Python Check If Key In Dictionary
In Python, dictionaries are essential data structures that store key-value pairs. Often, we need to check if a specific key exists in a dictionary before performing any operations on it. In this article, we will explore various ways to check if a key exists in a dictionary, along with some common scenarios and frequently asked questions.
1. Using the ‘in’ Operator:
The simplest way to check if a key exists in a dictionary is by using the ‘in’ operator. It returns a boolean value, True if the key exists, and False otherwise. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict:
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
2. Using the ‘get()’ Method:
The ‘get()’ method can be utilized to check if a key exists in a dictionary. It returns the value associated with the given key if it exists, otherwise it returns None. We can leverage this behavior to determine if a key exists. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.get(‘name’) is not None:
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
3. Using the ‘keys()’ Method:
The ‘keys()’ method returns a view object that holds the keys of the dictionary. To check if a key exists, we can convert the view object into a list and then use the ‘in’ operator. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in list(my_dict.keys()):
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
4. Using the ‘has_key()’ Function (deprecated in Python 3):
In older versions of Python (2.x), the ‘has_key()’ function was available to check if a key exists in a dictionary. However, it has been deprecated in Python 3 and should not be used anymore. It is recommended to use the ‘in’ operator instead.
5. Using Exception Handling with ‘try-except’ Block:
Another approach to check if a key exists in a dictionary is by using exception handling with a ‘try-except’ block. We can try to access the key and catch the ‘KeyError’ if it doesn’t exist. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
value = my_dict[‘name’]
print(“Key ‘name’ exists in the dictionary”)
except KeyError:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
6. Using the ‘in’ Operator with List Comprehension:
List comprehension can be used to create a list of all keys in the dictionary and then check if the desired key exists in that list using the ‘in’ operator. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in [key for key in my_dict]:
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
FAQs:
Q: How can I check if a key does not exist in a dictionary in Python?
A: You can use any of the mentioned methods, such as the ‘not in’ operator, the ‘get()’ method (which returns None if the key doesn’t exist), or exception handling with a ‘try-except’ block.
Q: How can I print all keys in a dictionary in Python?
A: You can use the ‘keys()’ method to get a view object of all keys in the dictionary. You can then convert this view object into a list and print it.
Q: How can I find an element in a dictionary in Python?
A: You can use any of the methods mentioned above to check if a specific key exists in the dictionary. If it does, you can access its corresponding value. If not, you can handle the situation accordingly.
Q: Can I check if a key exists in an array in Python?
A: No, dictionaries and arrays are different data structures in Python. The methods mentioned in this article are specifically for dictionaries. For arrays, you can check if an element exists using the ‘in’ operator or list comprehension.
Q: How can I check if a value exists in a dictionary in Python?
A: To check if a value exists in a dictionary, you can use the ‘in’ operator with the ‘values()’ method. For example, `if value in my_dict.values():`.
Q: What is a dictionary in Python?
A: A dictionary is an unordered collection of key-value pairs. It is mutable, iterable, and can contain elements of different data types.
Q: How can I iterate over key-value pairs in a dictionary in Python?
A: You can use the ‘items()’ method to get a view object of all key-value pairs in a dictionary. Then, you can iterate over this view object using a loop or list comprehension.
In conclusion, there are several ways to check if a key exists in a dictionary in Python. The ‘in’ operator, ‘get()’ method, ‘keys()’ method, exception handling, and list comprehension are all viable options. Choose the method that suits your specific needs and ensure the accuracy and efficiency of your code.
Python: Check If Value Exists In Dictionary
How To Check If Key Is Valid In Dictionary Python?
Python is a widely used and versatile programming language known for its simplicity and readability. One of its powerful built-in data structures is the dictionary. Dictionaries are unordered collections of key-value pairs, where each key is unique. They offer a convenient way of storing and accessing data, making them an essential tool for many Python developers.
When utilizing dictionaries, it is often necessary to perform key existence checks to avoid unexpected errors or exceptions. Therefore, understanding how to check if a key is valid in a Python dictionary is crucial. In this article, we will explore various methods to accomplish this, ensuring that you can efficiently handle dictionary operations.
Checking for Key Existence using the ‘in’ Operator:
The simplest method to verify whether a key exists in a dictionary is by using the ‘in’ operator. This operator checks if the specified key is present in the dictionary and returns a Boolean value, indicating whether the key is valid or not. Let’s look at a simple example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘orange’: 3}
if ‘apple’ in my_dict:
print(“Key ‘apple’ exists in the dictionary.”)
else:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we start by defining a dictionary called ‘my_dict’ that contains a few key-value pairs. Next, we use the ‘in’ operator to check if the key ‘apple’ exists in the dictionary. If the key is present, it will print “Key ‘apple’ exists in the dictionary”; otherwise, it will print “Key ‘apple’ does not exist in the dictionary.”
Using the get() Method:
Another elegant way to determine if a key is valid in a Python dictionary is by using the get() method. This method allows you to specify a default value to return if the key is not found, preventing any potential errors. Take a look at the following example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘orange’: 3}
result = my_dict.get(‘apple’, ‘Key not found.’)
print(result)
“`
In this code snippet, we use the get() method on ‘my_dict’ to check if the key ‘apple’ exists. If the key is found, the corresponding value (1 in this case) is returned. However, if the key is not present, the provided default value ‘Key not found.’ will be returned instead. The output in this case will be 1, as the key ‘apple’ exists in the dictionary.
Using exception handling with the try-except block:
Handling exceptions is a fundamental practice in Python programming. By using the try-except block, we can gracefully handle errors that may arise when accessing dictionary keys. Let’s consider the following code snippet:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘orange’: 3}
try:
result = my_dict[‘apple’]
print(“Key ‘apple’ exists in the dictionary.”)
except KeyError:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we attempt to access the value corresponding to the key ‘apple’ directly using square brackets. If the key exists, the corresponding value will be assigned to ‘result’, and the output will be “Key ‘apple’ exists in the dictionary.” However, if the key is not found, a KeyError exception will be raised. By using the except block, we can handle this exception and print “Key ‘apple’ does not exist in the dictionary.”
FAQs:
Q: How can I check if a key is not valid in a Python dictionary?
A: You can use the ‘not in’ operator in a similar way to the ‘in’ operator. For example:
“`python
if ‘mango’ not in my_dict:
print(“Key ‘mango’ does not exist in the dictionary.”)
“`
Q: Can I check if multiple keys exist in a dictionary at once?
A: Yes, you can check if multiple keys exist simultaneously by iterating over them. Here’s an example:
“`python
keys_to_check = [‘apple’, ‘banana’, ‘mango’]
for key in keys_to_check:
if key not in my_dict:
print(f”Key ‘{key}’ does not exist in the dictionary.”)
“`
Q: Which method should I use to check if a key is valid in a dictionary: ‘in’ operator, get() method, or exception handling?
A: The choice depends on the specific use case. If you simply want to check if a key exists without raising an exception, using the ‘in’ operator is the most straightforward approach. The get() method is useful when you want to handle non-existent keys gracefully by providing default values. Exception handling is ideal if you want to perform specific actions when a key does not exist or if you need to access the value immediately.
In conclusion, checking if a key is valid in a Python dictionary is a common task that can be accomplished using various methods. The ‘in’ operator, get() method, and exception handling with the try-except block provide flexible approaches based on your specific needs. By understanding and utilizing these techniques effectively, you can avoid unexpected errors and effectively manage dictionary operations in your Python programs.
How To Check If Variable Is Dictionary In Python?
Python is a dynamically typed language, meaning that variables can hold different types of data throughout the execution of a program. This flexibility is advantageous, but it can also create the need for type-checking in certain scenarios. One common situation is when we want to determine whether a variable is a dictionary or not. In this article, we will explore various methods to check if a variable is a dictionary in Python and examine their pros and cons.
Method 1: isinstance()
The isinstance() function is a built-in Python function used to determine whether an object belongs to a particular class or its subclass. It takes two arguments: the object we want to check, and the class (or tuple of classes) we want to compare against.
Here is an example of using isinstance() to check if a variable is a dictionary:
“`python
my_var = { ‘name’: ‘John’, ‘age’: 30 }
if isinstance(my_var, dict):
print(“The variable is a dictionary”)
else:
print(“The variable is not a dictionary”)
“`
The isinstance() function returns True if my_var is of the dict class (or a subclass of dict), and False otherwise.
Pros:
– It is a simple and straightforward method.
– The isinstance() function can also be used to check if a variable is an instance of a custom dictionary subclass.
Cons:
– The isinstance() function can be slow when dealing with large data sets, as it needs to traverse the inheritance hierarchy of the given object.
Method 2: type()
The type() function in Python returns the type of an object. We can use it to check if a variable is a dictionary.
Here is an example:
“`python
my_var = { ‘name’: ‘John’, ‘age’: 30 }
if type(my_var) == dict:
print(“The variable is a dictionary”)
else:
print(“The variable is not a dictionary”)
“`
The type() function returns the type of my_var, and we compare it to the dict type using the equality operator.
Pros:
– It is a simple and minimalistic approach.
Cons:
– Using type() can be less flexible compared to isinstance(), especially when dealing with subclasses.
Method 3: __class__
In Python, every object has a special attribute __class__ that refers to its class. We can use this attribute to determine if a variable is a dictionary.
Here is an example:
“`python
my_var = { ‘name’: ‘John’, ‘age’: 30 }
if my_var.__class__ == dict:
print(“The variable is a dictionary”)
else:
print(“The variable is not a dictionary”)
“`
Here, we directly access the __class__ attribute of my_var and compare it to the dict class.
Pros:
– It provides a direct way to access an object’s class.
Cons:
– Accessing special attributes like __class__ can be frowned upon in Python, as it goes against the concept of encapsulation.
FAQs:
Q: Can a variable belong to multiple classes in Python?
A: No, a variable can only belong to a single class. However, a class can inherit from multiple base classes.
Q: Are dictionaries the only way to store key-value pairs in Python?
A: No, Python provides other data structures, such as OrderedDict and defaultdict, for storing key-value pairs.
Q: Can I modify the __class__ attribute of an object?
A: Yes, you can modify the __class__ attribute of an object dynamically in Python. However, it is generally not recommended unless you have a specific reason to do so.
Q: How can we check if a variable is an empty dictionary?
A: To check if a variable is an empty dictionary, you can use the following condition: `if my_var == {}:`
In conclusion, verifying if a variable is a dictionary in Python can be accomplished using several methods, such as isinstance(), type(), or accessing the __class__ attribute. Each approach has its advantages and disadvantages, so it is essential to choose the most appropriate method based on your specific requirements.
Keywords searched by users: python check if key in dictionary Check if key not exists in dictionary Python, Print key in dictionary Python, Find element in dictionary Python, Python check key in array, Check value in dictionary Python, Dict in Python, For key-value in dict Python, Python check if object has key
Categories: Top 94 Python Check If Key In Dictionary
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
Introduction:
In Python, dictionaries are a powerful data structure used to store a collection of key-value pairs. As developers, it is crucial to know how to handle scenarios where we need to check if a specific key does not exist within a dictionary. In this article, we will explore various approaches to determine the absence of a key in a dictionary in Python and provide insights into using these techniques effectively.
Methods to Check if a Key Does Not Exist in a Dictionary:
Method 1: Using the ‘in’ Operator
Python provides the ‘in’ operator that allows us to check if a key exists in a dictionary. We can negate this operation with the ‘not’ keyword, which will return True if the key is not found. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘weight’ not in my_dict:
print(“Key does not exist!”)
“`
Method 2: Using the ‘get()’ Method
The ‘get()’ method is another approach to determine whether a key does not exist in a dictionary. If the specified key is absent, this method returns a default value of our choice. By checking the returned value against the default, we can verify if the key is missing. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
weight = my_dict.get(‘weight’, None)
if weight is None:
print(“Key does not exist!”)
“`
Method 3: Using ‘try-except’ Block
We can also use exception handling to check if a key does not exist in a dictionary. By attempting to access the key using the ‘[]’ notation and catching the ‘KeyError’ exception, we can conclude that the key is not present. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
weight = my_dict[‘weight’]
except KeyError:
print(“Key does not exist!”)
“`
Method 4: Using the ‘not’ Keyword with ‘dict.get()’
An alternative approach is to combine the ‘not’ keyword with the ‘dict.get()’ method. This technique returns ‘None’ if the key is not present. By simply checking if the returned value is ‘None’, we can determine whether the key exists in the dictionary. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.get(‘weight’) is None:
print(“Key does not exist!”)
“`
FAQs:
Q1. Can we use these methods to check if a key does not exist in nested dictionaries?
Yes, these methods work just as well with dictionaries nested inside other dictionaries. You can navigate through the nested structure by chaining the appropriate key checks or using recursion.
Q2. What happens if we try to access a nonexistent key directly without any of these methods?
If you attempt to access a nonexistent key directly using the ‘[]’ notation, it will raise a ‘KeyError’ exception. Therefore, it is recommended to use one of the methods mentioned above to handle key existence checks to avoid program termination.
Q3. Is there a preferred method for checking if a key does not exist in a dictionary?
The choice of method depends on the specific use case and personal preference. Some developers prefer the ‘in’ operator or ‘get()’ method, while others find the ‘try-except’ block more readable and precise. Consider the context and requirements of your application before deciding on the method.
Q4. Is it possible to check the absence of multiple keys simultaneously?
Yes, all the methods discussed in this article can be used to check the absence of multiple keys. Simply combine multiple key checks using logical operators like ‘and’ or ‘or’.
Q5. What if we want to retrieve a default value if a key is not present?
In situations where you want to retrieve a default value in case a key does not exist, the ‘get()’ method with a default value parameter is an excellent choice. Simply specify the default value as the second argument when invoking the method.
Conclusion:
Checking if a key does not exist in a Python dictionary is a common scenario when working with data structures. By using methods like the ‘in’ operator, ‘get()’, ‘try-except’ blocks, or the ‘not’ keyword, we can efficiently handle cases where the presence or absence of keys plays a significant role in decisions and program flow. Understanding these techniques and selecting the most appropriate one for your use case will ensure code accuracy and efficiency in Python programming.
Print Key In Dictionary Python
As we all know, a dictionary in Python is an unordered collection of elements that are stored as key-value pairs. Each key in a dictionary must be unique, while values can be of any data type. Accessing the keys and values from a dictionary is a fundamental operation, and the print key function simplifies this process by displaying the desired output.
The syntax for printing a dictionary key involves using the built-in print() function in combination with the .keys() method. The .keys() method returns a list-like object containing all the keys in the dictionary.
Let’s take a look at an example to better understand the implementation of the print key function:
“`python
my_dict = {1: ‘apple’, 2: ‘banana’, 3: ‘orange’}
print(my_dict.keys())
“`
Output:
“`
dict_keys([1, 2, 3])
“`
In the above example, we create a dictionary called `my_dict` with three key-value pairs. By using the `.keys()` method, we can print all the keys present in the dictionary. The output shows the list-like object `dict_keys` containing the keys `[1, 2, 3]`.
To further enhance the functionality of the print key method, we can iterate through the keys using a for loop:
“`python
my_dict = {1: ‘apple’, 2: ‘banana’, 3: ‘orange’}
for key in my_dict.keys():
print(key)
“`
Output:
“`
1
2
3
“`
In this example, we iterate through each key in the dictionary using the for loop and print each key on a separate line. This allows us to have more control over the format of the output.
Additionally, the print key function can be used to access and print the corresponding values of the keys. To achieve this, we can combine the .keys() method with a square bracket notation:
“`python
my_dict = {1: ‘apple’, 2: ‘banana’, 3: ‘orange’}
for key in my_dict.keys():
print(key, my_dict[key])
“`
Output:
“`
1 apple
2 banana
3 orange
“`
In the above code snippet, we use the square bracket notation `my_dict[key]` to access the value associated with a particular key. By printing both the key and its corresponding value in each iteration of the loop, we obtain an output that displays both the keys and their values.
Now, let’s address some frequently asked questions related to the print key in dictionary Python:
Q: Can the print key function be used with nested dictionaries?
A: Yes, the print key function can be used with nested dictionaries. By using nested for loops, we can iterate through the keys of each nested dictionary and print them accordingly.
Q: Is the order of the keys preserved when using the print key function?
A: No, the order of the keys is not preserved when using the print key function. As dictionaries are inherently unordered, the keys may appear in a different order than they were initially defined.
Q: Can we print only specific keys from a dictionary?
A: Yes, if you want to print specific keys from a dictionary, you can use conditional statements such as `if` to filter out the desired keys. By providing specific conditions, you can selectively print the keys that meet those conditions.
In conclusion, the print key function in dictionary Python is a convenient method to access and display the keys and values stored within a dictionary. By utilizing the print() function in conjunction with the .keys() method, we can easily obtain and print the keys, their corresponding values, or both. The examples provided in this article should give you a good understanding of how the print key function can be implemented in Python.
Find Element In Dictionary Python
Python provides several approaches to find an element in a dictionary. Let’s start by considering the most straightforward method: using the get() method. The get() method allows you to retrieve the value associated with a specified key in a dictionary. If the key is present, it returns the corresponding value; otherwise, it returns a default value, which is None by default. This method is quite handy when you are unsure whether a key exists in the dictionary or not, as it avoids raising a KeyError exception.
Here’s an example to demonstrate the get() method:
“`
my_dict = {‘apple’: 3, ‘banana’: 5, ‘orange’: 2}
print(my_dict.get(‘apple’))
# Output: 3
print(my_dict.get(‘grape’))
# Output: None
print(my_dict.get(‘grape’, ‘Not found’))
# Output: Not found
“`
In the example above, we have a dictionary called `my_dict` containing the counts of various fruits. We use the `get()` method to retrieve the value associated with the key `’apple’`, which returns `3`, the number of apples in the dictionary. When attempting to retrieve the value for the key `’grape’`, which doesn’t exist in the dictionary, the method returns `None`. Alternatively, we can specify a custom default value as the second parameter of the `get()` method, as shown in the last line of the example.
Another approach to find an element in a dictionary is by using the `in` keyword. This keyword allows you to check whether a key exists in a dictionary or not. It returns a boolean value: `True` if the key is present and `False` otherwise.
Here’s an example to illustrate the usage of the `in` keyword:
“`python
my_dict = {‘apple’: 3, ‘banana’: 5, ‘orange’: 2}
print(‘apple’ in my_dict)
# Output: True
print(‘grape’ in my_dict)
# Output: False
“`
In this example, we use the `in` keyword to check whether the key `’apple’` exists in the dictionary `my_dict`. Since the key is present, the output is `True`. Conversely, the key `’grape’` doesn’t exist in the dictionary, so the output is `False`.
Besides the methods mentioned above, it is also possible to find elements in a dictionary using list comprehension or generator expression. These techniques are particularly useful when you want to find multiple elements given a certain condition. For instance, you may want to retrieve all the fruits with a count higher than 2.
Here’s an example that illustrates the usage of list comprehension for finding elements in a dictionary:
“`python
my_dict = {‘apple’: 3, ‘banana’: 5, ‘orange’: 2}
fruits_with_count_higher_than_two = [key for key, value in my_dict.items() if value > 2]
print(fruits_with_count_higher_than_two)
# Output: [‘apple’, ‘banana’]
“`
In this example, we use list comprehension to create a new list, `fruits_with_count_higher_than_two`, that contains all the keys for fruits whose count is higher than 2.
Now let’s address some frequently asked questions about finding elements in a dictionary:
**Q1: Can I use the `in` keyword to check if a value exists in the dictionary?**
A1: No, the `in` keyword only checks for the presence of keys in a dictionary. To check if a value exists, you would need to use other methods such as `values()`, `items()`, or convert the values to a list or set before performing the check.
**Q2: What is the most efficient method to find an element in a dictionary?**
A2: The most efficient method depends on the specific use case and the size of the dictionary. The `get()` method is generally efficient, especially when combined with a default value. However, if you need to perform multiple lookups on a large dictionary, it might be more efficient to convert the dictionary to a list or set and perform the lookups on the keys or values directly.
**Q3: What happens if I try to retrieve a non-existent key using the `get()` method without providing a default value?**
A3: If the key is not present in the dictionary and you do not provide a default value, the `get()` method will return `None` by default. It is always a good practice to handle this case and provide appropriate default values or error handling mechanisms.
In conclusion, finding an element in a dictionary in Python can be accomplished using various methods. The `get()` method and the `in` keyword are commonly used to retrieve elements based on their keys. Alternatively, list comprehension or generator expression can be employed to find elements based on specific conditions. Understanding the advantages and disadvantages of each method allows you to choose the most appropriate approach for your particular use case.
Images related to the topic python check if key in dictionary
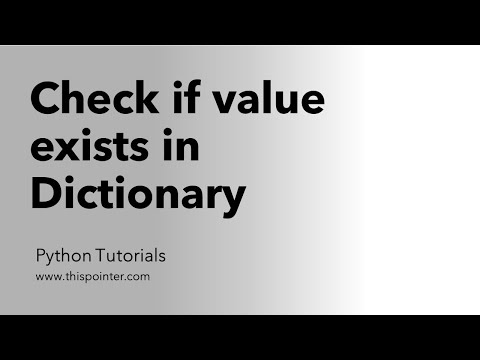
Found 17 images related to python check if key in dictionary theme
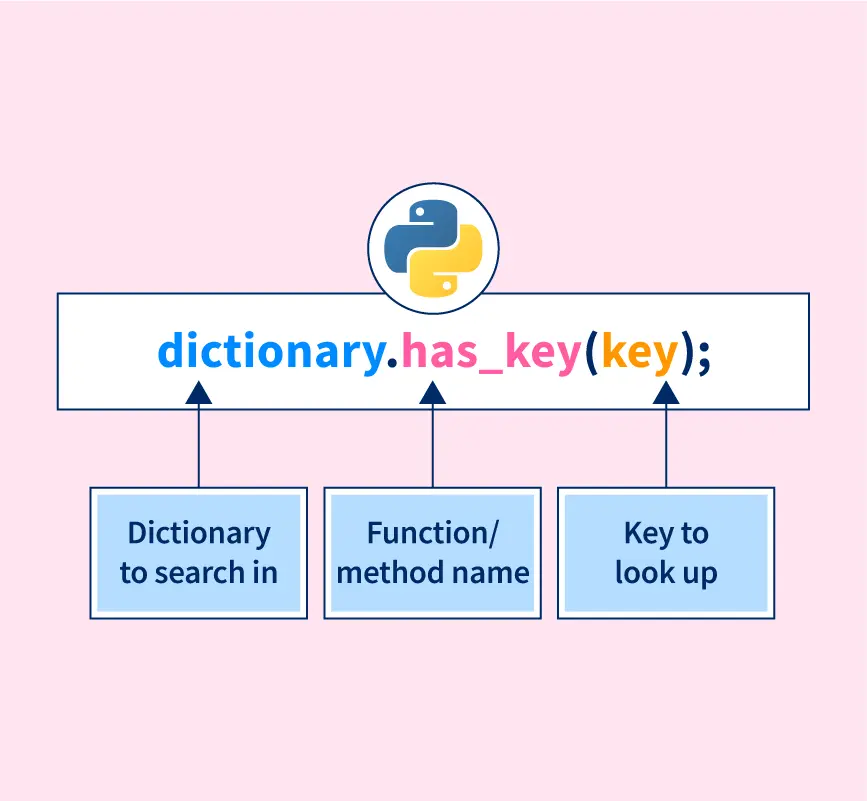
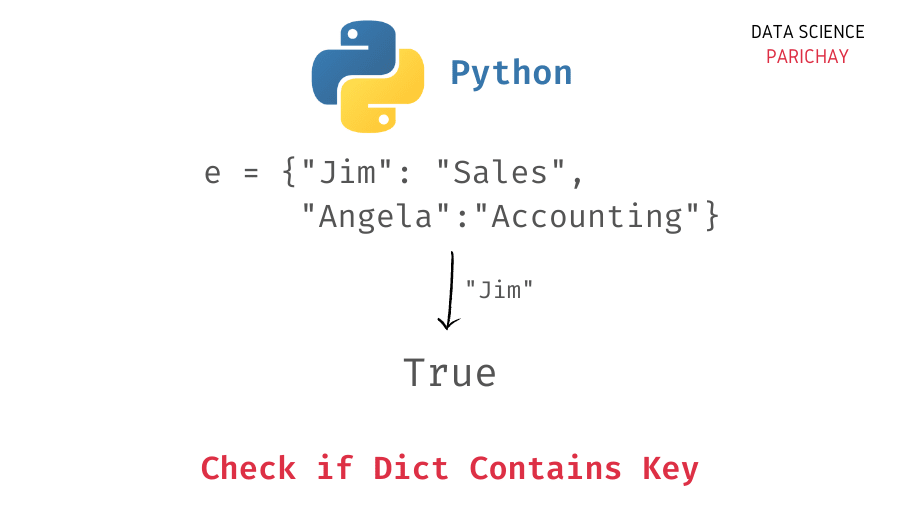
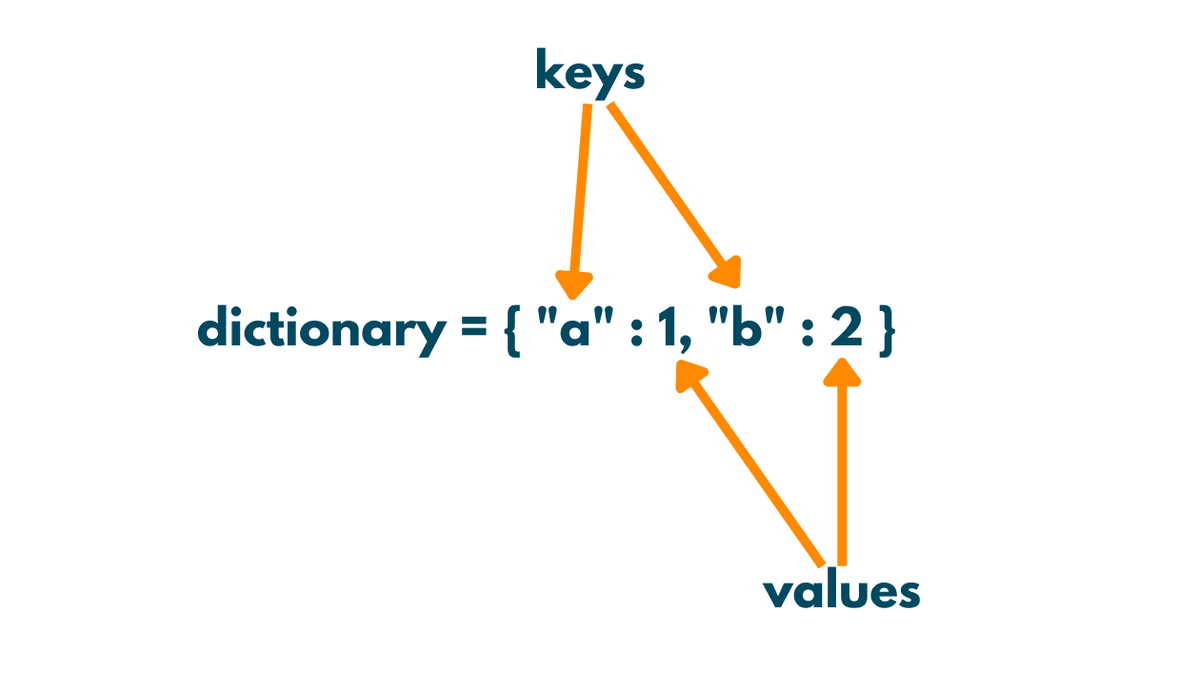
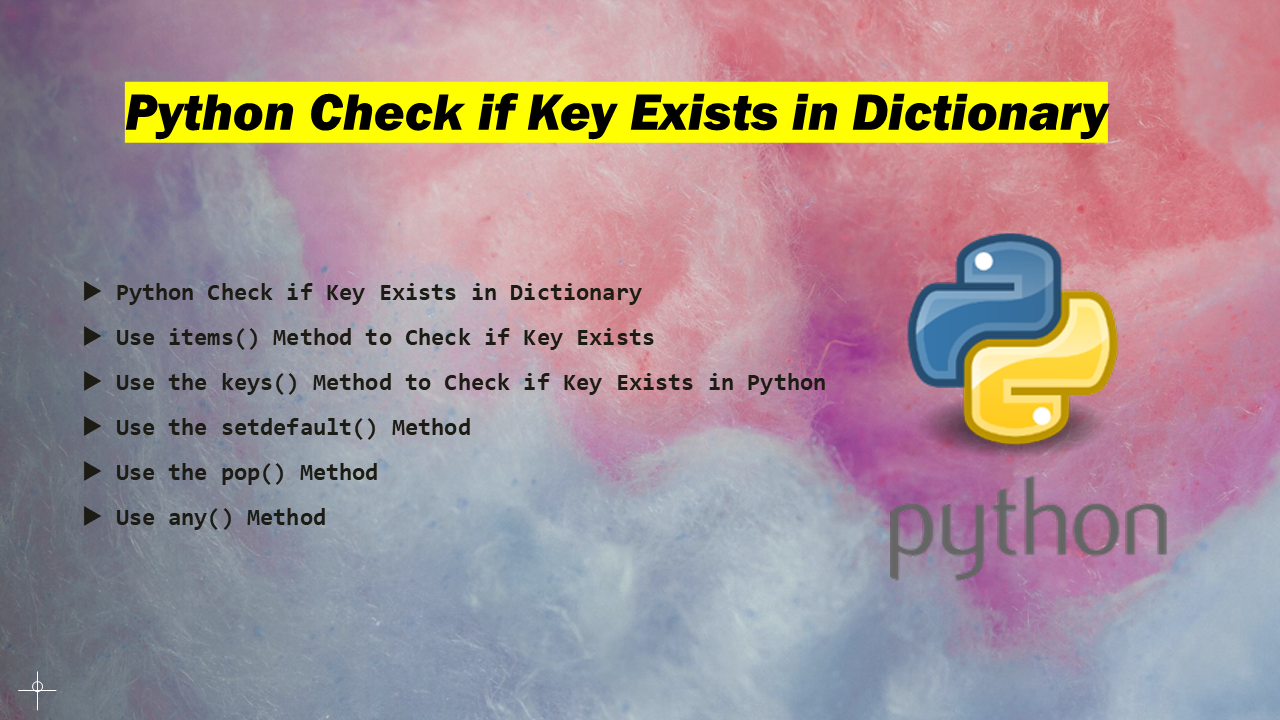

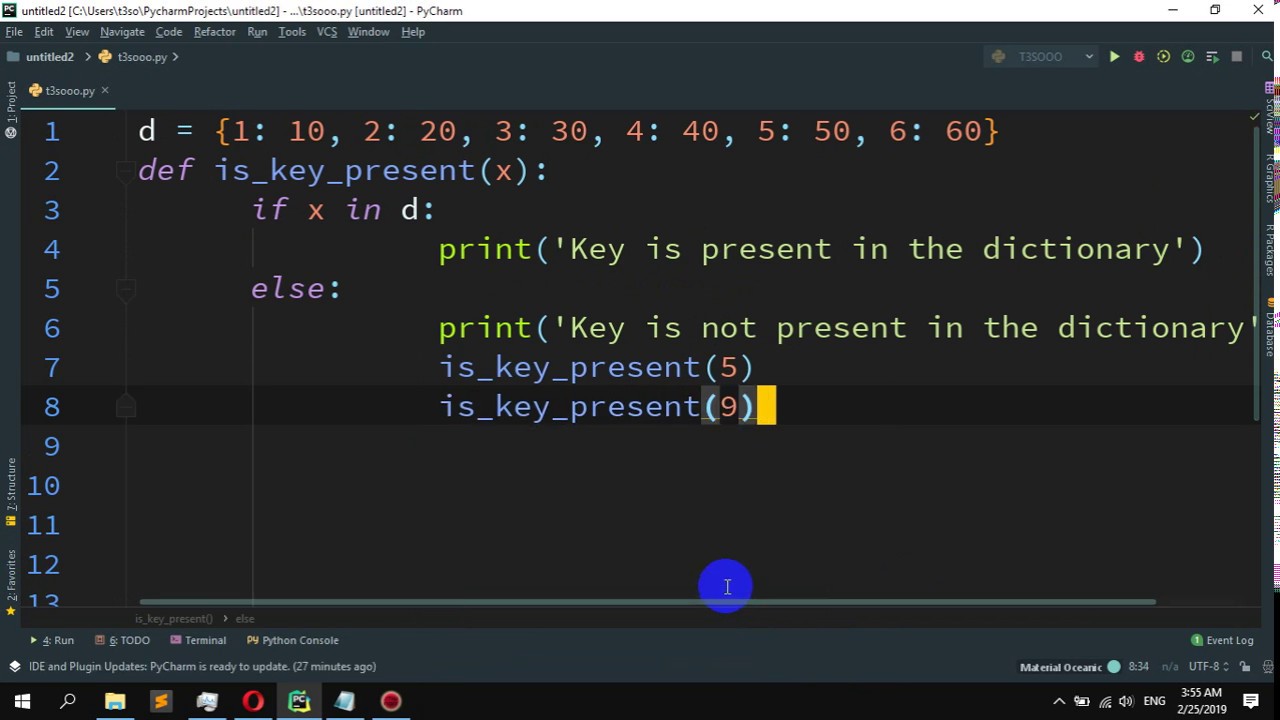
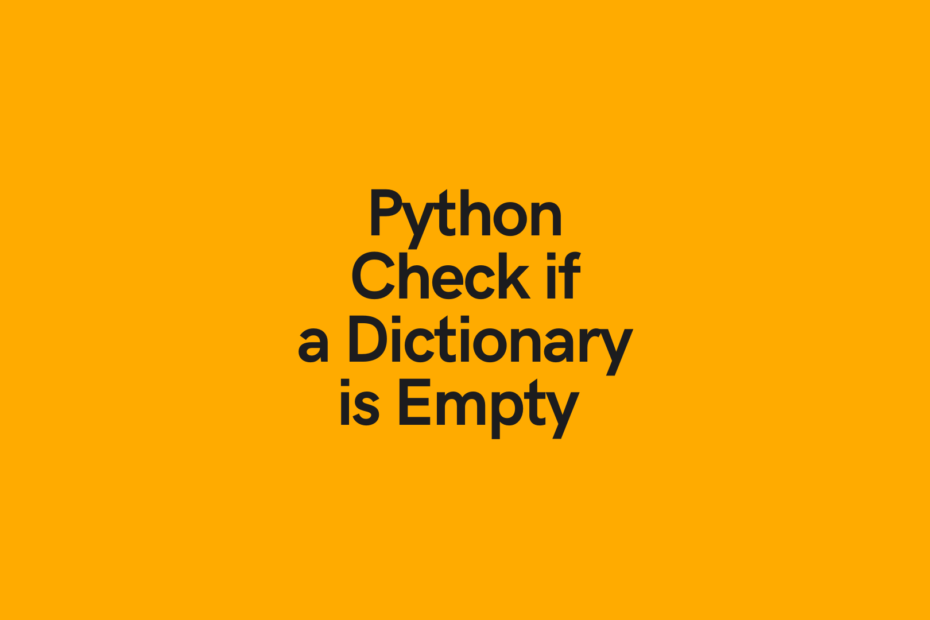
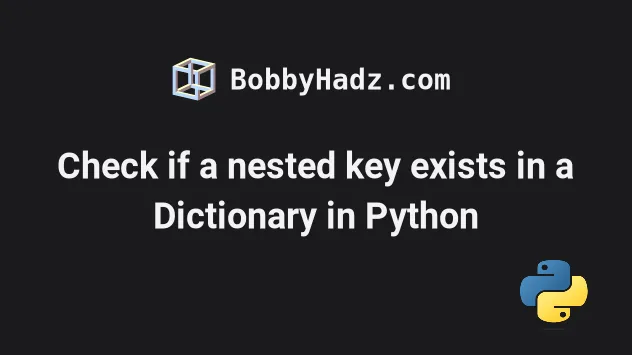
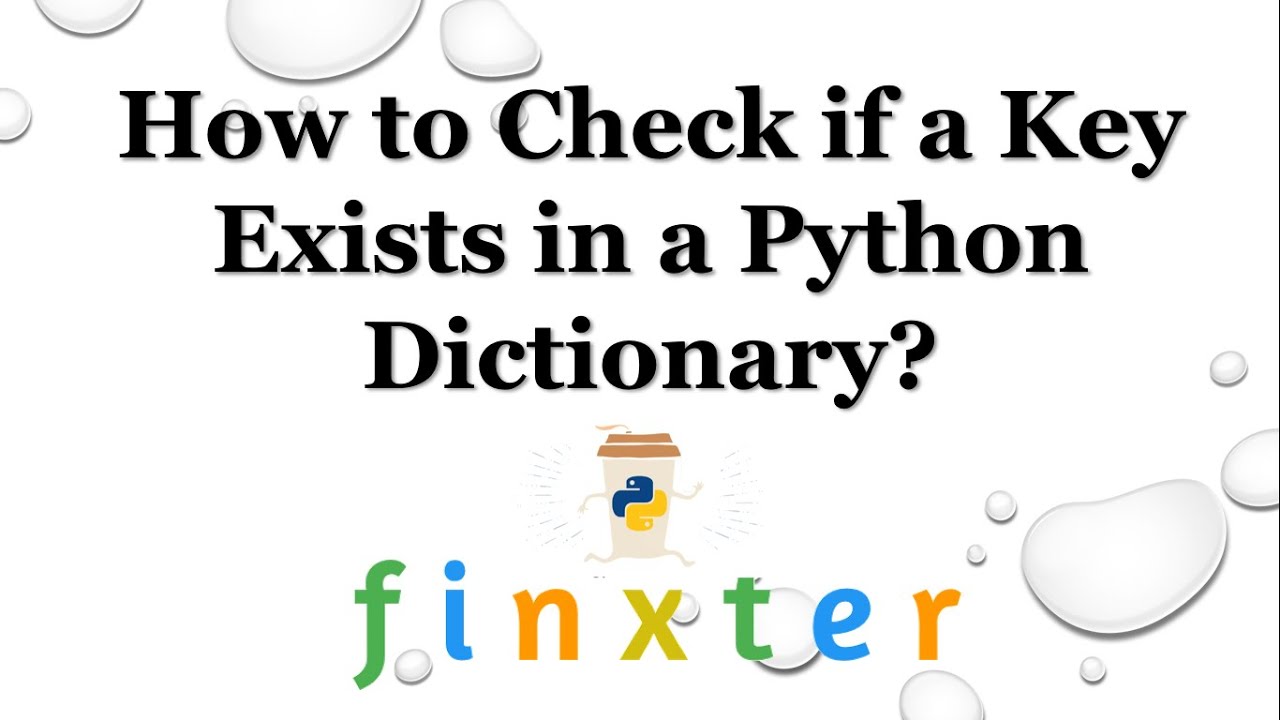
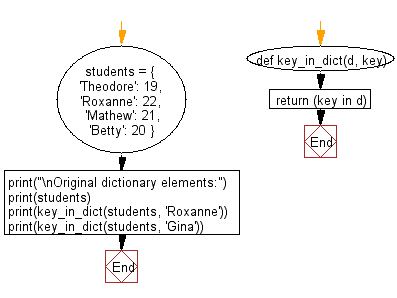

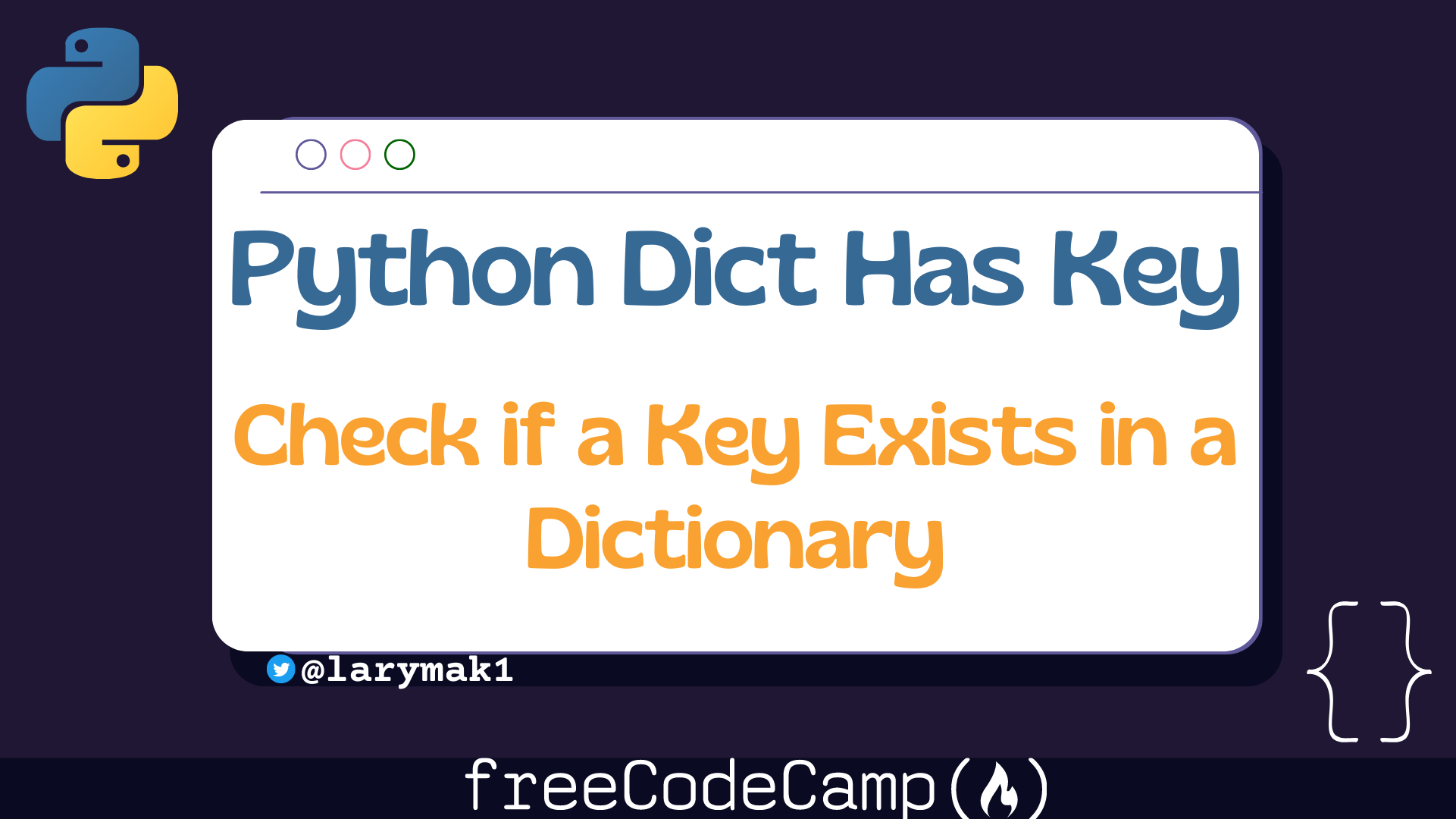

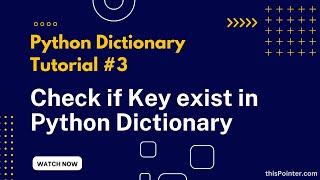
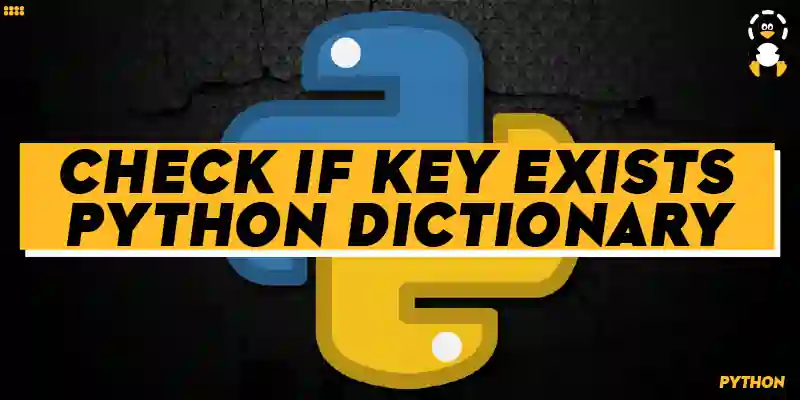


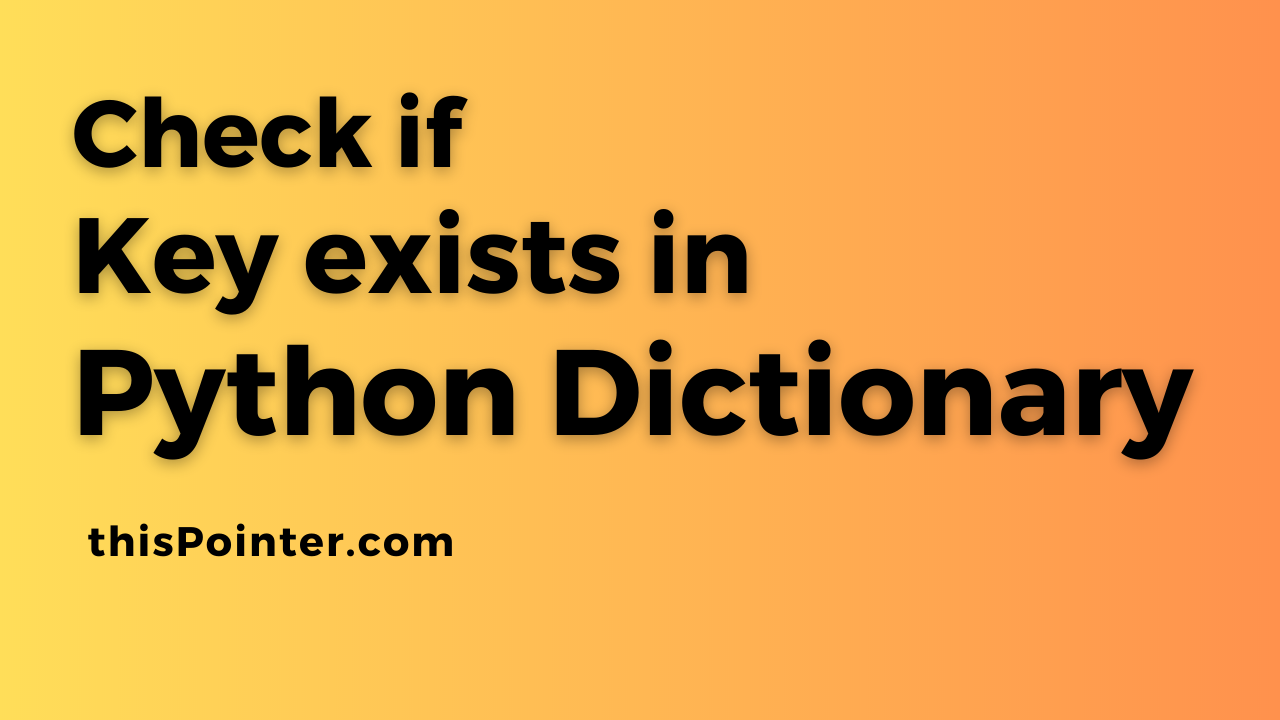
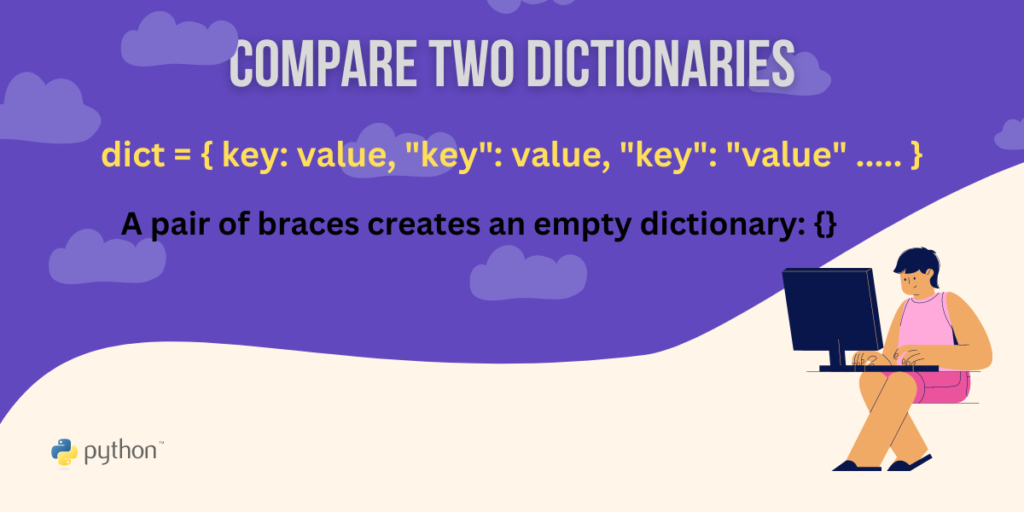


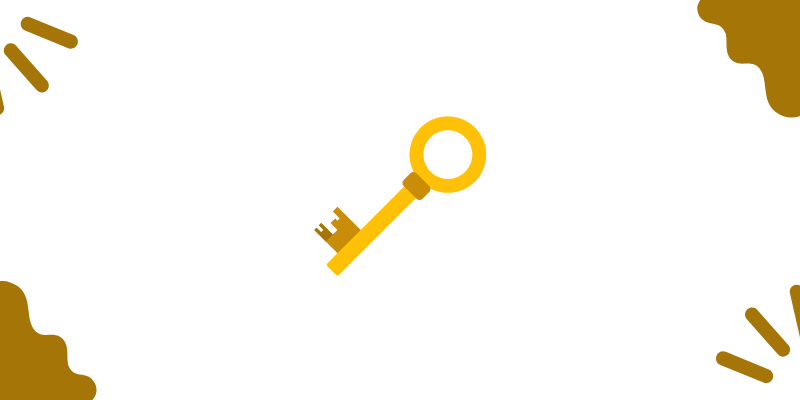
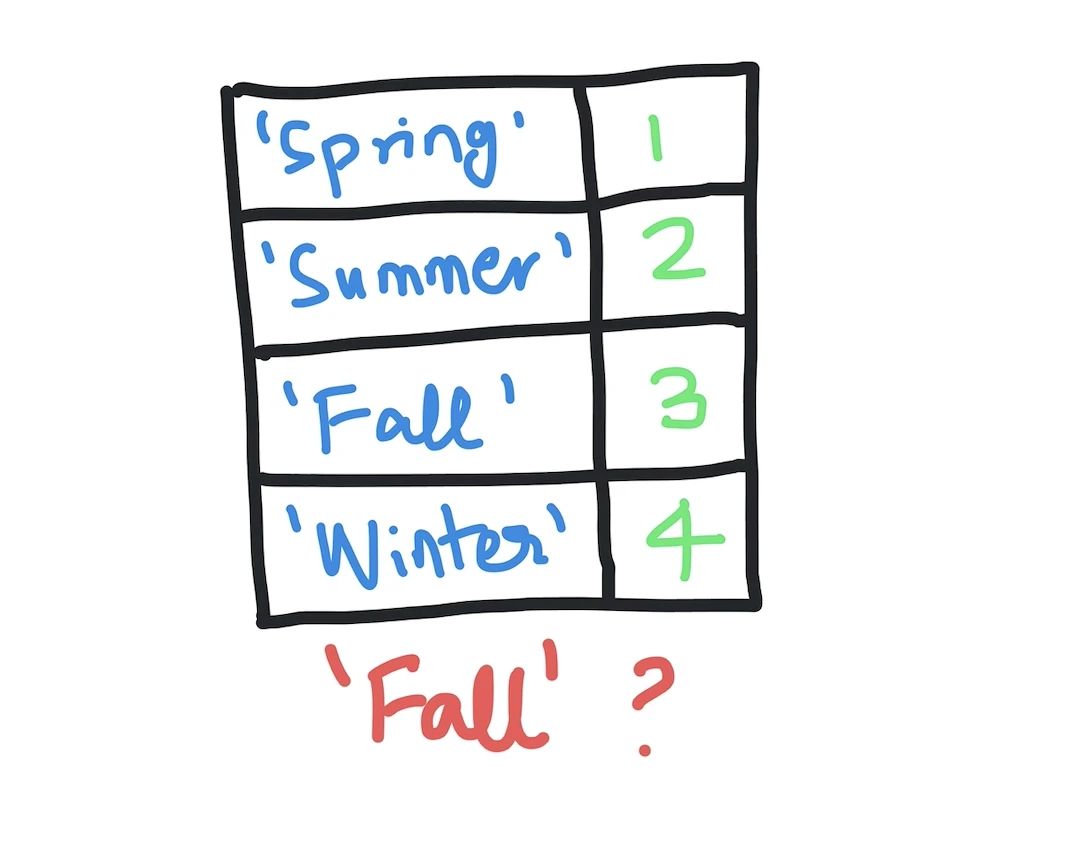
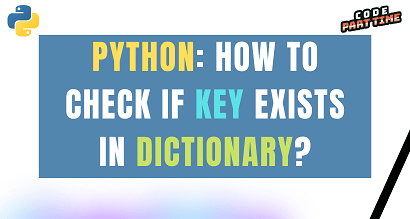
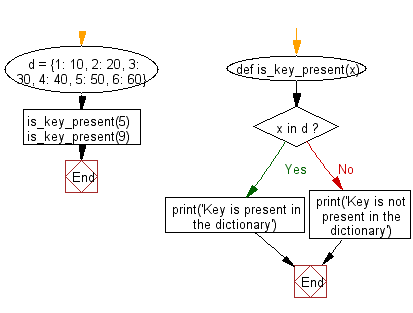
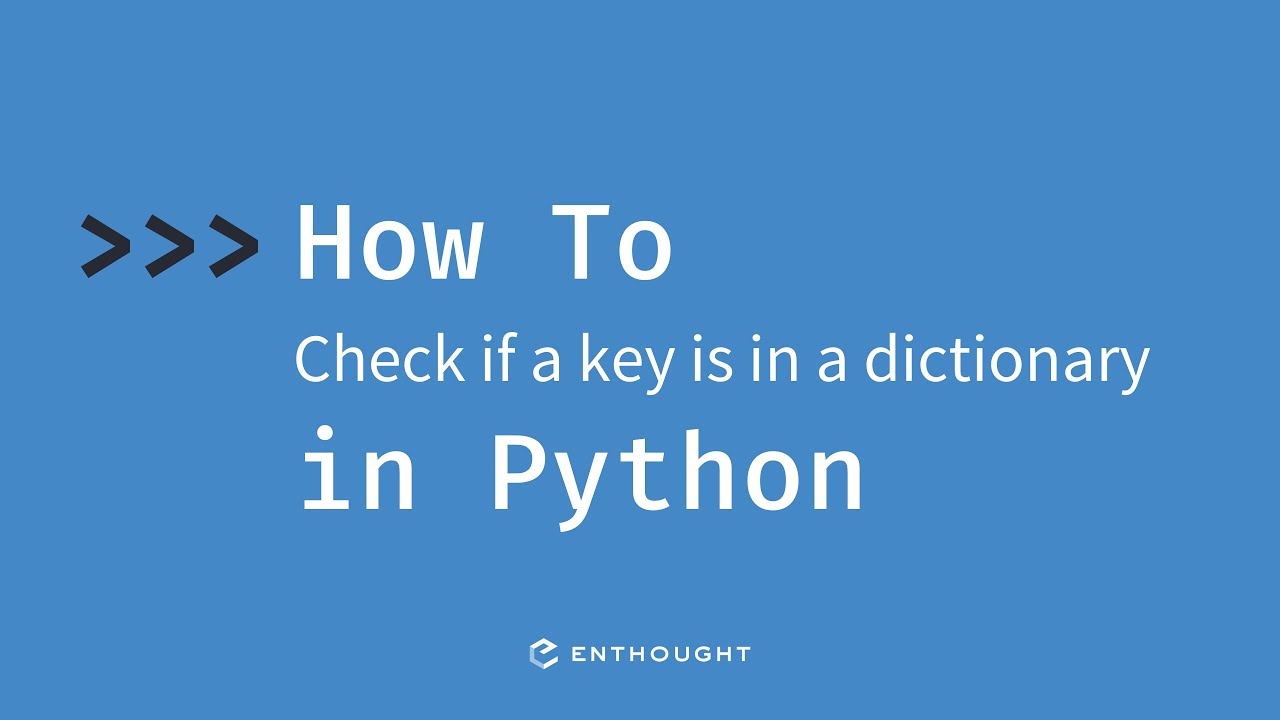
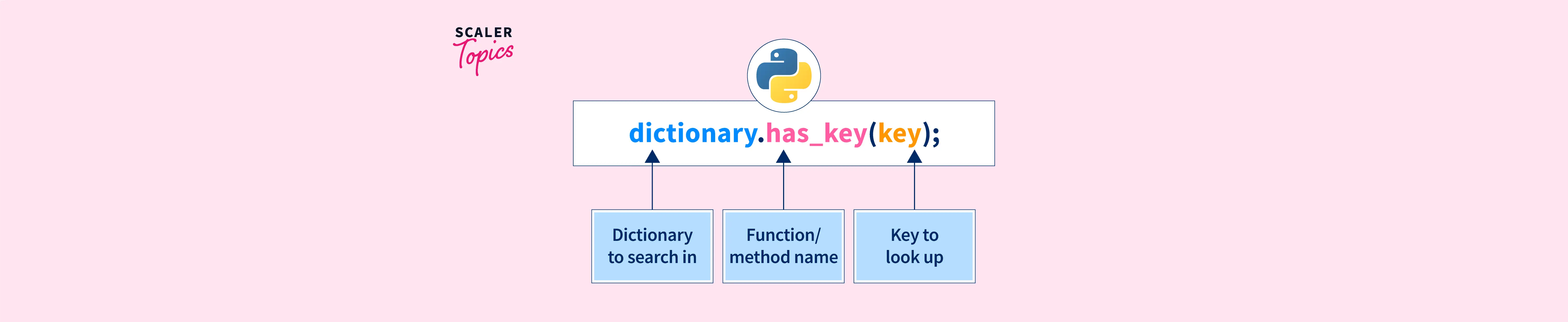
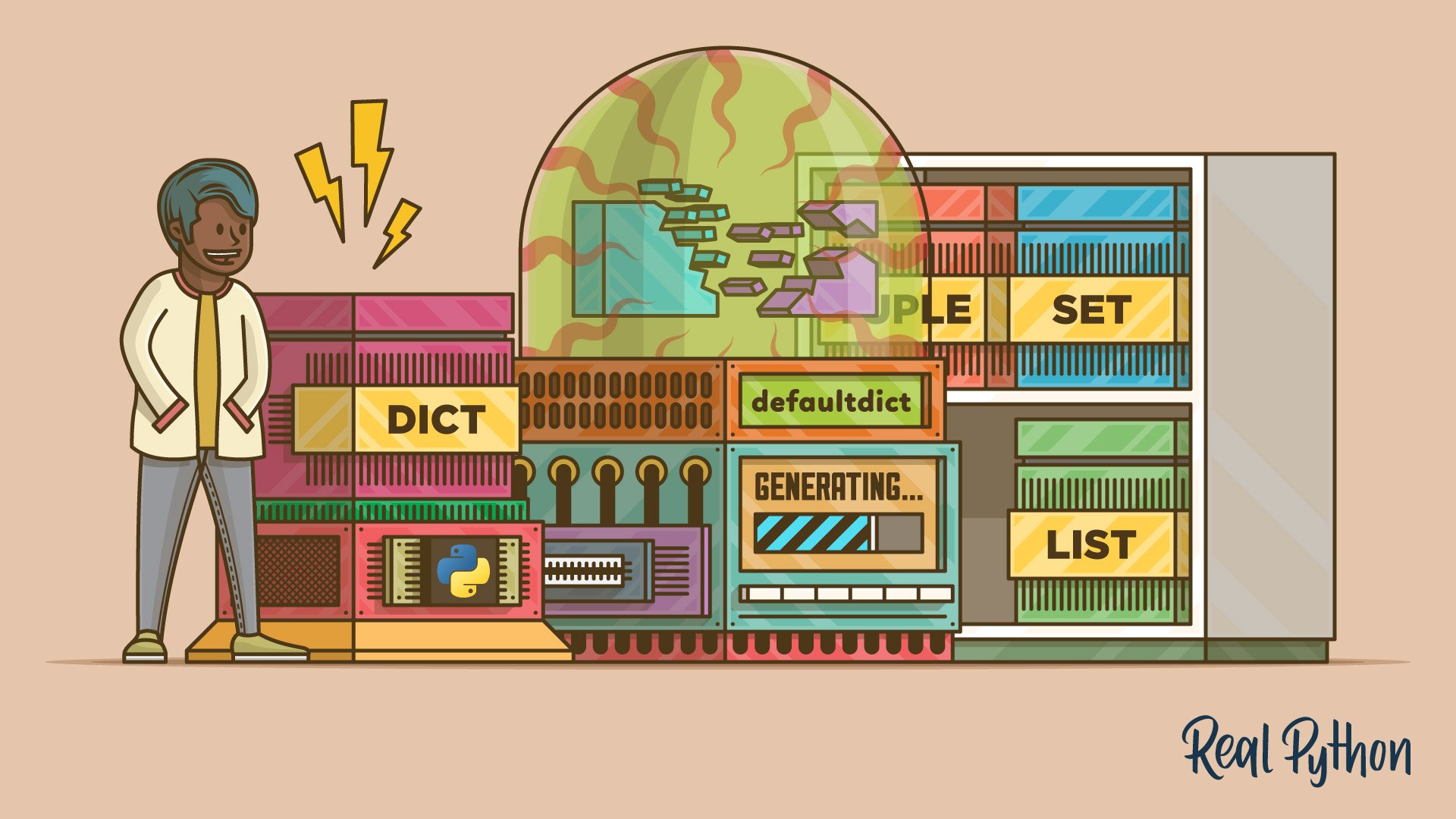
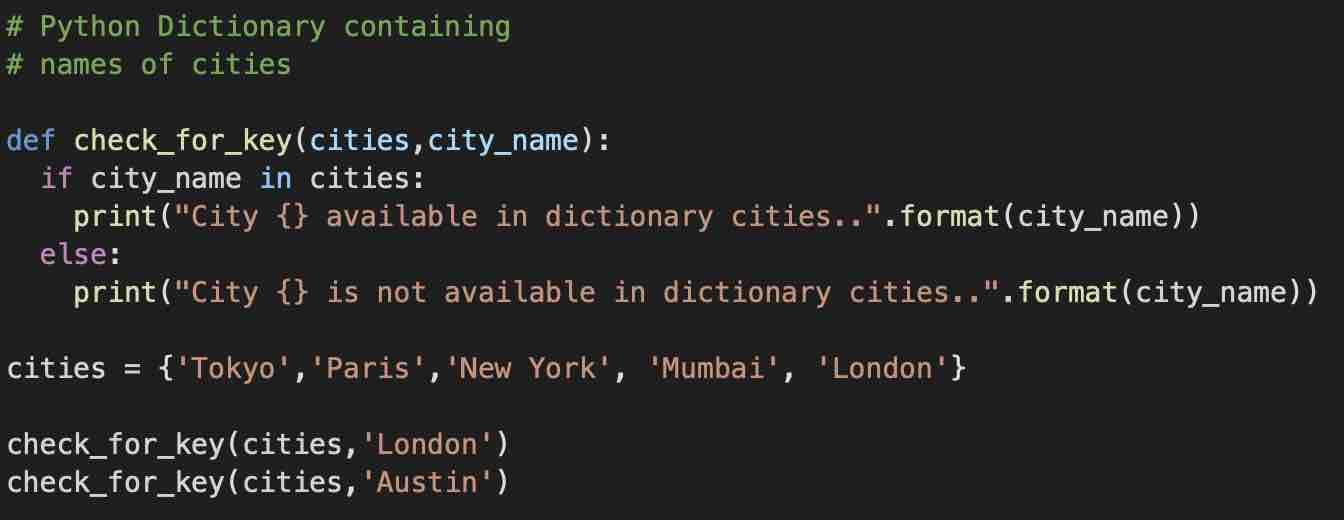
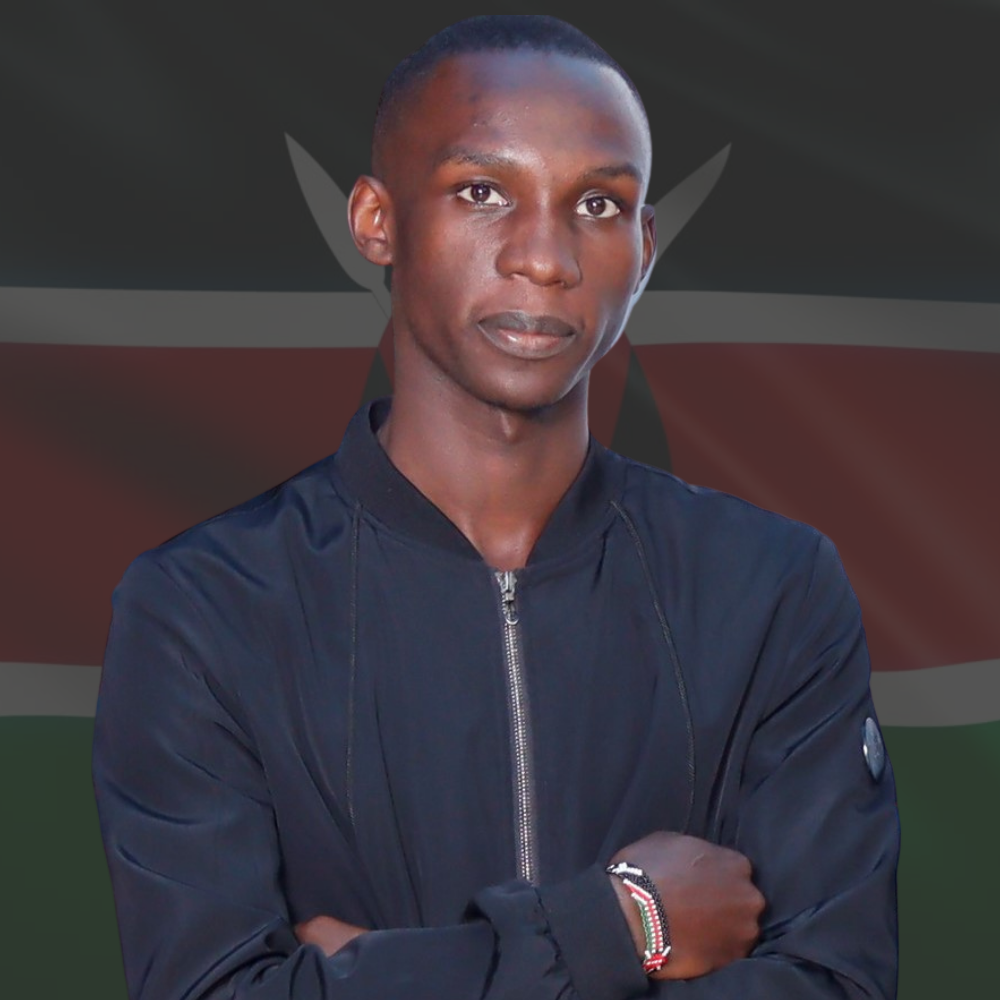

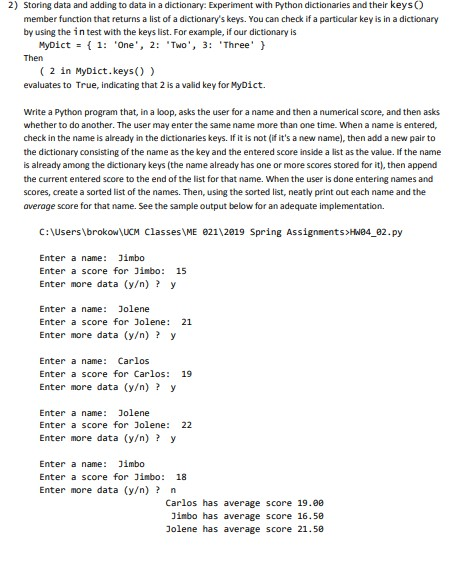

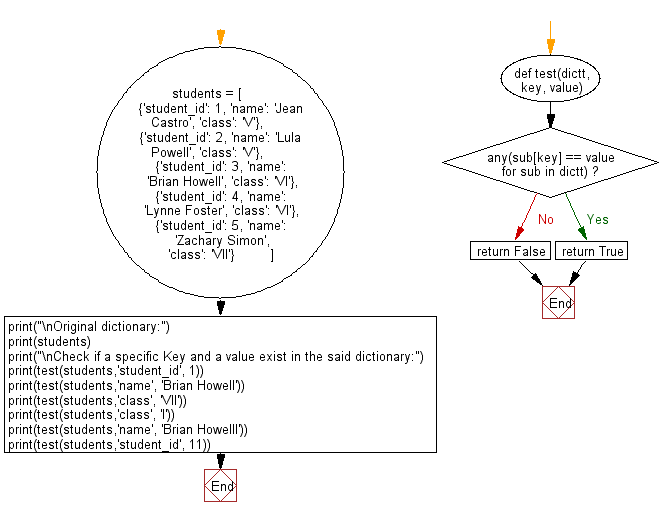
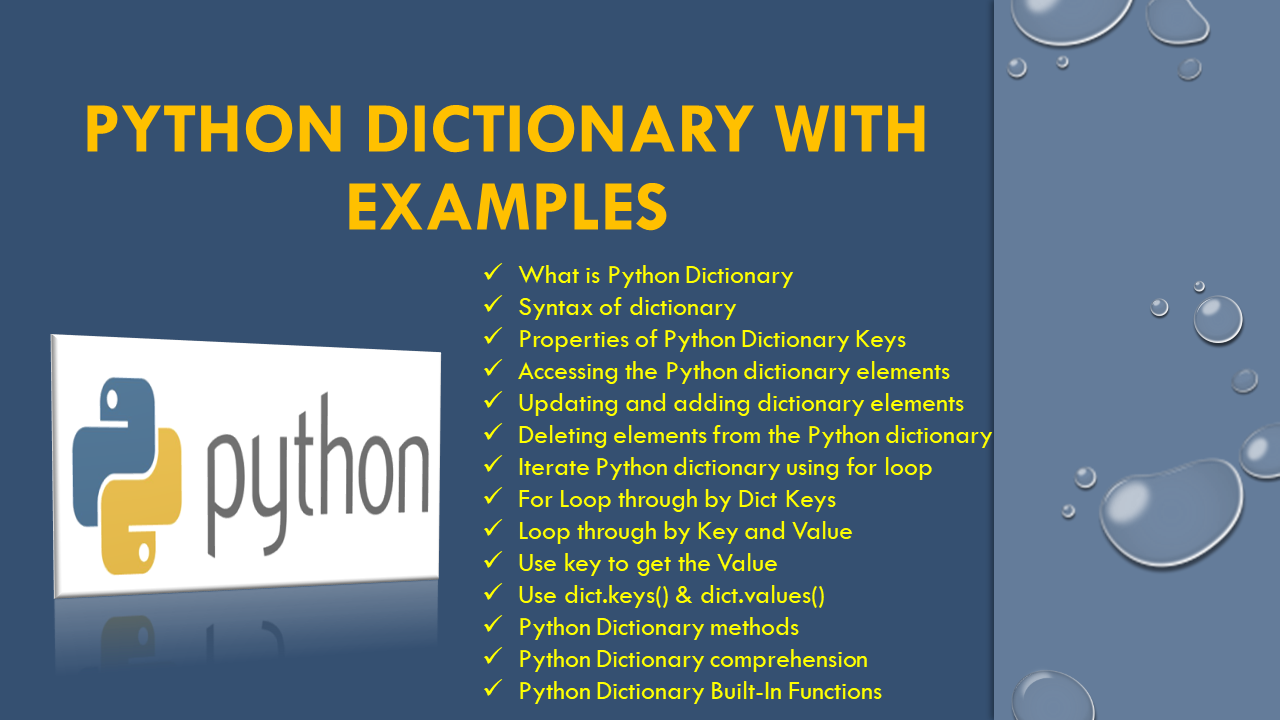

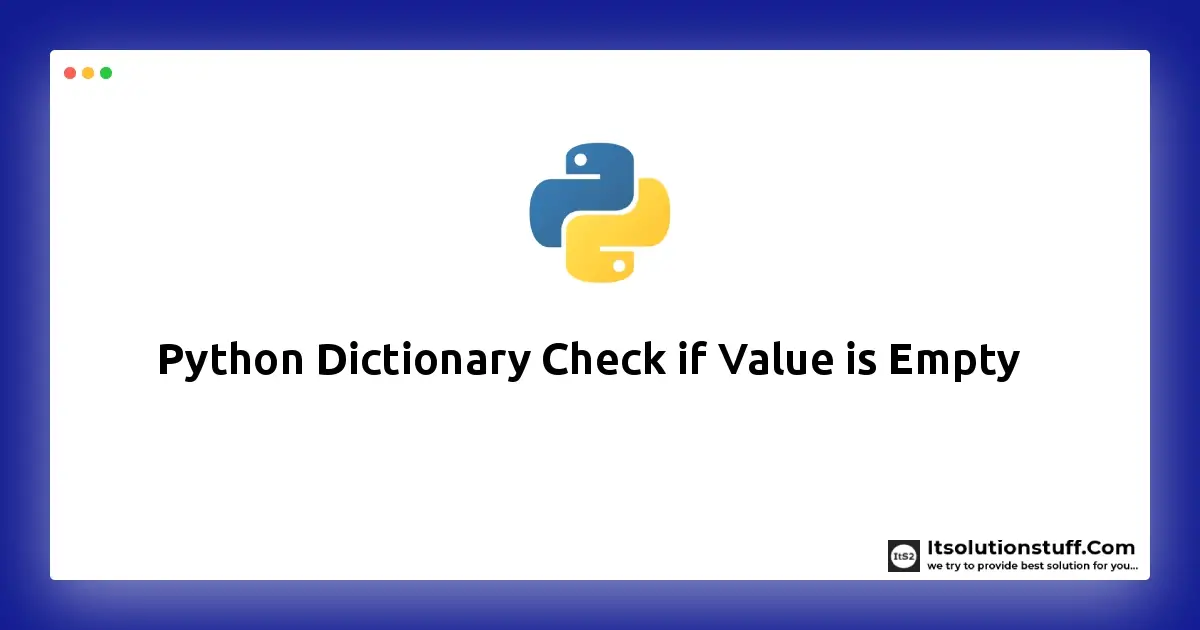

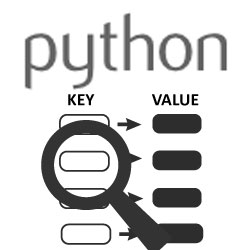
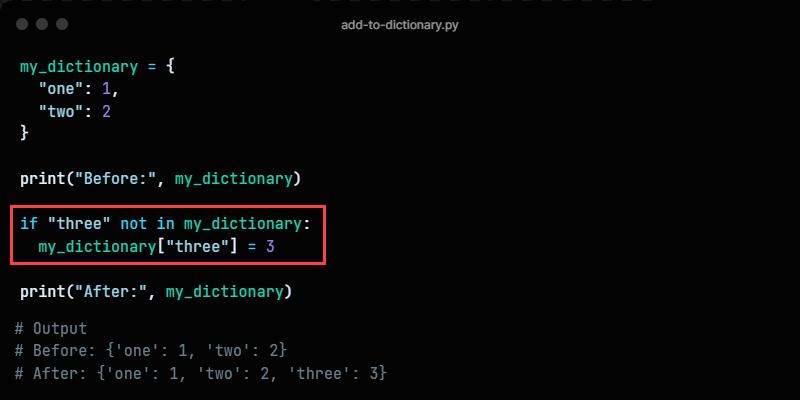
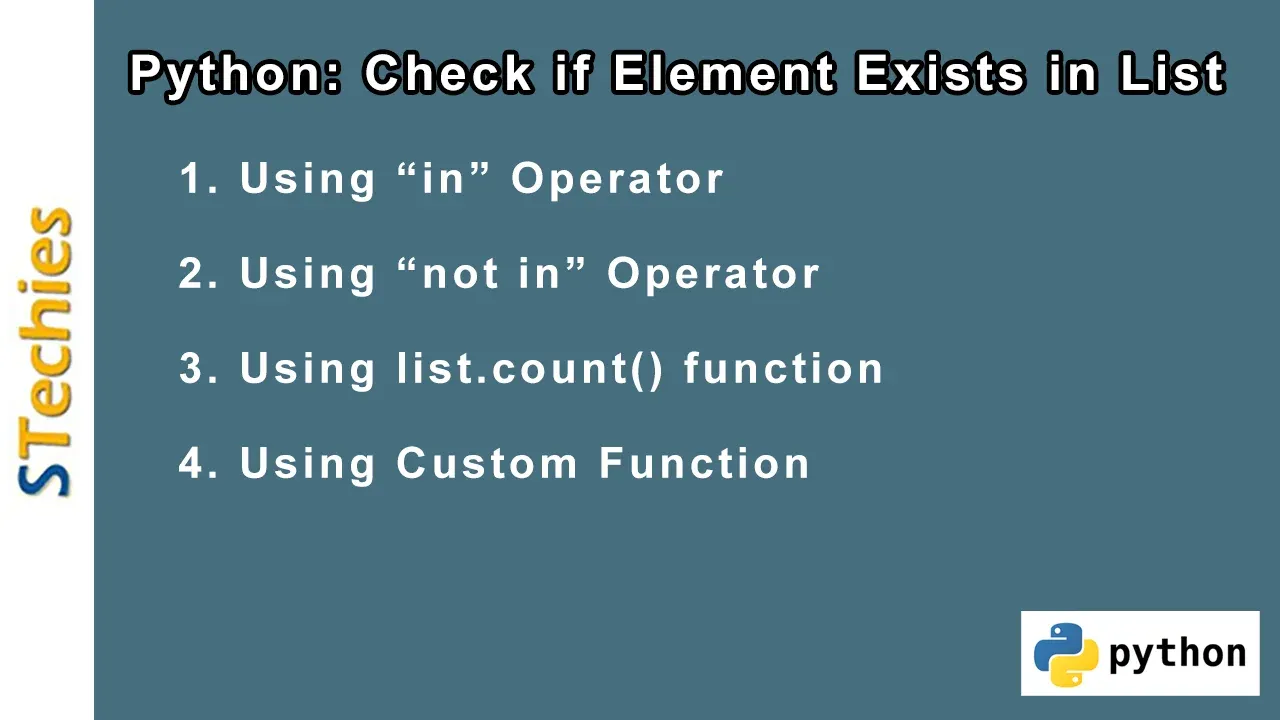
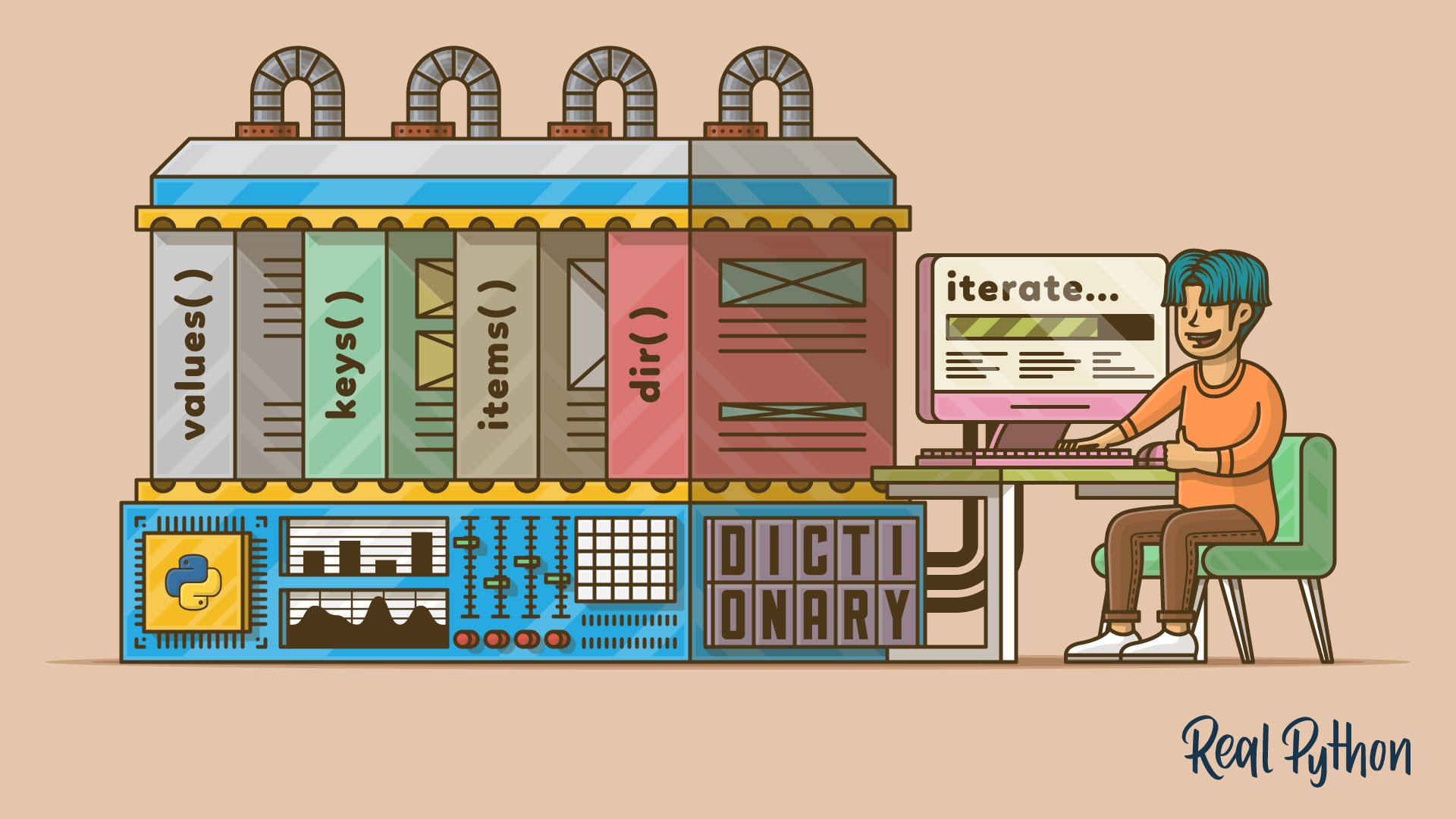
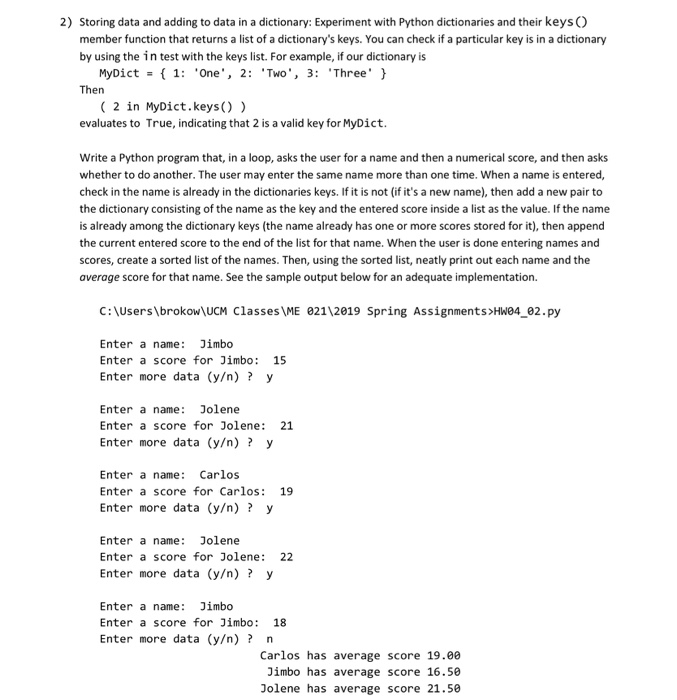
![Class 12] Julie has created a dictionary containing names and marks Class 12] Julie Has Created A Dictionary Containing Names And Marks](https://d1avenlh0i1xmr.cloudfront.net/374bed5d-d4bc-4d9f-b730-4c919131fae6/slide37.jpg)
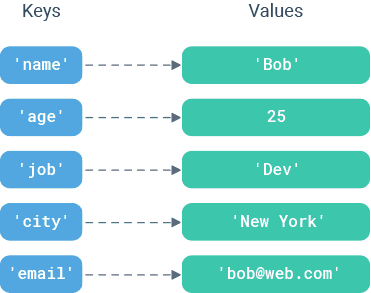
Article link: python check if key in dictionary.
Learn more about the topic python check if key in dictionary.
- How to check if key exists in a python dictionary? – Flexiple
- Check if a given key already exists in a dictionary
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check whether given Key already exists in a Python Dictionary
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Python: Check if Variable is a Dictionary
- Check if Value Exists in List of Dictionaries in Python (2 Examples)
- Python | Check if key has Non-None value in dictionary – GeeksforGeeks
- Check if key/value exists in dictionary in Python – nkmk note
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- Check whether given Key already exists in a Python Dictionary
- Check if Key exists in Dictionary (or Value) with Python code
- How To Check If Key Exists In Dictionary In Python?
See more: nhanvietluanvan.com/luat-hoc