Python Delete Dict Key
Introduction:
Python is a popular programming language known for its simplicity and versatility. One of its powerful features is the dictionary, a data structure that stores key-value pairs. In this article, we will explore various methods to delete a specific key from a dictionary in Python.
Understanding the Syntax and Basic Operations of Dictionaries in Python:
Before diving into deleting keys, let’s briefly discuss dictionaries in Python. A dictionary is declared using curly braces { }, with key-value pairs separated by a colon. Keys must be unique, while values can be of any data type. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “salary”: 5000}
“`
To access the value associated with a key, you can use the square bracket notation:
“`
print(my_dict[“name”]) # Output: John
“`
Using the “del” Keyword to Delete a Specific Key in a Dictionary:
The simplest way to delete a key from a dictionary is by using the “del” keyword. Consider the following code snippet:
“`
my_dict = {“name”: “John”, “age”: 25, “salary”: 5000}
del my_dict[“age”]
“`
In this example, the “age” key and its corresponding value are removed from the dictionary. If we try to access the “age” key again, a KeyError will be raised.
Utilizing the “pop()” Method to Remove a Specific Key-Value Pair from a Dictionary:
Another way to delete a key-value pair from a dictionary is by using the “pop()” method. This method not only removes the key but also returns the corresponding value. Consider the following code:
“`
my_dict = {“name”: “John”, “age”: 25, “salary”: 5000}
removed_value = my_dict.pop(“salary”)
print(removed_value) # Output: 5000
“`
In this example, the “salary” key-value pair is deleted from the dictionary, and the value, 5000, is assigned to the variable “removed_value”.
Handling Errors and Exceptions when Deleting a Key from a Dictionary:
When deleting a key from a dictionary, you need to handle potential errors, especially if the key does not exist. To avoid a KeyError, you can use the “in” keyword to check if a key exists before attempting to delete it. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “salary”: 5000}
key = “address”
if key in my_dict:
del my_dict[key]
else:
print(“Key does not exist!”)
“`
In this code snippet, the program first checks if the key “address” exists in the dictionary. If it does, the key is deleted; otherwise, an appropriate message is printed.
Deleting Multiple Keys using the “del” Keyword and Loops:
Python allows you to delete multiple keys from a dictionary using the “del” keyword and loops. Suppose we have a dictionary of student grades and we want to remove all students who scored below a certain threshold. Here’s how we can achieve that:
“`
grades = {“Alice”: 90, “Bob”: 75, “Charlie”: 80, “Dave”: 60}
threshold = 70
keys_to_delete = []
for student, grade in grades.items():
if grade < threshold:
keys_to_delete.append(student)
for student in keys_to_delete:
del grades[student]
```
In this example, a list, "keys_to_delete," is populated with the keys of students who scored below the threshold. We then iterate over this list and delete the corresponding keys from the dictionary.
Incorporating Conditional Statements to Delete a Key based on Specific Criteria:
Sometimes, we may need to delete a key from a dictionary based on certain conditions. To achieve this, we can incorporate conditional statements. Consider the following example:
```
inventory = {
"apple": {"color": "red", "quantity": 10},
"banana": {"color": "yellow", "quantity": 5},
"orange": {"color": "orange", "quantity": 15}
}
for item, details in inventory.items():
if details["quantity"] < 5:
del inventory[item]
```
In this example, we iterate over the dictionary and delete any key-value pair where the quantity is less than 5.
Tips and Best Practices for Efficient Key Deletion in Python Dictionaries:
When deleting keys from a dictionary in Python, it is essential to keep in mind a few tips and best practices:
1. Avoid modifying a dictionary while iterating over it: Modifying a dictionary while iterating can lead to a `RuntimeError: dictionary changed size during iteration`. Instead, create a separate list of keys to delete and iterate over that list.
2. Use the "in" keyword to check for key existence: Always check if a key exists in a dictionary before deleting it. This helps avoid KeyErrors and enhances the safety of your code.
3. Consider using the "pop()" method for situations where you need both the value and key removed. It simplifies your code and improves readability.
4. If deleting multiple keys based on a condition, consider using dictionary comprehension. It allows you to create a new dictionary without duplicating keys that should be deleted.
FAQs:
1. What is the meaning of "RuntimeError: dictionary changed size during iteration"?
This error occurs when you modify a dictionary while iterating over it. It is recommended to create a separate list of keys to delete and iterate over that list instead.
2. Can I delete a key from a dictionary while iterating over it?
Yes, you can delete a key from a dictionary while iterating over it, but you need to be cautious not to modify the dictionary's size during the iteration. Using a separate list to store keys and iterating over that list is a safe approach.
3. How can I check if a key exists in a dictionary before deleting it?
You can use the "in" keyword to check if a key exists in a dictionary. By doing so, you can avoid a KeyError when attempting to delete a non-existent key.
4. How can I remove the first key-value pair from a dictionary?
Dictionaries in Python do not preserve the order of insertion, as they are inherently unordered. Hence, there is no concept of a "first" key-value pair. However, you can retrieve the first key using the next(iter(my_dict)) method and then delete it using the "del" keyword.
5. How can I delete a specific element from a dictionary?
To delete a specific element from a dictionary, you need to specify the key associated with that element. Using the "del" keyword or the "pop()" method will remove the desired element from the dictionary.
I hope this article has provided you with a comprehensive understanding of deleting keys in a Python dictionary. By following the syntax and methods explained here, you can confidently manipulate dictionaries to suit your programming needs.
Python Tutorial – How To Delete Values In Dictionary | Del | Pop() | Clear()
Keywords searched by users: python delete dict key runtimeerror: dictionary changed size during iteration, Python delete dictionary item while iterating, Delete key in dictionary Python while iterating, Get first key in dictionary – Python, Remove element in dict Python, Check key in dict Python, Python list key-value, Remove dict in list python
Categories: Top 57 Python Delete Dict Key
See more here: nhanvietluanvan.com
Runtimeerror: Dictionary Changed Size During Iteration
In the world of programming, errors are inevitable. One such error that developers often encounter is the Runtime Error: Dictionary Changed Size During Iteration. This error occurs when a dictionary is modified (i.e., items added or removed) during a loop that is iterating over the same dictionary. In this article, we will delve deeper into this error, understand its causes, and explore possible solutions to resolve it.
Understanding the Error:
When a dictionary is changed while it is being iterated over, the interpreter encounters an unexpected situation. This leads to an inconsistency between the size of the dictionary and the iteration process, resulting in the Runtime Error: Dictionary Changed Size During Iteration. Let’s take a closer look at how this error can manifest in different scenarios.
Causes of Runtime Error: Dictionary Changed Size During Iteration:
1. Adding or removing items: One common cause of this error is when an item is added or removed from the dictionary during a loop that iterates over the same dictionary. This modification leads to a change in the dictionary’s size, which causes the iteration process to fail.
2. Nested loops: Another cause of this error is when nested loops are used, and modifications are made to the dictionary in the inner loop. As a result, the size of the dictionary changes, causing the outer loop to throw the runtime error.
3. Incorrect use of dictionary methods: Incorrect usage of dictionary methods, such as `del()` or `pop()`, within a loop that is iterating over the dictionary can also trigger this error. Modifying the dictionary while iterating disrupts the iteration process and raises the runtime error.
Resolving the Runtime Error: Dictionary Changed Size During Iteration:
To overcome this error, developers need to be cautious when modifying a dictionary during iteration. Here are some strategies to handle the RuntimeError gracefully:
1. Create a copy of the dictionary: Instead of modifying the dictionary directly, create a copy of it and iterate over the copy. This prevents any size changes in the original dictionary during iteration. To achieve this, use the `copy()` method or the dictionary copy constructor.
2. Use a list to store items to be deleted: In cases where items need to be removed from the dictionary during iteration, instead of deleting them directly, store the keys in a separate list. After the iteration is complete, iterate over the list and delete the corresponding items from the dictionary. This ensures that the iteration process is not affected by changes in the dictionary size.
3. Use thread synchronization mechanisms: If you are working with multi-threaded applications where multiple threads modify the dictionary, consider using thread synchronization mechanisms like locks or semaphores. These mechanisms help in preventing simultaneous modifications to the dictionary during iteration.
4. Convert the dictionary to a list: In situations where the order of iteration is not important, converting the dictionary to a list of tuples can be a viable solution. By doing so, you avoid the dictionary size changes during iteration, as lists can be safely modified in the loop.
Frequently Asked Questions (FAQs):
Q1. Can I modify a dictionary while using a for loop in Python?
A1. Technically, it is not recommended to modify a dictionary during a for loop in Python. However, if you must, there are ways to handle it properly, such as creating a copy of the dictionary or using a separate list to store items for deletion.
Q2. What other looping structures can I use without encountering this error?
A2. In addition to the for loop, alternatives like the while loop can be used effectively without triggering the Runtime Error: Dictionary Changed Size During Iteration. Be cautious about modifications during iteration, regardless of the looping structure used.
Q3. How can I avoid runtime errors while iterating over nested dictionaries?
A3. When dealing with nested dictionaries, it is essential to consider the depth of the iteration and the depth of modifications. Use temporary variables to store references to inner dictionaries and modify them outside the loop to avoid the runtime error.
Q4. Are there any tools available to detect and prevent this error?
A4. Various static code analyzers, such as pylint or pyflakes, can help detect some scenarios that may cause this error. However, it is ultimately the programmer’s responsibility to ensure proper handling of dictionary modifications during iteration.
In conclusion, the Runtime Error: Dictionary Changed Size During Iteration can be a common and frustrating issue for developers. By understanding the causes and implementing appropriate strategies, such as creating a copy of the dictionary or using a list for item deletion, developers can effectively overcome this error. Remember to be cautious when modifying dictionaries during iteration and consider alternative looping structures when necessary.
Python Delete Dictionary Item While Iterating
In Python, dictionaries are a versatile data structure that allows you to store key-value pairs. Sometimes, you may need to iterate over a dictionary and remove certain items based on specific conditions. However, deleting dictionary items while iterating can be a challenging task since it may lead to unexpected behavior or even runtime errors. This article will explore different approaches to safely delete dictionary items while iterating in Python, along with common pitfalls and best practices.
Understanding Dictionary Iteration in Python
Before diving into deleting dictionary items, let’s have a quick overview of how dictionary iteration works in Python. When you iterate over a dictionary using a `for` loop, the loop variable takes on the keys of the dictionary by default. To access the associated values, you need to use the keys to retrieve them from the dictionary explicitly.
“`python
my_dict = {‘apple’: 3, ‘banana’: 4, ‘orange’: 2}
for key in my_dict:
value = my_dict[key]
print(key, value)
“`
Output:
“`
apple 3
banana 4
orange 2
“`
Deleting Dictionary Items Safely
Now, let’s discuss different methods to delete dictionary items while iterating, ensuring safe and predictable behavior.
Method 1: Create a Copy of the Dictionary
One straightforward method is to create a copy of the dictionary and iterate over the copy while deleting items from the original dictionary. This approach avoids modifying the dictionary being iterated over, preventing potential errors or unexpected behavior.
“`python
my_dict = {‘apple’: 3, ‘banana’: 4, ‘orange’: 2}
for key in list(my_dict.keys()):
if my_dict[key] < 3:
del my_dict[key]
print(my_dict)
```
Output:
```
{'banana': 4}
```
Method 2: Using Dictionary Comprehension
Another elegant approach is to use dictionary comprehension, which allows you to create a new dictionary based on conditions applied to the original dictionary. By filtering out the unwanted items, you can effectively delete them.
```python
my_dict = {'apple': 3, 'banana': 4, 'orange': 2}
my_dict = {k: v for k, v in my_dict.items() if v >= 3}
print(my_dict)
“`
Output:
“`
{‘apple’: 3, ‘banana’: 4}
“`
Method 3: Using the `pop()` Method
The `pop()` method allows you to remove an item from the dictionary by specifying its key. To safely delete items while iterating, you can maintain a list of keys to be deleted and remove them after the iteration is complete.
“`python
my_dict = {‘apple’: 3, ‘banana’: 4, ‘orange’: 2}
keys_to_delete = []
for key, value in my_dict.items():
if value < 3:
keys_to_delete.append(key)
for key in keys_to_delete:
my_dict.pop(key)
print(my_dict)
```
Output:
```
{'banana': 4}
```
Frequently Asked Questions (FAQs)
Q1: Why is it a bad idea to delete dictionary items directly while iterating?
Iterating over a dictionary creates an iterator that keeps track of its internal state. If an item is deleted from the dictionary during iteration, it can disrupt the iterator's state, leading to unexpected behavior or even raising a `RuntimeError`. By avoiding direct deletion, you ensure the integrity and stability of the iteration process.
Q2: What happens if I delete items from a dictionary during iteration without using the suggested safe methods?
Deleting items without applying the safe methods can lead to unpredictable behavior. Some items may be skipped, while others may be processed multiple times. The result can be inaccurate or incorrect, causing subtle bugs that are difficult to identify and debug.
Q3: Can I modify dictionary items while iterating without deleting them?
Yes, you can modify dictionary items directly while iterating, as long as you do not change the dictionary's size. Modifying values or attributes of dictionary items will not disrupt the iteration process. However, if you add or remove items, it is recommended to use safe deletion methods.
Q4: Can I use these safe deletion methods in nested loops?
Yes, these methods can be used in nested loops without additional precautions. They ensure safe deletion regardless of the level of nesting. However, be mindful of the performance impact of nested deletion operations on larger dictionaries.
In conclusion, safely deleting dictionary items while iterating is an essential skill for Python programmers. By employing approaches like creating a copy, using dictionary comprehension, or utilizing the `pop()` method with careful handling, you can confidently delete dictionary items without introducing unexpected behavior or runtime errors. Adhering to these safe deletion practices ensures the integrity and correctness of your code when dealing with dictionaries in Python.
Delete Key In Dictionary Python While Iterating
When working with dictionaries in Python, it is common to iterate through the key-value pairs using loops or list comprehensions. The Delete key allows us to remove certain items from the dictionary while iterating, allowing us to filter out unwanted data.
To better understand this concept, let’s take a look at a simple example:
“`python
my_dict = {“apple”: 5, “banana”: 7, “orange”: 2, “mango”: 4}
for fruit, quantity in my_dict.items():
if quantity < 5:
del my_dict[fruit]
print(my_dict)
```
In this example, we have a dictionary `my_dict` with fruits as keys and their respective quantities as values. We want to delete any key-value pair where the quantity is less than 5.
Inside the loop, we use an `if` statement to check if the quantity is less than 5. If it is, we use the `del` keyword followed by the dictionary name and the key in square brackets. This deletes the key-value pair from the dictionary.
However, there is a potential issue with this approach. In Python, modifying the size of a dictionary while iterating over it can lead to unpredictable behavior. It may cause errors or skip certain items. To overcome this problem, we can create a copy of the dictionary and iterate over the copy instead. This way, we can safely delete key-value pairs without affecting the original dictionary.
Here's an example that demonstrates this approach:
```python
my_dict = {"apple": 5, "banana": 7, "orange": 2, "mango": 4}
for fruit, quantity in my_dict.copy().items():
if quantity < 5:
del my_dict[fruit]
print(my_dict)
```
By using the `copy()` method on the dictionary before iterating, we ensure that modifications to the dictionary do not affect the iteration process.
Now, let's address some frequently asked questions about using the Delete key in dictionary iteration:
Q: Can I delete key-value pairs while iterating using a list comprehension?
A: No, list comprehensions are not suitable for deleting items from a dictionary during iteration. You should use a traditional loop or the `copy()` method to perform deletions safely.
Q: What happens if I try to delete a key that doesn't exist in the dictionary?
A: If you try to delete a key that does not exist, it will raise a `KeyError` exception. To avoid such errors, you can use the `in` keyword to check if the key exists before deleting.
Q: Is it possible to delete multiple key-value pairs simultaneously?
A: Yes, it is possible to delete multiple key-value pairs at once by passing a list of keys to the `del` statement. For example:
```python
my_dict = {"apple": 5, "banana": 7, "orange": 2, "mango": 4}
keys_to_delete = ["banana", "orange"]
for key in keys_to_delete:
del my_dict[key]
print(my_dict)
```
This will delete the "banana" and "orange" key-value pairs from the dictionary.
Q: Are there any alternatives to the Delete key for removing dictionary items during iteration?
A: Yes, instead of deleting items while iterating, you can create a new dictionary that only contains the desired key-value pairs. This can be done by using a dictionary comprehension with a filtering condition.
Here's an example that demonstrates this approach:
```python
my_dict = {"apple": 5, "banana": 7, "orange": 2, "mango": 4}
new_dict = {k: v for k, v in my_dict.items() if v >= 5}
print(new_dict)
“`
In this case, we create a new dictionary `new_dict` where only key-value pairs with a value equal to or greater than 5 are included.
In conclusion, the Delete key is a useful tool for removing specific key-value pairs during iteration in Python dictionaries. However, it is important to be cautious when modifying the dictionary size while iterating to avoid unexpected behavior. By using the `copy()` method or creating a new filtered dictionary, you can safely delete items without affecting the original dictionary.
Images related to the topic python delete dict key
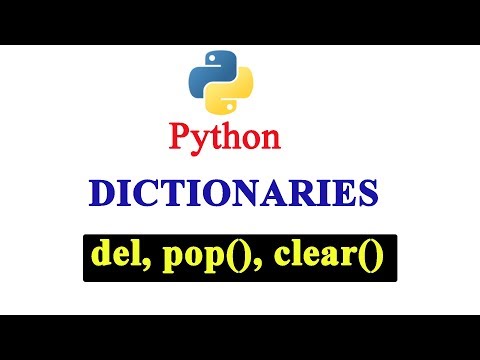
Found 41 images related to python delete dict key theme




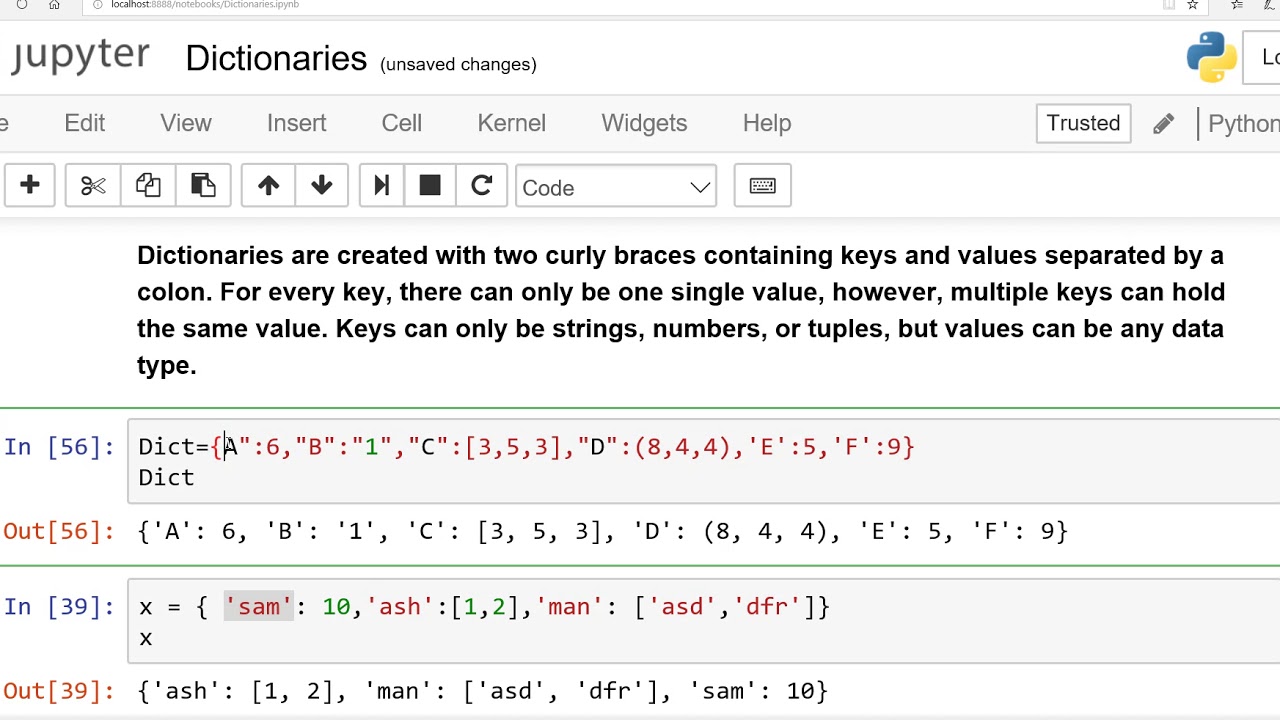
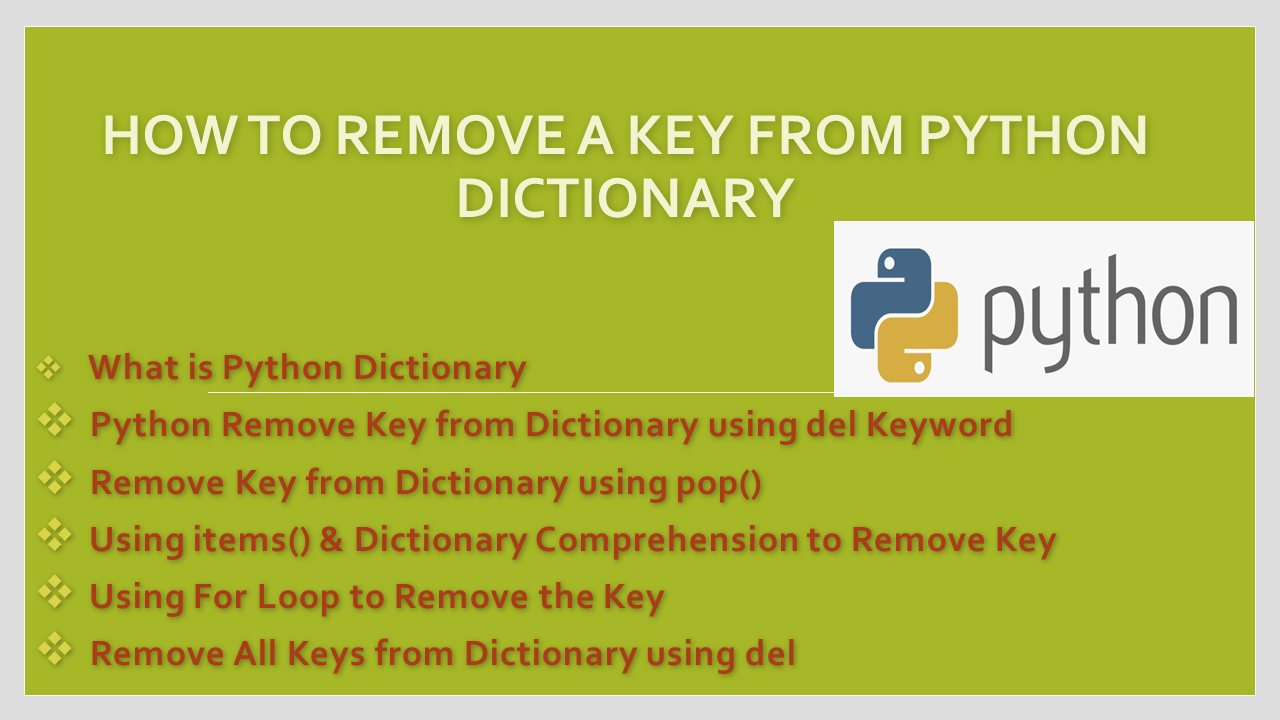

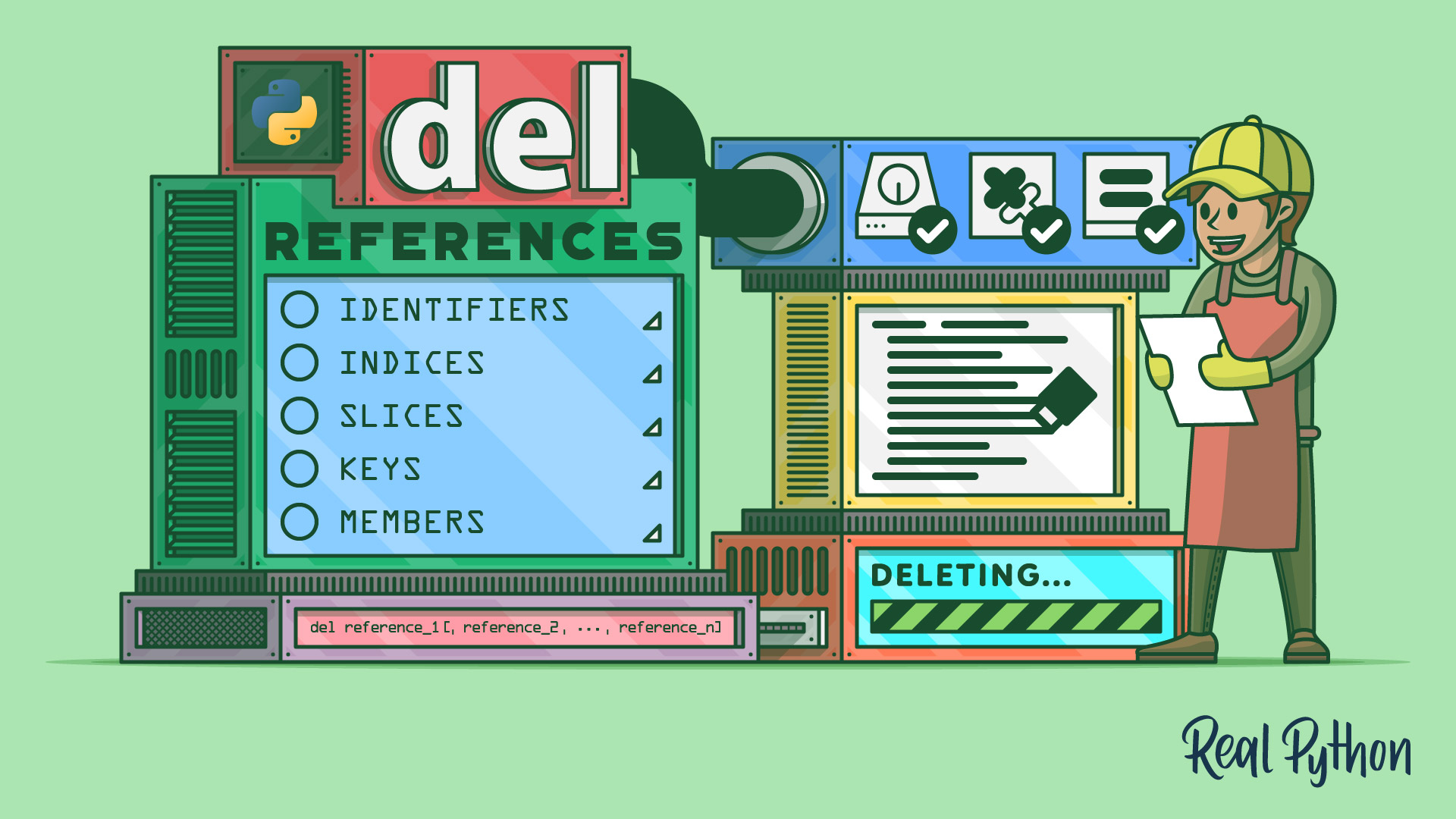
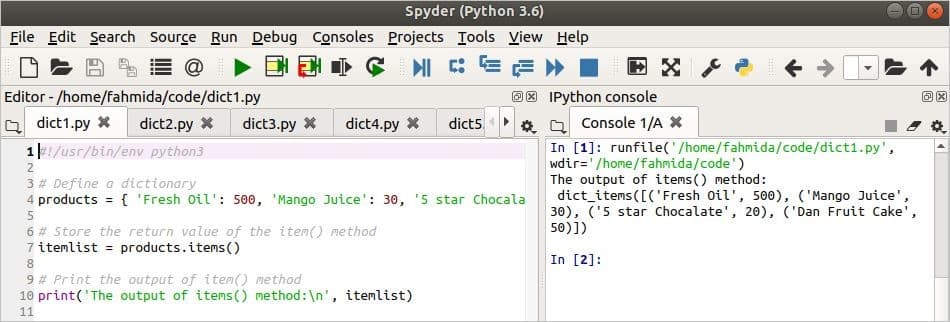
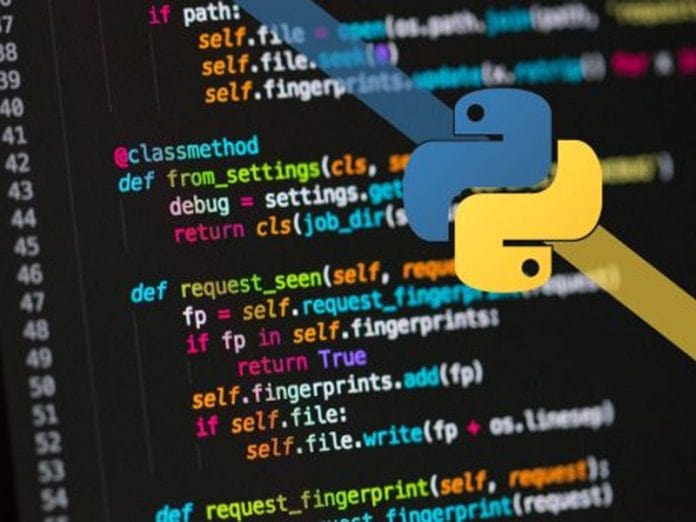

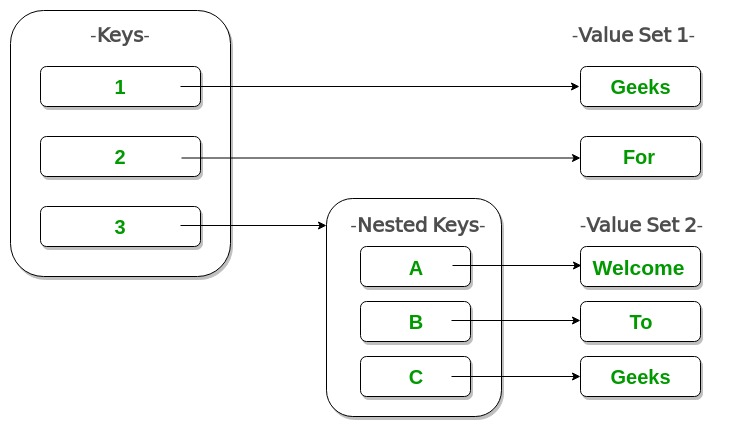
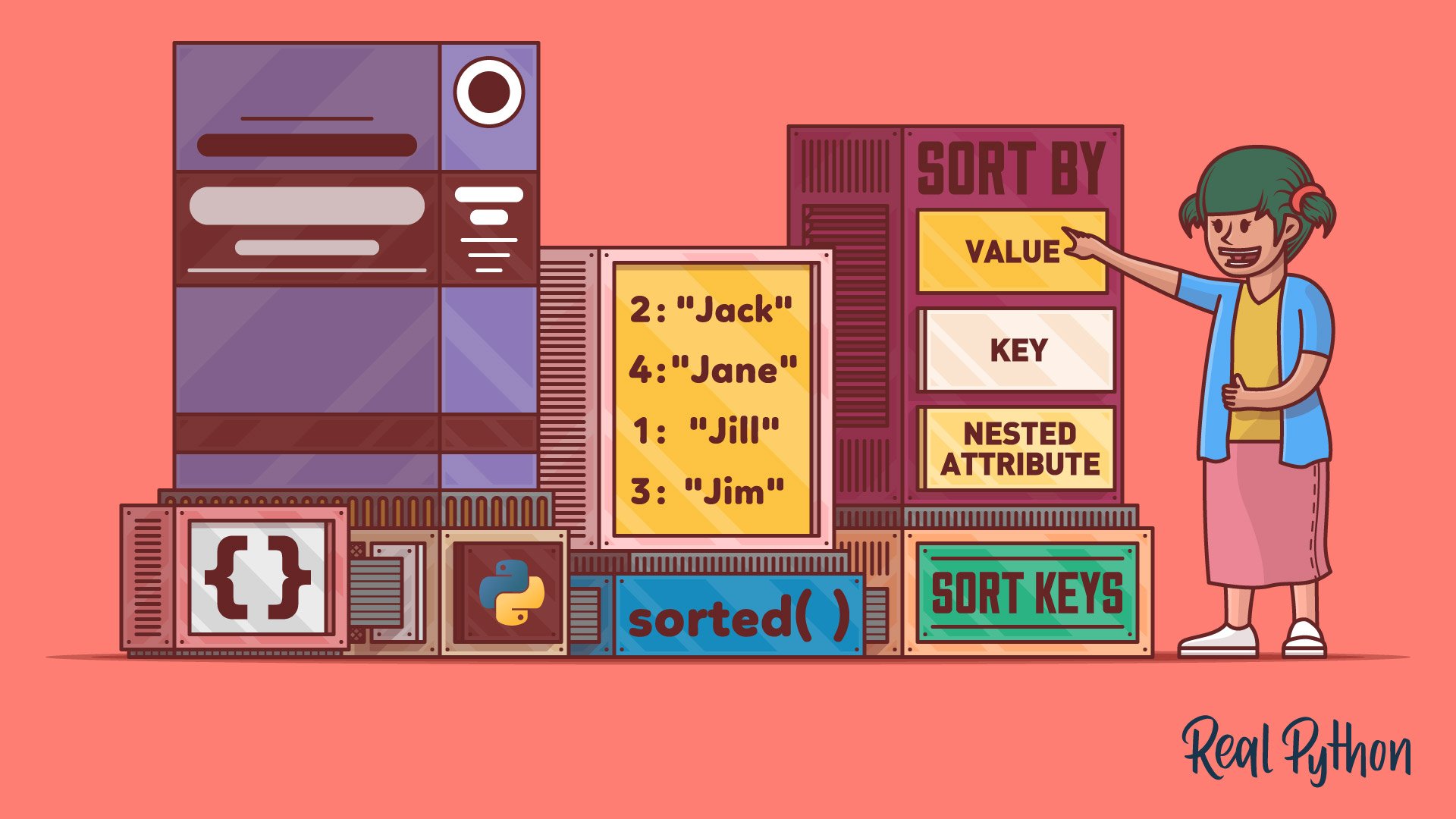


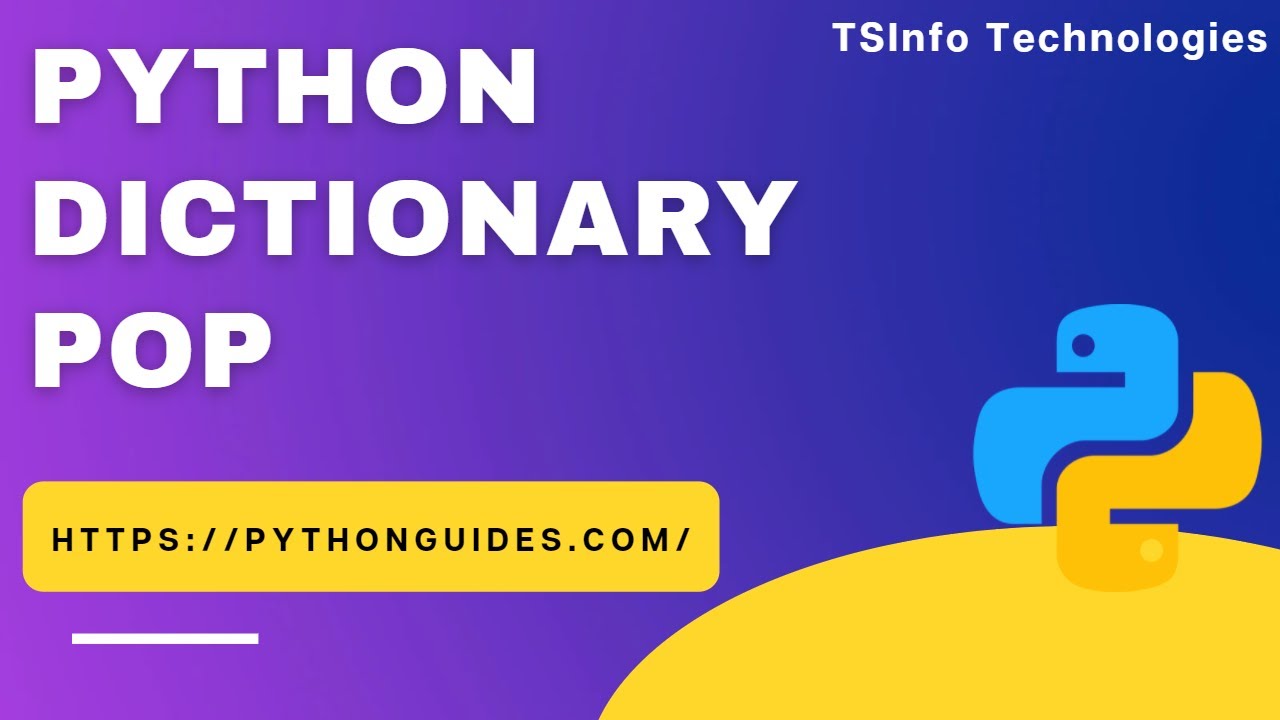
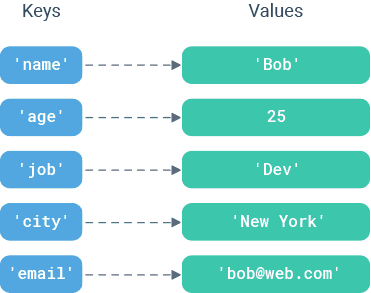
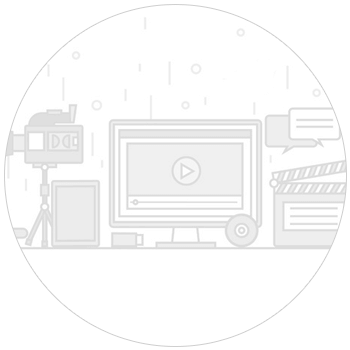
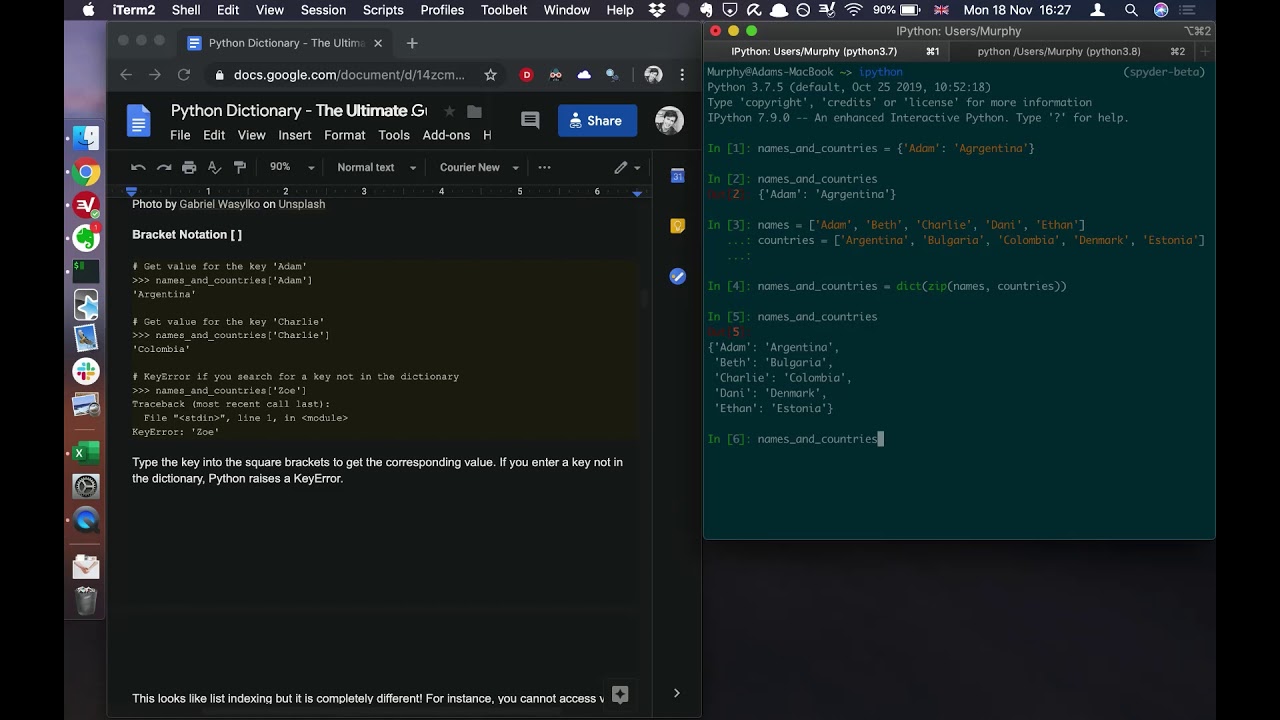
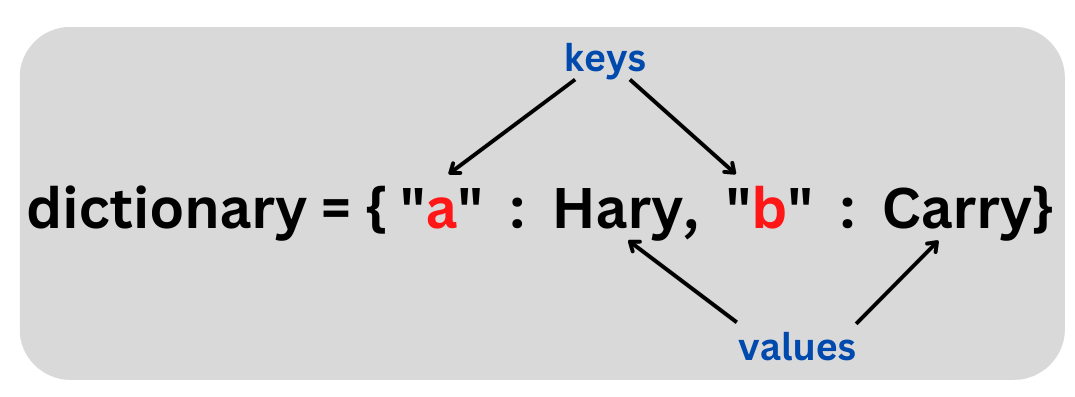
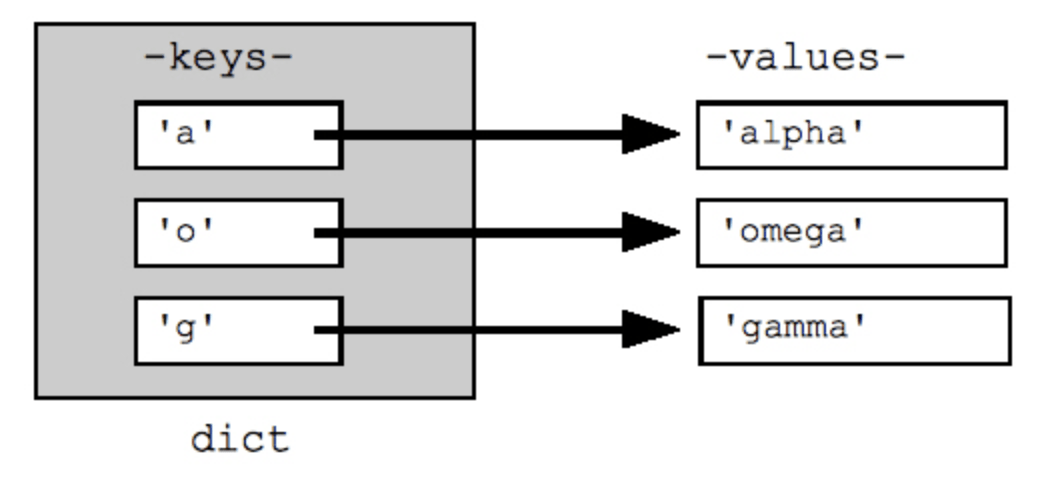
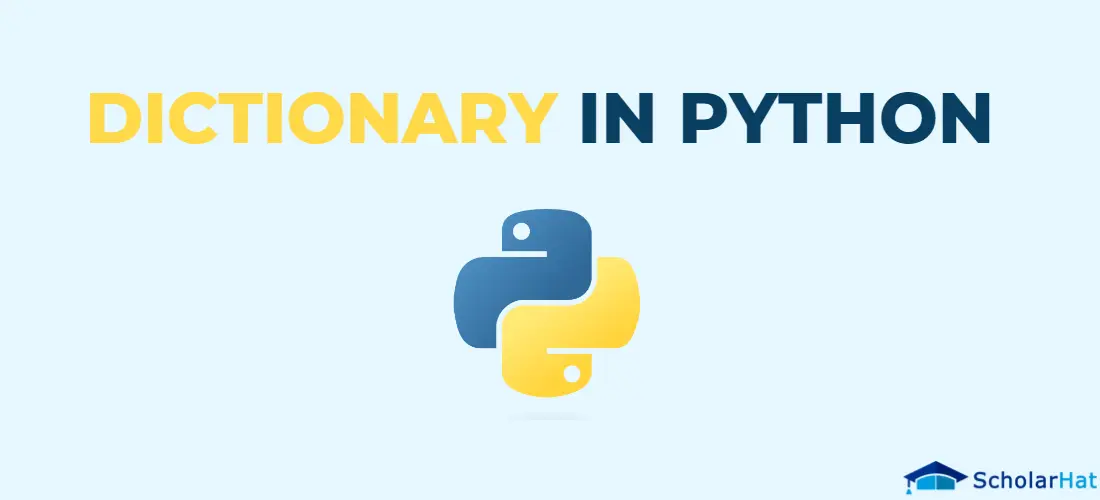




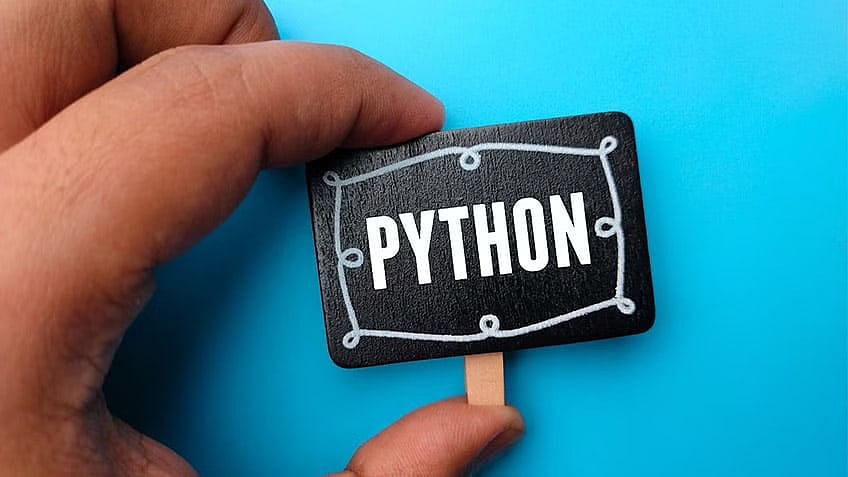
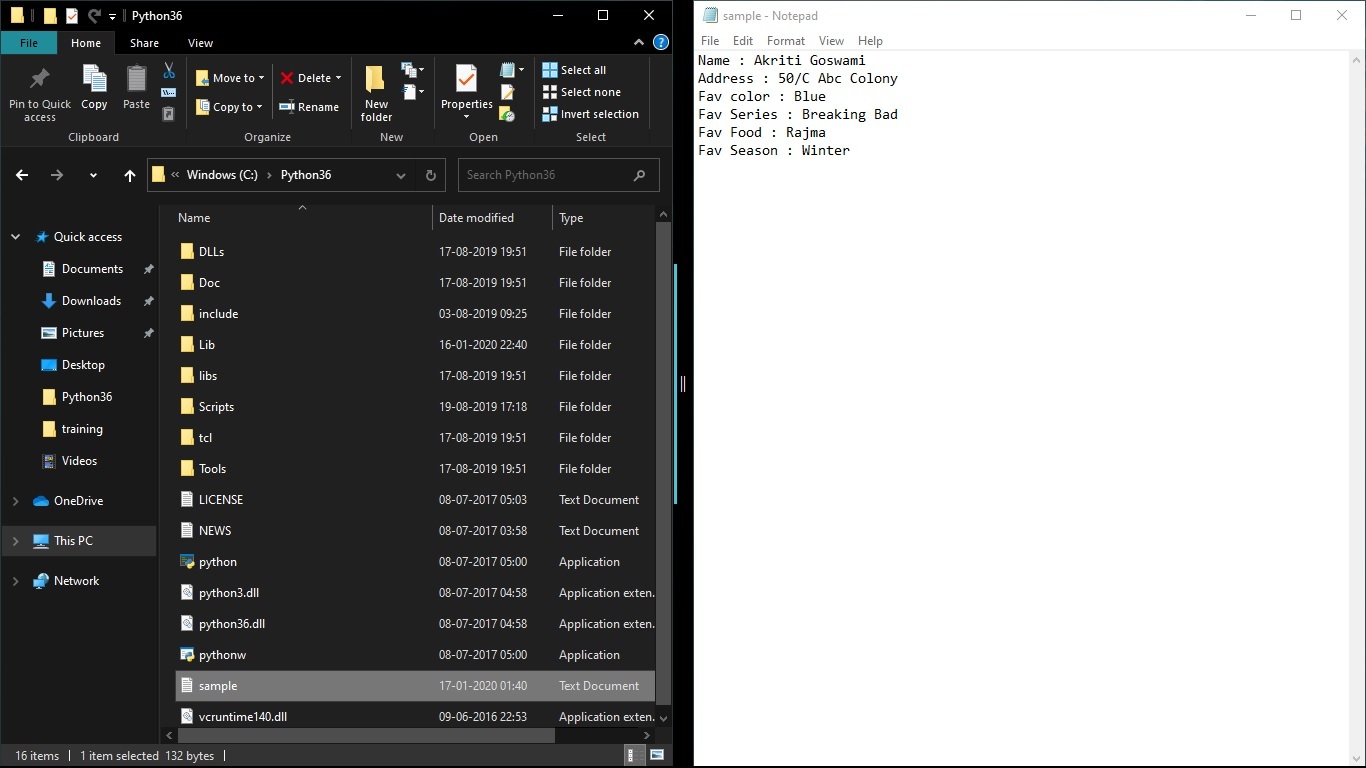
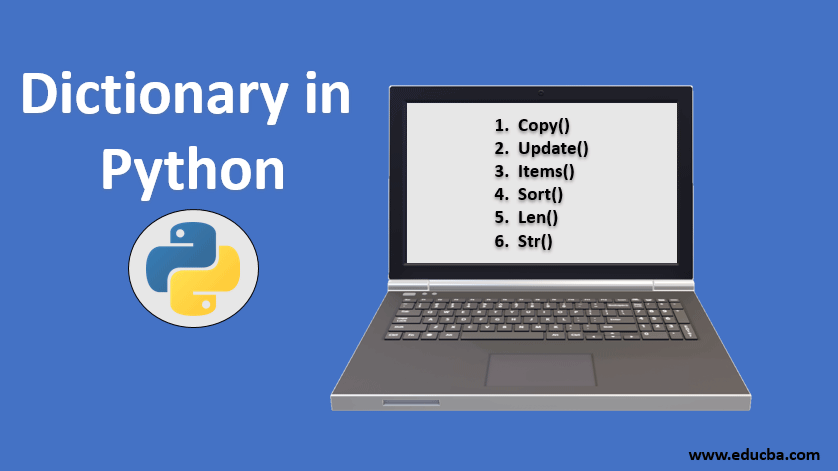

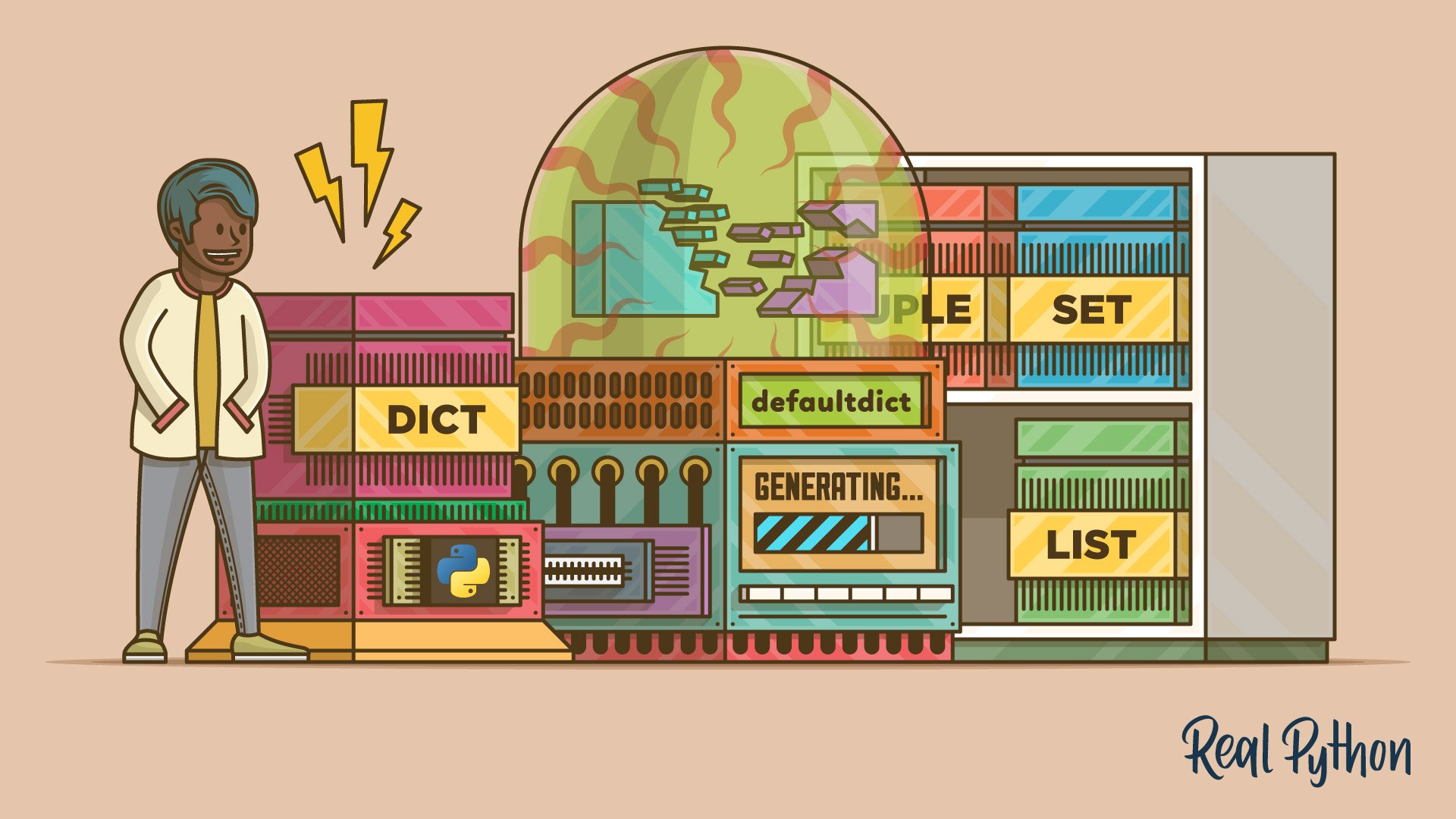
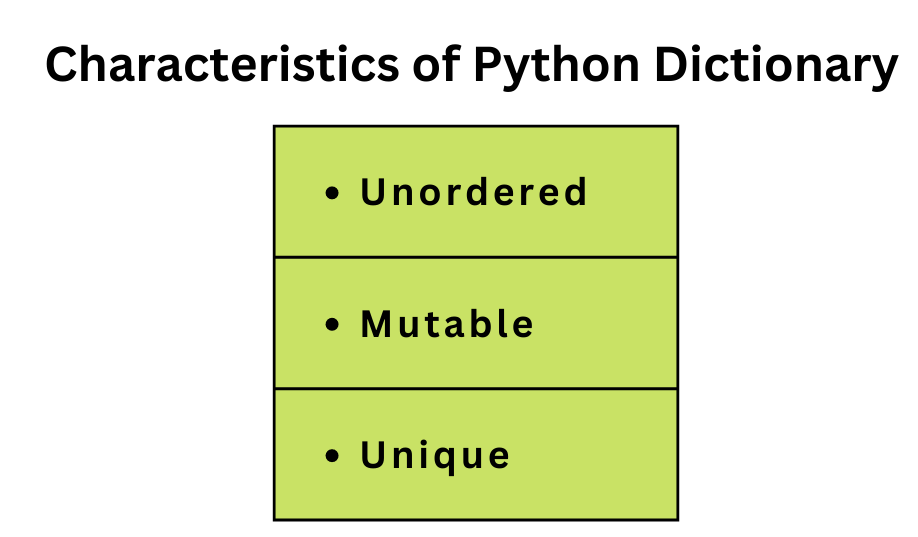
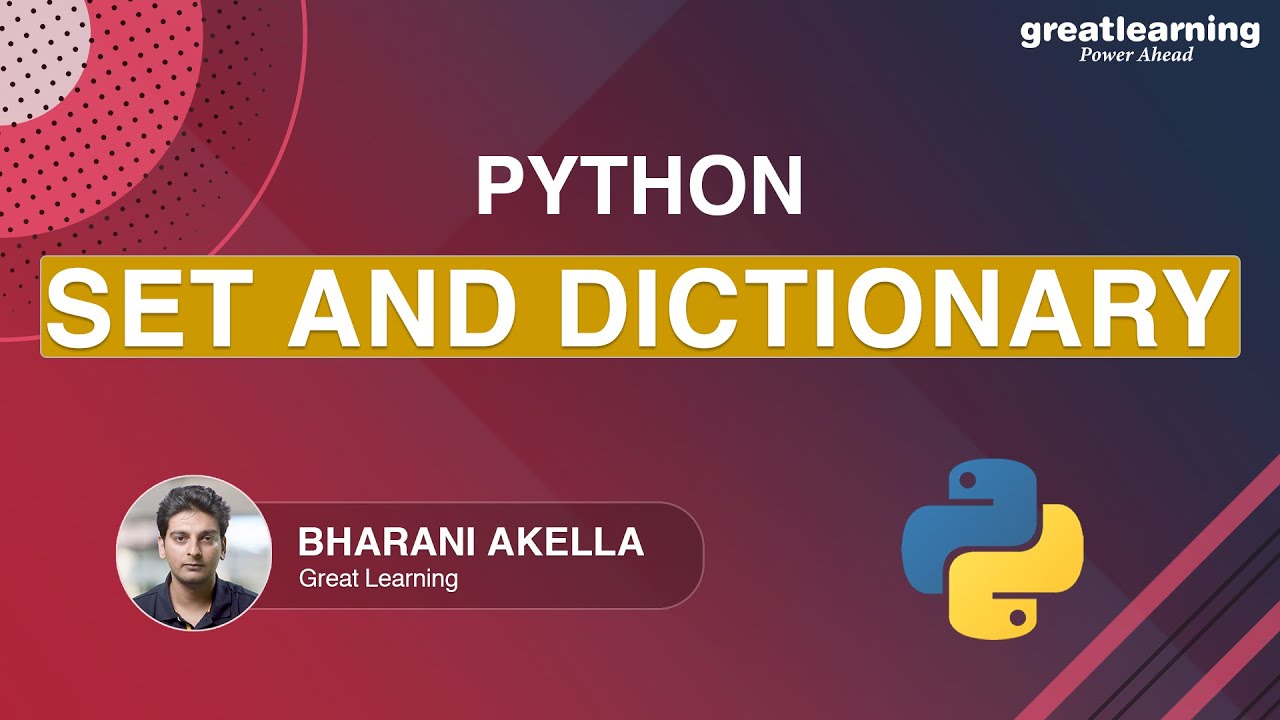
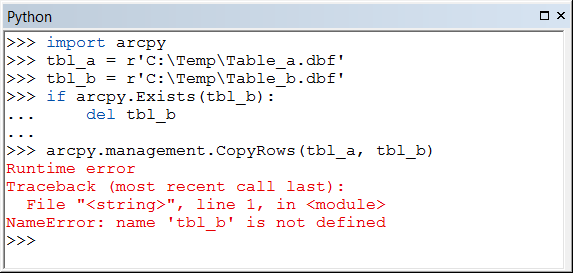
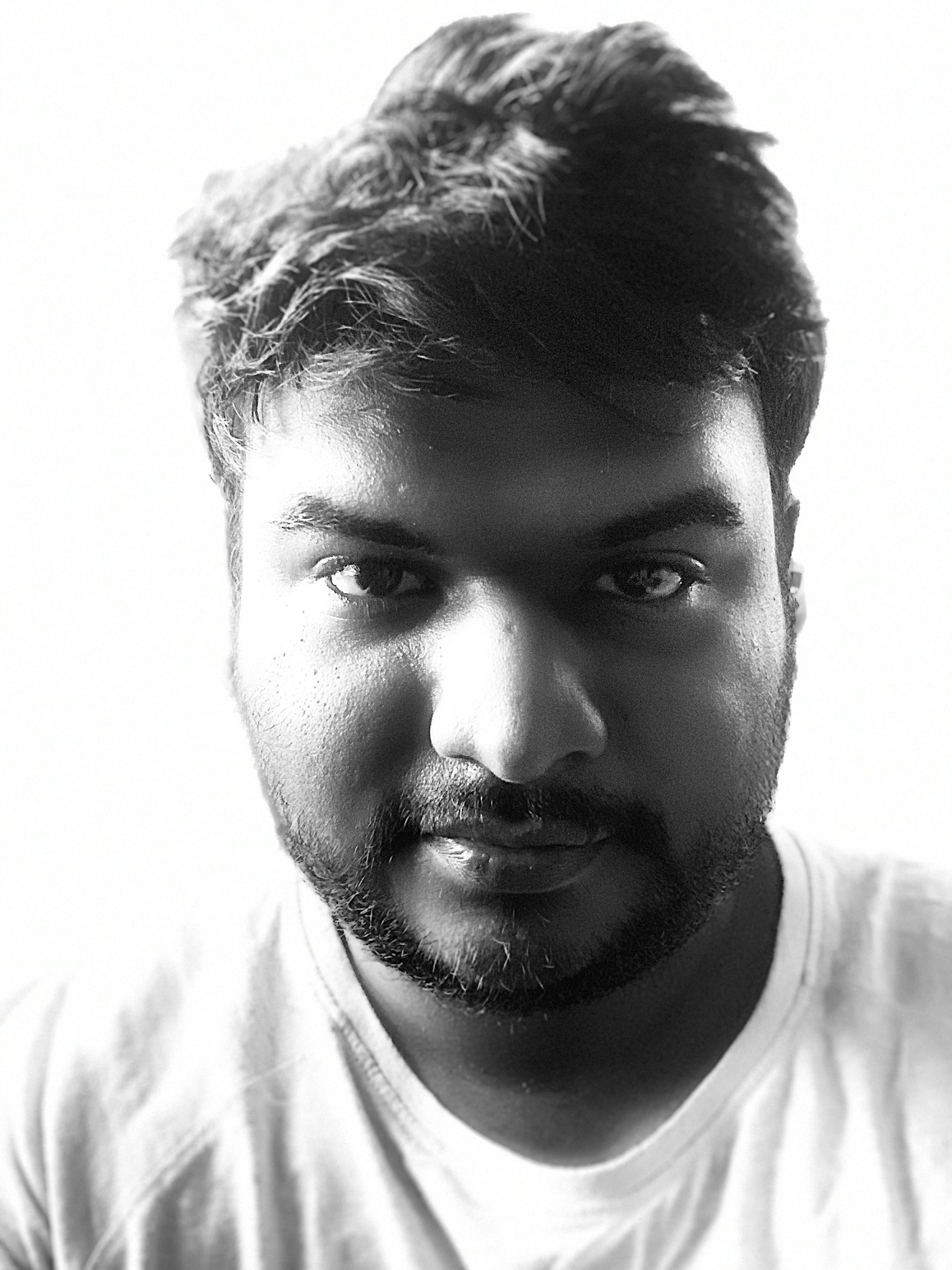
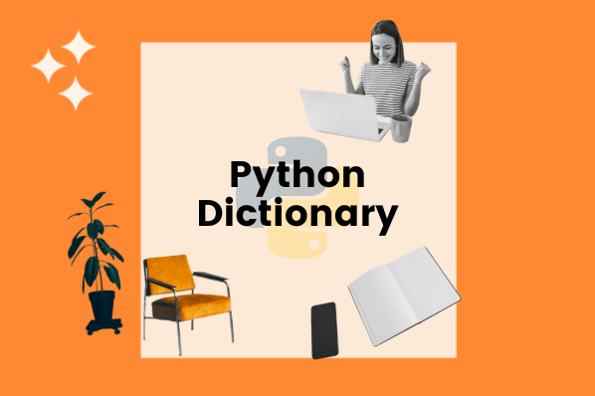

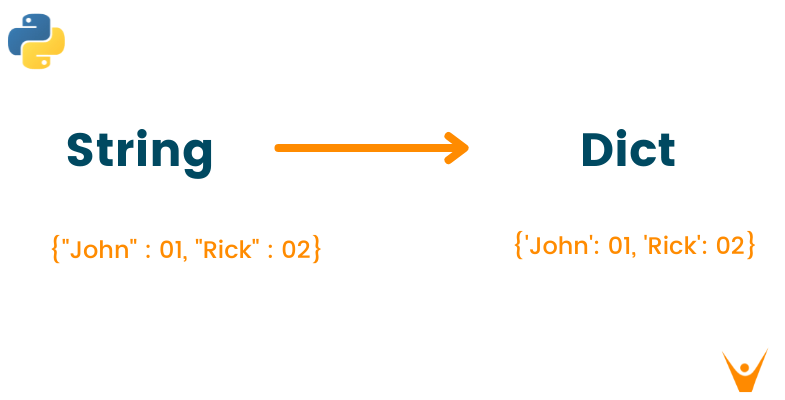
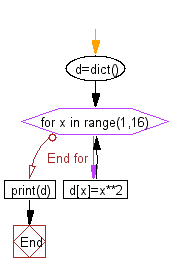

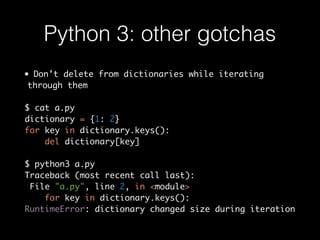
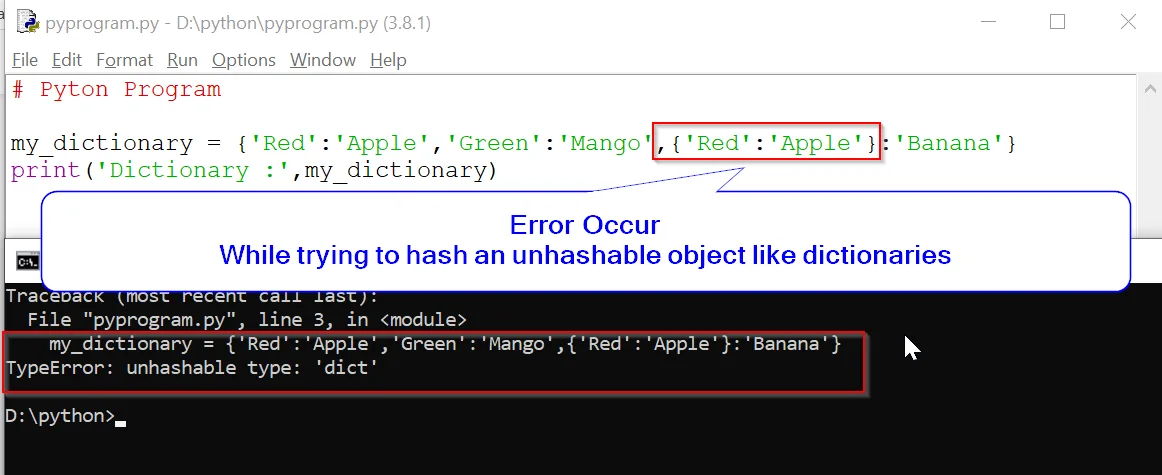
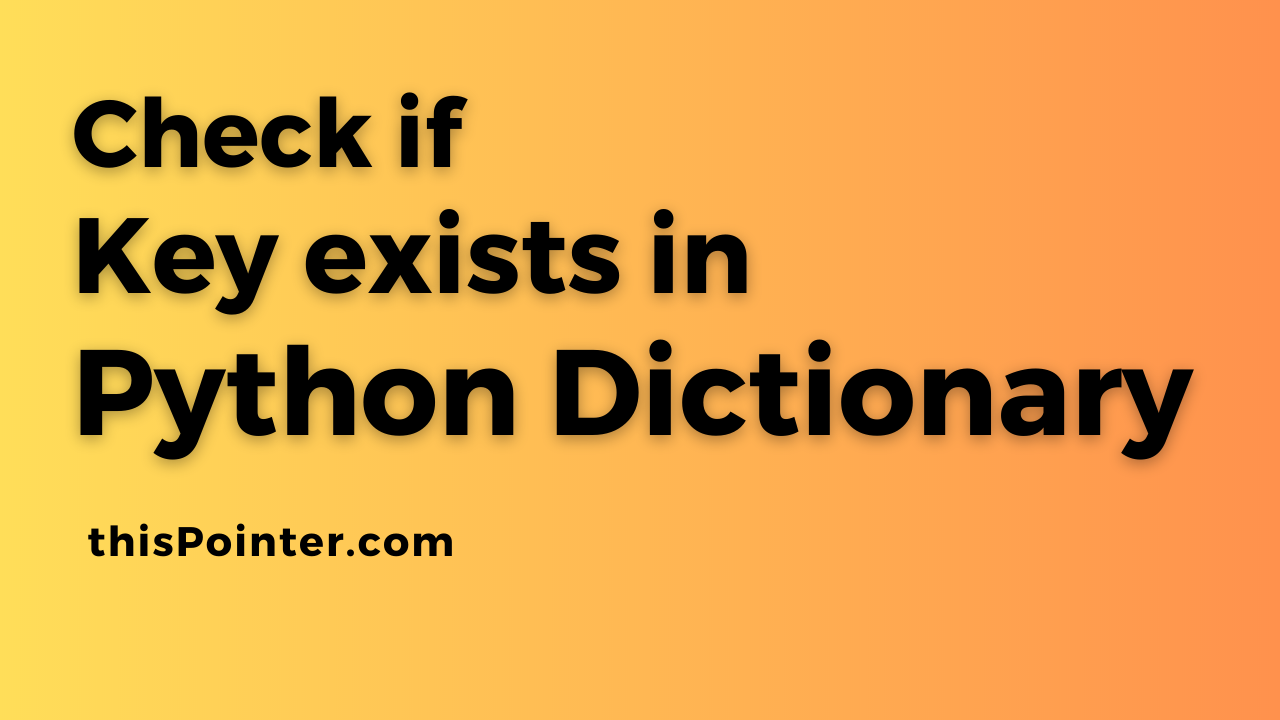
Article link: python delete dict key.
Learn more about the topic python delete dict key.
- remove key from dictionary python | Scaler Topics
- Python Remove Key from Dictionary – How to Delete Keys …
- Python Remove Key from a Dictionary: A Complete Guide
- How can I remove a key from a Python dictionary?
- Python | Ways to remove a key from dictionary – GeeksforGeeks
- Python: Remove Key from Dictionary (4 Different Ways) – Datagy
- How to remove items from a dictionary in Python – Educative.io
- Remove an item from a dictionary in Python (clear, pop …
- Remove key from python dictionary | Board Infinity
- How to Remove a Key from Python Dictionary
See more: nhanvietluanvan.com/luat-hoc