Python Check If Keys In Dict
A dictionary in Python is a powerful data structure that allows you to store and retrieve key-value pairs efficiently. It is a fundamental data structure in the language and is used extensively in various applications. In this article, we will explore different methods to check if keys exist in a Python dictionary.
Iterating over Keys in a Python Dictionary
One of the simplest ways to check for keys in a dictionary is by iterating over its keys. This can be done using a for loop as follows:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key in my_dict:
print(key)
“`
This will print all the keys in the dictionary: ‘name’, ‘age’, and ‘city’. If you want to perform some operations based on the keys, you can add your logic inside the loop.
Using the “in” Operator to Check for Key Existence
The “in” operator is another convenient way to check for key existence in a dictionary. It returns a boolean value indicating whether the specified key is present in the dictionary or not. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict:
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
This will print “Key ‘name’ exists in the dictionary” since the key ‘name’ is present in the dictionary.
Using the “get()” Method to Check for Key Existence
The “get()” method is commonly used to retrieve values from a dictionary based on a key. However, it can also be used to check for key existence. If the specified key is present in the dictionary, the method will return its value. Otherwise, it will return a default value (which can be specified as the second argument of the method). Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
name = my_dict.get(‘name’)
print(name)
country = my_dict.get(‘country’, ‘Unknown’)
print(country)
“`
In this example, the first “get()” method call will return the value associated with the key ‘name’ (i.e., ‘John’). The second “get()” method call will return the default value ‘Unknown’ since the key ‘country’ does not exist in the dictionary.
Using the “keys()” Method to Generate a List of Keys
If you want to obtain a list of all the keys in a dictionary, you can use the “keys()” method. This method returns a view object that contains all the keys in the dictionary, which can be converted to a list using the “list()” function. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
keys = list(my_dict.keys())
print(keys)
“`
This will print [‘name’, ‘age’, ‘city’], which is a list of all the keys in the dictionary.
Using the “items()” Method to Iterate over Dictionary Keys and Values
The “items()” method is a versatile way to iterate over both the keys and values in a dictionary simultaneously. It returns a view object that contains tuples of key-value pairs, which can be unpacked in a for loop. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(key, value)
“`
This will print the key-value pairs of the dictionary: ‘name John’, ‘age 25’, and ‘city New York’. If you only want to iterate over the keys, you can modify the loop accordingly.
Using the “has_key()” Method to Check for Key Existence (Python 2 only)
Note: This method is only available in Python 2 and has been removed in Python 3.
In Python 2, you can use the “has_key()” method to check for key existence in a dictionary. This method returns a boolean value indicating whether the specified key is present in the dictionary or not. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.has_key(‘name’):
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
This will print “Key ‘name’ exists in the dictionary” since the key ‘name’ is present in the dictionary. However, it is important to note that this method is deprecated and should not be used in Python 3.
Using Exception Handling to Check for Key Existence
Another approach to check for key existence in a dictionary is by using exception handling. You can try to access the value of a key using the key indexing syntax, and if a KeyError exception is raised, it means the key does not exist in the dictionary. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
name = my_dict[‘name’]
print(“Key ‘name’ exists in the dictionary”)
except KeyError:
print(“Key ‘name’ does not exist in the dictionary”)
“`
This will print “Key ‘name’ exists in the dictionary” since the key ‘name’ is present in the dictionary. If you try to access a non-existent key, the exception block will handle the KeyError and print “Key ‘name’ does not exist in the dictionary”.
FAQs:
Q: How can I check if a key does not exist in a Python dictionary?
A: You can use the “in” operator or the “get()” method to check for key existence in a dictionary. If the key is not present, both methods will return False or the specified default value.
Q: Is it possible to check if a key exists in a dictionary without iterating over all the keys?
A: Yes, you can use the “in” operator or the “get()” method to quickly check for key existence in a dictionary. These methods are efficient and provide a convenient way to perform key existence checks.
Q: Can I use the “in” operator with nested dictionaries?
A: Yes, the “in” operator can be used with nested dictionaries to check for key existence at any level. However, you need to access the nested dictionaries using their respective key paths.
Q: Is there a performance difference between using the “in” operator and the “get()” method for key existence checks?
A: In general, using the “in” operator is slightly faster than using the “get()” method for key existence checks. However, the difference in performance is negligible unless you are performing a large number of key checks.
Q: How can I find a specific element in a dictionary based on its value?
A: You can use a for loop to iterate over the key-value pairs in a dictionary and perform a value comparison to find the desired element. If the value matches, you can access the corresponding key.
Q: Can I check if a value exists in a dictionary using similar methods?
A: Yes, you can use similar methods like the “in” operator or the “values()” method to check for value existence in a dictionary. However, keep in mind that values can be duplicated, so these methods will return True if the value exists at least once.
In conclusion, Python provides various methods to check for key existence in a dictionary. Each method has its advantages and can be chosen based on the specific requirements of your code. By understanding these methods, you can efficiently handle key checks and manipulate dictionaries in Python.
Python: Check If Value Exists In Dictionary
How To Check If Key Exists In Dict Python?
Python is a versatile programming language that offers a wide range of data structures to work with. One of the most commonly used data structures in Python is the dictionary. A dictionary, also known as a hashmap or associative array, is an unordered collection of key-value pairs. It provides a fast and efficient way to store and retrieve data. However, before accessing or manipulating the values associated with a particular key in a dictionary, it is essential to check if the given key exists in the dictionary. In this article, we will explore various methods to check if a key exists in a dictionary in Python.
Method 1: Using the “in” Operator
The simplest approach to determine if a key is present in a dictionary is by using the “in” operator. The “in” operator returns True if the given key is present in the dictionary, and False otherwise. Here is an example that demonstrates the usage of the “in” operator:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘grape’: 10}
if ‘apple’ in my_dict:
print(“Key ‘apple’ exists!”)
else:
print(“Key ‘apple’ does not exist!”)
“`
Output:
“`
Key ‘apple’ exists!
“`
Method 2: Using the get() Method
Another way to check the existence of a key in a dictionary is by using the get() method. The get() method returns the value associated with the specified key if it exists. If the key is not found, it returns a default value, which is None by default. By checking if the returned value is None, we can determine if the key exists in the dictionary. Here is an example:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘grape’: 10}
value = my_dict.get(‘apple’)
if value is not None:
print(“Key ‘apple’ exists!”)
else:
print(“Key ‘apple’ does not exist!”)
“`
Output:
“`
Key ‘apple’ exists!
“`
Method 3: Using the keys() Method
Python dictionaries provide a keys() method that returns a list containing all the keys present in the dictionary. To check if a specific key exists, we can simply check if that key is present in the list returned by the keys() method. Here is an example:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘grape’: 10}
if ‘apple’ in my_dict.keys():
print(“Key ‘apple’ exists!”)
else:
print(“Key ‘apple’ does not exist!”)
“`
Output:
“`
Key ‘apple’ exists!
“`
Method 4: Using the has_key() Method (Python 2.x Only)
In older versions of Python (2.x), the has_key() method was available to check if a key exists in a dictionary. However, this method is now deprecated and removed in Python 3.x. It is recommended to use the “in” operator or the get() method instead.
Additional Tips:
1. Avoid using the has_key() method in Python 2.x as it is no longer supported in Python 3.x.
2. It is generally more efficient to use the “in” operator or the get() method to check if a key exists in a dictionary, rather than converting the keys to a list using the keys() method.
FAQs:
Q: What happens if I try to access a key that doesn’t exist in a dictionary using the square bracket notation?
A: If you try to access a key that doesn’t exist using the square bracket notation, Python will raise a KeyError. To handle this situation, you can either use the “in” operator or the get() method to check if the key exists before accessing it.
Q: Can I use the “in” operator to check if a value exists in a dictionary?
A: No, the “in” operator checks if a key exists in a dictionary, not a value. To check if a value exists in a dictionary, you can use the values() method followed by the “in” operator.
Q: What is the time complexity of checking if a key exists in a dictionary?
A: The time complexity of checking if a key exists in a dictionary is O(1), regardless of the size of the dictionary. This makes dictionary lookups extremely fast and efficient.
In conclusion, there are multiple methods available to check if a key exists in a dictionary in Python. Whether you choose to use the “in” operator, the get() method, or the keys() method, it is essential to ensure that the key is present before attempting to access or modify the associated value. By employing these techniques, you can write more robust and error-free Python code.
How To Check Value By Key In Dict Python?
In Python, a dictionary is an unordered collection of key-value pairs where each key is unique. One of the most common tasks when working with dictionaries is to check if a certain key exists and retrieve its value. This article will guide you through various methods to check the value by key in a dictionary in Python.
Accessing dictionary values using keys is both simple and efficient due to the nature of dictionary data structures. It allows you to quickly retrieve values based on their corresponding keys, making dictionaries an invaluable tool for managing data in Python.
Here are some methods you can use to check the value by key in a dictionary:
1. Using the square bracket notation:
The simplest approach is to use the square bracket notation along with the key to access the value directly. Let’s consider an example of a dictionary with some key-value pairs:
“`python
my_dict = {“name”: “John”, “age”: 30, “city”: “New York”}
“`
To check the value associated with the key “name,” you can simply use:
“`python
value = my_dict[“name”]
print(value) # Output: John
“`
This method works as long as the dictionary contains the specified key. If the key is not present, it will raise a KeyError. To avoid this, you can use the `get()` method.
2. Using the `get()` method:
The `get()` method allows you to retrieve the value for a given key without raising an error if the key is not present in the dictionary. Instead, it returns None or a specified default value. Here’s an example:
“`python
value = my_dict.get(“name”)
print(value) # Output: John
“`
If the key “name” is not found, it will return None by default. However, you can supply a default value as the second argument to `get()`:
“`python
value = my_dict.get(“address”, “Not found”)
print(value) # Output: Not found
“`
3. Using the `in` keyword:
Python provides the `in` keyword, which allows you to check the existence of a key in a dictionary. It returns True if the key is present; otherwise, it returns False. Here’s an example:
“`python
key_exists = “name” in my_dict
print(key_exists) # Output: True
“`
You can combine this method with an `if` statement to handle cases where the key is not found:
“`python
if “address” in my_dict:
value = my_dict[“address”]
else:
value = “Not found”
print(value) # Output: Not found
“`
4. Using the `keys()` method:
The `keys()` method returns a view object that contains all the keys of the dictionary. By converting it into a list, you can then check if a specific key exists. Here’s an example:
“`python
key_exists = “name” in list(my_dict.keys())
print(key_exists) # Output: True
“`
However, note that this method is less efficient compared to the previous ones, as it involves creating an additional list object.
5. Using the `dict.get()` method combined with `in` keyword:
Another approach is to combine the `get()` method with the `in` keyword, which allows you to retrieve a value while simultaneously checking if the key exists. Here’s an example:
“`python
value_exists = my_dict.get(“name”) is not None
print(value_exists) # Output: True
“`
This method returns True if the value exists and False if it does not. By using `is not None`, you explicitly check if the value is not None, which indicates its existence.
FAQs about Checking Value by Key in Dict Python:
Q1. What happens if you try to access a non-existing key using square brackets?
A1. If you try to access a non-existing key using square brackets, a KeyError will be raised. To handle this, you can use the `get()` method or the `in` keyword.
Q2. Can dictionaries have multiple keys with the same value?
A2. Yes, dictionaries can have multiple keys with the same value. However, each key must be unique within the dictionary.
Q3. What is the difference between `dict.get()` and `dict[key]`?
A3. The `dict.get()` method allows you to retrieve dictionary values while providing a default value if the key is not found. On the other hand, using `dict[key]` directly will raise a KeyError if the key does not exist.
Q4. Which method is the most efficient for checking if a key exists in a dictionary?
A4. The most efficient method for checking if a key exists in a dictionary is using the `in` keyword directly. It does not involve creating any additional objects and provides a simple boolean result.
Q5. Can dictionaries contain complex objects as values?
A5. Yes, dictionaries in Python can contain complex objects as values, including other dictionaries, lists, tuples, or even user-defined objects.
In conclusion, accessing and checking values by key in Python dictionaries is straightforward and efficient. You can use various methods such as the square bracket notation, the `get()` method, the `in` keyword, or a combination of these techniques. Choose the method that best suits your requirements based on whether you need to handle missing keys or retrieve default values.
Keywords searched by users: python check if keys in dict Check if key not exists in dictionary Python, Dict in Python, Check key in dict Python, Python check key in array, Python check if object has key, Find element in dictionary Python, Check value in dictionary Python, For key-value in dict Python
Categories: Top 89 Python Check If Keys In Dict
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
Dictionaries are one of the most versatile and useful data structures in Python. They allow us to store data in key-value pairs, providing fast and efficient access to the values based on their associated keys. However, there are instances when we need to check if a certain key does not exist in the dictionary. In this article, we will explore different approaches to accomplish this task in Python.
Method 1: Using the ‘in’ Operator
One simple and intuitive way to check if a key does not exist in a dictionary is by using the ‘in’ operator. This operator returns True if the specified key is present in the dictionary and False otherwise. By negating the result using the ‘not’ keyword, we can determine if the key does not exist. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ not in my_dict:
print(“Key ‘name’ does not exist.”)
else:
print(“Key ‘name’ exists.”)
“`
In this case, since the key ‘name’ exists in the dictionary, the output will be “Key ‘name’ exists.” However, if we modify the dictionary to exclude the key ‘name’, the output will be “Key ‘name’ does not exist.”
Method 2: Using the get() Method
Another approach to check if a key does not exist in a dictionary is by using the get() method. This method returns the value associated with the specified key if it exists in the dictionary. Otherwise, it returns None. By comparing the result to None, we can determine if a key does not exist. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.get(‘name’) is None:
print(“Key ‘name’ does not exist.”)
else:
print(“Key ‘name’ exists.”)
“`
In this example, the result of the get() method for the key ‘name’ is not None, indicating that it exists in the dictionary. Therefore, the output will be “Key ‘name’ exists.” However, if we modify the dictionary to exclude the key ‘name’, the output will be “Key ‘name’ does not exist.”
Method 3: Using the try…except Block
Python provides a robust error handling mechanism through the use of try…except blocks. We can leverage this feature to check if a key does not exist. By attempting to access the key within a try block and catching the KeyError exception in an except block, we can conclude that the key does not exist if the exception is raised. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
value = my_dict[‘name’]
print(“Key ‘name’ exists.”)
except KeyError:
print(“Key ‘name’ does not exist.”)
“`
In this example, since the key ‘name’ exists in the dictionary, the output will be “Key ‘name’ exists.” However, if we modify the dictionary to exclude the key ‘name’, the output will be “Key ‘name’ does not exist.”
Frequently Asked Questions:
Q1: Can I use the ‘not in’ operator to check if a key does not exist in a dictionary?
A1: No, the ‘not in’ operator does not directly work for dictionaries. It is specifically designed to be used with sequence types like strings, lists, or tuples. For dictionaries, it is recommended to use the ‘not’ keyword in combination with the ‘in’ operator.
Q2: Which method should I use to check if a key does not exist in a dictionary?
A2: The choice of method depends on your specific requirements. If you simply want to check the existence of a key, using the ‘in’ operator is a straightforward and concise option. However, if you need to handle the absence of a key in a specific way (e.g., returning a default value), using the get() method or try…except block might be more suitable.
Q3: What happens if I try to access a non-existent key directly without using any of the mentioned methods?
A3: If you try to access a non-existent key directly, Python will raise a KeyError exception. It is recommended to handle this exception to prevent program termination.
Q4: Can a dictionary have multiple keys with the same name?
A4: No, each key in a dictionary must be unique. If you attempt to assign multiple values to the same key, the last assigned value will overwrite the previous one.
Q5: How can I remove a key-value pair from a dictionary if the key exists?
A5: You can use the del keyword followed by the key to remove a key-value pair from a dictionary. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
del my_dict[‘name’]
“`
After executing the code, the key-value pair with the key ‘name’ will be removed from the dictionary.
In conclusion, checking if a key does not exist in a dictionary in Python is a common task, and there are multiple approaches to accomplish it. The ‘in’ operator, the get() method, and the try…except block provide different alternatives depending on the desired behavior. By understanding these methods, you can efficiently handle situations where you need to determine if a key is absent from a dictionary.
Dict In Python
Dictionaries in Python are versatile and powerful data structures that allow us to store and retrieve data efficiently. They provide a unique way of organizing and manipulating data, making them an essential tool in any Python programmer’s arsenal. In this article, we will delve deep into dictionaries, exploring their features, functionality, and some real-world use cases. So, let’s dive in!
Understanding Dictionaries:
A dictionary in Python is an unordered collection of key-value pairs, enclosed in curly braces {}. The keys in a dictionary are unique and immutable, whereas the values can be of any type and mutable. Dictionaries are also commonly referred to as associative arrays, maps, or hashes in other programming languages.
Creating a Dictionary:
To create a dictionary, we simply initialize it using empty curly braces, like this:
“`python
my_dict = {}
“`
Alternatively, we can also create a dictionary with some pre-filled data using the key-value syntax:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
“`
Accessing Values in a Dictionary:
To access a specific value from a dictionary, we use the corresponding key. For example:
“`python
name = my_dict[‘name’]
“`
This retrieves the value associated with the key `’name’` and assigns it to the variable `name`. If the key does not exist in the dictionary, a `KeyError` will be raised. To avoid this, we can either check if the key exists using the `in` operator or utilize the `get()` method, which returns `None` if the key is not found:
“`python
if ‘age’ in my_dict:
age = my_dict[‘age’]
“`
“`python
age = my_dict.get(‘age’)
“`
Modifying Values in a Dictionary:
Since dictionaries are mutable, we can easily modify the values associated with existing keys or add new key-value pairs. For example:
“`python
my_dict[‘age’] = 26 # Update the value of an existing key
my_dict[‘language’] = ‘Python’ # Add a new key-value pair
“`
Removing Values from a Dictionary:
To remove a key-value pair from a dictionary, we can use the `del` statement followed by the key:
“`python
del my_dict[‘age’]
“`
This deletes the key `’age’` along with its associated value from the dictionary.
Dictionary Methods and Operations:
Python dictionaries come with a plethora of built-in methods and operations for various purposes. Some commonly used methods include:
– `.keys()`: Returns a view object of all keys in the dictionary.
– `.values()`: Returns a view object of all values in the dictionary.
– `.items()`: Returns a view object with all key-value pairs in the dictionary as tuples.
– `.update()`: Updates the dictionary with new key-value pairs from another dictionary or iterable.
– `.pop()`: Removes and returns the value associated with a given key.
In addition to these methods, we can also use dictionary comprehensions, which follow a similar concept to list comprehensions, to create dictionaries in a concise and elegant manner.
Real-World Use Cases:
Dictionaries find applications in various domains and scenarios. Some common real-world use cases include:
1. Data Manipulation: Dictionaries provide an efficient way to manipulate and organize data. They can be used to store and retrieve data from databases, represent JSON-like structures, or construct complex data sets.
2. Caching: Dictionaries can act as a cache, storing previously computed values and avoiding expensive operations. By mapping inputs to their corresponding outputs, dictionaries enable quick and efficient calculations.
3. Counting Frequencies: With dictionaries, it becomes effortless to count the frequency of elements in a collection. For instance, we can count the occurrences of words in a text or track the frequency of various items in an inventory.
4. Configuration Settings: Dictionaries are often utilized to store and manage configuration settings for applications. They allow easy retrieval of specific settings based on their corresponding keys, enabling customization and flexibility.
FAQs:
Q1. Can dictionaries contain duplicate keys?
No, dictionaries cannot contain duplicate keys. If you try to add a duplicate key, the new value will replace the existing one.
Q2. Are dictionaries ordered in Python?
Dictionaries were traditionally unordered in Python versions before 3.7. From Python 3.7 onwards, dictionaries maintain the order of elements, similar to how they were inserted.
Q3. Can we nest dictionaries inside other dictionaries?
Yes, dictionaries can be nested inside other dictionaries, allowing for hierarchical and structured data representations.
Q4. How are dictionaries different from lists?
Dictionaries differ from lists in terms of their storage mechanism and retrieval of values. While lists are indexed by integers, dictionaries are indexed by unique keys. This enables faster access to values using descriptive keys rather than integer indices.
Q5. Are dictionaries mutable?
Yes, dictionaries are mutable, meaning their values can be modified, entries can be added, and entries can be removed.
In conclusion, dictionaries in Python provide a powerful, flexible, and efficient way to store and manipulate data. Their unique key-value mapping allows for quick lookups and enables various real-world use cases. Understanding dictionaries and their methods grants Python developers the ability to leverage this data structure effectively, leading to cleaner code and improved performance.
Check Key In Dict Python
Python is a versatile and powerful programming language that provides various data structures for efficient programming. One such data structure is the dictionary, which allows us to store and retrieve key-value pairs. When working with dictionaries, it is often necessary to check whether a specific key exists or not. The ability to efficiently check for the presence of a key in a dictionary is essential for performing various operations and ensuring correct program behavior. In this article, we will explore different methods to check for a key in a dictionary using Python.
Checking key existence in a dictionary can be particularly useful when dealing with large datasets or when designing algorithms that handle user input. It allows us to handle different cases depending on whether a key is present or not. Python provides several methods to check for a key in a dictionary, each with its advantages and use cases.
1. Using ‘in’ Operator:
The simplest way to check for the existence of a key in a dictionary is by using the ‘in’ operator. The syntax is as follows:
“`
if key in dictionary:
# Key is present
else:
# Key is not present
“`
This method returns a boolean value, True if the key exists, and False otherwise. It is a straightforward and concise approach that works efficiently for most cases. However, it may not be the most performant method when dealing with very large dictionaries.
2. Using get() Method:
Python dictionaries come with a built-in method called get() that allows us to retrieve the value associated with a specific key. Additionally, this method also provides a way to handle cases where the key is not found. The syntax is as follows:
“`
value = dictionary.get(key, default)
“`
Here, the get() method takes two parameters: the key to be retrieved and a default value to be returned if the key is not present in the dictionary. If the key exists, the method returns the associated value; otherwise, it returns the default value. We can use this method to check if a key exists and simultaneously retrieve its value.
3. Using the ‘KeyError’ Exception:
Python provides built-in exception handling mechanisms that allow us to handle errors gracefully. One such exception is the KeyError, which is raised when trying to access a non-existent key in a dictionary. We can leverage this behavior to check for the existence of a key. The syntax is as follows:
“`
try:
value = dictionary[key]
# Key is present
except KeyError:
# Key is not present
“`
This method involves directly accessing the key from the dictionary using square brackets. If the key is not found, a KeyError exception is raised, which can be caught and handled accordingly. Although this method may seem less intuitive, it provides a way to handle the absence of a key in a more controlled manner.
4. Using ‘keys()’ Method:
Python dictionaries provide a method called keys() that returns a list of all the keys present in the dictionary. We can use this method to check for the existence of a key. The syntax is as follows:
“`
if key in dictionary.keys():
# Key is present
else:
# Key is not present
“`
This method converts all the keys into a list and performs a check in that list. While this method works correctly for small-sized dictionaries, it may not be the most efficient approach for large-sized dictionaries as it creates an additional list of keys.
5. Using ‘dict.get()’ Method with No Default Value:
Another variation of using the get() method involves omitting the default value parameter. When the key is not found, this method returns None instead of a default value. We can then use this behavior to check whether the key exists or not. The syntax is as follows:
“`
value = dictionary.get(key)
if value is not None:
# Key is present
else:
# Key is not present
“`
Here, we directly store the value obtained from the get() method and check if it is None or not. Although this method works, it can be less clear and may cause confusion if None is a valid value in the dictionary.
FAQs:
Q1. What is the most efficient method to check for a key in a dictionary?
A1. The most efficient method depends on the specific use case and the size of the dictionary. Generally, using the ‘in’ operator is a simple and efficient way to check for key existence. However, if the dictionary is very large, using the ‘KeyError’ exception method might be more performant.
Q2. What happens if we try to access a non-existent key using square brackets directly?
A2. When trying to access a non-existent key using square brackets, a KeyError exception is raised. To handle this exception, you can use a try-except block or use the get() method with a default value.
Q3. Can we check for the existence of a value instead of a key in a dictionary?
A3. Yes, we can check for the existence of a value in a dictionary by using the ‘in’ operator along with the values() method. The syntax would be as follows: ‘if value in dictionary.values():’.
In conclusion, checking for the presence of a key in a dictionary is a common operation in Python programming. Python provides various methods, each with its advantages and use cases. By using the appropriate method based on the specific requirements, we can ensure efficient and error-free operations on dictionaries.
Images related to the topic python check if keys in dict
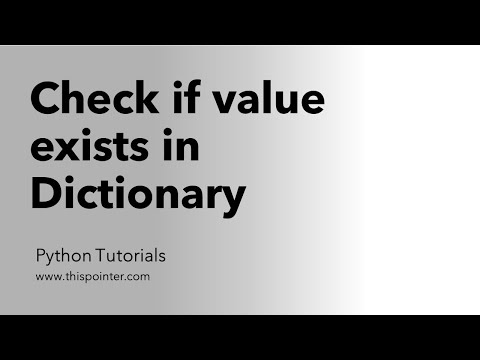
Found 43 images related to python check if keys in dict theme
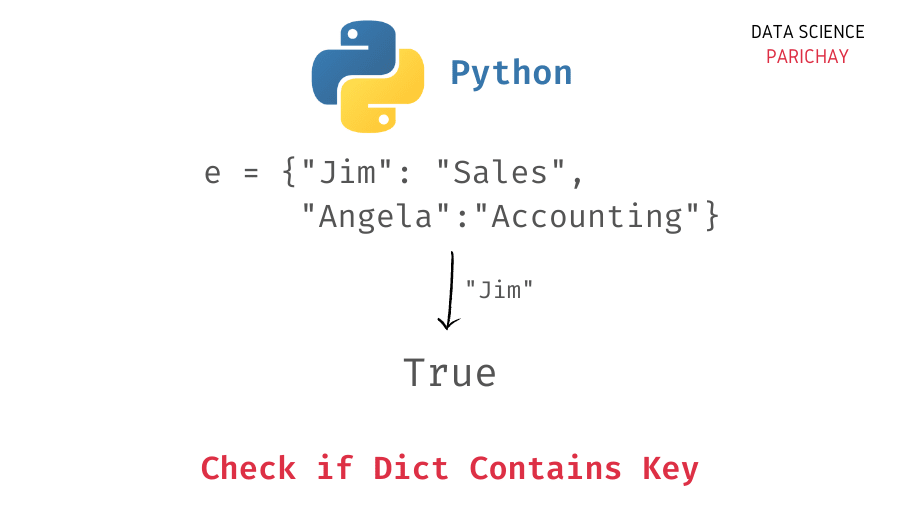


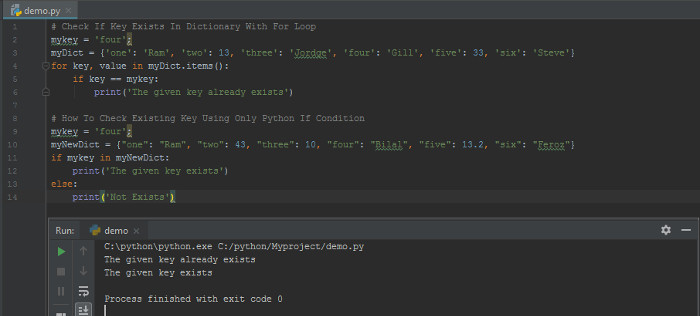
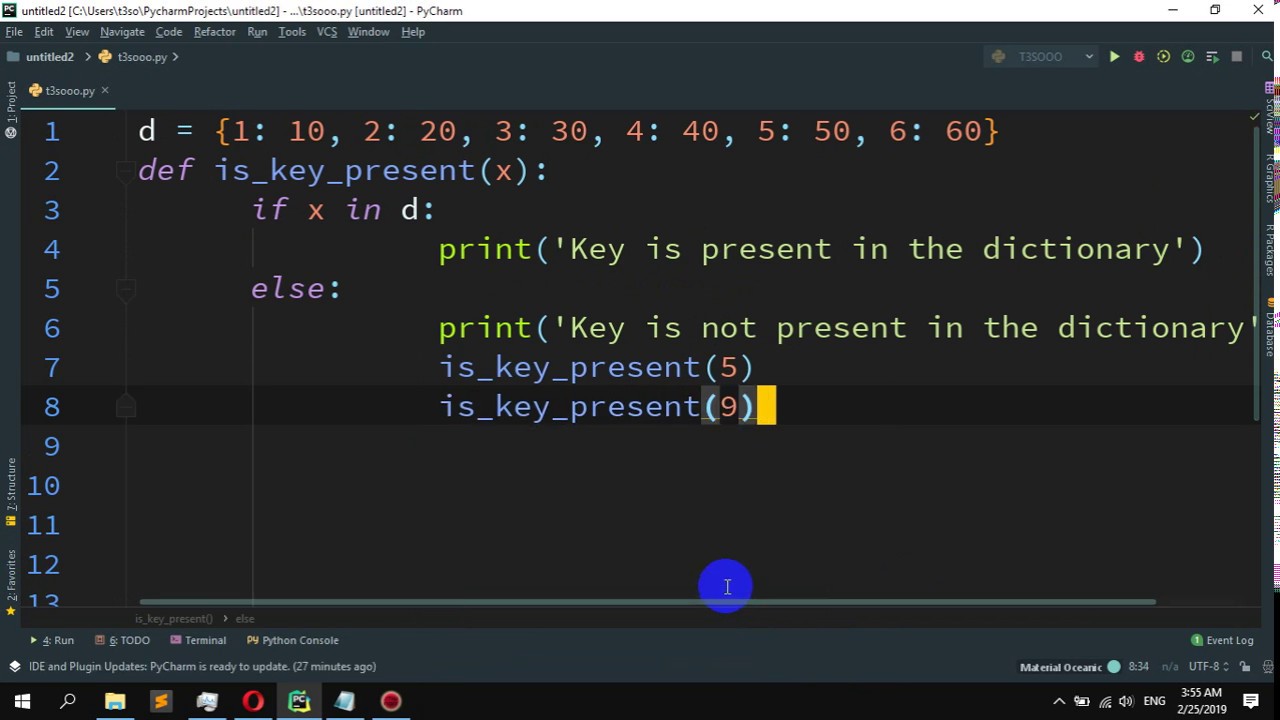
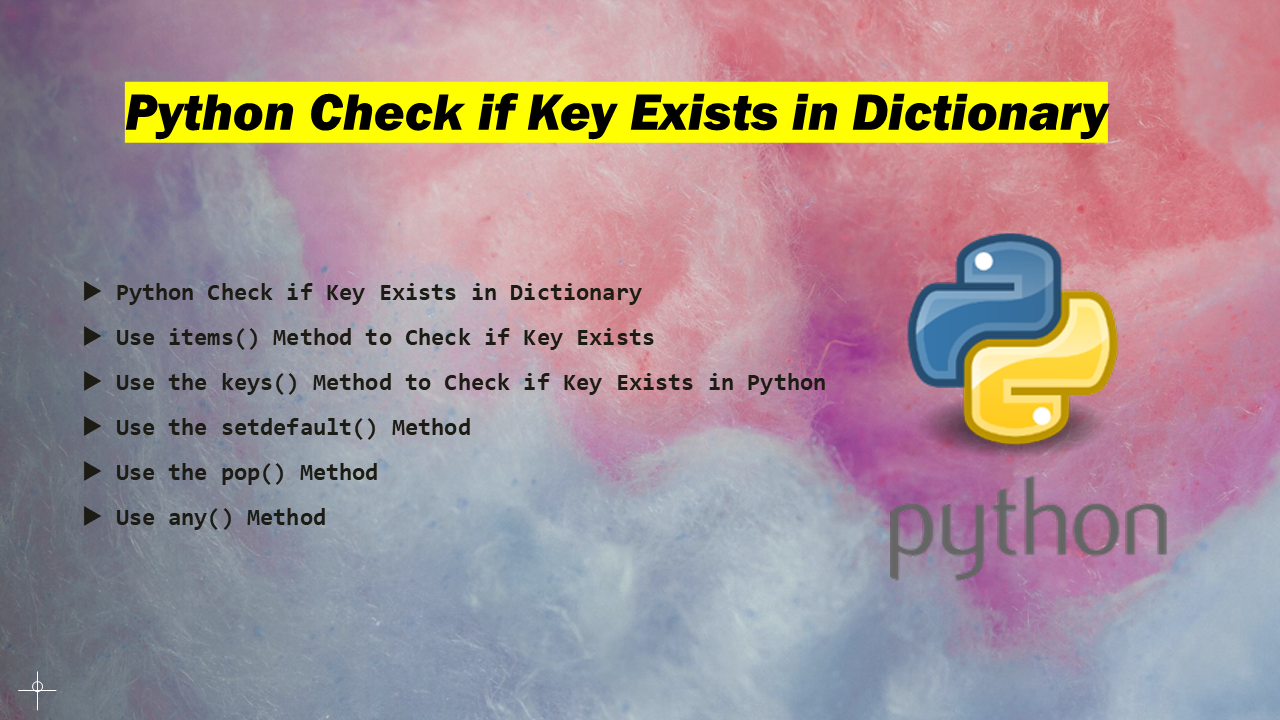
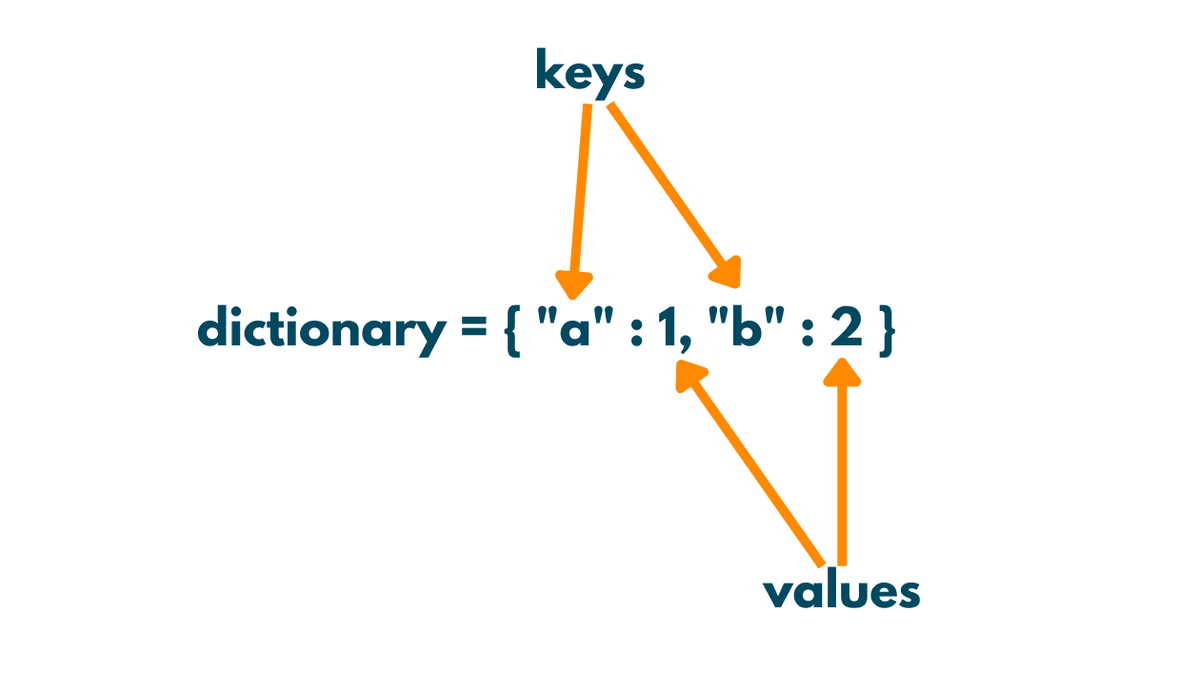
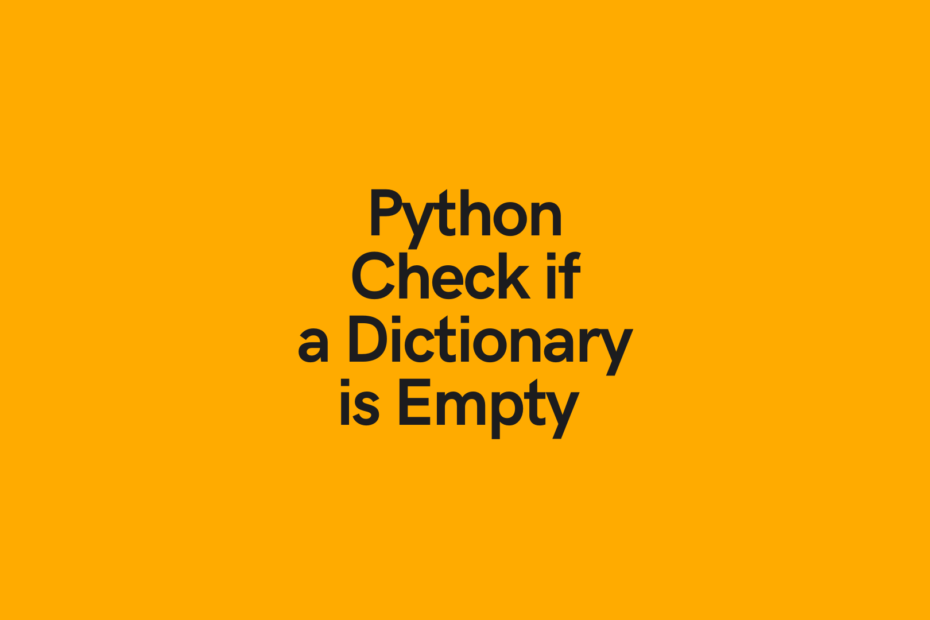

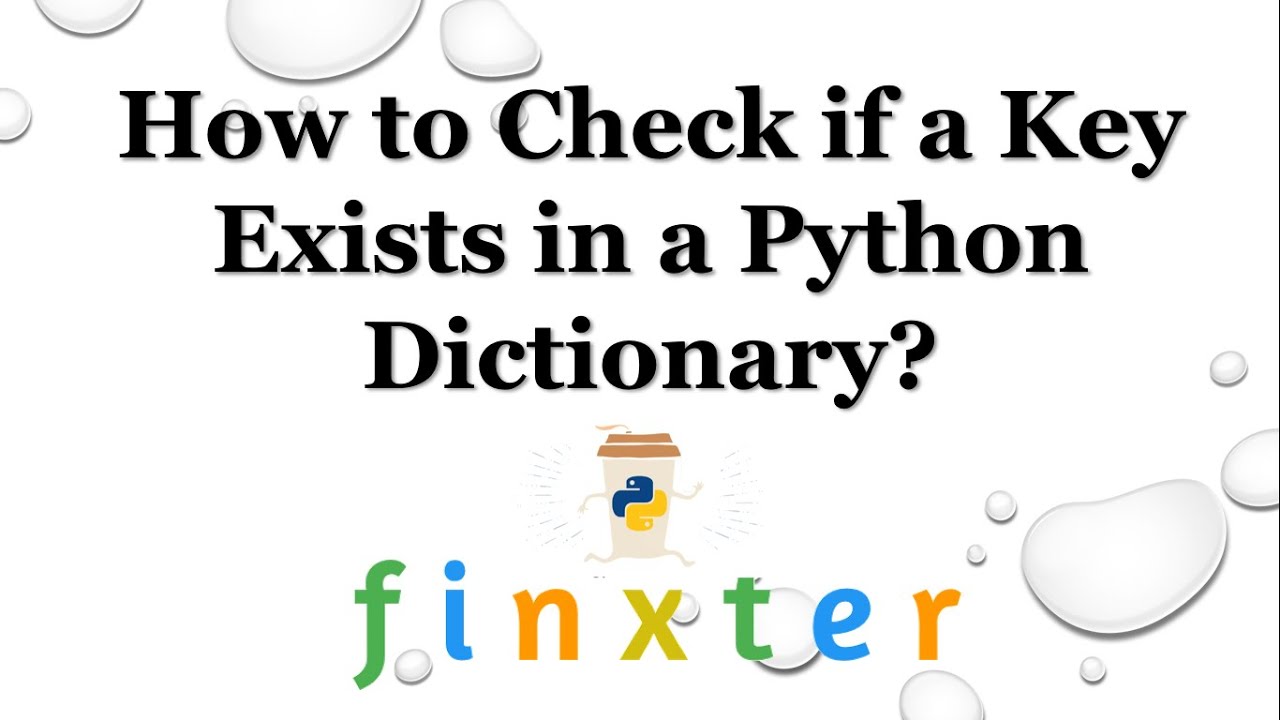
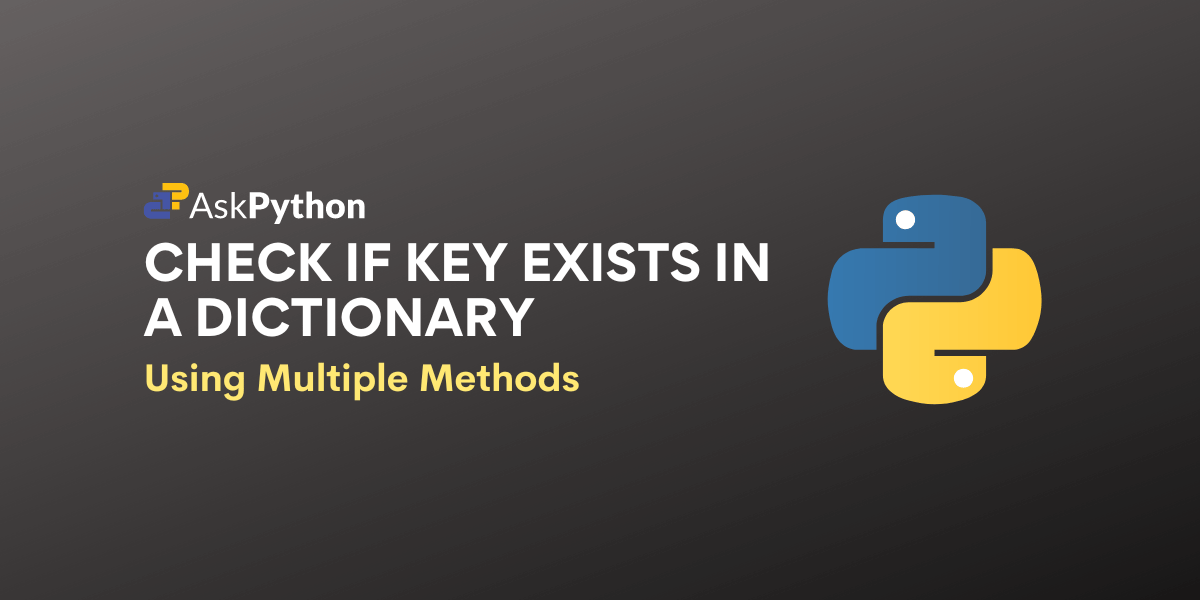
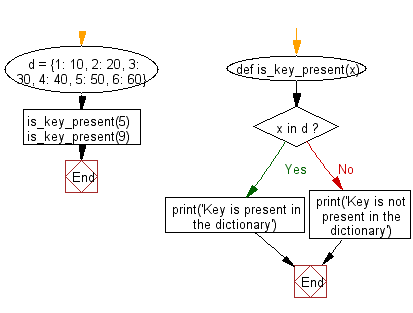
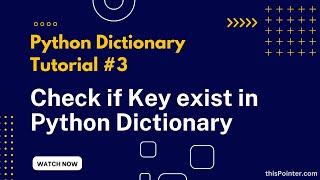

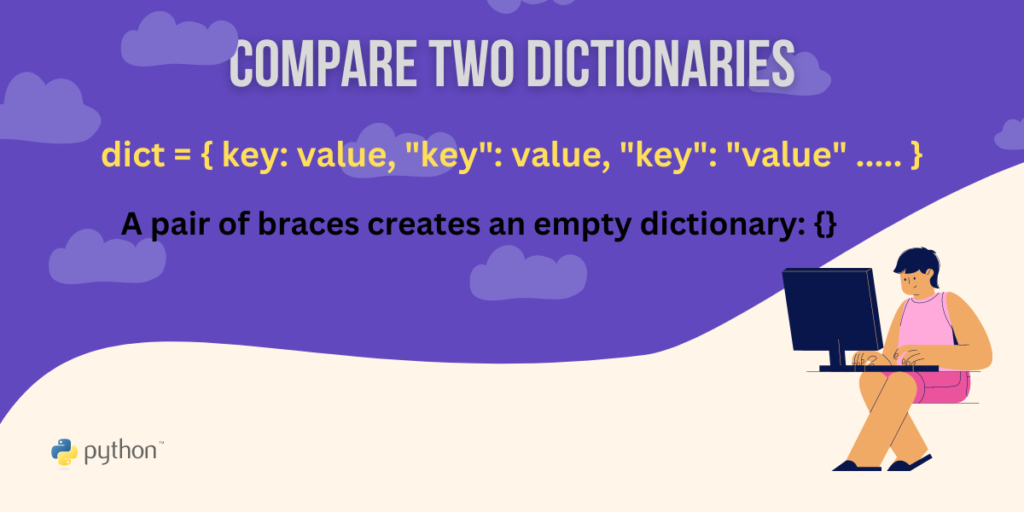


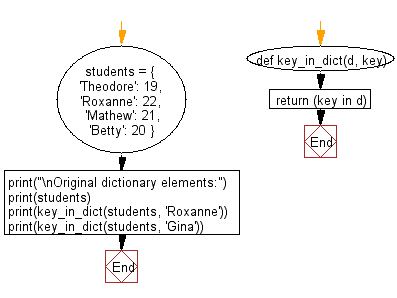
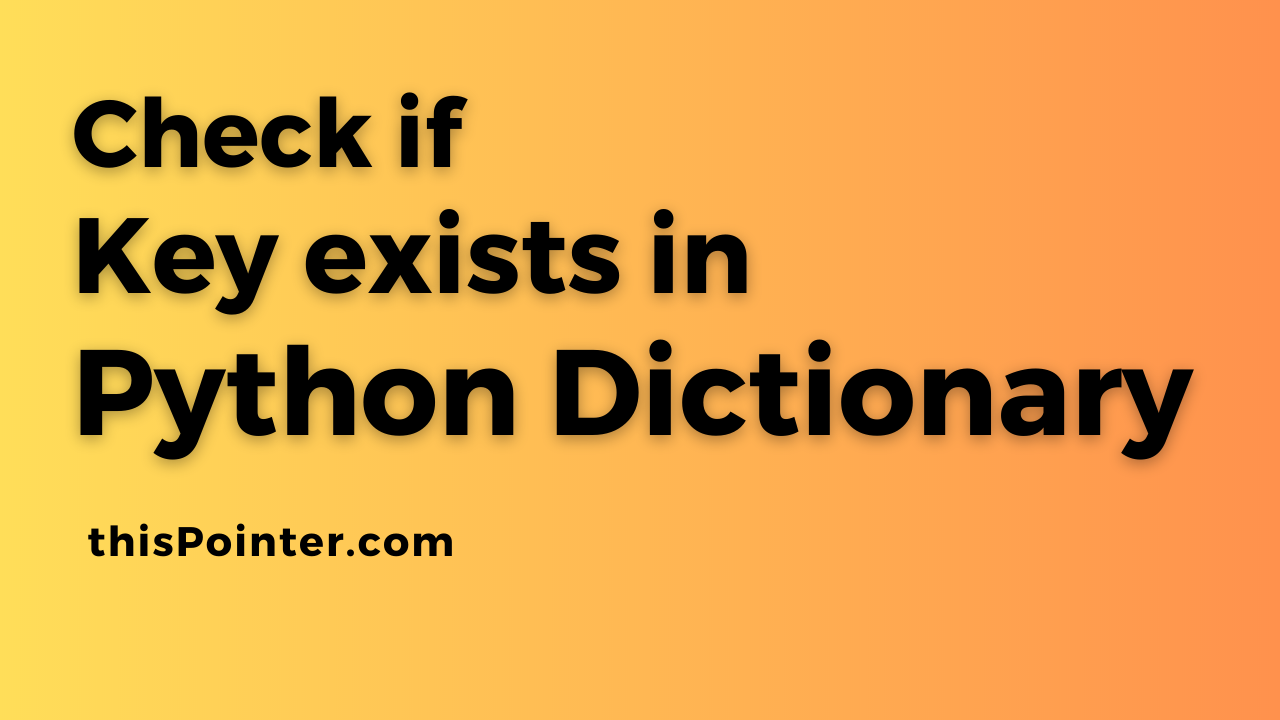

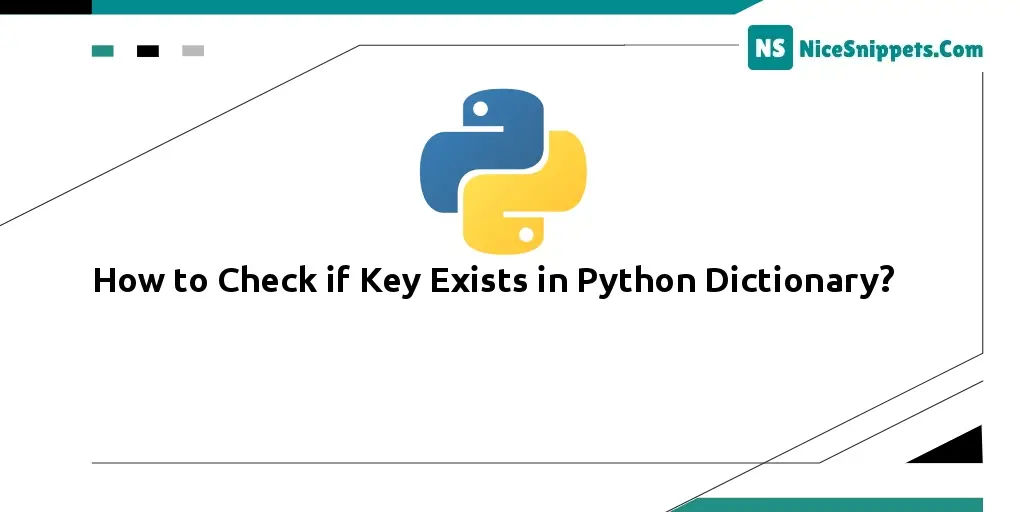
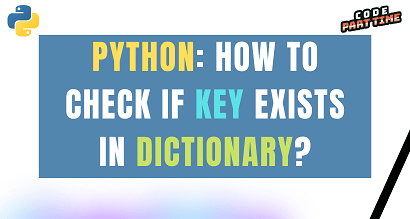
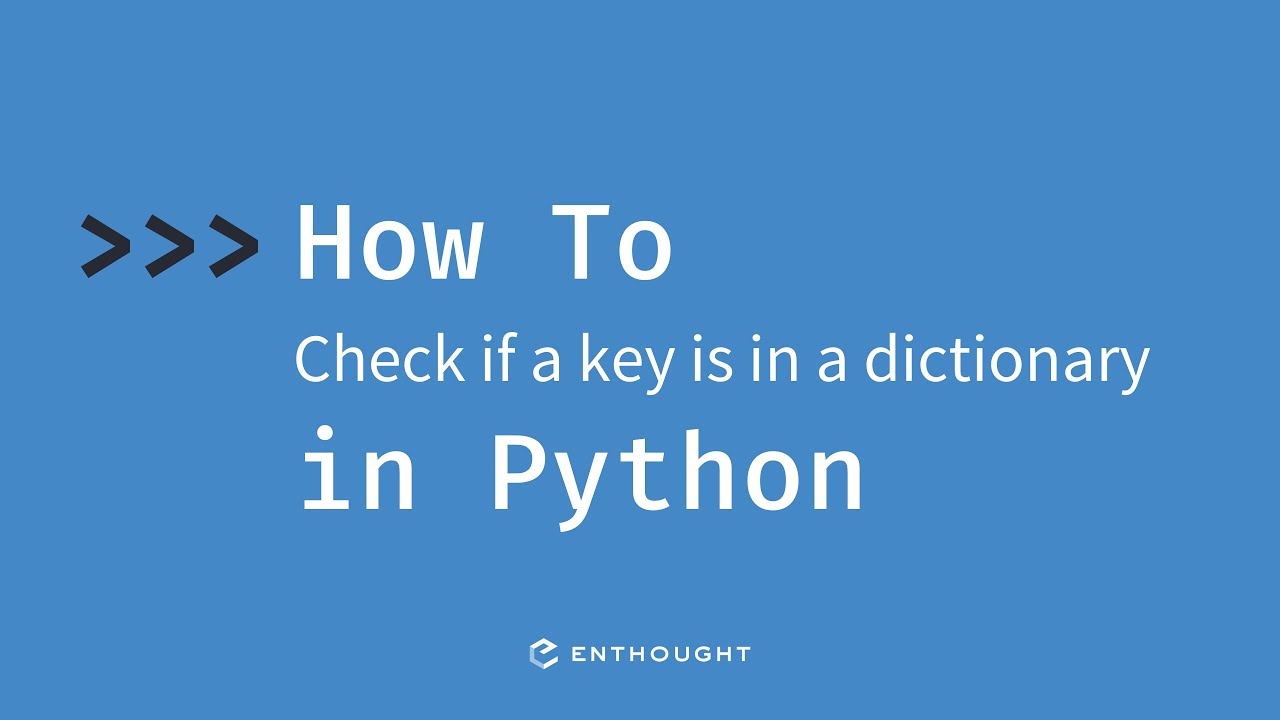
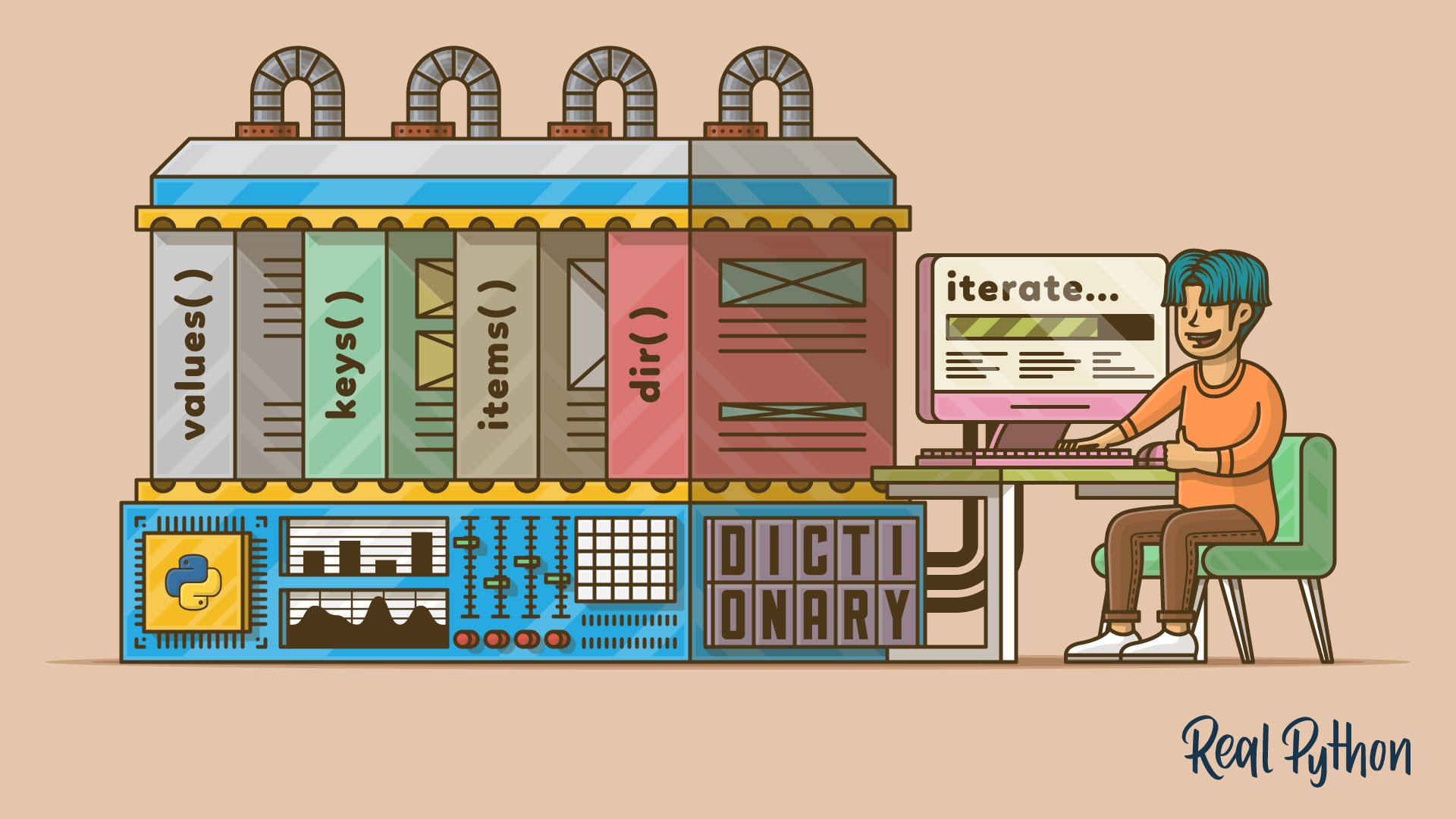
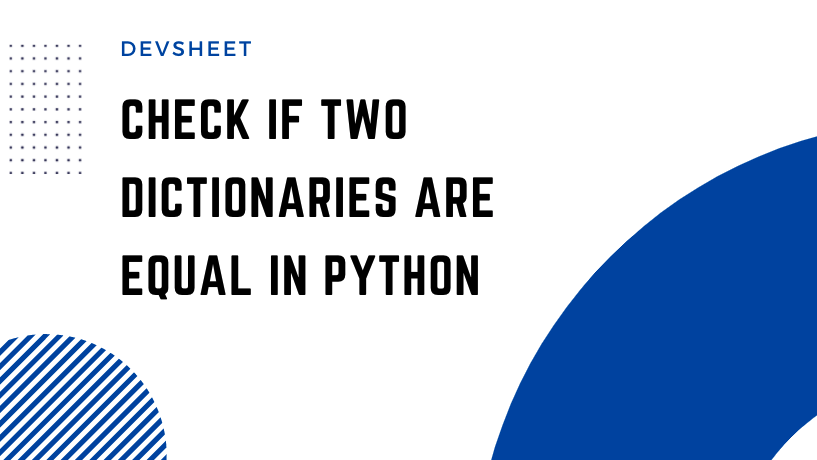
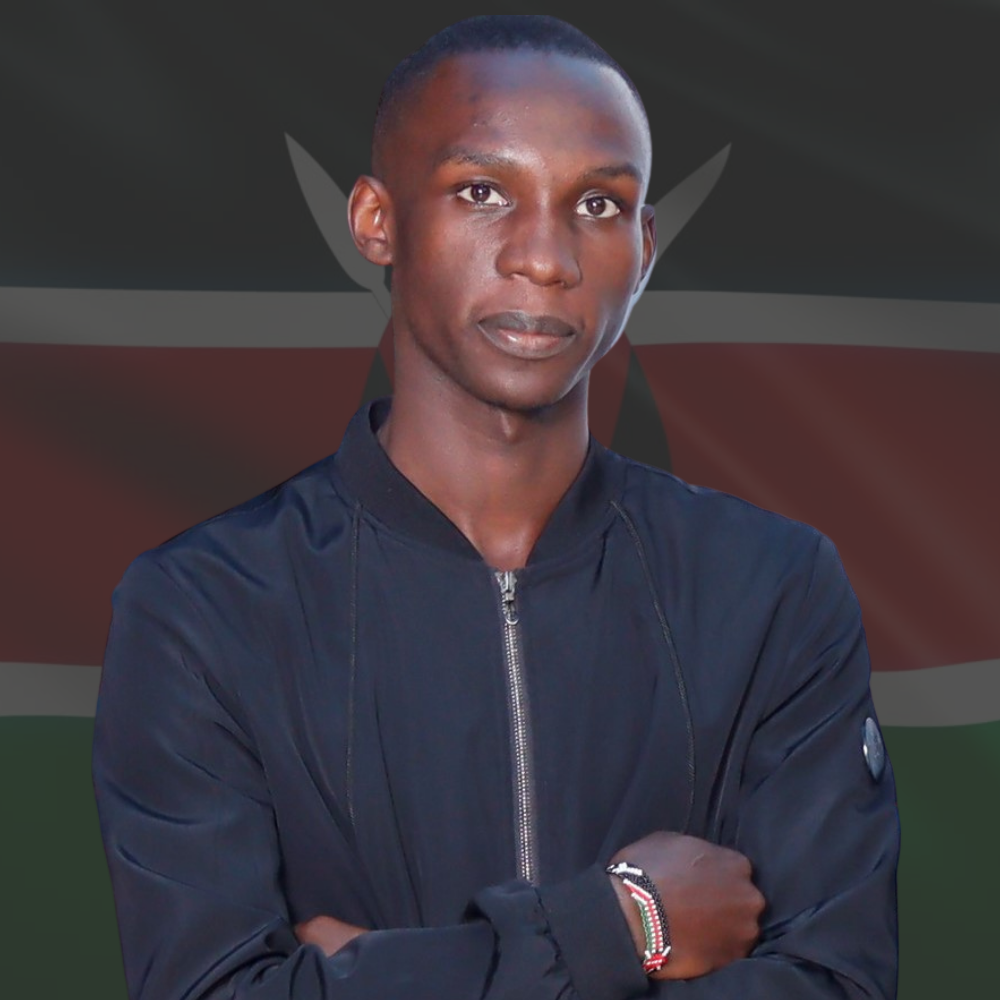
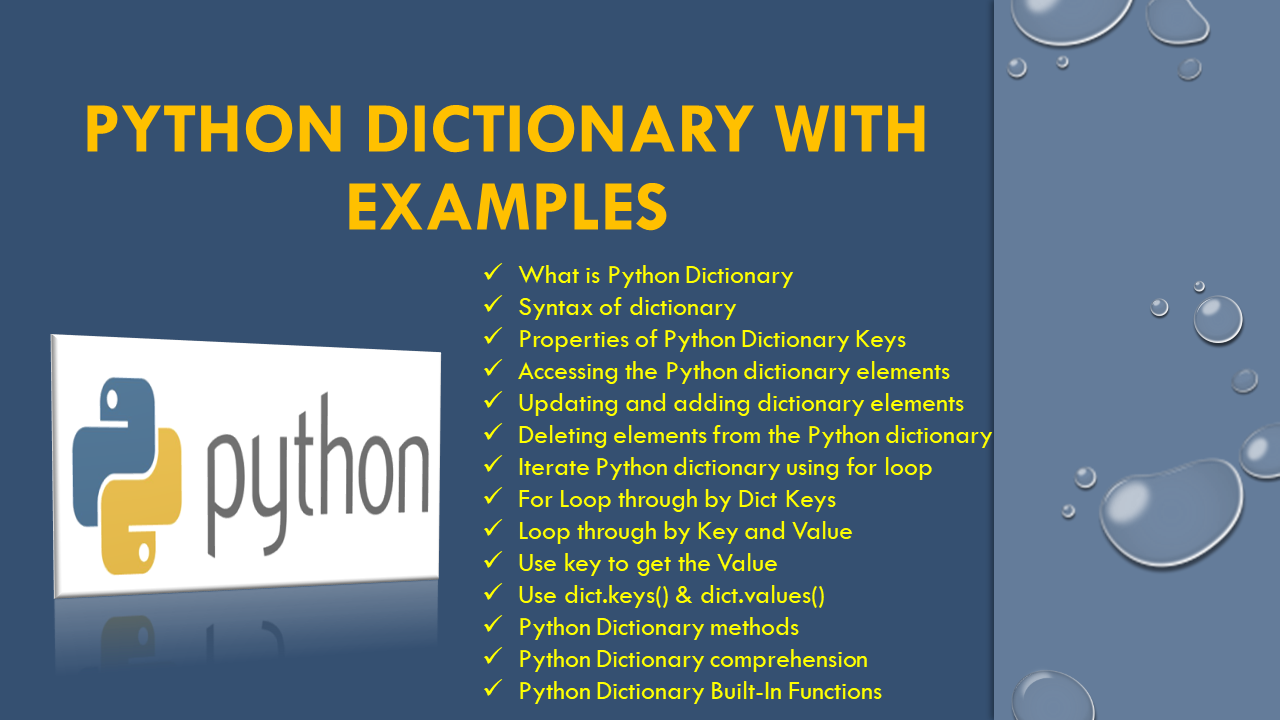


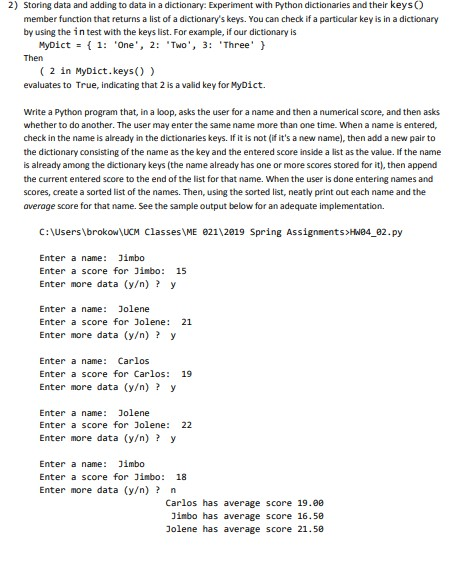
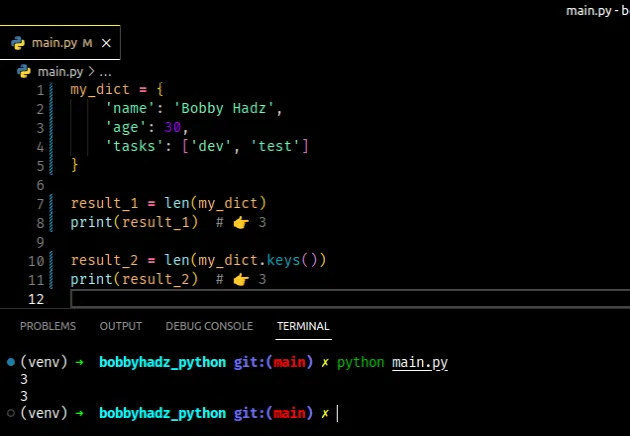

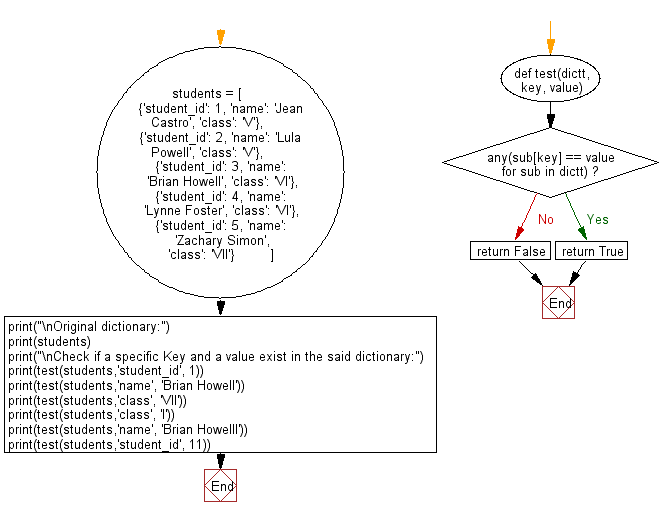
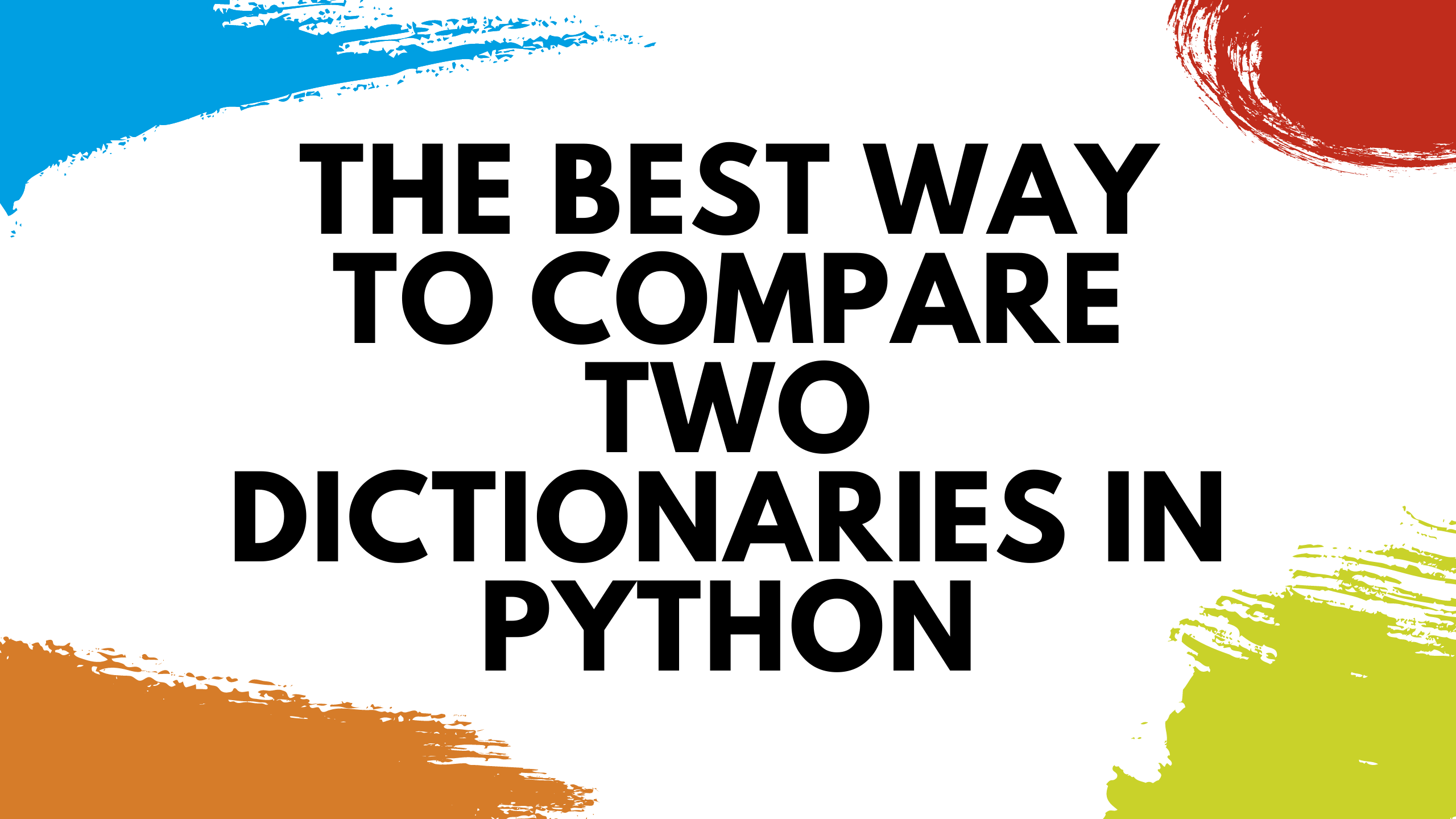
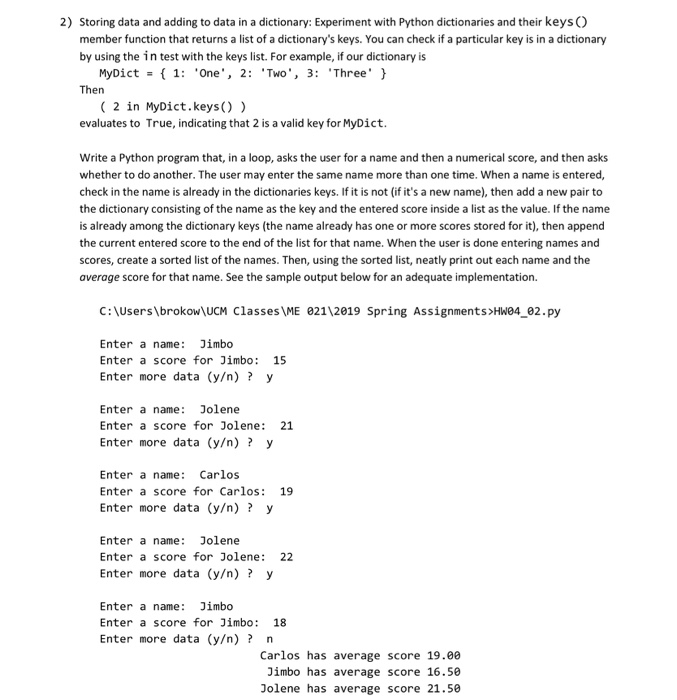


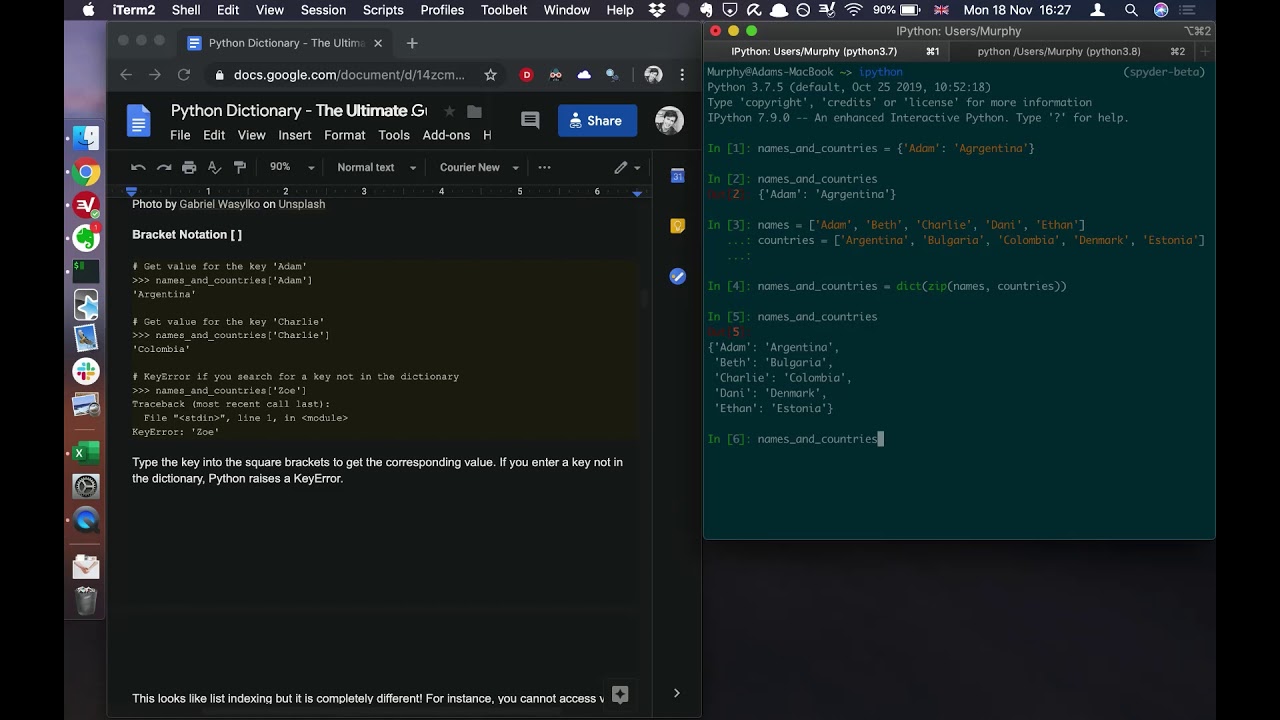
![Class 12] Julie has created a dictionary containing names and marks Class 12] Julie Has Created A Dictionary Containing Names And Marks](https://d1avenlh0i1xmr.cloudfront.net/374bed5d-d4bc-4d9f-b730-4c919131fae6/slide37.jpg)
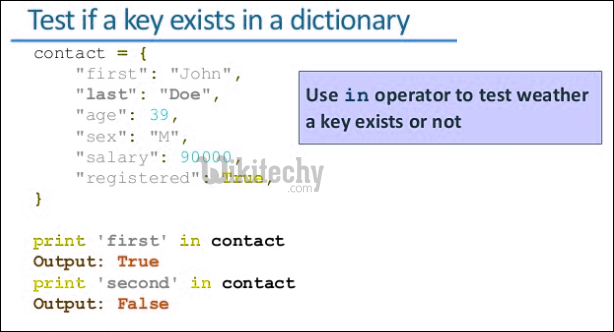
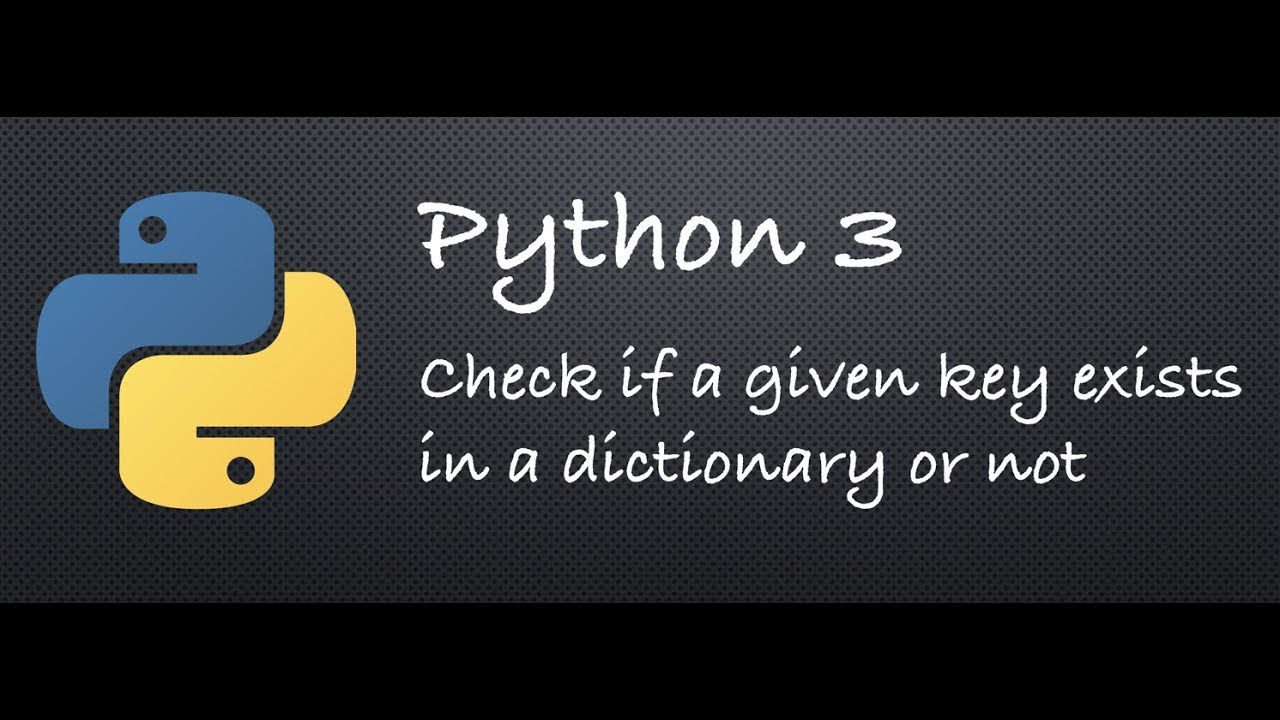
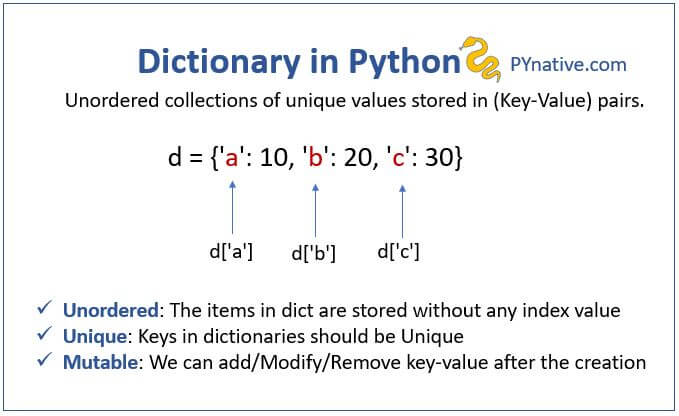
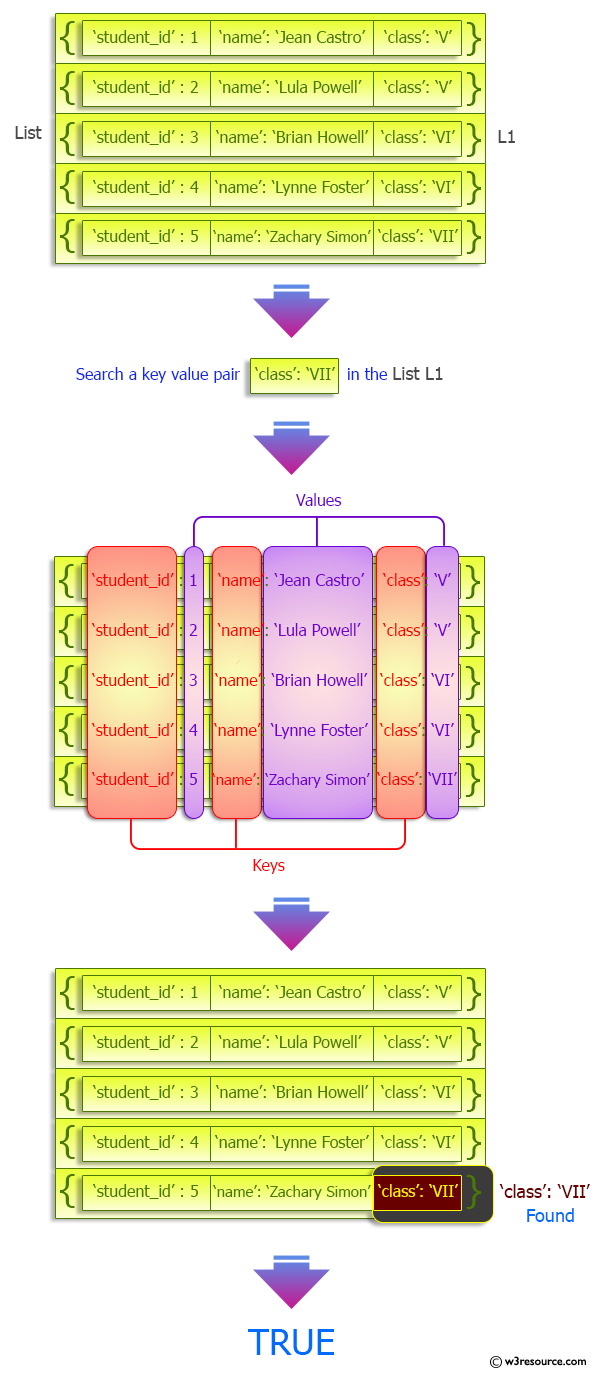
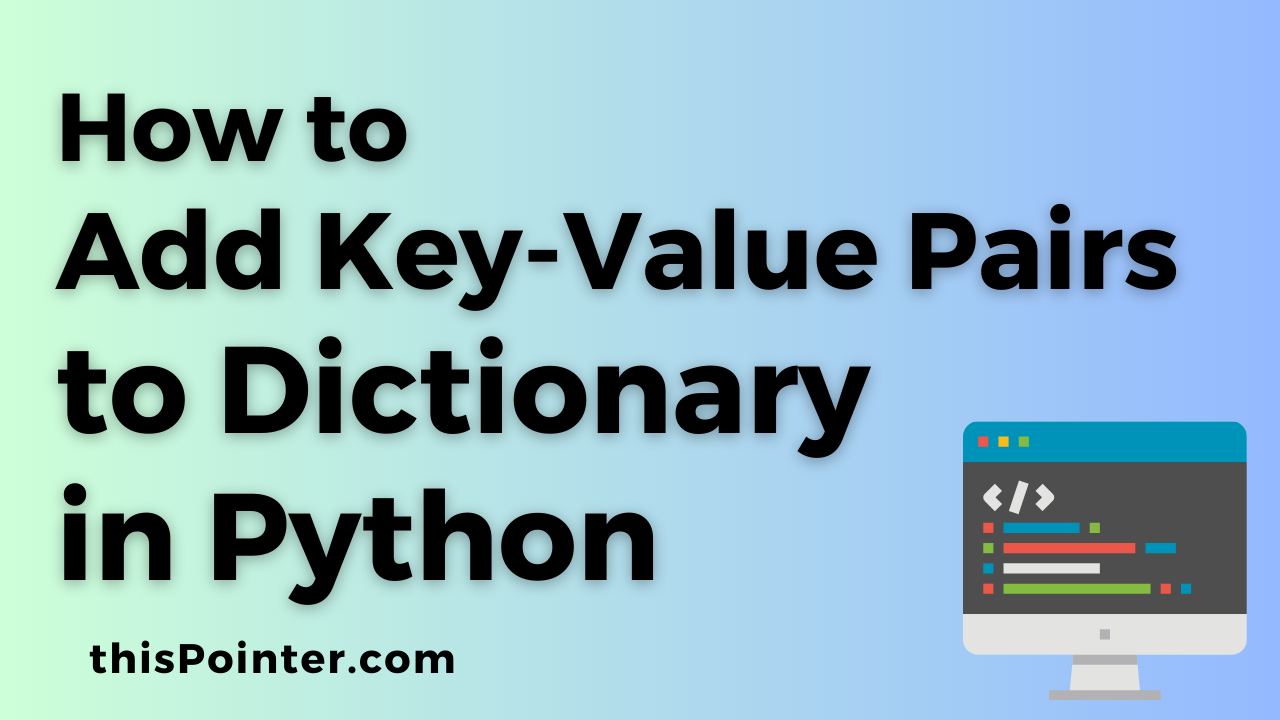
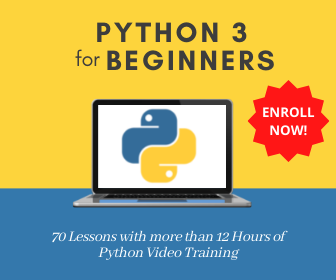
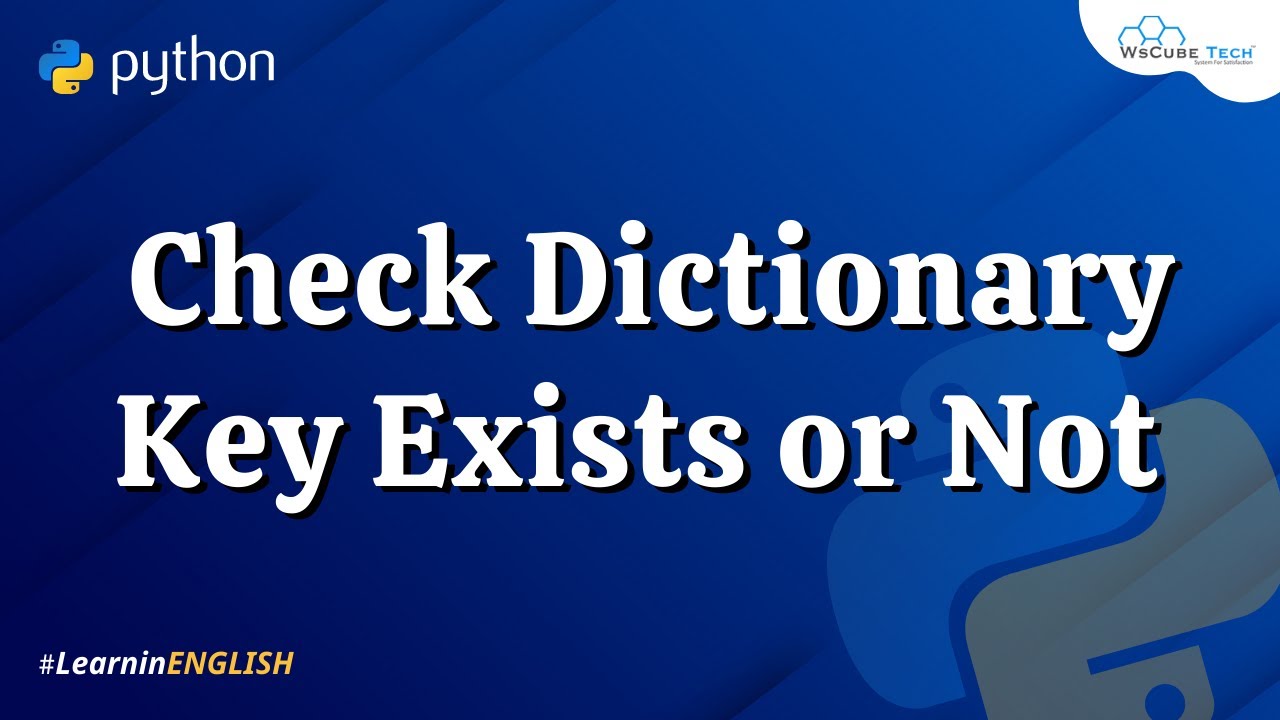
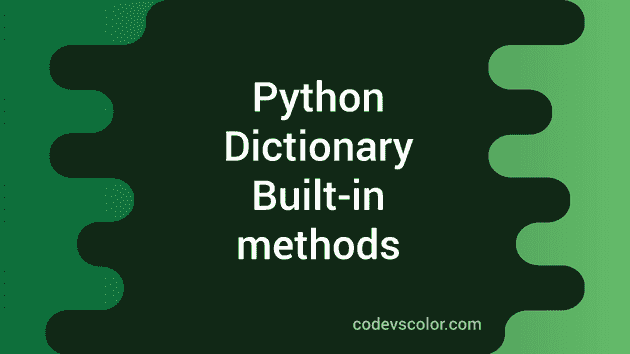
Article link: python check if keys in dict.
Learn more about the topic python check if keys in dict.
- Check if a given key already exists in a dictionary
- How to check if key exists in a python dictionary? – Flexiple
- Check if Key Exists in Dictionary Python – Scaler Topics
- How to check if a key exists in a Python dictionary – Educative.io
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if key/value exists in dictionary in Python – nkmk note
- Python | Check if key has Non-None value in dictionary – GeeksforGeeks
- Check whether given Key already exists in a Python Dictionary
- How To Check If Key Exists In Dictionary In Python?
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- Check whether given Key already exists in a Python Dictionary
- Check if Key exists in Dictionary (or Value) with Python code
- How to Check if a Key Exists in a Dictionary in Python
See more: nhanvietluanvan.com/luat-hoc