Python String To Boolean
Introduction to Python Strings and Booleans
In Python programming language, strings and booleans are both fundamental data types. While strings are used to represent text, booleans are used to represent logical values, i.e., either True or False. The ability to convert strings to booleans in Python can be immensely useful, especially when dealing with user inputs or reading data from external sources.
Understanding the Concept of Boolean Data Type in Python
A boolean data type represents truth values, where True represents a condition that is satisfied or a statement that holds true, and False represents a condition that is not satisfied or a statement that is false. Booleans are primarily used in control structures such as if-else statements and while loops, where the code execution depends on the truth value of certain conditions.
Converting a String to Boolean using the `bool()` Function
Python provides a built-in function called `bool()` that allows us to convert various data types, including strings, to boolean values. When the `bool()` function is applied to a string, it evaluates the string and returns a boolean value based on certain rules.
Converting Empty Strings and Non-Empty Strings to Boolean Values
When converting a string to a boolean using the `bool()` function, an empty string (”) is considered as False, while a non-empty string is evaluated as True. This means that if the string contains any characters, spaces, or special characters, it will be considered as True.
Handling Case-Insensitive String Comparisons for Boolean Conversion
By default, string comparisons in Python are case-sensitive. However, when converting strings to booleans, it may be necessary to perform case-insensitive comparisons. To achieve this, you can use the `lower()` or `upper()` methods of the string before applying the `bool()` function.
Handling Specific String Values for Boolean Conversion
In some cases, you may want to convert specific string values to a boolean, irrespective of their length or case. For example, you may want to treat the strings “yes”, “true”, “on”, or “1” as True, and “no”, “false”, “off”, or “0” as False. In such scenarios, you can compare the string with the desired values and convert them accordingly.
Using Comparison Operators for String to Boolean Conversion
In addition to using the `bool()` function, you can also use comparison operators to convert strings to booleans.
Evaluating String Equality for Boolean Conversion
To convert a string to a boolean based on its equality to another string, you can use the `==` operator. If the strings are equal, it will return True, and if they are not equal, it will return False.
Evaluating String Inequality for Boolean Conversion
Similarly, if you want to convert a string based on its inequality to another string, you can use the `!=` operator. If the strings are not equal, it will return True, and if they are equal, it will return False.
Considerations when Converting Numbers Represented as Strings to Booleans
When dealing with numbers represented as strings, there are some additional considerations to keep in mind.
Converting String Representations of Integers to Booleans
When converting a string representation of an integer to a boolean, any non-zero string will be considered as True, while “0” (zero) will be considered as False.
Converting String Representations of Floating-Point Numbers to Booleans
When converting a string representation of a floating-point number to a boolean, any non-zero value, including decimal fractions, will be considered as True, while “0.0” (zero) will be considered as False.
Converting Other Types of Data Contained in Strings to Booleans
In some cases, strings may contain data types other than just numbers or alphabets. For example, a string representation of a list or a dictionary. When converting such strings to booleans, the `bool()` function will treat them as True, regardless of their contents.
Evaluating String Representations of True or False for Boolean Conversion
If a string directly represents the boolean values “True” or “False”, the `bool()` function will convert them accordingly, returning True if the string is “True” and False if the string is “False”.
Handling Exceptions when Converting Strings to Booleans
It is important to handle exceptions when converting strings to booleans, especially when dealing with user inputs or inputs from external sources. Invalid string inputs that cannot be evaluated as boolean values may result in errors. To handle such cases, you can use exception handling techniques such as try-except blocks to gracefully handle any potential errors.
Best Practices and Tips for String to Boolean Conversion in Python
1. Always validate and sanitize user inputs before converting them to booleans to ensure reliable conversions.
2. Use the `lower()` or `upper()` methods to perform case-insensitive string comparisons if required.
3. Clearly define and handle specific string values that need to be converted to booleans.
4. Consider the consequences of converting numbers represented as strings, and ensure it aligns with your intended logic.
5. Use comparison operators for more customized string to boolean conversions.
6. Implement exception handling to gracefully handle any invalid string inputs for boolean conversion.
7. Use descriptive variable names and add comments to improve code readability.
In conclusion, Python offers several methods to convert strings to boolean values, providing flexibility and versatility when working with user inputs or external data. By using the `bool()` function, comparison operators, and considering specific requirements, developers can effectively convert strings to booleans and utilize them in their Python programs.
FAQs
Q1. How can I turn a string into a boolean in Python?
To turn a string into a boolean in Python, you can use the `bool()` function. It will evaluate the string and return a boolean value based on certain rules.
Q2. How can I convert a boolean to a string in Python?
To convert a boolean to a string in Python, you can use the `str()` function. It will convert the boolean value into its string representation.
Q3. How can I input a boolean value in Python?
In Python, you can input a boolean value by either assigning it directly to a variable or obtaining it from user input. For example, you can assign `True` or `False` to a variable or prompt the user to enter a boolean value using the `input()` function.
Q4. How can I convert a string to a boolean in JavaScript?
In JavaScript, you can use the `Boolean()` function to convert a string to a boolean. However, the conversion rules may differ compared to Python, so be sure to consult the JavaScript documentation for specific details.
Q5. How can I change a string to a boolean in JavaScript?
To change a string to a boolean in JavaScript, you can use the `Boolean()` function. It will evaluate the string and return a boolean value based on JavaScript’s conversion rules.
Q6. How can I check the type of a boolean value in Python?
In Python, you can check the type of a boolean value using the `type()` function. For example, `type(True)` will return `
Q7. What is the `bool()` function in Python?
The `bool()` function in Python is a built-in function that allows you to convert various data types to boolean values. When applied to a string, it evaluates the string and returns either True or False based on certain rules.
Q8. How can I evaluate user input as Python code and convert it to a boolean?
To evaluate user input as Python code and convert it to a boolean, you can use the `eval()` function. However, it is essential to be cautious when using `eval()` as it can execute potentially harmful code. Always validate and sanitize user inputs before using `eval()`.
In conclusion, understanding and effectively converting strings to booleans is an important skill in Python programming. By utilizing the provided functions, comparison operators, and considering the specific requirements, developers can seamlessly incorporate string to boolean conversions in their Python programs.
How To Convert From String To Boolean In Python
Keywords searched by users: python string to boolean Turn string into boolean python, Convert boolean to string Python, Input boolean in python, Convert string to boolean js, How to change string to boolean in javascript, Check type boolean python, Bool() function in Python, Eval(input Python)
Categories: Top 22 Python String To Boolean
See more here: nhanvietluanvan.com
Turn String Into Boolean Python
Introduction
In Python, a boolean variable can have two values: True or False. However, when working with user inputs or data from external sources, we often encounter strings that represent boolean values. This article aims to provide a comprehensive guide on how to turn a string into a boolean in Python, covering various methods and scenarios.
Table of Contents:
1. Converting String to Boolean
2. Common Scenarios and Methods
3. Handling Case Sensitivity
4. FAQs
1. Converting String to Boolean:
Python provides a built-in function called `bool()` that allows us to convert a string into a boolean value. The `bool()` function takes a string as an argument and returns `True` if the string represents a `True` value and `False` otherwise.
For example:
“`
string_true = “True”
boolean_true = bool(string_true)
print(boolean_true) # Output: True
string_false = “False”
boolean_false = bool(string_false)
print(boolean_false) # Output: False
“`
2. Common Scenarios and Methods:
a. Converting String with “True” or “False”:
When dealing with strings explicitly representing boolean values, as shown in the example above, the `bool()` function works perfectly. It evaluates the string and returns the corresponding boolean value.
b. Converting String with “0” or “1”:
Sometimes, instead of “True” or “False”, we encounter strings representing boolean values using “1” for `True` and “0” for `False`. In such cases, we can first convert the string to an integer using `int()`, and then convert the integer to a boolean using the `bool()` function.
For example:
“`
string_true = “1”
boolean_true = bool(int(string_true))
print(boolean_true) # Output: True
string_false = “0”
boolean_false = bool(int(string_false))
print(boolean_false) # Output: False
“`
c. Converting String with Other Representations:
If the string representing a boolean value is expressed differently, such as “on” for `True` and “off” for `False`, we can utilize if-else statements to handle these cases. We can convert the string to lowercase for easy comparison and use logical conditions to assign the corresponding boolean value.
Example:
“`
string_on = “on”
boolean_on = False
if string_on.lower() == “on”:
boolean_on = True
print(boolean_on) # Output: True
“`
3. Handling Case Sensitivity:
By default, the `bool()` function is not case-sensitive. It evaluates the string “true” the same way it evaluates “True”, returning `True` in both cases. However, this may not be the desired behavior in certain situations, where case sensitivity is crucial.
To handle case-sensitive conversions, we can use the `str.lower()` method or explicitly compare the string with both possible representations.
For example:
“`
string_true = “tRuE”
boolean_true = None
if string_true.lower() == “true”:
boolean_true = True
print(boolean_true) # Output: True
“`
4. FAQs:
Q1. What happens if we use `bool()` on an empty string?
A1. When an empty string is passed to the `bool()` function, it returns `False`. This behavior is because an empty string is considered as False in Python.
Q2. Can we use `bool()` on strings that are not representing boolean values?
A2. Yes, `bool()` can be used on any string. If the string is empty, it returns `False`. Otherwise, it returns `True`. However, for strings explicitly representing boolean values, direct conversion techniques are recommended.
Q3. What happens if we pass a non-boolean string to `bool()`?
A3. When a string other than “True”, “False”, “1”, or “0” is passed to `bool()`, it will return `True` since any non-empty string is considered `True` in Python.
Q4. How can we handle unexpected input values when converting to boolean?
A4. To handle unexpected input values, it is recommended to utilize if-else statements, explicitly defining the expected representations and providing fallback options for unanticipated cases.
Q5. Are there any third-party libraries available for converting strings to boolean?
A5. While there might be some third-party libraries that provide specific functionalities for converting strings to boolean, utilizing the built-in functions and techniques mentioned in this article should suffice for most use cases.
Conclusion:
In Python, converting a string into a boolean value is essential when handling user inputs or working with data from external sources. Understanding how to perform this conversion using the built-in `bool()` function and additional techniques enables efficient and accurate data processing. By following the methods discussed in this comprehensive guide, you will be able to handle various scenarios when turning strings into booleans in Python.
Convert Boolean To String Python
In Python, boolean values are represented by the True and False keywords. Occasionally, you may come across situations where you need to convert a boolean value to a string for various purposes, such as printing, logging, or manipulating the data. This article will walk you through the different methods available to convert a boolean to a string in Python, along with examples and explanations.
Method 1: Using the str() function
The str() function in Python is a built-in function that converts values to a string representation. It can be used to convert a boolean value to its string equivalent. Here’s an example:
“`
value = True
str_value = str(value)
print(str_value)
“`
Output:
“`
True
“`
In this method, the str() function converts the boolean value True to the string value “True”. Similarly, you can convert the boolean value False to a string by replacing True with False in the above code.
Method 2: Using the format() method
Python’s format() method provides another way to convert a boolean value to a string. You can specify the boolean value as an argument inside curly braces {} and use the format() method to convert it to a string. Let’s see an example:
“`
value = True
str_value = “{}”.format(value)
print(str_value)
“`
Output:
“`
True
“`
By using the format() method, the boolean value True is converted to the string value “True”. You can apply the same approach to convert the boolean value False to a string.
Method 3: Using string interpolation
String interpolation is a powerful feature in Python, which allows you to insert variables directly into strings using placeholders. String interpolation can be used to convert a boolean value to a string by including the boolean variable inside curly braces {}. Here’s an example:
“`
value = True
str_value = f”{value}”
print(str_value)
“`
Output:
“`
True
“`
In this method, the boolean value True is converted to the string value “True” using string interpolation. Similarly, you can convert the boolean value False to a string by replacing True with False in the above code.
FAQs:
Q1. Why would I need to convert a boolean to a string in Python?
A. There can be several scenarios where you may need to convert a boolean to a string. For example, when you want to print or log the boolean value, or when you need to manipulate it in a specific string format, converting it to a string becomes necessary.
Q2. Can I directly use a boolean value in string concatenation?
A. No, you cannot directly concatenate a boolean value with a string in Python. However, by converting the boolean to a string using any of the mentioned methods, you can concatenate the converted string with other strings.
Q3. Are there any differences between the different methods discussed?
A. All of the methods mentioned above achieve the same goal of converting a boolean to a string. However, they differ in syntax and usage. Choose the method that best suits your coding style and requirements.
Q4. Can I use the same methods to convert strings to booleans?
A. No, the methods discussed in this article are used to convert boolean values to strings. If you want to convert a string to a boolean in Python, you can use the bool() function or comparison operators.
Q5. Can I customize the string representation of boolean values during conversion?
A. Yes, you can customize the conversion process by adding conditional statements. For example, if you want to convert True to “YES” and False to “NO”, you can use an if-else statement or a dictionary to achieve the desired string representation.
In conclusion, converting a boolean value to a string in Python is essential in many scenarios. By using functions like str(), format(), and string interpolation, you can easily achieve this conversion. Make use of the provided examples and FAQs to clarify any doubts you may have encountered while exploring this topic. Finally, choose the method that best fits your needs and coding style, ensuring a seamless conversion process.
Input Boolean In Python
Python is a versatile programming language that offers numerous tools and features to simplify the development process. One such feature is input boolean, which allows users to interact with their code through simple yes/no or true/false responses. In this article, we will dive deep into input booleans in Python, exploring their purpose, implementation, and potential use cases. Additionally, a section of frequently asked questions (FAQs) will be provided to address common queries and misunderstandings.
Understanding Input Booleans:
An input boolean is a mechanism in Python that enables a program to receive boolean values from users. These values help shape the program’s execution and decision-making process. This feature is particularly useful when creating interactive programs or when user input is needed to guide the code’s flow.
Implementation of Input Booleans:
Implementing input booleans in Python is a straightforward process. The built-in input() function serves as the foundation for obtaining user input. By combining input() with some conditional statements and logical operators, we can create robust input boolean structures.
Let’s take a look at a simple example:
“`python
user_input = input(“Do you like pizza? (yes/no) “)
if user_input.lower() == “yes”:
print(“Awesome! Who doesn’t like pizza?”)
else:
print(“Oh, that’s sad. Let’s have pizza anyway!”)
“`
In this example, we prompt the user with a question and store their response in the `user_input` variable. Using lower() helps in case-insensitive comparison. The input boolean is then tested using an if-else statement, allowing different execution paths based on the user’s response.
Use Cases for Input Booleans:
1. Configuring Program Behavior: Input booleans are commonly used in user configuration scenarios. By asking users simple yes/no questions, a program can be customized to suit their preferences. For instance, a game could ask whether to enable sound effects or not, enhancing the gaming experience according to the player’s choice.
2. Making Decisions in Programs: Input booleans allow users to guide the program’s decision-making process. For example, an e-commerce application may ask if the customer wants to receive promotional emails. The user’s response can determine whether the code sends future marketing messages.
3. Building Interactive Interfaces: By collecting user input through input booleans, Python programs can create interactive command-line interfaces. This makes programs more user-friendly and allows users to interact with the code in real-time.
FAQs:
Q1. Can input booleans only accept yes/no responses?
A1. No, input booleans can accept any string representing a true/false value. The comparison is usually done using logical operators such as `==` or `!=` to evaluate the user’s input.
Q2. How do I handle invalid user input?
A2. It is crucial to handle scenarios where users input unexpected or invalid values. You can use while loops to repeatedly prompt the user until a valid input is given. Additionally, the `try-except` block can be utilized to gracefully handle exceptions and display appropriate error messages.
Q3. Can input booleans be used with graphical user interfaces (GUI)?
A3. Yes, input booleans can be adapted for use with GUI frameworks like Tkinter or PyQt. They provide graphical elements such as checkboxes or radio buttons, simplifying boolean input collection.
Q4. Is there a way to simplify repetitive input boolean code?
A4. Yes, repetitive code can be streamlined using functions or classes. By encapsulating input boolean functionality, you can reuse the same code multiple times throughout your program, making it more readable and maintainable.
Q5. Are there alternative methods for gathering user input?
A5. Apart from input booleans, Python offers other input mechanisms, such as inputting integers, floating-point numbers, or strings. The choice of input mechanism depends on the type of data required from the user.
Conclusion:
Input booleans provide a valuable means of collecting user input in Python programs. By leveraging this feature, developers can create interactive programs, adjust program behavior, and efficiently gather valuable user information. Understanding how to implement and utilize input booleans expands the possibilities of Python programming. So, go ahead and incorporate input booleans into your code to make it more engaging and collaborative with users.
Images related to the topic python string to boolean
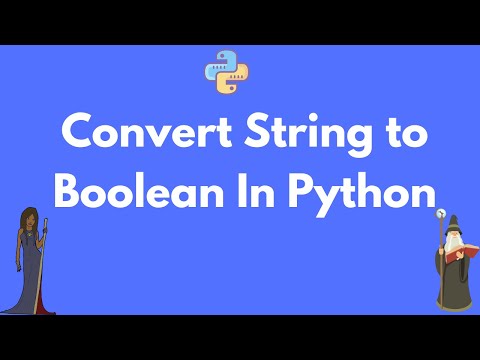
Found 44 images related to python string to boolean theme


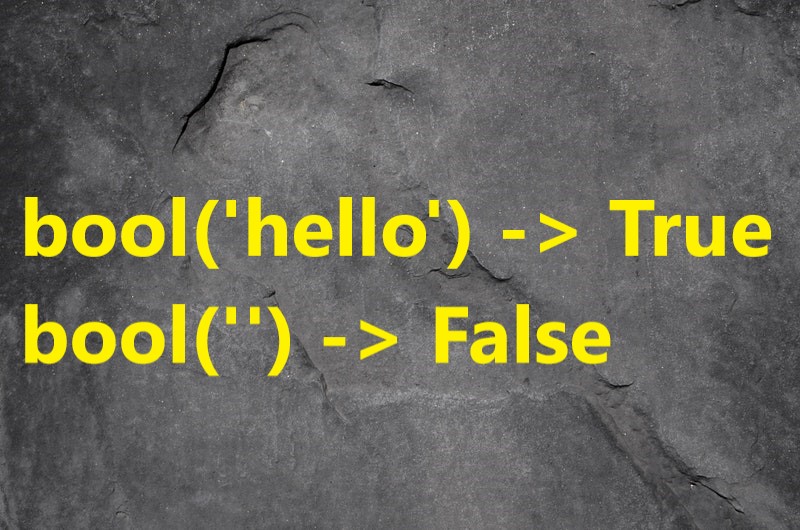


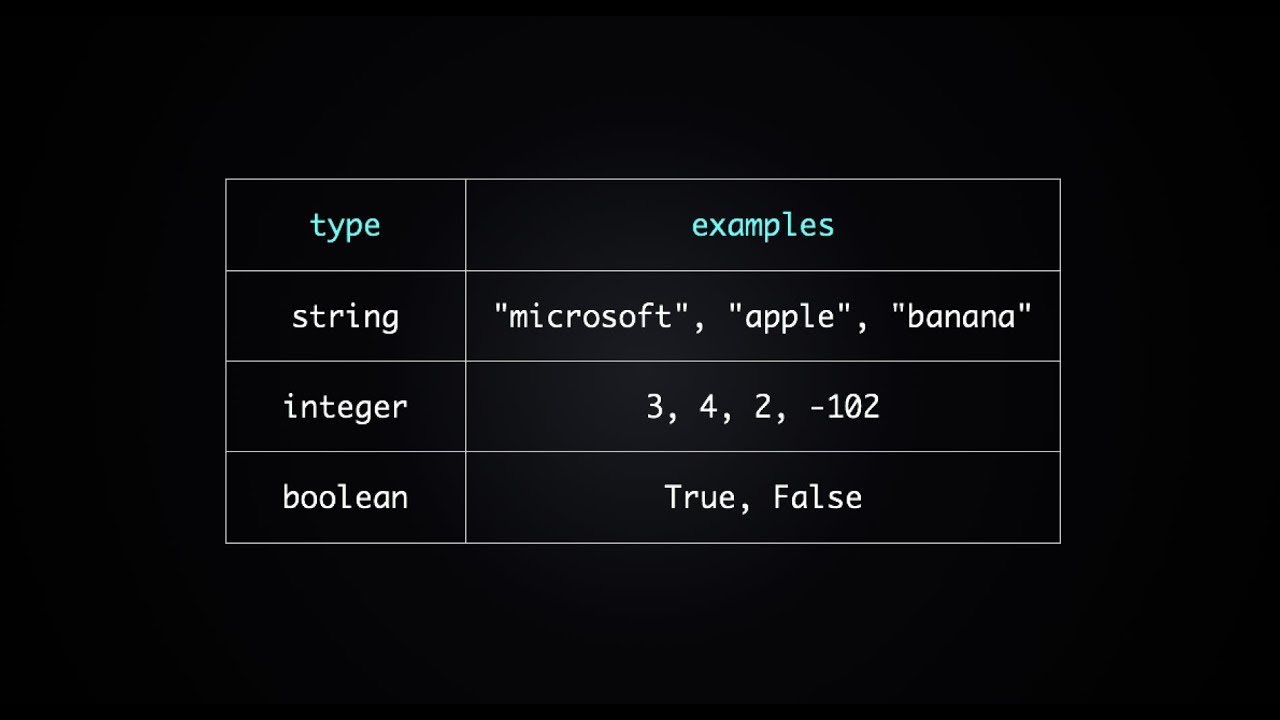
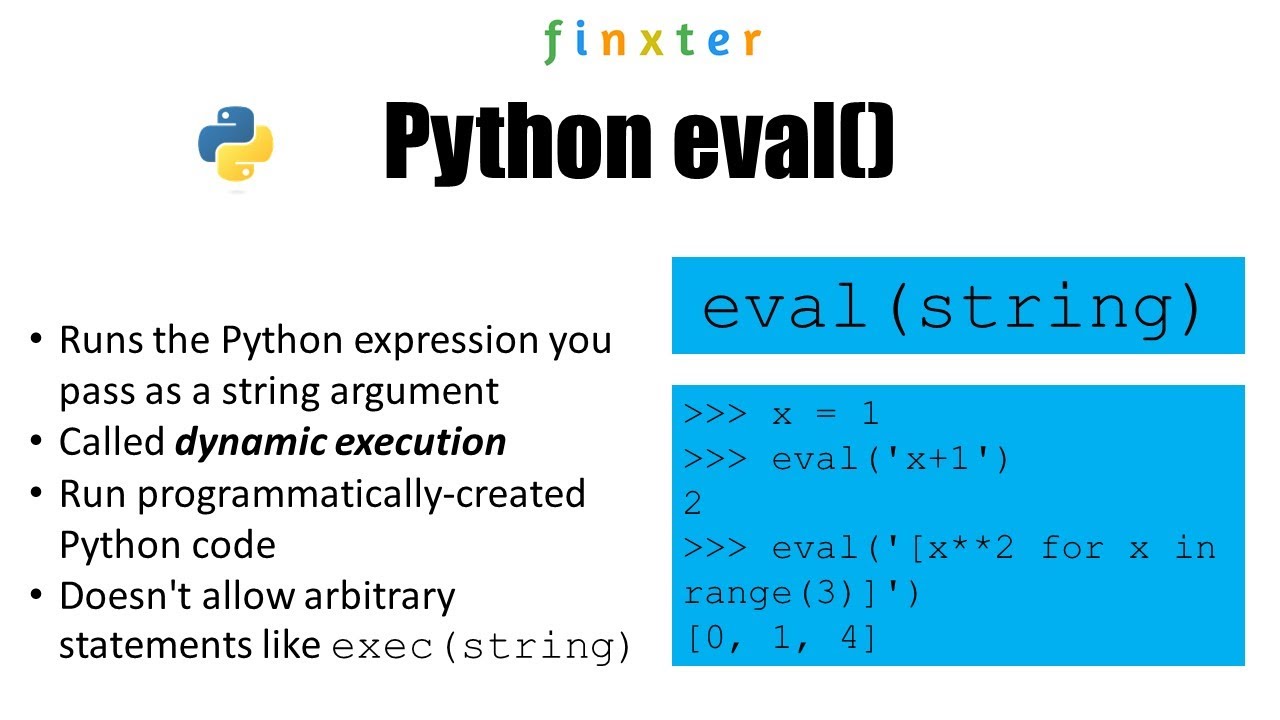

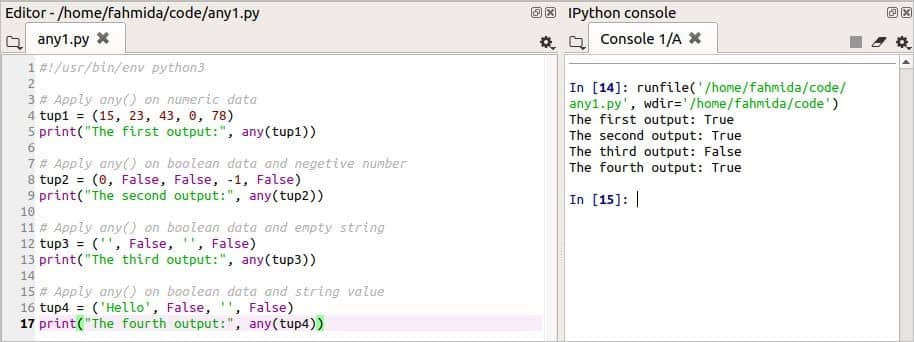


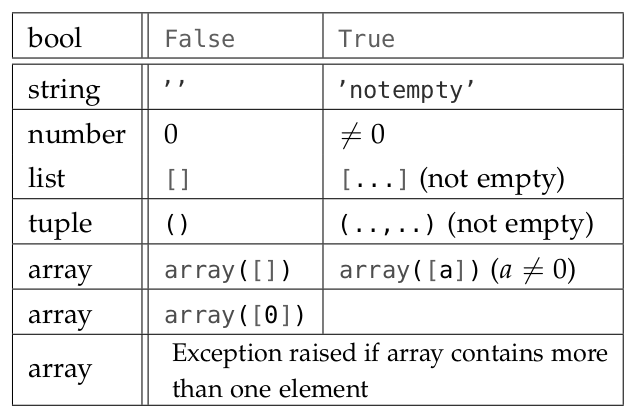

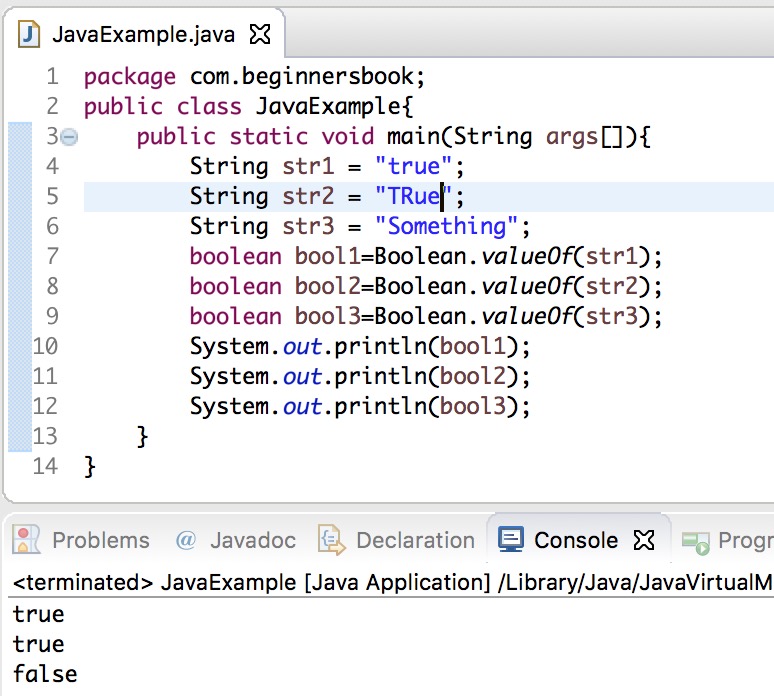
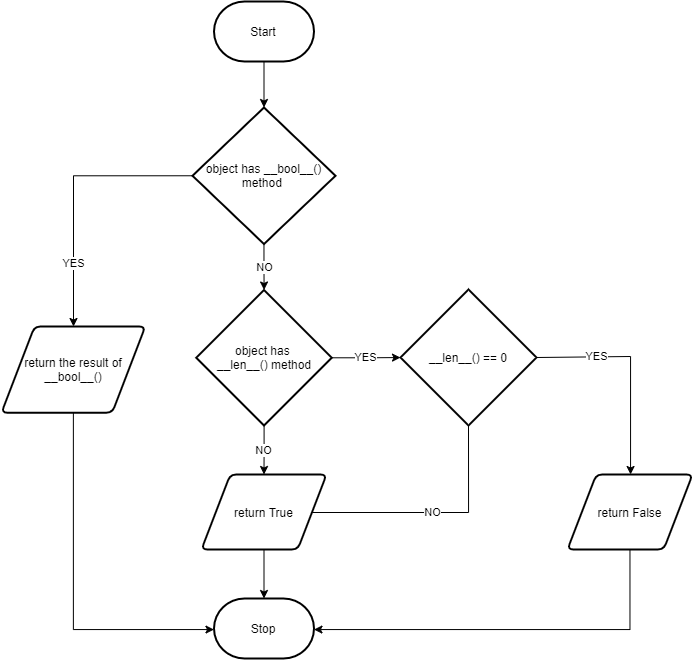
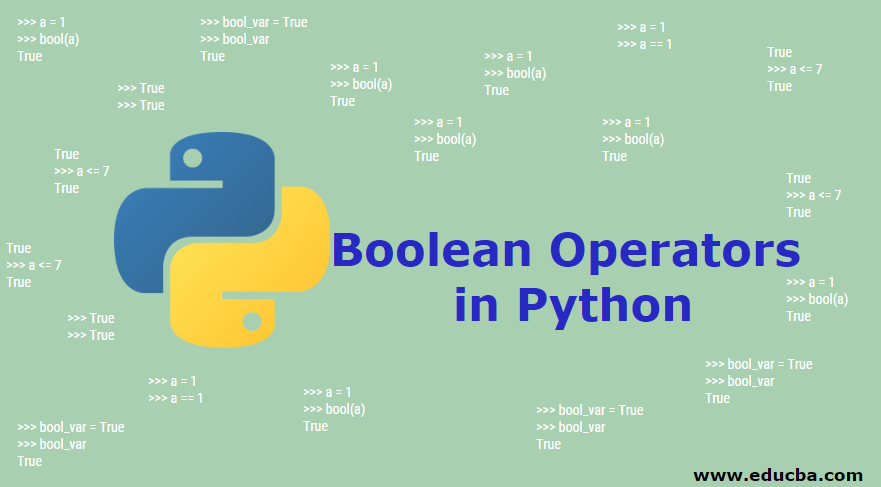


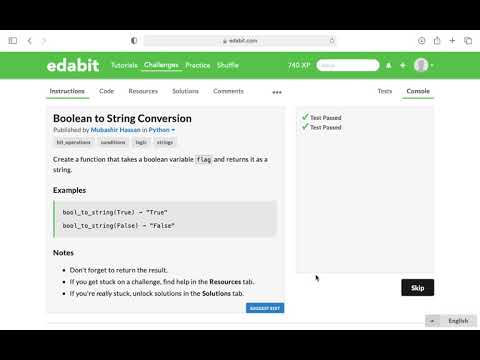
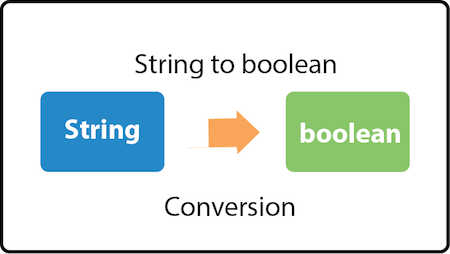
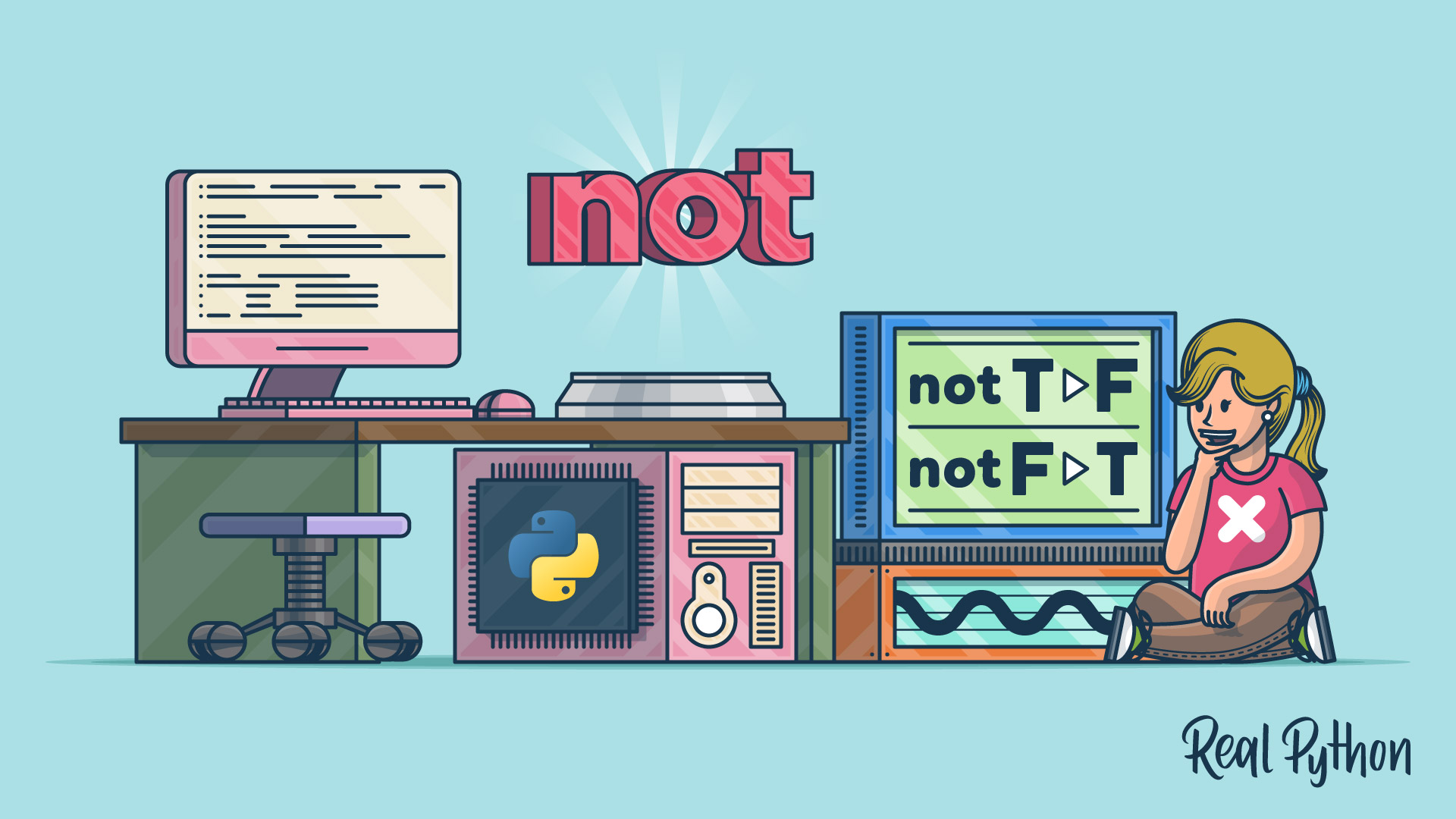

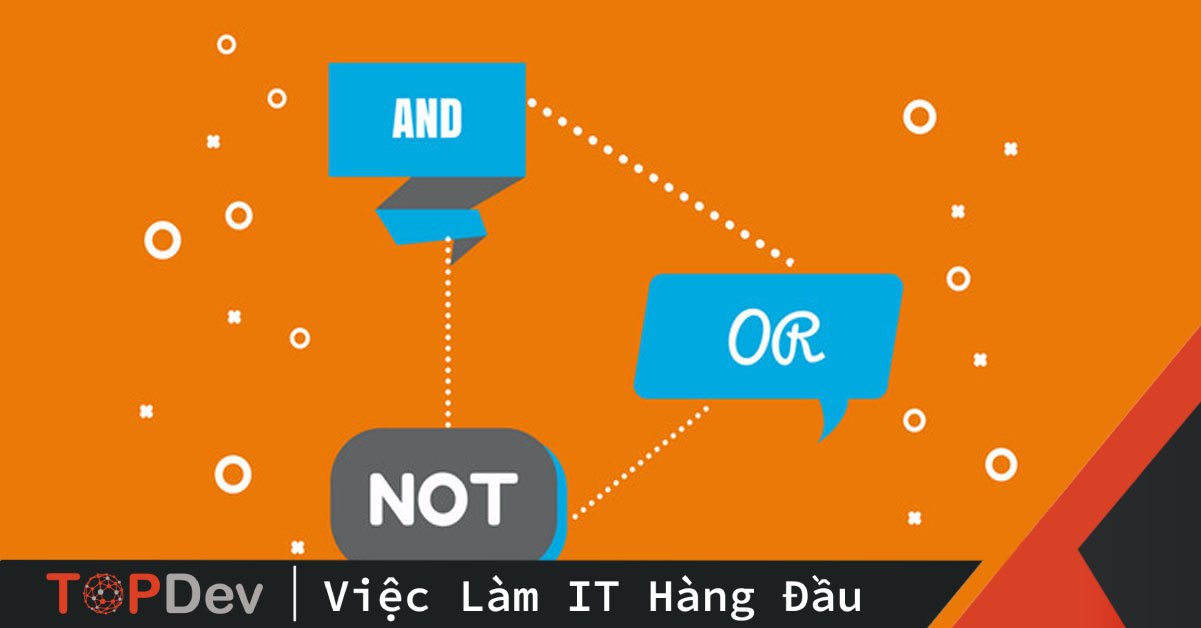
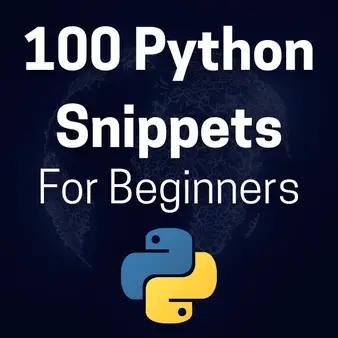
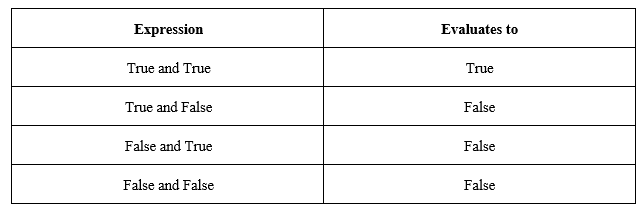
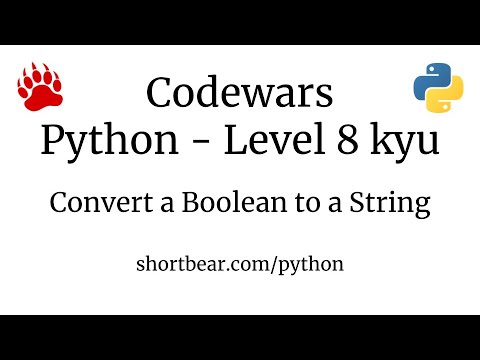
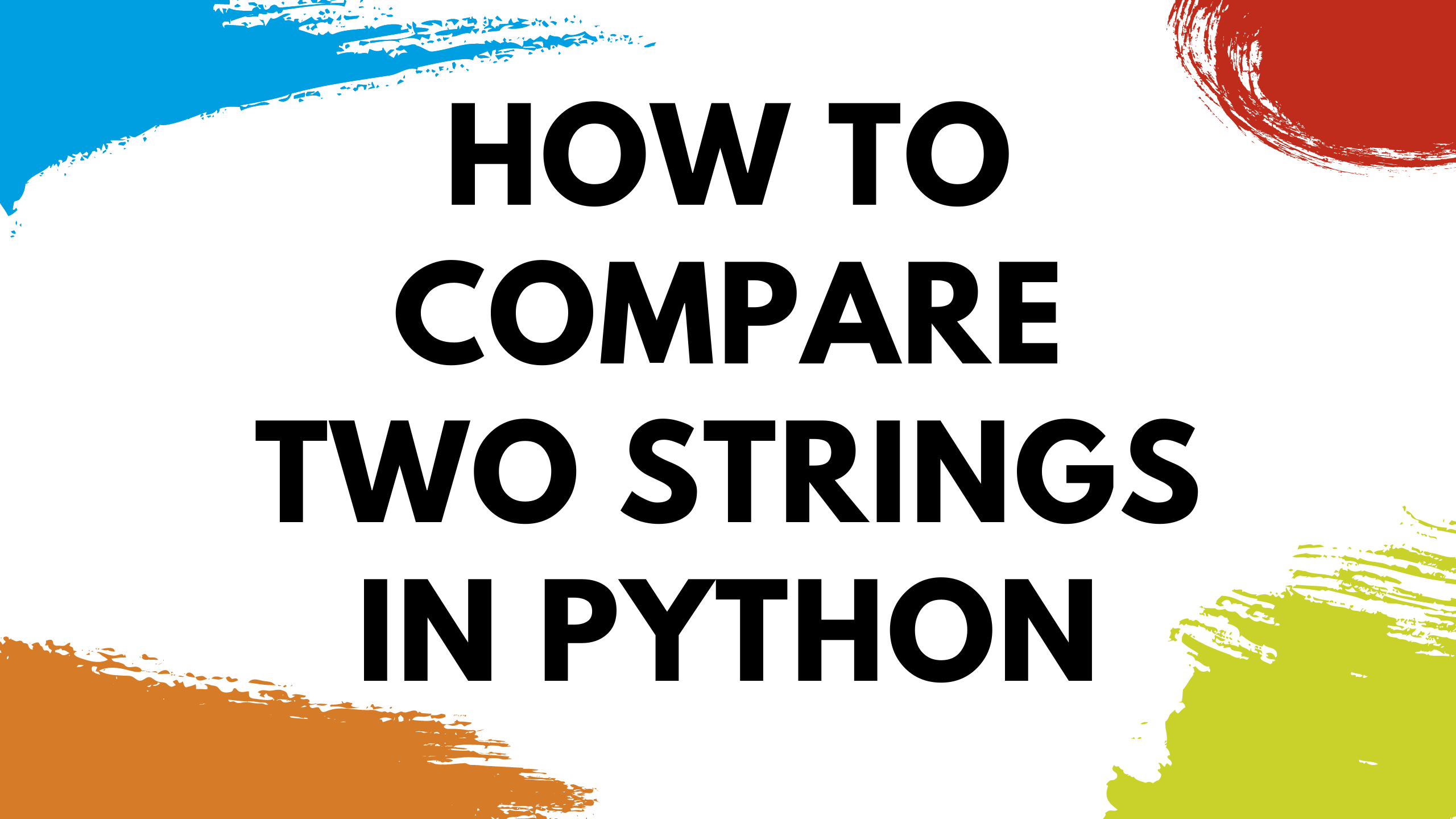
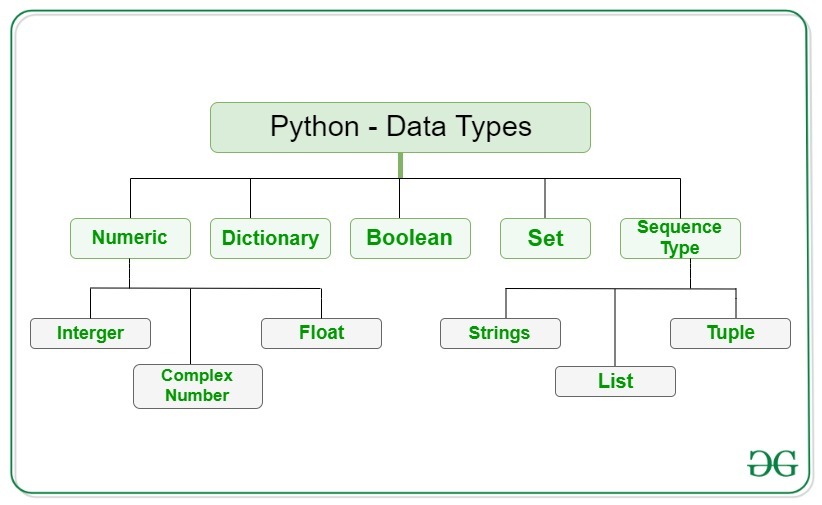


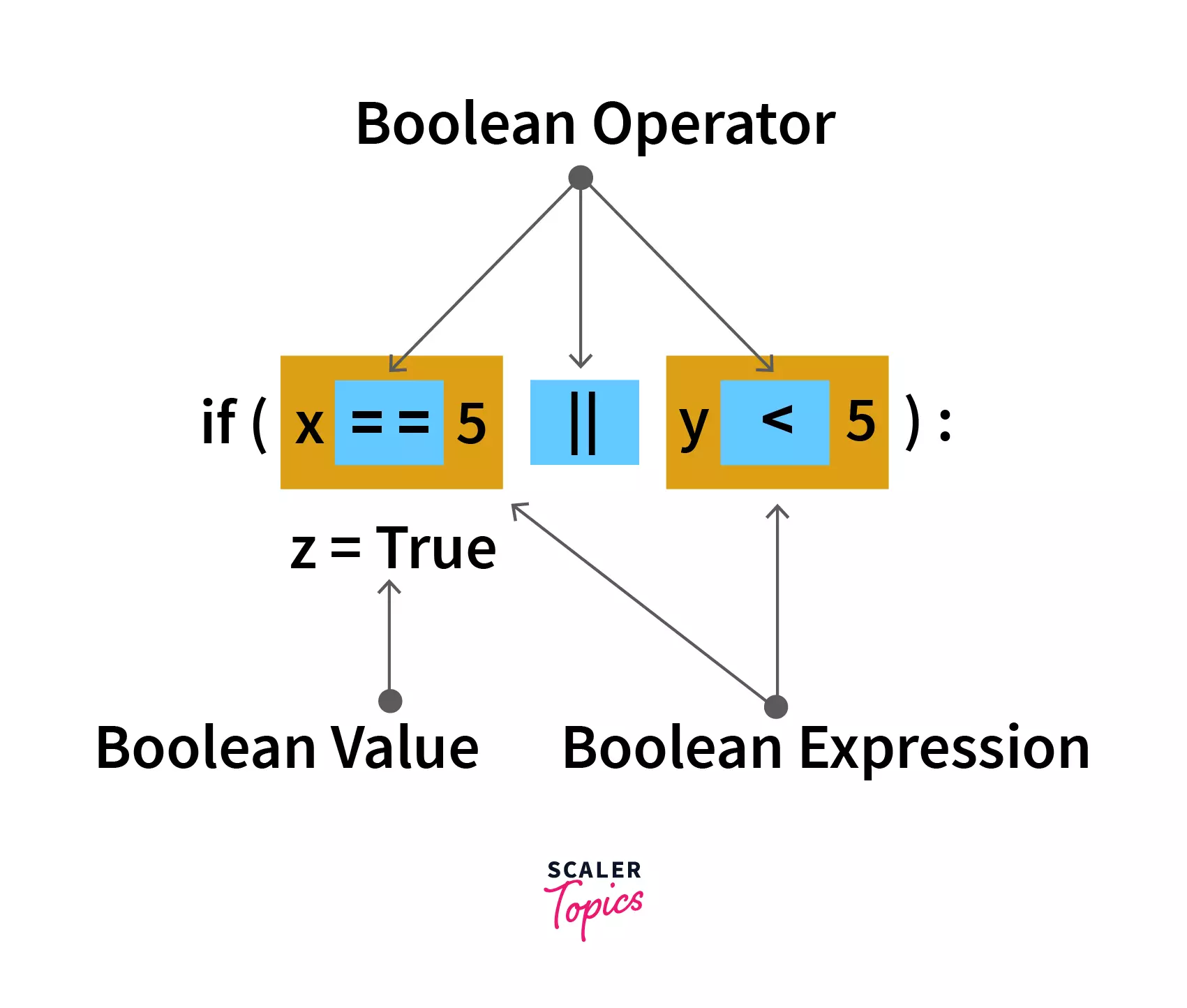
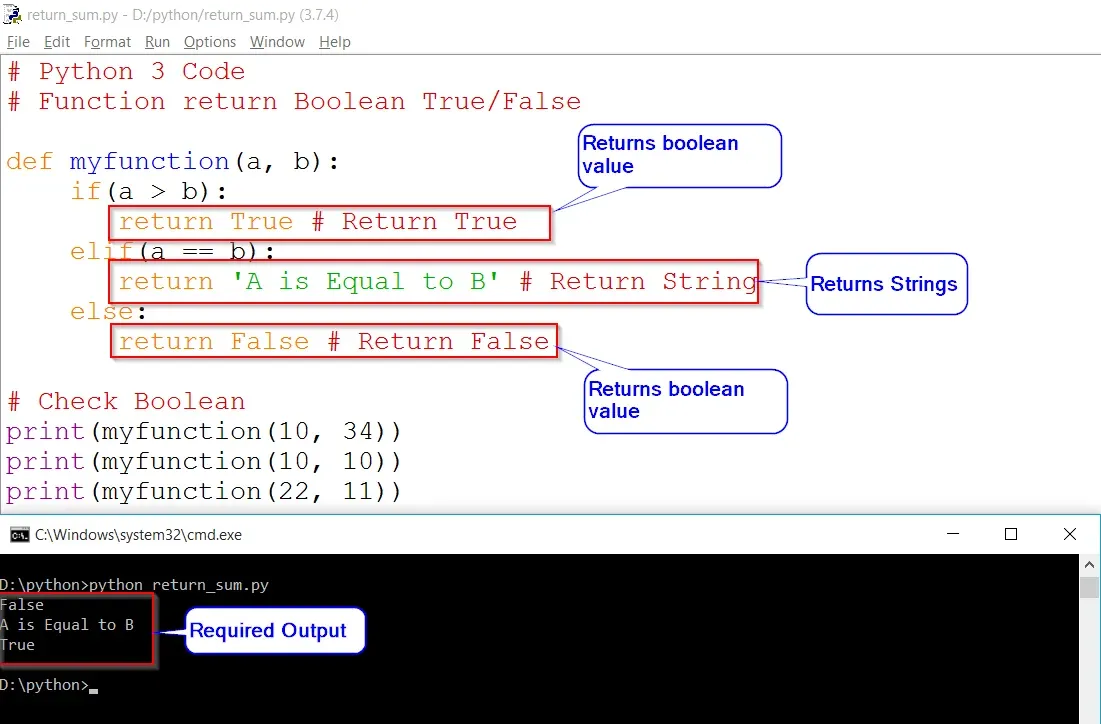
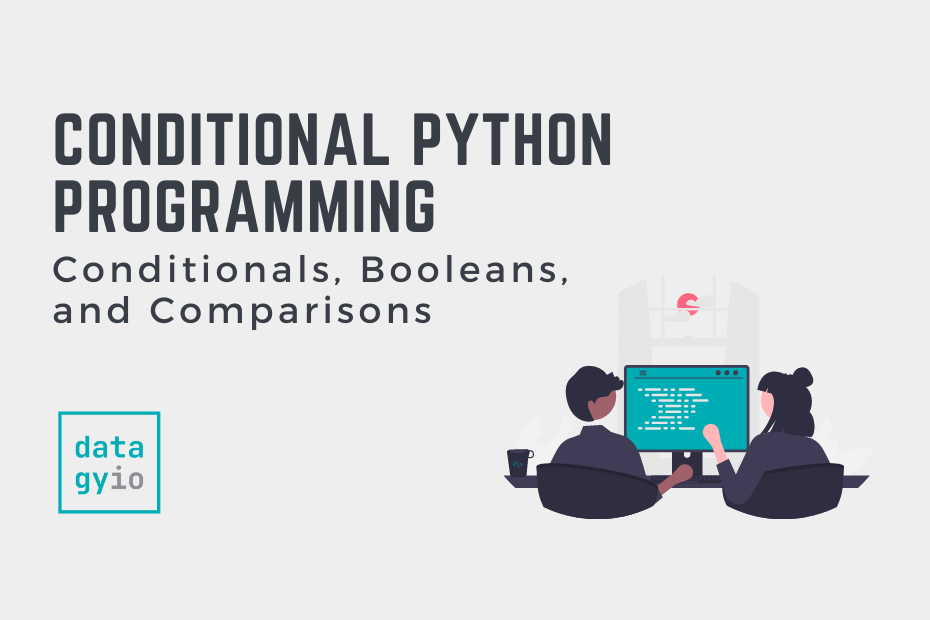

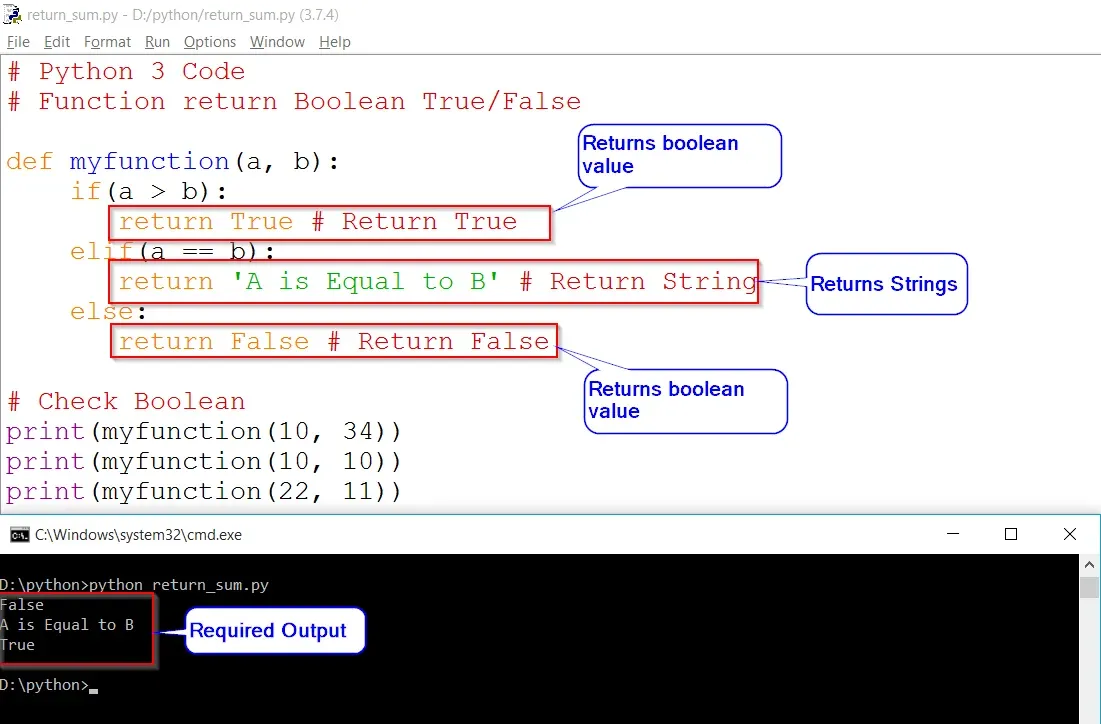
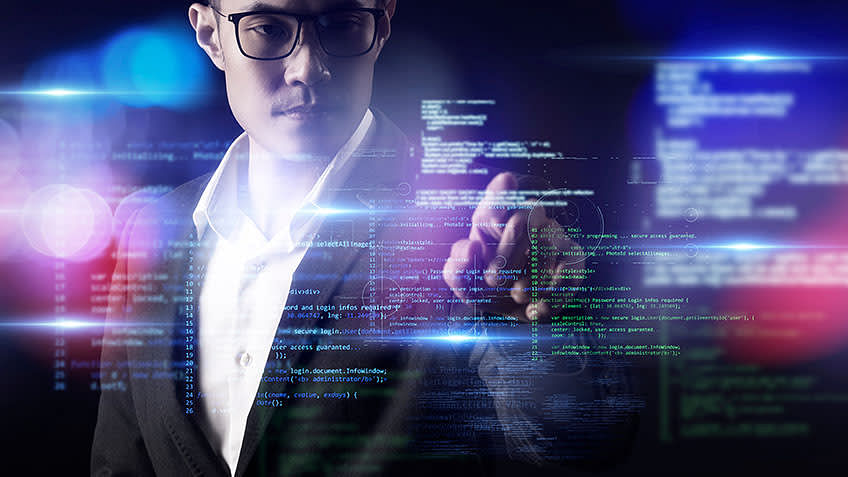

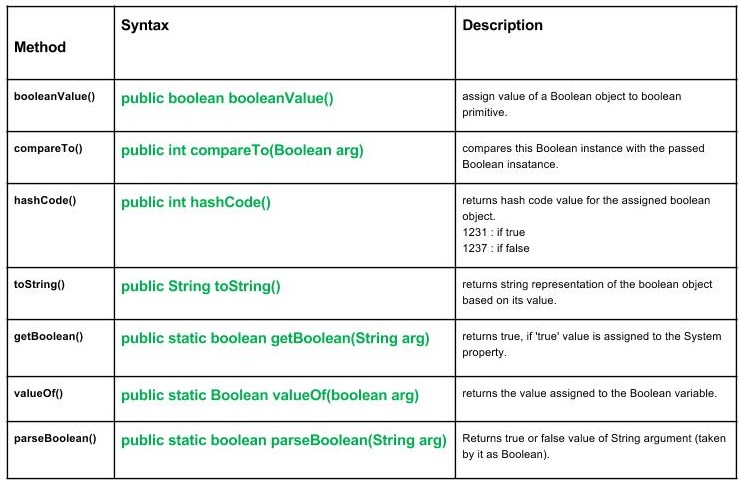
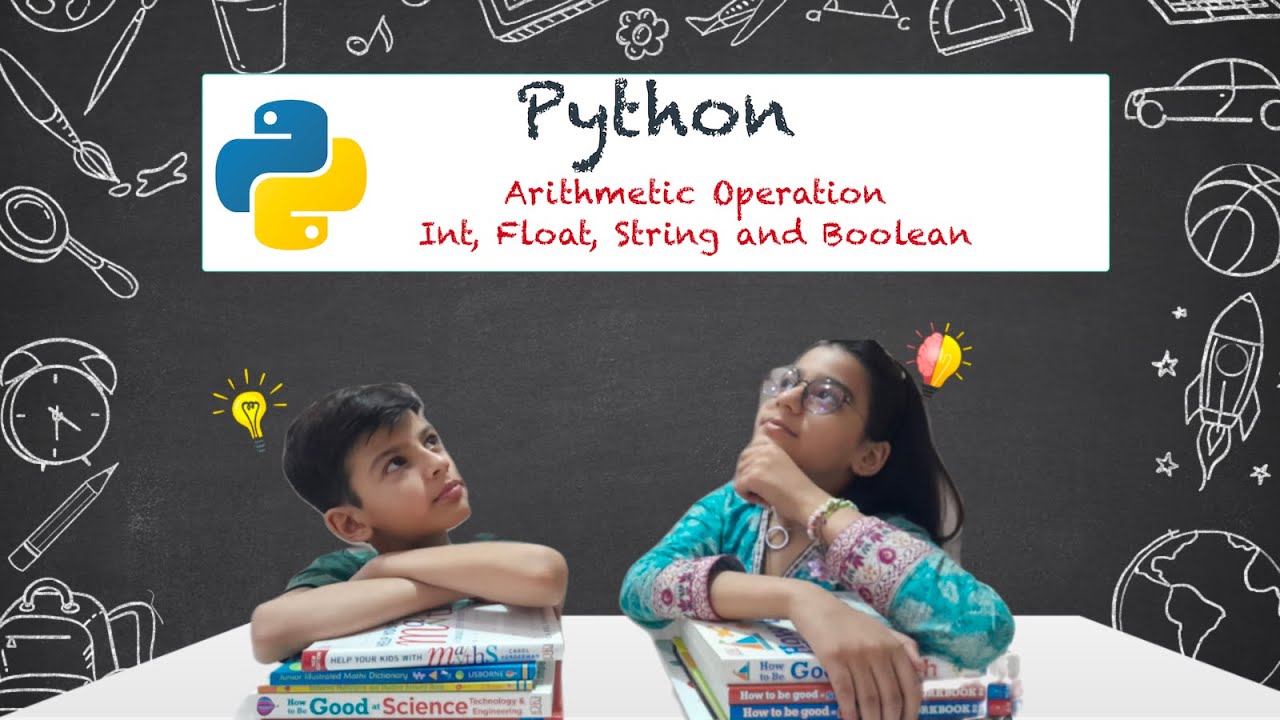
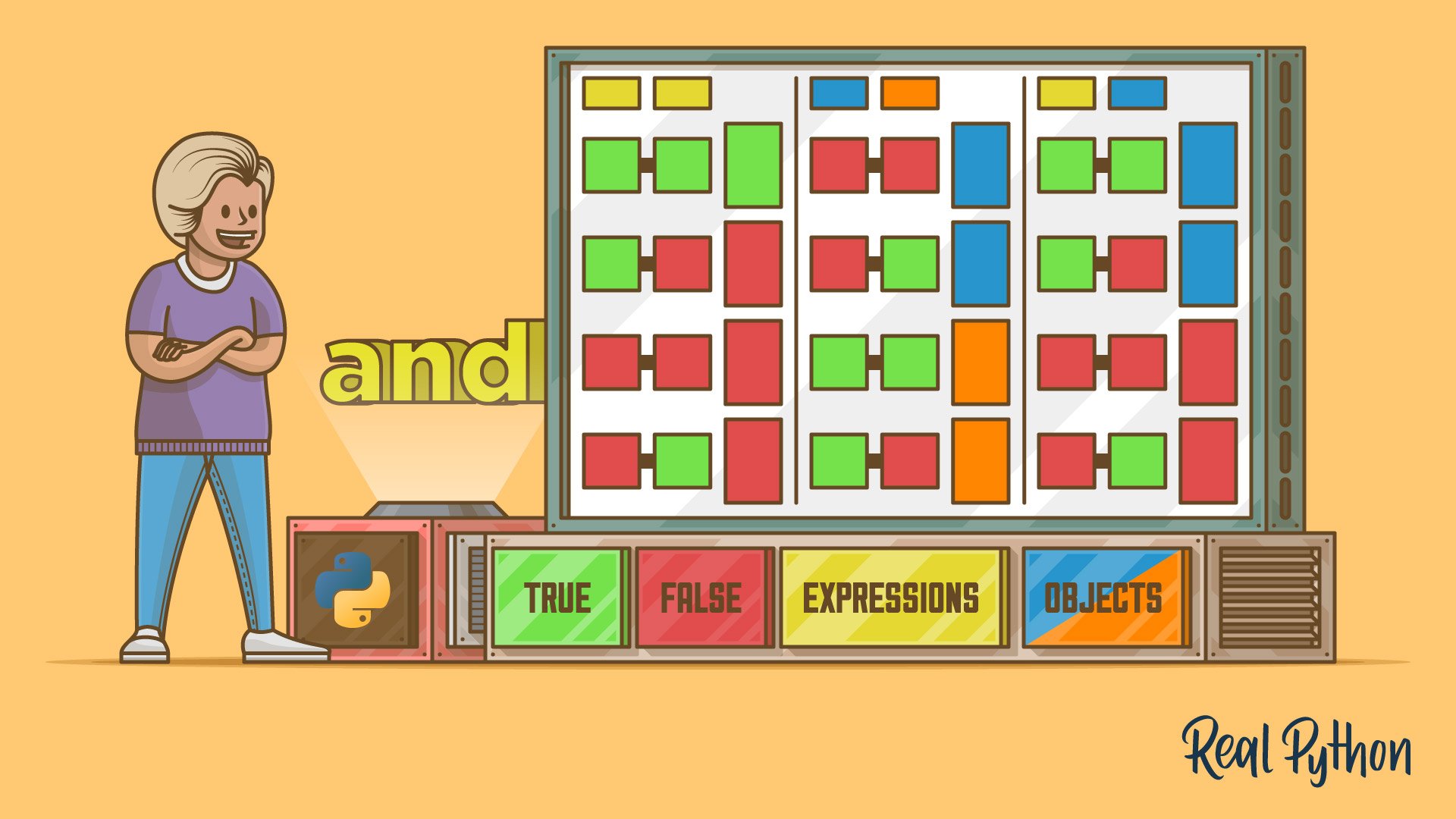



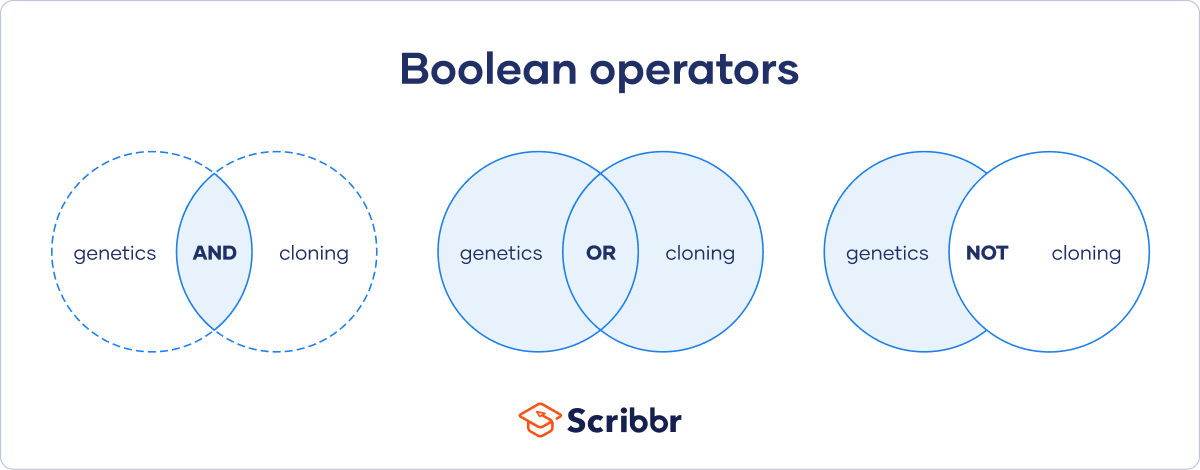


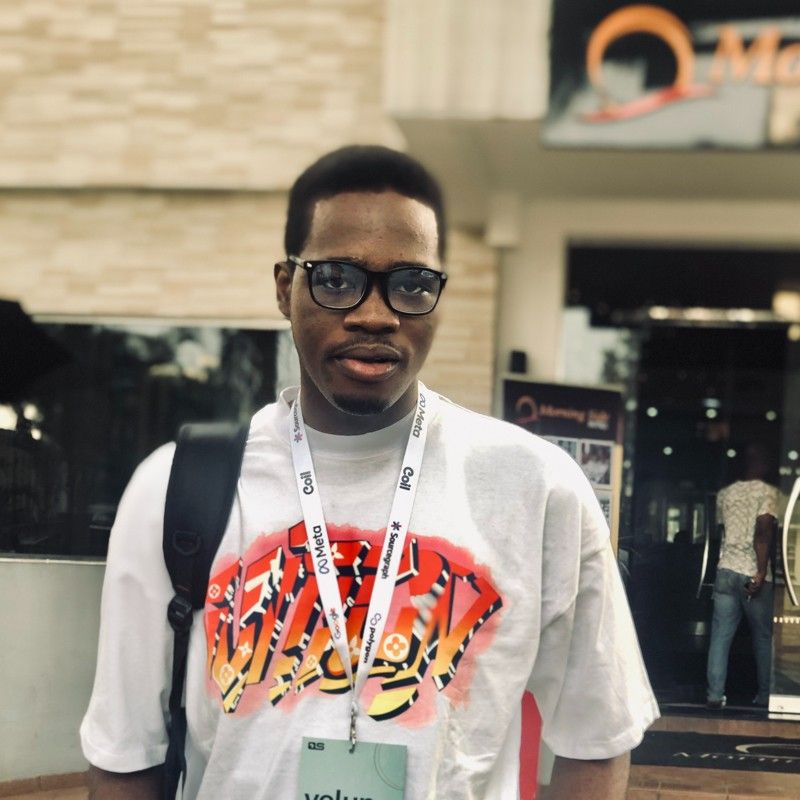
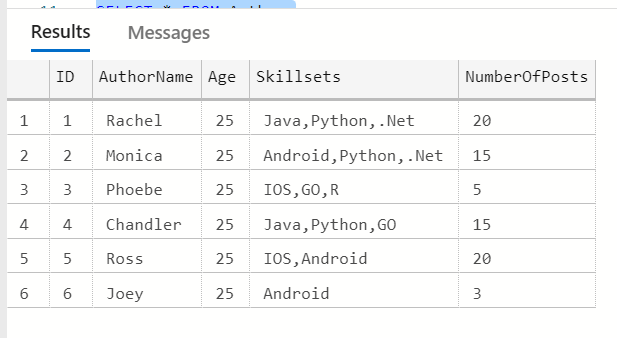

Article link: python string to boolean.
Learn more about the topic python string to boolean.
- Converting from a string to boolean in Python – Stack Overflow
- Convert String to Boolean in Python: A step-by-step guide
- Python – Convert String Truth values to Boolean
- How to convert string to boolean in Python – sebhastian
- Convert String to Boolean in Python | Delft Stack
- Converting from a string to boolean in Python – W3docs
- How to convert a string to a boolean in Python – Adam Smith
- How to convert a string to a boolean in Python? – thisPointer
- How to Convert String to Bool in Python? – Shiksha Online
- how to convert a string to boolean in python – Entechin
See more: https://nhanvietluanvan.com/luat-hoc