Python Split Multiple Delimiters
Python is a versatile programming language that offers a wide range of built-in functions and methods, making it easy to manipulate and work with strings. One such method is the split() method, which allows us to split strings based on specific delimiters. In this article, we will explore how to split strings in Python using multiple delimiters, and also address some frequently asked questions regarding this topic.
Overview of the split() Method in Python
The split() method in Python is used to split a given string into a list of substrings, based on a specified delimiter. By default, the delimiter used is a whitespace character. The syntax for using the split() method is as follows:
string.split(separator, maxsplit)
Here, ‘string’ refers to the string that we want to split, ‘separator’ is the delimiter that identifies where the string should be split, and ‘maxsplit’ is an optional parameter that specifies the maximum number of splits to be performed.
Splitting Strings using a Single Delimiter
To split a string using a single delimiter, we simply pass the delimiter as an argument to the split() method. For example, consider the following code snippet:
“`python
string = “Hello,World”
result = string.split(“,”)
print(result)
“`
Output:
[‘Hello’, ‘World’]
In this example, we split the string “Hello,World” using the comma (‘,’) as the delimiter. The split() method returns a list with two elements: ‘Hello’ and ‘World’.
Splitting Strings using Multiple Delimiters
Python does not offer a built-in method to split strings using multiple delimiters directly. However, we can achieve this by using a combination of the split() method and list comprehension. Let’s take a look at an example:
“`python
import re
string = “Hello;World|Python”
delimiters = re.compile(“[;,|]”)
result = delimiters.split(string)
print(result)
“`
Output:
[‘Hello’, ‘World’, ‘Python’]
In this example, we use the re (regular expression) module to create a pattern that matches the delimiters we want to split the string with. The pattern ‘[;,|]’ matches the characters ‘;’, ‘,’ and ‘|’. The split() method is then used with this pattern to split the string.
Splitting Strings with Multiple Delimiters in a Single Step
If you want to split a string using multiple delimiters in a single step, you can use the re.split() function from the re module. This function allows us to split a string using multiple delimiters directly. Let’s see an example:
“`python
import re
string = “Hello;World|Python”
result = re.split(“[;,|]”, string)
print(result)
“`
Output:
[‘Hello’, ‘World’, ‘Python’]
Here, we use the re.split() function to split the string using the delimiters ‘[;,|]’. The result is the same as the previous example, but this method allows us to split the string in a more concise way.
Handling Empty Strings and Leading/Trailing Delimiters
When splitting strings, it is important to consider how empty strings and leading/trailing delimiters are handled. By default, the split() method and re.split() function remove empty strings from the resulting list. However, if we want to include empty strings, we can use the split() method with an additional argument.
“`python
string = “Hello;World;;;Python”
result = string.split(“;”)
print(result)
“`
Output:
[‘Hello’, ‘World’, ”, ”, ‘Python’]
In this example, the string contains multiple consecutive delimiters. The split() method includes the empty strings between these delimiters in the resulting list.
Conclusion
In this article, we explored how to split strings in Python using multiple delimiters. We learned about the split() method and the re.split() function, which can be used to split strings based on single or multiple delimiters. We also discovered how to handle empty strings and leading/trailing delimiters. By understanding these techniques, you’ll be able to efficiently split strings and manipulate the resulting substrings in your Python programs.
FAQs
Q: Can I split a string into multiple substrings based on a regular expression pattern?
A: Yes, you can use the re.split() function from the re module to split a string based on a regular expression pattern. This allows for more complex splitting scenarios.
Q: What is the use of the maxsplit parameter in the split() method?
A: The maxsplit parameter specifies the maximum number of splits to be performed. If not provided, all occurrences of the delimiter will be used to split the string.
Q: How can I split a column in a pandas DataFrame by multiple delimiters?
A: In pandas, you can use the str.split() method with regular expressions to split a column by multiple delimiters. This allows you to split strings within a DataFrame easily.
Q: Is it possible to split a string into a specific number of substrings in Python?
A: Yes, you can use the maxsplit parameter in the split() method to specify the maximum number of splits to be performed. This allows you to control the number of resulting substrings.
Q: Can I split a string using a regular expression pattern in Python?
A: Yes, you can use the re.split() function from the re module to split a string using a regular expression pattern. This gives you more flexibility in defining the delimiters.
Python Regex: How To Split A String On Multiple Characters
Can I Split A String With Multiple Delimiters Python?
Working with strings is a fundamental aspect of programming, and Python provides a robust set of tools to manipulate them. One common task in string processing is splitting a string into multiple parts, or tokens, based on certain delimiters. Python offers several methods to achieve this, and in this article, we will explore how to split a string using multiple delimiters.
Splitting Strings with split()
Python’s built-in string method, `split()`, is a versatile tool for dividing strings. By default, it splits a string into a list of substrings based on whitespace, also known as word splitting. However, it can also take a parameter called `sep` to specify a delimiter other than whitespace. For example:
“`python
string = “Hello, world! Welcome to Python.”
result = string.split(“,”)
print(result)
“`
In this case, the `split()` method will divide the string at every comma, resulting in the following output:
“`
[‘Hello’, ‘ world! Welcome to Python.’]
“`
Splitting Strings with re.split()
While `split()` is useful for simple delimiters, it lacks flexibility when it comes to more complex patterns. For such cases, the `re` module offers the `split()` function, which uses regular expressions to split strings. This method allows you to specify multiple delimiters using regular expression patterns.
Here’s an example that splits a string using both commas and periods as delimiters:
“`python
import re
string = “Hello, world! Welcome to Python.”
result = re.split(“[,.]”, string)
print(result)
“`
The output will be the following:
“`
[‘Hello’, ‘ world! Welcome to Python’, ”]
“`
Notice that the split occurred at both commas and periods, and an empty string was added as the last element because there was a delimiter at the end of the original string.
Splitting Strings with re.split() and Multiple Delimiters
To split a string with multiple delimiters using `re.split()`, you can combine the delimiters into a single regular expression pattern by using the `|` symbol, which acts as an “or” operator in regular expressions.
Here’s an example that splits a string using commas, periods, and exclamation marks as delimiters:
“`python
import re
string = “Hello, world! Welcome to Python.”
result = re.split(“[,.!]”, string)
print(result)
“`
The output will be as follows:
“`
[‘Hello’, ‘ world’, ‘ Welcome to Python’, ”]
“`
You can see that the string has been split at every comma, period, and exclamation mark, and an empty string was added as the last element because there was a delimiter at the end of the original string.
FAQs
Q: Can I split a string with multiple delimiters in Python using the `split()` method?
A: Yes, you can use the `split()` method to split a string based on a single delimiter. However, for multiple delimiters or more complex patterns, it is better to use the `re.split()` function.
Q: Are there any performance considerations when using `re.split()`?
A: The `re` module can be slower than the built-in `split()` method because regular expressions require additional processing. If performance is a concern, and you only need to split the string at a fixed set of delimiters, it may be more efficient to use the `split()` method.
Q: Can I split a string with multiple delimiters in a case-insensitive manner?
A: Yes, you can make the regular expression case-insensitive by using the `re.IGNORECASE` flag as the second argument for `re.split()`. For example: `re.split(“[,.!]”, string, flags=re.IGNORECASE)`.
Q: How can I split a string with both delimiters and keep the delimiters as separate tokens?
A: You can enclose the delimiters with parentheses inside the regular expression pattern. This will cause `re.split()` to include the delimiters as separate elements in the resulting list. For example: `re.split(“([,.!])”, string)`.
Q: Can I split a string with multiple delimiters of varying lengths?
A: Yes, you can use regular expressions to handle delimiters of varying lengths. For example, if you want to split a string at both “abc” and “def”, you can use the pattern “abc|def” in `re.split()`.
Can Split () Take 2 Arguments?
The split() function is a popular method used in many programming languages, including Python and JavaScript, to split a string into a list of substrings based on a specified separator. However, the split() function typically takes only one argument, which is the separator. So, can split() indeed take two arguments?
To answer this question, let’s first take a look at the basic syntax of the split() function. In both Python and JavaScript, the syntax is as follows:
In Python:
“`python
string.split(separator, maxsplit)
“`
In JavaScript:
“`javascript
string.split(separator, limit)
“`
From the syntax alone, it is evident that the split() function can indeed take two arguments. The second argument is specified as either `maxsplit` in Python or `limit` in JavaScript.
The primary purpose of this additional argument is to provide a limit on the number of splits that should be performed on the string. In other words, it determines the maximum number of elements that the resulting list of substrings can have.
For instance, consider the following example in Python:
“`python
string = “Welcome to OpenAI, the leading AI research lab”
result = string.split(” “, 3)
print(result)
“`
Here, we are using the split() function to split the given string into substrings using a space as a separator. However, we also specify the `maxsplit` argument as 3. This means that the resulting list will have at most 4 elements, with the third occurrence of the separator being the last split.
The output of the above code will be:
“`
[‘Welcome’, ‘to’, ‘OpenAI,’, ‘the leading AI research lab’]
“`
Similarly, in JavaScript, we can use the `limit` argument to achieve the same result. Here is the equivalent example in JavaScript:
“`javascript
let string = “Welcome to OpenAI, the leading AI research lab”;
let result = string.split(” “, 3);
console.log(result);
“`
The output will be the same as in Python:
“`
[‘Welcome’, ‘to’, ‘OpenAI,’, ‘the leading AI research lab’]
“`
As you can see, by using the second argument of the split() function, we were able to limit the number of splits performed and control the number of elements in the resulting list. This can be particularly useful in scenarios where we only need a certain number of substrings or want to extract specific portions of a string.
FAQs:
Q: Can split() take more than two arguments?
A: No, the split() function is designed to take only two arguments. The first argument is the separator, and the second argument controls the maximum number of splits.
Q: What happens if we don’t specify the second argument?
A: If the second argument is not specified, the split() function will consider it as unlimited and split the string into as many substrings as possible.
Q: What if the number of splits exceeds the limit specified by the second argument?
A: If the number of splits exceeds the limit, the remaining part of the string will be treated as a whole and added as the last element of the resulting list.
Q: Can we use regular expressions as separators in the split() function?
A: Yes, both Python and JavaScript allow the use of regular expressions as separators in the split() function. However, this introduces additional complexity and is beyond the scope of this article.
Q: Are there any performance implications of using the second argument?
A: In general, the performance impact of specifying the second argument is negligible. However, it is important to note that excessive splitting can result in a larger number of substrings, which may impact memory usage.
In conclusion, the split() function can indeed take two arguments – the separator and the maximum number of splits. By utilizing the second argument, you can control the number of resulting substrings and achieve more precise string manipulation. Understanding how to use the split() function effectively can greatly enhance your string handling capabilities in Python and JavaScript.
Keywords searched by users: python split multiple delimiters Split multiple characters Python, Python split maxsplit, Python split multi space, Split column by multiple delimiters pandas, Split n Python, Python split regex, Split python parameters, Split multiple characters js
Categories: Top 47 Python Split Multiple Delimiters
See more here: nhanvietluanvan.com
Split Multiple Characters Python
The split() function in Python is a versatile tool that can be used to split a string into multiple characters based on a specified delimiter. By default, the delimiter is a space character. However, you can specify any delimiter you want by passing it as an argument to the split() function.
Let’s start by looking at a basic example:
“`python
string = “Hello World”
split_string = string.split()
print(split_string)
“`
In the above code, we have a string “Hello World”. We use the split() function to split the string into individual characters. Since we didn’t provide any delimiter, it splits the string based on the space character. The output of the code will be:
“`
[‘Hello’, ‘World’]
“`
As you can see, the split() function has divided the string into two parts: “Hello” and “World”. Each part is stored as an element in a list.
If you want to split a string using a different delimiter, you can pass it as an argument to the split() function. For example:
“`python
string = “John,Doe,Jr.”
split_string = string.split(“,”)
print(split_string)
“`
In the above code, we have a string “John,Doe,Jr.”. We use the split() function with a comma (“,”) as the delimiter. It splits the string based on the comma and stores each part as an element in a list. The output of the code will be:
“`
[‘John’, ‘Doe’, ‘Jr.’]
“`
Another way to split multiple characters in Python is by using regular expressions. The re module in Python provides the split() function, allowing you to split a string using a regex pattern.
Here’s an example:
“`python
import re
string = “Python;Java;C#”
split_string = re.split(“;|,”, string)
print(split_string)
“`
In the above code, we import the re module and use the split() function with a regex pattern `;|,` as the delimiter. This pattern matches either a semicolon or a comma. It splits the string based on the matched pattern and stores each part as an element in a list. The output of the code will be:
“`
[‘Python’, ‘Java’, ‘C#’]
“`
Using regular expressions for splitting strings provides more flexibility, as you can define complex patterns to split the string based on your requirements.
Now, let’s address some frequently asked questions about splitting multiple characters in Python:
**Q: Can I split a string based on multiple delimiters?**
A: Yes, you can split a string based on multiple delimiters by using regular expressions with the re.split() function. For example, `re.split(“;|,|-“, string)` splits the string based on semicolon, comma, or hyphen.
**Q: What if I want to limit the number of splits performed?**
A: By default, the split() function splits the entire string. However, you can limit the number of splits performed by providing the `maxsplit` parameter. For example, `string.split(“,”, 1)` splits the string at the first occurrence of a comma.
**Q: Can I split the string from right to left?**
A: Yes, you can split the string from right to left by using the rsplit() function instead of split(). It works in the same way but starts splitting from the right side of the string.
**Q: What if I want to split a string into individual characters?**
A: If you want to split a string into individual characters, you can use an empty string as the delimiter. For example, `list(string)` will convert the string into a list of individual characters.
**Q: Are there any performance considerations when splitting large strings?**
A: Splitting large strings can have performance implications, especially when using regular expressions. If you’re working with very large strings, it’s worth considering other string manipulation methods or optimizing your regular expressions for better performance.
In conclusion, splitting multiple characters in Python is a straightforward task using the split() function or regular expressions. The flexibility provided by these methods allows you to split strings based on various delimiters and complex patterns. Keep in mind the performance considerations, especially when dealing with large strings.
Python Split Maxsplit
First, let’s understand what the split function does in Python. The split function is used to split a string into multiple substrings based on a specified delimiter. By default, the delimiter is a space character, but you can also specify a different delimiter. The split function returns a list of substrings after splitting the original string.
The syntax for the split function is as follows:
“`
string.split(separator, maxsplit)
“`
– `string` is the input string that needs to be split.
– `separator` is the delimiter used for splitting the string. It can be a string or a character. If not specified, the default delimiter is a space character.
– `maxsplit` is an optional parameter that specifies the maximum number of splits. It must be a non-negative integer. If not specified, the default value is -1, which means all occurrences of the delimiter will be considered for splitting.
Now, let’s explore the usage of the maxsplit parameter. The maxsplit parameter allows us to control the number of splits performed on the input string. This is beneficial when we only want to split the string into a specific number of parts, rather than splitting it completely.
Let’s consider an example to understand this better. Suppose we have the following string:
“`
text = “Python is a powerful and versatile programming language”
“`
If we use the split function with the default delimiter (space character) and don’t specify the maxsplit parameter, all occurrences of the space character will be considered for splitting. Thus, the resulting list would be:
“`
[‘Python’, ‘is’, ‘a’, ‘powerful’, ‘and’, ‘versatile’, ‘programming’, ‘language’]
“`
However, if we specify a maxsplit value of 2, the string will only be split into two parts, resulting in the following list:
“`
[‘Python’, ‘is a powerful and versatile programming language’]
“`
In this example, the first two occurrences of the space character were considered for splitting, and the rest of the string remained unsplit.
Now, let’s take a look at a few more examples to understand the behavior of the maxsplit parameter in different scenarios:
1. Splitting a string into individual characters:
“`
word = “Hello”
result = word.split(”, maxsplit=len(word)-1)
print(result)
“`
Output:
“`
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’]
“`
In this example, the empty string is used as the delimiter, causing the string to be split into its individual characters.
2. Splitting a string at specified occurrences:
“`
path = “/usr/local/bin/python3″
result = path.split(‘/’, maxsplit=2)
print(result)
“`
Output:
“`
[”, ‘usr’, ‘local/bin/python3’]
“`
In this example, the input string is split at the first two occurrences of the forward slash character (“/”). The resulting list contains three parts: an empty string, “usr”, and “local/bin/python3”.
3. Splitting a string without limiting the number of splits:
“`
sentence = “This is a sample sentence.”
result = sentence.split(‘ ‘)
print(result)
“`
Output:
“`
[‘This’, ‘is’, ‘a’, ‘sample’, ‘sentence.’]
“`
In this example, the input string is split at each occurrence of the space character, resulting in a list of individual words.
Now that we have covered the usage and examples of Python split maxsplit, let’s address some frequently asked questions about this topic.
**FAQs**
**Q:** What is the default value of the maxsplit parameter?
**A:** If the maxsplit parameter is not specified, the default value is -1, which means all occurrences of the delimiter will be considered for splitting.
**Q:** Can the maxsplit value be zero?
**A:** No, zero is not a valid value for the maxsplit parameter. It must be a non-negative integer.
**Q:** How does the split function handle consecutive occurrences of the delimiter?
**A:** Consecutive occurrences of the delimiter are treated as a single occurrence. They will only lead to a single split in the resulting list.
**Q:** Can the delimiter string be more than one character long?
**A:** Yes, the delimiter string can be of any length. If it is longer than one character, all occurrences of the entire string will be considered for splitting.
**Q:** What happens if the maxsplit value is greater than the number of occurrences of the delimiter?
**A:** If the maxsplit value exceeds the number of occurrences, the split function will perform as many splits as there are occurrences of the delimiter. The remaining part of the string will not be split.
In conclusion, Python split maxsplit is a useful function for dividing strings and sequences based on a specific delimiter. By using the maxsplit parameter, we can control the number of splits performed. Understanding the behavior and usage of this parameter allows us to manipulate strings more effectively in Python.
Images related to the topic python split multiple delimiters
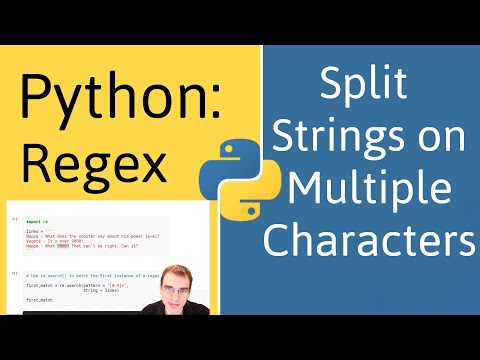
Found 7 images related to python split multiple delimiters theme


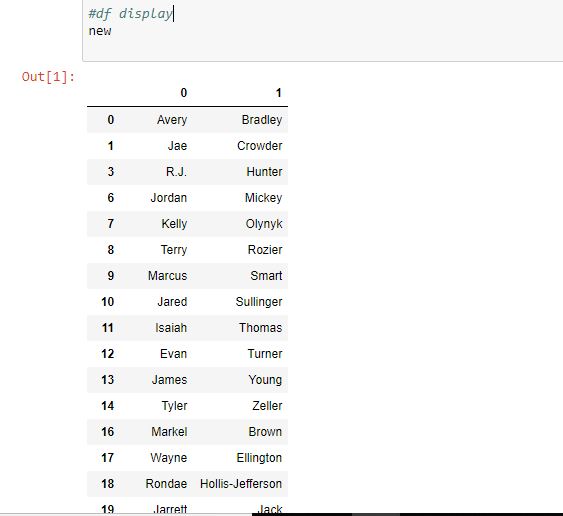
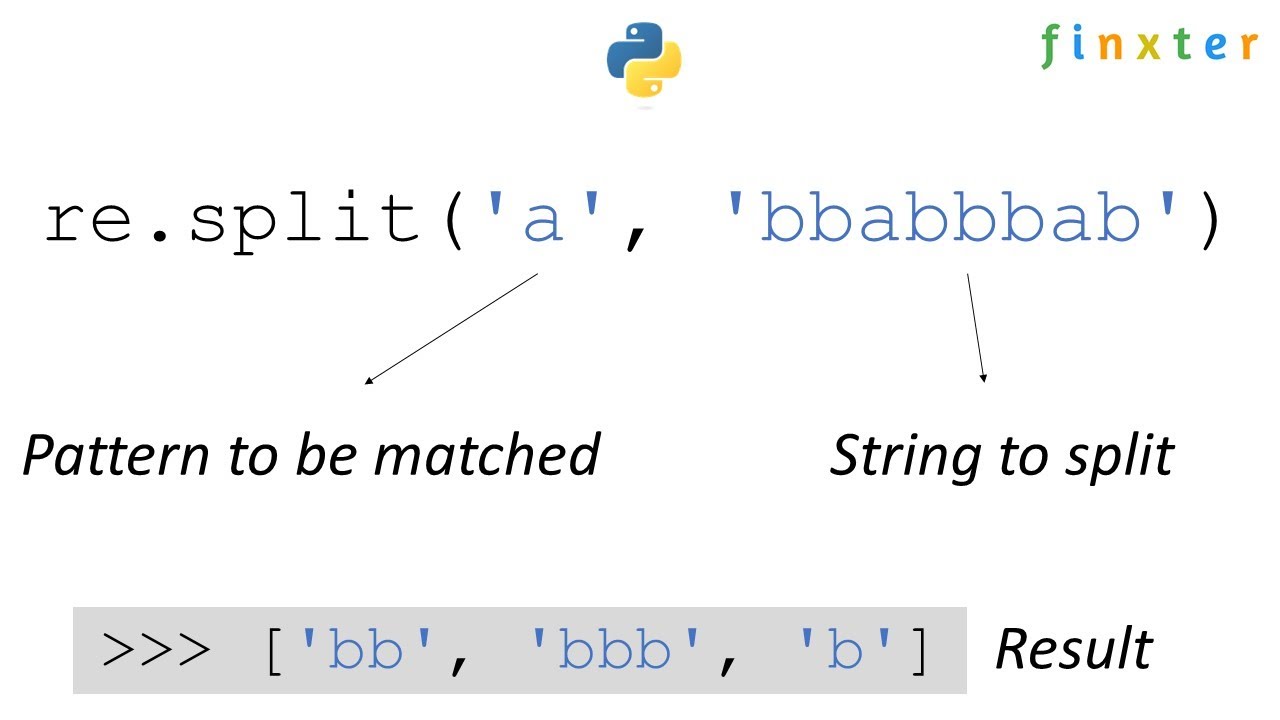
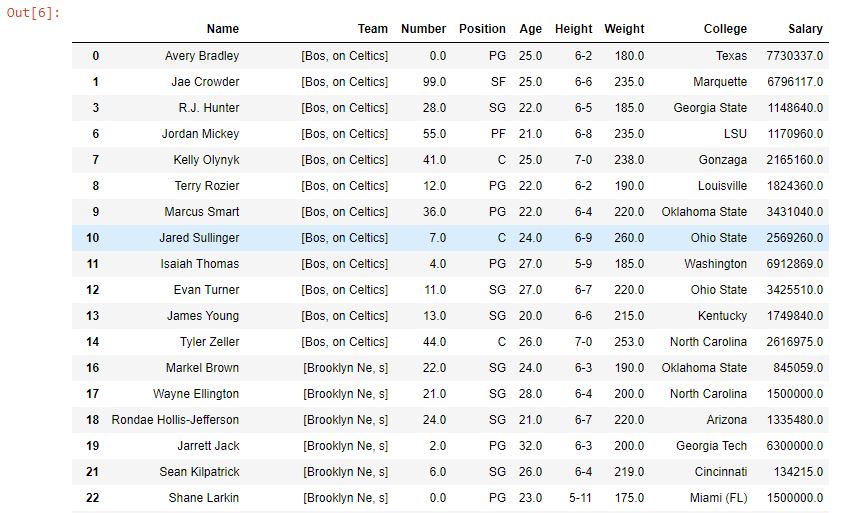
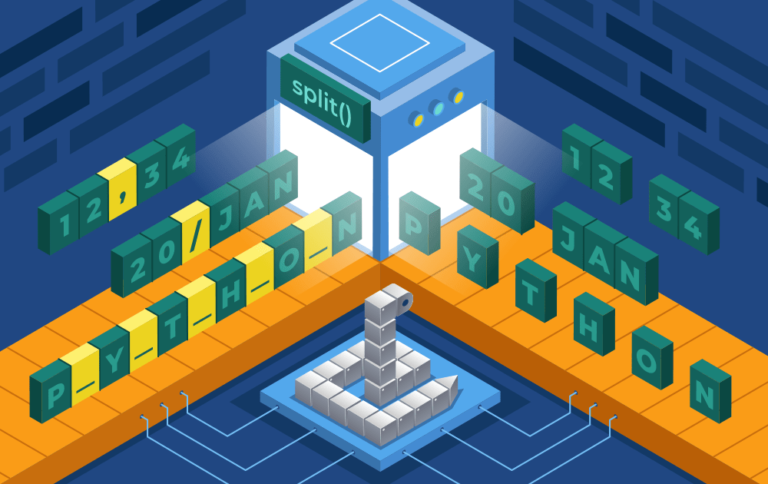
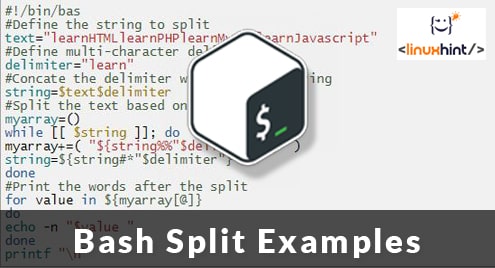
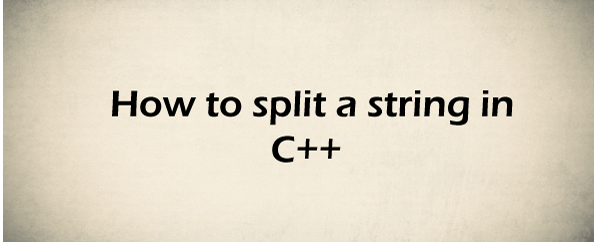
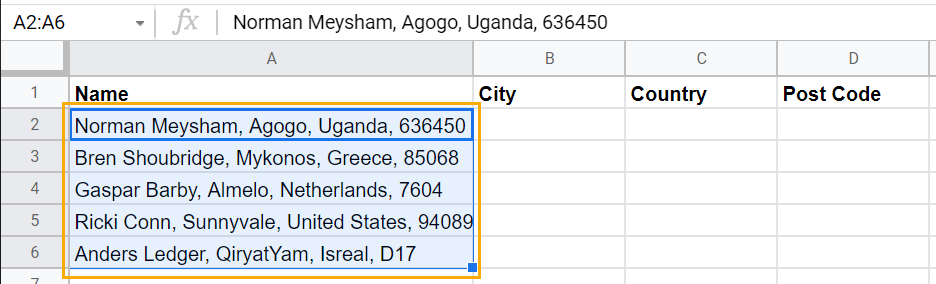
![Split in Python: An Overview of Split() Function [Updated] Split In Python: An Overview Of Split() Function [Updated]](https://www.simplilearn.com/ice9/free_resources_article_thumb/Cassandra_Data_Types.jpg)
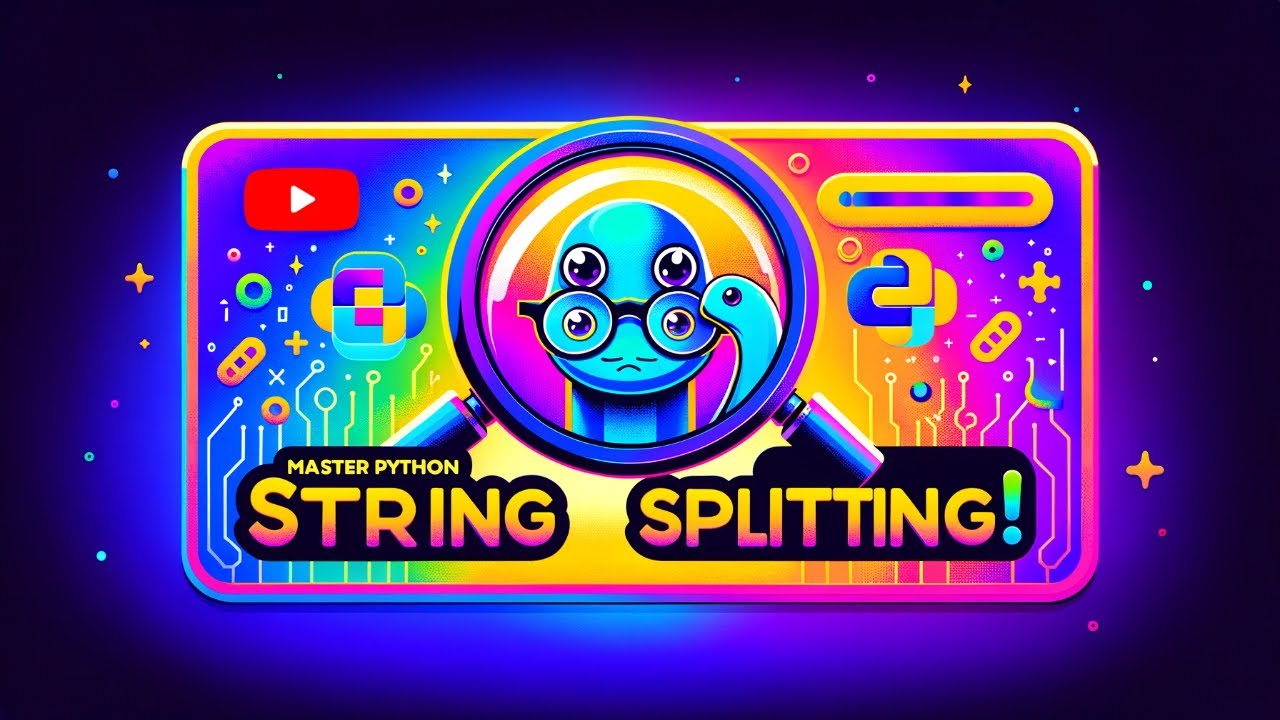
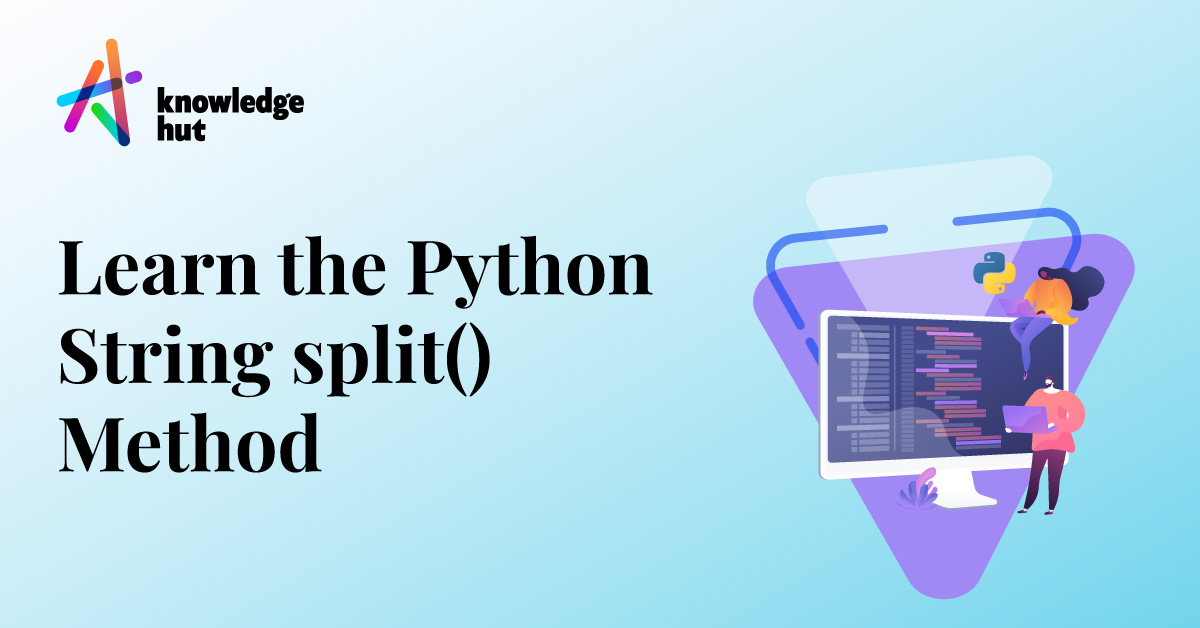
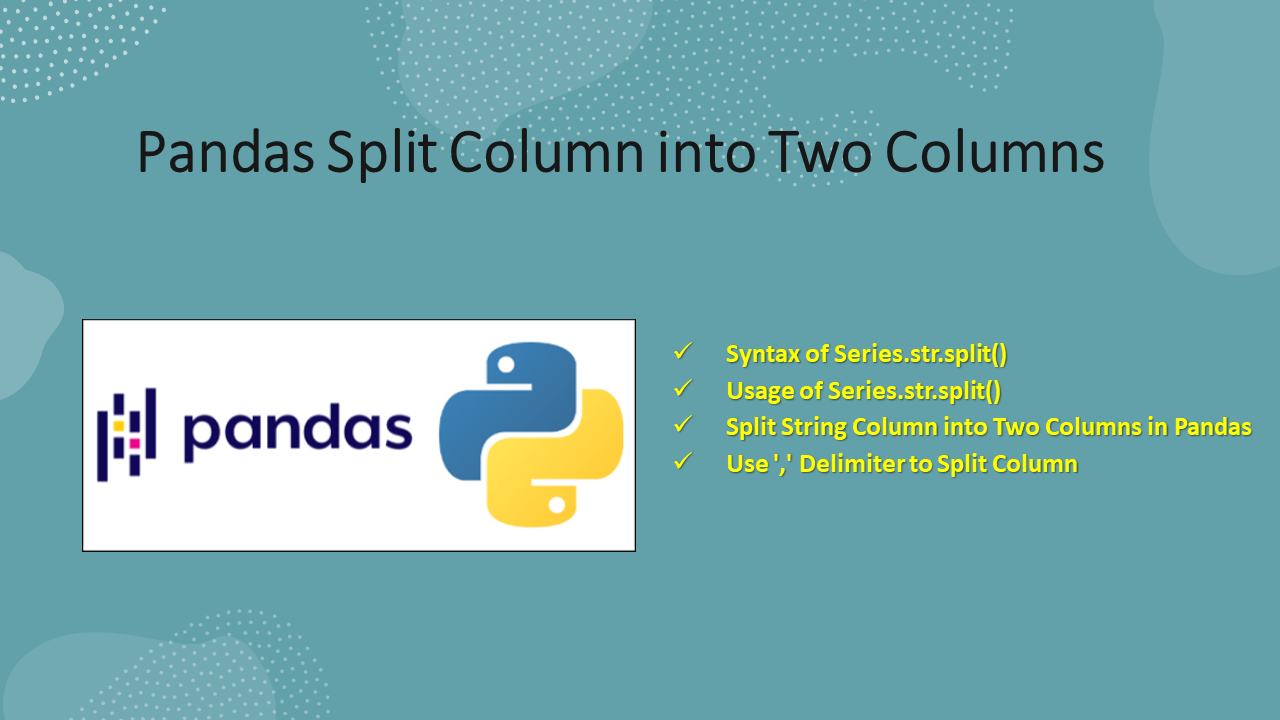
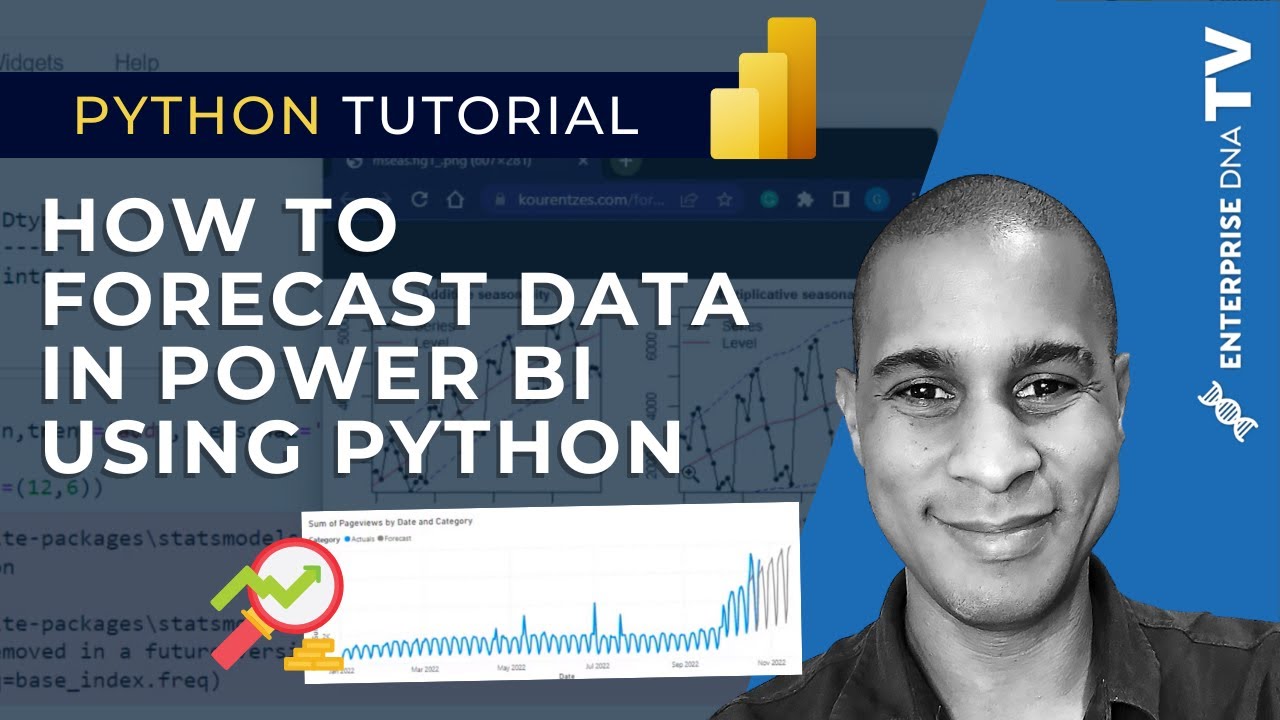
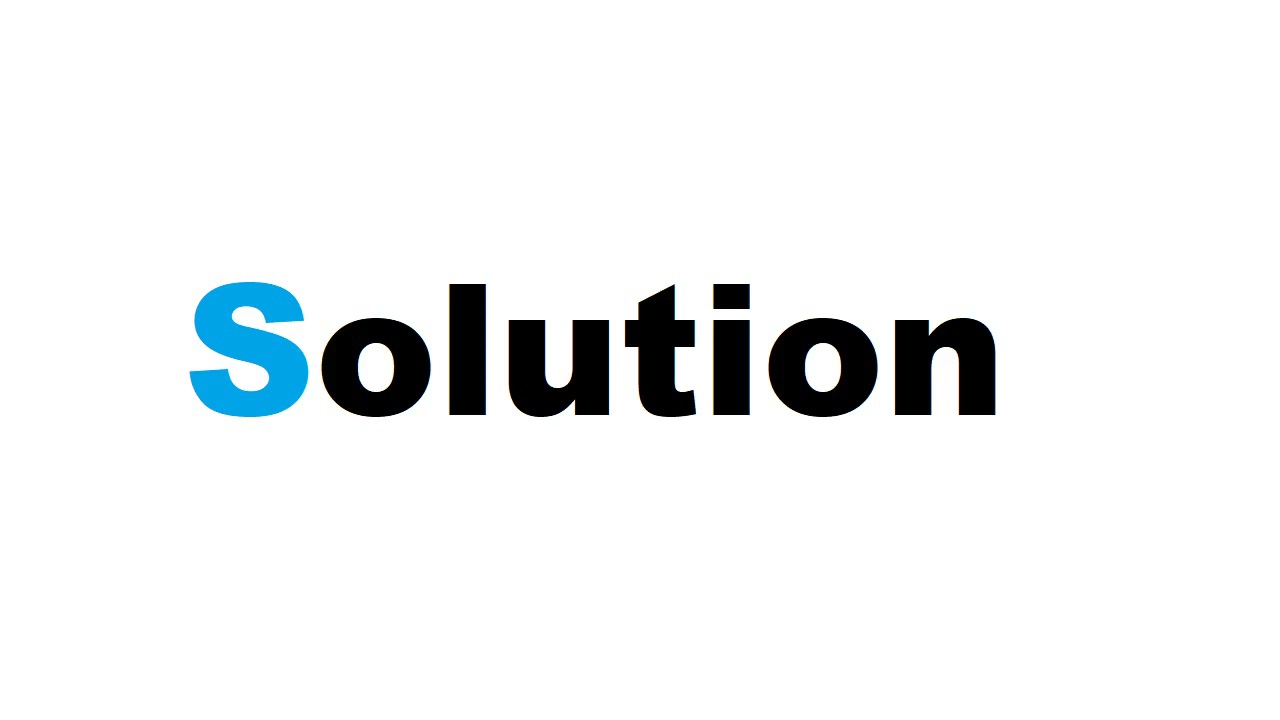
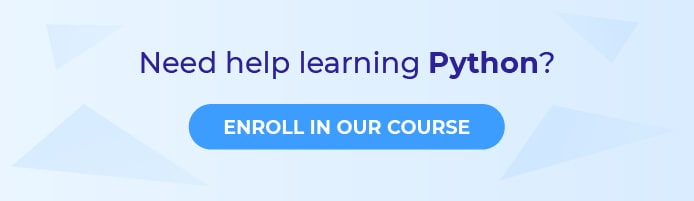
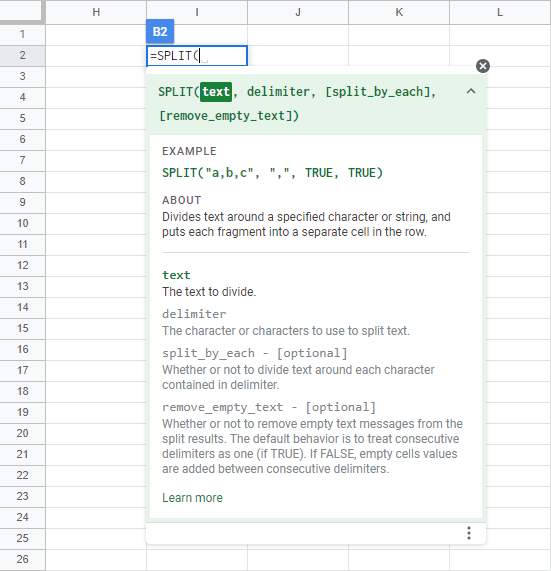
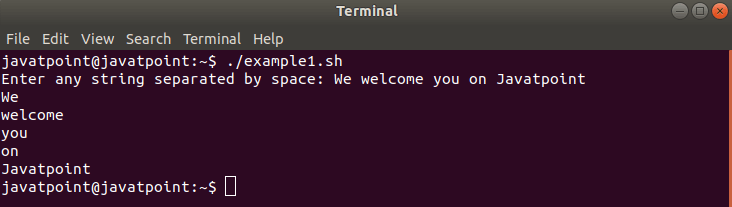
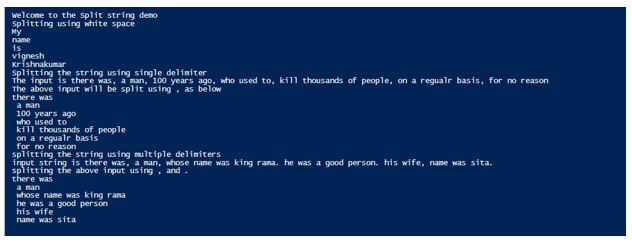
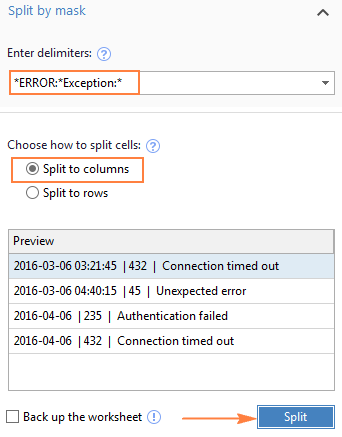
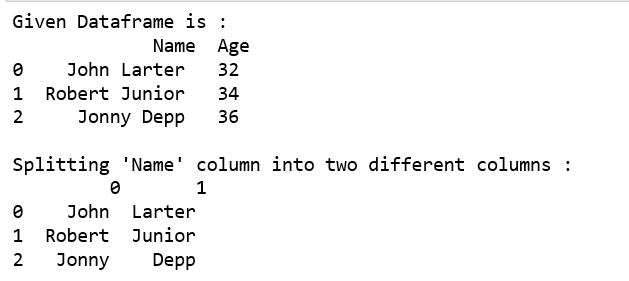
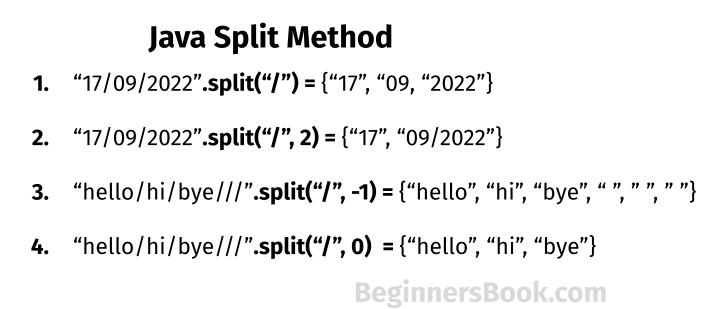


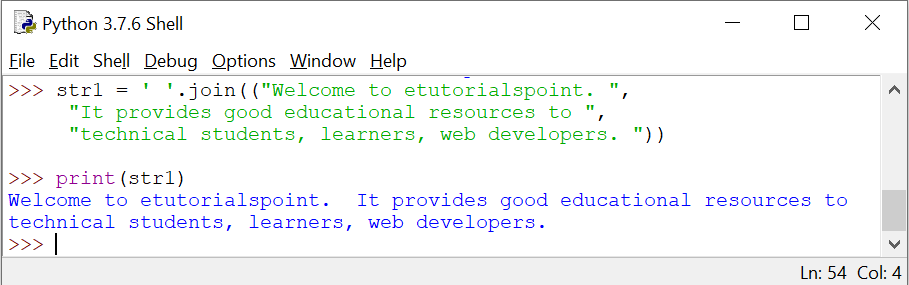
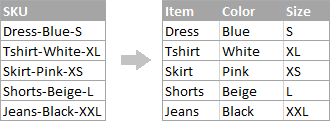
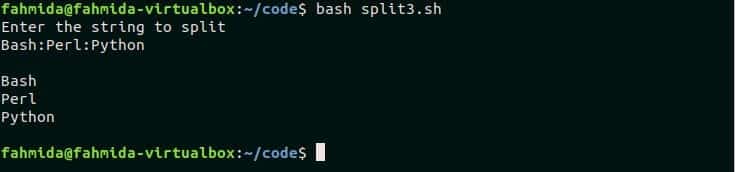
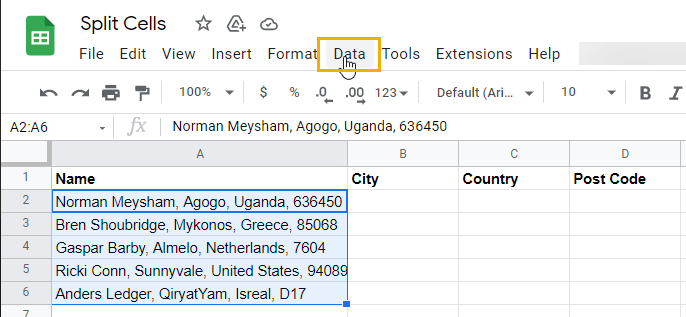
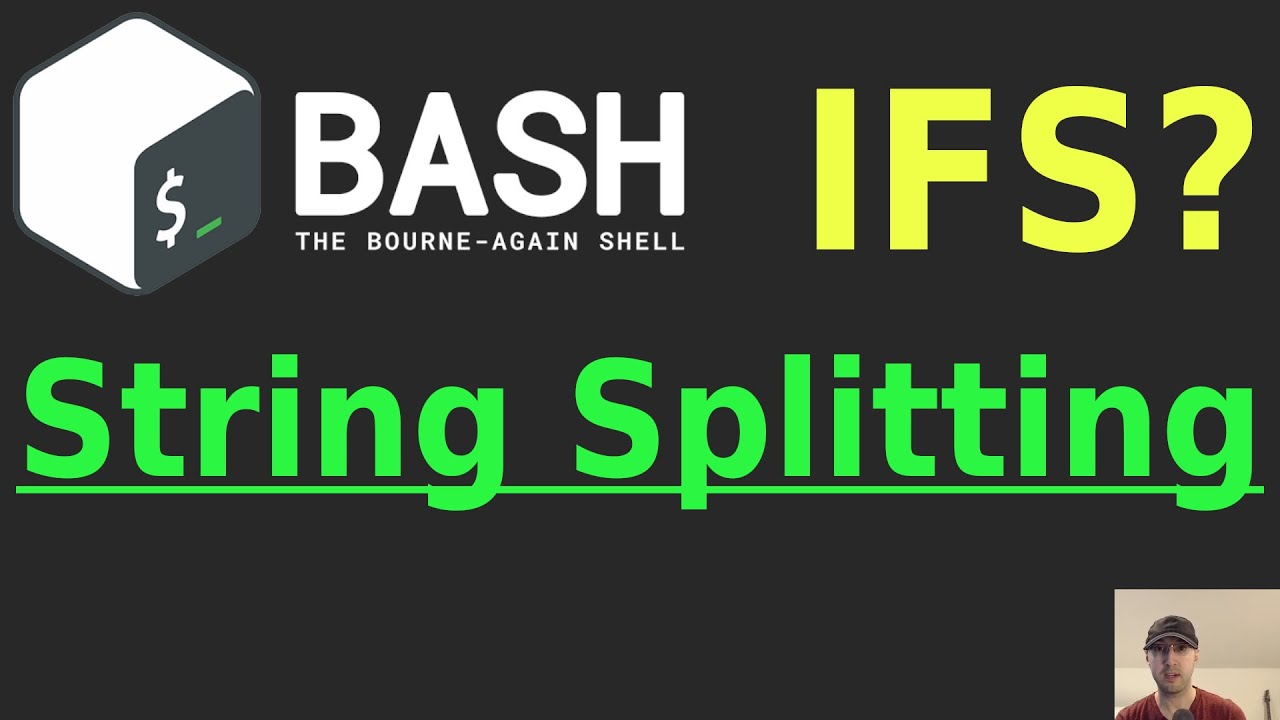
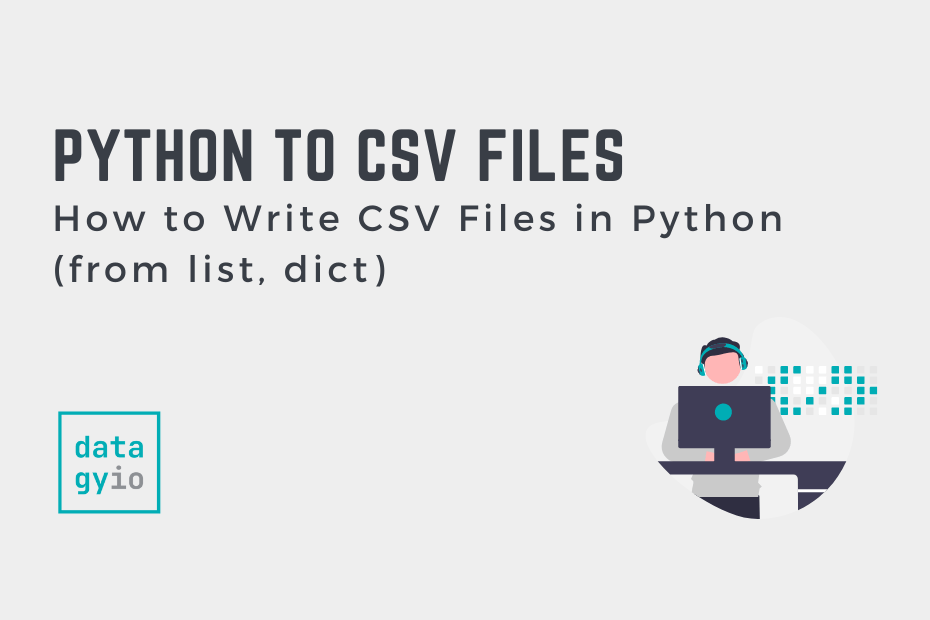

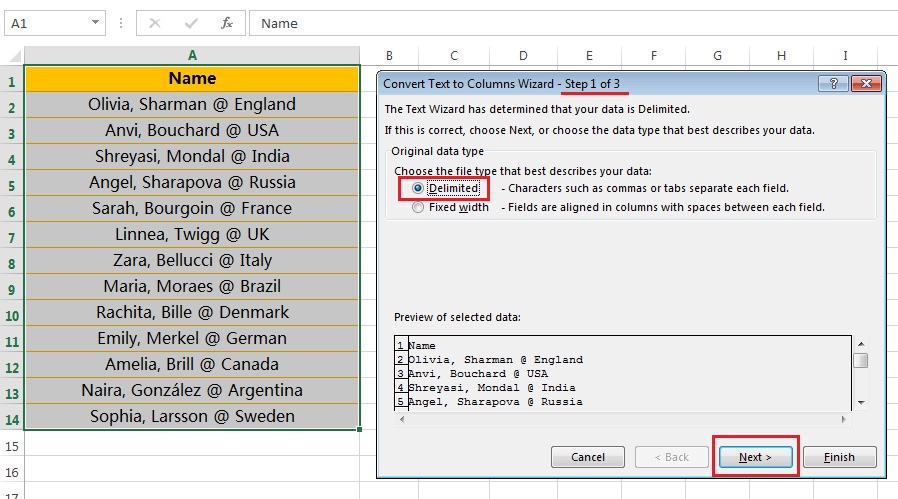
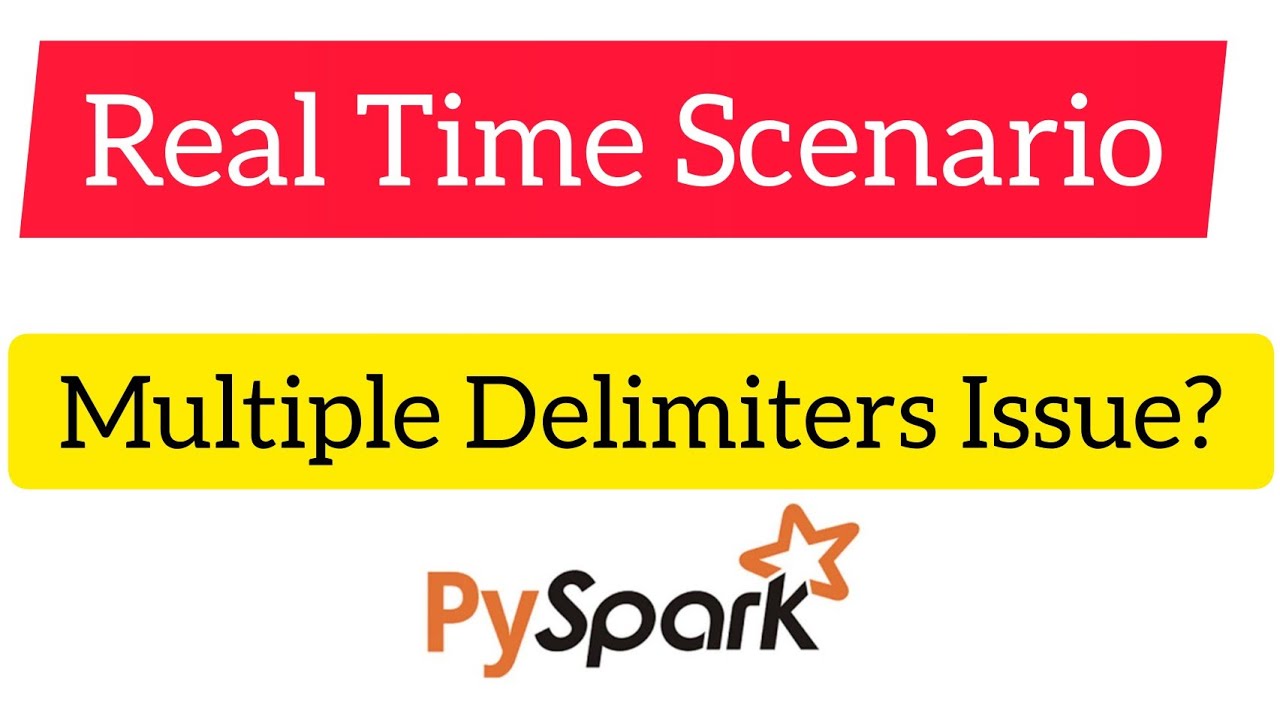
Article link: python split multiple delimiters.
Learn more about the topic python split multiple delimiters.
- Split string with multiple delimiters in Python – Stack Overflow
- Python: Split a String on Multiple Delimiters – Datagy
- How to Split String on Multiple Delimiters in Python
- Python .split() – Splitting a String in Python – freeCodeCamp
- Split in Python: An Overview of Split() Function – Simplilearn
- Split a string with multiple delimiters in Python – bobbyhadz
- How to Split String on Multiple Delimiters in Python
- How to Split String with Multiple Delimiters in Python
- How can I use Python regex to split a string by multiple …
- Python: Split a string with multiple delimiters – w3resource
- How To Split A String With Multiple Delimiters In Python
- Split string with multiple delimiters in Python – W3docs
- How can I split a string by multiple delimiters in Python?
See more: https://nhanvietluanvan.com/luat-hoc