Python Append Multiple Items To List
Appending a Single Item to a List
Appending a single item to a list in Python is straightforward. You can use the `append()` method, which adds the provided item to the end of the list. Here’s an example:
“`python
my_list = [“apple”, “banana”, “cherry”]
my_list.append(“date”)
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”]
“`
Appending Multiple Items Using a Loop
If you have a collection of items that you want to add to a list, you can use a loop to iterate through the collection and append each item individually. This is useful when you have a fixed set of items or when you want to modify the items before appending them. Here’s an example:
“`python
my_list = [“apple”, “banana”, “cherry”]
fruits = [“date”, “elderberry”, “fig”]
for fruit in fruits:
my_list.append(fruit)
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”, “elderberry”, “fig”]
“`
Appending Multiple Items Using `extend()`
The `extend()` method allows you to append multiple items to a list at once. It takes an iterable as an argument and adds each item in the iterable to the end of the list. Here’s an example:
“`python
my_list = [“apple”, “banana”, “cherry”]
fruits = [“date”, “elderberry”, “fig”]
my_list.extend(fruits)
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”, “elderberry”, “fig”]
“`
Appending Multiple Items Using `+=`
In Python, you can also use the `+=` operator to append multiple items to a list. This operator is shorthand and is equivalent to using the `extend()` method. Here’s an example:
“`python
my_list = [“apple”, “banana”, “cherry”]
fruits = [“date”, “elderberry”, “fig”]
my_list += fruits
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”, “elderberry”, “fig”]
“`
Appending Multiple Items Using List Comprehension
List comprehension is a concise and powerful way to create lists in Python. It can also be used to append multiple items to an existing list. Here’s an example:
“`python
my_list = [“apple”, “banana”, “cherry”]
fruits = [“date”, “elderberry”, “fig”]
my_list += [fruit for fruit in fruits]
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”, “elderberry”, “fig”]
“`
Appending Items from Another List
If you have another list that you want to append to an existing list, you can use the same methods described above. Whether you choose to use a loop, `extend()`, `+=`, or list comprehension depends on your specific needs. Here’s an example using `extend()`:
“`python
my_list = [“apple”, “banana”, “cherry”]
other_list = [“date”, “elderberry”, “fig”]
my_list.extend(other_list)
print(my_list)
“`
Output:
“`
[“apple”, “banana”, “cherry”, “date”, “elderberry”, “fig”]
“`
FAQs
Q: How do I append multiple strings to a list in Python?
A: To append multiple strings to a list, you can use any of the methods described above. Simply represent each string as an individual item in an iterable such as a list, then use the desired method to append the items to the list.
Q: Can I append multiple elements at once in Python?
A: Yes, Python provides several methods like `extend()` and `+=` to append multiple elements to a list at once.
Q: How can I append multiple columns to a list in Python?
A: To append multiple columns to a list, you can create a list of lists where each inner list represents a column. Then, use the desired method like `extend()` to append the columns to the list.
Q: How do I append an item multiple times in Python?
A: If you want to append an item multiple times to a list, you can use the methods described above in a loop by specifying the number of times you want to append the item.
Q: What is the difference between extend() and append() in Python?
A: The `extend()` method adds multiple items to the end of a list, while the `append()` method adds a single item. So, if you want to add multiple items at once, you should use `extend()`.
Q: How can I insert a list into another list in Python?
A: To insert a list into another list, you can use the `extend()` method or the `+=` operator to add the items from one list to another.
Q: How can I append all elements of a list in Python?
A: To append all elements of a list to another list, you can use the `extend()` method or the `+=` operator with the desired list. This will add each item individually to the target list.
How To Append To A List In Python
Can You Append Multiple Items To A List Python At Once?
Python, being a versatile and powerful programming language, offers various ways to efficiently manipulate lists. Lists are commonly used to store multiple items in a single variable, and often developers need to append multiple elements to a list at once. In this article, we will explore different techniques and approaches to achieve this goal.
Understanding Lists in Python
Before diving into the specifics of appending multiple items to a list in Python, let’s have a quick overview of lists. Lists are ordered, mutable collections of items, enclosed within square brackets and separated by commas. They can store any data type, including integers, strings, or even other lists.
Appending Single Items to a List
In Python, the append() method is used to add individual elements to an existing list. This method takes a single argument, the item to be added, and appends it to the end of the list. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
“`
Appending Multiple Items to a List
Python provides multiple approaches to append multiple items to a list simultaneously. Let’s explore some of these methods:
1. Using the “+=” Operator: The “+=” operator, also known as the augmented assignment operator, allows concatenating lists together. By using this operator, we can append multiple items to an existing list in a single line of code. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list += [4, 5, 6]
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
“`
2. Using the extend() Method: The extend() method in Python appends all items from a given iterable to the end of an existing list. It accepts an iterable, such as a list or tuple, as its argument. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
“`
3. Using List Concatenation: Another way to append multiple items to a list is by utilizing list concatenation. This involves creating a new list by concatenating the existing list with a new list containing the desired items. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list = my_list + [4, 5, 6]
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
“`
Selecting the appropriate method depends on the specific requirements of a given scenario. While the above techniques serve the purpose of appending multiple items to a list, each method has its own advantages and considerations.
FAQs
Q: Can I append multiple items of different data types?
A: Yes, Python allows appending items of different data types to a list. Lists in Python are flexible in that they can store a mix of integers, strings, other lists, or any other valid data type.
Q: Can I append multiple items to an empty list?
A: Absolutely. Whether you have an existing list or an empty list, you can append multiple items using the methods mentioned above. For an empty list, appending multiple items will populate the list with the given elements.
Q: Which method is the most efficient for appending multiple items?
A: When it comes to efficiency, using the extend() method is often the most performant approach. This is because extend() iterates over the given iterable and adds each element to the list individually.
Q: Can I append items from another list created on-the-fly?
A: Yes, you can create a new list within the append or extend methods, effectively appending items from another list without explicitly defining it as a separate variable. This allows for more concise and readable code.
Q: Is it possible to append items in specific positions within a list?
A: No, by default, appending items to a list adds them to the end. If you need to insert items at specific positions, you can use the insert() method or slice assignment instead.
In conclusion, Python provides several convenient ways to append multiple items to a list. Whether using the augmented assignment operator, extend() method, or list concatenation, developers can efficiently expand the contents of a list without the need for complicated loops or extra code. Understanding and utilizing these methods will greatly enhance your programming productivity.
Can Append Take Multiple Values?
In programming, the ability to add elements to a list or an array is often achieved using the “append” function. This function allows developers to append, or add, elements to the end of a sequence. However, one common question that arises is whether the append function can take multiple values and add them to the sequence at once.
The answer to this question depends on the programming language being used. In some languages, such as Python, the append function can indeed take multiple values and add them to the sequence. Let’s explore this concept further.
Appending Multiple Values in Python:
Python is a popular programming language known for its simplicity and readability. In Python, the append function is used to add elements to a list. This function can take one value at a time and add it to the end of the list. However, if you want to append multiple values to the list, you can achieve this by using the concept of unpacking.
In Python, you can use the asterisk (*) operator to unpack multiple values from a tuple or a list. For example, suppose you have two lists, list1 and list2, and you want to append all the elements from list2 to list1. You can do this by unpacking list2 using the asterisk operator and passing it as arguments to the append function.
Here’s an example:
“`
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.append(*list2)
print(list1)
“`
Output:
[1, 2, 3, 4, 5, 6]
As you can see, the elements from list2 (4, 5, 6) are added to list1 using the append function. By unpacking the list using the asterisk operator, we can pass the individual elements to the function.
Appending Multiple Values in Other Languages:
While Python allows appending multiple values using the unpacking technique, not all programming languages provide this functionality. In some languages, the append function can only accept one value at a time and add it to the sequence. In such cases, you would need to loop over the values you want to append and call the append function for each element.
For example, in languages like JavaScript or Java, you would need to iterate over the values and call the append function inside a loop. This would add each value to the sequence one by one.
Here’s a JavaScript example:
“`
let list = [1, 2, 3];
let valuesToAdd = [4, 5, 6];
for (let value of valuesToAdd) {
list.push(value);
}
console.log(list);
“`
Output:
[1, 2, 3, 4, 5, 6]
As shown in the example, we iterate over the valuesToAdd array using a loop and call the push function (equivalent to append in this case) to add each value to the list.
FAQs:
Q: Can append take multiple values in all programming languages?
A: No, the ability to append multiple values using the append function depends on the programming language. Some languages, like Python, allow appending multiple values using unpacking techniques, while others require looping over the values.
Q: Why would I need to append multiple values at once?
A: Appending multiple values at once can be useful when you want to add a whole sequence of elements to an existing list or array without the need for individual append calls.
Q: Are there any performance implications of appending multiple values?
A: The performance implications may vary depending on the programming language and the size of the list or array. In some cases, appending all the values at once may be more efficient compared to multiple append calls within a loop.
Q: Can I append multiple values to the beginning of a sequence?
A: The append function typically adds elements to the end of a sequence. To add multiple values at the beginning, you may need to use a different function or technique, such as concatenation or inserting the values at the desired position.
In conclusion, the ability to append multiple values using the append function depends on the programming language. Python provides the flexibility to append multiple values using the unpacking technique, while other languages may require looping over the values. Understanding the capabilities and limitations of the append function in your chosen language is crucial to effectively manipulate sequences.
Keywords searched by users: python append multiple items to list python append multiple strings to list, Append multiple elements Python, Multiple list Python, append multiple columns to list python, Python append multiple times, Insert list to list Python, List append list Python, Append all elements of a list Python
Categories: Top 86 Python Append Multiple Items To List
See more here: nhanvietluanvan.com
Python Append Multiple Strings To List
To append multiple strings to a list in Python, there are several techniques that you can use. Let’s explore some of the most common ones:
1. Using the append() method:
The append() method is a built-in function in Python that allows you to add elements to the end of a list. To append a single string, you can simply call this method with the string as the argument. However, when dealing with multiple strings, you can use a for loop to iterate over the strings and append them one by one. Here’s an example:
“`python
my_list = []
strings = [‘Hello’, ‘World’, ‘Python’]
for string in strings:
my_list.append(string)
print(my_list)
“`
This will result in the list `[‘Hello’, ‘World’, ‘Python’]`.
2. Using the extend() method:
Similar to the append() method, the extend() method also adds elements to the end of a list. However, the extend() method takes an iterable as an argument, which allows you to append multiple strings at once. Here’s an example:
“`python
my_list = []
strings = [‘Hello’, ‘World’, ‘Python’]
my_list.extend(strings)
print(my_list)
“`
This will also result in the list `[‘Hello’, ‘World’, ‘Python’]`.
3. Using the ‘+’ operator:
Python allows you to concatenate lists using the ‘+’ operator. By using this operator, you can easily merge multiple lists together. To append multiple strings to a list using this approach, you need to convert each string into a list and then concatenate them. Here’s an example:
“`python
my_list = []
strings = [‘Hello’, ‘World’, ‘Python’]
for string in strings:
my_list += list(string)
print(my_list)
“`
This will also result in the list `[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’, ‘P’, ‘y’, ‘t’, ‘h’, ‘o’, ‘n’]`. Note that the strings are split into individual characters.
4. Using a list comprehension:
List comprehensions offer a concise way to create lists in Python. By using a list comprehension, you can create a new list by iterating over each string and appending it to the list. Here’s an example:
“`python
strings = [‘Hello’, ‘World’, ‘Python’]
my_list = [string for string in strings]
print(my_list)
“`
This will also result in the list `[‘Hello’, ‘World’, ‘Python’]`.
These are just a few of the approaches to append multiple strings to a list in Python. Choose the method that best suits your needs and the complexity of your problem.
**FAQs:**
Q1. Can I append strings of different lengths into a list?
Yes, you can append strings of different lengths into a list. Python treats strings as sequences of characters, so you can append strings of any length to a list without any issues.
Q2. Can I append other types of data, such as numbers or booleans, to a list using these techniques?
Yes, you can append other types of data, such as numbers, booleans, or even other lists, using these techniques. The append() method, extend() method, ‘+’ operator, and list comprehensions can handle various types of data, not just strings.
Q3. Is there a specific order in which the strings are appended to the list?
Yes, the order in which the strings are appended to the list depends on the order in which you provide the strings or how you iterate over them. The examples provided in this article preserve the order of the original strings.
Q4. Can I append an empty string to a list using these techniques?
Yes, you can append an empty string to a list using the append() method, extend() method, ‘+’ operator, and list comprehensions. Python treats an empty string as a valid element and will append it to the list accordingly.
In conclusion, Python offers several convenient techniques to append multiple strings to a list. Whether you prefer using the append() or extend() method, the ‘+’ operator, or list comprehensions, you have the flexibility to choose the approach that best suits your needs. By mastering these techniques, you can efficiently manipulate lists in your Python programs.
Append Multiple Elements Python
In Python, the append() method is commonly used to add elements to a list. However, what if you want to append multiple elements to a list at once? Thankfully, Python provides us with multiple ways to achieve this. In this article, we will explore various techniques to append multiple elements in Python, including using the extend() method, list concatenation, and list comprehension.
Using the extend() Method
The extend() method allows you to append multiple elements to an existing list. It takes an iterable (such as a list, tuple, or string) as an argument and appends each element of the iterable to the end of the original list. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list)
“`
Output:
“`
[1, 2, 3, 4, 5, 6]
“`
In the above code, we first initialize a list called `my_list` with three elements. Then, we use the `extend()` method to append the elements `[4, 5, 6]` to the original list. Finally, the updated list is printed, showing all the appended elements.
List Concatenation
Another way to append multiple elements in Python is by using list concatenation. This involves creating a new list by combining the original list with the desired elements. Here’s an example:
“`python
my_list = [1, 2, 3]
appended_list = my_list + [4, 5, 6]
print(appended_list)
“`
Output:
“`
[1, 2, 3, 4, 5, 6]
“`
In the above code, we use the `+` operator to concatenate `my_list` with `[4, 5, 6]` and assign the result to a new list called `appended_list`. As a result, the appended elements are added to the end of the original list.
List Comprehension
List comprehension is a concise and elegant way to perform various operations on lists, including appending multiple elements. By utilizing list comprehension, we can append elements based on certain conditions or from another iterable. Here’s an example:
“`python
my_list = [1, 2, 3]
appended_list = my_list + [x for x in range(4, 7)]
print(appended_list)
“`
Output:
“`
[1, 2, 3, 4, 5, 6]
“`
In the above code, we use list comprehension to create a new list `[x for x in range(4, 7)]`, which generates elements from 4 to 6. Then, we concatenate `my_list` with this new list and assign the result to `appended_list`. As a result, the elements from the range are appended to the original list.
FAQs (Frequently Asked Questions):
Q: Can we append multiple elements to a list using the append() method?
A: No, the append() method is designed to append only a single element at a time. To append multiple elements, you can utilize the extend() method, list concatenation, or list comprehension.
Q: Are there any performance differences between these methods?
A: Yes, there can be performance differences depending on the size of the original list and the number of elements to append. The extend() method has better performance compared to list concatenation since it directly modifies the original list. List concatenation creates a new list, resulting in additional memory usage.
Q: Can we append elements from different data types?
A: Yes, all these methods can append elements of different data types to a list.
Q: Is it possible to append elements to a list on condition?
A: Yes, you can use list comprehension to append elements based on certain conditions. For example, you can append only the elements that satisfy a specific condition or append elements that meet specific criteria from another iterable.
Q: Can we append elements to a list at a specific position?
A: While the examples in this article focus on appending elements to the end of a list, you can insert elements at a specific position using the insert() method.
Conclusion
Appending multiple elements to a list in Python is a common task, and there are several approaches to achieve it. The extend() method is the most straightforward method for appending multiple elements, followed by list concatenation and list comprehension. Depending on the requirements and performance considerations, you can choose the most suitable method to append multiple elements to your lists in Python.
Multiple List Python
Multiple List Python refers to the capability of using multiple lists in various operations, such as iteration, concatenation, and comparison. It allows developers to perform operations on multiple lists at once, eliminating the need for repetitive code or complex logic.
One of the most common use cases of Multiple List Python is iterating over multiple lists simultaneously. Let’s consider an example where we have two lists, `list1` and `list2`, both containing the same number of elements. We can easily iterate over both lists simultaneously with a single loop using the built-in `zip()` function, as shown below:
“`python
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’, ‘c’]
for x, y in zip(list1, list2):
print(x, y)
“`
The output of this code will be:
“`
1 a
2 b
3 c
“`
The `zip()` function takes multiple lists as input and returns an iterator that generates tuples containing elements from each list. In the above example, `x` and `y` are variables that hold the current elements from `list1` and `list2`, respectively, in each iteration of the loop.
Another useful operation that can be performed with Multiple List Python is concatenation. With the `+` operator, we can concatenate multiple lists into a single list efficiently. For instance:
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
concatenated_list = list1 + list2 + list3
print(concatenated_list)
“`
The output will be:
“`
[1, 2, 3, 4, 5, 6, 7, 8, 9]
“`
By using the `+` operator, we can combine the elements from all three lists into a single list called `concatenated_list`. It is important to note that this operation does not modify the original lists; instead, it produces a new list.
Multiple List Python also provides the ability to compare lists. We can compare multiple lists element-wise using comparison operators like `==` (equality), `!=` (inequality), `<` (less than), `>` (greater than), etc. When comparing two or more lists, the result will be a boolean value indicating whether the comparison is true or false.
“`python
list1 = [1, 2, 3]
list2 = [1, 2, 3]
list3 = [4, 5, 6]
print(list1 == list2) # Output: True
print(list1 != list3) # Output: True
print(list1 < list3) # Output: True
```
In this example, the first comparison `list1 == list2` returns `True` because all elements in both lists are equal. The second comparison `list1 != list3` returns `True` because at least one element between the two lists is different. And finally, the third comparison `list1 < list3` returns `True` because the first element of `list1` is less than the first element of `list3`.
Now let's address some frequently asked questions about Multiple List Python:
**Q: Can I iterate over more than two lists simultaneously?**
A: Yes, you can iterate over any number of lists simultaneously using the `zip()` function. Simply provide the additional lists as arguments to `zip()`, and the resulting iterator will generate tuples containing elements from all the lists.
**Q: Does the `zip()` function stop when it reaches the end of the shortest list?**
A: Yes, `zip()` stops iterating as soon as it reaches the end of the shortest list. If the lists have different lengths, the resulting iterator will only contain tuples up to the length of the shortest list.
**Q: Can I assign the result of `zip()` to a variable for later use?**
A: Yes, you can assign the result of `zip()` to a variable, and that variable will hold the iterator object. You can then iterate over the iterator in a loop or convert it to a list using the `list()` function if you need random access to the elements.
**Q: Can I perform mathematical operations on multiple lists?**
A: Yes, you can perform mathematical operations like addition, subtraction, multiplication, etc., on multiple lists element-wise. The operation will be applied to each corresponding pair of elements from the lists.
In conclusion, Multiple List Python is a powerful tool that allows developers to work with multiple lists simultaneously. It brings convenience, efficiency, and simplicity to the code by eliminating the need for repetitive logic. Whether it's iterating over lists, concatenating them, or comparing their elements, Multiple List Python proves to be a valuable feature in the Python programming language.
Images related to the topic python append multiple items to list
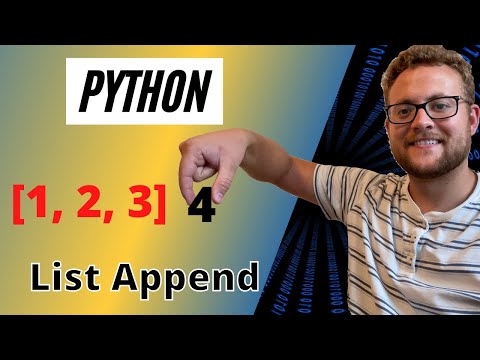
Found 17 images related to python append multiple items to list theme


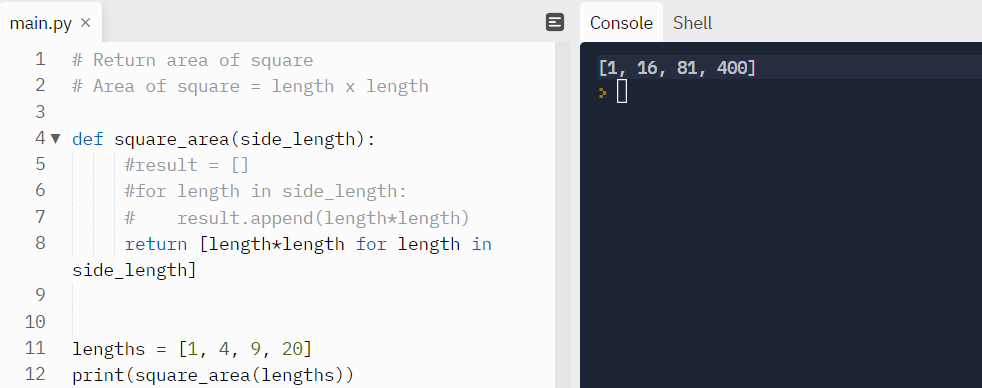
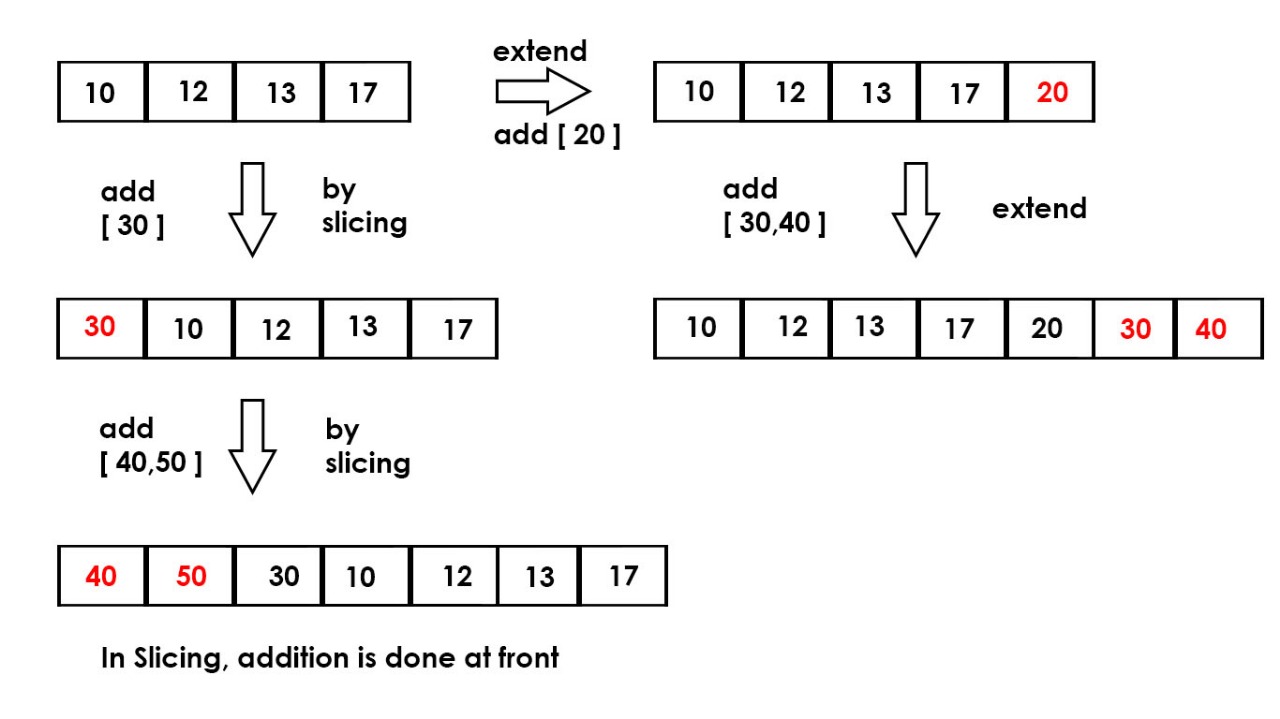
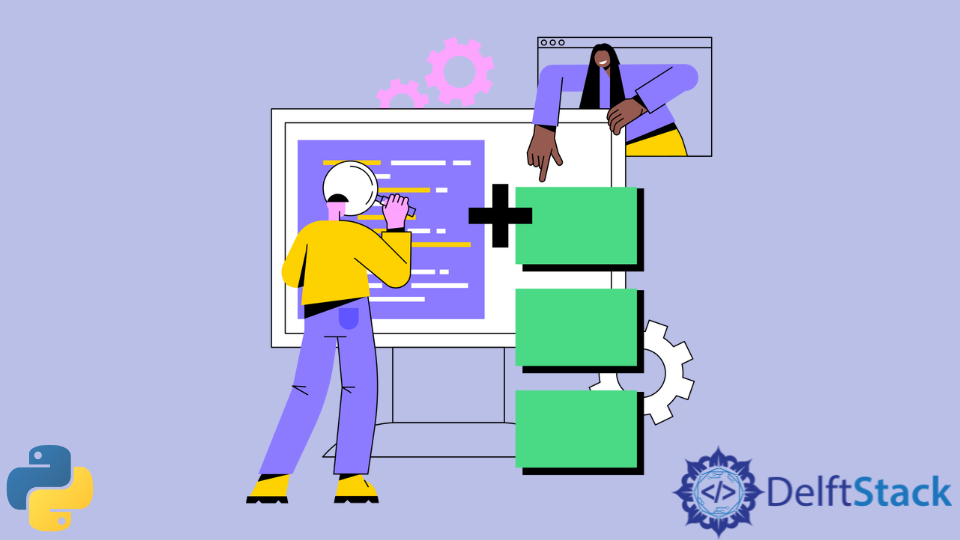
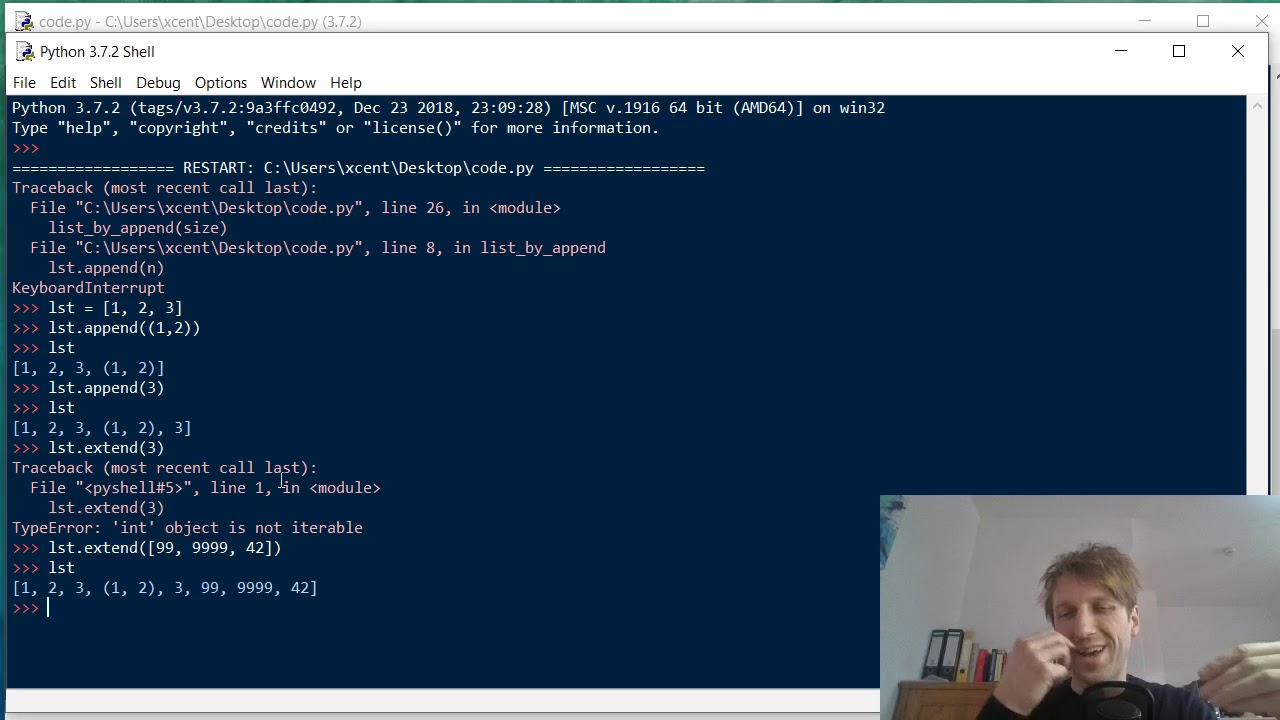
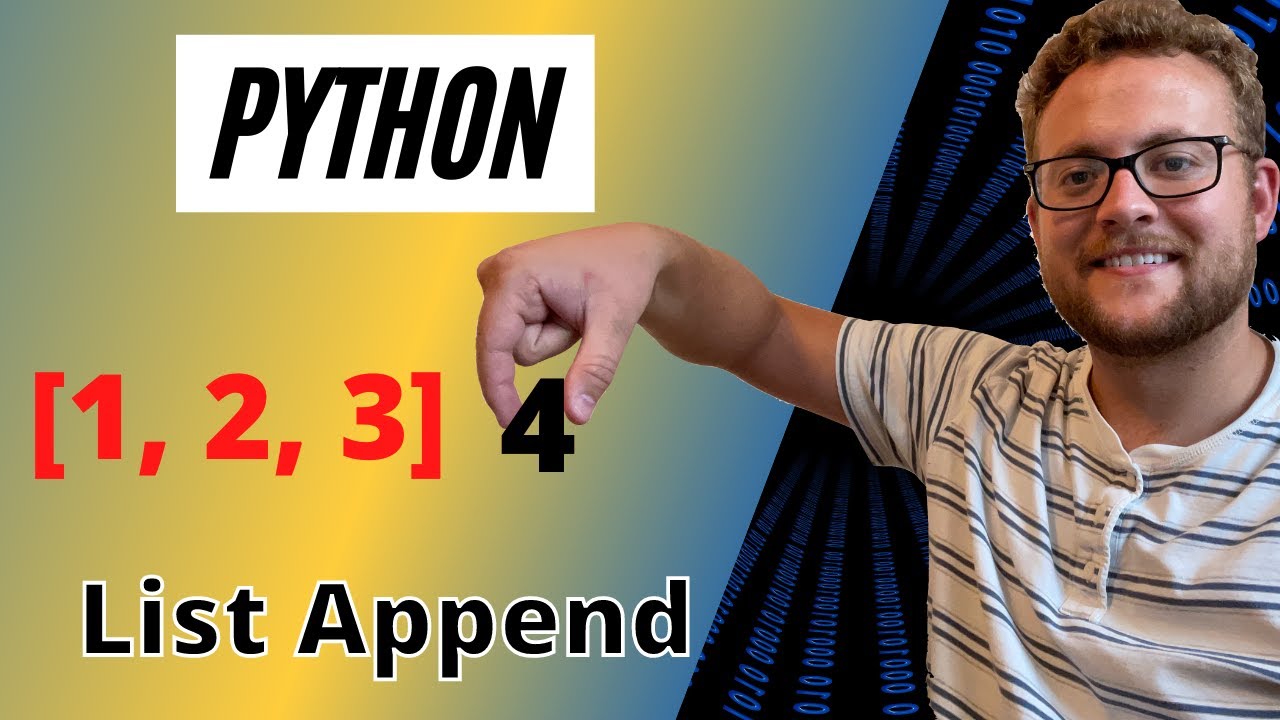




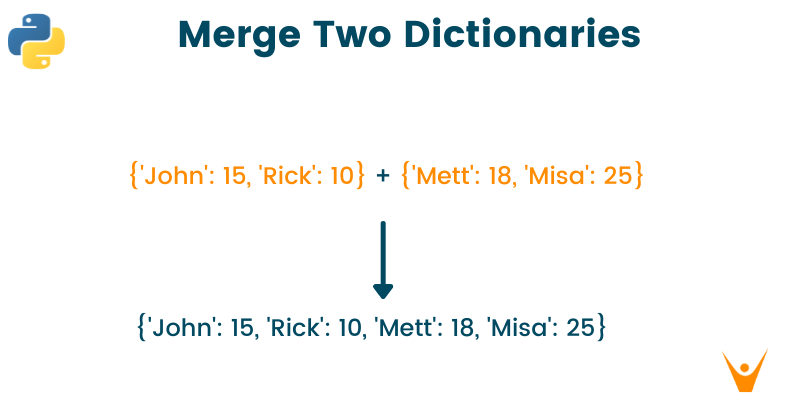
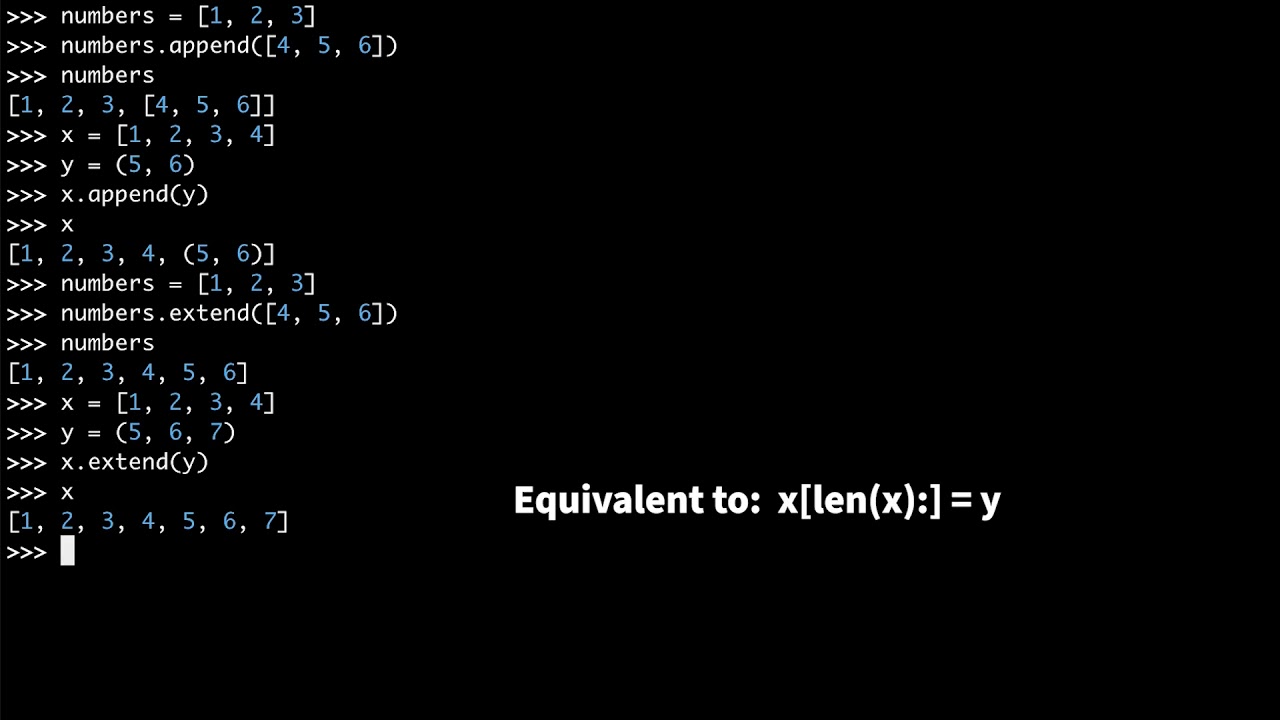
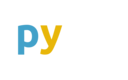
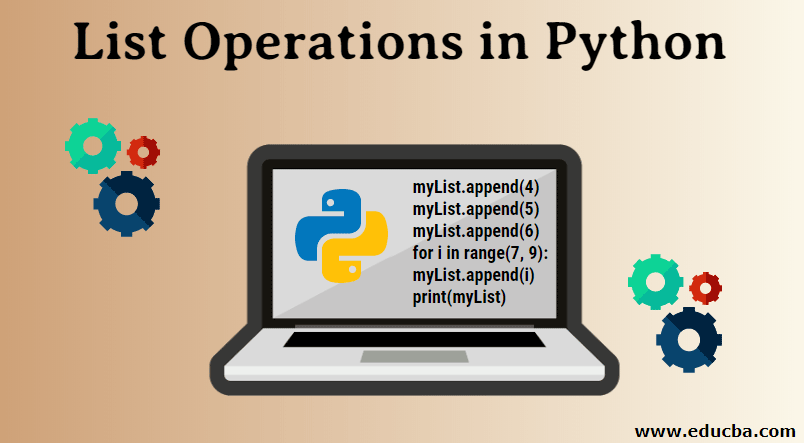


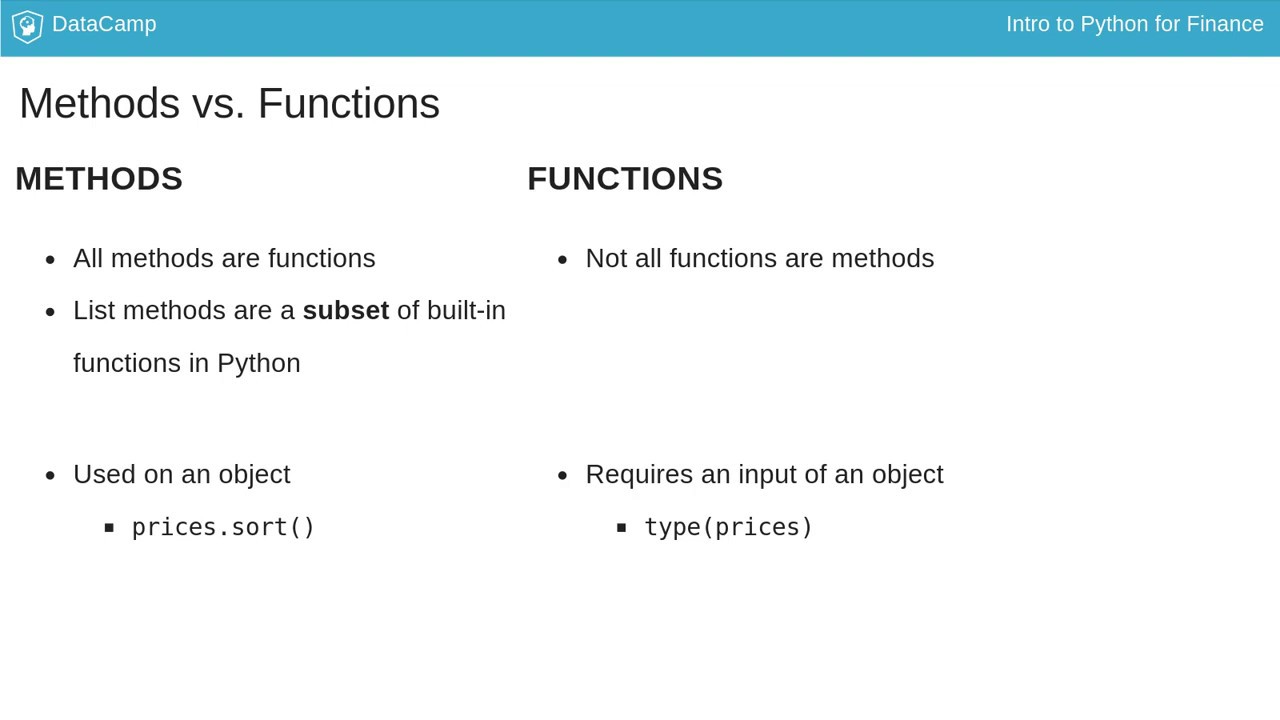
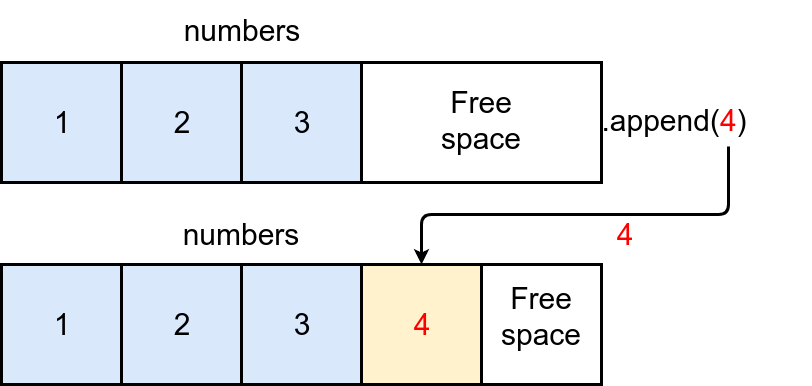

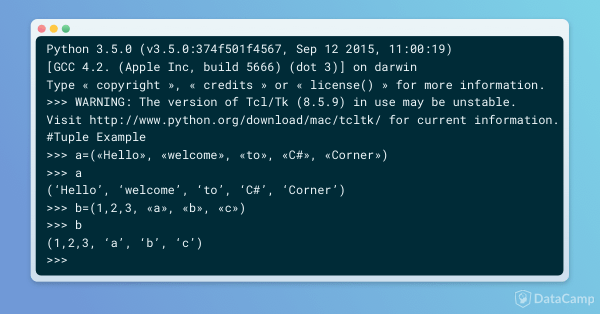

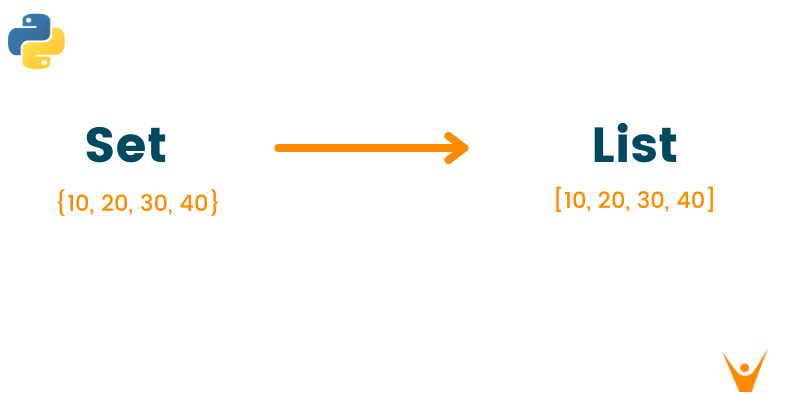

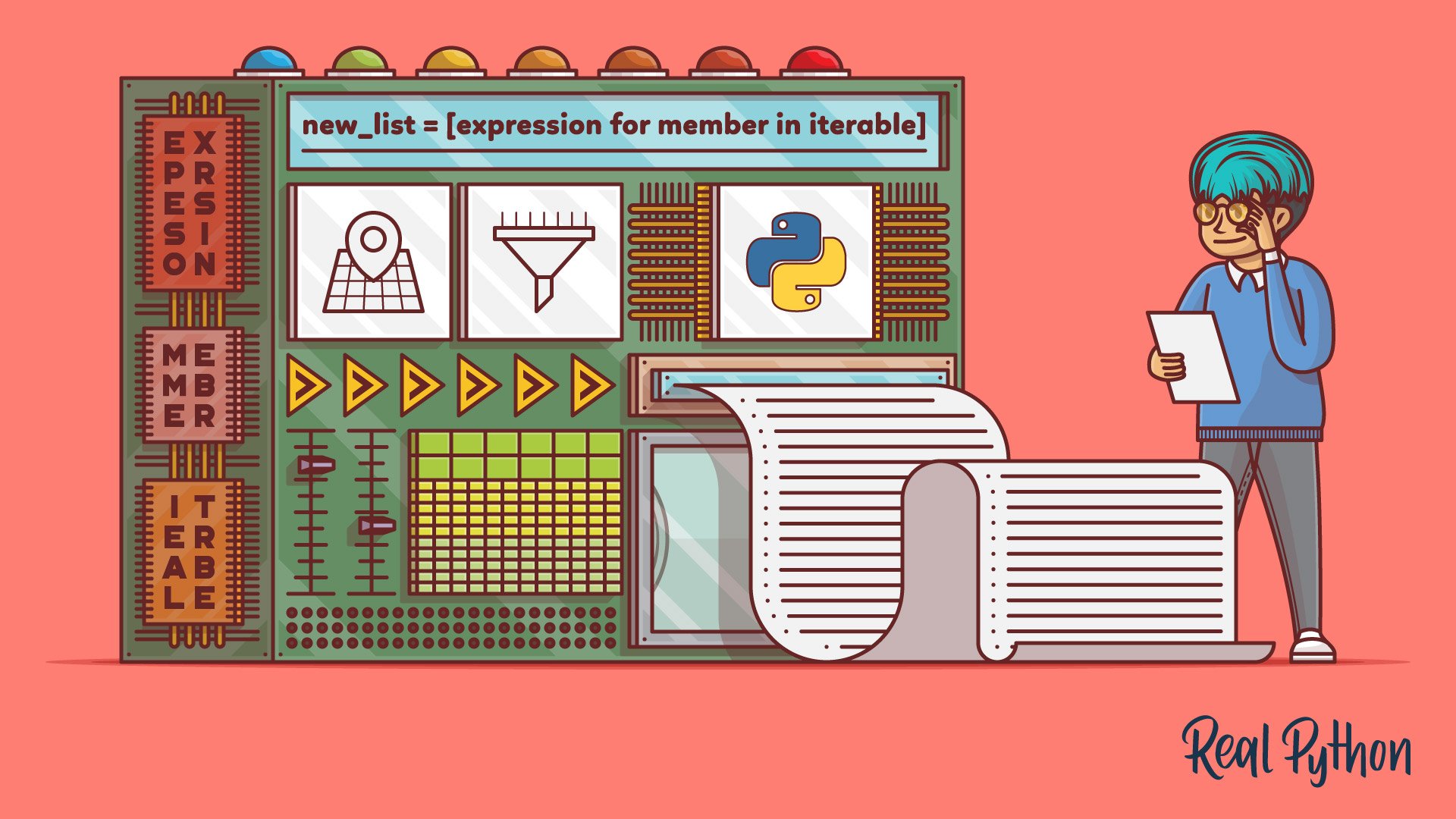
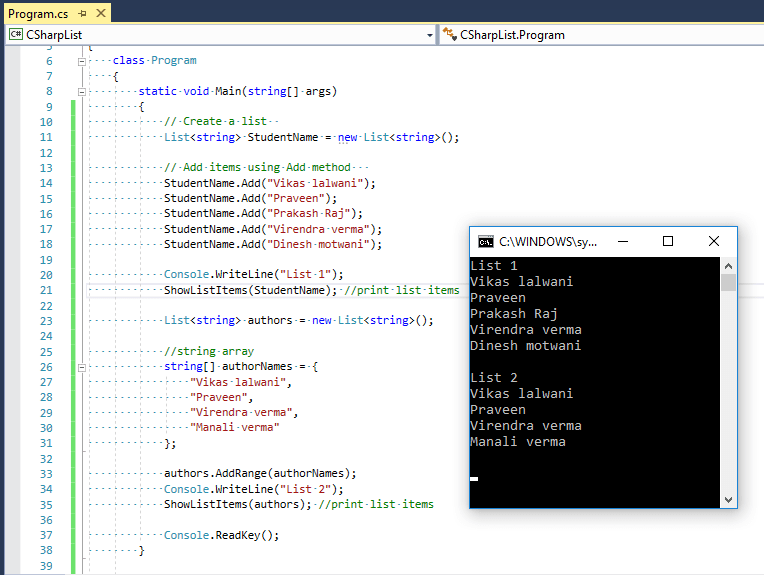
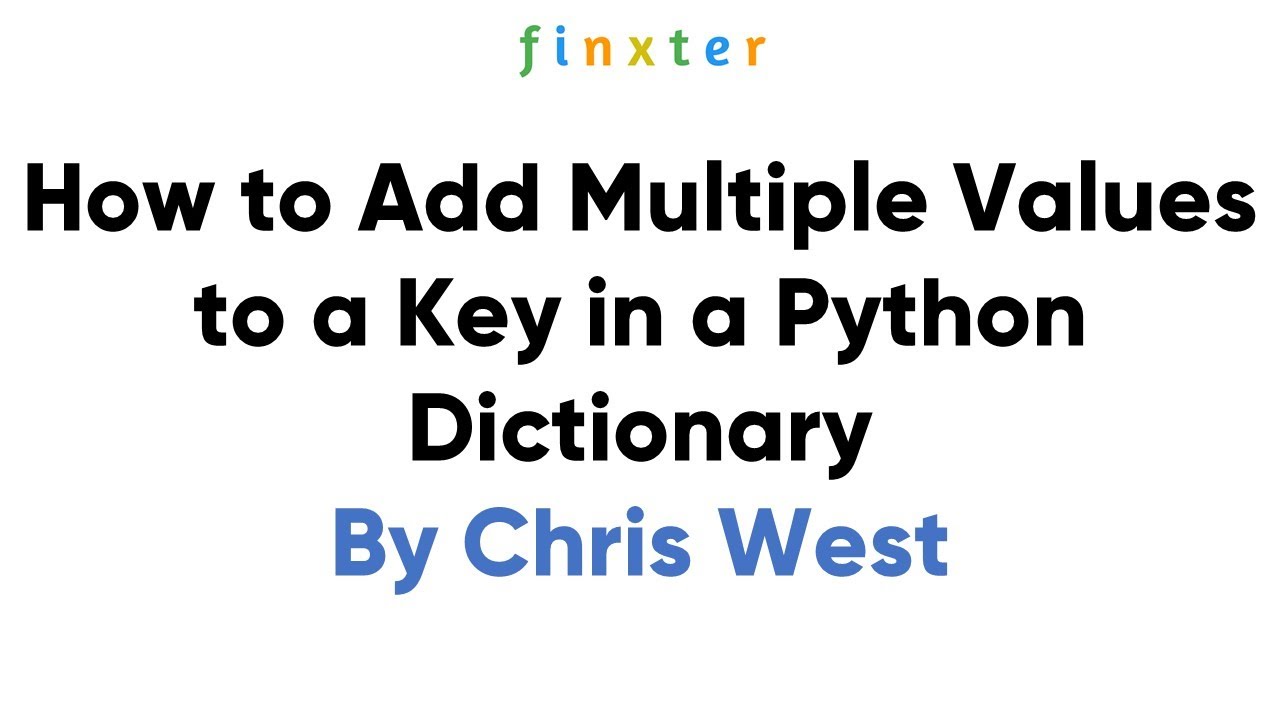

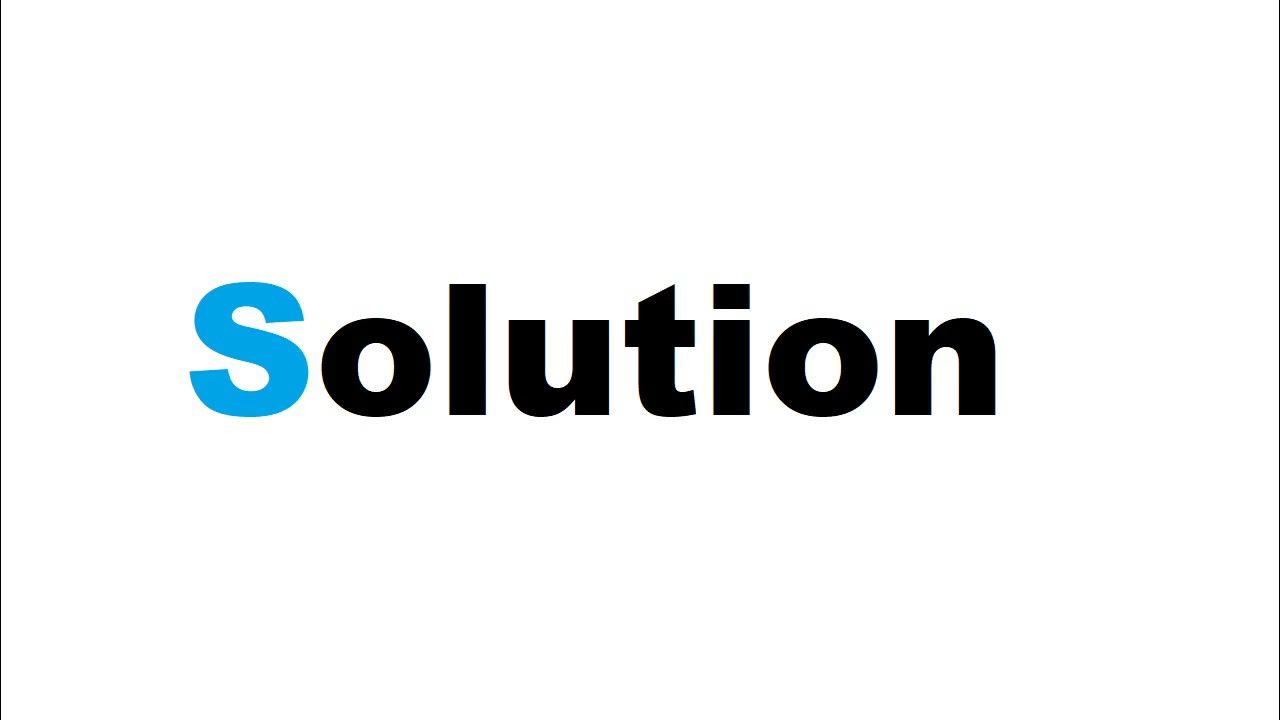


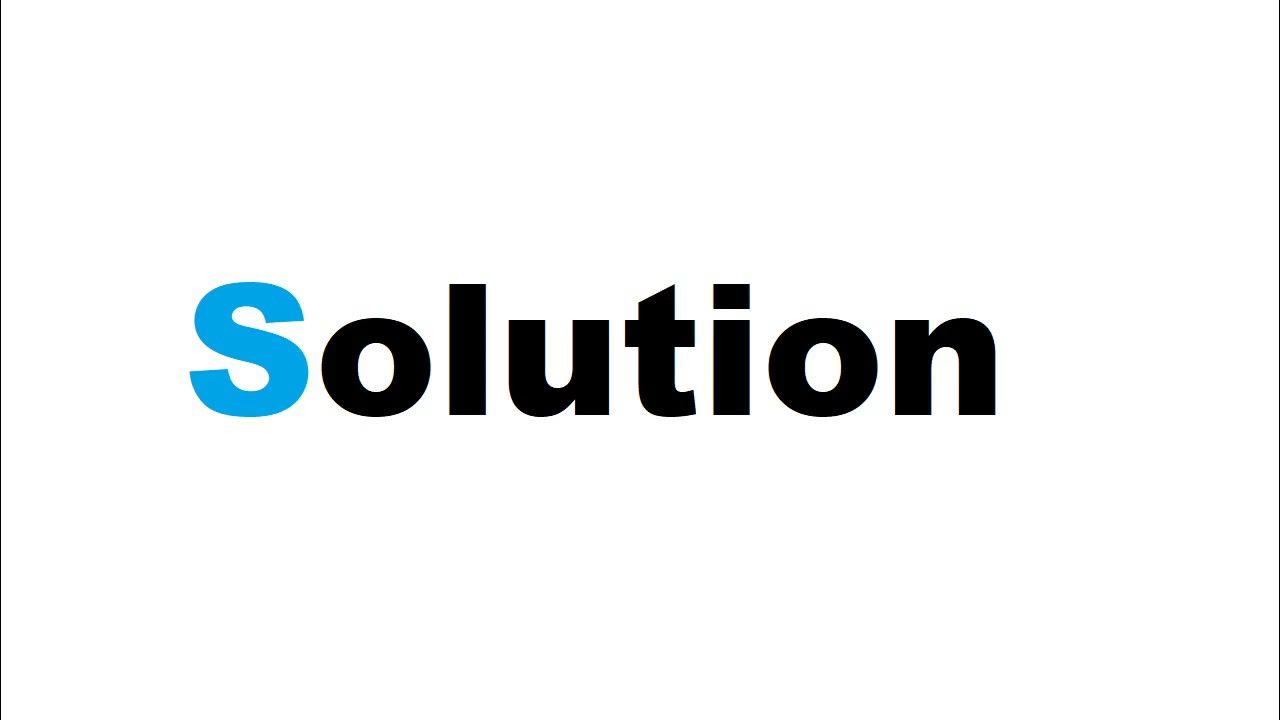
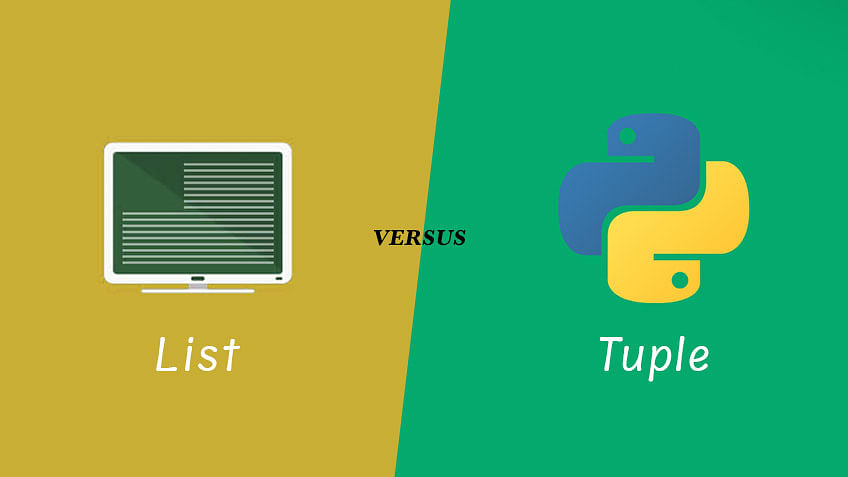
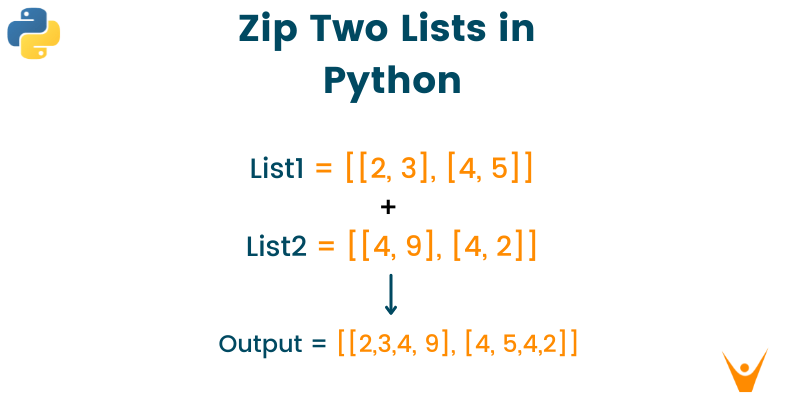
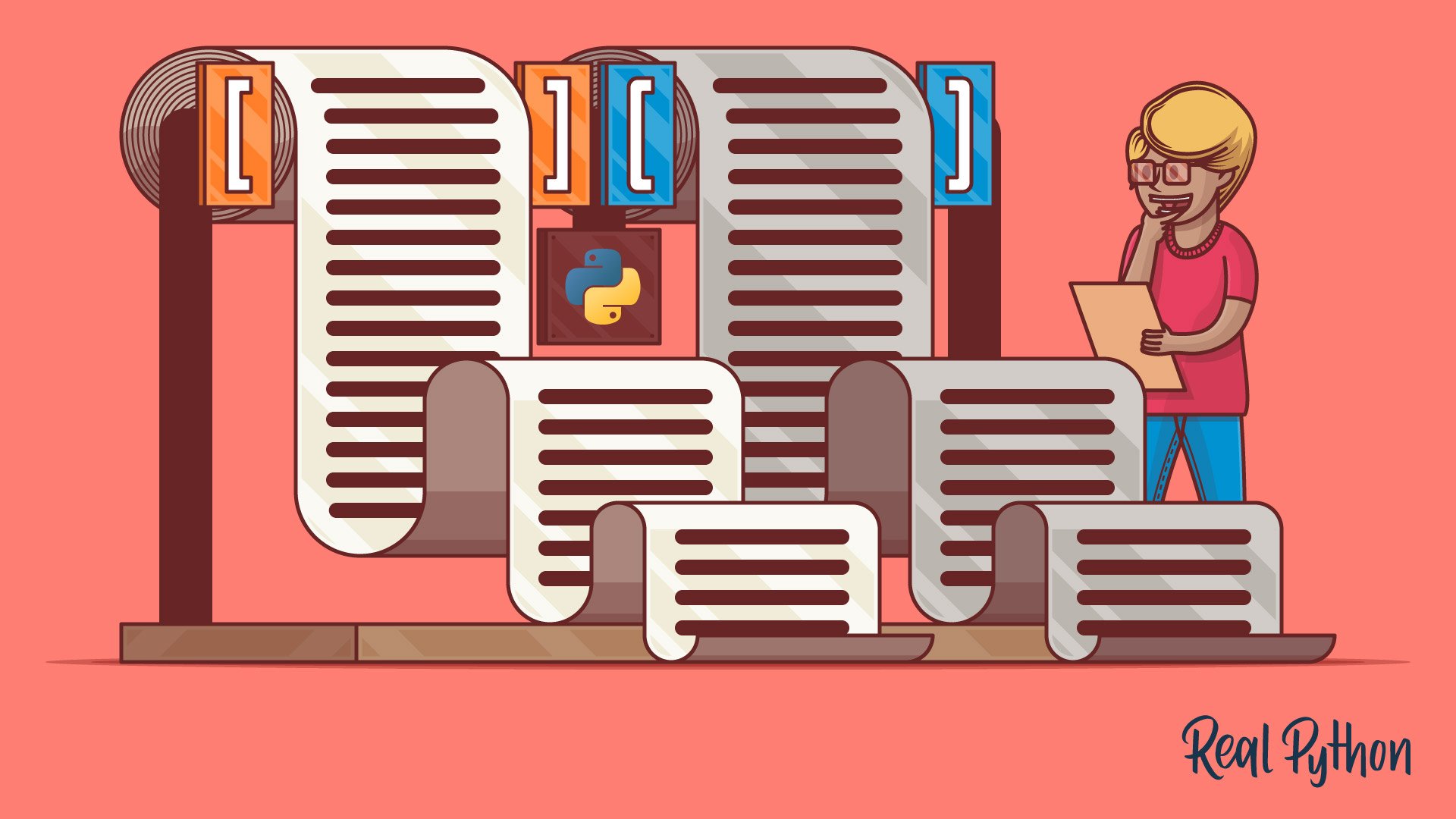

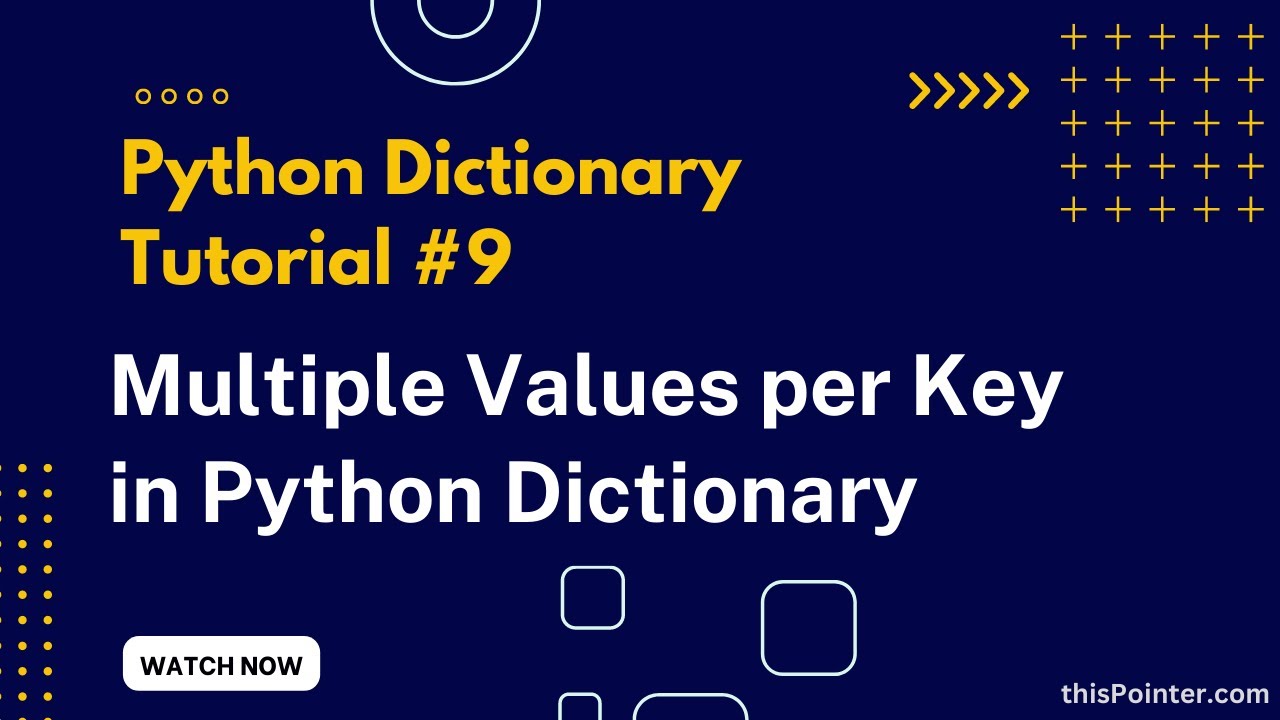
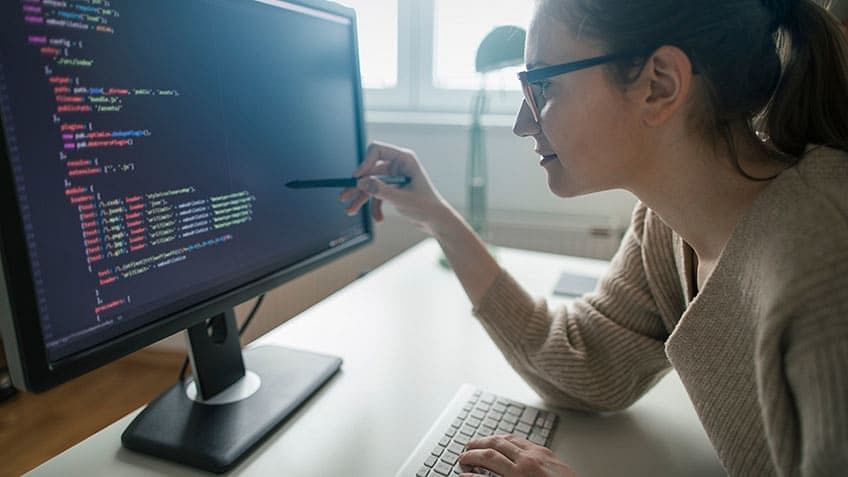
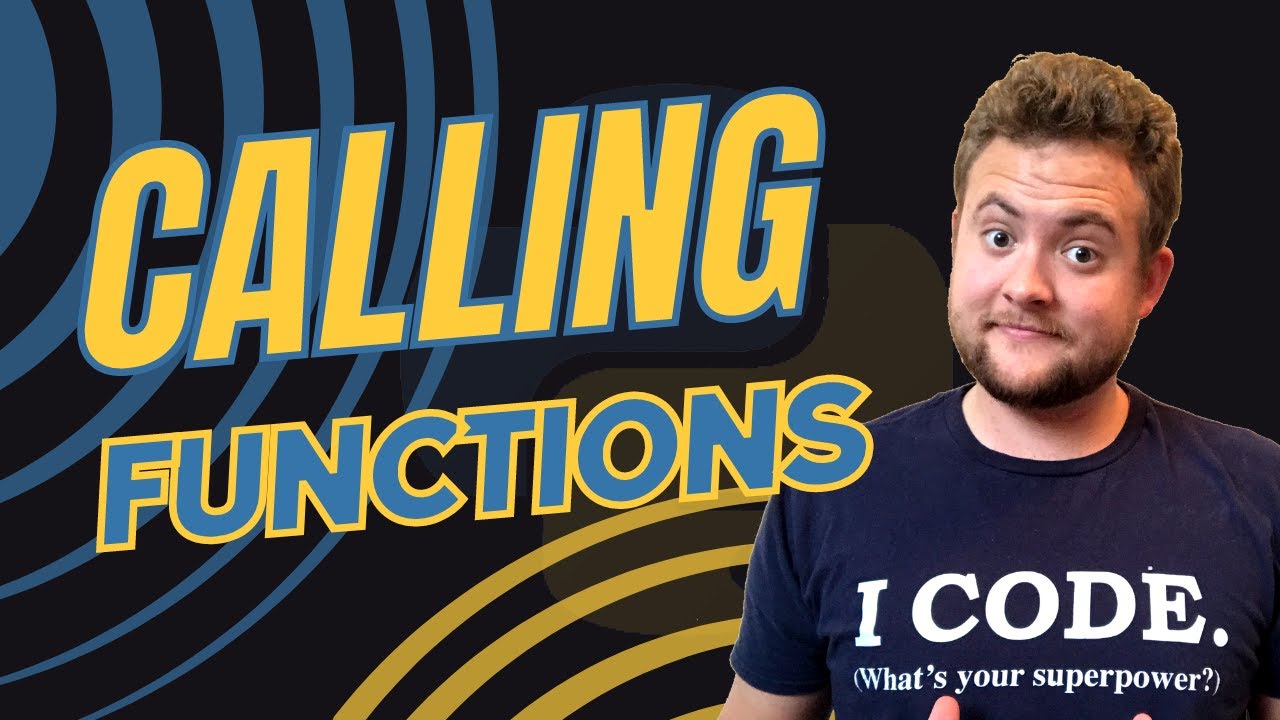
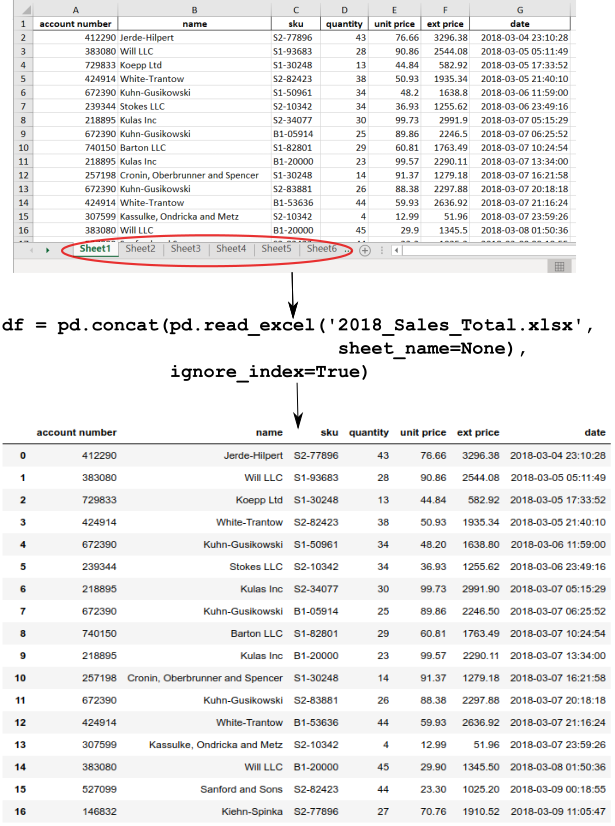
Article link: python append multiple items to list.
Learn more about the topic python append multiple items to list.
- How to append multiple values to a list in Python
- Python Append Items Elements to List – Spark By {Examples}
- Can .append(#1,#2) take two or more arguments ? | Codecademy
- Python Append Items Elements to List – Spark By {Examples}
- How to append multiple Values to a List in Python – bobbyhadz
- How to Append Multiple Items to List at Once in Python
- How to Append Multiple Items to List in Python – PyTutorial
- Python Tutorial: How to append multiple items to a list in Python
- How To add Elements to a List in Python – DigitalOcean
- Append Multiple Elements to List in Python | Delft Stack
- Python List Extend: Append Multiple Items to a List – Datagy
- Python Append Multiple Items To List – AppDividend
See more: nhanvietluanvan.com/luat-hoc