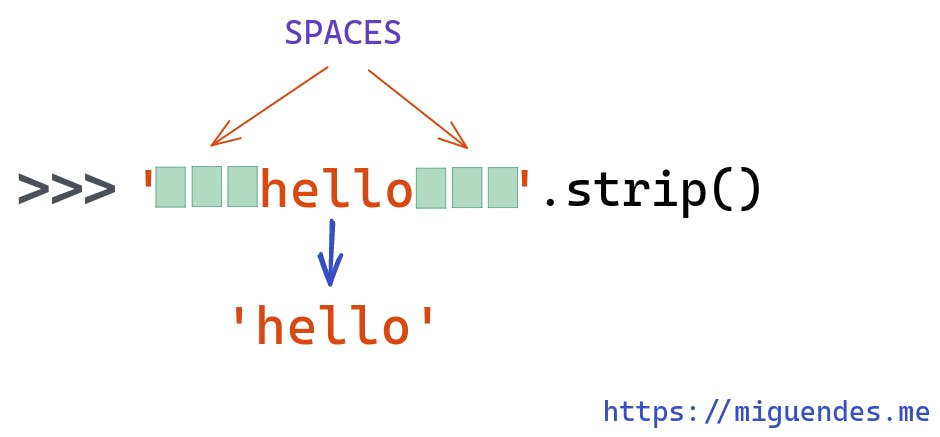
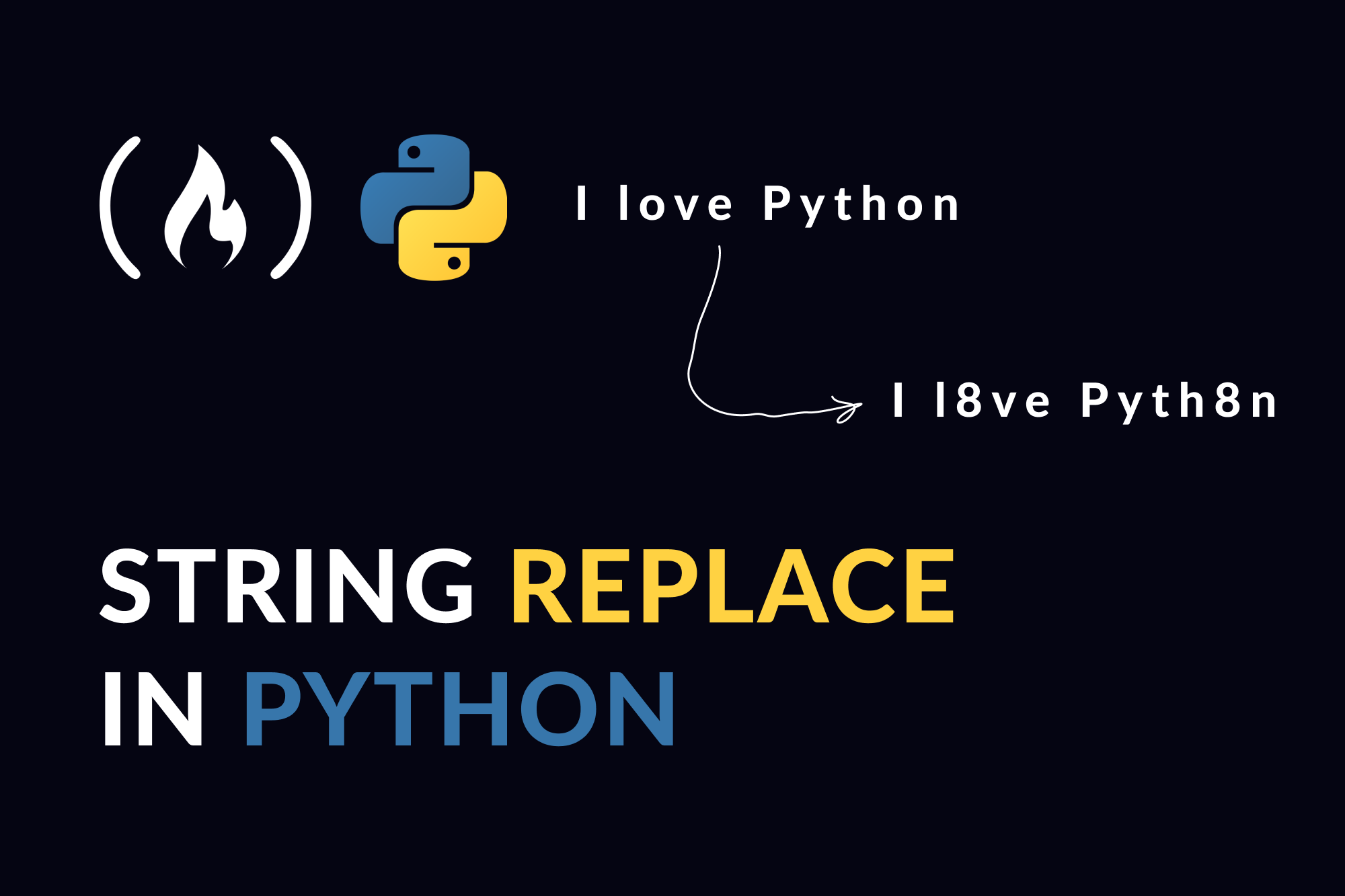
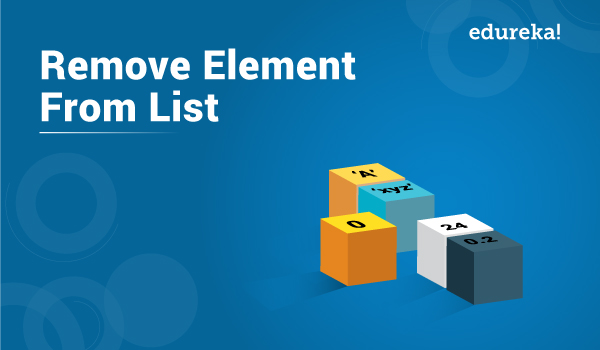
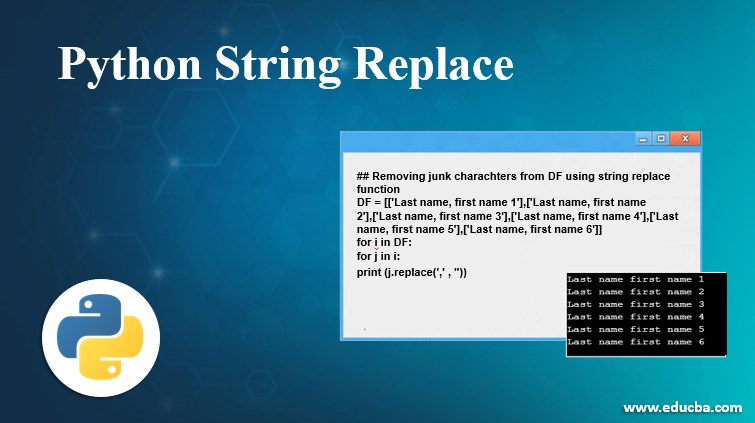

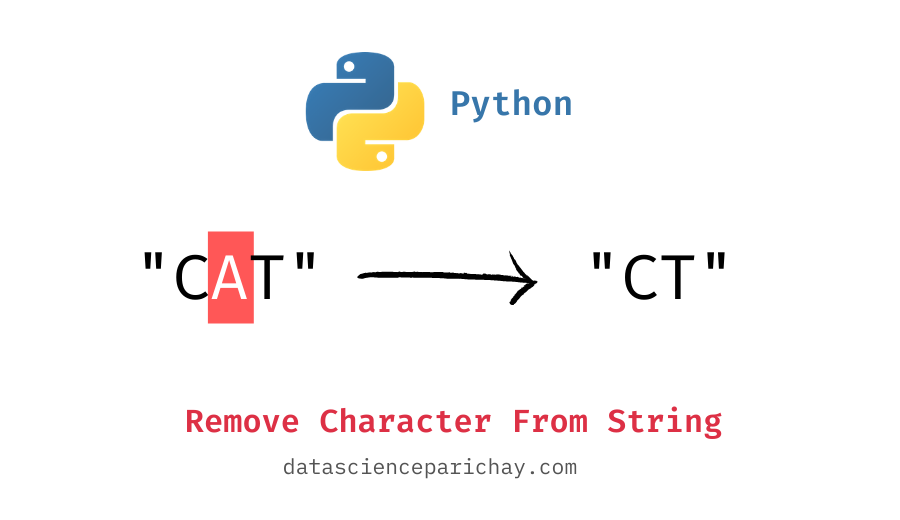
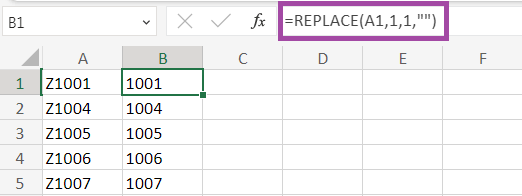
![Python Delete Lines From a File [4 Ways] – PYnative Python Delete Lines From A File [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/python_delete_lines_from_file.png)
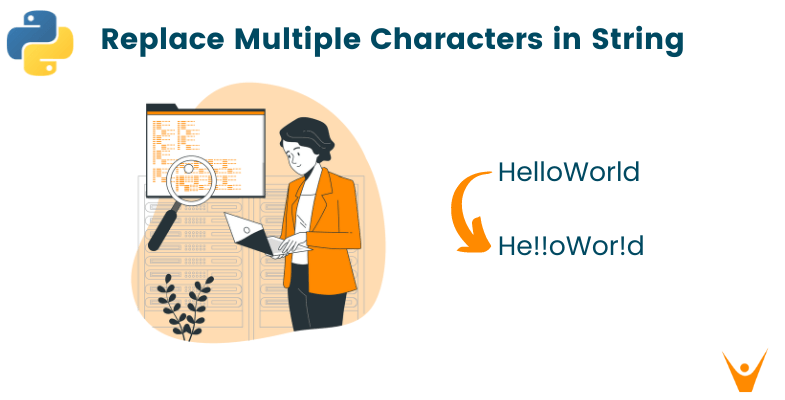
Article link: python remove first character from string.
Learn more about the topic python remove first character from string.
- Remove the first character of a string – python – Stack Overflow
- How To Remove First Character From A String In Python
- Remove First Character From String in Python – Linux Hint
- Remove first character from a Python string – Techie Delight
- Remove the First Character From the String in Python
- How to Remove the First and Last Character from a String in …
- Remove First Character from String in Python – Javatpoint
See more: https://nhanvietluanvan.com/luat-hoc