Raw Use Of Parameterized Class
Parameterized classes, also known as generic classes, are classes that can be defined with one or more type parameters. These type parameters serve as placeholders for actual types that will be supplied when instances of the class are created. The concept of parameterized classes is a fundamental feature of many modern programming languages, including Java, C#, and Python.
1. Defining parameterized classes: Understanding the concept and purpose
A parameterized class allows for the creation of reusable code that can work with different types of data without sacrificing type safety. By defining a class with type parameters, developers can write code that is independent of specific data types. This enhances code flexibility and promotes code reuse. It also makes the code easier to maintain and reduces the likelihood of errors due to incompatible data types.
2. Advantages of using parameterized classes: Increased flexibility and reusability
The use of parameterized classes offers several advantages. Firstly, it increases code flexibility by allowing the same class to work with different data types. This not only reduces code duplication but also enables developers to write generic algorithms that can be used with various data types. Secondly, parameterized classes promote code reusability. By defining a class once and supplying different type arguments, developers can save time and effort in writing similar code for different data types. Lastly, parameterized classes enhance code readability and maintainability by clearly defining the types of data that can be used with a class.
3. Creating parameterized classes in different programming languages: Syntax and techniques
The syntax for creating parameterized classes may vary slightly across different programming languages. In Java, for example, type parameters are enclosed in angle brackets and are typically denoted by a single uppercase letter. In C#, type parameters are also enclosed in angle brackets but can have more descriptive names. Python, on the other hand, uses square brackets to denote type parameters. Despite these minor syntax differences, the underlying concept and purpose of parameterized classes remain the same.
4. Implementing generic algorithms with parameterized classes: Enabling code efficiency and modularity
One of the main benefits of parameterized classes is the ability to implement generic algorithms. Generic algorithms are algorithms that can operate on different types of data without the need for duplication or modification. By using parameterized classes, developers can write algorithms that are independent of specific types and can work with a wide range of data. This promotes code efficiency and modularity, as the same algorithm can be used in different contexts without requiring any changes to the core logic.
5. Handling different data types with parameterized classes: Dynamic adaptability and type safety
Parameterized classes provide dynamic adaptability by allowing the use of different data types. This is particularly useful when dealing with collections of data, such as lists or maps. For example, the raw use of the parameterized class HashMap in Java allows for the creation of a map that can store key-value pairs of any type, without the need for explicit type casting. This dynamic adaptability not only simplifies code but also ensures type safety, as the compiler can check that the correct data types are being used.
6. Practical examples of parameterized classes: Solving real-world programming challenges
Parameterized classes can be used to solve a wide range of programming challenges. For example, a parameterized class called “genericcontainer” can be used to create a container that can hold objects of any type, providing a flexible storage solution. Another practical example is the use of the parameterized class “ResponseEntity” in Spring Boot, which allows for the creation of generic HTTP responses that can be customized based on different data types. These examples demonstrate the versatility and usefulness of parameterized classes in solving real-world programming challenges.
7. Best practices for using parameterized classes: Guidelines for efficient and maintainable code
When working with parameterized classes, it is important to follow best practices to ensure efficient and maintainable code. Firstly, it is recommended to always specify the type parameters explicitly when creating instances of parameterized classes, rather than relying on the raw use of the class. This helps to ensure type safety and avoid potential runtime errors. Secondly, it is important to use descriptive type parameter names that reflect the purpose or characteristics of the data being used. This improves code readability and makes it easier for other developers to understand the code.
8. Common pitfalls and challenges in working with parameterized classes: Troubleshooting and avoiding potential issues
While parameterized classes offer many advantages, there are also common pitfalls and challenges to be aware of. One common issue is the “raw use of parameterized classes,” where type parameters are not specified explicitly. This can lead to type safety issues and potential runtime errors. Another challenge is properly handling generic types, such as arrays or ArrayLists, to ensure that type parameters are correctly specified to avoid raw types. Being aware of these potential issues and following best practices can help developers avoid pitfalls and ensure the smooth implementation and use of parameterized classes.
FAQs:
Q: What is the raw use of a parameterized class?
A: The raw use of a parameterized class refers to using the class without specifying type parameters explicitly. This can lead to type safety issues and potential runtime errors.
Q: Can a raw type reference a generic type?
A: Yes, a raw type can reference a generic type. However, it is recommended to always specify type parameters explicitly to ensure type safety.
Q: Can a parameterized class work with different data types?
A: Yes, a parameterized class can work with different data types by supplying different type arguments when creating instances of the class.
Q: Is it necessary to specify type parameters when working with parameterized classes?
A: It is recommended to specify type parameters explicitly when working with parameterized classes to ensure type safety and avoid potential errors.
Q: How can parameterized classes enhance code flexibility?
A: Parameterized classes enhance code flexibility by allowing the same class to work with different data types, reducing code duplication, and enabling the creation of generic algorithms.
Q: How can parameterized classes improve code reusability?
A: Parameterized classes improve code reusability by allowing the same class to be used with different data types, reducing the need to write similar code for each specific type.
Q: What are some best practices for using parameterized classes?
A: Some best practices for using parameterized classes include always specifying type parameters explicitly, using descriptive type parameter names, and ensuring that type safety is maintained.
Java Fundamentals Generics 28 Raw Types
What Is Raw Type And Parameterized Type?
In programming, especially in object-oriented languages like Java, types play a crucial role in defining and manipulating data. When it comes to generics, Java provides two concepts known as raw types and parameterized types, which are essential for understanding and utilizing the power of generics effectively. This article will delve into the definitions and differences between raw types and parameterized types to provide a comprehensive understanding of these concepts.
Defining Raw Types:
A raw type, also known as a non-generic type or a generic type without type parameters, refers to using a generic class or interface as if it were a non-generic one. In essence, a raw type is an instance of a generic class or interface without providing the necessary type information. This implies that the generic type’s benefits, such as type safety and compile-time checks, are not utilized in the code using raw types.
To understand raw types better, consider the following example:
“`
List list = new ArrayList(); // Raw type
“`
In this example, `List` is a raw type because it lacks the necessary type parameter. As a result, you can add any type of object to this list, disregarding type safety checks enforced by generics. This absence of type information makes dealing with raw types prone to typecasting errors at runtime, as the compiler cannot enforce type checks.
Understanding Parameterized Types:
On the other hand, a parameterized type, as the name suggests, refers to a generic class or interface that is instantiated with a specific type parameter. With parameterized types, the type information is provided at the time of object creation, enabling the compiler to enforce type safety and perform compile-time checks.
Consider the following example:
“`
List
“`
In this example, `List
The Advantages of Parameterized Types:
Parameterized types provide several advantages when compared to raw types. Some of these advantages are:
1. Type Safety: Parameterized types ensure type safety by allowing the compiler to perform type checks at compile-time. This reduces the likelihood of runtime errors related to incompatible types.
2. Code Readability: By explicitly specifying the type parameter, parameterized types enhance code readability and self-documentation, making code maintenance and debugging easier.
3. Reduced Type Casting: Compared to raw types, parameterized types minimize the need for explicit type casting since the compiler has already validated the types.
FAQs:
Q: Can I use raw types and parameterized types interchangeably?
A: While it is possible to mix raw types and parameterized types, it is generally recommended to use parameterized types to leverage the benefits of generics. Raw types should be used sparingly and only when necessary to maintain backward compatibility.
Q: What happens if I use a raw type inadvertently?
A: If you accidentally use a raw type, the code will compile, but you may encounter runtime errors related to incompatible types as the compiler cannot enforce type checks.
Q: Can I convert a raw type to a parameterized type?
A: Converting a raw type to a parameterized type requires modifying the code by adding appropriate type parameters. However, it is essential to consider potential impacts on backward compatibility and thoroughly test the modified code.
Q: Are raw types considered deprecated in Java?
A: Raw types are not officially deprecated in Java; however, their usage is generally discouraged in favor of parameterized types due to their increased safety and readability.
In conclusion, raw types and parameterized types are fundamental concepts in Java’s generics. While raw types provide a way to use generic classes and interfaces without specifying type parameters, parameterized types offer increased type safety, code readability, and reduced type casting. By understanding the differences between raw types and parameterized types, developers can make informed decisions about which approach to employ in their code, ultimately improving code quality and maintainability.
What Is > In Java Generics?
Java generics are a powerful feature introduced in Java 5 to enhance the type safety of collections and eliminate the need for explicit casting. Generics allow developers to define classes, interfaces, and methods that can handle different types of objects, while still ensuring type safety at compile time. One significant aspect of generics in Java is the wildcard operator, denoted by >, which has an intriguing purpose. Let’s dive deeper into what the wildcard operator is and how it is used in Java generics.
Understanding Wildcards in Generics
Java generics primarily revolve around the idea of specifying types using a combination of angle brackets (<>) and type parameters. For example, if we want to create a generic class to represent a box that can hold any type of object, we can define it as follows:
“`java
public class Box
private T content;
public void setContent(T content) {
this.content = content;
}
public T getContent() {
return content;
}
}
“`
Here, the type parameter `
“`java
Box
Box
“`
This way, the box can only hold a `String` in the `stringBox` instance and an `Integer` in the `intBox` instance. However, there are scenarios where we may want to allow the box to hold any type of object, and this is where the wildcard operator comes into play.
The Wildcard Operator – >
The wildcard operator in Java generics, denoted by `>`, represents an unknown type. It is a placeholder for any type that can be specified at runtime. This allows us to define methods or classes that can work with any type of object. Let’s take a look at an example using the Box class defined earlier:
“`java
public class Box
private T content;
public void setContent(T content) {
this.content = content;
}
public T getContent() {
return content;
}
public boolean isContentEqualTo(Box> otherBox) {
return content.equals(otherBox.getContent());
}
}
“`
In this updated version of the Box class, we have added a new method `isContentEqualTo()` that takes another box as a parameter. Instead of specifying a concrete type for the other box, we use the wildcard operator to indicate that it can be any type. This allows us to compare the content of two boxes regardless of their types. For example:
“`java
Box
Box
stringBox.setContent(“Hello”);
intBox.setContent(42);
Box
floatBox.setContent(42.0f);
System.out.println(stringBox.isContentEqualTo(intBox)); // Output: false
System.out.println(intBox.isContentEqualTo(floatBox)); // Output: true
“`
Frequently Asked Questions (FAQs):
Q1. Can I add elements to a collection with wildcard type `>`?
A1. No, you cannot add elements to a collection with a wildcard type, `>`. This is because the type is unknown at compile time, and it may not match the type expected by the collection. However, you can still retrieve elements from the collection.
Q2. What is the difference between `>` and `
Q3. Can I use multiple wildcards in a single generic declaration?
A3. Yes, you can use multiple wildcards in a single generic declaration. For example, you can have a method that accepts two boxes with different wildcards:
“`java
public static void compareBoxes(Box extends Number> box1, Box super Integer> box2) {
// Perform comparison logic
}
“`
In this case, `box1` can accept any box of a subtype of `Number`, and `box2` can accept any box of a supertype of `Integer`.
Q4. Why would I use a wildcard instead of a concrete type?
A4. Wildcards provide flexibility when you want to work with multiple types, especially when the specific type is unknown or doesn’t matter. This allows for more generic and reusable code. Additionally, wildcards facilitate polymorphism by allowing you to write code that operates on a common base type.
Conclusion
The wildcard operator `>` in Java generics plays an essential role in creating generic, type-safe code that can handle unknown types at runtime. It allows developers to define methods and classes that can work with any type of object, providing flexibility and reusability. By understanding and utilizing the wildcard operator effectively, developers can harness the full power of Java generics.
Keywords searched by users: raw use of parameterized class Raw use of parameterized class, Raw use of parameterized class HashMap, Raw use of parameterized class ResponseEntity, Raw use of parameterized class genericcontainer, Is a raw type references to generic type should be parameterized, Class, Generic class Java, Arraylist is a raw type references to generic type arraylist e should be parameterized
Categories: Top 88 Raw Use Of Parameterized Class
See more here: nhanvietluanvan.com
Raw Use Of Parameterized Class
In the world of programming, flexibility and reusability are highly valued. One of the ways this is achieved is through the use of parameterized classes. A parameterized class, also known as a generic class, is a class that can operate on more than one type. It allows developers to write code that can be reused with different data types, without duplicating the entire class for each type. In this article, we will explore the raw use of parameterized classes, their benefits, and some common questions about them.
Understanding Parameterized Classes
To understand the raw use of parameterized classes, let’s first delve into what a parameterized class actually is. In simple terms, a parameterized class is a class that has one or more type parameters. These type parameters act as placeholders for the actual data types that will be used when creating instances of the class. For example, consider a generic class called `Box`:
“`
public class Box
private T content;
public Box(T content) {
this.content = content;
}
public T getContent() {
return content;
}
public void setContent(T content) {
this.content = content;
}
}
“`
In this example, the class `Box` is parameterized with a type parameter `T`. This means that when we create an instance of the `Box` class, we can specify the type of data that the box will hold. For instance, we can create a box of integers (`Box
The Raw Use of Parameterized Classes
Now that we have a basic understanding of generic classes, let’s explore the concept of raw use. Raw use refers to using a generic class without specifying the type parameter(s). When a generic class is used in this manner, it is treated as if it were a non-generic class. For example, we can create a raw instance of the `Box` class like this:
“`
Box rawBox = new Box(“Raw”);
“`
In this case, the type parameter `T` is omitted when creating the `rawBox` instance. Consequently, the `rawBox` instance is treated as if it were a `Box
Benefits of Parameterized Classes
Parameterized classes offer several benefits in terms of code reuse, type safety, and performance optimization. Let’s take a closer look at each of these benefits:
1. Code Reuse: By using parameterized classes, developers can write generic code that can be reused with different data types. This promotes code modularity and reduces code duplication, leading to more maintainable and efficient programs.
2. Type Safety: Generics provide compile-time type checking, ensuring that the correct types are used in the code. This helps detect errors early on and eliminates the need for explicit type casting, resulting in safer and more reliable code.
3. Performance Optimization: Parameterized classes can improve performance by avoiding unnecessary object boxing and unboxing operations. With generics, primitive types can be used directly, without the overhead of converting them to their corresponding wrapper classes.
FAQs about Raw Use of Parameterized Classes
Q: Can I use the raw type after specifying the type parameter initially?
A: Technically, you can use the raw type even if you have specified the type parameter earlier. However, it is not recommended since it defeats the purpose of using parameterized classes.
Q: Are there any risks associated with raw use of parameterized classes?
A: Yes, using raw types increases the chances of type errors and may lead to unexpected runtime exceptions if incompatible types are used. It is always better to use type-safe generics.
Q: Are parameterized classes limited to Java programming language?
A: No, parameterized classes are a concept supported by various programming languages, including C++, C#, and Kotlin, to name a few.
Q: Are there any performance differences between raw and parameterized types?
A: Using raw types might lead to a slight improvement in performance due to the elimination of type checking and boxing/unboxing operations. However, the primary goal of using generics is code reuse and type safety, rather than performance optimization.
In conclusion, the raw use of parameterized classes provides the ability to use generic classes without specifying the type parameter(s). While it can be tempting to use raw types for convenience, it is generally recommended to avoid them and benefit from the strong type checking and code reuse that parameterized classes offer. By embracing generics, developers can create more maintainable, type-safe, and efficient code.
Raw Use Of Parameterized Class Hashmap
In programming, data structures play a fundamental role in organizing and storing large amounts of information efficiently. One such data structure is the HashMap, which is a parameterized class widely used in many programming languages, including Java. This article aims to explore the raw use of the parameterized class HashMap, explaining its implementation, features, and common use cases. But before diving into the details, let’s understand what a HashMap is.
What is a HashMap?
A HashMap is a data structure that provides a way to store and retrieve key-value pairs efficiently. It is part of the Java Collections Framework and is widely used due to its high performance and flexibility. In a HashMap, each key is unique, and it maps to a corresponding value. This means that given a key, you can quickly retrieve the associated value. HashMaps are implemented using an array of linked lists, where each list represents a bucket that holds elements with the same hash code.
Raw Use of HashMap:
A raw use of HashMap refers to using the class without specifying the type of its key-value pairs, also known as the type parameters. In this case, the HashMap treats all keys and values as Objects. While using the raw form of HashMap eliminates the compile-time type-checking benefits provided by generics, it is still functional and can be useful in certain scenarios.
Initialization and Usage:
To create an instance of a raw HashMap, you simply use the default constructor, as shown below:
“`
HashMap map = new HashMap();
“`
Once initialized, you can populate the HashMap with key-value pairs using the `put()` method, like so:
“`
map.put(key1, value1);
map.put(key2, value2);
“`
To retrieve the value associated with a key, you can use the `get()` method:
“`
Object value = map.get(key);
“`
Similarly, you can remove a key-value pair using the `remove()` method:
“`
Object removedValue = map.remove(key);
“`
It is important to note that when using a raw HashMap, you need to ensure type safety manually, as the compiler won’t be able to provide any checks. This can lead to runtime errors if incorrect types are used for keys or values. Therefore, it is generally recommended to use the parameterized form of HashMap, which provides compile-time type checking.
Common Use Cases:
While using the raw form of HashMap may not be the best practice, there are scenarios where it can still be applicable. One such situation is when dealing with legacy code or when migrating from older versions of Java that do not support generics. Another case is when the exact type of keys and values is not known, and you need to work with general Objects. However, caution is essential in these situations to prevent type-related errors and unexpected behavior.
FAQs:
Q: Can a raw HashMap store different types of keys and values?
A: Yes, a raw HashMap can store keys and values of any object type since it treats them as Objects. However, type-related errors might occur if care is not taken while retrieving the values.
Q: Is there a performance difference between a generic HashMap and a raw HashMap?
A: In terms of performance, there is usually no significant difference between the two. The raw HashMap might even have a slight edge due to not having to perform type checks during runtime.
Q: Can I use the raw HashMap alongside other generic collections?
A: Mixing raw and generic collections in the same codebase might lead to type-safety issues and is generally discouraged. It is recommended to migrate to using generics consistently.
Q: Can I iterate over a raw HashMap in a type-safe manner?
A: Since the raw HashMap does not maintain type information, iterating over it in a type-safe manner is not possible. You would need to manually check and cast values appropriately.
Q: Is it safe to use a raw HashMap in a multi-threaded environment?
A: No, using a raw HashMap in a multi-threaded environment can lead to unpredictable results and data corruption. It is advisable to use thread-safe alternatives, such as ConcurrentHashMap.
Concluding Thoughts:
While the raw use of the parameterized class HashMap should be avoided in most cases, it still provides functionality when type information is unknown or when dealing with older code. However, it is crucial to handle type safety manually and be cautious of potential pitfalls. As the use of generics is more prevalent and recommended, it is advisable to migrate to the parameterized form of HashMap for improved compile-time type checking and better code readability.
Raw Use Of Parameterized Class Responseentity
The use of RESTful services has become increasingly popular in the world of software development. As a result, developers often find themselves in need of handling the HTTP response returned by these services. ResponseEntity, a parameterized class in the Java Spring framework, offers a flexible and powerful way to handle such responses. In this article, we will delve into the raw use of the ResponseEntity class, exploring its features, benefits, and how it can simplify the handling of HTTP responses.
What is ResponseEntity?
ResponseEntity is a class provided by the Java Spring framework that represents an HTTP response. It can hold not only the actual response body but also custom headers, response status codes, and other relevant information. This class allows developers to have complete control over the returned HTTP response and enables them to define the required behavior based on the specific use case.
Understanding Parameterized Classes
Before diving deeper into the raw use of ResponseEntity, it is important to understand the concept of parameterized classes in Java. Parameterized classes, or generics, allow developers to create a class that can be customized for different data types. In the case of ResponseEntity, it can be parameterized with the desired response body type – for example, ResponseEntity>.
Raw Use of ResponseEntity
When using ResponseEntity in its raw form, without specifying the type of the response body, developers gain flexibility in handling various types of responses. They can retrieve the response body as a raw String, an Object, or even as a byte array. This raw use is particularly useful when the response body is of an unknown type or when the response contains binary data.
Benefits of Raw Use
The raw use of ResponseEntity provides several benefits to developers working with RESTful services. First and foremost, it allows for easy handling of responses without the need to define complex and specific response body types. This ensures greater flexibility when working with different types of responses.
Additionally, the raw use of ResponseEntity simplifies error handling by allowing the retrieval of error messages or stack traces from the response body. This is often useful when dealing with bad requests or server errors, as it enables developers to extract and display meaningful information to the user.
Furthermore, the raw use of ResponseEntity facilitates testing and debugging of RESTful services. Since it is not necessary to define specific response body types, developers can quickly test different scenarios and examine the returned responses directly. This helps detect and troubleshoot issues in a timely manner.
Best Practices and Example Use Cases
While the raw use of ResponseEntity offers flexibility, it is important to follow some best practices to ensure clean and robust code. One best practice is to use explicit type casting when retrieving the response body, as it allows for better clarity and avoids potential runtime errors.
Example Use Case: Raw String Response
Consider a scenario where a RESTful service is expected to return a plain String response. By utilizing the raw use of ResponseEntity, developers can easily retrieve and process this response. Below is an example code snippet demonstrating this use case:
“`java
RestTemplate restTemplate = new RestTemplate();
ResponseEntity
if (response.getStatusCode().is2xxSuccessful()) {
String responseBody = response.getBody();
// Process and handle the response accordingly
} else {
// Handle error response
}
“`
In this example, the getForEntity method of RestTemplate returns a ResponseEntity
FAQs:
1. Can I use ResponseEntity without parameterizing its type?
Yes, you can use ResponseEntity without specifying the type of the response body. This allows for flexible handling of various response types.
2. How does the raw use of ResponseEntity simplify error handling?
By retrieving the response body as a raw String or Object, developers can easily extract error messages or stack traces, enabling better error handling and user feedback.
3. Are there any drawbacks to using the raw form of ResponseEntity?
While the raw use of ResponseEntity provides flexibility, it may affect type safety. It is important to ensure proper type casting and validation to avoid potential runtime errors.
4. Can ResponseEntity be used in asynchronous programming?
Yes, ResponseEntity can be used in asynchronous programming when combined with frameworks like CompletableFuture or reactive programming libraries like Reactor.
In conclusion, the raw use of ResponseEntity in Java Spring offers developers a powerful and flexible way to handle HTTP responses in RESTful services. It simplifies response handling, error management, and facilitates testing and debugging. By following best practices and leveraging the raw use of ResponseEntity, developers can optimize their code and create robust and efficient applications.
Images related to the topic raw use of parameterized class
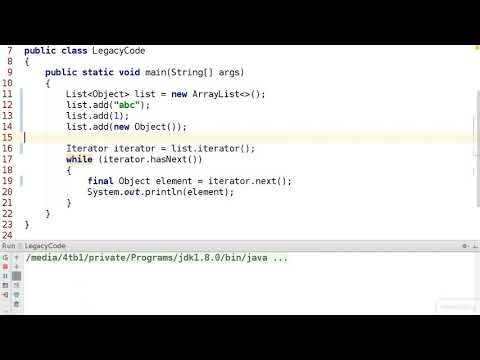
Found 47 images related to raw use of parameterized class theme


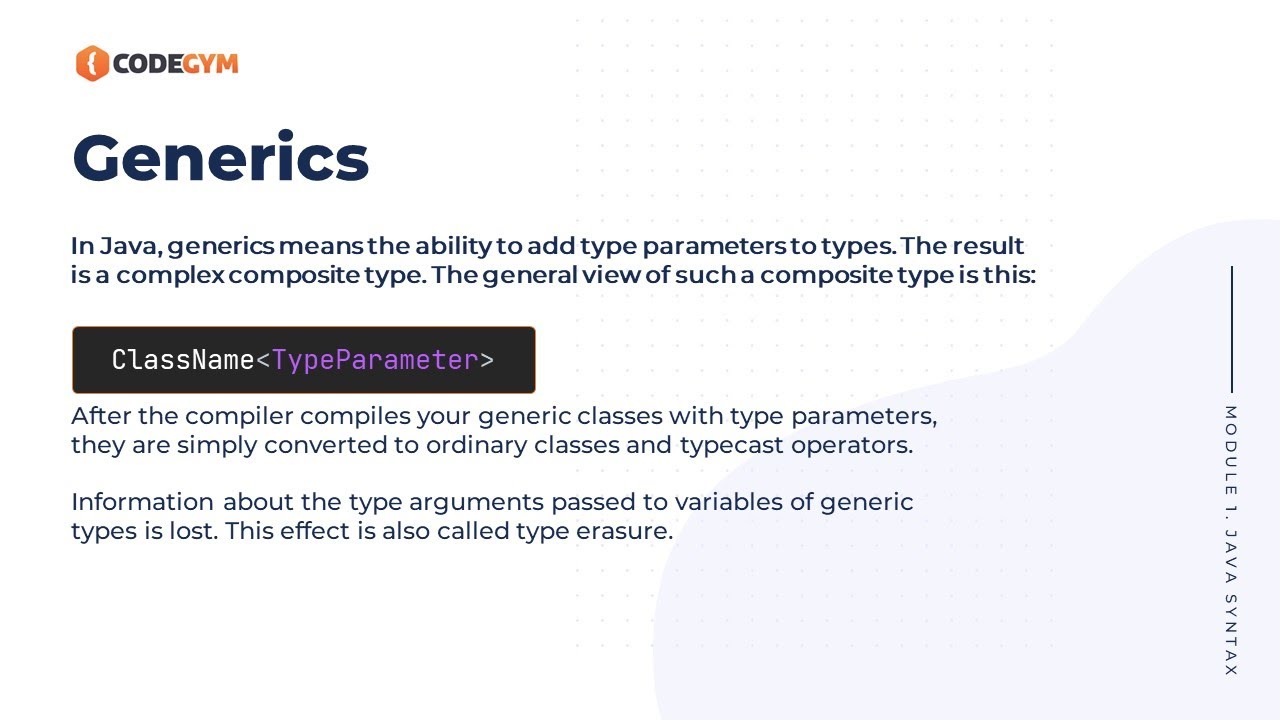
Article link: raw use of parameterized class.
Learn more about the topic raw use of parameterized class.
- Raw use of parameterized class – java – Stack Overflow
- Inspection ‘Raw use of parameterized class’ should provide …
- Raw Types in Java | Baeldung
- What are Generics in Java? – Educative.io
- Java Generics – Classes – Tutorialspoint
- How to write parametrized Generic class and method in Java Example
- Raw Types in Java | Baeldung
- Raw Types – Learning the Java Language
- Java – Don’t use raw types – Sebastian Weber
- Avoid raw types – Java Practices
- Java static code analysis: Raw types should not be used
- Java Generics Example Tutorial – Generic Method, Class …
- Spring / Intellij: Raw use of parameterized class ‘HttpEntity’
- Solve Java warning “Raw use of parameterized class” – GitHub
See more: https://nhanvietluanvan.com/luat-hoc