Node Check If File Exists
1. Checking for file existence using Node.js built-in fs module:
The fs module in Node.js provides several methods for working with the file system. One such method is `fs.existsSync()`, which checks if a file exists synchronously. This method returns a boolean value indicating whether the file exists or not. Here’s an example of how to use it:
“`javascript
const fs = require(‘fs’);
const filePath = ‘/path/to/file.txt’;
const fileExists = fs.existsSync(filePath);
if (fileExists) {
console.log(‘File exists’);
} else {
console.log(‘File does not exist’);
}
“`
2. Implementing synchronous file existence check:
The synchronous approach can be useful when you want your application to wait until the file existence check is complete. However, keep in mind that synchronous methods can block the execution flow, so use them judiciously.
3. Async file existence check with fs.access method:
To perform an asynchronous file existence check, Node.js provides the `fs.access()` method. This method checks if the file exists and if the user has permission to access it. It uses a callback function to handle the result. Here’s an example:
“`javascript
const fs = require(‘fs’);
const filePath = ‘/path/to/file.txt’;
fs.access(filePath, fs.constants.F_OK, (err) => {
if (err) {
console.log(‘File does not exist’);
} else {
console.log(‘File exists’);
}
});
“`
In the above example, we pass `fs.constants.F_OK` as the second argument to check for the existence of the file.
4. Determining file existence using fs.stat method:
Another way to check if a file exists in Node.js is by using the `fs.stat()` method. This method retrieves the file status, including information about the file’s existence, size, and other properties. Here’s an example:
“`javascript
const fs = require(‘fs’);
const filePath = ‘/path/to/file.txt’;
fs.stat(filePath, (err, stat) => {
if (err) {
console.log(‘File does not exist’);
} else {
console.log(‘File exists’);
}
});
“`
The `fs.stat()` method returns an error if the file does not exist, allowing you to handle the scenario accordingly.
5. Cross-platform file existence check with the path module:
The `path` module in Node.js provides utility functions for working with file and directory paths. One of them is `path.existsSync()`, which is a cross-platform method to check if a file exists. Here’s an example:
“`javascript
const path = require(‘path’);
const filePath = ‘/path/to/file.txt’;
const fileExists = path.existsSync(filePath);
if (fileExists) {
console.log(‘File exists’);
} else {
console.log(‘File does not exist’);
}
“`
This method returns a boolean value indicating whether the file exists or not.
6. File existence check with fs.promises in Node.js v10 and above:
If you are using Node.js version 10 or above, you can take advantage of the `fs.promises` API to perform asynchronous file existence checks using promises. Here’s an example:
“`javascript
const fs = require(‘fs’).promises;
const filePath = ‘/path/to/file.txt’;
fs.access(filePath, fs.constants.F_OK)
.then(() => {
console.log(‘File exists’);
})
.catch(() => {
console.log(‘File does not exist’);
});
“`
The `fs.promises` API provides promise-based versions of various fs methods, including `access` for file existence checks.
7. Handling error scenarios during file existence check:
When checking if a file exists, it’s essential to handle errors gracefully. File operations can fail due to various reasons, such as permission issues, invalid file paths, or disk failures. Make sure to capture and handle any potential errors during file existence checks. For example:
“`javascript
const fs = require(‘fs’);
const filePath = ‘/path/to/file.txt’;
try {
fs.accessSync(filePath, fs.constants.F_OK);
console.log(‘File exists’);
} catch (err) {
console.log(‘File does not exist’);
}
“`
In the above example, we use a try-catch block to catch any potential errors thrown by the `fs.accessSync()` method.
8. Best practices for file existence check in Node.js programs:
– Use asynchronous methods (like `fs.access()` or promises with `fs.promises`) whenever possible to avoid blocking the execution flow.
– Handle errors gracefully and provide appropriate error messages.
– Validate file paths before performing existence checks to avoid unexpected errors.
– Use cross-platform compatible methods and modules to ensure your code works consistently across different operating systems.
– Consider using relative paths instead of absolute paths to make your code more flexible and portable.
– Leverage caching mechanisms if your application requires frequent file existence checks to optimize performance.
FAQs:
Q: Can I use the file existence check methods in JavaScript/React?
A: Yes, you can use these methods in JavaScript and React applications as long as you have access to the Node.js environment.
Q: What is the purpose of `mkdirSync` in file existence checks?
A: `mkdirSync` is not directly related to file existence checks. It is a synchronous method provided by the fs module to create a new directory. However, creating a directory before checking if a file exists can be a useful practice in certain scenarios.
Q: How can I check if a file exists in a specific folder using Node.js?
A: You can check if a file exists in a specific folder by providing the full path to the file in the file existence check methods. For example: `const filePath = ‘/path/to/folder/file.txt’;`
Q: Is file existence check possible without using the fs module in Node.js?
A: The fs module is the standard way to work with the file system in Node.js. While there might be other third-party modules or libraries available, it is recommended to use the built-in fs module for file existence checks.
In conclusion, file existence checks are a common requirement in many Node.js applications. Node.js provides various methods and modules to perform these checks efficiently. Use the appropriate method based on your specific use case and consider the best practices mentioned in this article to ensure smooth file existence checks in your Node.js programs.
How To Check A File Exists With Node.Js Tutorial
How To Check If A File Exists In Nodejs?
When working with file systems in Node.js, it is often necessary to check if a file exists before performing any operations on it. Fortunately, Node.js provides several built-in methods that allow you to easily determine the existence of a file. In this article, we will explore different approaches to verify the existence of a file in Node.js and demonstrate how to utilize these methods effectively.
Method 1: fs.stat()
One of the most common ways to check if a file exists in Node.js is by using the `fs.stat()` method. This method provides information about a file or directory specified by the given path. By checking the error object returned by `fs.stat()`, we can determine whether the file exists or not.
Here’s an example that showcases the usage of `fs.stat()`:
“`javascript
const fs = require(‘fs’);
fs.stat(‘path/to/file.txt’, (err, stats) => {
if (err) {
if (err.code === ‘ENOENT’) {
console.log(‘File does not exist’);
} else {
console.error(‘An error occurred while checking the file existence’);
}
} else {
console.log(‘File exists!’);
}
});
“`
In this snippet, `fs.stat()` is called with the file path as the first argument. The callback function receives an error object as the first parameter and the file stats as the second parameter. If the file exists, no error will be present, and we can assume that the file exists.
Method 2: fs.access()
Another way to check if a file exists in Node.js is by employing the `fs.access()` method. This method determines the accessibility of a file or directory specified by the provided path. By using the `fs.constants.F_OK` flag, we can specifically check if the file exists.
Here’s an example that demonstrates the usage of `fs.access()`:
“`javascript
const fs = require(‘fs’);
fs.access(‘path/to/file.txt’, fs.constants.F_OK, (err) => {
if (err) {
console.log(‘File does not exist’);
} else {
console.log(‘File exists!’);
}
});
“`
In this code snippet, `fs.access()` is called with the file path, `fs.constants.F_OK` flag, and a callback function. If the file exists, no error will be thrown, indicating its existence.
Method 3: fs.existsSync()
The `fs.existsSync()` method provides a synchronous way to check if a file exists in Node.js. This method returns a Boolean value that indicates whether the file exists or not.
Here’s an example showcasing `fs.existsSync()`:
“`javascript
const fs = require(‘fs’);
const fileExists = fs.existsSync(‘path/to/file.txt’);
if (fileExists) {
console.log(‘File exists!’);
} else {
console.log(‘File does not exist’);
}
“`
In this snippet, `fs.existsSync()` is used to check if the file exists. If the returned value is `true`, the file exists; otherwise, it does not.
FAQs
Q1. What are the possible errors that can occur while checking file existence?
A1. When checking the existence of a file, you may encounter various errors. The most common error is `ENOENT` (Error NO ENTry), which indicates that the file or directory does not exist. Additionally, you might encounter other errors like permission-related errors or invalid file paths.
Q2. What should I do if I receive a permission-related error while checking file existence?
A2. If you are facing permission-related errors, ensure that the file or directory you are trying to access has appropriate read permissions for the user running the Node.js application.
Q3. How can I check if a file exists in a different directory?
A3. To check if a file exists in a different directory, provide the full path to the file when calling the file existence methods (`fs.stat()`, `fs.access()`, or `fs.existsSync()`).
Q4. Can I check the existence of a directory using these methods?
A4. Yes, the same approach can be used to check the existence of a directory. The methods `fs.stat()`, `fs.access()`, and `fs.existsSync()` work for both files and directories.
In conclusion, determining whether a file exists in Node.js is crucial before performing any operations on it. By utilizing the provided methods (`fs.stat()`, `fs.access()`, or `fs.existsSync()`), you can easily check the existence of a file and handle any errors that may arise. Remember to consider both synchronous and asynchronous approaches depending on your specific use case.
How To Check If A File Exist In Js?
When working with JavaScript, there may be times when you need to determine whether a file exists on a server or in a local directory before performing any further actions. This article aims to provide a comprehensive guide on checking the existence of a file in JavaScript, covering various scenarios and techniques.
Before diving into the specifics, it’s important to understand that JavaScript is primarily a client-side scripting language, meaning it runs within a user’s web browser. However, there are strategies to determine the existence of a file both on the client-side and server-side.
Checking File Existence on the Client-Side:
1. XMLHttpRequest:
One of the most commonly used methods for checking file existence on the client-side is by using the XMLHttpRequest (XHR) object. This object allows us to communicate with a server and retrieve data without refreshing the entire web page.
To check if a file exists on the server, you can send a HEAD request using the XHR object. The server will respond with the file’s metadata if it exists, or return an error status code (e.g., 404 – Not Found) if it doesn’t. Here’s an example:
“`javascript
function checkFileExists(url, callback) {
var xhr = new XMLHttpRequest();
xhr.open(‘HEAD’, url, true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
callback(true);
} else {
callback(false);
}
}
};
xhr.send();
}
// Usage:
checkFileExists(‘path/to/file.txt’, function(exists) {
console.log(exists ? ‘File exists!’ : ‘File does not exist!’);
});
“`
2. Fetch API:
Another approach, introduced in modern browsers, is using the Fetch API to make network requests. The Fetch API provides a more flexible and intuitive way of handling HTTP requests compared to the XHR object. With the Fetch API, you can send a HEAD request to check if a file exists:
“`javascript
function checkFileExists(url) {
return fetch(url, { method: ‘HEAD’ })
.then(response => {
return response.ok;
})
.catch(() => {
return false;
});
}
// Usage:
checkFileExists(‘path/to/file.txt’)
.then(exists => {
console.log(exists ? ‘File exists!’ : ‘File does not exist!’);
});
“`
Checking File Existence on the Server-Side:
Checking file existence on the server-side typically requires a backend scripting language such as Node.js. Here are two common approaches:
1. fs Module:
In Node.js, you can utilize the built-in ‘fs’ module to perform file system operations, including checking if a file exists. The ‘fs’ module provides an ‘access’ function that allows you to check the accessibility of a file or directory. Here’s an example:
“`javascript
const fs = require(‘fs’);
function checkFileExists(filepath) {
return new Promise(resolve => {
fs.access(filepath, fs.constants.F_OK, (error) => {
resolve(!error);
});
});
}
// Usage:
checkFileExists(‘path/to/file.txt’)
.then(exists => {
console.log(exists ? ‘File exists!’ : ‘File does not exist!’);
});
“`
2. HTTP Request:
You can also leverage HTTP requests on the server-side to check file existence. For instance, using Node.js and the ‘http’ module, you can send a HEAD request to the server and receive the response headers. If the HTTP status code is 200, it means the file exists. Here’s an example:
“`javascript
const http = require(‘http’);
function checkFileExists(url) {
return new Promise(resolve => {
http.request(url, { method: ‘HEAD’ }, (res) => {
resolve(res.statusCode === 200);
}).end();
});
}
// Usage:
checkFileExists(‘http://example.com/path/to/file.txt’)
.then(exists => {
console.log(exists ? ‘File exists!’ : ‘File does not exist!’);
});
“`
Frequently Asked Questions (FAQs):
Q: Can JavaScript check if a file exists without making a server request?
A: No, JavaScript cannot directly check file existence without some form of server interaction. However, client-side techniques like XMLHttpRequest allow you to send a request and receive a response indicating file existence.
Q: Is there a way to check file existence in a local directory?
A: Unfortunately, JavaScript cannot directly access a user’s local file system due to security limitations enforced by web browsers. The file existence check should be primarily focused on server-side or remote files.
Q: What should I do if the file existence check fails due to cross-origin restrictions?
A: When the file you want to check exists on a different domain, you may encounter cross-origin restrictions. In such cases, you’ll need to enable Cross-Origin Resource Sharing (CORS) on the server hosting the file or use alternative server-side methods for checking file existence.
In conclusion, checking the existence of a file in JavaScript can be achieved through different approaches based on whether you are working on the client-side or server-side. By using techniques like XMLHttpRequest, Fetch API, or backend modules like ‘fs’ in Node.js, you can determine if a file exists before performing other operations. However, it’s important to consider any security restrictions, cross-origin limitations, and the specific environment in which your JavaScript code runs.
Keywords searched by users: node check if file exists Nodejs check file exists, JavaScript Check local file exists, Mkdirsync, React check file exists, Fs check file exists, JavaScript path check if file exists, Fs exists, Check file exist in folder nodejs
Categories: Top 16 Node Check If File Exists
See more here: nhanvietluanvan.com
Nodejs Check File Exists
### Why do we need to check if a file exists?
Before diving into the methods, let’s understand the importance of checking if a file exists in Node.js. When working with file systems, it is essential to verify the existence of a file before performing any subsequent operations, such as reading, writing, or updating it. By ensuring that a file exists, developers can avoid errors and handle such scenarios gracefully within their applications.
### Method 1: Synchronous Approach
In Node.js, the `fs` module provides synchronous methods to interact with the file system. The `fs.existsSync()` method is one such approach to check whether a file exists or not. This function takes the file path as an argument and returns a boolean value indicating the file’s existence.
Here’s an example demonstrating the usage of `fs.existsSync()`:
“`
const fs = require(‘fs’);
const filePath = ‘path/to/your/file.txt’;
const fileExists = fs.existsSync(filePath);
if (fileExists) {
console.log(‘File exists!’);
} else {
console.log(‘File does not exist!’);
}
“`
### Method 2: Asynchronous Approach
Node.js also provides an asynchronous alternative to checking file existence using the `fs` module. The `fs.access()` method can be used to asynchronously check if a file exists. It takes the file path and a callback function as arguments. The callback function is called with an error object if the file does not exist; otherwise, it is called without an error.
Here’s an example demonstrating the usage of `fs.access()`:
“`javascript
const fs = require(‘fs’);
const filePath = ‘path/to/your/file.txt’;
fs.access(filePath, (err) => {
if (err) {
console.log(‘File does not exist!’);
} else {
console.log(‘File exists!’);
}
});
“`
### Method 3: Promisified Approach
If your codebase uses promises or you prefer working with promises for asynchronous operations, you can utilize the `util.promisify()` method to convert the `fs.access()` method into a promise-based approach.
Here’s an example showcasing the promisified approach:
“`javascript
const fs = require(‘fs’);
const { promisify } = require(‘util’);
const filePath = ‘path/to/your/file.txt’;
const access = promisify(fs.access);
access(filePath)
.then(() => {
console.log(‘File exists!’);
})
.catch((err) => {
console.log(‘File does not exist!’);
});
“`
### FAQs
**Q1: Can I check if a file exists without using the ‘fs’ module in Node.js?**
No, as file system operations are core functionalities, you need to use the ‘fs’ module or any other module built on top of it to check if a file exists in Node.js.
**Q2: Is there any performance difference between synchronous and asynchronous approaches?**
Yes, there is a performance difference between synchronous and asynchronous approaches. Asynchronous methods do not block the execution flow, allowing other operations to proceed in the meantime. Synchronous methods, on the other hand, halt the execution until the operation completes. Therefore, if performance is a concern for your application, it is recommended to use the asynchronous approach.
**Q3: How can I handle permission-related errors while checking file existence?**
To handle errors related to permissions while accessing files, you can wrap the checking operations within a `try-catch` block. In case of any error, you can handle them gracefully and take appropriate actions, such as providing a meaningful error message to the users or retrying the operation with elevated permissions.
### Conclusion
In this article, we explored different methods to check if a file exists in Node.js. We discussed the synchronous, asynchronous, and promisified approaches using the `fs` module. It is essential to verify the existence of a file before performing any further operations to ensure error-free and robust code. By utilizing these methods, developers can build more reliable file handling mechanisms within their Node.js applications.
Javascript Check Local File Exists
Introduction:
When working with JavaScript, it is often necessary to check if a local file exists. This can be particularly useful when handling file uploads, reading external data, or performing file manipulation tasks. In this article, we will explore various methods to accomplish this task using JavaScript and delve into their advantages and disadvantages.
Methods to Check Local File Existence:
1. XMLHttpRequest:
One of the most common methods to check if a local file exists is to use the XMLHttpRequest object. This object allows JavaScript to make HTTP requests, which can include local file paths. By sending a HEAD request to the file’s URL, we can check the response status code to determine if the file exists or not. Here is an example:
“`javascript
function checkFileExists(url, callback) {
var xhr = new XMLHttpRequest();
xhr.open(“HEAD”, url, true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
callback(xhr.status === 200);
}
};
xhr.send();
}
checkFileExists(“path/to/file.txt”, function(exists) {
console.log(“File exists:”, exists);
});
“`
Advantages: This method is widely supported and can be used in both modern and older browsers.
Disadvantages: The file path must be accessible via a URL, as XMLHttpRequest cannot access local directories directly.
2. Fetch API:
Introduced in modern browsers, the Fetch API provides another approach to check if a file exists. By using the fetch() function with the “HEAD” method, we can check the response status code similar to the XMLHttpRequest method. Here is an example:
“`javascript
function checkFileExists(url) {
return fetch(url, { method: “HEAD” })
.then(response => response.ok)
.catch(() => false);
}
checkFileExists(“path/to/file.txt”)
.then(exists => {
console.log(“File exists:”, exists);
});
“`
Advantages: The Fetch API provides a more streamlined approach with a Promise-based interface.
Disadvantages: As with the XMLHttpRequest method, the file path must be accessible via a URL.
3. Node.js fs module:
If you are working with JavaScript on the server-side, particularly with Node.js, you can use the built-in fs (file system) module to check if a local file exists. Here is an example:
“`javascript
const fs = require(“fs”);
function checkFileExists(path) {
try {
fs.accessSync(path);
return true;
} catch (e) {
return false;
}
}
console.log(“File exists:”, checkFileExists(“path/to/file.txt”));
“`
Advantages: This method can be used with Node.js, enabling file existence checks in server-side applications.
Disadvantages: Limited to server-side usage and not applicable for client-side JavaScript running in the browser.
Frequently Asked Questions (FAQs):
Q1. Can JavaScript check if a local file exists without using external libraries?
Yes, both the XMLHttpRequest and Fetch API methods described above do not require any external libraries. They utilize built-in browser functionalities.
Q2. How do I handle cross-origin issues when checking file existence?
If you encounter cross-origin issues when using the XMLHttpRequest or Fetch API methods, you may need to configure appropriate CORS (Cross-Origin Resource Sharing) headers on the server hosting the file. This allows the browser to make requests to the file from different origins.
Q3. Can JavaScript check file existence on the client’s local filesystem?
No, JavaScript running in the browser cannot directly access the client’s local filesystem for security reasons. It can only interact with files accessible via URLs or submitted through file input fields.
Q4. Is it possible to check file existence asynchronously?
Yes, both the XMLHttpRequest and Fetch API methods provide asynchronous operations, allowing you to perform other tasks while waiting for the file existence check to complete.
Conclusion:
Checking if a local file exists using JavaScript is a crucial task in many scenarios, such as handling file uploads or fetching external data. In this article, we explored three different methods to achieve this goal: the XMLHttpRequest, Fetch API, and Node.js fs module. Each method has its own advantages and disadvantages, depending on whether you are working on the client-side or server-side. By understanding these methods, you will be able to determine the best approach for your specific use case and effectively handle local file existence checks using JavaScript.
Mkdirsync
Introduction
When it comes to managing file systems and directories in a complex computing environment, efficient and reliable directory creation is crucial. Mkdirsync, a powerful tool developed primarily for Linux systems, provides a convenient way to create directories in a synchronous manner. In this article, we will delve into the ins and outs of Mkdirsync, discussing its main features, benefits, and potential drawbacks. Additionally, a FAQs section will address common queries and provide further clarity on this topic.
Understanding Mkdirsync
Mkdirsync is a command-line utility that facilitates the creation of directories synchronously, meaning that it ensures the completion of one directory creation before starting on another. This approach ensures that the creation of directories is done in a well-ordered and synchronized manner, eliminating the possibility of concurrency-related issues during the process.
Key Features of Mkdirsync
1. Atomic Directory Creation: Mkdirsync guarantees atomic directory creation, making it a reliable choice for handling critical operations. The atomicity ensures that either all directories are successfully created, or none at all, preventing any partial or inconsistent creation.
2. Recursive Mode: The recursive mode of Mkdirsync allows for the creation of nested directories effortlessly. With a single command, you can create an entire directory tree, eliminating the need for multiple operations.
3. Error Handling: Mkdirsync provides comprehensive error handling capabilities. It detects issues during the directory creation process and reports detailed error messages, enabling users to identify and address any problems that may arise.
4. Durability: The tool is designed to be resilient, even in the face of system failures or interruptions during the creation process. It ensures that directories are created reliably and can recover from potential errors or system failures seamlessly.
Benefits of Using Mkdirsync
1. Ensures Data Integrity: By guaranteeing atomic directory creation, Mkdirsync safeguards data integrity and consistency in the file system. Any potential interruption or error during the process does not compromise the overall state of the directory structure.
2. Simplifies Complex Directory Creation: Mkdirsync simplifies the creation of complex directory structures with its recursive mode. Whether you need to create multiple directories or a deep directory hierarchy, Mkdirsync reduces the effort and time required for these operations.
3. Enhances System Reliability: The robust error handling and durability of Mkdirsync contribute to overall system reliability. Even in the face of system failures or interruptions, Mkdirsync can help maintain the stability and consistency of the file system.
4. Efficient and Automated: Mkdirsync allows for automating directory creation tasks, thereby saving time and effort. With a single command, users can create multiple directories simultaneously, increasing productivity and efficiency.
Drawbacks and Limitations of Mkdirsync
1. Platform-Specific: Mkdirsync is primarily developed for Linux systems, which means it may not be directly available for other operating systems. Users of different platforms may need to explore alternative tools or consider cross-platform compatibility options.
2. Command-Line Interface: While the command-line interface provides flexibility, it may not be as intuitive for all users. Those unfamiliar with using command-line tools might require a learning curve to effectively leverage Mkdirsync’s features.
3. File System Dependencies: Certain file systems may not fully support all the features of Mkdirsync, leading to potential compatibility issues. It is advisable to verify compatibility with the specific file system in use before relying heavily on Mkdirsync.
Frequently Asked Questions (FAQs)
Q1. Can Mkdirsync be used on operating systems other than Linux?
A1. Mkdirsync is primarily developed for Linux systems but can potentially be used on other Unix-like operating systems. However, direct support for other platforms may be limited, requiring potential workarounds or alternative tools.
Q2. How does Mkdirsync handle errors during the directory creation process?
A2. Mkdirsync provides detailed error messages, allowing users to identify and troubleshoot any errors encountered during directory creation. It ensures that partial or inconsistent directory structures are not created.
Q3. Is Mkdirsync suitable for creating a large number of directories?
A3. Yes, Mkdirsync is designed to handle directory creation efficiently, even for large numbers. Its atomicity and error handling capabilities make it a reliable choice for managing a significant volume of directories.
Q4. Does Mkdirsync support the creation of nested directories?
A4. Yes, Mkdirsync supports the creation of nested directories using the recursive mode. This feature simplifies the process of creating complex directory structures effortlessly.
Q5. Can Mkdirsync recover from system failures during the directory creation process?
A5. Mkdirsync’s durability mechanisms ensure that it can recover from system failures or interruptions during the directory creation process. It maintains the overall integrity of the file system.
Conclusion
Mkdirsync is a powerful tool designed for synchronous directory creation in Linux systems. With its atomicity, error handling capabilities, and easy recursive mode, Mkdirsync simplifies complex directory creation tasks and ensures data integrity in the file system. While it may have platform-specific limitations and require familiarity with command-line tools, Mkdirsync enables users to create directories efficiently and reliably. By addressing potential FAQs, we hope this article has provided comprehensive insights into Mkdirsync, highlighting its features, benefits, and limitations.
Images related to the topic node check if file exists
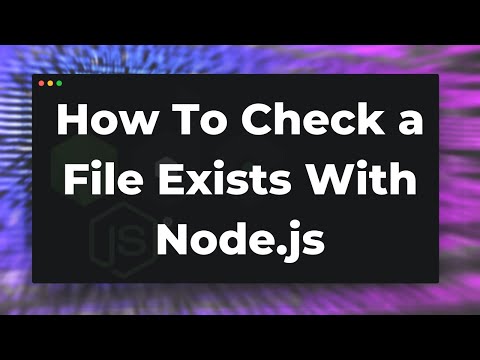
Found 10 images related to node check if file exists theme
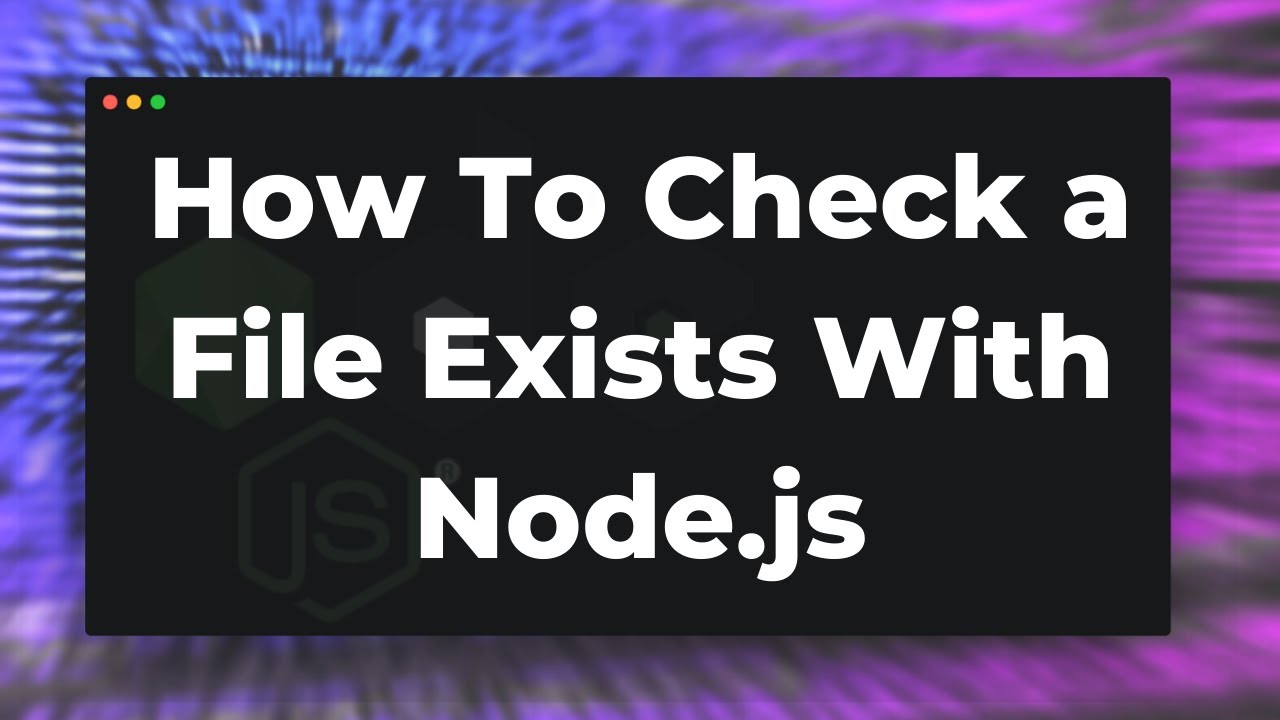
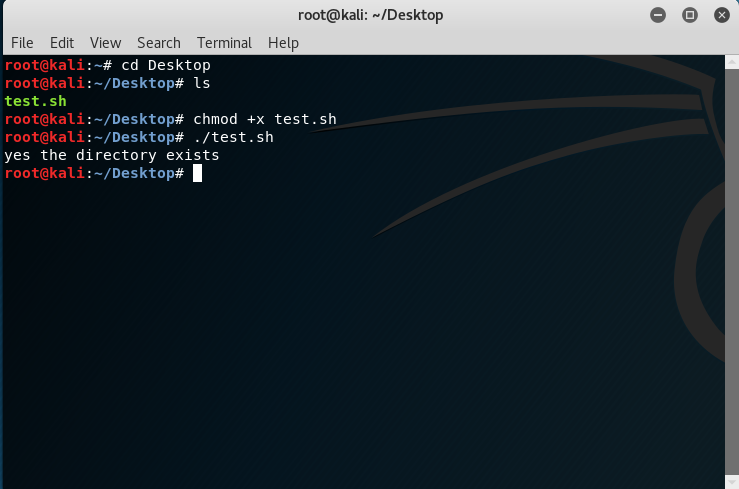


![How to check if a Directory exists in Node.js [6 Ways] | bobbyhadz How To Check If A Directory Exists In Node.Js [6 Ways] | Bobbyhadz](https://bobbyhadz.com/images/blog/check-if-directory-exists-in-node-js/check-if-directory-exists-using-fs-statsync.webp)
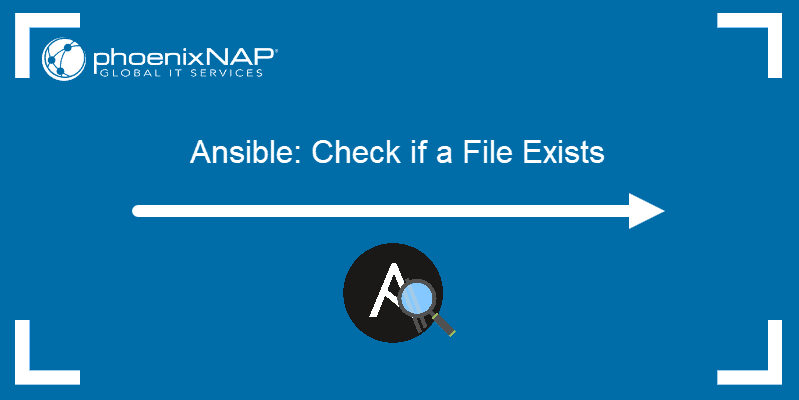
![How to check if a Directory exists in Node.js [6 Ways] | bobbyhadz How To Check If A Directory Exists In Node.Js [6 Ways] | Bobbyhadz](https://bobbyhadz.com/images/blog/check-if-directory-exists-in-node-js/check-if-directory-exists-using-fs-access.webp)

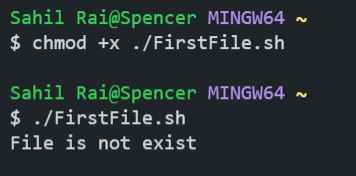
![SOLVED] Check if file or directory exists in Node.js | GoLinuxCloud Solved] Check If File Or Directory Exists In Node.Js | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/NODEJS_CHECK_FILE_DIR_EXISTS.jpg)
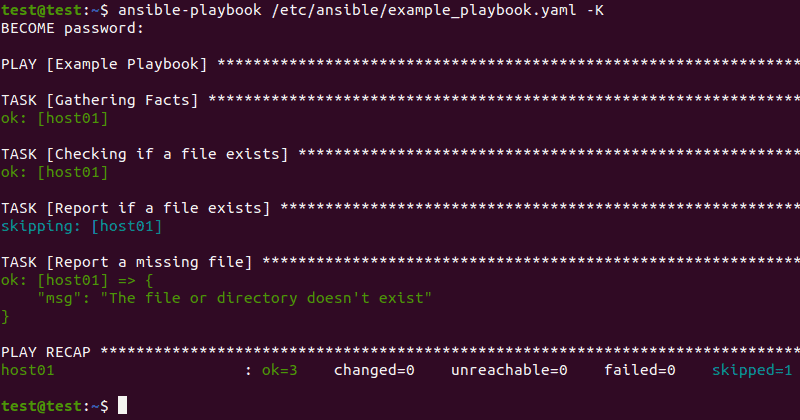

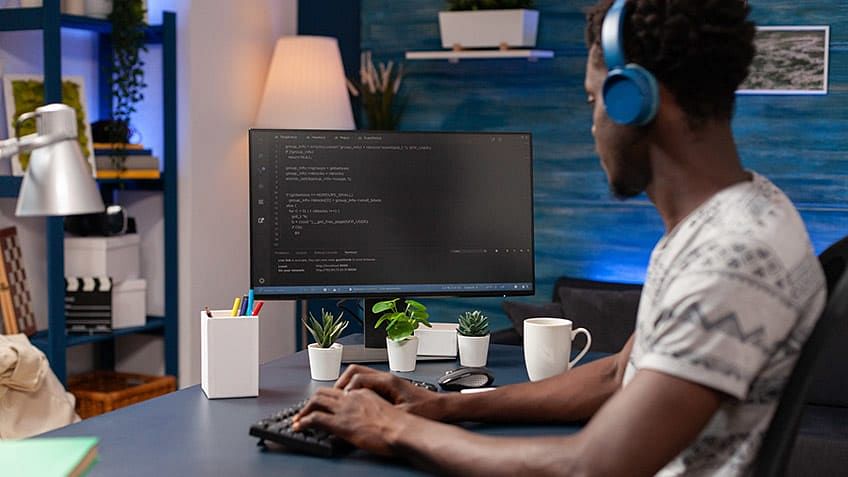

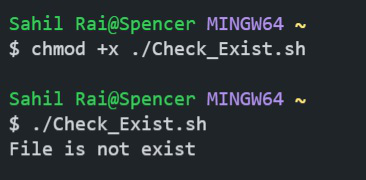
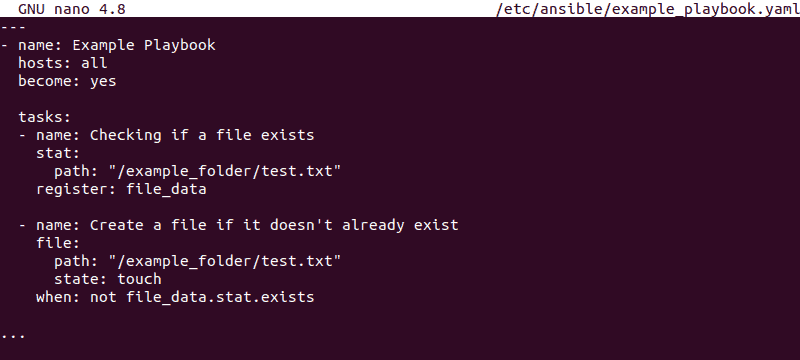
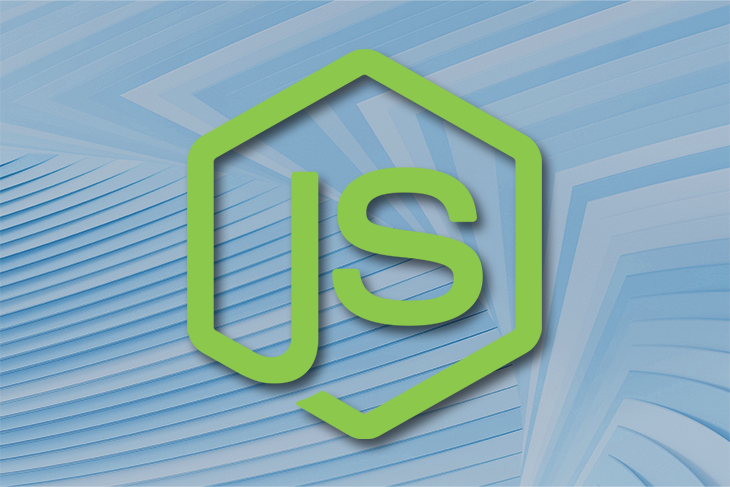


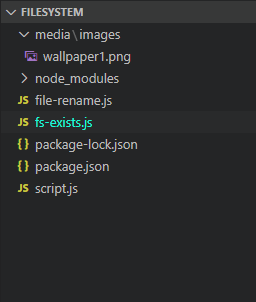

![SOLVED] Check if file or directory exists in Node.js | GoLinuxCloud Solved] Check If File Or Directory Exists In Node.Js | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/lab-setup-2-3.jpg)


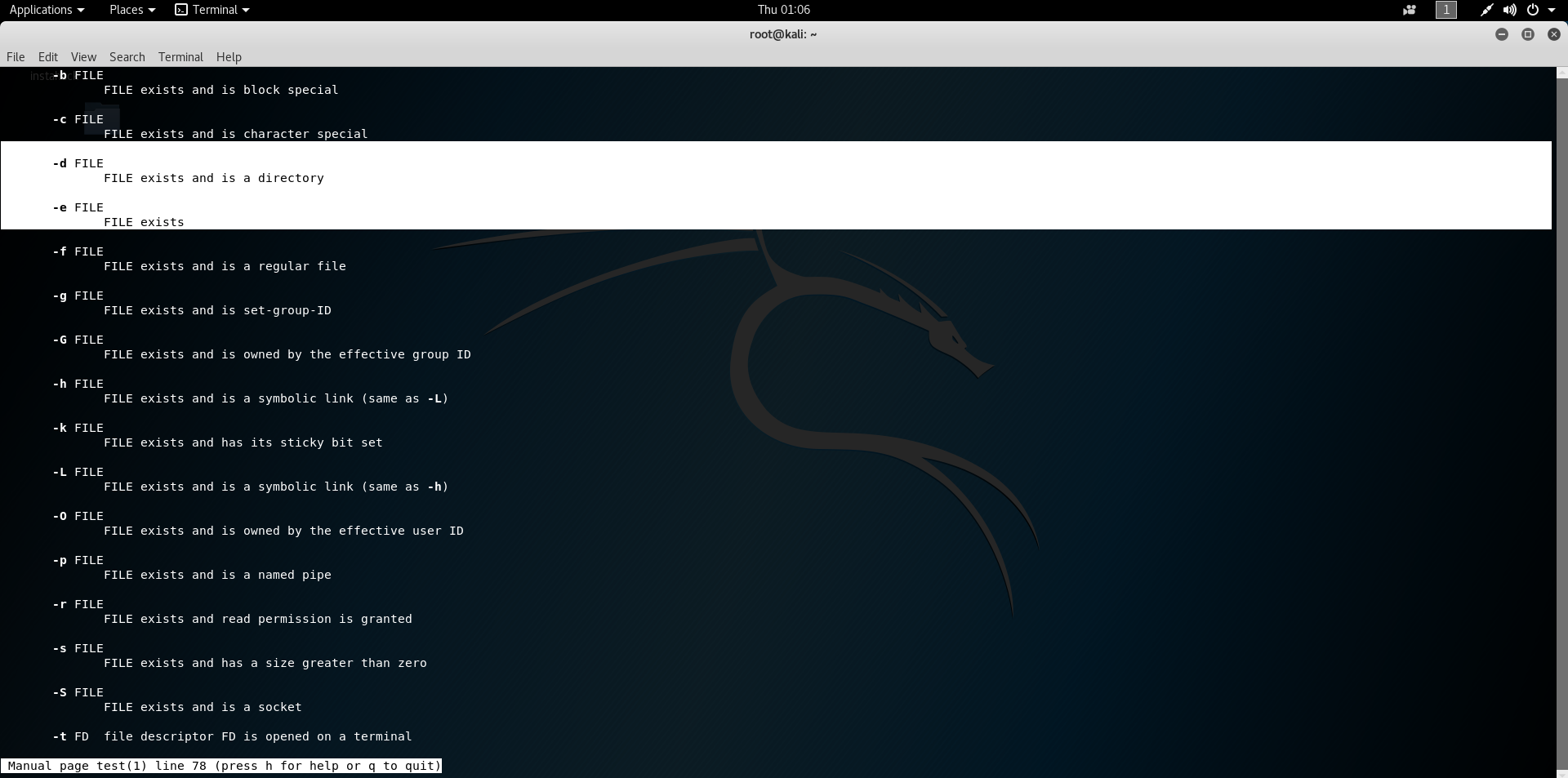

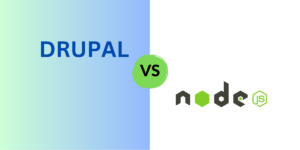
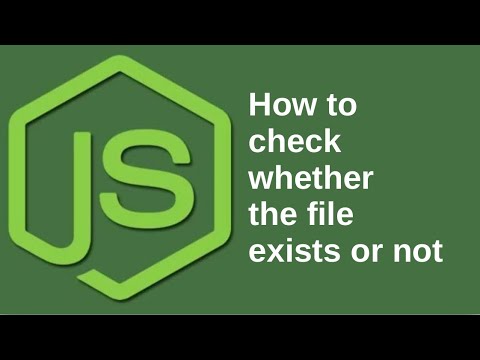
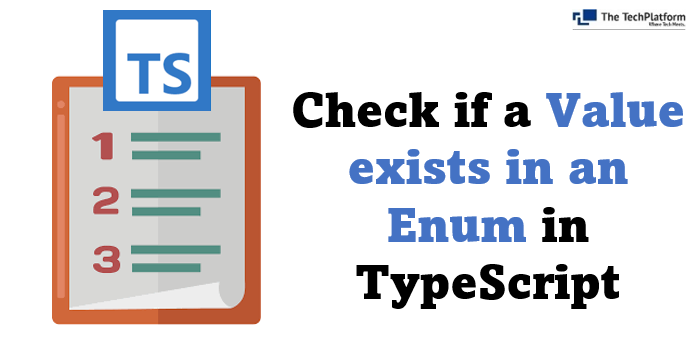
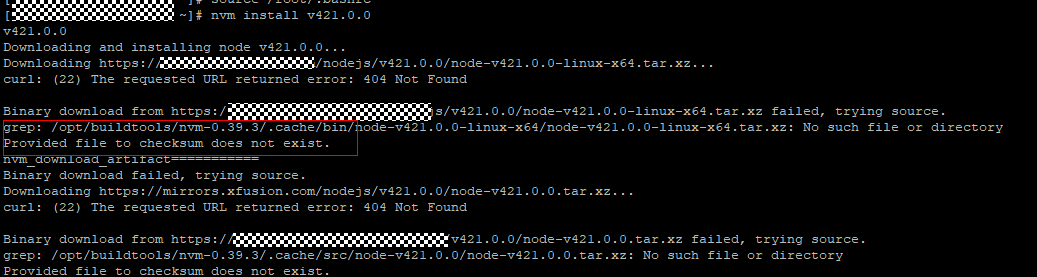
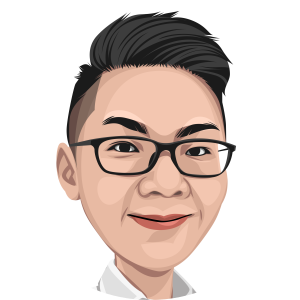
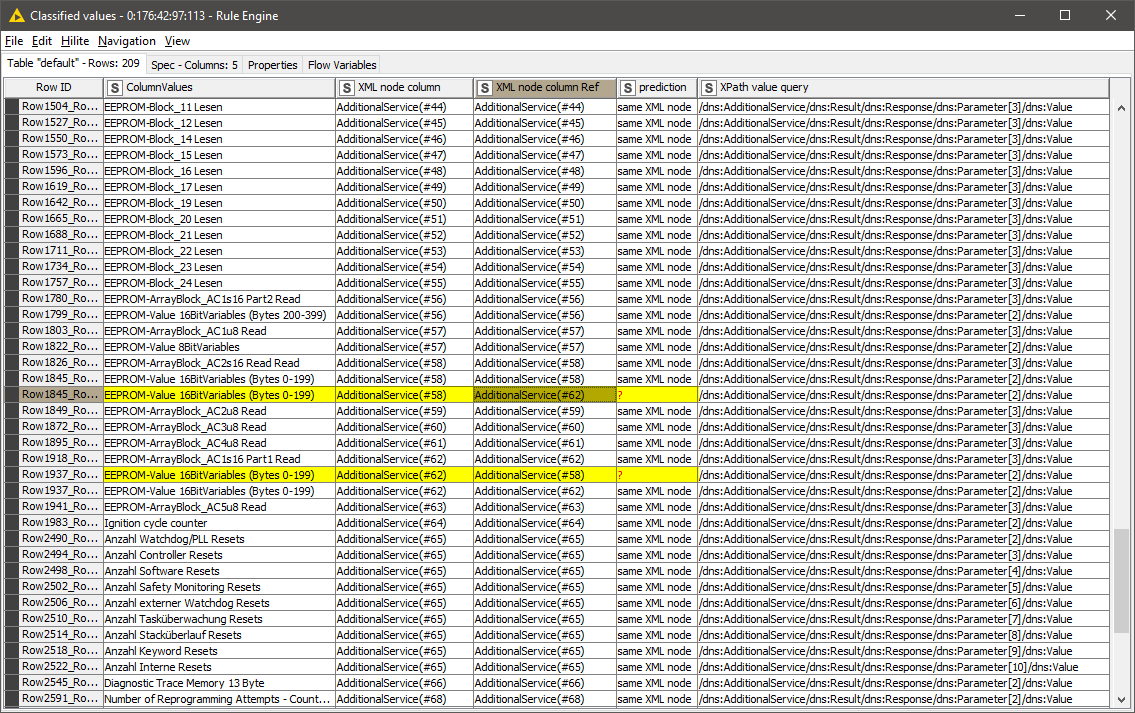
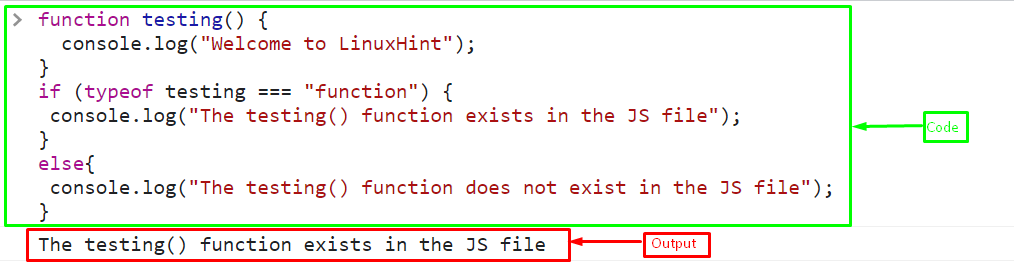
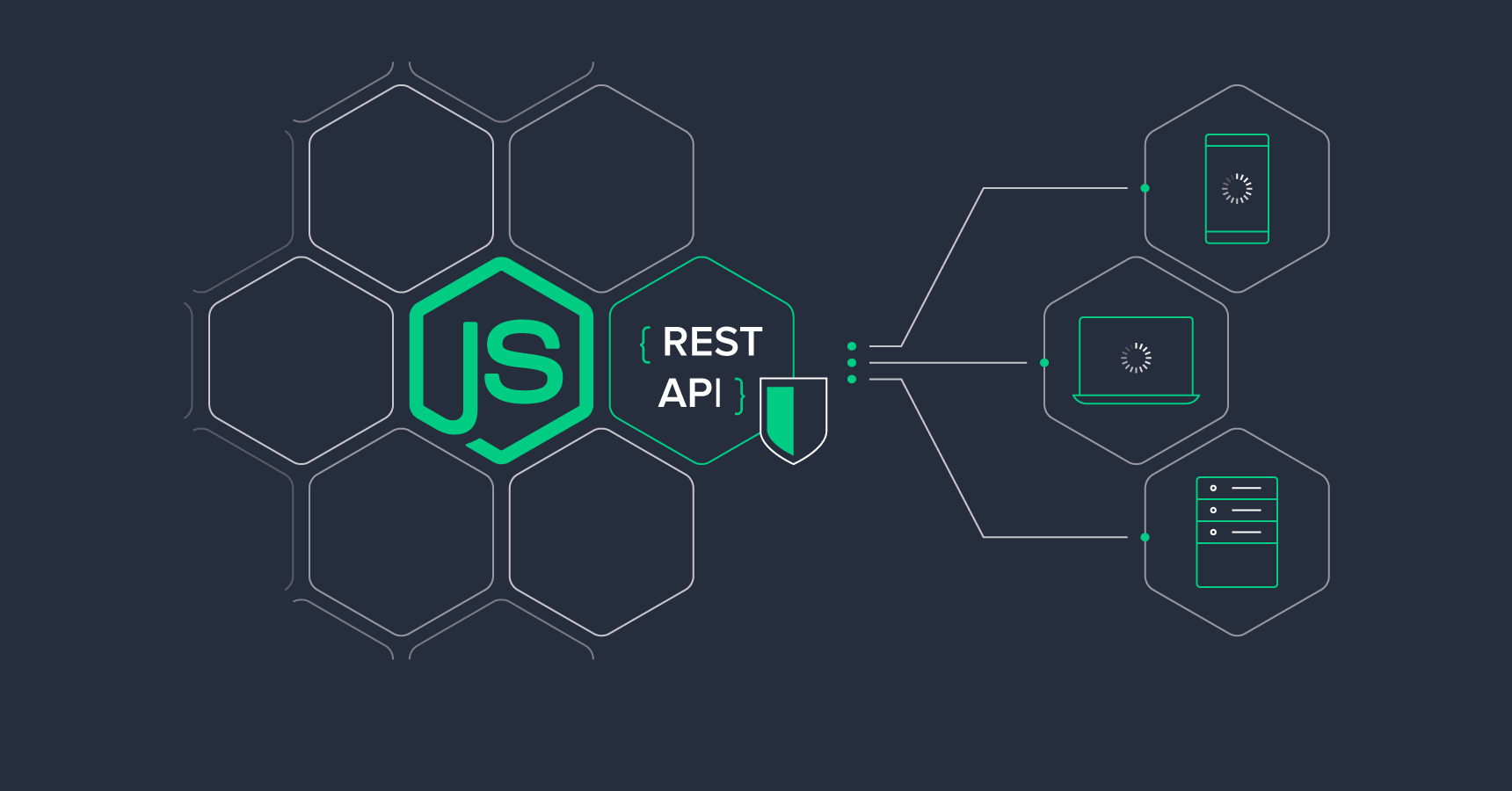
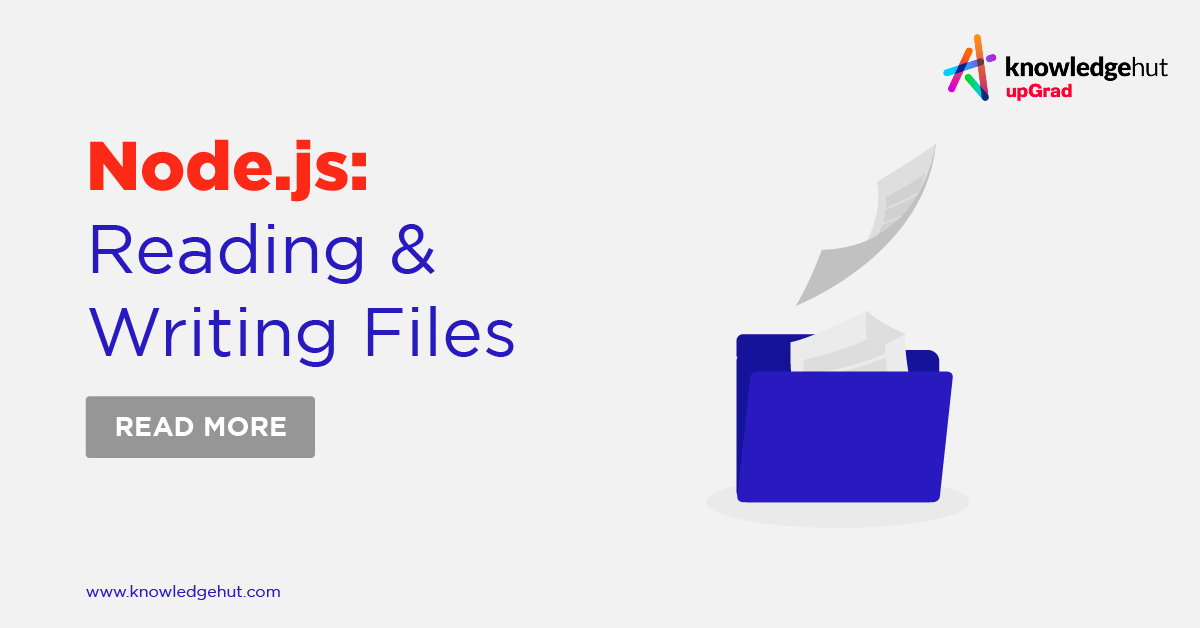
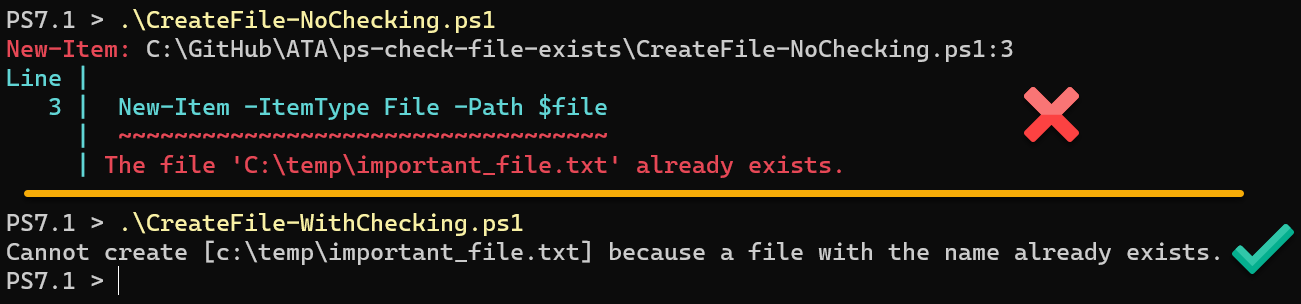
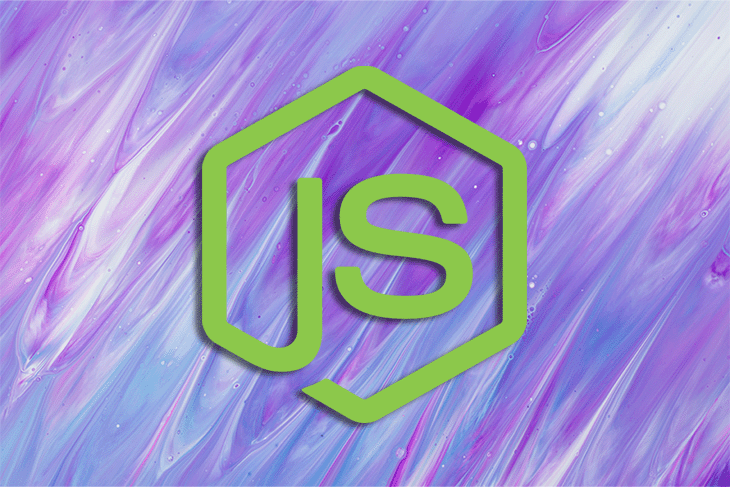
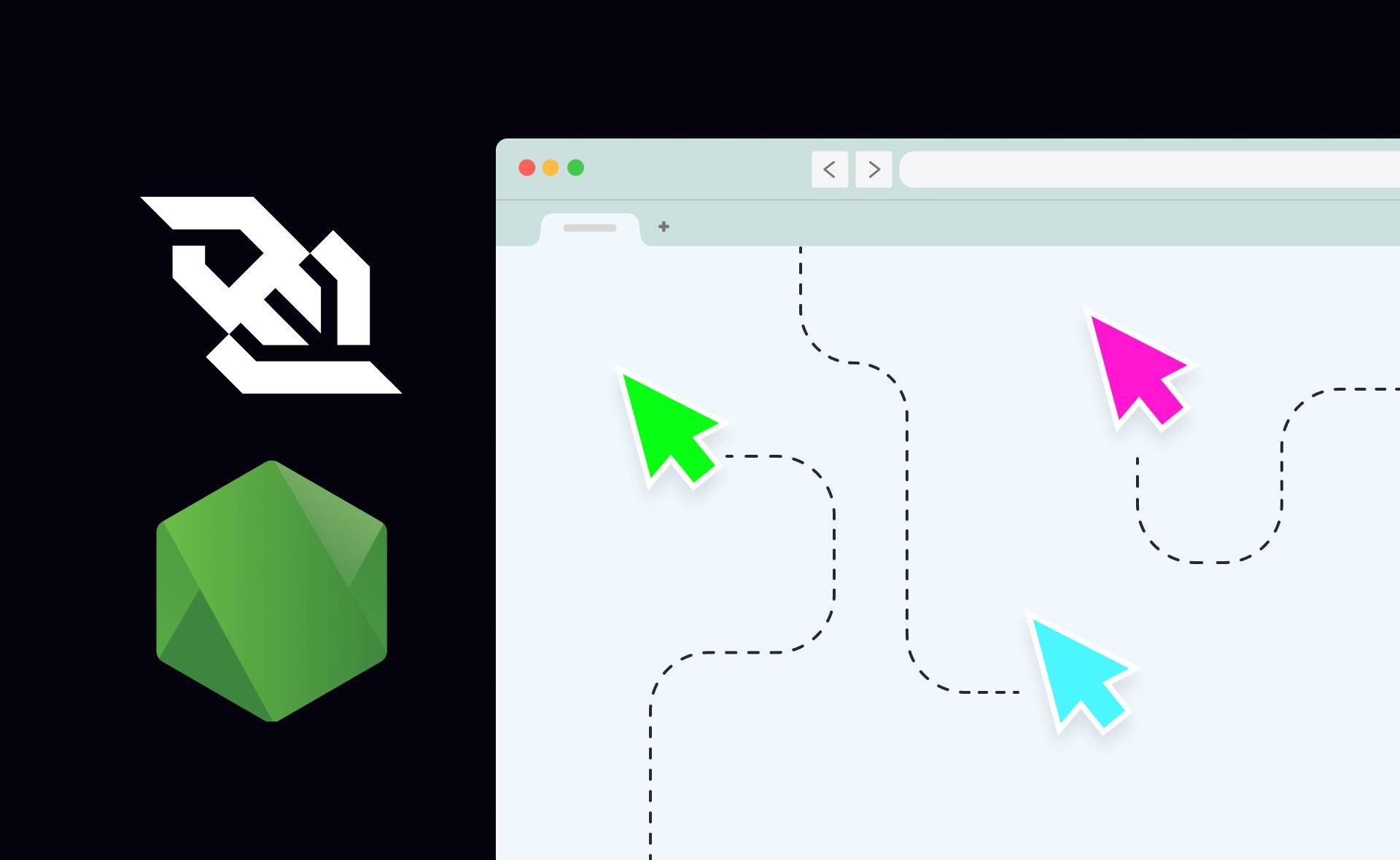
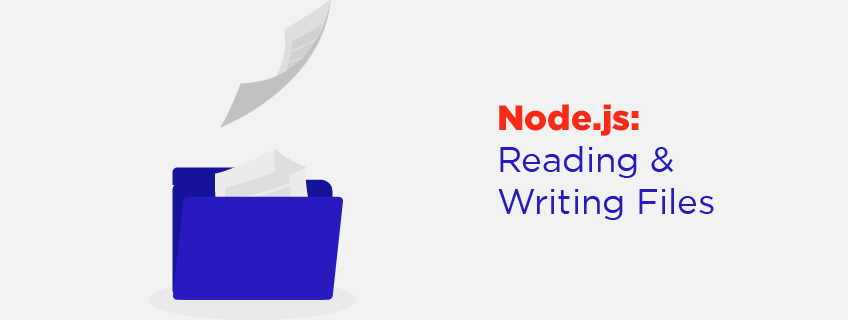
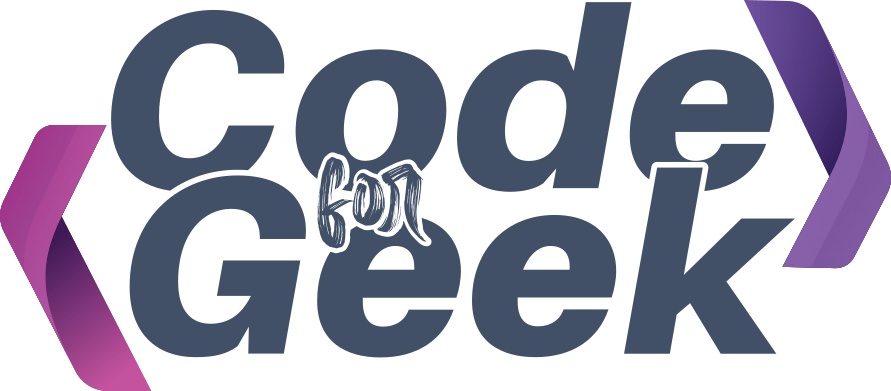
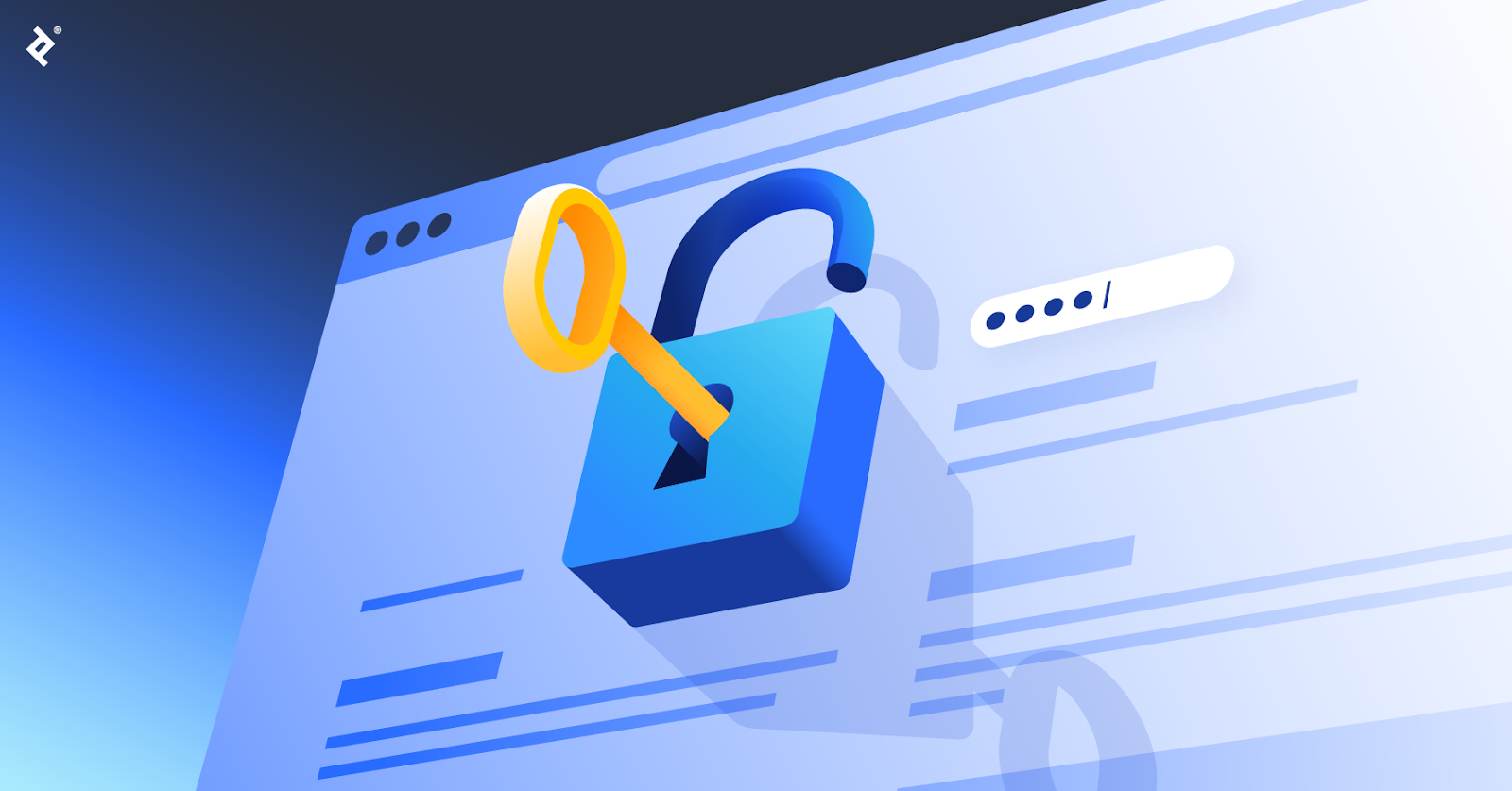
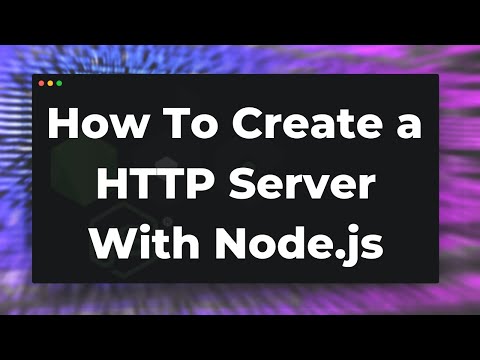
Article link: node check if file exists.
Learn more about the topic node check if file exists.
- How to check if a file exists in Node.js – Flavio Copes
- Node.js check if file exists – fs – Stack Overflow
- How To Check If A File Exists In NodeJS
- Node.js — Check If a Path or File Exists – Future Studio
- How to check if a file exists in Node.js? – DEV Community
- NodeJS – How to check if a file exists tutorial – sebhastian
- How To Check If A File Exists In NodeJS
- File.exists() – Pure JavaScript [Book] – O’Reilly
- Working with folders in Node.js – NodeJS Dev
- Node: Check if File or Directory is Empty – Stack Abuse
- How to check if file exists in Node.js – byby.dev
- How to Check If a File Exists in Node.js – CodeForGeek
- How to Check If a File Exists in Node.js (Best Methods) | WM
- How to Check if File Exists in NodeJS – Fedingo
See more: https://nhanvietluanvan.com/luat-hoc/