Reverse A String C#
Reversing a string is a common task in programming, and it involves changing the order of characters in a given string. In C#, there are various approaches to reversing a string, from traditional methods using loops to utilizing built-in functions and recursion. This article will explore these different techniques and also provide insights into preserving word order, handling special characters, using the StringBuilder class, and performance considerations when reversing a string in C#.
II. Understanding the concept of reversing a string in C#
A. What is a string?
A string is a sequence of characters that is used to store and manipulate text in programming languages. In C#, strings are immutable, meaning that once created, they cannot be modified. To change a string, a new string object needs to be created.
B. What does it mean to reverse a string?
Reversing a string simply means to change the order of its characters, so the last character becomes the first, the second-to-last character becomes the second, and so on.
C. Importance of reversing a string in certain applications
Reversing a string is a task that finds its applications in various scenarios. It can be used in password encryption, searching and sorting algorithms, data analysis, and text processing, to name a few. Understanding how to reverse a string is fundamental in programming.
III. Traditional approach to reversing a string in C#
The traditional approach to reversing a string involves using loops to iterate through the string’s characters and then reversing them character by character.
A. Using a loop to iterate through the string
A loop, such as a for loop, can be used to iterate through the characters of a string from the end to the beginning and store them in a new string variable.
B. Reversing the string character by character
The characters of the original string can be accessed individually and stored in a new string variable in reverse order.
C. Implementing the traditional approach in C#
In C#, the traditional approach to reversing a string can be achieved by converting the string to a character array, iterating through the array, and then constructing a new reversed string.
IV. Using built-in functions to reverse a string in C#
C# provides built-in functions that simplify the task of reversing a string. These functions include the String.Reverse() method and the Array.Reverse() method.
A. Exploring the String.Reverse() method
The String.Reverse() method is a member of the System.Linq namespace. It allows for reversing the characters of a string by creating a new string object.
B. Utilizing the Array.Reverse() method
The Array.Reverse() method is a member of the System namespace. It provides a way to reverse the elements of an array, including a character array.
C. Advantages and limitations of using built-in functions
Using built-in functions can simplify the process of reversing a string in C#, as they are optimized for performance and eliminate the need for custom implementations. However, they may not be suitable for more specific scenarios that involve preserving word order or dealing with special characters.
V. Reversing a string using recursion in C#
A. Understanding the concept of recursion
Recursion is a programming technique where a function calls itself repeatedly until a base condition is met. It can be used to reverse a string by breaking it down into smaller substrings.
B. Developing a recursive function to reverse a string
A recursive function can be created to reverse a string by calling itself with a substring that excludes the first character, and then appending the first character at the end.
C. Analyzing the performance of recursive approach
The recursive approach to reversing a string can be simpler to implement but may have a higher time complexity compared to other methods. It is important to consider the performance implications when dealing with large strings.
VI. Reverse a string preserving word order in C#
A. Challenges of reversing a string while preserving the order of words
When reversing a string, preserving the order of words becomes a challenge as the reversed string would also reverse the word order.
B. Algorithm for reversing a string with preserved word order
To reverse a string while preserving word order, the string can be split into words, each word can be reversed individually, and then the words can be concatenated in reverse order.
C. Implementing the algorithm in C#
Implementing the algorithm involves using string splitting, reversing each word, and then rejoining the reversed words in the correct order.
VII. Reverse a string with special characters in C#
A. Dealing with special characters during string reversal
When reversing a string that contains special characters, it is important to handle them correctly to preserve their positions.
B. Algorithm for reversing a string containing special characters
An algorithm for reversing a string containing special characters would involve identifying and preserving the positions of these special characters during the reversal process.
C. Coding the algorithm in C#
The algorithm can be implemented by identifying the special characters, reversing the remaining characters, and inserting the special characters back into their respective positions.
VIII. Reverse a string using StringBuilder class in C#
A. Introduction to the StringBuilder class
The StringBuilder class in C# is a mutable string type that provides efficient string manipulation operations. It can be used to reverse a string efficiently.
B. Utilizing the StringBuilder class to reverse a string
The StringBuilder class provides a Reverse() method that can directly reverse the characters in the string builder object.
C. Advantages and disadvantages of using StringBuilder for string reversal
Using the StringBuilder class can be more memory-efficient, especially when dealing with large strings. However, it may not be the best choice if the program requires preserving word order or handling special characters.
IX. Performance considerations when reversing a string in C#
A. Time complexity analysis of various approaches
Different string reversal techniques have different time complexities, and it’s important to consider the efficiency of these approaches when dealing with large strings.
B. Space complexity comparison of different string reversal techniques
The space complexity of different string reversal techniques can vary depending on whether a new string object is created or the original string is modified in place.
X. Conclusion
Reversing a string in C# is a common task that can be achieved using different techniques, including traditional approaches using loops, built-in functions, recursion, preserving word order, handling special characters, and utilizing the StringBuilder class. Each approach has its advantages and limitations, and the choice depends on the specific requirements of the program. Understanding the various techniques and considering the performance and memory implications can help in optimizing code and achieving efficient string reversal in C#.
FAQs:
Q1: How to reverse a string in C++?
A1: In C++, you can reverse a string by using the reverse() function from the algorithm library or by manually iterating through the string and swapping characters from both ends.
Q2: How to reverse a string in Java?
A2: In Java, you can reverse a string by converting it to a StringBuilder object and using its reverse() method, or by manually iterating through the string and constructing a new string in reverse order.
Q3: Hàm reverse string C++ là gì?
A3: Hàm reverse trong C++ là một hàm trong thư viện algorithm dùng để đảo ngược các phần tử trong một chuỗi.
Q4: How to input a string and print it in reverse order in C?
A4: In C, you can input a string using the scanf() function and then iterate through the string from the end to the beginning to print it in reverse order.
Q5: How to input a string in C?
A5: In C, can use the gets() function or the fgets() function to input a string from the standard input.
Q6: How to reverse words in a given string in C?
A6: To reverse words in a given string in C, you can split the string into words, reverse each word individually, and then concatenate the reversed words in the correct order.
Q7: How to write a program in C to print a string in reverse using a pointer?
A7: You can write a program in C to print a string in reverse using a pointer by iterating through the string using a pointer and printing the characters in reverse order.
Reverse A String | C Programming Example
Keywords searched by users: reverse a string c# Reverse a string C++, Reverse(String Java), Hàm reverse string C++, Input a string and print it in reverse order, Scanf string C, How to input string in C, Reverse words in a given string in C, Write a program in C to Print a string in reverse using a pointer
Categories: Top 11 Reverse A String C#
See more here: nhanvietluanvan.com
Reverse A String C++
When programming in C++, you may often come across situations where you need to reverse a string. Reversing a string simply means changing the order of its characters, so the first character becomes the last, the second becomes the second last, and so on. In this article, we will explore different approaches to reverse a string in C++, discuss their efficiency, and provide some useful tips and FAQs.
Approaches to Reverse a String
1. Using a temporary variable:
One straightforward approach to reverse a string is by using a temporary variable. We iterate through half of the string and swap the characters at corresponding positions. For example, if we have a string “hello,” we would swap ‘h’ with ‘o’ and ‘e’ with ‘l’. This approach is quite efficient, as it only requires one iteration through the string and performs a constant number of swaps.
2. Using the reverse() function:
C++ provides a built-in function called `reverse()` in the `
3. Using recursion:
Another interesting approach to reversing a string is by using recursion. We define a recursive function that takes a string and its length as parameters. The base case is when the length is 0 or 1, in which case we return the same string. Otherwise, we recursively call the function on a substring that excludes the first character and concatenate it with the first character. This approach has a simple and elegant recursive implementation, but it may not be the most efficient solution for large strings due to the overhead of function calls.
Efficiency Considerations
When reversing a string, it is crucial to consider the efficiency of the chosen approach, particularly if dealing with large strings. The first approach, using a temporary variable, is the most efficient in terms of runtime and memory usage. It requires a single iteration through the string and performs a constant number of swaps, resulting in a time complexity of O(n) and space complexity of O(1).
On the other hand, using the `reverse()` function has a time complexity of O(n) and space complexity of O(n), as it needs to create a copy of the original string. While this approach might be suitable for smaller strings, it may not be the optimal choice for more substantial inputs due to the extra space requirement.
The recursive approach has a time complexity of O(n) as well, but it has a space complexity of O(n) due to the recursive function calls on substrings. This approach can be elegant for shorter strings, but its performance may degrade for larger ones.
Tips for Reversing a String
1. Consider the requirements of your program: Depending on your specific use case, choose the appropriate approach for reversing a string. If efficiency is crucial, use the first approach with a temporary variable.
2. Be mindful of the input size: If dealing with large strings, avoid the `reverse()` function or recursive approach, as they may consume excessive memory. Stick to the first approach or consider utilizing more optimized algorithms or data structures if performance is critical.
3. Understand string manipulations: Reversing a string is not only useful in its own right but also helps build a solid foundation for other string manipulation operations. Mastering string manipulation techniques can be beneficial in solving various programming challenges.
FAQs
Q: Can I reverse a C++ string in-place, without using additional memory?
A: Yes, you can reverse a string in-place by using the first approach mentioned earlier. This approach swaps the characters within the original string, without requiring any additional memory.
Q: Why is the recursive approach less efficient for large strings?
A: The recursive approach involves multiple function calls and creates new substrings, leading to increased memory usage. Additionally, the overhead of function calls can impact the performance, making it less efficient compared to other approaches.
Q: Can I reverse a string using the built-in `reverse()` function without creating a new string?
A: No, the `reverse()` function in C++ reverses the order of elements in a range of iterators. It does not modify the string in-place but rather creates a copy of the original string with reversed elements.
In conclusion, reversing a string in C++ can be accomplished through various approaches, each with its own advantages and efficiency considerations. By understanding the available techniques and assessing the requirements of your program, you can choose the most suitable method for reversing strings efficiently and without unnecessary memory consumption.
Reverse(String Java)
In the Java programming language, the reverse() method is used to reverse the order of characters in a given string. It is a commonly used function in programming as it allows developers to manipulate strings efficiently. In this article, we will explore the reverse(String Java) function in detail, discussing its syntax, usage, and various examples. Additionally, we will address some frequently asked questions to provide a comprehensive understanding of this method.
Syntax:
The syntax for using the reverse(String Java) function is as follows:
“`java
public static String reverse(String str)
“`
The reverse() method takes a string as input and returns a new string with characters reversed. Here, `str` represents the string that needs to be reversed.
Usage:
The reverse() method is defined in the `java.lang.StringBuilder` class, which is a mutable sequence of characters. To use the reverse() method, we first need to create an instance of the StringBuilder class, passing the input string argument to its constructor. Then, we can call the reverse() method on the StringBuilder object.
Example:
“`java
String originalString = “Hello, World!”;
StringBuilder reversedString = new StringBuilder(originalString).reverse();
System.out.println(reversedString);
“`
Output:
“`
!dlroW ,olleH
“`
In this example, we define the `originalString` variable, which stores the string “Hello, World!”. We pass this string to the StringBuilder constructor, creating a StringBuilder object. Then, we call the reverse() method on the StringBuilder object, reversing the characters in the string. Finally, we print the reversed string using System.out.println().
FAQs:
Q1. Can the reverse() method be used with immutable strings?
Yes, while the reverse() method is defined in the StringBuilder class, it can be used with immutable strings as well. However, using the reverse() method directly on immutable strings would require converting the string to a mutable StringBuilder object and then reversing it.
Q2. Is there any alternative method to reverse a string in Java?
Yes, apart from using the reverse() method, there are alternative approaches to reverse a string in Java. One common approach is to convert the string into a character array, iterate over it, and swap the characters from both ends until the middle is reached.
Example:
“`java
String originalString = “Hello, World!”;
char[] charArray = originalString.toCharArray();
int start = 0;
int end = charArray.length – 1;
while (start < end) { char temp = charArray[start]; charArray[start] = charArray[end]; charArray[end] = temp; start++; end--; } String reversedString = new String(charArray); System.out.println(reversedString); ``` Output: ``` !dlroW ,olleH ``` In this alternative approach, we convert the original string into a character array using the toCharArray() method. Then, we create two pointers, `start` and `end`, pointing to the first and last elements of the array, respectively. We swap the characters in the array using these pointers until they meet in the middle. Finally, we construct a new string from the modified character array and print the reversed string. Q3. Does the reverse() method modify the original string? No, the reverse() method does not modify the original string. It creates a new string with the characters in reverse order, leaving the original string unchanged. This is because the String class in Java is immutable, meaning its value cannot be modified after it is created. Q4. Can the reverse() method reverse non-alphabetic characters? Yes, the reverse() method can reverse any characters, whether they are alphabetic, numeric, or special characters. It handles characters of any type and order in the same way. Q5. Are there any limitations to the reverse() method? The reverse() method does not have any specific limitations. However, it is important to note that as the length of the input string increases, the time complexity of the method also increases. It is generally efficient, but for very large strings, alternative approaches might be more suitable to optimize performance. In conclusion, the reverse(String Java) method is an effective way to reverse the characters in a given string in Java. It allows developers to manipulate strings efficiently and achieve desired results. Whether used with immutable strings or mutable character arrays, this method provides a straightforward solution to the problem of string reversal.
Images related to the topic reverse a string c#
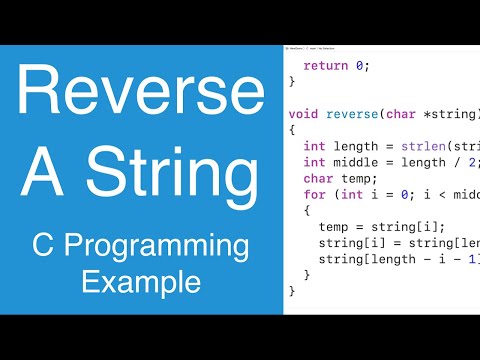
Article link: reverse a string c#.
Learn more about the topic reverse a string c#.
- Reverse a String in C – Javatpoint
- C Program to Reverse A String Using Different Methods
- Reverse a String in C – Scaler Topics
- C program to reverse a string – Programming Simplified
- Reverse a String in C – W3schools
- Reverse a String in C – Coding Ninjas
- strrev() function in C – GeeksforGeeks
- Reverse String in C++ – DigitalOcean
- Program to Reverse a String in C – Studytonight
- Program of Reverse string in C – PrepInsta
See more: https://nhanvietluanvan.com/luat-hoc