Python Writing List To File
Writing lists to a file is a common task in many programming projects. Whether you need to store data for future retrieval or share information with others, Python provides a simple and efficient way to accomplish this task. In this article, we will explore the basic concepts of writing lists to a file, various scenarios of writing different types of lists, and how to handle errors and exceptions along the way. Let’s dive in!
1. Basic Concepts of Writing Lists to a File
Before we start exploring the different scenarios, let’s understand the basic concepts of writing lists to a file in Python. Simply put, writing a list to a file means saving the contents of the list onto a disk, allowing it to be accessed later. A file can be in various formats, such as a plain text file (.txt), a comma-separated values file (.csv), or even a binary file (.dat).
When writing a list to a file, each element of the list is written as a separate line or row in the file. This helps in maintaining the structure and readability of the data. Depending on the type of data stored in the list, different methods are used to write it to a file. Now, let’s explore each scenario in detail.
2. Opening a File in Python for Writing
The first step to writing a list to a file is opening the file. In Python, you can open a file using the `open()` function. This function takes two arguments: the file name/path and the mode in which to open the file. To write data to a file, we need to open it in “write” mode, denoted by the character `’w’`.
Here’s an example code snippet to open a file in write mode:
“`python
file = open(“data.txt”, “w”)
“`
In this example, we open a file named “data.txt” in write mode. If the file does not exist, Python will create a new file with the specified name. However, if the file already exists, its previous content will be overwritten.
3. Writing a List of Strings to a File
One of the most common scenarios is writing a list of strings to a file. Python provides an easy way to write each string in the list as a separate line in the file using the `write()` method.
Here’s an example code snippet that demonstrates writing a list of strings to a file:
“`python
data = [“apple”, “banana”, “cherry”, “date”]
with open(“fruits.txt”, “w”) as file:
for item in data:
file.write(item + “\n”)
“`
In this example, the list `data` contains four strings. We open a file named “fruits.txt” in write mode using a `with` statement, which automatically handles closing the file after writing. Then, we iterate over each item in the list and write it to the file using the `write()` method. The `”\n”` character creates a new line after each string, maintaining the structure.
4. Writing a List of Numeric Values to a File
If you have a list containing numeric values, you can convert them to strings using the `str()` function before writing them to a file. This ensures that the values are written as human-readable text.
Here’s an example code snippet that demonstrates writing a list of numeric values to a file:
“`python
data = [1, 2, 3, 4, 5]
with open(“numbers.txt”, “w”) as file:
for item in data:
file.write(str(item) + “\n”)
“`
In this example, the list `data` contains five numeric values. We use the `str()` function to convert each item to a string before writing it to the file. The resulting file, “numbers.txt,” will have each value on a separate line.
5. Writing a List of Lists to a File
In some cases, you may have a list of lists, where each inner list represents a row or record. To write this structured data to a file, you can use the `csv` module, which provides functionality to handle comma-separated values.
Here’s an example code snippet that demonstrates writing a list of lists to a CSV file:
“`python
import csv
data = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
with open(“matrix.csv”, “w”, newline=””) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
In this example, we import the `csv` module and create a list of lists named `data`. We open a file named “matrix.csv” in write mode and create a `csv.writer` object using the `writer()` method. Then, we use the `writerows()` method to write the entire list of lists to the file. The resulting CSV file will have each inner list as a separate row, with values separated by commas.
6. Writing a List of Dictionaries to a File
If you have a list of dictionaries, where each dictionary represents a record with key-value pairs, you can use the `csv` module’s `DictWriter` class to write the data to a CSV file.
Here’s an example code snippet that demonstrates writing a list of dictionaries to a CSV file:
“`python
import csv
data = [
{“Name”: “John”, “Age”: 25, “City”: “London”},
{“Name”: “Jane”, “Age”: 32, “City”: “New York”},
{“Name”: “Bob”, “Age”: 45, “City”: “Sydney”}
]
keys = data[0].keys()
with open(“records.csv”, “w”, newline=””) as file:
writer = csv.DictWriter(file, fieldnames=keys)
writer.writeheader()
writer.writerows(data)
“`
In this example, we import the `csv` module and create a list of dictionaries named `data`. We extract the keys from the first dictionary using `data[0].keys()` to set the fieldnames for the `DictWriter` object. We open a file named “records.csv” in write mode and create a `csv.DictWriter` object using the `DictWriter()` method. We write the header using the `writeheader()` method and then write the list of dictionaries using the `writerows()` method.
7. Handling Errors and Exceptions while Writing Lists to a File
While writing lists to a file, it’s essential to handle errors and exceptions gracefully to avoid unexpected program termination. One common source of errors is if the file is read-only or if the disk space is full. Python provides built-in mechanisms for handling such errors using the `try-except` block.
Here’s an example code snippet that demonstrates error handling while writing a list to a file:
“`python
try:
with open(“data.txt”, “w”) as file:
file.write(“Hello, World!”)
except IOError as e:
print(“An error occurred while writing the file:”, str(e))
“`
In this example, we wrap the file writing code inside a `try` block. If an error occurs during the file writing process, Python raises an `IOError` exception. We catch the exception using the `except` block and print an error message that includes the specific error message obtained from the exception.
FAQs:
Q: How do I write a list to a file in Python?
A: To write a list to a file in Python, you need to open the file in write mode using the `open()` function, then iterate over each item in the list and write it to the file using the `write()` method. Don’t forget to close the file after writing.
Q: How do I read and write a list to a file in Python?
A: To read and write a list to a file, you need to open the file in read mode or read-write mode using the appropriate flags. Read the contents of the file into a list using methods like `readlines()`, modify the list as needed, and then write it back to the file using the `write()` method.
Q: How do I save a list of numbers to a file in Python?
A: You can save a list of numbers to a file in Python by converting them to strings using the `str()` function and then writing each number as a separate line in the file using the `write()` method.
Q: How do I write an array to a file in Python?
A: To write an array to a file in Python, you can convert it to a list using the `tolist()` method, then apply the same techniques mentioned earlier for writing lists to a file.
Q: How do I write to a text file in Python?
A: To write to a text file in Python, you need to open the file in write mode using the `open()` function, then use the `write()` method to write the desired content to the file. Close the file after writing to ensure proper handling.
Q: How do I write a log to a text file in Python?
A: To write a log to a text file in Python, you can use the `logging` module. Configure the module to write logs to a file using a `FileHandler` and customize the log format if needed. Then, use the `logging` functions like `info()`, `warning()`, or `error()` to write log messages to the file.
Q: How do I write a list to a CSV file in Python?
A: To write a list to a CSV (comma-separated values) file in Python, you need to use the `csv` module. Open the file in write mode, create a `csv.writer` object, and use the `writerows()` method to write the list to the file.
Q: How do I save a list in Python?
A: In Python, you can save a list by writing it to a file using the techniques discussed in this article. By saving a list to a file, you can preserve its contents for future use or share it with other programs or individuals.
How To Write To A Text .Txt File In Python! Processing Lists, And Outputting Data!
How To Create Text File In Python?
Python is a versatile programming language that offers a wide range of functionalities, including the ability to create, read, and modify text files. Text files are simple yet powerful tools for storing and organizing data, making them an essential part of any programming project. In this article, we will explore various ways to create text files in Python, along with some common questions and answers related to this topic.
Understanding Text Files in Python:
Before diving into the specifics of creating text files in Python, let’s briefly discuss what text files are and why they are useful. A text file is a plain and simple file that contains unformatted text without any specific structure or styling. It typically consists of human-readable characters and can be opened and modified using any text editor. Text files are widely used for storing configuration settings, log files, data dumps, and other textual information.
Creating a Text File in Python:
Python provides multiple ways to create text files, and we will explore a few of them in this section.
1. Using the open() Function:
The most common method to create a text file in Python is by using the `open()` function. This function is versatile and allows you to perform various file operations, including creating, reading, and writing files. To create a text file using the `open()` function, you need to specify the filename and the mode as `w` (write). For example:
“`python
file = open(“myfile.txt”, “w”)
# Do something with the file
file.close()
“`
In the example above, we created a text file called “myfile.txt” in write mode. After performing the necessary operations, it is important to close the file using the `close()` function to release any system resources associated with it.
2. Using the with Statement:
The `with` statement is a more elegant way to create text files in Python. It automatically handles opening and closing the file, ensuring proper resource management. Here’s an example of creating a text file using the `with` statement:
“`python
with open(“myfile.txt”, “w”) as file:
# Do something with the file
pass
“`
In this example, the file will be automatically closed at the end of the `with` block.
FAQs:
Q: Can I create a text file in a specific directory?
A: Yes, you can specify the path of the text file while providing the filename. For example, to create a file named “myfile.txt” in the directory “C:/folder/”, you would use `open(“C:/folder/myfile.txt”, “w”)`. If the directory doesn’t exist, Python will raise an error.
Q: How can I check if a text file already exists before creating it?
A: You can use the `os.path.exists()` function to check if a file exists at a specified path. For example:
“`python
import os
if not os.path.exists(“myfile.txt”):
with open(“myfile.txt”, “w”) as file:
# Do something with the file
pass
“`
Q: What if I want to append data to an existing text file instead of overwriting it?
A: To append data to an existing file, you can use the mode `a` (append) instead of `w`. For example:
“`python
with open(“myfile.txt”, “a”) as file:
file.write(“New Data\n”)
“`
The `write()` function is used to append new data to the file.
Q: Can I create a text file with a specific encoding?
A: By default, Python uses the system’s default encoding for creating text files. However, you can specify a specific encoding by providing the `encoding` argument to the `open()` function. For example:
“`python
with open(“myfile.txt”, “w”, encoding=”utf-8″) as file:
# Do something with the file
pass
“`
In this example, we used the UTF-8 encoding.
In conclusion, creating a text file in Python allows you to store and organize data efficiently. Whether it’s for logging information, storing user preferences, or any other purpose, Python provides intuitive ways to create and manipulate text files. By understanding the different methods presented in this article, you can choose the approach that best suits your needs and utilize the versatility and simplicity of text file handling in Python.
Keywords searched by users: python writing list to file Write list to file Python, Read and write list to file Python, Python save list of numbers to file, Write array to file Python, Python write to txt file, Python write log to text file, Write list to CSV file Python, Save list Python
Categories: Top 82 Python Writing List To File
See more here: nhanvietluanvan.com
Write List To File Python
Python, as a versatile and powerful programming language, offers a plethora of functionalities to efficiently handle data. One such task frequently encountered in programming is saving a list of data to a file for future use. In this article, we will explore various methods and techniques to write a list to a file in Python, ensuring that you can do so effortlessly for any project. So, let’s dive in!
Methods to Write a List to a File:
1. Using the `write()` method:
One of the simplest approaches to write a list to a file is by using the built-in `write()` method. Here’s an example showcasing this method:
“`python
data = [“apple”, “banana”, “orange”]
with open(“output.txt”, “w”) as file:
for item in data:
file.write(item + “\n”)
“`
In this example, we create a list called `data` with three elements. We then open a file called “output.txt” in write mode (“w”) using the `open()` function. Next, we iterate over each element of the list and write it to the file, appending a newline character (“\n”) after each item. Finally, we close the file using the `with` statement.
2. Utilizing the `writelines()` method:
Another approach to write a list to a file is by using the `writelines()` method. This method accepts an iterable of strings and writes them to the file. Here’s an example:
“`python
data = [“apple”, “banana”, “orange”]
with open(“output.txt”, “w”) as file:
file.writelines(item + “\n” for item in data)
“`
In this example, we first create the list `data`. Then, using the `with` statement, we open a file named “output.txt” in write mode (“w”). The `writelines()` method is then applied to the file, with the generator expression `item + “\n” for item in data` passed as an argument. This expression appends a newline character to each item before writing it to the file.
3. Applying the `json` module:
If your list contains complex or nested data structures, writing it to a file in a readable and portable format can be accomplished using the `json` module. This module allows us to save Python objects as JSON files. Here’s an example:
“`python
import json
data = [“apple”, “banana”, “orange”]
with open(“output.json”, “w”) as file:
json.dump(data, file)
“`
In this example, we import the `json` module and create a list called `data`. Then, using the `with` statement, we open a file called “output.json” in write mode (“w”). The `json.dump()` method is used to write the list to the file in JSON format, resulting in a more structured representation of the data.
Frequently Asked Questions (FAQs):
Q1: How can I append a list to an existing file?
A: To append a list to an existing file, open the file in append mode (“a”) instead of write mode (“w”). The following code snippet demonstrates this:
“`python
data = [“apple”, “banana”, “orange”]
with open(“existing_file.txt”, “a”) as file:
file.writelines(item + “\n” for item in data)
“`
Q2: How can I write a list of integers to a file?
A: Writing a list of integers follows the same principles as writing any other list. You can utilize either the `write()` or `writelines()` methods to write the integer values to the file. Here’s an example:
“`python
data = [1, 2, 3, 4, 5]
with open(“output.txt”, “w”) as file:
file.writelines(str(item) + “\n” for item in data)
“`
Q3: How can I write a list of lists to a file?
A: Writing a list of lists requires a similar approach. You can iterate over the outer list and, within that loop, iterate over the inner lists to write their contents to the file. Here’s an example:
“`python
data = [[1, 2, 3], [“apple”, “banana”, “orange”], [True, False]]
with open(“output.txt”, “w”) as file:
for sublist in data:
file.writelines(str(item) + “\n” for item in sublist)
“`
Wrapping Up:
Writing a list to a file is a fundamental skill that can greatly enhance your ability to handle data in Python. By utilizing various methods such as `write()`, `writelines()`, or even the `json` module, you can efficiently store data for future use or analysis. Remember to choose the suitable approach based on your requirements, and keep exploring the vast capabilities of Python for even more data-handling tasks.
Read And Write List To File Python
Python is a widely-used programming language known for its simplicity and versatility. One of its key strengths lies in its ability to read and write data to files effortlessly. In this article, we will explore how to read and write list data to a file using Python, and provide a comprehensive guide for users of all proficiency levels.
Part 1: Reading List Data from a File
To begin with, let’s understand how to read list data from a file in Python. The process involves opening a file, reading its contents, and converting the data into a list format. Here’s a step-by-step breakdown of the process:
Step 1: Open the file
Use the built-in `open()` function to open the file in the desired mode (read, write, append, etc.). For reading, specify the mode as `”r”`.
Step 2: Read the file contents
Once the file is opened, use the `read()` or `readlines()` method to access the data. The `read()` method reads the entire file as a single string, while `readlines()` splits the content into individual lines as a list.
Step 3: Convert the data into a list
If you used `readlines()`, you already have the data as a list, ready to be utilized within your program. However, if you used `read()`, you need to further process the string to separate values into a list format. You can utilize the `split()` method to split the string and convert it into a list.
Part 2: Writing List Data to a File
In this section, we will look at how to write list data to a file. Writing list data involves creating a file, converting the list elements into a string, and storing the data in the file. Below are the steps involved:
Step 1: Open the file
Similar to reading, start by opening the file using the `open()` function, this time specifying the mode as `”w”` (write).
Step 2: Prepare the list data
Before writing to the file, ensure that the list data is in a format that can be easily stored. This typically involves converting the list elements into a single string. You can use methods like `join()` or a loop to achieve this.
Step 3: Write to file
Now that the data is ready, use the `write()` method to write the string to the opened file. Be mindful to include newline characters (`\n`) if you want each list element to be on a separate line.
Part 3: FAQs
Q1: How can I append list data to an existing file?
A: To append list data to an existing file, open the file using the `”a”` mode instead of `”w”`. This ensures that the data is added to the end of the file without overwriting the existing content.
Q2: Can I read and write other data structures, such as dictionaries or sets?
A: Absolutely! Python provides various methods and strategies to handle different data structures. For dictionaries, you can use the `json` module to convert them to JSON (JavaScript Object Notation) format and store them in a file. Sets can also be converted into lists and stored using the techniques described earlier.
Q3: How can I handle large data sets efficiently when reading or writing to files?
A: When working with large data sets, it is advisable to read or write the data in chunks rather than all at once. For instance, when reading, use a loop to iterate over lines of the file instead of using `read()` to extract the entire content. Similarly, when writing, process and write the data in smaller portions to avoid memory issues.
Q4: Can I read and write multiple lists to a single file?
A: Yes, you can read and write multiple lists to a single file. To write multiple lists, convert each list into a separate string with appropriate formatting (e.g., using separators like commas or tabs). While reading, retrieve the strings from the file and convert them back into lists using the reverse process.
Conclusion
In this article, we explored the process of reading and writing list data to a file in Python. We discussed the step-by-step procedure for both tasks, providing insights for beginners and advanced users alike. Additionally, we answered some frequently asked questions regarding file manipulation. By following the guidelines and understanding the concepts presented here, you will be equipped to handle list data efficiently in your Python programs.
Images related to the topic python writing list to file
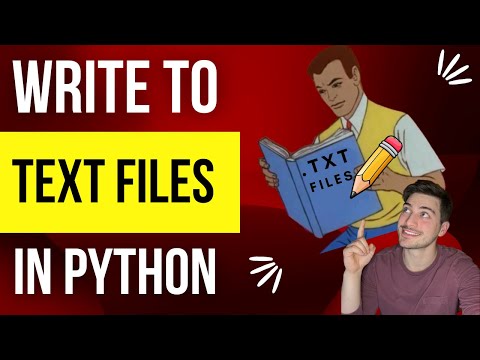
Found 8 images related to python writing list to file theme
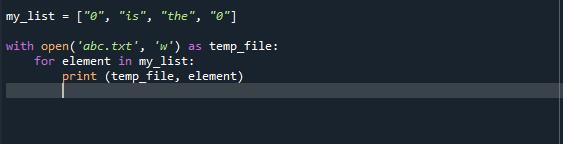
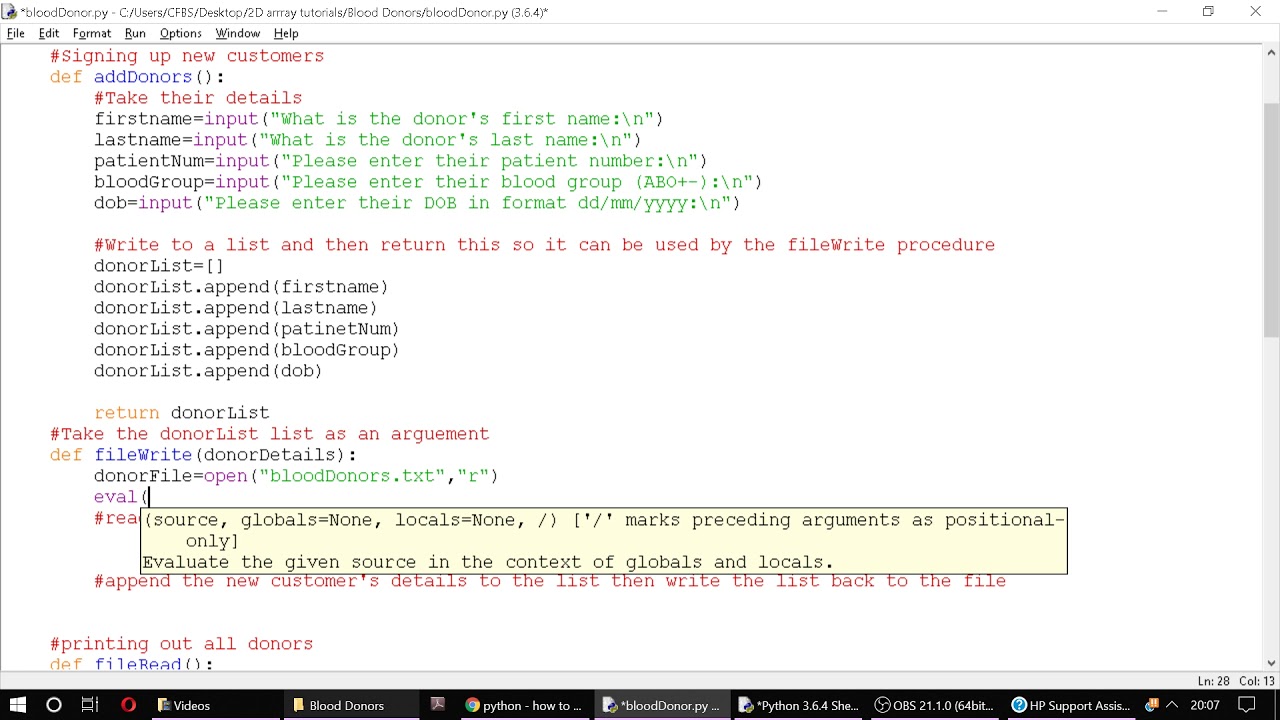
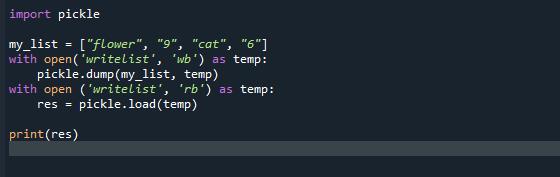
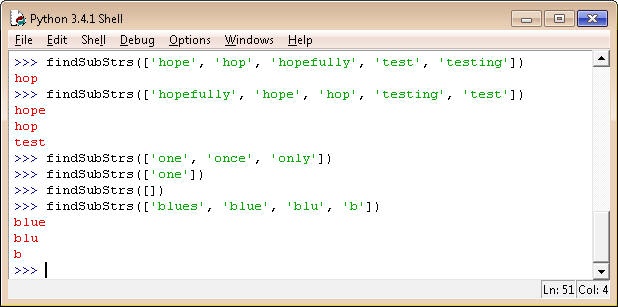
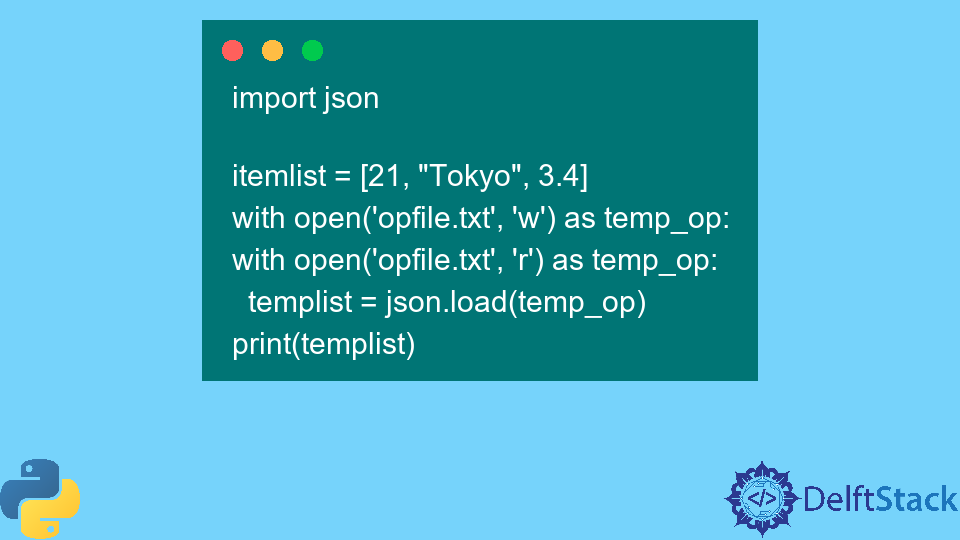
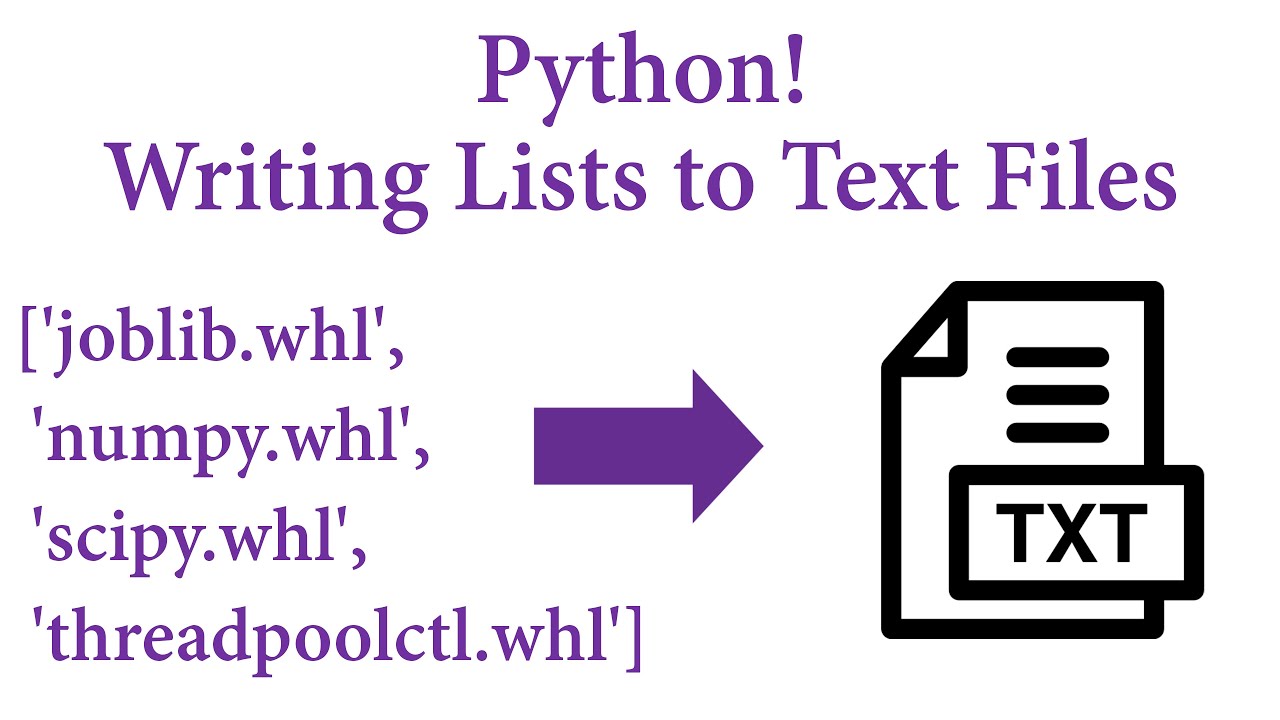

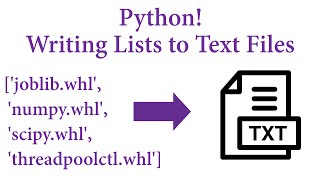
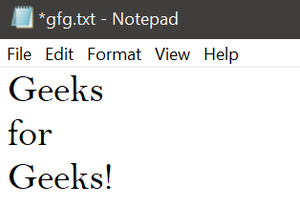
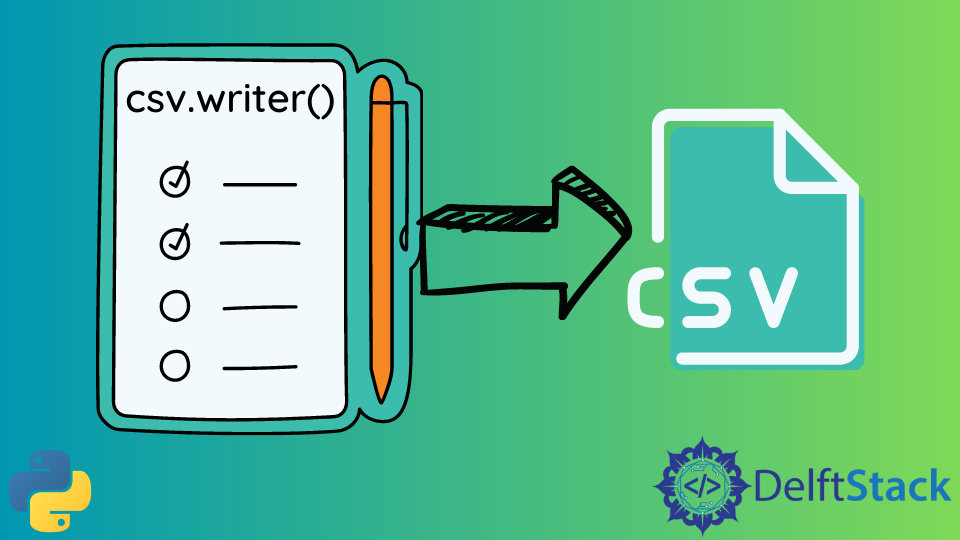
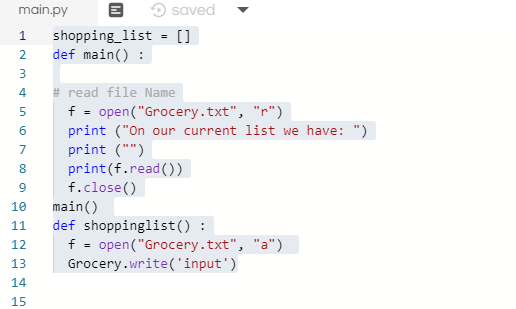

![File Handling in Python [Complete Series] – PYnative File Handling In Python [Complete Series] – Pynative](https://pynative.com/wp-content/uploads/2021/07/file_handling_in_python.png)


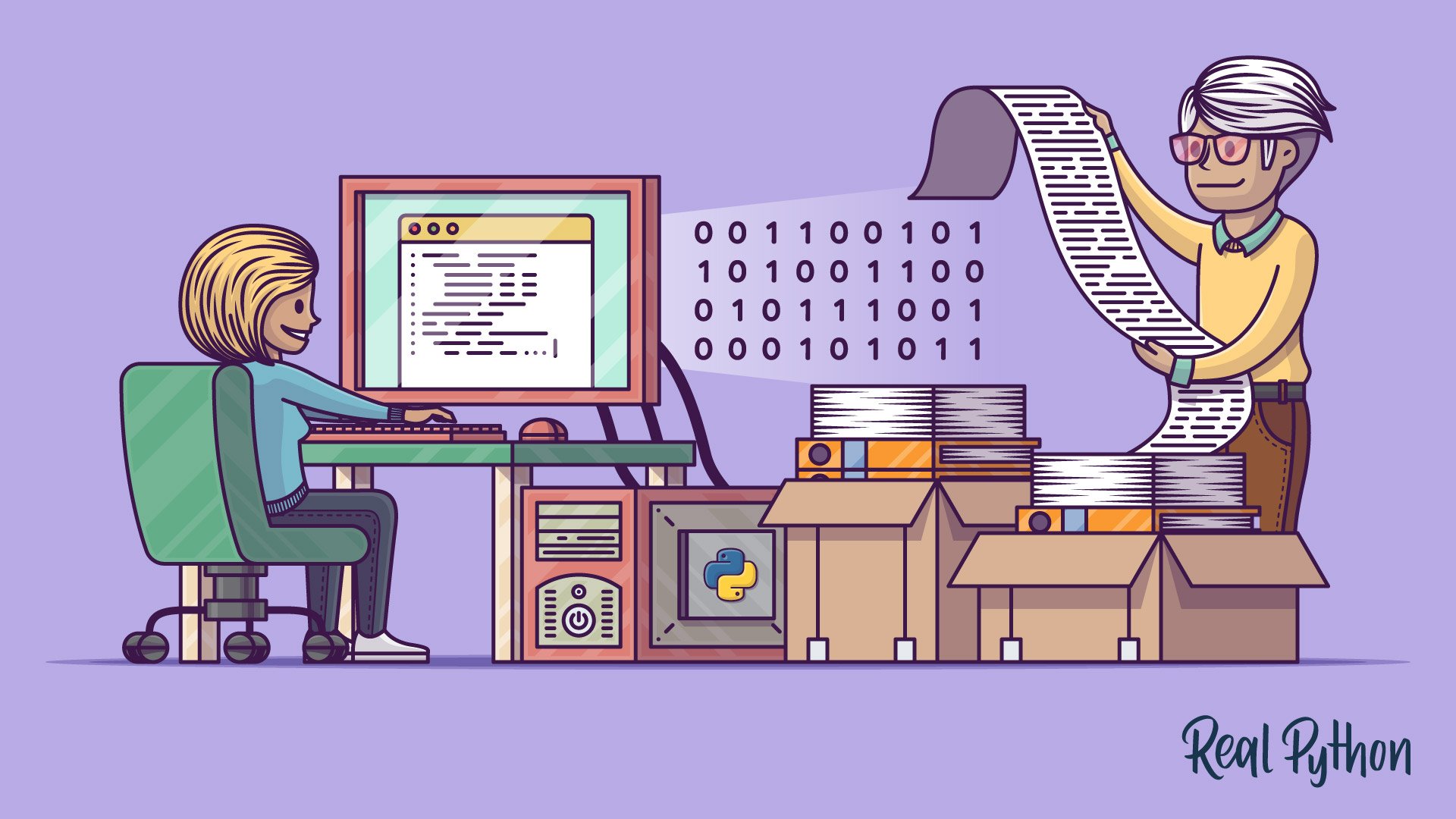
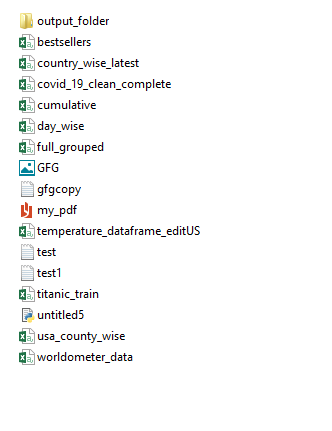
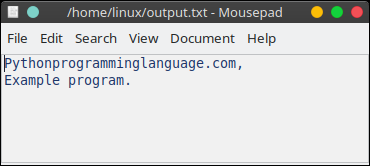
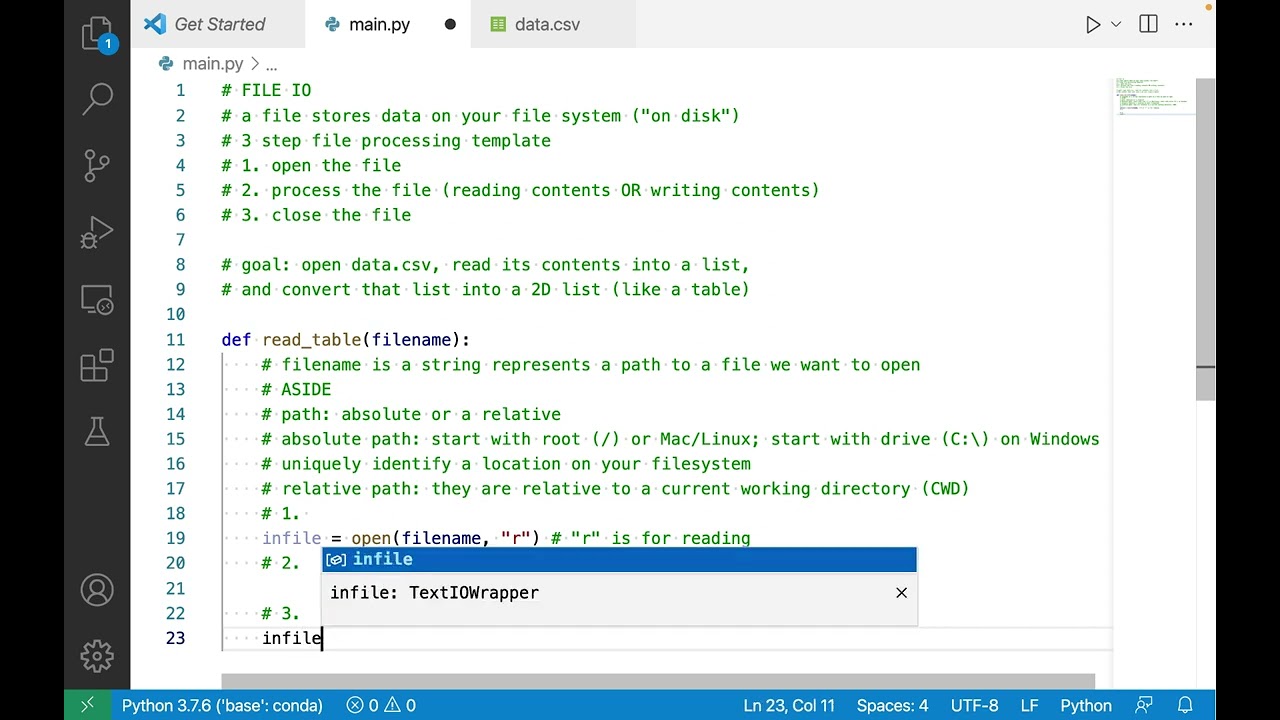

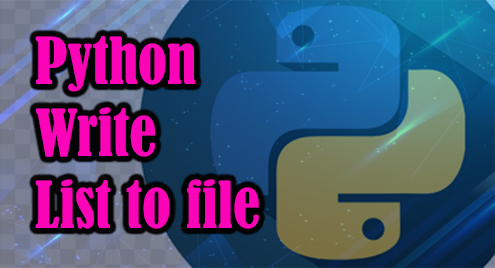


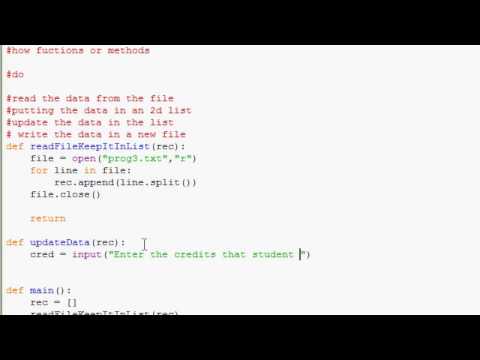
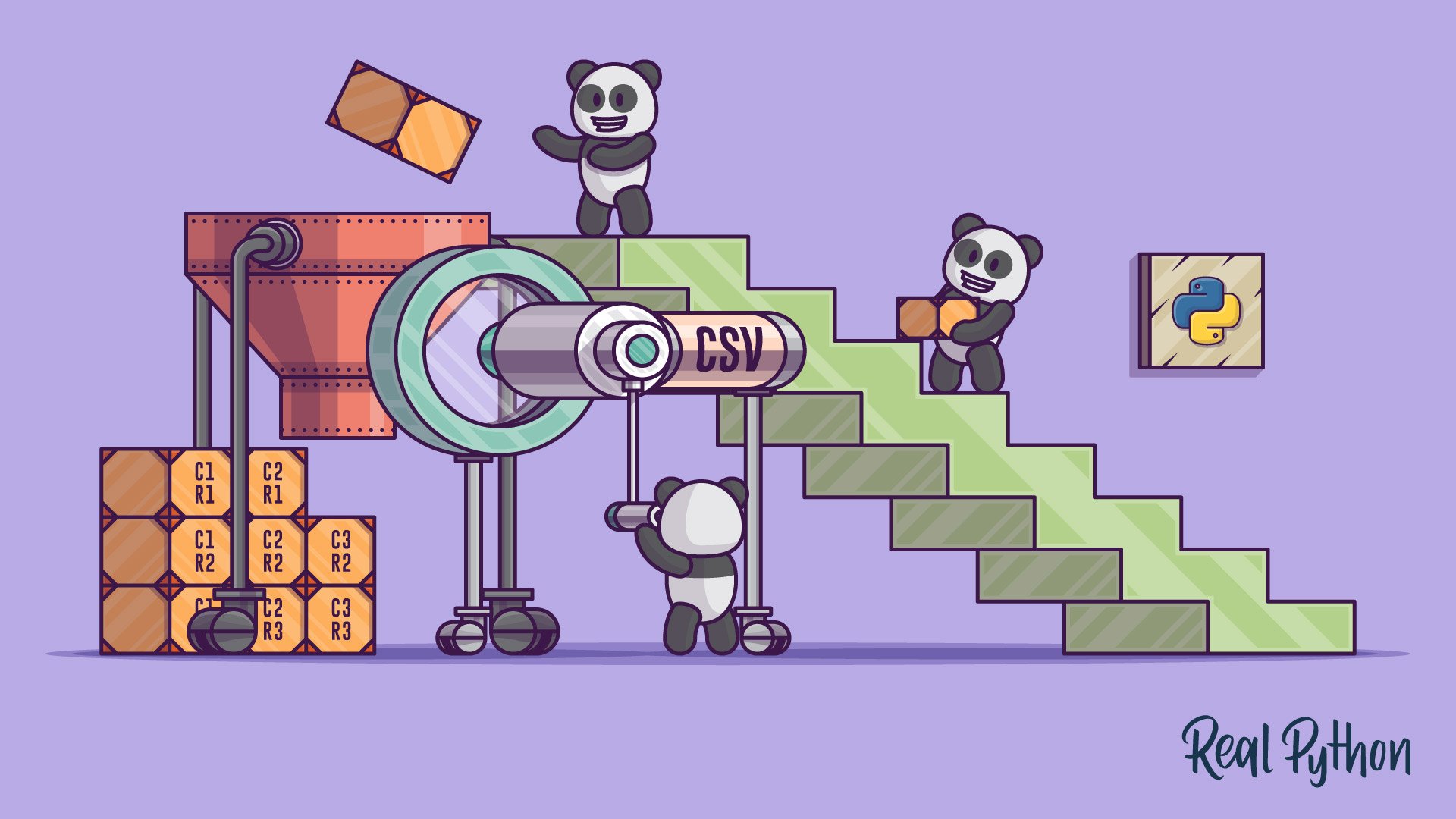
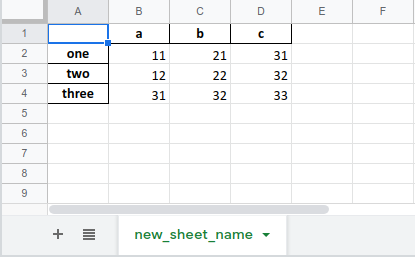
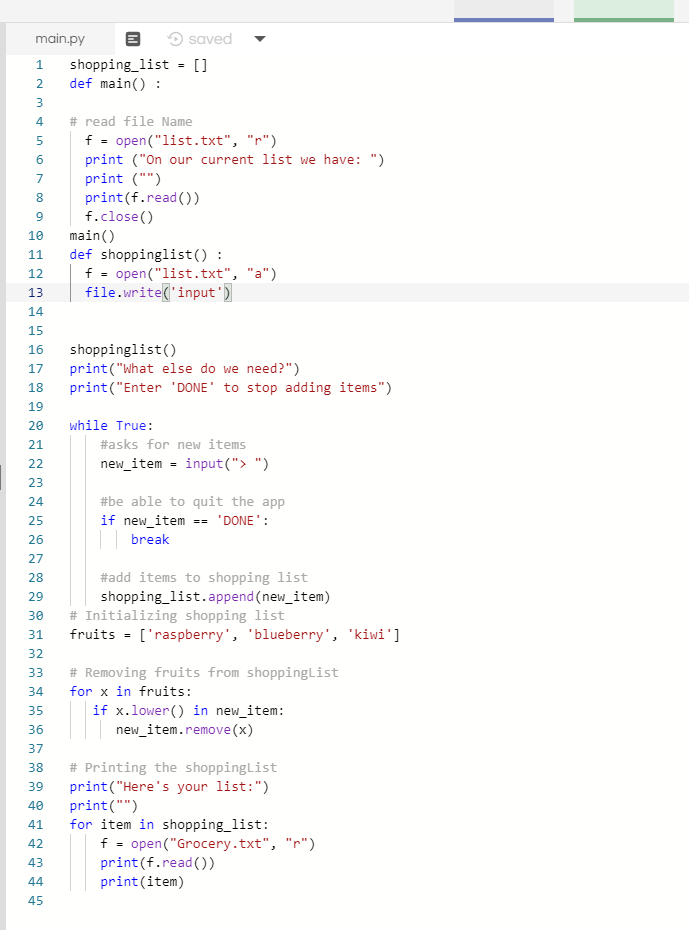
![How to Write a List to a File in Python [3 Methods] How To Write A List To A File In Python [3 Methods]](https://linuxhandbook.com/content/images/2020/06/Python-Scripting-Tips.png)

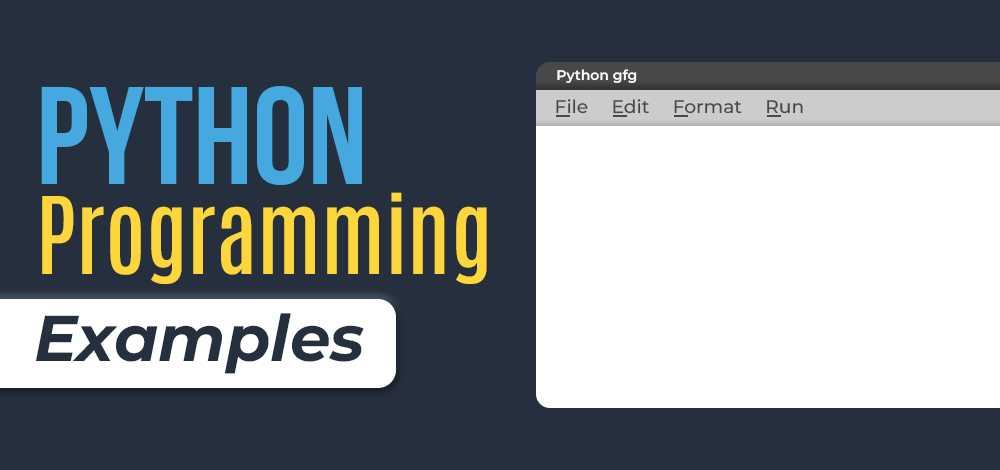
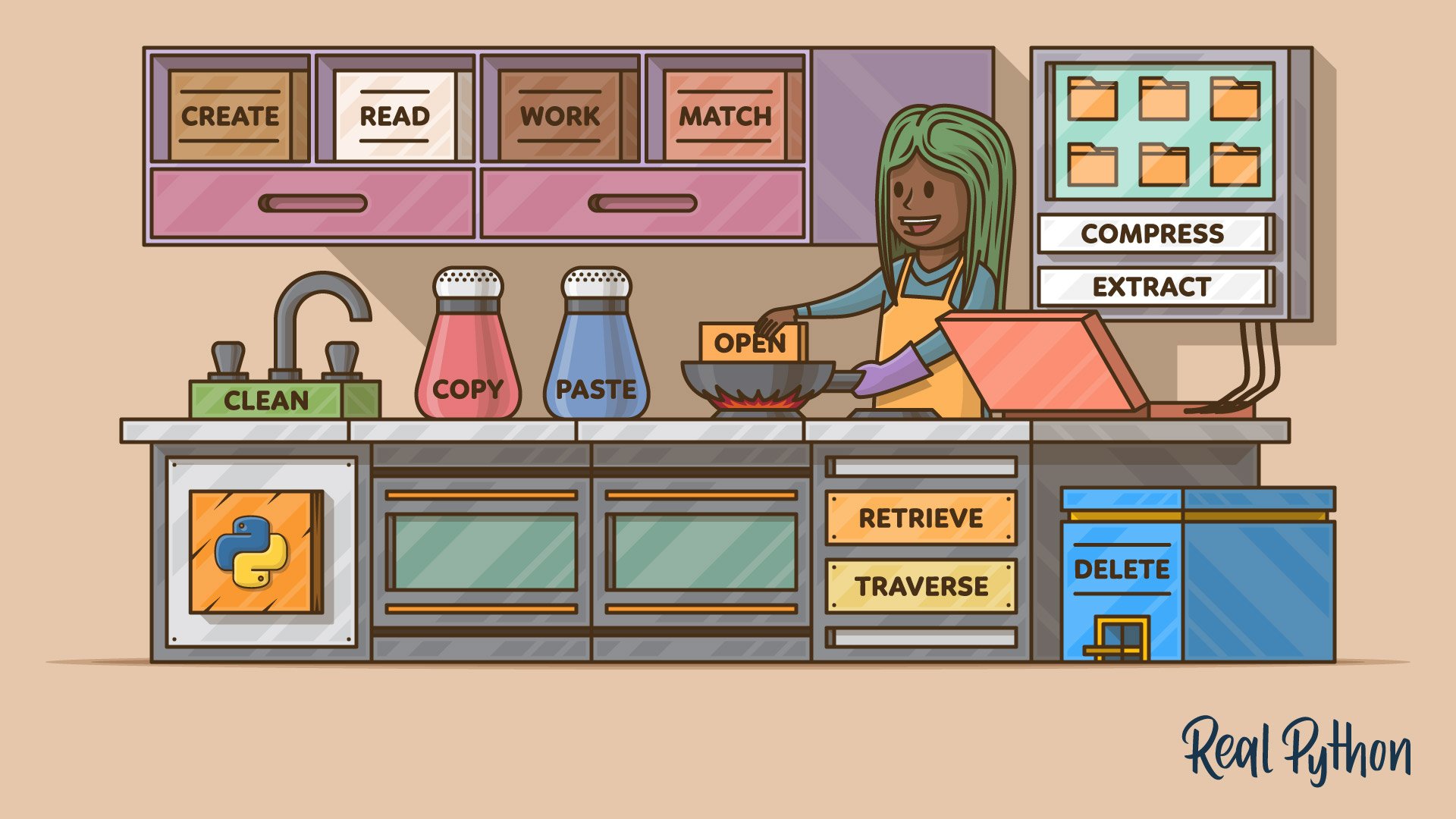
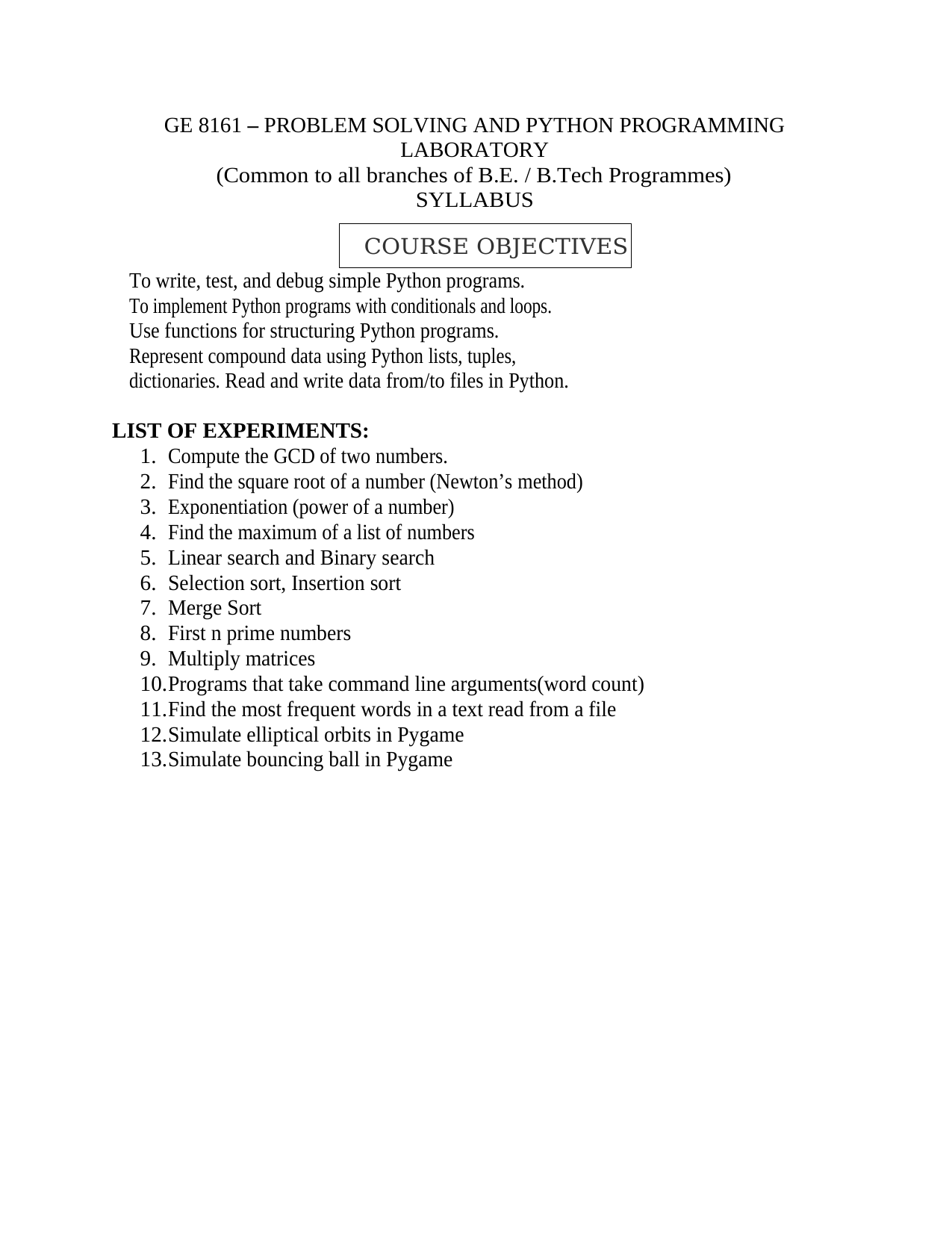
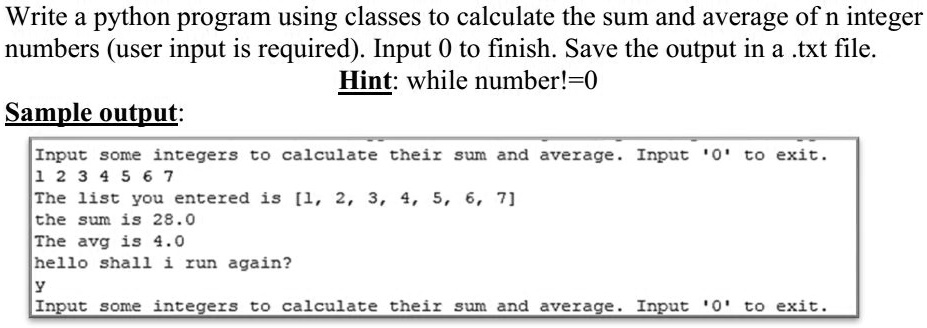
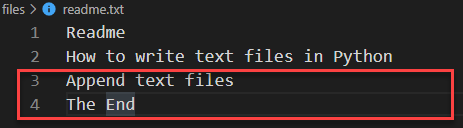
![Sample Paper] Kabir is a Python programmer working in a school. Sample Paper] Kabir Is A Python Programmer Working In A School.](https://d1avenlh0i1xmr.cloudfront.net/6447e807-9ecc-4410-9e08-0392f6f8ecab/python-program-(with-comments)-to-update-and-display-the-records-in-a-csv-file---teachoo.png)
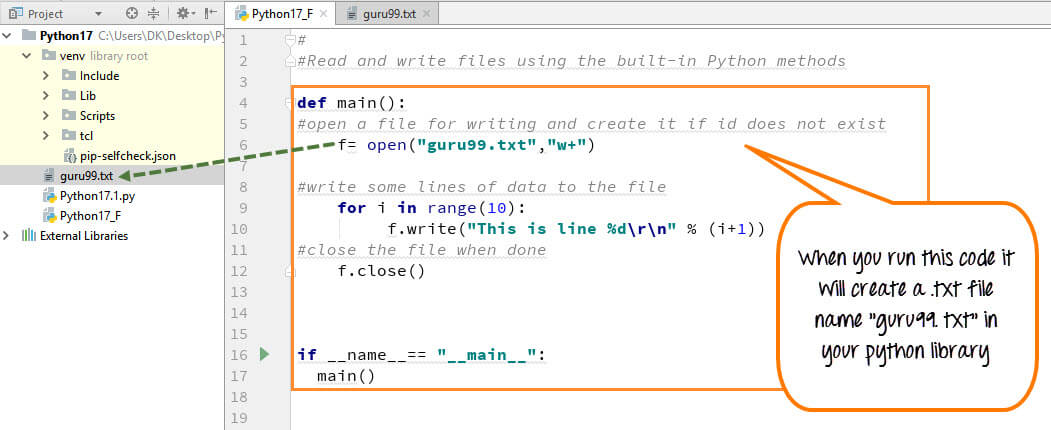

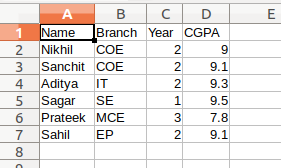
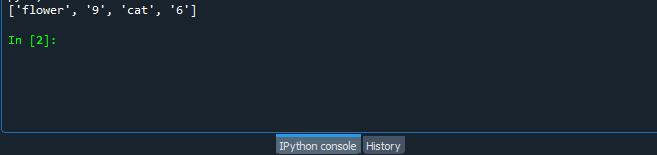
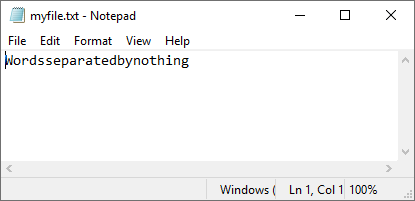
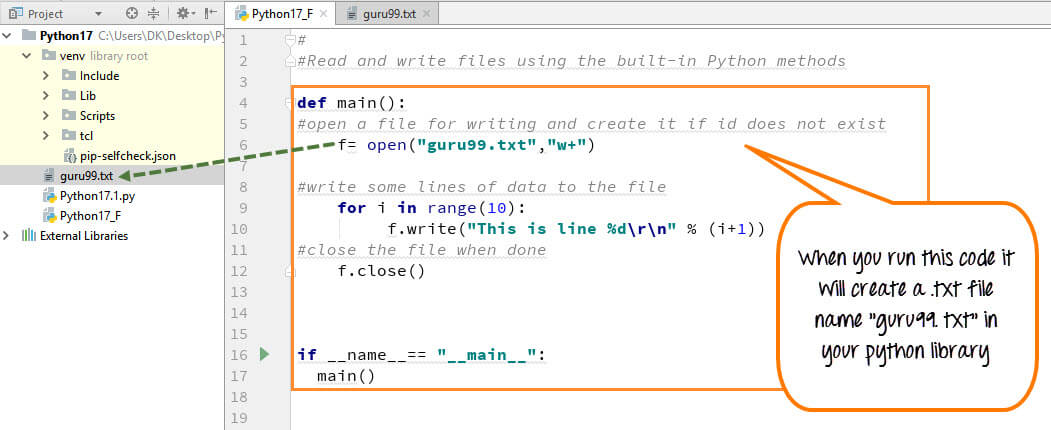




Article link: python writing list to file.
Learn more about the topic python writing list to file.
- Writing List to a File in Python – PYnative
- Python Program to Write List to File – Scaler Topics
- Writing a list to a file with Python, with newlines – Stack Overflow
- Reading and Writing Lists to a File in Python – Stack Abuse
- Reading and Writing lists to a file in Python – GeeksforGeeks
- File Handling in Python – How to Create, Read, and Write to a File
- Python Program To Write List To File
- Writing a List to a File in Python — A Step-by-Step Guide
- 3 Ways to Write a List to a File in Python – Linux Handbook
- How to Write a List Content to a File using Python
- Write List of Strings to File in Python
See more: https://nhanvietluanvan.com/luat-hoc