Runtimeerror: Working Outside Of Application Context
In Flask, the application context is an essential concept that ensures proper execution and functionality of the application. The application context represents the environment in which the Flask application is running and provides access to resources such as the request and the database connection. It is essential to understand the application context to prevent errors like “RuntimeError: Working outside of application context.”
The Importance of Application Context
The application context is crucial because Flask is designed to be lightweight and thread-safe. Each request in Flask runs in a separate thread, and the application context ensures that the state of the application is maintained correctly during the request-response cycle. It allows Flask to manage resources efficiently and prevent conflicts between multiple requests.
Causes of Working outside of Application Context
1. Callback Functions and Flask Request Context
Callback functions are commonly used in Flask to handle events such as route handling or request processing. These functions are executed within the request context. However, when callback functions are invoked outside of the request context, it can lead to the “RuntimeError: Working outside of application context” error. This occurs because the necessary resources and context required for proper execution are not available.
Understanding the Flask Request Context
The Flask request context contains information about the current request being processed, such as the request headers, form data, and session. The request context is created and pushed onto the application context stack before processing a request, and it is removed once the request is completed. Accessing Flask-specific features, such as the request object, outside of the request context will result in errors.
Potential issues when using callback functions outside of application context
When callback functions are used outside of the Flask application context, critical resources become inaccessible. For example, if a callback function tries to access the request object, which is only available within the request context, an error will occur. This can lead to data corruption, incorrect behavior, or even application crashes.
2. Threaded Execution and Global Variables
In Flask, threaded execution allows multiple requests to be processed simultaneously. However, using global variables in a multi-threaded environment can lead to issues with the application context. Global variables are shared across threads and can result in conflicts or a loss of application context.
Exploring threaded execution in Flask
Threaded execution in Flask enables the application to handle multiple requests concurrently. By default, Flask uses a single-threaded development server. However, for production environments, a multi-threaded server or a WSGI server like Gunicorn or uWSGI is recommended.
How global variables can lead to working outside of application context
When global variables are used in Flask, their values become shared across threads, making it difficult to maintain proper application context. Accessing or modifying global variables outside of their intended context can result in unexpected behavior or errors. It is crucial to use Flask’s built-in mechanisms, such as the request context and session objects, instead of relying on global variables.
Strategies for handling threaded execution properly
To ensure proper handling of threaded execution in Flask, it is recommended to use thread-local variables. Thread-local variables are unique to each thread, allowing the application to maintain separate contexts for each request. Flask provides the `Local` object to handle thread-local variables. By leveraging this mechanism, each request can have its own context, preventing conflicts and errors with shared data.
3. Asynchronous Tasks and Flask Context Preservation
Asynchronous programming is becoming increasingly popular in web development to improve performance and scalability. However, when dealing with asynchronous tasks in Flask, preserving the application context can be challenging. If the application context is not properly maintained, errors such as “RuntimeError: Working outside of application context” may occur.
Overview of asynchronous tasks in Flask
Flask provides several mechanisms for handling asynchronous tasks, such as using the `async` and `await` keywords, employing third-party libraries like Celery, or utilizing Flask extensions like Flask-Mail or Flask-SocketIO. These techniques allow developers to perform time-consuming operations without blocking the main thread.
Challenges of maintaining application context in asynchronous tasks
When working with asynchronous tasks, the application context may be suspended or lost during the execution. If the application context is not properly preserved, accessing resources such as the database, request context, or session can result in errors or unexpected behavior.
Techniques for preserving Flask context in asynchronous tasks
To ensure the proper preservation of the Flask application context during asynchronous tasks, developers can employ techniques such as using the `app.app_context()` method, which creates a context manager that can be used to push the application context manually. Additionally, libraries like `flask.ctx` can help preserve the application context when working with asynchronous tasks.
4. Improper Use of Flask Extensions and Decorators
Flask’s extensibility allows developers to enhance the functionality of their applications. However, improper usage of Flask extensions and decorators can lead to errors, including “RuntimeError: Working outside of application context.”
Common mistakes related to Flask extensions and decorators
One common mistake is not initializing or configuring Flask extensions properly. Each extension may have specific requirements or initialization steps that need to be followed to work within the Flask application context. Failing to do so can result in errors.
How improper usage can result in working outside of application context
When Flask extensions or decorators are used incorrectly, they may access or modify resources that are only available within the application context. This can lead to unexpected behavior, data corruption, or crashes.
Best practices for utilizing Flask extensions and decorators correctly
To avoid errors related to Flask extensions and decorators, it is crucial to refer to the official documentation and follow the recommended practices. Each extension may have specific instructions for initialization and usage. By adhering to best practices, developers can ensure that the extensions and decorators work seamlessly within the Flask application context.
Approaches to Resolving Runtime Errors: Working outside of Application Context
1. Utilizing Flask’s Application Context Stack
Flask provides the application context stack, which allows developers to push and pop application contexts as needed. By using the `app.app_context()` method, developers can manually push the application context onto the stack when necessary and pop it once the task is completed. This ensures that the application context is available when working with resources like the database or request objects.
Understanding the concept of application context stack in Flask
The application context stack in Flask is a way to manage the application context efficiently. It is a stack data structure that stores multiple application contexts. Each new request creates a new context, and it is pushed onto the stack. When the request is completed, the context is popped from the stack, allowing the next request to use the application context.
How to push and pop application context when necessary
To push and pop the application context in Flask, developers can use the `with app.app_context():` statement. Any code within this block will have access to the application context. Once the block is exited, the application context is automatically popped from the stack.
Examples of correct usage of the application context stack
To illustrate the correct usage of the application context stack, consider the following example:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def index():
with app.app_context():
# Code that requires the application context
return ‘Hello, World!’
“`
By using the `with app.app_context():` block, the code within it can access resources and functionalities only available within the application context.
2. Implementing Proper Asynchronous Patterns
When working with asynchronous tasks in Flask, it is crucial to follow recommended patterns to preserve the application context. Techniques such as thread-local contexts and event loops can be used to ensure the correct handling of asynchronous tasks.
Recommended patterns for handling asynchronous tasks in Flask
In Flask, it is recommended to use libraries like `flask.ctx` or techniques like thread locals to store the application context. By doing so, asynchronous tasks can access the application context when needed, without triggering the “RuntimeError: Working outside of application context” error.
Techniques such as thread-local contexts and event loops
Thread-local contexts allow for separate storage of variables and application context for each thread. This ensures that each request maintains its own context and resources without interfering with other requests.
Event loops can be used to manage asynchronous tasks and callbacks. By utilizing libraries like `asyncio` or Flask-specific extensions, developers can handle multiple tasks concurrently while preserving the application context.
Examples showcasing appropriate handling of asynchronous patterns
Consider the following example to demonstrate proper handling of asynchronous tasks in Flask:
“`python
import asyncio
from flask import Flask, current_app
app = Flask(__name__)
@app.route(‘/’)
def index():
asyncio.ensure_future(do_async_task())
return ‘Hello, World!’
async def do_async_task():
with app.app_context():
# Perform asynchronous task with access to the application context
pass
“`
In this example, the `asyncio.ensure_future()` method schedules the `do_async_task()` function as an asynchronous task. Within that task, the application context is manually pushed, allowing access to resources like the database or request object.
3. Adhering to Flask’s Documentation and Best Practices
Flask provides comprehensive documentation that covers various aspects of application development, including handling the application context correctly. It is crucial to refer to this documentation and follow Flask’s recommended practices to avoid errors related to working outside of the application context.
Importance of referring to Flask’s official documentation
Flask’s official documentation serves as a reliable source of information for developers. It provides detailed explanations of Flask’s features, including how to handle the application context correctly. Referring to the documentation ensures that developers understand the best practices and techniques to avoid errors.
Following Flask’s recommended practices for handling application context
Flask’s documentation offers guidance on how to handle the application context correctly. It provides examples and explanations on various aspects, from using callbacks and asynchronous tasks to working with extensions and decorators. By following Flask’s recommended practices, developers can minimize the risk of encountering “RuntimeError: Working outside of application context” errors.
Resources for further learning and understanding Flask’s best practices
To delve deeper into understanding Flask’s best practices and how to handle the application context correctly, developers can explore additional resources. These resources include Flask’s official documentation, online tutorials, blog posts, and community forums where developers can seek assistance and share insights.
FAQs
Q: What does “Working outside of application context” mean in Flask?
A: “Working outside of application context” is an error that occurs when code tries to access or modify Flask-specific resources, such as the request object or the database connection, outside of the application context. The application context ensures that Flask functions properly by providing access to crucial resources during the request-response cycle.
Q: How can I fix “RuntimeError: Working outside of application context” in Flask?
A: To fix this error, you need to ensure that your code is always executed within the application context. This can be achieved by using the `app.app_context()` block or following Flask’s recommended patterns for handling asynchronous tasks. It is also important to refer to Flask’s documentation and best practices to avoid common mistakes and ensure proper usage of extensions and decorators.
Q: Can “Working outside of application context” errors lead to data corruption?
A: Yes, “Working outside of application context” errors can potentially lead to data corruption. When code operates outside of the application context, it may not have access to the necessary resources to handle and process data correctly. This can result in unexpected changes to the data or even data loss.
Q: Are there any specific Flask extensions or decorators that commonly cause “Working outside of application context” errors?
A: Several Flask extensions and decorators can potentially cause “Working outside of application context” errors if not used properly. Some common examples include Flask-Mail, Flask-SQLAlchemy, Flask-SocketIO, Flask-Cache, and Flask-Login. It is important to refer to the documentation of each extension and decorator to understand their specific requirements and how to utilize them correctly within the Flask application context.
Flask – Runtimeerror: Working Outside Of Application Context #Flask #Python #Flaskruntimeerror
What Is Test Runtimeerror Working Outside Of Request Context?
In the realm of web development, working with request contexts is a common practice. It allows developers to interact with the current request being made by a user and access necessary data or perform specific actions accordingly. However, sometimes you may encounter a situation where you receive a test runtime error stating that you are working outside of the request context. In this article, we will dive into this issue, exploring its causes, potential solutions, and address some frequently asked questions.
Understanding the Error:
When you see a test runtime error stating that you are working outside of the request context, it means that you are trying to access or modify certain objects or variables that are only available within the context of a request. These objects are typically initialized and made available by web frameworks such as Flask or Django.
The request context provides a scope within which you can access various globally available objects, such as the current application, request, session, or database connections. It helps ensure that each request is processed independently without interfering with concurrent requests. However, when working outside of this context, attempts to access these objects will result in the mentioned error.
Causes of the Error:
1. Running Code Outside of Request Context: The primary cause of this error is running code in a place where it is not intended to be executed, i.e., outside of the request context. This often occurs when you attempt to access or modify request-related objects in a separate thread or outside of a view function.
2. Asynchronous Code Execution: Nowadays, asynchronous programming has gained popularity due to its ability to improve performance in certain scenarios. However, when using asynchronous frameworks or libraries, there is a higher chance of encountering a runtime error if it is not handled properly. Asynchronous code execution can sometimes disrupt the request context, leading to the error.
3. Incorrect Configuration or Setup: In some cases, the error may be caused by incorrect configuration or setup of your web framework. This could be due to missing or misconfigured middleware, decorators, or other components that affect the request context.
Solutions:
1. Ensure Proper Execution Context: The most important step in resolving this error is to verify that your code is being executed within the request context. Make sure you are running your code in the appropriate location, such as within a view function or a context manager provided by your web framework.
2. Avoid Asynchronous Code Outside of Request Context: If you are using asynchronous programming techniques, ensure that you are handling the request context correctly. Check if your web framework offers asynchronous support or utilize techniques such as using async middleware or decorators specifically designed for the framework you are using.
3. Review Configuration and Setup: If the error persists, review your configuration and setup for any potential issues. Double-check that you have correctly configured any required middleware or decorators that manage the request context. Additionally, ensure that you are importing and initializing your web framework correctly.
FAQs:
Q1. Can this error occur in any web framework?
A1. Yes, this error can occur in any web framework that utilizes request contexts to provide access to request-related objects. It is important to understand how your chosen web framework handles request contexts to effectively resolve this error.
Q2. Can multiple threads accessing the request context cause this error?
A2. Yes, multiple threads accessing the request context simultaneously can lead to this error. It is important to ensure thread safety and handle concurrent requests appropriately to avoid such issues.
Q3. Are there any tools or libraries available to debug this error?
A3. Yes, most web frameworks provide tools or libraries for debugging and troubleshooting request context-related issues. Consult the documentation of your specific web framework for recommended debugging techniques.
Q4. Can this error occur when writing tests?
A4. Yes, this error can occur when writing tests if the testing environment does not properly handle request contexts. Ensure that your testing framework has mechanisms in place to handle request contexts or consider mocking the necessary objects and variables.
In conclusion, encountering a test runtime error stating that you are working outside of the request context is a common issue in web development. It occurs when you attempt to access or modify request-related objects outside of their intended execution context. By understanding the causes and implementing the appropriate solutions, you can effectively resolve this error and ensure the smooth execution of your web applications.
What Is The Application Context Of Active Flask?
When developing web applications, it is essential to have a solid understanding of the application context in order to properly manage and handle the resources and variables associated with each request. Flask, a popular micro web framework for Python, provides an application context that allows developers to access request and application-specific data whenever needed. In this article, we will explore the application context of active Flask and delve into its importance and practical usage.
Understanding Flask’s Application Context:
Flask’s application context is an essential component that manages and holds the state of the current application. It ensures that multiple requests can be safely handled while keeping the necessary resources and variables isolated. The application context is created when a request is received and is destroyed at the end of the request/response cycle. This makes Flask an efficient and scalable framework for handling web applications.
Key Features and Benefits of Flask’s Application Context:
1. Global Access to Request and Application Context: The application context provides a mechanism for accessing request and application-specific data globally within Flask. This allows developers to store and retrieve data that is necessary for the execution of various functions and views throughout the application.
2. Resource Management: Flask’s application context effectively manages resources by creating and tearing down resources for each request. It ensures the proper clean-up of resources between requests, preventing resource leaks and improving the overall performance and stability of the application.
3. Context Locals: Context locals are a common concept in Flask’s application context. It allows developers to define variables within the application context that can be accessed across the entire application. These context locals are particularly useful for storing user-specific data or sharing commonly used objects such as database connections or configuration settings.
4. Thread Safety: Flask’s application context is designed to be thread-safe, allowing multiple requests to be processed simultaneously or in parallel. Each request is associated with its own isolated application context, ensuring that concurrent requests do not interfere with each other.
Practical Usage and Implementation of Flask’s Application Context:
1. Request-specific Data Access: The application context is particularly useful for accessing request-specific data such as the loaded user session, request headers, or cookies. Developers can conveniently access this data from anywhere within the application by using the `flask.request` object.
2. Database Connections: Flask’s application context is commonly employed when dealing with database connections. It allows developers to maintain a persistent database connection throughout the request/response cycle, ensuring that the connection is properly closed at the end of each request.
3. Authentication and Authorization: When implementing authentication and authorization mechanisms, Flask’s application context aids in managing user sessions and their associated data. Developers can store and retrieve session-specific information using context locals, enabling secure access control within the application.
4. Custom Context Processors: Flask provides a mechanism called context processors that allows developers to inject custom data or functions within the application context. These custom context processors are executed for every request and can add additional data or functionality for processing views or templates.
FAQs:
Q1. Can I access the application context outside of Flask’s views?
A1. Yes, Flask’s application context can be accessed outside of the views by utilizing the `current_app` object. The `current_app` object provides a reference to the active application context, allowing access to various application-specific resources.
Q2. Can Flask’s application context be used in multi-threaded environments?
A2. Yes, Flask’s application context is designed to be thread-safe, making it suitable for multi-threaded environments. Each request is associated with its own isolated application context, ensuring concurrent requests do not interfere with each other.
Q3. How is the lifespan of the application context managed?
A3. Flask’s application context is created at the start of a request and is destroyed at the end of the request/response cycle. It ensures proper resource management and prevents resource leaks by creating and tearing down resources for each request.
Q4. Are there any performance implications of using Flask’s application context?
A4. Flask’s application context is optimized for performance and has a minimal impact on the overall speed of the application. It provides a lightweight mechanism for managing resources and facilitates efficient request handling.
In conclusion, Flask’s application context plays a crucial role in managing and handling resources within web applications. Its ability to provide global access to request-specific and application-specific data makes Flask an efficient and scalable framework. By understanding and properly utilizing the application context, developers can build robust and secure web applications.
Keywords searched by users: runtimeerror: working outside of application context Working outside of application context pytest, Application context Flask, flask sqlalchemy working outside of application context, runtimeerror: working outside of request context pytest, App app_context, flask-apscheduler working outside of application context, Db create_all not working Flask, flask context
Categories: Top 21 Runtimeerror: Working Outside Of Application Context
See more here: nhanvietluanvan.com
Working Outside Of Application Context Pytest
Introduction:
Pytest is a popular testing framework for Python applications that allows developers to write test cases in a simple and concise manner. It provides various features and functionalities to streamline the testing process and ensure the quality and reliability of the codebase. However, there may be instances where you need to work outside of the application context in pytest. This article will discuss the concept of working outside of application context in pytest, its use cases, and provide a detailed explanation of how to do it.
Understanding the Application Context in pytest:
Before diving into the concept of working outside of the application context, it is essential to understand what the application context is in pytest. The application context is a crucial component of pytest that provides access to various resources, such as the database, configuration, and request context.
The application context is typically created and managed by pytest itself, allowing you to perform actions within the context of your application. This is particularly useful for testing scenarios that require access to resources that are initialized during the application’s runtime.
Working outside of Application Context in pytest:
There are instances where you may need to perform actions outside the application context in pytest. This can be useful in cases where you want to test functionality that doesn’t rely on resources provided within the application context or when you want to interact with external services directly.
To work outside of the application context in pytest, you can make use of fixtures. Fixtures in pytest provide a way to set up and tear down resources required by your test cases. By leveraging fixtures, you can create a custom fixture that allows you to work outside the application context.
Creating a Custom Fixture to Work Outside of Application Context:
To create a custom fixture that enables working outside the application context, you can make use of the `pytest.fixture` decorator. This decorator enables the configuration of the fixture and provides the necessary setup and teardown functions.
Here’s an example of creating a custom fixture to work outside the application context:
“`python
import pytest
@pytest.fixture
def my_fixture():
# Setup code outside of application context
yield
# Teardown code outside of application context
“`
In the setup code block of the custom fixture, you can perform any necessary actions outside the application context that you require for your test case. This can include interacting with external services, initializing resources, or any other logic that needs to be executed.
Similarly, in the teardown code block, you can clean up or release any resources that were initialized in the setup code block.
Using the Custom Fixture:
To utilize the custom fixture for working outside the application context, you can simply include it as an argument in your test case:
“`python
def test_my_function(my_fixture):
# Test case logic
“`
The custom fixture will be automatically invoked and set up before the test case execution begins. Once the test case execution is complete, the teardown code block within the fixture will be triggered.
Frequently Asked Questions (FAQs):
Q: When should I consider working outside the application context in pytest?
A: Working outside the application context is useful when you need to test functionality that doesn’t rely on resources provided within the application context or when you want to interact with external services directly.
Q: Can I have multiple custom fixtures for working outside the application context?
A: Yes, you can create multiple custom fixtures, each serving different purposes, to work outside the application context. Just ensure that you include the required fixture as an argument in your test cases accordingly.
Q: Can I access resources from the application context while working outside it?
A: No, when working outside the application context, you won’t have direct access to resources provided by the application context. In cases where you need to access those resources, consider working within the application context itself.
Conclusion:
Working outside the application context in pytest can be beneficial in scenarios where you need to test functionality that doesn’t require resources within the application context or when you want to interact with external services directly. By utilizing custom fixtures, you can easily set up and tear down resources to work efficiently outside the application context. Consider the use cases and guidelines discussed in this article to leverage the flexibility and power of pytest in your testing efforts.
Application Context Flask
Flask, a popular microframework for building web applications in Python, offers a wide range of powerful features and tools to streamline development processes. One such feature is the application context, which plays a crucial role in managing resources and maintaining state throughout the lifespan of an application. In this article, we will delve into the concept of application context in Flask, discuss its benefits, and explore some use cases.
Understanding Application Context:
In Flask, an application context represents the state and configuration of an application at a specific point in time. It serves as a container for global variables and provides an environment for executing specific tasks within the application. Every Flask application has one application context associated with it, which gets activated whenever a request is received.
The application context ensures that resources like database connections, current user information, and other application-specific settings are available throughout the request-response cycle. It eliminates the need to repeatedly recreate or fetch these resources, thereby improving efficiency and reducing overhead.
Benefits of Application Context:
1. Resource Management: One of the key advantages of using application context is managing resources efficiently. By creating these resources once and keeping them within the application context, Flask can reuse them across multiple requests. This avoids the overhead of creating new connections or fetching data repeatedly, resulting in faster response times.
2. Context Preservation: Since the application context persists throughout the lifespan of a request, it allows the application to maintain data integrity and handle concurrency issues effectively. For instance, if multiple requests are accessing the same resource simultaneously, Flask ensures mutual exclusion by toggling the application context across those requests.
3. Global Variable Access: Flask’s application context serves as a container for storing global variables that need to be accessed across different modules or functions within an application. By utilizing global context-local proxies, developers can access these variables seamlessly without the need for explicit parameter passing.
4. Simplified Testing: Application context makes testing Flask applications easier by providing a consistent environment for executing tests. Developers can utilize context managers or decorators to automatically handle context initialization and teardown, ensuring that the application state is reset before and after each test.
Use Cases for Application Context:
1. Database Operations: In applications that involve frequent database operations, the application context proves to be invaluable. By maintaining database connections within the application context, Flask eliminates the need to open and close connections on each request. This significantly improves performance while reducing the chances of connection leaks.
2. Authentication and Authorization: Application context can store the current user’s information and authentication details, enabling efficient authorization of requests. It allows Flask to retrieve user-specific data without redundant retrieval from the database or external sources.
3. Caching: When it comes to caching, the application context can be utilized effectively. By storing cached data within the context, Flask can serve subsequent requests for the same data directly from the cache, reducing the load on the application and enhancing response times.
4. Configuration Handling: Flask’s application context is also helpful for managing configuration settings. In complex applications, different configurations may be required for specific requests or modules. By utilizing the application context, developers can easily switch between various configurations without affecting the rest of the application.
FAQs:
1. How is the application context different from the request context in Flask?
While the application context represents the state of the entire application, the request context is specific to an individual request. The application context remains active throughout the lifespan of the application, while the request context is created and destroyed for each request.
2. Can I have multiple application contexts in a Flask application?
No, Flask allows only one application context per application. However, multiple request contexts can exist simultaneously, serving different users or requests.
3. How do I access the application context within a view function?
Flask provides the “current_app” proxy, allowing developers to access the application context within view functions or any other code within the application. The “current_app” proxy automatically references the correct application context for the specific execution path.
4. Does Flask handle race conditions automatically with the application context?
Flask does not handle race conditions automatically. However, it ensures mutual exclusion by toggling the application context across concurrent requests, thereby preventing simultaneous access to shared resources.
In conclusion, the application context in Flask is a powerful mechanism that allows for efficient resource management, enhanced scalability, and simplified testing. By maintaining the state and configuration of an application, Flask can reuse resources and handle concurrent requests effectively. Understanding and utilizing the application context can significantly optimize the performance and maintainability of Flask applications.
Images related to the topic runtimeerror: working outside of application context
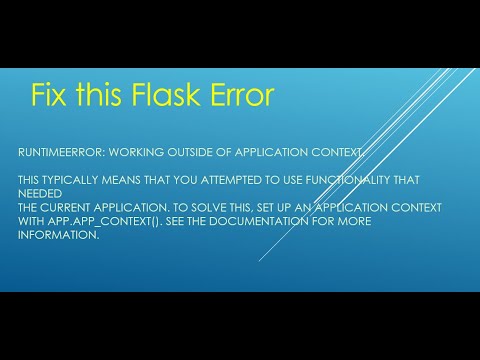
Found 21 images related to runtimeerror: working outside of application context theme
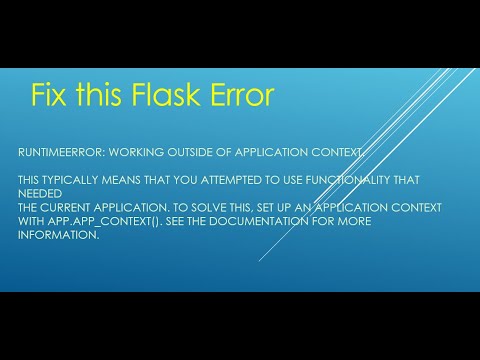
![runtimeerror: working outside of application context. [SOLVED] Runtimeerror: Working Outside Of Application Context. [Solved]](https://itsourcecode.com/wp-content/uploads/2023/05/runtimeerror-working-outside-of-application-context.png)
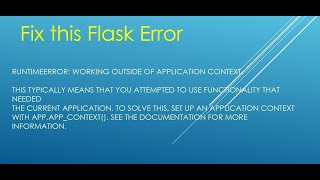
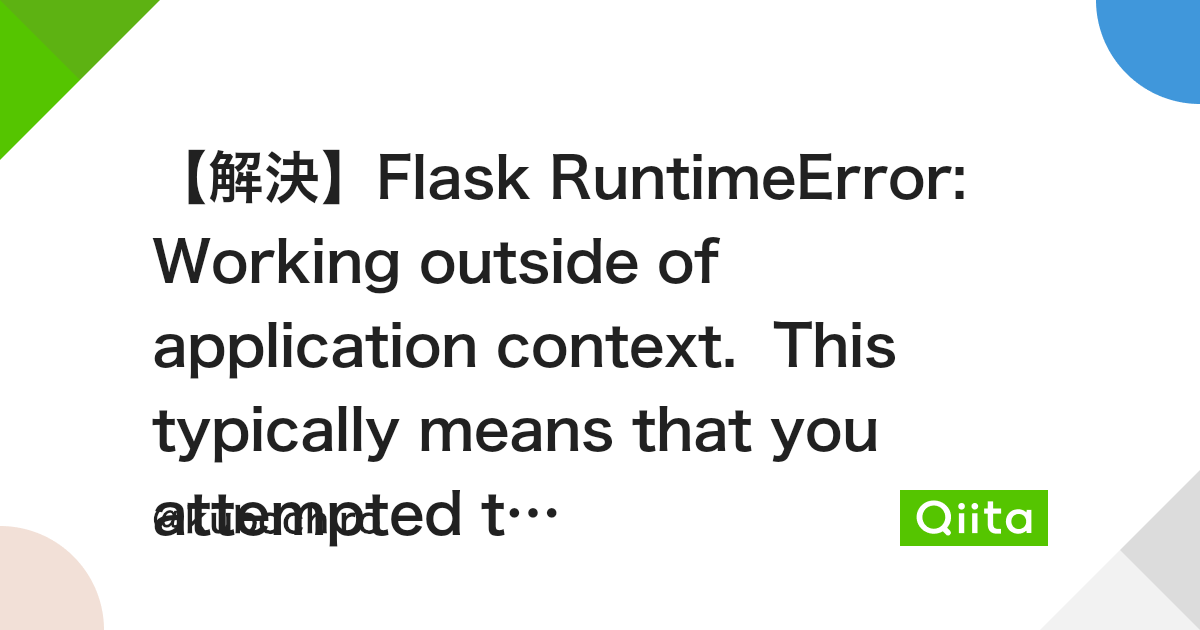
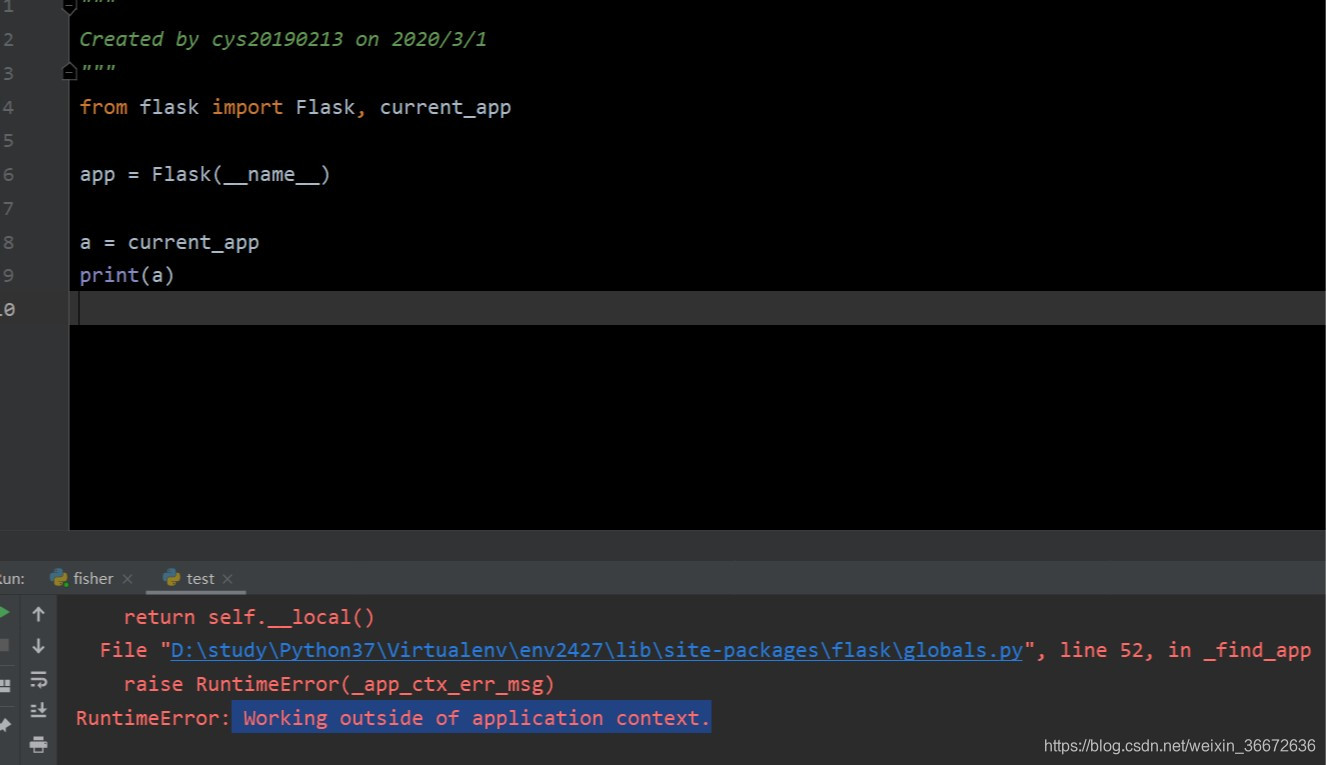


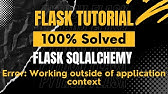
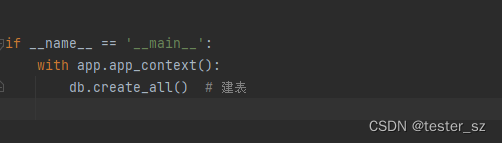
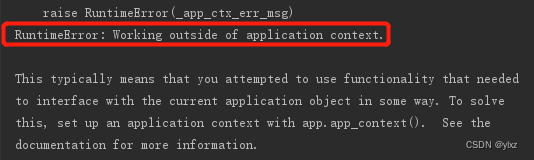
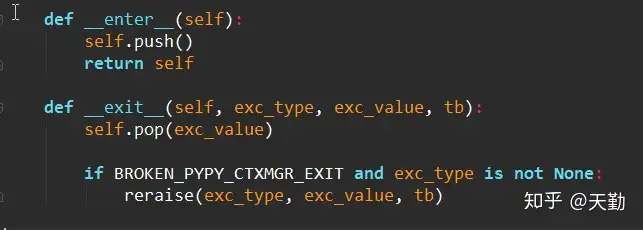
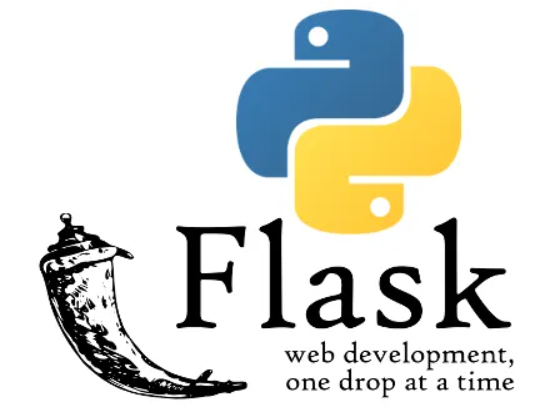
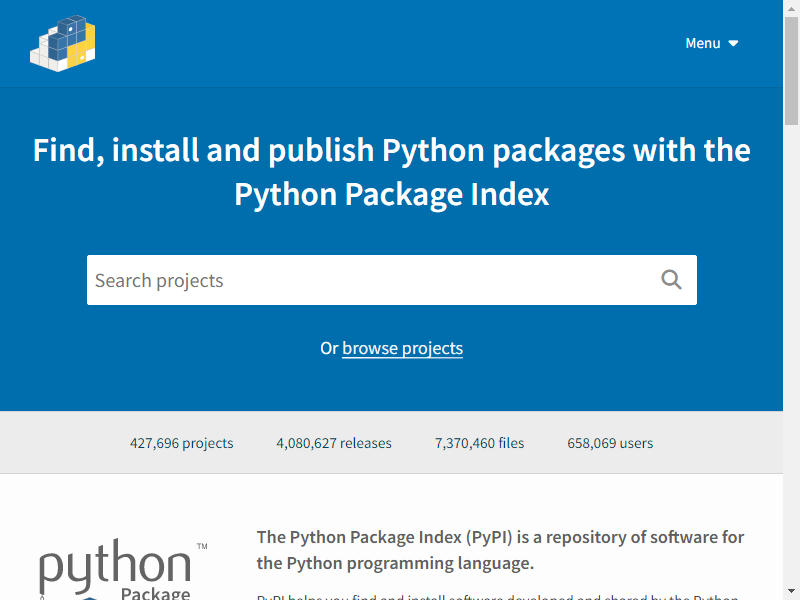

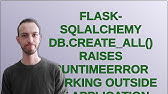

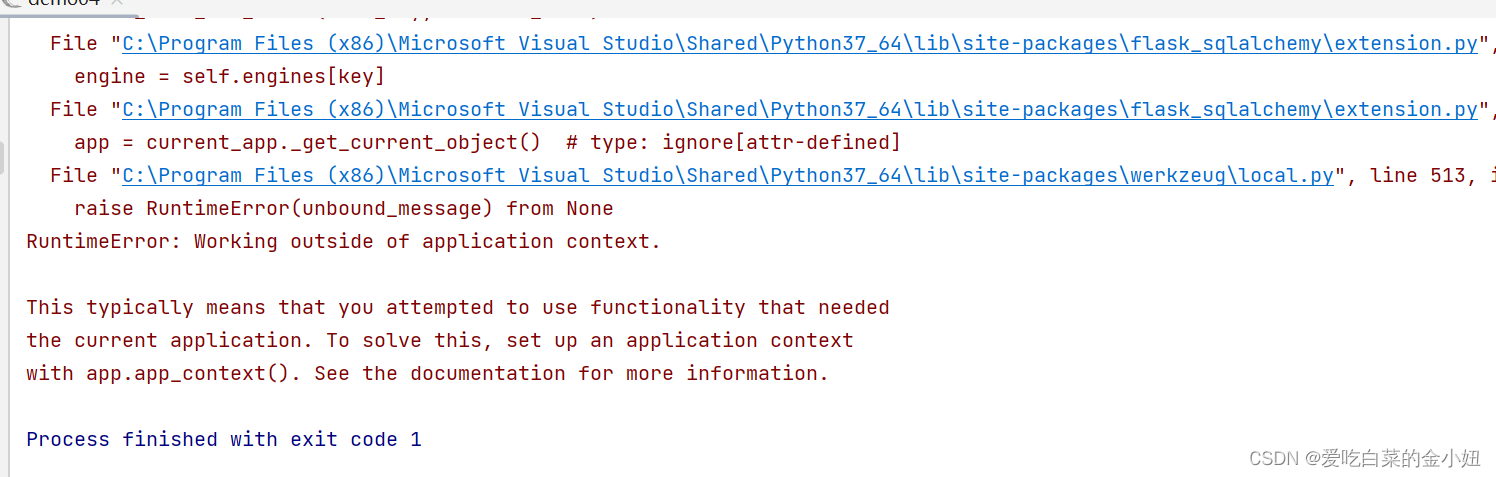
![runtimeerror: working outside of application context. [SOLVED] Runtimeerror: Working Outside Of Application Context. [Solved]](https://itsourcecode.com/wp-content/uploads/2021/01/IT-SOURCECODE_ICON-07.jpg)
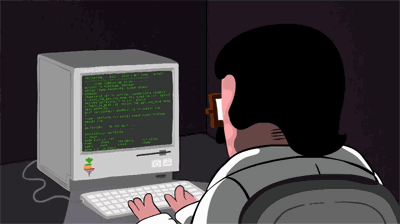
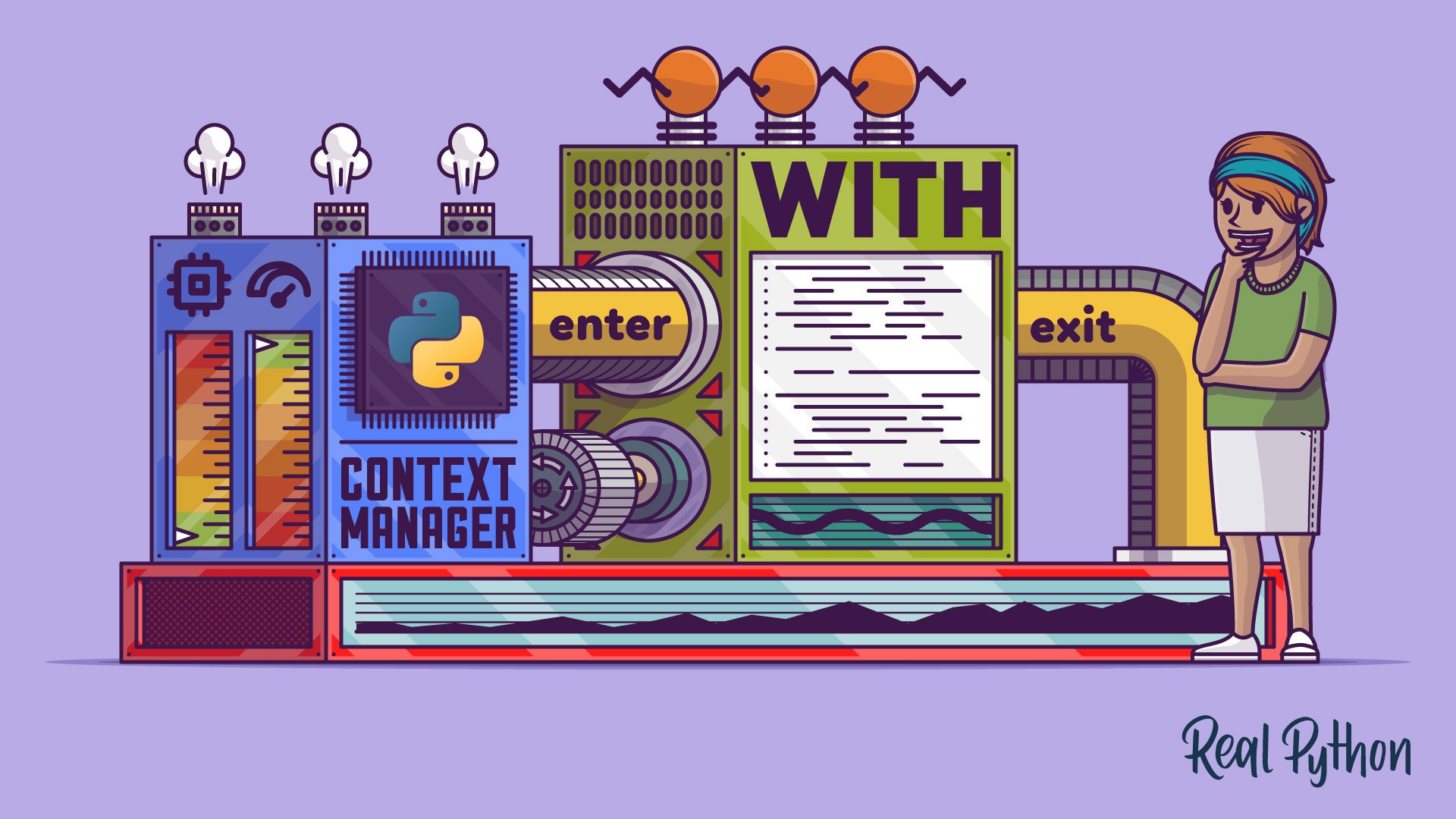
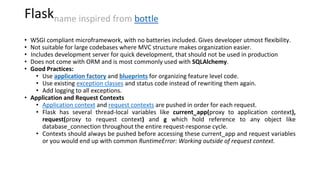


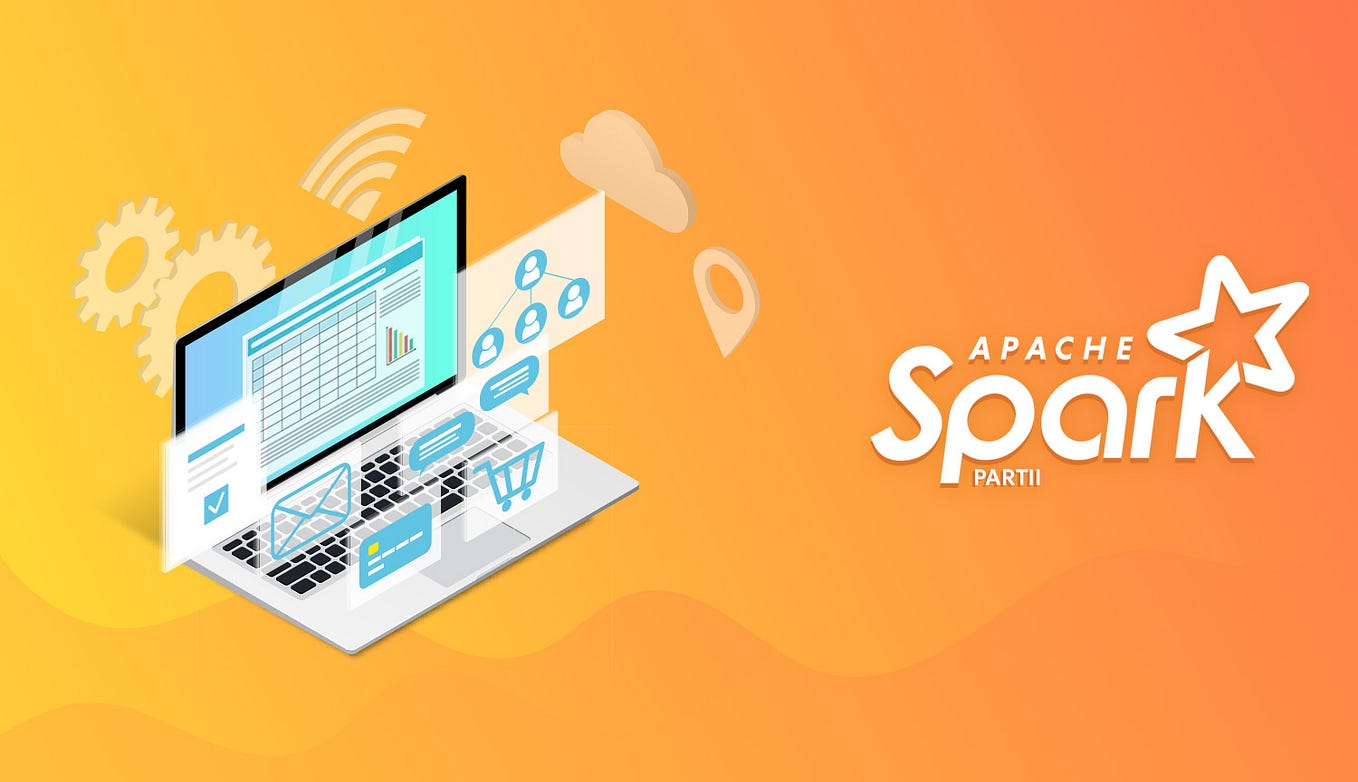

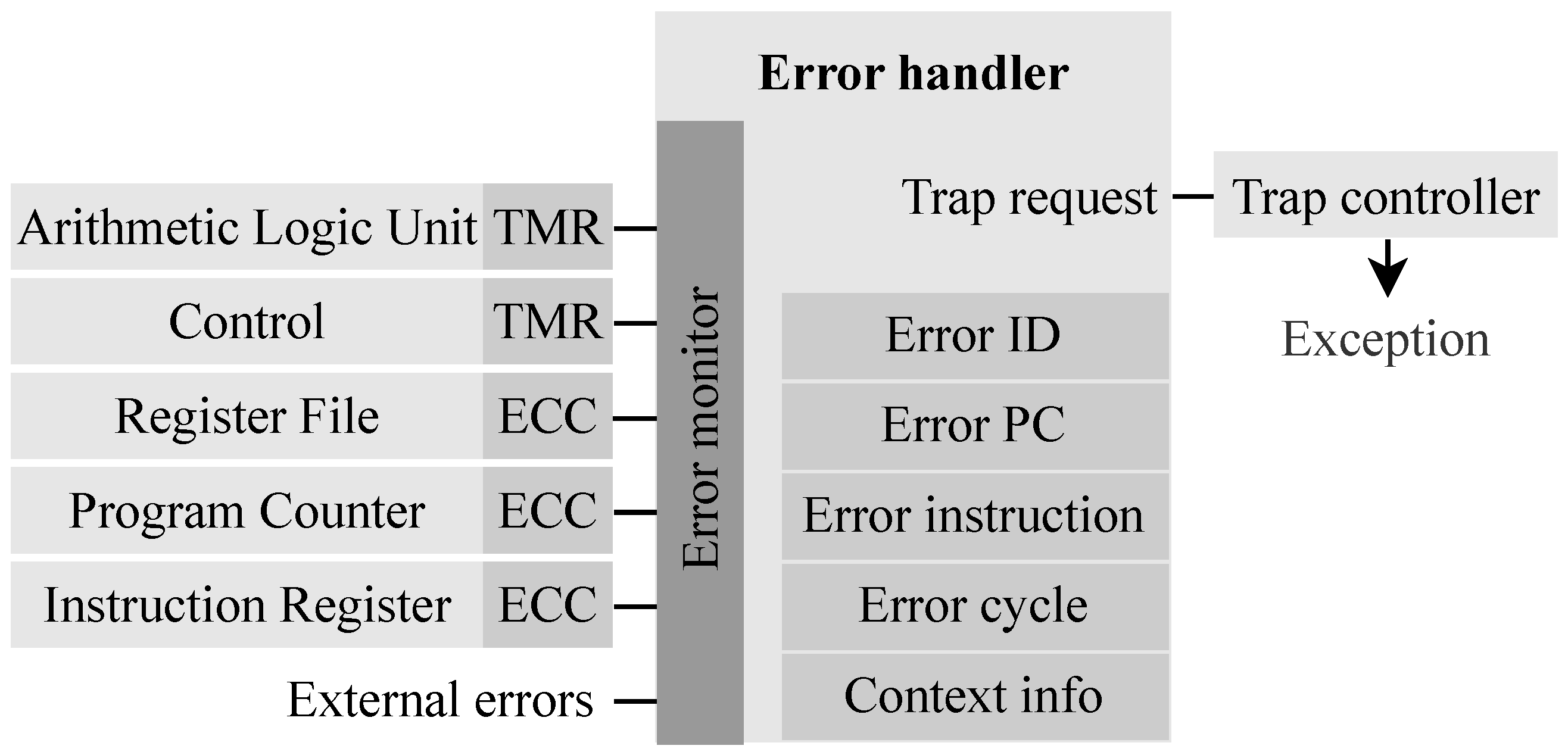
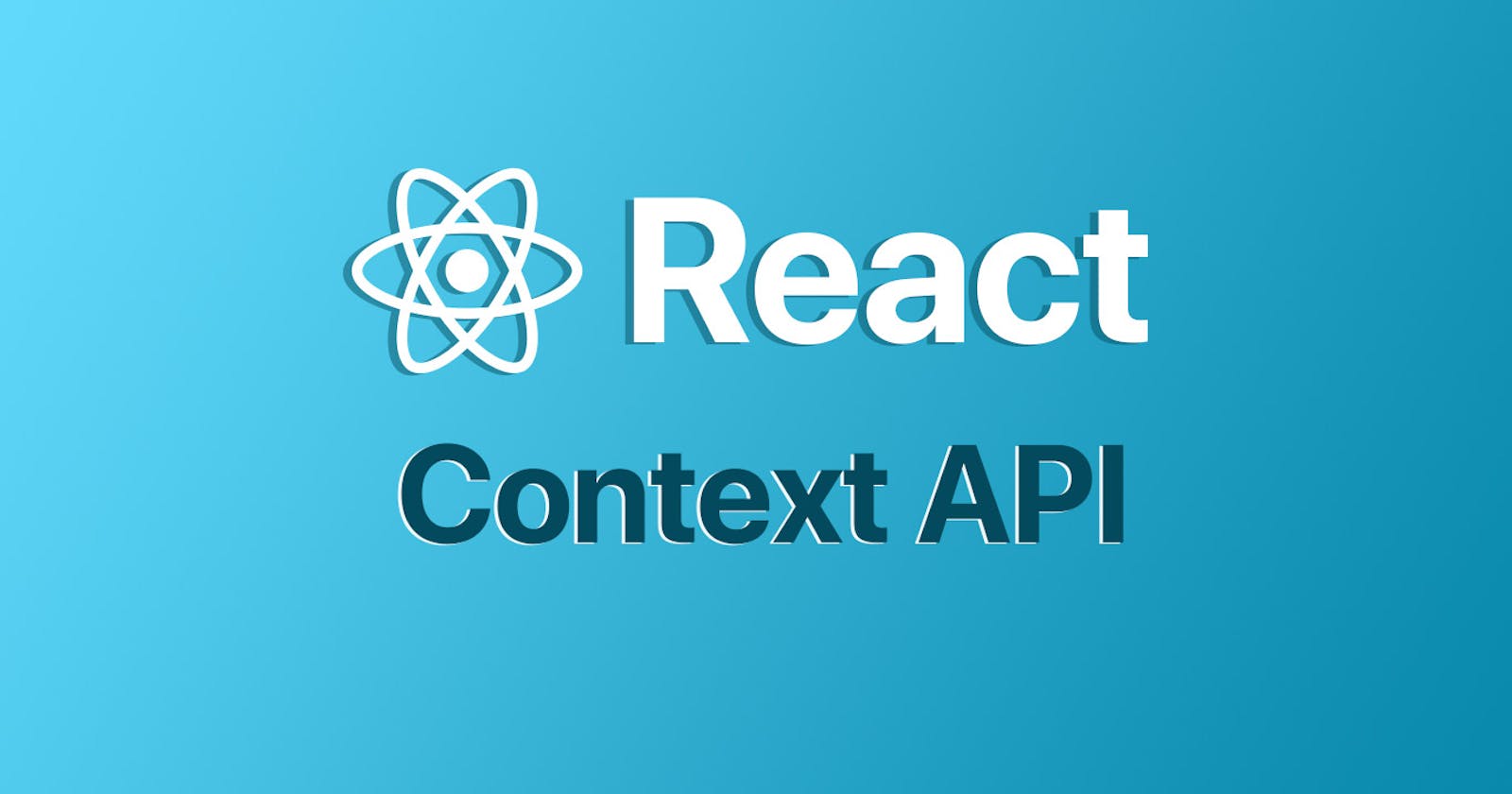

Article link: runtimeerror: working outside of application context.
Learn more about the topic runtimeerror: working outside of application context.
- RuntimeError: working outside of application context
- The Application Context — Flask Documentation (2.3.x)
- RuntimeError: Working Outside of Application Context – Sentry
- The Request Context — Flask Documentation (2.3.x)
- Flask-SQLAlchemy Documentation (3.0.x) » Flask Application Context
- flask.globals current_app Example Code – Full Stack Python
- Understanding Contexts in Flask – Abacus
- Flask: RuntimeError: Working outside of application context.
- RuntimeError: Working outside of application context in flask
- Run time error: working outside of application context. : r/flask
- 8. The Application Context — Flask API – GitHub Pages
- runtimeerror: working outside of application context. [SOLVED]
- RuntimeError: Working outside of application context – Python
See more: https://nhanvietluanvan.com/luat-hoc/