Python Dictionary Is Empty
Python dictionaries are a powerful data structure that allows you to store and retrieve key-value pairs. However, there may be times when you need to check if a dictionary is empty, i.e., it has no key-value pairs. In this article, we will explore different methods to accomplish this task.
Checking using the len() function:
The len() function is a built-in Python function that returns the number of elements in a given object. In the case of a dictionary, it returns the count of key-value pairs present in it. We can utilize this function to check if a dictionary is empty.
To implement the len() function and check if the dictionary is empty, use the following code:
“`python
my_dict = {}
if len(my_dict) == 0:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the above example, we assign an empty dictionary `my_dict = {}`. We then use the len() function to get the count of items in the dictionary. If the length is 0, it indicates that the dictionary is empty, and the respective message is printed.
Using the not operator:
The not operator is a logical operator in Python that returns the opposite boolean value. By using the not operator with the dictionary, it will return True if the dictionary is empty, and False otherwise.
To utilize the not operator and check if a dictionary is empty, you can use the following code:
“`python
my_dict = {}
if not my_dict:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the above code, we apply the not operator to the dictionary `my_dict`. Since it is empty, the condition becomes True, and the corresponding message is printed.
Using the bool() function:
The bool() function is a built-in Python function that returns the boolean value of an object. In the case of an empty dictionary, the bool() function will return False. We can utilize this function to determine if a dictionary is empty.
To apply the bool() function and check if a dictionary is empty, use the following code:
“`python
my_dict = {}
if bool(my_dict) == False:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the above example, we pass the dictionary `my_dict` to the bool() function. If the dictionary is empty, the bool() function will return False, and the respective message will be printed.
Using the keys() method:
The keys() method is a built-in Python method that returns a list of all the keys present in a dictionary. By utilizing this method, we can check if the dictionary is empty by checking the length of the list.
To utilize the keys() method and check if a dictionary is empty, use the following code:
“`python
my_dict = {}
if len(my_dict.keys()) == 0:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the code above, we apply the keys() method on the dictionary `my_dict` and retrieve a list of all the keys. By checking the length of this list, we can determine whether the dictionary is empty or not.
Using the values() method:
The values() method is a built-in Python method that returns a list of all the values present in a dictionary. By utilizing this method, we can check if the dictionary is empty by checking the length of the list.
To implement the values() method and check if a dictionary is empty, use the following code:
“`python
my_dict = {}
if len(my_dict.values()) == 0:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the above code, we utilize the values() method to retrieve a list of all the values from the dictionary `my_dict`. By checking the length of this list, we can determine if the dictionary is empty or not.
Using the items() method:
The items() method is a built-in Python method that returns a list of all the key-value pairs present in a dictionary. By utilizing this method, we can check if the dictionary is empty by checking the length of the list.
To utilize the items() method and determine if a dictionary is empty, use the following code:
“`python
my_dict = {}
if len(my_dict.items()) == 0:
print(“Dictionary is empty.”)
else:
print(“Dictionary is not empty.”)
“`
In the above code, we apply the items() method on the dictionary `my_dict` to get a list of all the key-value pairs. By checking the length of this list, we can determine if the dictionary is empty or not.
FAQs:
Q: How do I create an empty dictionary with keys?
A: To create an empty dictionary with specific keys, you can use the following code:
“`python
keys = [‘key1’, ‘key2’, ‘key3’]
empty_dict = {key: None for key in keys}
“`
Q: How can I remove an element from a dictionary?
A: To remove an element from a dictionary, you can use the del keyword followed by the key of the element you want to remove. Here is an example:
“`python
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
del my_dict[‘key1’]
“`
Q: How can I check if a specific key exists in a dictionary?
A: You can use the `in` operator to check if a specific key exists in a dictionary. Here is an example:
“`python
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
if ‘key1’ in my_dict:
print(“Key exists.”)
else:
print(“Key does not exist.”)
“`
Q: How can I get the value associated with a specific key in a dictionary?
A: You can use the `get()` method to retrieve the value associated with a specific key in a dictionary. If the key does not exist, you can provide a default value to return. Here is an example:
“`python
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
value = my_dict.get(‘key1’, ‘default’)
“`
Q: How can I create an empty dictionary in Python?
A: To create an empty dictionary, simply use the following code:
“`python
my_dict = {}
“`
In conclusion, checking if a Python dictionary is empty can be accomplished using various methods such as the len() function, the not operator, the bool() function, and the keys(), values(), and items() methods. Choose the method that fits your needs and your code’s readability.
How To Create An Empty Dictionary In Python
Keywords searched by users: python dictionary is empty Check dict empty Python, Create empty dict with keys, Empty dictionary Python, How to check a key in dictionary Python, Remove element in dict Python, Python dict get non existing key, Create empty dict Python, Check value in dictionary Python
Categories: Top 24 Python Dictionary Is Empty
See more here: nhanvietluanvan.com
Check Dict Empty Python
In Python, a dictionary (dict) is a mutable collection of key-value pairs. It is widely used to store and manipulate data efficiently. Often, there is a need to check whether a dictionary is empty or not, as this condition affects the subsequent operations or logic flow in a program. In this article, we will explore various ways to check if a dictionary is empty in Python.
Method 1: Using the len() function
The simplest and most straightforward approach to check if a dictionary is empty is by using the len() function. The len() function returns the number of elements in an object, and in the case of a dictionary, it returns the number of key-value pairs present.
Here’s an example showing how to use the len() function to check if a dictionary is empty:
“`python
my_dict = {}
if len(my_dict) == 0:
print(“The dictionary is empty”)
else:
print(“The dictionary is not empty”)
“`
In this example, an empty dictionary `my_dict` is created. The len() function is used to get the length of this dictionary, and if the length is 0, it means the dictionary is empty. Otherwise, it is considered non-empty.
Method 2: Using the bool() function
Another way to check if a dictionary is empty is by using the bool() function. The bool() function converts a value to its corresponding boolean value – True or False. For dictionaries, an empty dictionary evaluates to False, whereas a non-empty dictionary evaluates to True.
Here’s an example demonstrating the usage of bool() to check the emptiness of a dictionary:
“`python
my_dict = {}
if bool(my_dict):
print(“The dictionary is not empty”)
else:
print(“The dictionary is empty”)
“`
In this example, the bool() function is applied to the dictionary `my_dict`. If the result is True, it means that the dictionary is not empty, and if it is False, it indicates that the dictionary is empty.
Method 3: Using the keys() method
Python dictionaries have a built-in keys() method that returns a view object of all the keys in the dictionary. By checking the truthiness of this view object, we can determine if the dictionary is empty or not.
Have a look at the following example:
“`python
my_dict = {}
if my_dict.keys():
print(“The dictionary is not empty”)
else:
print(“The dictionary is empty”)
“`
In this example, the keys() method is called on the dictionary `my_dict`. If the keys view object is not empty, it implies that the dictionary is not empty. Conversely, if the keys view object is empty, it suggests that the dictionary is empty.
Method 4: Directly checking with if statement
Python allows us to directly check the emptiness of a dictionary using the if statement. As empty dictionaries are considered False in a boolean context, we can write an if statement that evaluates the dictionary directly.
Check out the following example:
“`python
my_dict = {}
if not my_dict:
print(“The dictionary is empty”)
else:
print(“The dictionary is not empty”)
“`
In this example, the if statement checks if the dictionary `my_dict` is empty using the `not` keyword. If the dictionary is empty, it proceeds to execute the block of code inside the if statement. Otherwise, it executes the code inside the else block, indicating that the dictionary is not empty.
Frequently Asked Questions (FAQs):
Q1. Can we use any of these methods on non-empty dictionaries as well?
A1. Absolutely! These methods are applicable to both empty and non-empty dictionaries. However, the condition inside the if statement will differ depending on whether the dictionary is empty or not.
Q2. Are there any performance differences among these methods?
A2. In terms of performance, the len() function tends to be slightly faster than the bool() function. However, the difference is negligible unless you are working with large datasets. Therefore, choose the method that suits your coding style and requirements.
Q3. How can I check if a nested dictionary is empty?
A3. To check if a nested dictionary is empty, you can use any of these methods on the nested dictionary itself. The emptiness of the outer dictionary will be determined by the emptiness of the nested dictionary.
Q4. Can I check the emptiness of a dictionary while iterating over it?
A4. Yes, it is possible to check the emptiness of a dictionary while iterating over it. However, it is important to note that the dictionary may change during the iteration. Therefore, it is generally advised to store the length of the dictionary in a variable before iterating over it to avoid unexpected behavior.
Q5. Are there any other built-in methods or functions to check the emptiness of a dictionary?
A5. Apart from the methods mentioned in this article, you can also use the `==` operator to check if a dictionary is empty. For example: `if my_dict == {}:`, but it is less preferred due to readability concerns.
In conclusion, checking if a dictionary is empty in Python is a commonly encountered task. Fortunately, Python provides several convenient ways to accomplish this. You can rely on the len() function, bool() function, keys() method, or the if statement itself to determine if a dictionary is empty or not. Choose the method that best suits your coding style and requirement, and use it confidently in your Python programs.
Create Empty Dict With Keys
A dictionary in Python is an incredibly useful data structure that allows us to store and retrieve values using keys. When working with dictionaries, often we need to create an empty dictionary and specify the keys beforehand. In this article, we will explore the various ways to create an empty dictionary with keys in English and provide a comprehensive understanding of this topic.
## Table of Contents
1. Creating an empty dictionary
2. Adding keys to an empty dictionary
3. Initializing an empty dictionary with default values
4. Frequently Asked Questions (FAQs)
## 1. Creating an empty dictionary
There are multiple ways to create an empty dictionary in Python, all of which involve using curly brackets ({}):
### Method 1: Using curly brackets
“`
my_dict = {}
“`
This creates an empty dictionary called `my_dict`.
### Method 2: Using the `dict()` function
“`
my_dict = dict()
“`
Similarly, this also creates an empty dictionary called `my_dict`.
## 2. Adding keys to an empty dictionary
Once we have created an empty dictionary, we can start adding keys to it. Here are a few ways to do that:
### Method 1: Direct assignment
“`
my_dict[‘key1’] = value1
my_dict[‘key2’] = value2
“`
This method allows us to assign values to specific keys directly by using the assignment operator (=). Replace `key1`, `key2`, `value1`, and `value2` with the appropriate keys and their respective values.
### Method 2: Using the `update()` method
“`
my_dict.update({‘key1’: value1, ‘key2’: value2})
“`
The `update()` method allows us to add multiple keys and their values to the dictionary at once. Replace `key1`, `key2`, `value1`, and `value2` with the corresponding keys and values.
### Method 3: Dictionary comprehension
“`
my_dict = {key: value for key, value in zip(keys_list, values_list)}
“`
Using dictionary comprehension, we can create a dictionary by mapping keys from a list (`keys_list`) to the corresponding values from another list (`values_list`). You can modify the lists with your desired keys and values.
## 3. Initializing an empty dictionary with default values
In some cases, we may need to initialize an empty dictionary with default values. Here’s how to achieve that:
### Method 1: Using the `fromkeys()` method
“`
my_dict = dict.fromkeys(keys_list, default_value)
“`
This method initializes the dictionary with the given `keys_list` as keys, and assigns all the keys a default value (`default_value`). You can replace `keys_list` and `default_value` with your desired values.
### Method 2: Dictionary comprehension with default values
“`
my_dict = {key: default_value for key in keys_list}
“`
Similar to the previous method, this also initializes the dictionary with the desired keys and assigns them a default value.
## Frequently Asked Questions (FAQs)
Q1. Can I add keys to a dictionary after creating it?
Yes, you can add keys to a dictionary after creating it. You can use any of the methods mentioned in Section 2 to add keys to an existing dictionary.
Q2. Can I create an empty dictionary with keys that have default values?
Yes, you can create an empty dictionary with default values using the methods described in Section 3.
Q3. How can I check if a key exists in a dictionary?
To check if a key exists in a dictionary, you can use the `in` keyword as follows:
“`python
if ‘key’ in my_dict:
# Key exists in the dictionary
else:
# Key does not exist in the dictionary
“`
Q4. Can I create an empty dictionary with predefined keys and values in one step?
Yes, you can use any of the methods mentioned in Section 2 to create an empty dictionary and assign keys and values at the same time.
In conclusion, dictionaries are versatile structures in Python that allow us to store and retrieve values using keys. Creating an empty dictionary with keys in English can be done using methods like direct assignment, `update()` method, and dictionary comprehension. Additionally, we can initialize an empty dictionary with default values. Understanding these techniques provides a solid foundation for working with dictionaries and enhances the efficiency of our code.
Images related to the topic python dictionary is empty
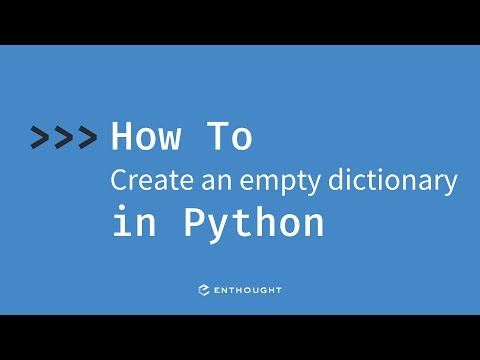
Found 28 images related to python dictionary is empty theme
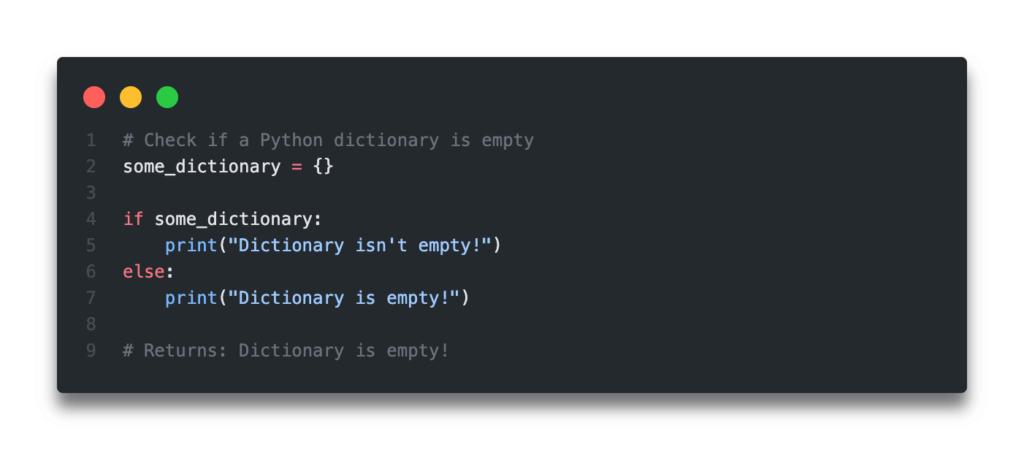
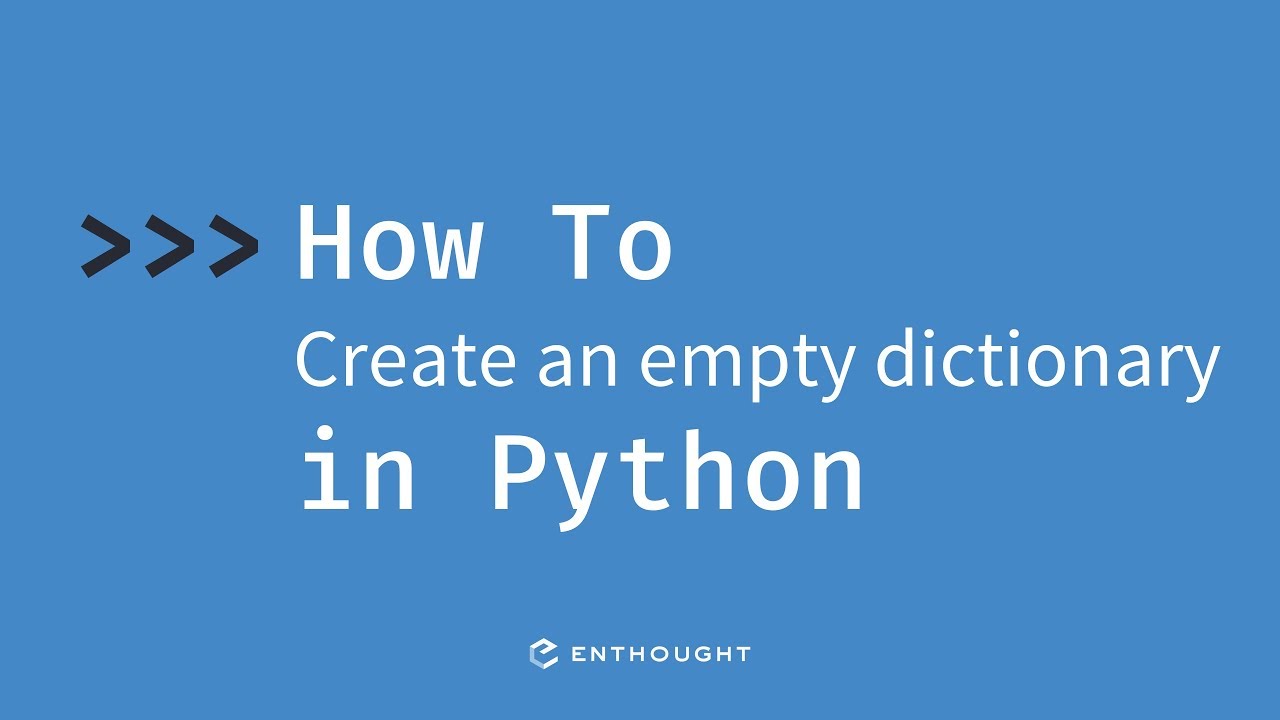
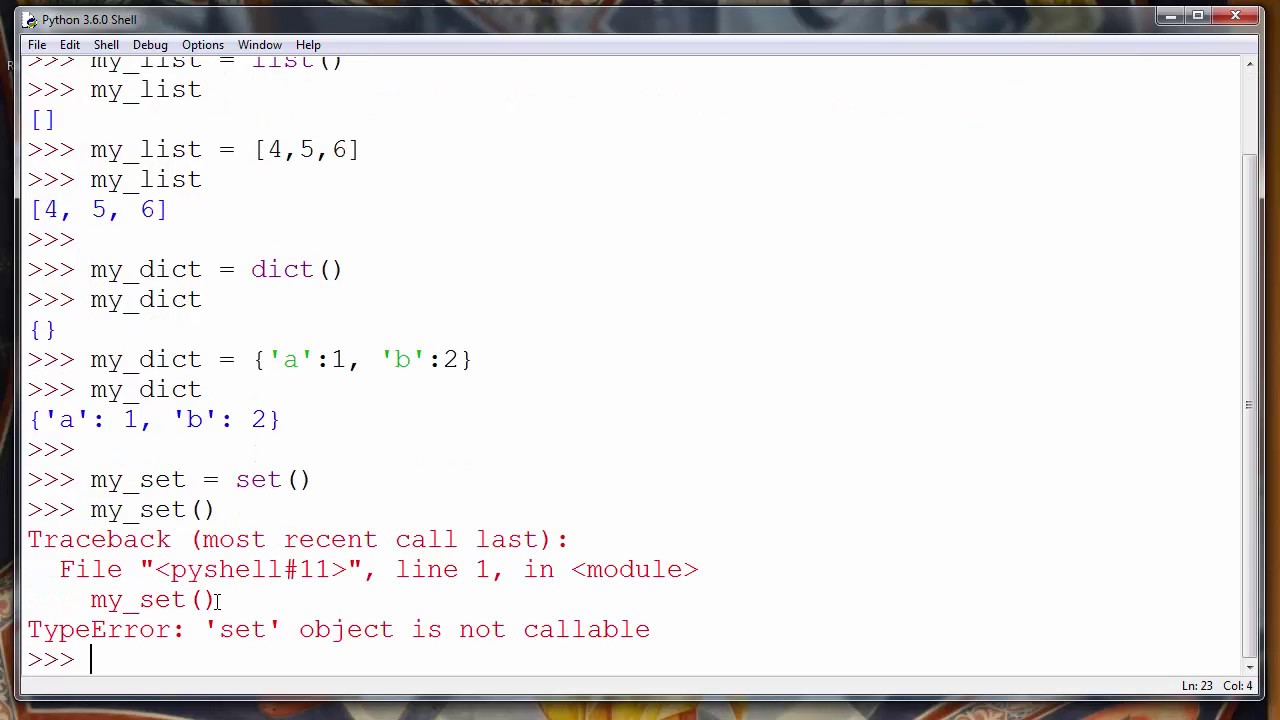
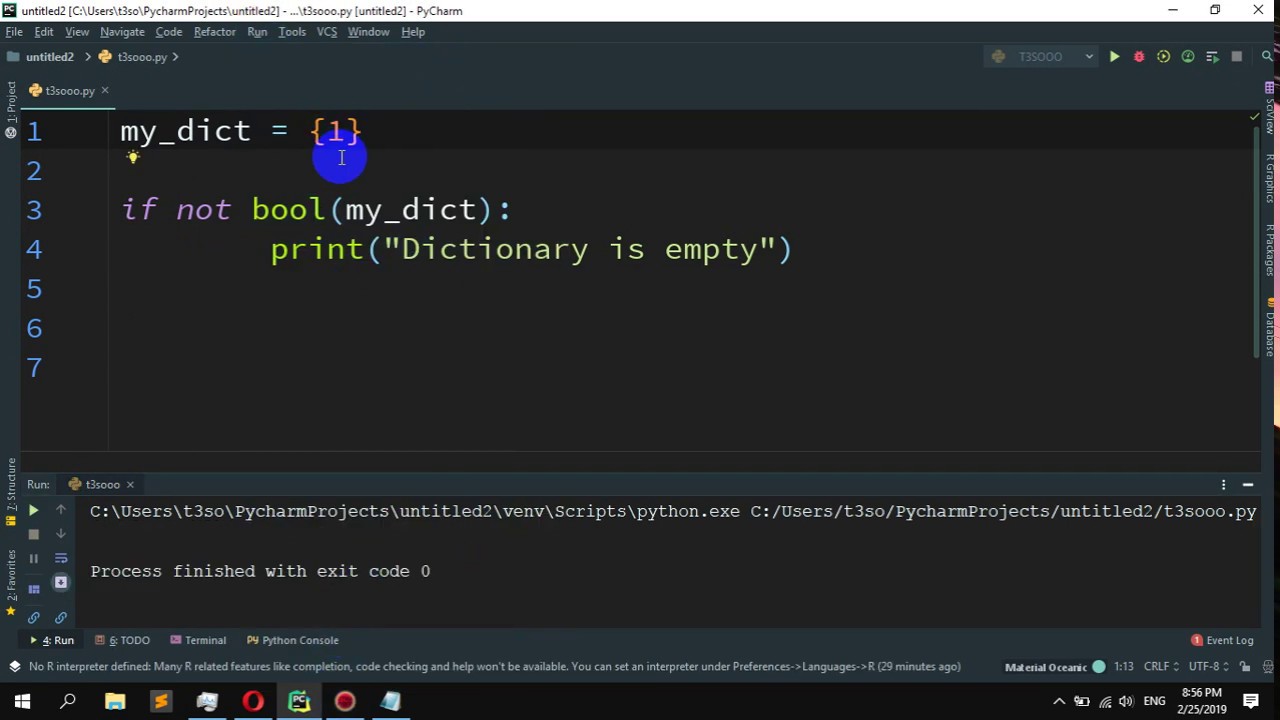

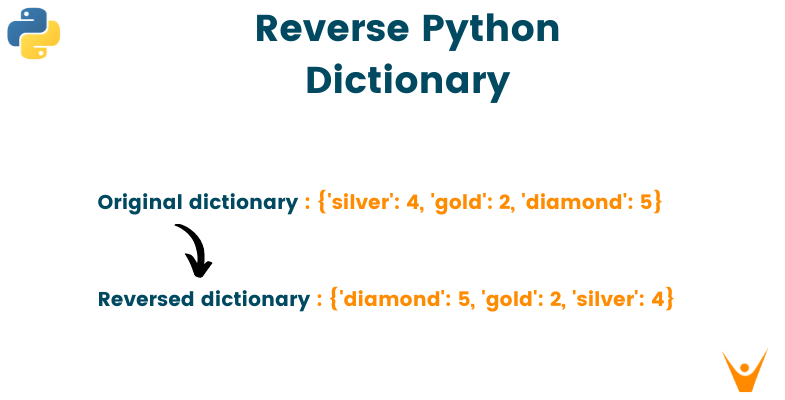


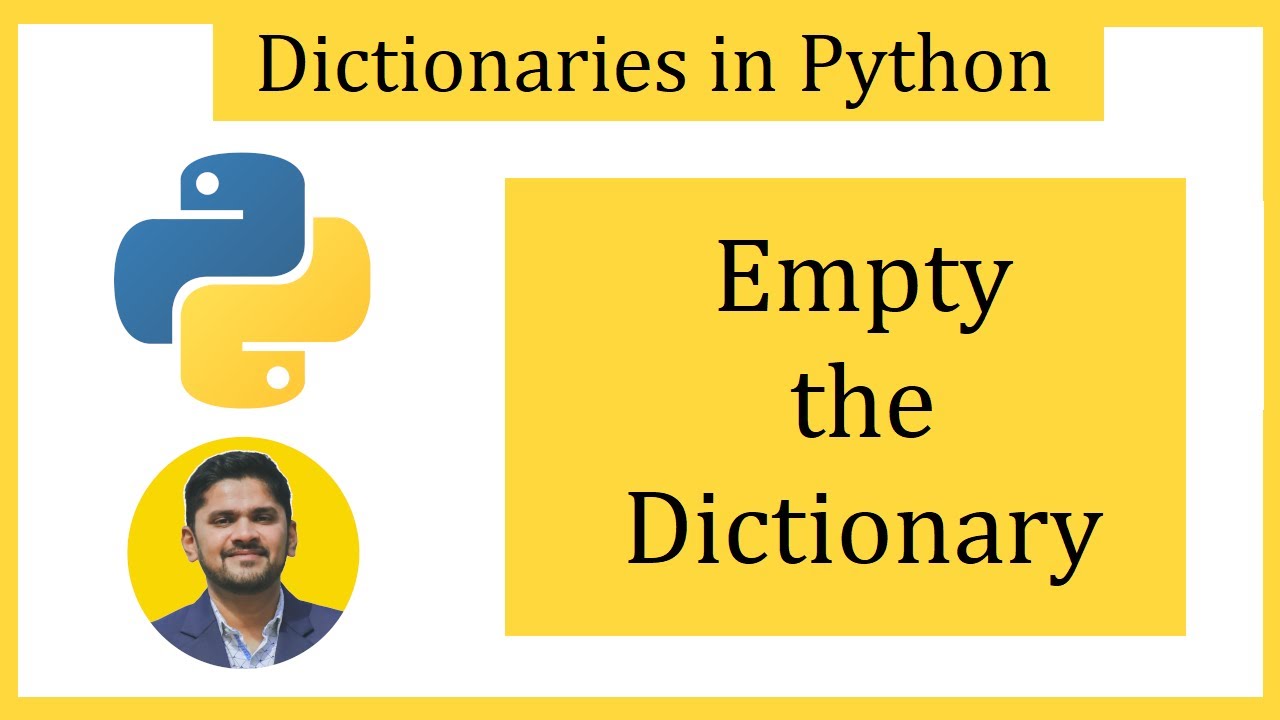

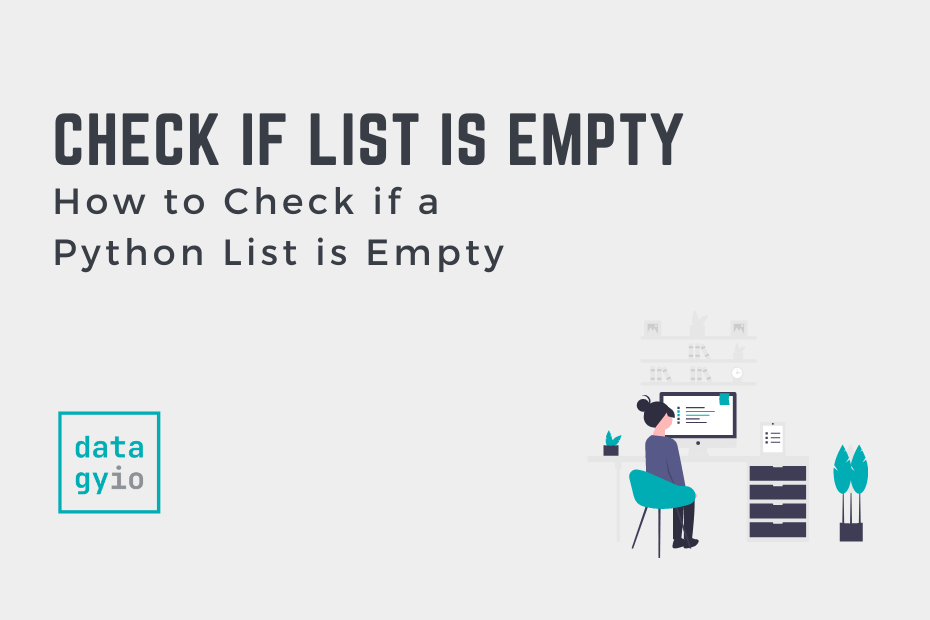
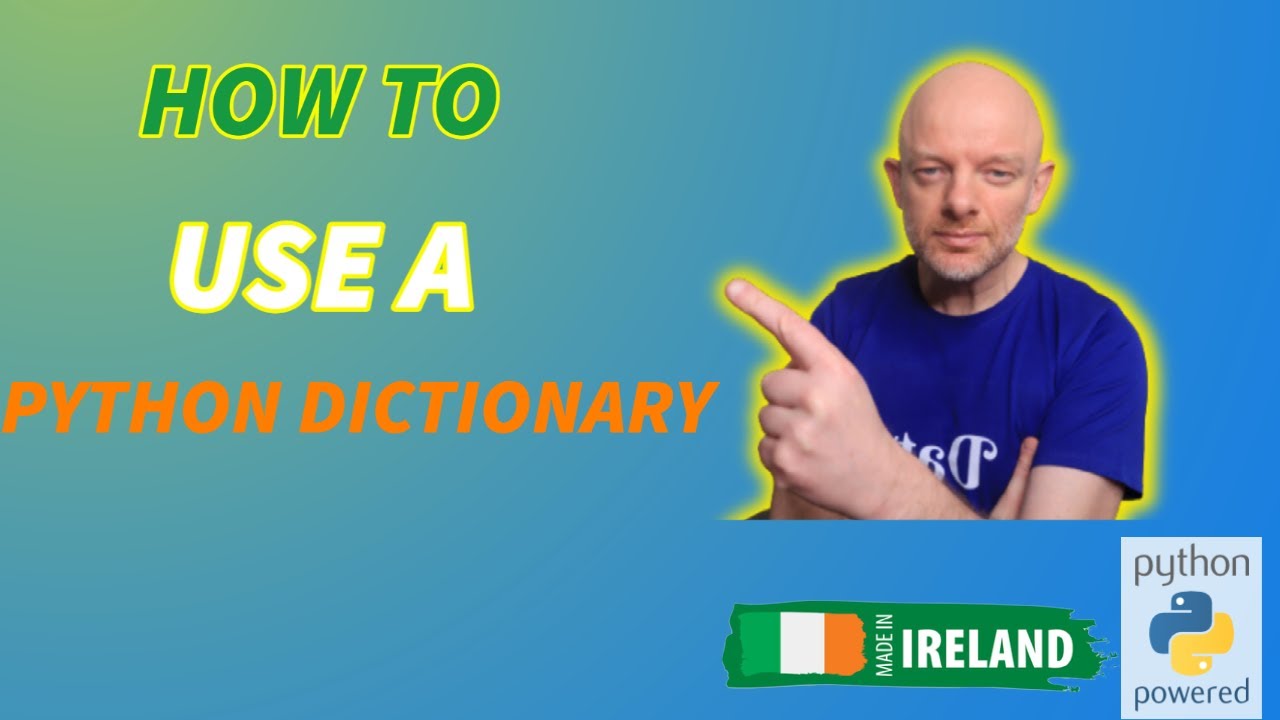



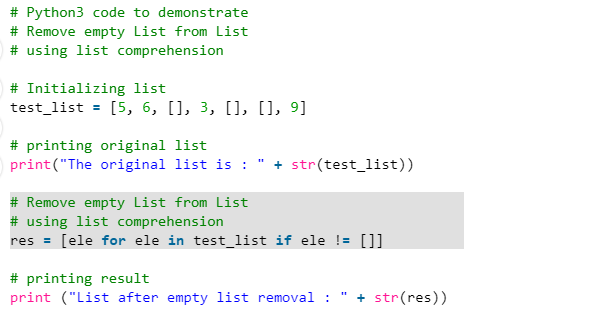

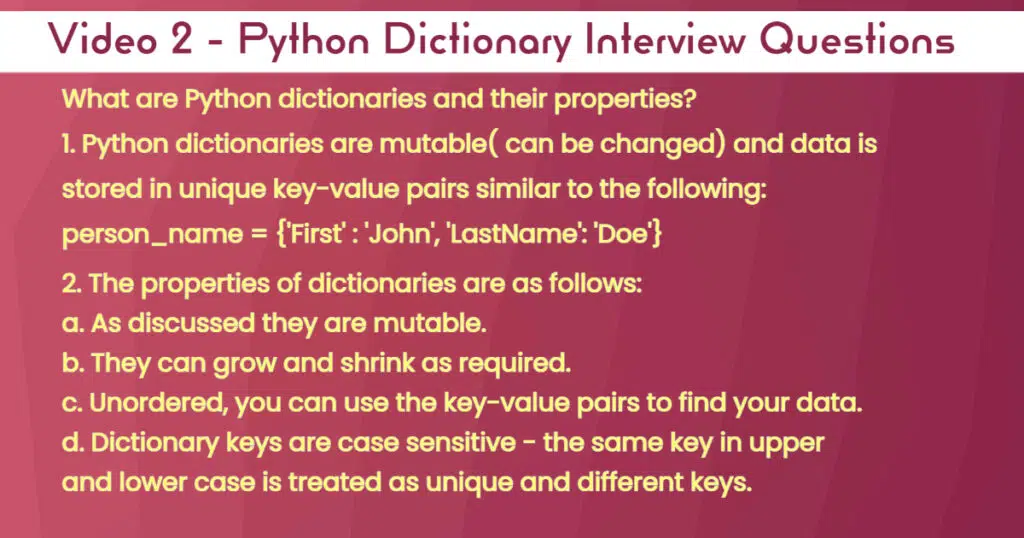
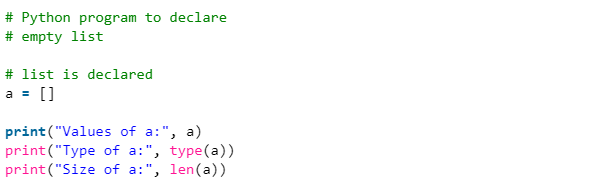
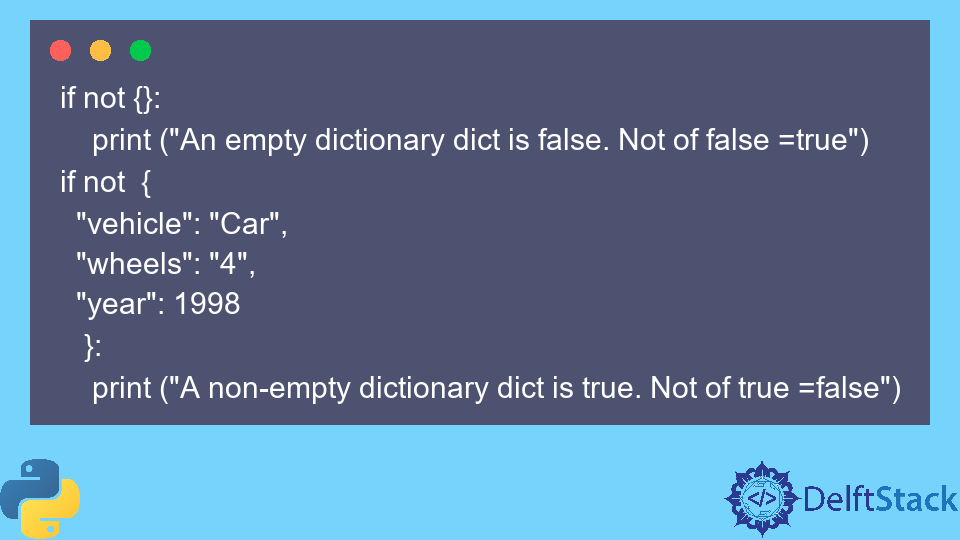
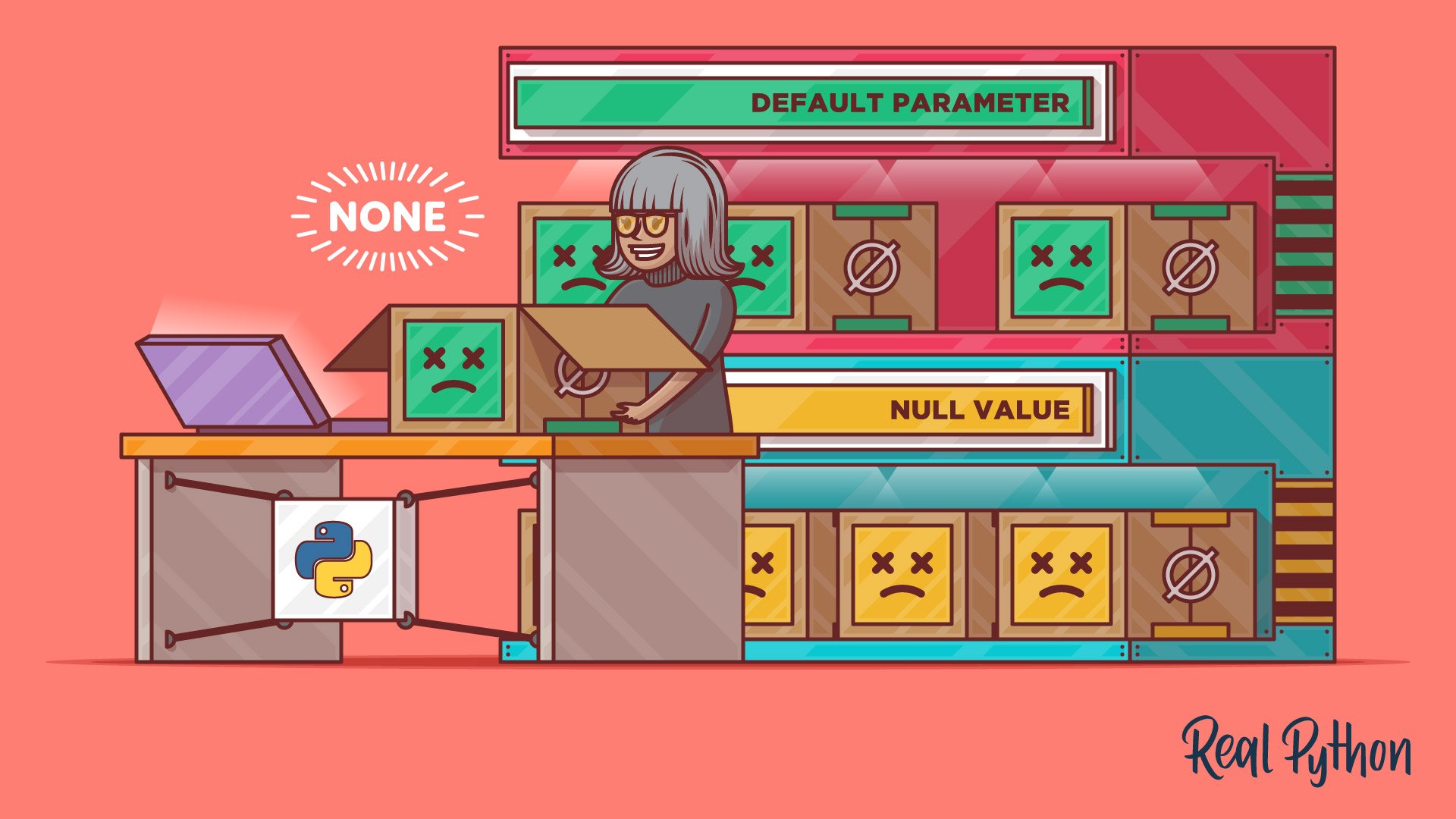
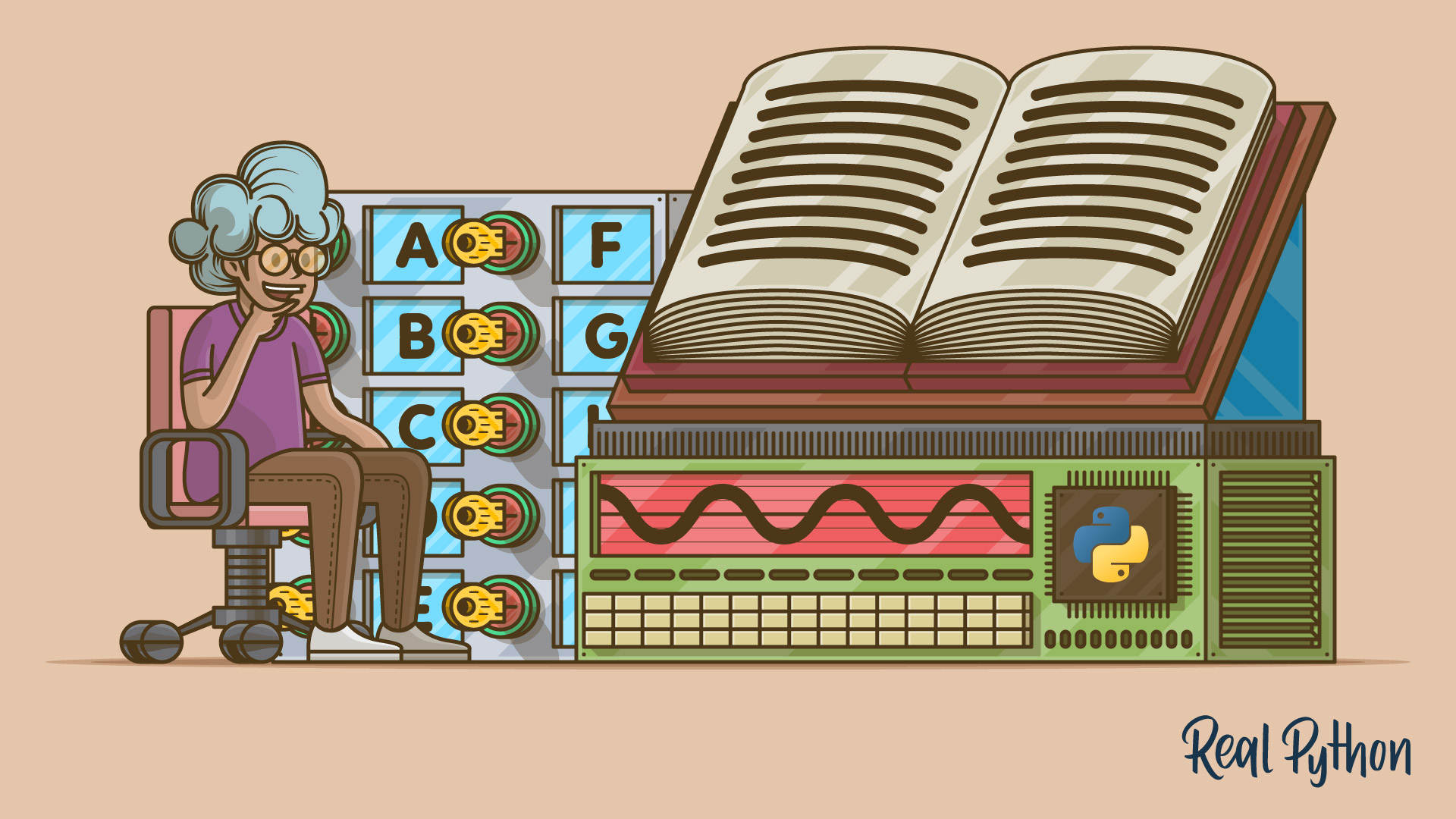
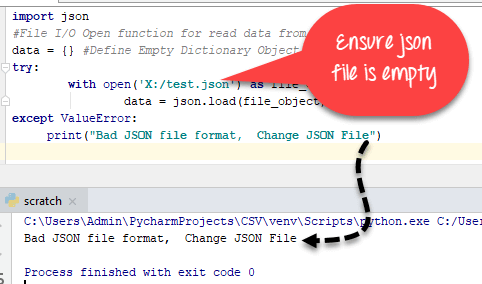
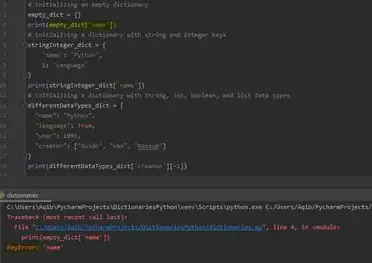
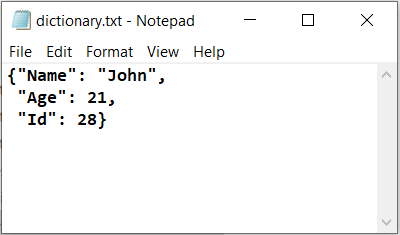




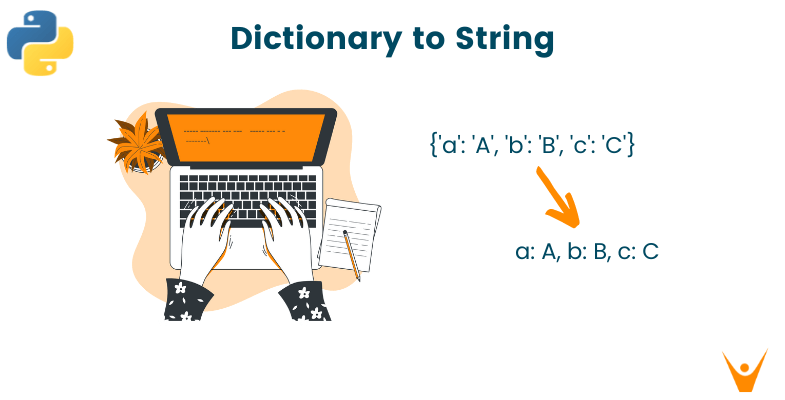
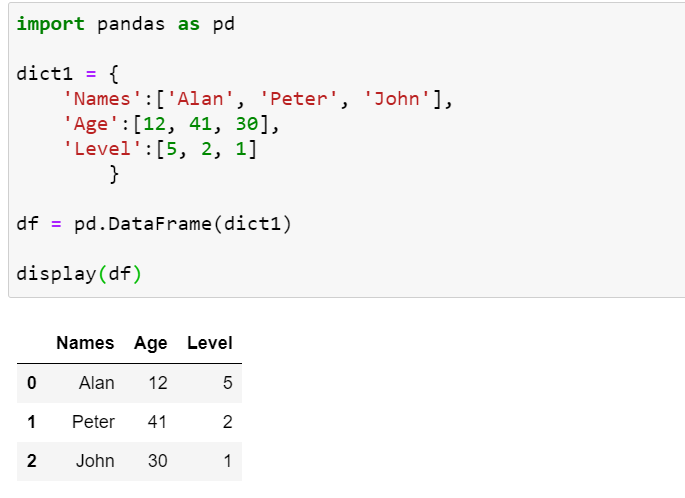

Article link: python dictionary is empty.
Learn more about the topic python dictionary is empty.
- Python: Check if a Dictionary is Empty (5 Ways!) – Datagy
- How to check if a dictionary is empty? – Stack Overflow
- How can I determine if a dictionary is empty? – Python FAQ
- Create Empty Dictionary in Python (5 Easy Ways) – FavTutor
- How to check if a dictionary is empty in python – Javatpoint
- Create a Dictionary in Python – Python Dict Methods
- How To Create An Empty Python Dictionary
- Python – Check if dictionary is empty – Tutorialspoint
See more: nhanvietluanvan.com/luat-hoc