Non-Default Argument Follows Default Argument
1. Understanding default arguments in programming
In programming, default arguments allow us to define values for parameters in a function, which can be used if the caller does not provide a specific argument when calling the function. This helps simplify the code and make it more flexible by providing default values for certain parameters.
2. The role of non-default arguments
On the other hand, non-default arguments are those that require a specific value to be passed by the caller. They cannot rely on default values and are necessary for the proper execution of the function. Non-default arguments are essential when the function needs specific input to produce the expected output.
3. Limitations and restrictions of default arguments
Although default arguments are widely used and helpful in many cases, they come with limitations and restrictions. One significant limitation is that default arguments can only be specified at the end of the list of arguments. This means that if a function has multiple arguments, all the ones following the default argument must also be default arguments.
4. The potential issues caused by non-default arguments following default arguments
The main issue that arises when a non-default argument follows a default argument is that it can lead to ambiguity or unexpected behavior. When calling the function, the caller might not provide a value for the non-default argument, assuming it will take its default value. However, instead of considering the default value, the argument will be assigned to the next argument in the list, resulting in incorrect behavior.
5. Common scenarios where non-default arguments follow default arguments
There are several common scenarios where non-default arguments follow default arguments. One example is when a function has optional parameters that can be omitted by the caller, but the required parameters should always be provided. Another example is when a function has a mixture of optional and mandatory parameters, and the optional ones are placed before the mandatory ones.
6. Best practices for dealing with non-default arguments following default arguments
To avoid potential issues caused by non-default arguments following default arguments, it is important to follow certain best practices. Initially, it is recommended to avoid such scenarios whenever possible. Design the function in a way that all non-default arguments come before any default arguments. By doing so, you ensure that the required input is always provided, and the function behaves as intended.
7. Advanced techniques to avoid or handle issues with non-default arguments following default arguments
If it is not possible to avoid non-default arguments following default arguments, there are some advanced techniques that can be used to address potential issues. One popular technique is to use keyword arguments when calling the function. By explicitly specifying the argument names and their values, you can overcome the positional ambiguity and ensure that each argument is assigned correctly.
Additionally, it is essential to thoroughly test the function with different input scenarios to identify any unexpected behavior caused by the non-default arguments following default arguments. By detecting and addressing these issues early on, you can ensure that the function operates correctly and avoids any unexpected errors.
FAQs:
Q: Can I have multiple default arguments in a function?
A: Yes, you can have multiple default arguments in a function. However, all the default arguments must be specified at the end of the argument list, as non-default arguments cannot follow default arguments.
Q: What happens if I don’t provide a value for a non-default argument?
A: If you don’t provide a value for a non-default argument, and it follows a default argument, the argument will be assigned to the next argument in the list. This can lead to unexpected behavior and should be avoided.
Q: How can I handle non-default arguments following default arguments in Python?
A: To handle non-default arguments following default arguments in Python, it is recommended to either rearrange the arguments or use keyword arguments when calling the function. Rearranging the arguments ensures that non-default arguments come before default arguments, while keyword arguments explicitly specify the names and values of the arguments, avoiding positional ambiguity.
Q: Are there any other programming languages that allow non-default arguments to follow default arguments?
A: The limitation of non-default arguments following default arguments is not specific to any programming language. It is a convention followed by many programming languages to avoid ambiguity and unexpected behavior.
Python : Syntaxerror: Non-Default Argument Follows Default Argument
What Does Non-Default Argument Follows Default Argument Mean In Python?
In Python, a common error that developers encounter is the “non-default argument follows default argument” error message. This error occurs when defining a function with both default and non-default arguments, and the function call is ambiguous due to the order in which the arguments are provided. Understanding the cause of this error and how to resolve it is crucial for Python programmers.
To understand this error, let’s first look at function arguments in Python. Arguments in Python functions can be divided into two types: positional arguments and keyword arguments. Positional arguments are defined in the function signature without specifying any default values, while keyword arguments have default values assigned to them.
When defining a function, the arguments with default values are known as default arguments, whereas the arguments without default values are known as non-default arguments. The order in which arguments are defined in the function signature determines their position.
Here’s an example illustrating this concept:
“`python
def greet(name, message=”Hello”):
print(message, name)
greet(“John”)
“`
In this example, the `greet` function has two arguments: `name` and `message`. The `message` argument is a default argument as it has a default value of “Hello”. If we call the `greet` function without providing a value for `message`, it will use the default value.
The output of the above code will be:
“`
Hello John
“`
Now, let’s explore the error that can occur when defining functions with both default and non-default arguments:
“`python
def greet(message=”Hello”, name):
print(message, name)
greet(“John”)
“`
Executing this code will result in a `SyntaxError` with the message: “non-default argument follows default argument”. This error occurs because when a default argument is used, all the arguments to its right must also have default values. In the above code, the argument `name` does not have a default value, and it comes after the default argument `message`.
To resolve this error, we have two options. The first option is to reorder the arguments so that the default argument appears after the non-default argument:
“`python
def greet(name, message=”Hello”):
print(message, name)
greet(“John”)
“`
By rearranging the arguments, we ensure that the default argument `message` comes after the non-default argument `name`. This resolves the “non-default argument follows default argument” error, and the code will execute without any issues.
The second option is to explicitly provide the keyword argument when calling the function. By specifying the argument using its keyword, we can bypass the order restriction:
“`python
def greet(message=”Hello”, name):
print(message, name)
greet(name=”John”)
“`
In this example, we explicitly provide the value for the argument `name` using the keyword `name=”John”`. By doing so, we overcome the order restriction imposed by default arguments, and the code will run without any errors.
Frequently Asked Questions (FAQs):
Q: Is it mandatory to have default arguments in a function?
A: No, default arguments are not mandatory. You can have functions with only non-default arguments.
Q: Can I mix positional and keyword arguments in a function call?
A: Yes, in Python, you can mix both positional and keyword arguments while calling a function. However, when using a mix of both, remember that positional arguments must come before keyword arguments.
Q: Can I specify values for some arguments and use default values for others in a function call?
A: Yes, you can selectively provide values for certain arguments and use default values for the rest. By using keyword arguments and specifying their values, you can override the defaults for those specific arguments.
Q: Can I provide a value for a default argument in a function call without specifying the argument name?
A: Yes, you can provide values for default arguments without explicitly specifying the argument name by relying on the positional order of the arguments. However, it is considered good practice to use keyword arguments to improve code readability.
In conclusion, the “non-default argument follows default argument” error in Python occurs when defining a function with both default and non-default arguments and violating the order restriction. To resolve this error, ensure that the default arguments appear after the non-default arguments. Alternatively, you can provide values for the arguments using their keywords. Understanding this error and its resolution is essential for writing error-free Python code.
Which Cannot Have Default Arguments?
In the world of programming, default arguments provide a convenient way to assign initial values to function parameters. By specifying default values, programmers can avoid the need to provide arguments for all parameters every time a function is called. However, not all function parameters can have default arguments in programming languages. In this article, we will explore the concept of default arguments, discuss why certain parameters cannot have defaults, and answer some frequently asked questions on this topic.
Understanding Default Arguments:
Default arguments are values assigned to parameters in a function declaration. They are used when no explicit value is provided by the caller while invoking the function. This feature enhances code flexibility and allows programmers to design functions with optional parameters. For example, consider a function that calculates the area of a rectangle. The function may have two parameters representing length and width, with default values of 1.0 for both. This means that if the user invokes the function without providing any arguments, it will calculate the area of a unit square.
Parameters That Cannot Have Default Arguments:
While default arguments are a powerful feature, it is important to note that not all function parameters can have defaults. Let us explore the reasons why certain parameters are excluded from this provision:
1. Output Parameters: Parameters that serve as output variables cannot have default arguments. Output parameters are designed to return specific values to the calling function, and default values go against their intended purpose. They should always be explicitly assigned values by the caller.
2. Reference Parameters: Parameters that are passed by reference cannot have default arguments. In many programming languages, references allow direct manipulation of the original variable passed to the function. Since the original variable can be modified within the function, providing a default value may lead to confusion and unintended consequences.
3. Variadic Parameters: Variadic parameters, which are used to accept a variable number of arguments, cannot have default values. Their purpose is to handle a flexible number of inputs, making it impractical to set a default value. For example, a function that concatenates multiple strings into a single string cannot have a default delimiter, as the number of strings can vary.
4. Constructors: Constructors, which are special functions used to initialize objects of a class, cannot have default arguments for their parameters. This limitation ensures that objects are initialized correctly with explicit and defined values. Constructors play a crucial role in defining the state of objects, making it vital to eliminate any ambiguity by avoiding default arguments.
5. Pure Virtual Functions: In object-oriented programming, pure virtual functions define interfaces that must be implemented by derived classes. These functions cannot have default arguments, as the derived classes should explicitly determine the specific functionality of the function. Default arguments would undermine the purpose of pure virtual functions, making them less abstract and harming the design of the class hierarchy.
FAQs:
Q1. Can a function have multiple parameters with default arguments?
Yes, functions can have multiple parameters with default arguments. It allows flexibility as the user can choose to pass values for specific parameters while relying on default values for the remainder.
Q2. Is it possible to set default arguments in any programming language?
Most modern programming languages support default arguments. However, there are some older or low-level languages that may not have this feature. It is important to consult the documentation of the specific language being used to confirm its availability.
Q3. Can default arguments be assigned to any data type?
In general, default arguments can be assigned to any valid data type supported by the programming language. However, there might be limitations or restrictions on specific types, such as literals or complex objects.
Q4. Why are constructors not allowed to have default arguments?
Constructors are responsible for initializing objects and ensuring their valid state. By excluding default arguments, object creation is forced to be explicit and avoids any ambiguity in initialization.
Q5. Are default arguments checked at compile-time or runtime?
Default arguments are resolved at compile-time. The compiler uses the specified default values to generate appropriate function call instructions. Therefore, any errors or conflicts related to default arguments are typically detected during the compilation process.
In conclusion, default arguments are a valuable feature that simplifies programming tasks, but not all parameters can have default values. Understanding the limitations associated with default arguments helps developers write more robust and maintainable code. By considering the nature and purpose of each parameter, programmers can make informed decisions around the use of default arguments in their functions.
Keywords searched by users: non-default argument follows default argument Positional argument follows keyword argument, Default parameter Python, Got multiple values for argument
Categories: Top 30 Non-Default Argument Follows Default Argument
See more here: nhanvietluanvan.com
Positional Argument Follows Keyword Argument
In Python, argument passing plays a pivotal role in enhancing the flexibility and efficiency of code. Python functions provide two ways to pass arguments: positional arguments and keyword arguments. While positional arguments are the traditional and most widely used method, keyword arguments offer more control and clarity. However, there may be cases where you encounter an error message stating “Positional argument follows keyword argument.” This article will delve into this error, explain its cause, and provide strategies to resolve it.
Understanding Positional and Keyword Arguments
Before we delve into the issue of “Positional argument follows keyword argument,” let’s take a moment to understand positional and keyword arguments.
Positional arguments are arguments passed to a function based on their position, in the same order as they appear in the function signature. This is the classical way of passing arguments, where their value is assigned to respective parameters based on their order.
On the other hand, keyword arguments are passed with the parameter name followed by a colon and the argument value. This allows for flexibility in argument position, as long as the parameter name is specified. Using keyword arguments is highly recommended when there are a large number of arguments or when defaults are given to some parameters.
The Error: “Positional argument follows keyword argument”
When you encounter the error “Positional argument follows keyword argument,” it means that you have passed a positional argument to a function after a keyword argument. Let’s consider an example to understand this error better:
“`Python
def divide_numbers(a, b, precision=2):
result = a / b
return round(result, precision)
divide_numbers(10, precision=3, b=2)
“`
In this example, the function `divide_numbers` calculates the result of dividing `a` by `b` up to a specified precision. When we call this function, we pass the values of `a` and `precision` as keyword arguments, indicating their respective parameter names. However, we mistakenly placed the positional argument `b` after the keyword argument `precision`. As a result, we encounter the error “Positional argument follows keyword argument.”
The Cause of the Error and How to Resolve It
To understand why this error occurs, we need to consider how Python interprets both positional and keyword arguments. Python processes positional arguments first, followed by keyword arguments. Thus, if a positional argument is encountered after a keyword argument, it violates this logical sequence, leading to the error.
To resolve this error, you have two options:
1. Reorder the arguments: The simplest way to resolve the error is to reorder the arguments so that positional arguments are passed before keyword arguments. In the previous example, we can correct the code as follows:
“`Python
divide_numbers(10, b=2, precision=3)
“`
2. Pass all arguments as keyword arguments: Another option is to make all arguments keyword arguments. This ensures that the order of arguments does not matter. By rewriting the function call as:
“`Python
divide_numbers(a=10, b=2, precision=3)
“`
With these strategies, you can prevent the error “Positional argument follows keyword argument” and ensure the proper execution of your code.
FAQs
Q1. What does “Positional argument follows keyword argument” mean in Python?
The error message “Positional argument follows keyword argument” indicates that you have passed a positional argument to a function after a keyword argument. Since Python processes positional arguments first, followed by keyword arguments, this violates the logical sequence, resulting in the error message.
Q2. How can I fix the “Positional argument follows keyword argument” error?
To resolve this error, you can either reorder the arguments so that positional arguments precede keyword arguments or pass all arguments as keyword arguments. These simple adjustments will ensure that the error is resolved, and your code executes as expected.
Q3. Why should I use keyword arguments instead of positional arguments?
Keyword arguments offer several advantages over positional arguments. They enhance code clarity, as the use of parameter names makes the code more self-explanatory. Moreover, keyword arguments provide flexibility by allowing arguments to be passed in any order as long as the parameter names are specified. This becomes particularly useful when dealing with functions that have numerous arguments or have significant default values.
Q4. Are there any cases where the order of positional and keyword arguments does not matter?
Yes, when all arguments are passed as keyword arguments, the order in which they are specified becomes irrelevant. By consistently using keyword arguments, you can ensure that the sequence of arguments does not affect the execution of your code.
In conclusion, the error “Positional argument follows keyword argument” indicates that you have passed a positional argument after a keyword argument, violating Python’s logical sequence of argument processing. By understanding the cause of this error and applying simple strategies to fix it, you can enhance the efficiency and clarity of your code. Embracing keyword arguments offers greater flexibility and control, making your code more maintainable in the long run.
Default Parameter Python
In Python, default parameters are a powerful feature that allows you to assign a default value to a function parameter if no value is provided during function call. This flexibility simplifies the code and makes it more readable, reducing the need for lengthy if-else statements to handle various scenarios. Understanding and using default parameters effectively can greatly enhance your coding abilities and improve the efficiency of your programs.
Defining Default Parameters
To define a default parameter in Python, you simply assign a value to the parameter in the function’s definition. Consider the following example:
“`python
def greet(name, message=”Hello!”):
print(f”{message} {name}!”)
“`
In this code snippet, the `message` parameter is assigned a default value of “Hello!” using the assignment operator (=). The `greet` function takes two parameters, `name` and `message`. If the `message` argument is not provided during the function call, it will default to “Hello!”.
Using Default Parameters
When you call a function with default parameters, you have the option to pass values for any or all parameters. If you only provide specific arguments, the default values will be used for the rest. Let’s see how this works with the `greet` function:
“`python
greet(“John”) # Output: “Hello John!”
greet(“Emma”, “Hi!”) # Output: “Hi Emma!”
“`
In the first function call, only the `name` argument is provided, so the default value of `message` is used. In the second call, both `name` and `message` arguments are provided, so the default value is overridden.
Overriding Default Parameters
Default parameters can be overridden simply by providing a value during function call. This allows you to customize your function’s behavior without modifying the original function definition. Consider the following example:
“`python
def multiply(a, b=2):
return a * b
print(multiply(3)) # Output: 6
print(multiply(3, 4)) # Output: 12
“`
In this case, the `multiply` function multiplies two numbers, `a` and `b`. If `b` is not provided during the function call, it defaults to 2. The first call multiplies 3 by the default value 2, giving an output of 6. The second call overrides the default value by passing 4 as the second argument, resulting in an output of 12.
Default Parameter Evaluation
One important thing to note when using default parameters is that the expression used as the default value is only evaluated once when the function is defined. This means that the default value is retained across multiple function calls. Consider the following example:
“`python
def increment_list(value, lst=[]):
lst.append(value)
return lst
print(increment_list(10)) # Output: [10]
print(increment_list(20)) # Output: [10, 20]
“`
In this code snippet, the `increment_list` function appends a value to a list. If `lst` is not provided during the function call, it defaults to an empty list. However, since the default value is evaluated once and retained across function calls, subsequent calls to the function will append values to the same list instead of creating a new list. To avoid this behavior, it is recommended to use immutable default values, such as `None`, and handle the default value inside the function using conditional statements.
FAQs
Q1. Can I use any type of value as a default parameter?
Yes, you can use any valid Python value as a default parameter, including numbers, strings, lists, dictionaries, and even objects.
Q2. Can I modify the default value within the function?
Modifying the default value within the function can lead to unexpected results. It is generally recommended to avoid modifying default values directly, as it might affect future function calls.
Q3. Can I specify default values for some parameters and not others?
Yes, you can assign default values to any number of parameters in a function. Parameters without default values are required during function calls, while those with default values are optional.
Q4. Can I use variables as default parameter values?
Yes, you can use variables as default parameter values, as long as those variables are defined before the function is called.
Q5. Can I change the order of default parameters during function call?
No, you cannot change the order of default parameters during function calls. The arguments must be provided in the same order as they are defined in the function’s parameter list.
In conclusion, default parameters in Python provide a powerful way to simplify function calls and handle various scenarios without the need for extensive if-else statements. By understanding how to define, use, and override default parameters, you can enhance the readability and efficiency of your Python code. Remember to be cautious when modifying default values and consider using immutable default values to avoid unwanted behavior.
Got Multiple Values For Argument
The “Got multiple values for argument” error message appears when a function or method is called with more arguments than it expects. The error message typically shows the name of the argument and the number of values provided. For example, if we have a function called “multiply” that accepts two arguments, we might encounter this error if we try to call it with three arguments: multiply(2, 3, 4).
So, why does this error occur? Well, Python functions and methods are designed to accept a specific number of arguments. When you call a function with too many arguments, Python sees this as a mismatch between the function’s definition and the way it is being used. Python raises an error to alert you to this mismatch so that you can correct it.
Let’s look at an example to understand this error better:
“`python
def add_numbers(a, b):
return a + b
result = add_numbers(2, 3, 4)
print(result)
“`
In this code snippet, the `add_numbers` function is defined to accept two arguments, `a` and `b`. However, when we try to call this function with three arguments, Python raises the “Got multiple values for argument” error. This error message indicates that we provided too many values for the function arguments.
To fix this error, we need to ensure that we call the function with the correct number of arguments. In the above example, we can fix the error by simply removing the extra argument: `result = add_numbers(2, 3)`.
Sometimes, this error can also occur due to incorrect usage of keyword arguments. Keyword arguments allow us to specify the argument values by their names instead of their positions. If you pass multiple values for a keyword argument, Python raises this error as well. Let’s take a look at an example:
“`python
def get_message(name, message):
return f”Hello {name}, {message}”
result = get_message(name=”John”, message=”Welcome!”, name=”Jane”)
print(result)
“`
In this code, we are calling the `get_message` function with the keyword argument `name` provided twice. Python raises the “Got multiple values for argument” error, indicating that the `name` argument received multiple values. To fix this error, we should only provide the argument once with the desired value: `result = get_message(name=”Jane”, message=”Welcome!”)`.
Now, let’s address some frequently asked questions about the “Got multiple values for argument” error:
**Q: Can this error occur with built-in Python functions?**
A: Yes, this error can occur with built-in Python functions as well. While the specific error message may vary, the root cause is the same: providing more arguments than expected.
**Q: How can I avoid this error in my code?**
A: To avoid this error, carefully review the function’s documentation to understand the expected number and order of arguments. Make sure to provide the correct number of arguments while calling the function.
**Q: Can this error occur with default arguments?**
A: No, this error will not occur with default arguments. If a function has default values for some of its arguments, you can omit them while calling the function. Python will assign the default values to those arguments automatically.
**Q: What if I need to pass a variable number of arguments to a function?**
A: Python provides a mechanism for handling variable-length argument lists in functions. By using the `*args` or `**kwargs` notation, you can pass a variable number of arguments to a function. This way, you can avoid the “Got multiple values for argument” error when the number of arguments may vary.
In conclusion, the “Got multiple values for argument” error in Python occurs when a function or method is called with more arguments than it expects. This error message is an indication of a mismatch between the function’s definition and its usage. By carefully reviewing the function’s documentation and providing the correct number of arguments, you can avoid this error in your code. Additionally, understanding how to handle variable-length argument lists can help you overcome this error when working with functions that expect a varying number of arguments.
Images related to the topic non-default argument follows default argument
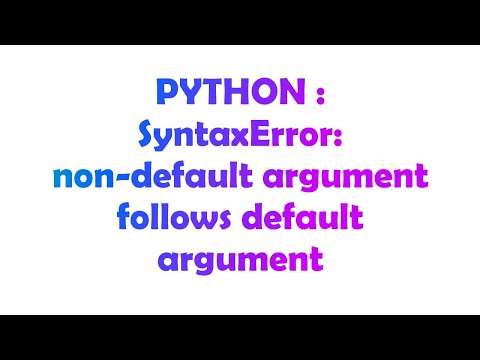
Found 44 images related to non-default argument follows default argument theme
![Solved] SyntaxError: non-default argument follows default argument - Clay-Technology World Solved] Syntaxerror: Non-Default Argument Follows Default Argument - Clay-Technology World](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2020/06/image-40.png?ssl=1)

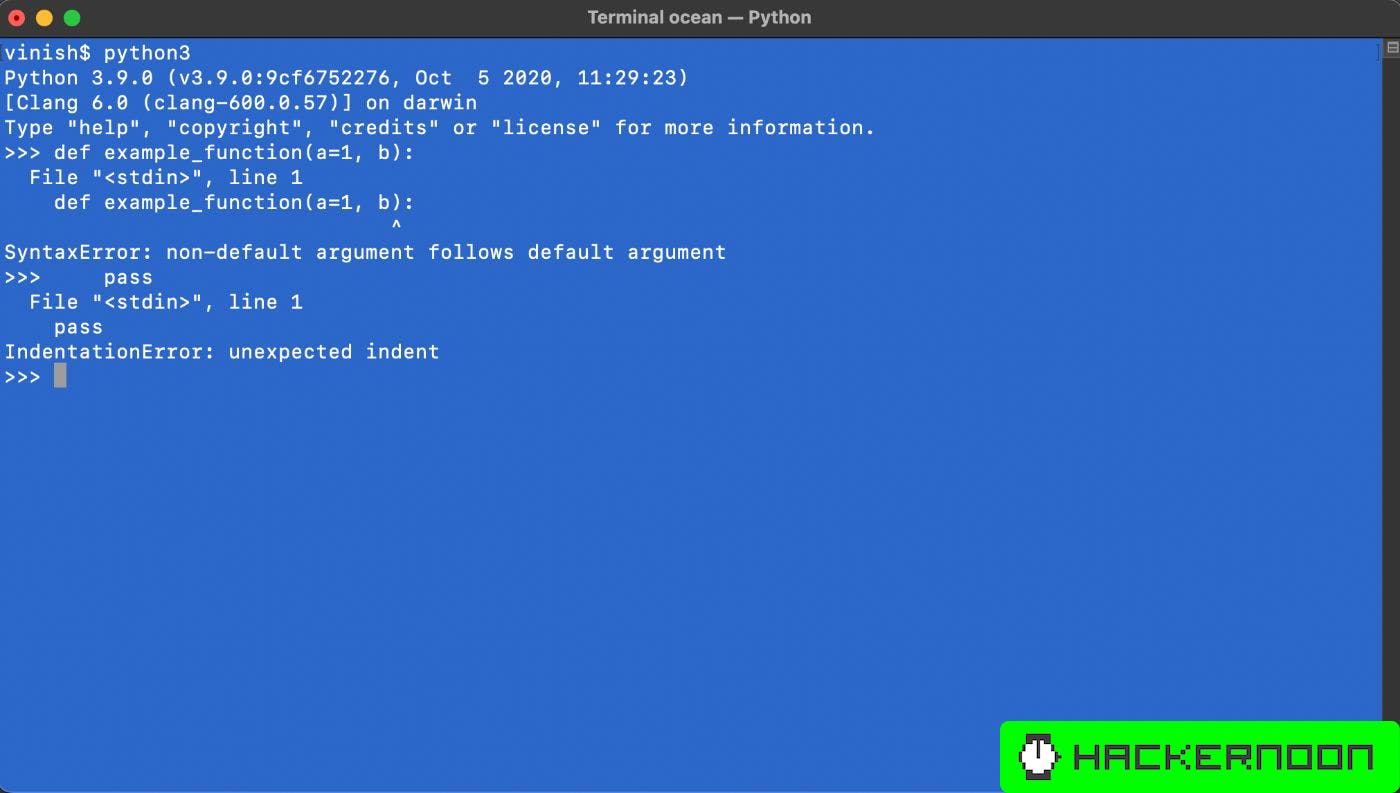

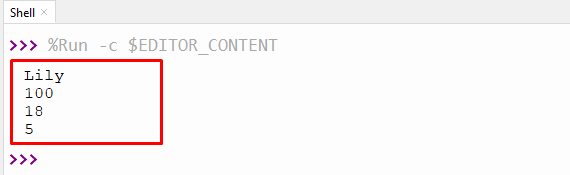
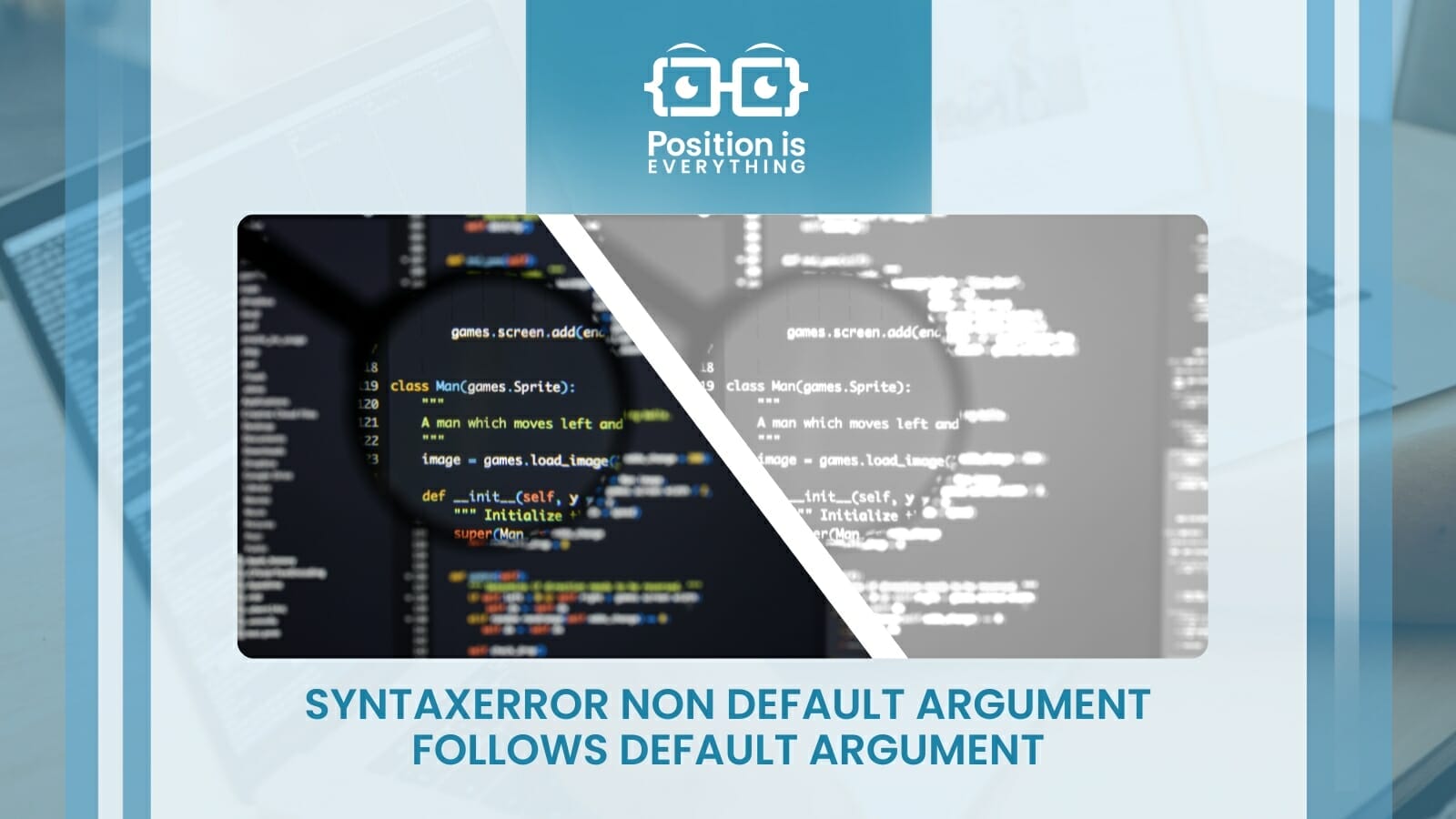
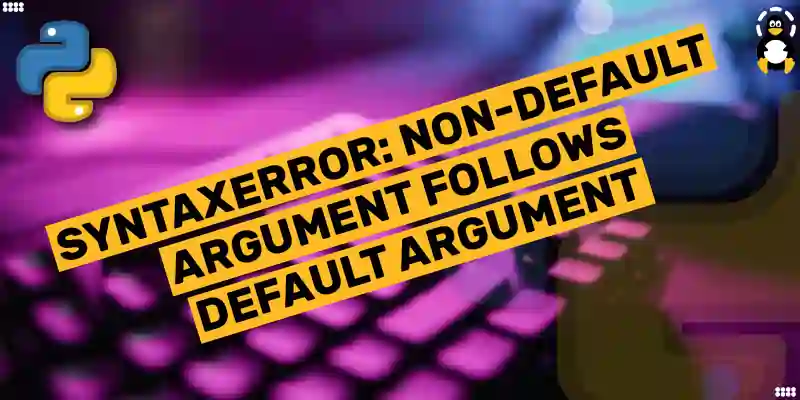
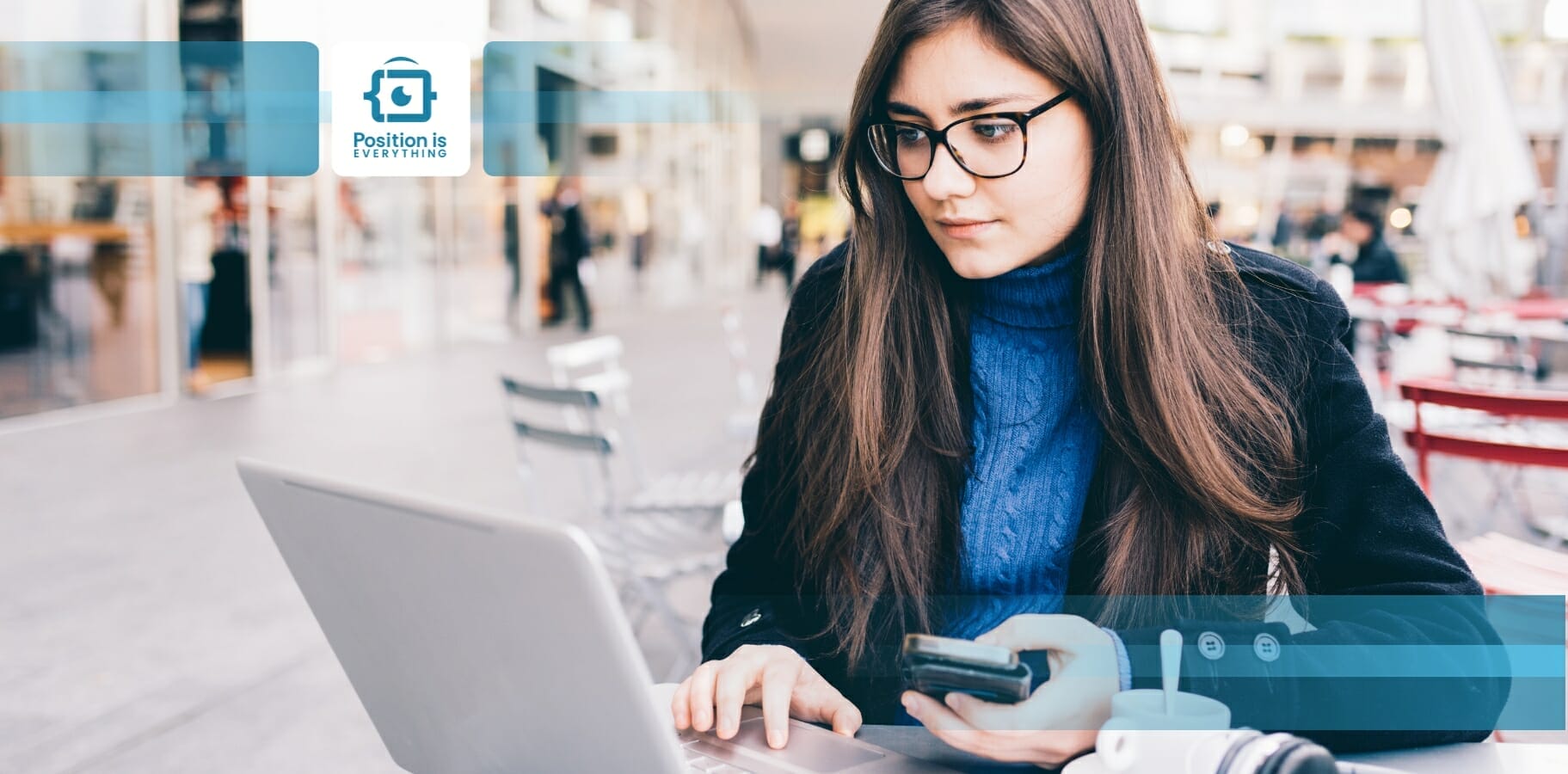
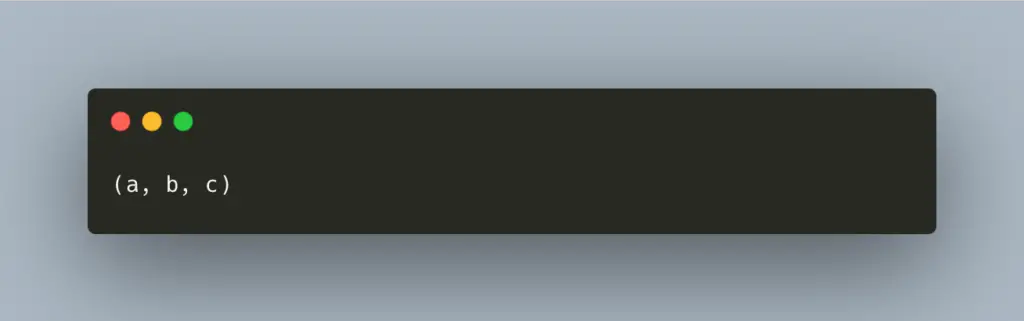
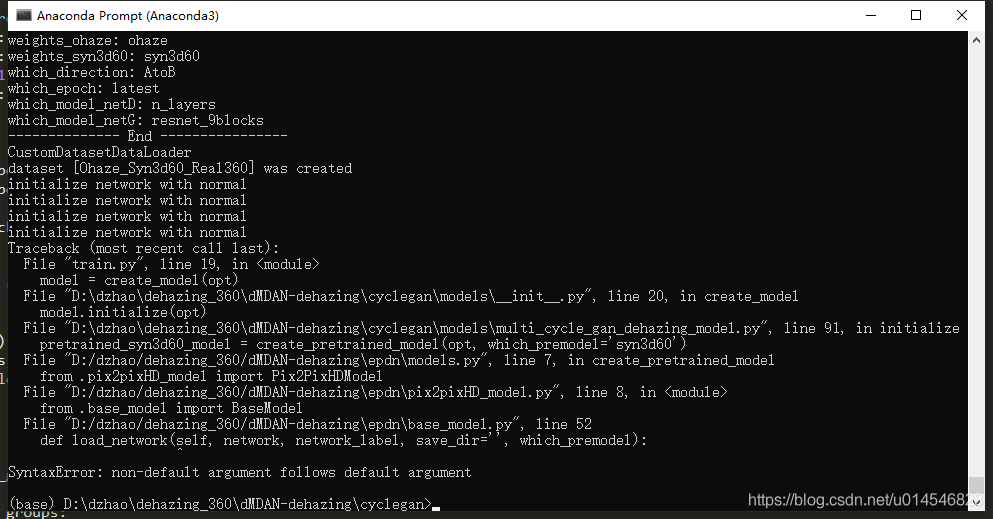

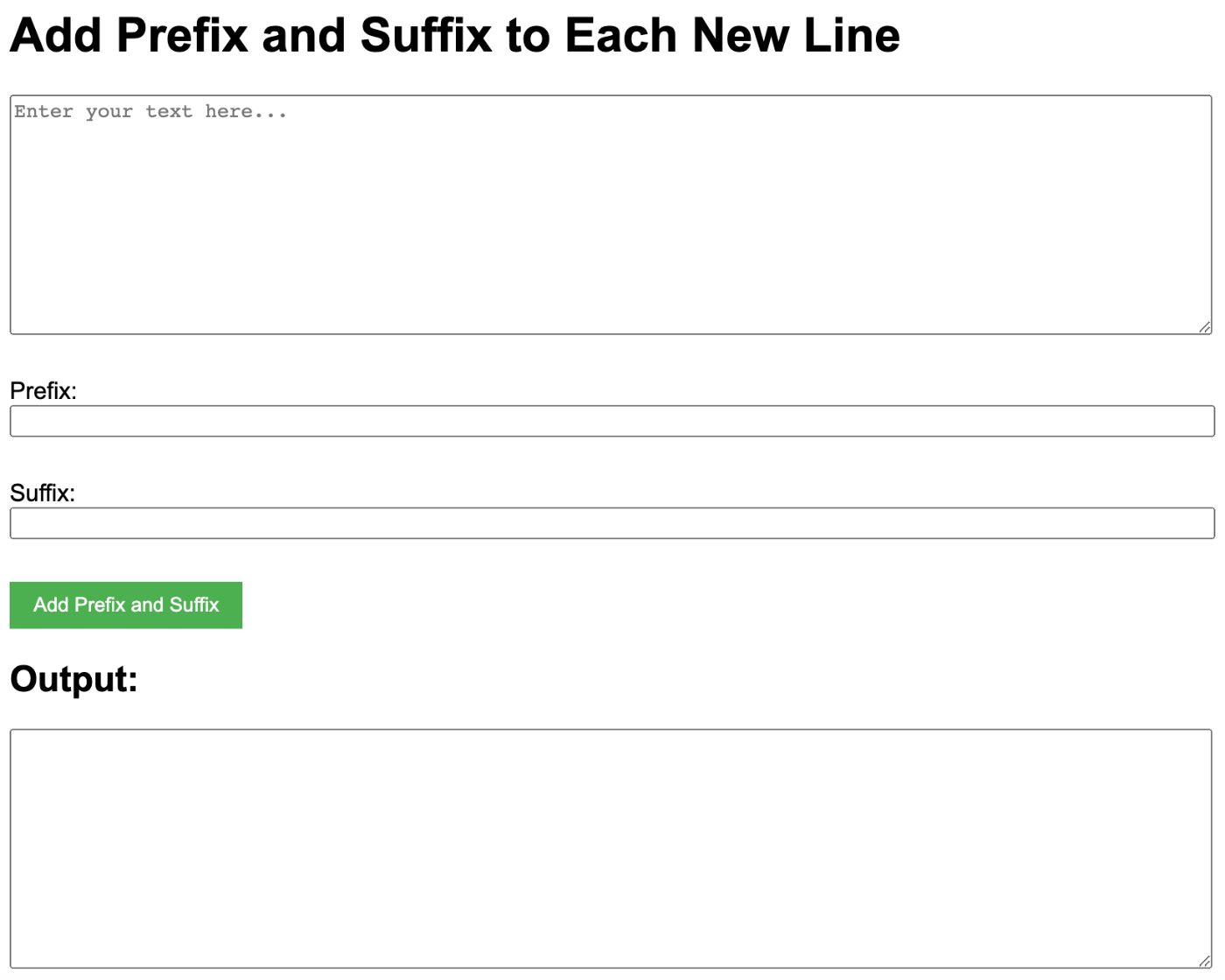
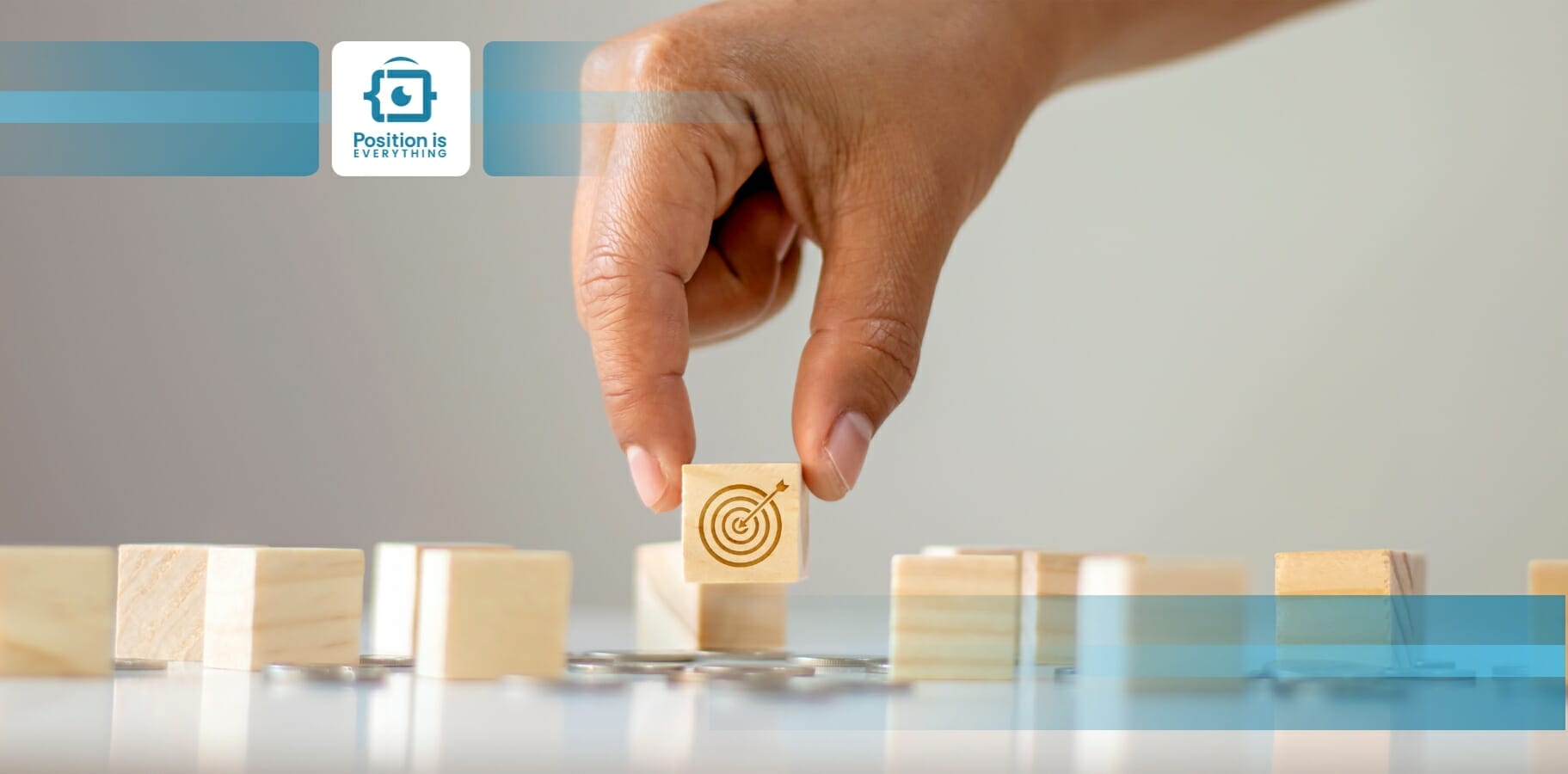
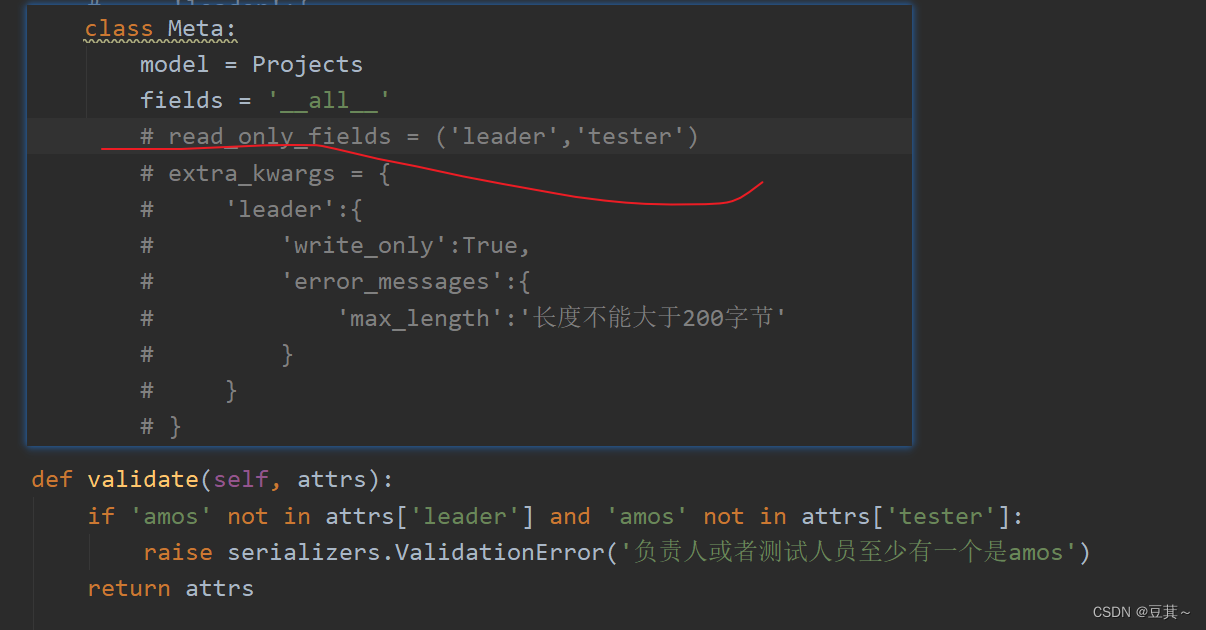
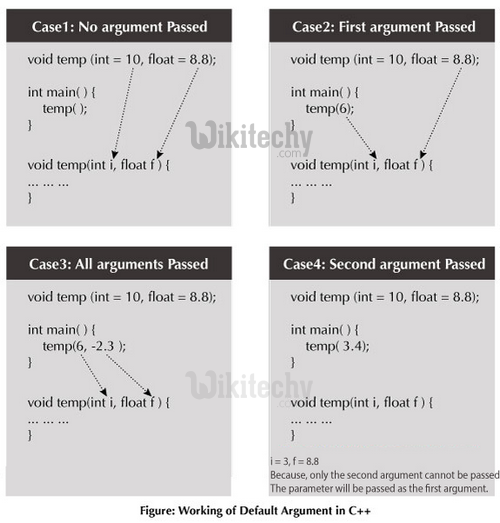
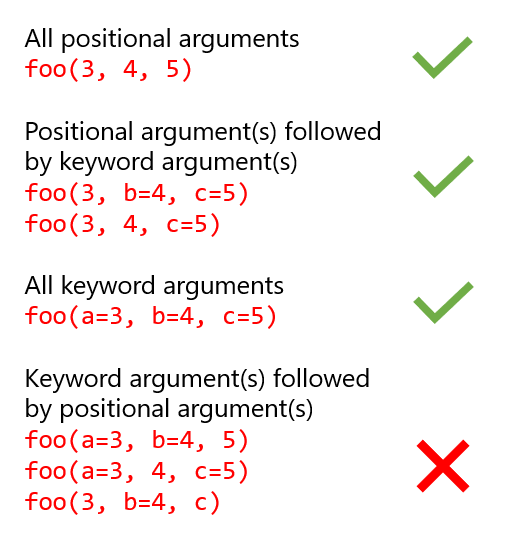
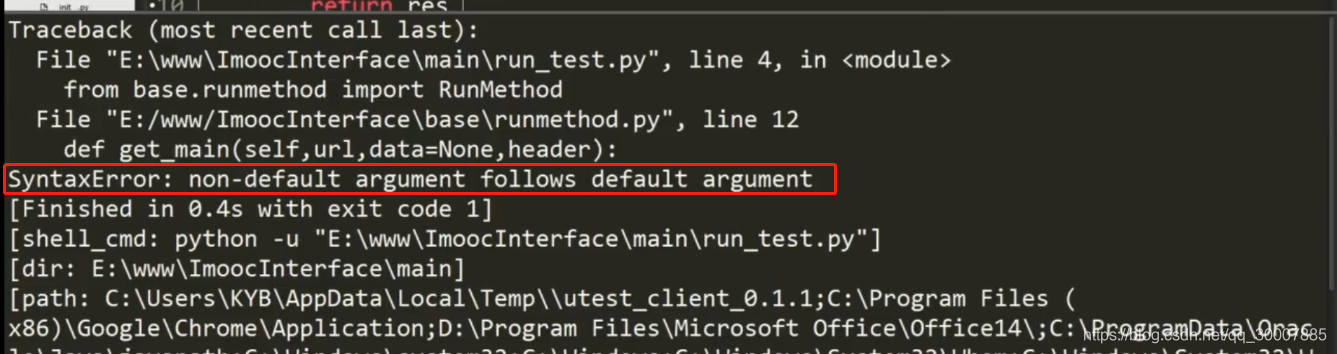

![Python Function Arguments [4 Types] – PYnative Python Function Arguments [4 Types] – Pynative](https://pynative.com/wp-content/uploads/2022/08/python_function_arguments_types.jpg)
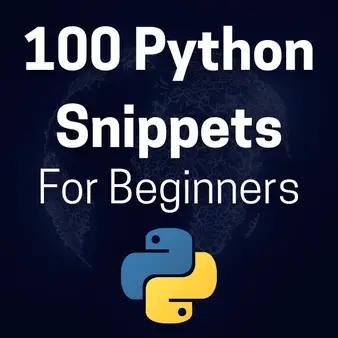
![Solved] SyntaxError: non-default argument follows default argument - Clay-Technology World Solved] Syntaxerror: Non-Default Argument Follows Default Argument - Clay-Technology World](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2021/03/cropped-output.png?fit=350%2C350&ssl=1)
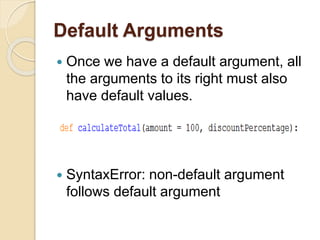


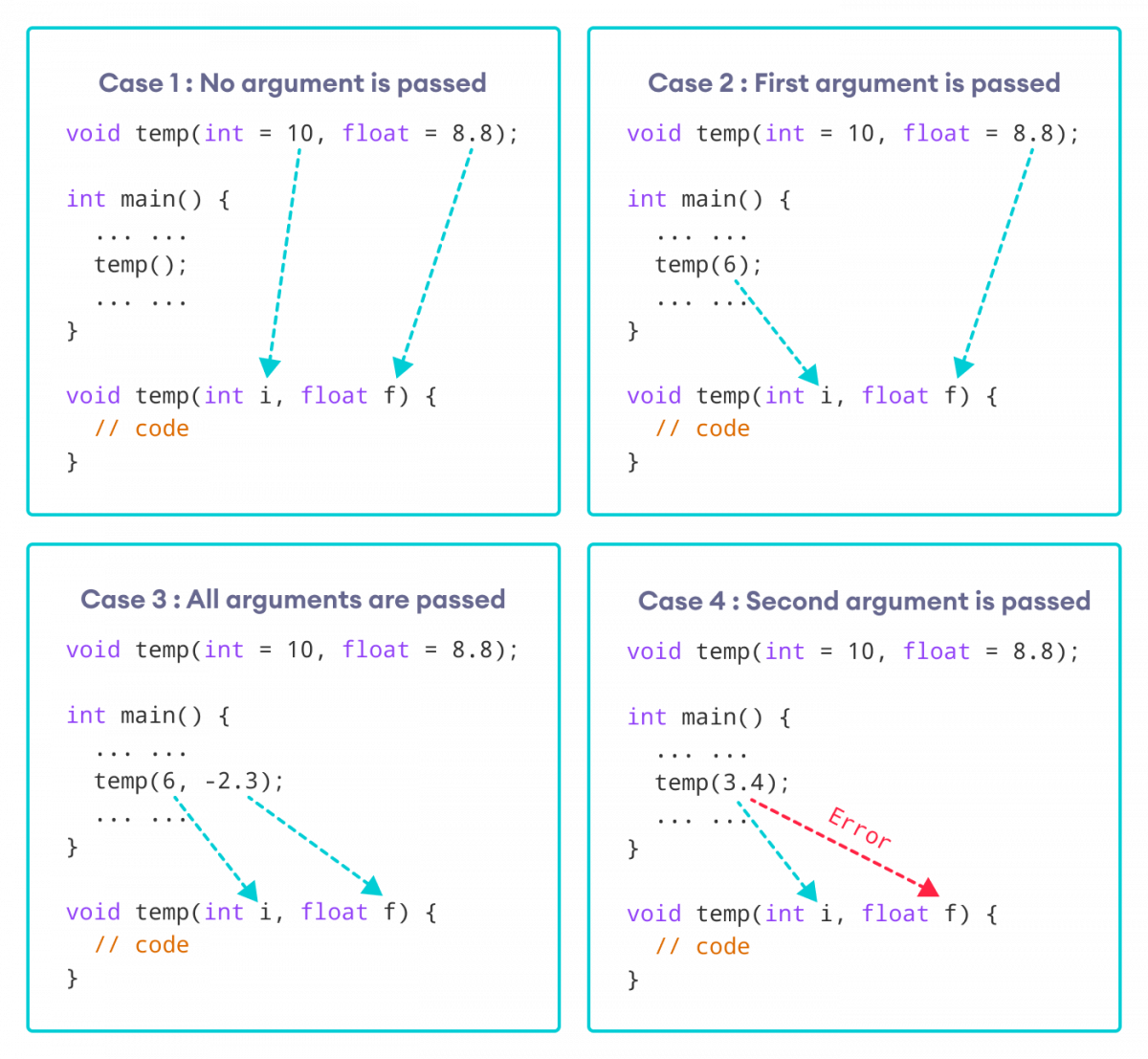
![Solved][Python] SyntaxError: non-default argument follows default argument Solved][Python] Syntaxerror: Non-Default Argument Follows Default Argument](https://img1.daumcdn.net/thumb/C176x176/?fname=https://blog.kakaocdn.net/dn/cz06uj/btqEspU1TLH/vttwhkkmaU5iJynx83z991/img.png)
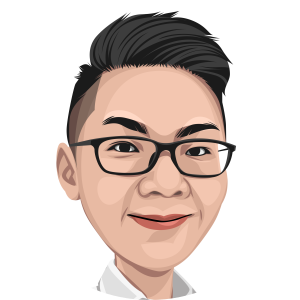
![Python Function Arguments [4 Types] – PYnative Python Function Arguments [4 Types] – Pynative](https://pynative.com/wp-content/uploads/2022/08/python_function_argument_and_parameter.jpg)
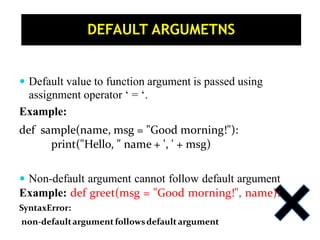
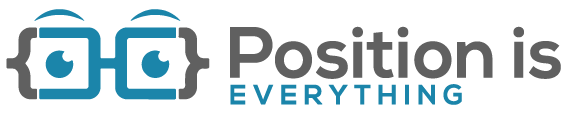
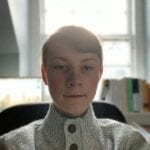
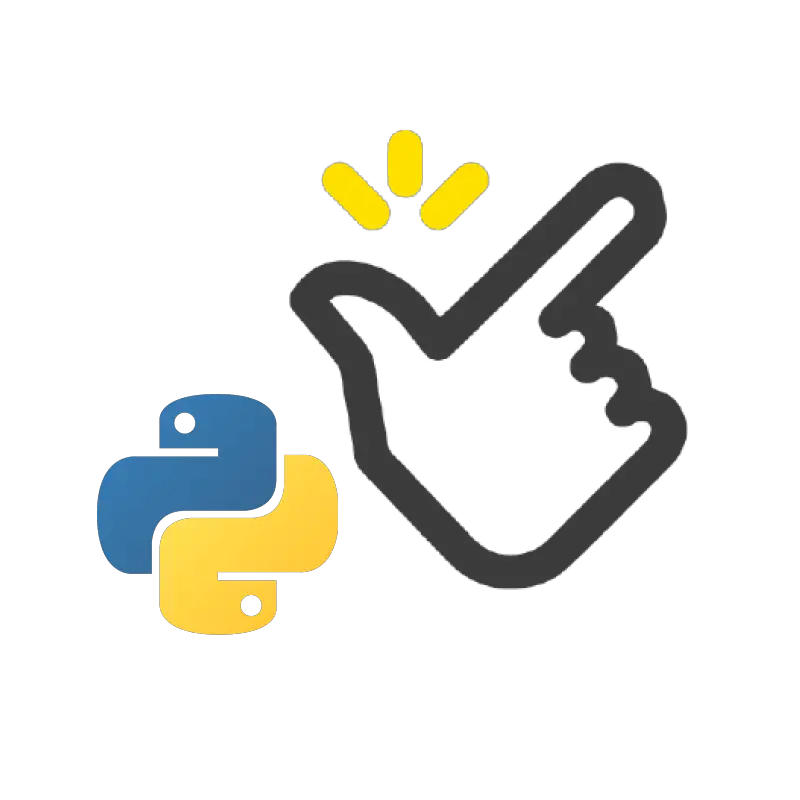
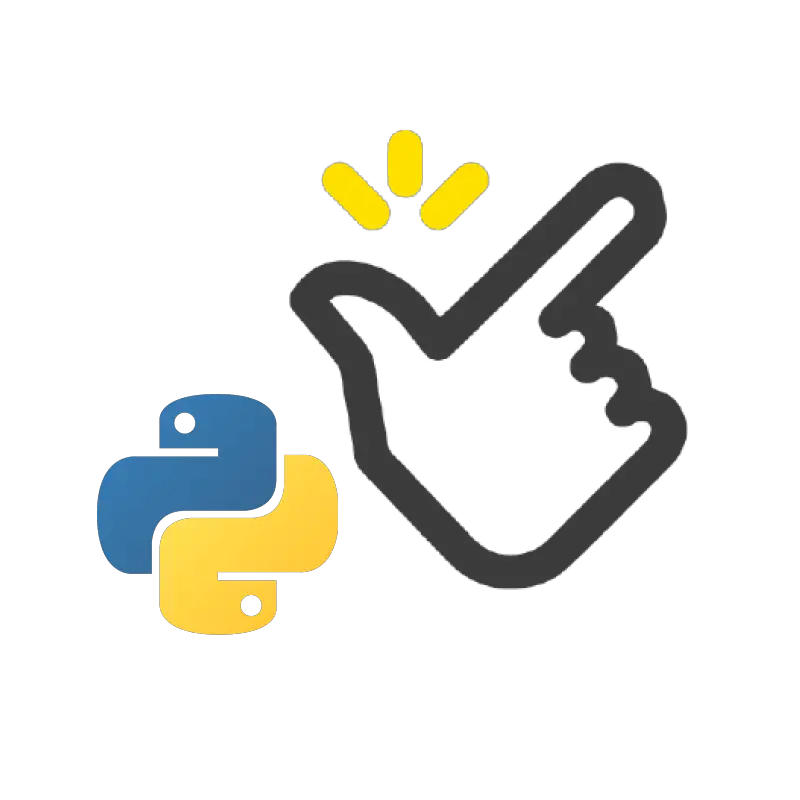
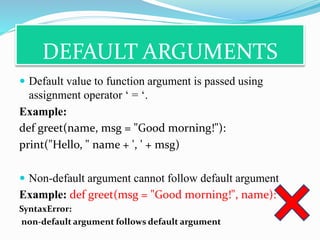
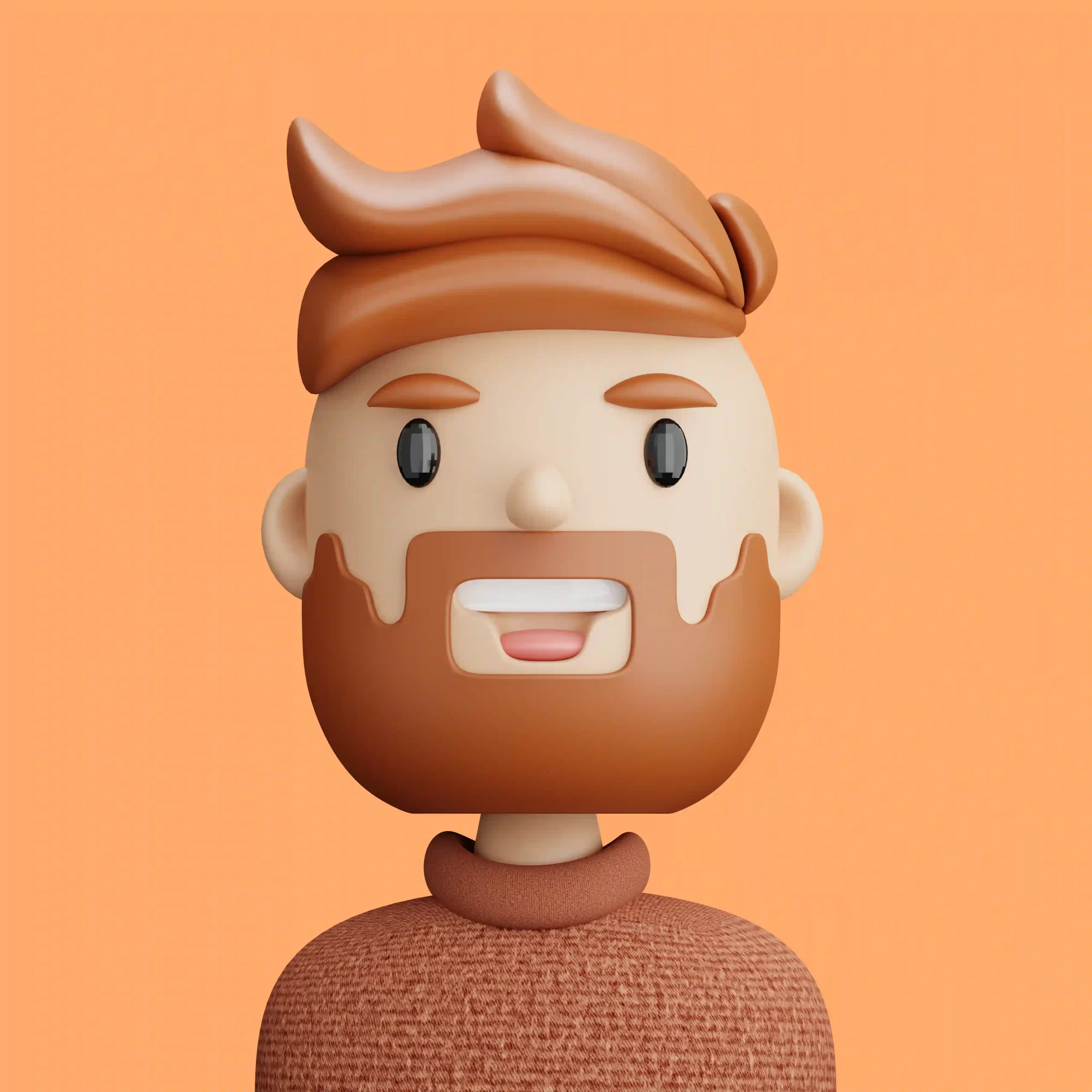
![Python] The Preview Version 3.10 Support The Python] The Preview Version 3.10 Support The](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2020/08/python.jpg?fit=768%2C457&ssl=1&resize=350%2C200)

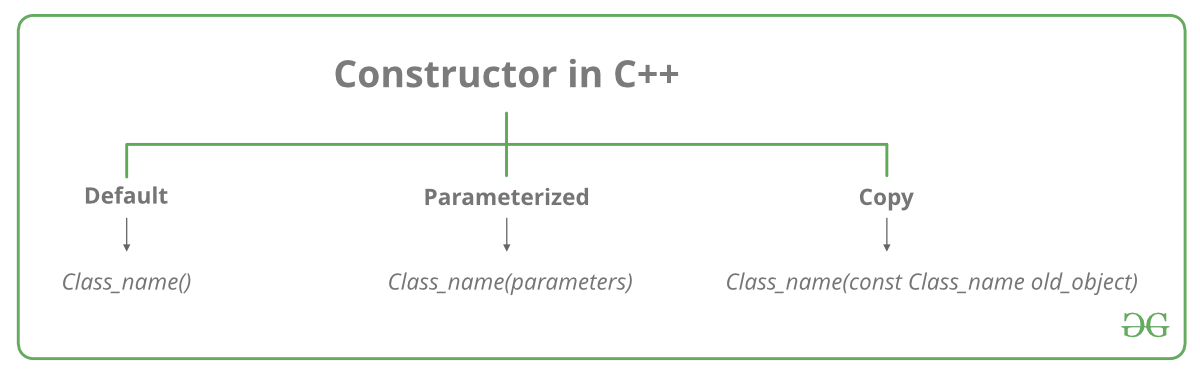

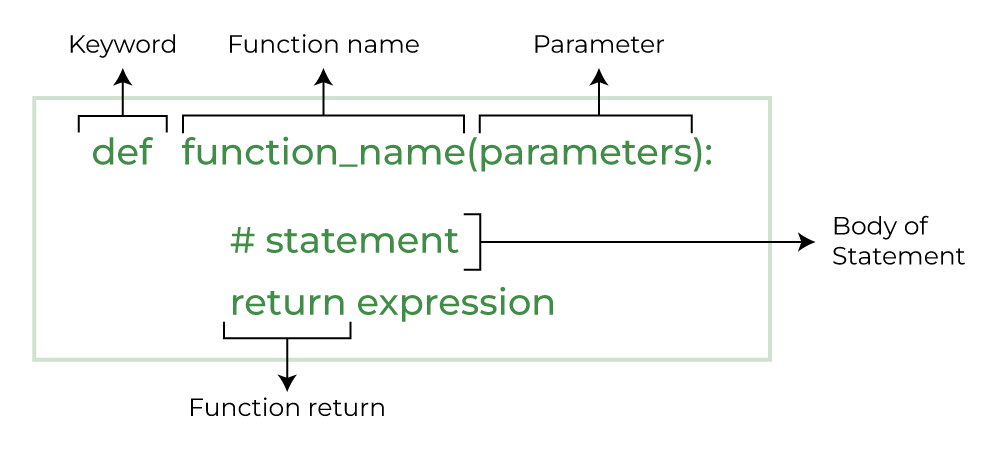
Article link: non-default argument follows default argument.
Learn more about the topic non-default argument follows default argument.
- How to Resolve the “SyntaxError: Non-Default Argument …
- SyntaxError: non-default argument follows default argument
- SyntaxError: non-default argument follows … – bobbyhadz
- 4 Ways to Fix Python SyntaxError: Non-Default Argument …
- Default arguments – cppreference.com – C++ Reference
- Default Arguments in C++ – GeeksforGeeks
- Python Function Arguments (Default, Keyword and Arbitrary) – Toppr
- Python SyntaxError: non-default argument follows default
- 4 Ways to Fix Python SyntaxError: Non-Default Argument …
- Solve Python SyntaxError: non-default argument follows …
- [Solved]: non-default argument follows default argument
- SyntaxError: non-default argument follows default argument
See more: https://nhanvietluanvan.com/luat-hoc/