Syntaxerror: Non-Default Argument Follows Default Argument
Introduction
In programming, default arguments are a valuable feature that allows you to assign a default value to a function parameter if no argument is provided when calling the function. This saves developers time by reducing the need to explicitly specify values for all parameters in every function call. However, there are certain rules and limitations when it comes to using default arguments in programming languages, such as Python. One common syntax error that programmers may encounter is the “non-default argument follows default argument” error. In this article, we will explore this error, its causes, and how to avoid it.
Explanation of the Syntax Error: Non-Default Argument Follows Default Argument
The “non-default argument follows default argument” error occurs when a function definition includes a non-default argument after a default argument. In other words, any argument that does not have a default value must come before any arguments that have default values in the function signature.
This error is raised because the Python interpreter cannot reliably determine which arguments correspond to which parameters when calling the function. It is important to note that this error is specific to Python and may not exist in other programming languages.
Examples Illustrating the Syntax Error
Let’s examine some code examples to better understand this error. Consider the following function definition:
“`python
def greet(name=”John”, age):
print(“Hello, ” + name + “! You are ” + str(age) + ” years old.”)
“`
In this example, the parameter “name” is assigned a default value of “John”, while “age” does not have a default value. When attempting to define this function, a syntax error will be raised due to the non-default argument “age” following the default argument “name”.
Common Scenarios That Lead to This Error
There are several common scenarios that can lead to the “non-default argument follows default argument” error. Some of these include:
1. Incorrect order of arguments: As previously mentioned, the error occurs when a non-default argument follows a default argument. This often happens when developers accidentally rearrange the order of arguments when defining their functions.
2. Forgetting to provide default values: Another common mistake is forgetting to assign default values to all the function parameters. If you attempt to define a function where a non-default argument follows a default argument and you have not provided default values for all the parameters, the error will be triggered.
3. Ignoring function signature rules: Python has specific rules for defining function signatures, and non-compliance with these rules can lead to syntax errors. Notably, the rule that no non-default arguments should follow default arguments is one such rule.
Recommendations and Best Practices to Avoid the Error
To avoid encountering the “non-default argument follows default argument” error, consider the following recommendations and best practices:
1. Maintain the correct order of arguments: Ensure that all non-default arguments are placed before any default arguments when defining your functions. This will prevent the error from occurring.
2. Provide default values for all arguments: Make sure to assign default values to all function parameters that you intend to be optional. This will help avoid the error and ensure that your function behaves as expected when default values are not explicitly provided.
3. Regularly review and test function signatures: Take the time to review and test your function signatures to ensure they adhere to the rules and conventions of the programming language you are using. By being diligent in this regard, you can catch any potential syntax errors, including the “non-default argument follows default argument” error, before they become problematic.
Conclusion
In conclusion, the “non-default argument follows default argument” error is a syntax error that occurs when a non-default argument follows a default argument in a function definition. This error is specific to Python and can lead to confusion and unexpected behavior when calling functions. By following best practices and adhering to the rules of function signatures, developers can avoid this error and write more reliable code.
FAQs
Q: What is the difference between positional argument and keyword argument?
A: Positional arguments are arguments provided to a function based on their position or order in the function call. Keyword arguments, on the other hand, are arguments provided to a function based on their name, allowing developers to explicitly specify which parameter each argument corresponds to.
Q: What are default parameters in Python?
A: Default parameters, also known as default arguments, are function parameters that are assigned a default value in the function signature. If no argument is provided for a parameter with a default value, the function will use the default value instead.
Q: What does the error message “Got multiple values for argument” mean?
A: The “Got multiple values for argument” error occurs when a function is called with multiple values assigned to the same argument. This is often caused by incorrect function call syntax or passing arguments in the wrong order.
Python : Syntaxerror: Non-Default Argument Follows Default Argument
Keywords searched by users: syntaxerror: non-default argument follows default argument Positional argument follows keyword argument, Default parameter Python, Got multiple values for argument
Categories: Top 41 Syntaxerror: Non-Default Argument Follows Default Argument
See more here: nhanvietluanvan.com
Positional Argument Follows Keyword Argument
In Python programming, the order and arrangement of arguments play a crucial role in defining the behavior and functionality of a function. One common error that many developers encounter is the “Positional argument follows keyword argument” exception. This error occurs when a positional argument is placed after a keyword argument in a function call. To avoid this error, it is important to understand the basics of positional and keyword arguments, as well as their differences and best practices.
Positional arguments are the most fundamental type of arguments in Python. They are defined by their position or order in a function call. When a function is defined, its parameters are listed in a specific order. These parameters can be assigned default values, or they can expect values to be passed when invoking the function. When calling a function, positional arguments must be provided in the same order as they are defined in the function signature.
On the other hand, keyword arguments are defined by their name. Instead of relying on their position, keyword arguments are provided with a name followed by a value in the function call. Unlike positional arguments, keyword arguments can be provided in any order as long as their names are correctly specified. This flexibility makes them useful when dealing with functions that have a large number of parameters, as it allows you to explicitly state which argument corresponds to which value without relying on position alone.
The “Positional argument follows keyword argument” error occurs when a positional argument is placed after a keyword argument in a function call. To illustrate this, let’s consider the following function:
“`
def greet(name, age):
print(f”Hello {name}, you are {age} years old!”)
“`
If we want to call this function using keyword arguments, we can simply provide the parameter names along with their corresponding values:
“`
greet(name=”Alice”, age=25)
“`
This will correctly output: “Hello Alice, you are 25 years old!”.
However, if we mistakenly provide a positional argument after a keyword argument:
“`
greet(age=25, “Alice”)
“`
Python will raise the “Positional argument follows keyword argument” exception. In this case, we should either provide all arguments as positional arguments or reorder the arguments to ensure positional arguments precede keyword arguments:
“`
greet(“Alice”, age=25)
“`
Now, let’s address some frequently asked questions regarding the “Positional argument follows keyword argument” error:
Q: What is the reason behind this exception?
A: Python enforces a strict ordering of arguments to avoid ambiguity. Placing a positional argument after a keyword argument can lead to confusion and incorrect results, hence the interpreters’ behavior to raise an exception.
Q: How can I prevent this error from occurring?
A: To avoid this error, ensure that all positional arguments precede any keyword arguments in a function call. Alternatively, you can explicitly provide all arguments using either positional or keyword style.
Q: Can I use only keyword arguments for function calls?
A: Yes, you can use only keyword arguments, as long as the function’s signature allows for it. However, it is important to note that the use of only keyword arguments can make the code less readable and less intuitive for other developers.
Q: Is this error specific to Python?
A: No, this error is not exclusive to Python. Some other programming languages may exhibit similar behavior or raise similar exceptions for misplaced arguments when using keywords and positional parameters.
Q: How can I debug or trace this error?
A: Debugging this error is relatively straightforward. Check the function call where the error occurs and ensure all positional arguments are placed before any keyword arguments. Modifying the order of arguments or explicitly providing names and values can resolve the issue.
In conclusion, understanding the concept of positional and keyword arguments is essential for writing efficient and error-free Python code. The “Positional argument follows keyword argument” exception highlights the importance of providing arguments in the correct order. By following best practices and being aware of this error, developers can write more robust and maintainable code.
Default Parameter Python
Introduction
In Python, functions play a crucial role in organizing and reusing code. They allow you to encapsulate a set of actions into a reusable block of code, which can be called multiple times with different arguments. One feature that makes Python functions more powerful is the ability to define default parameter values. This enables you to provide a default value for a parameter, which is used when the argument for that parameter is not specified during function call. In this article, we will explore default parameters in Python in depth and showcase various ways of using them effectively.
Understanding Default Parameters
To comprehend default parameters, let’s first delve into the anatomy of a function. In Python, a function is defined using the `def` keyword, followed by the function name and a set of parentheses. Inside the parentheses, you can specify the function’s parameters, which act as placeholders for values that will be passed to the function when called. Consider the following simple function that adds two numbers:
“`python
def add_numbers(a, b):
return a + b
“`
In this case, `a` and `b` are the parameters of the `add_numbers` function. When you call this function, you need to provide arguments that match the order and number of the parameters. For example, `add_numbers(3, 5)` will return `8`.
Now, let’s say you want to modify the `add_numbers` function so that the second number can have a default value of 2. To achieve this, you can define the parameter `b` with a default value of 2:
“`python
def add_numbers(a, b=2):
return a + b
“`
With this modification, if you call the function without passing any arguments for `b`, it will automatically use the default value of 2. For instance, `add_numbers(3)` will now return `5`.
Benefits of Default Parameters
Default parameters provide flexibility and convenience when working with functions. Here are some key benefits they offer:
1. Avoiding errors and providing flexibility: By providing default values, you make function calls simpler, as users don’t need to remember or specify every parameter. If arguments are not provided, default values ensure the function still executes without errors.
2. Enhanced readability: Default parameters can improve the readability of functions by reducing clutter when calling them. When a function has several parameters, specifying default values allows you to focus on the required arguments only.
3. Enabling optional functionality: Default parameters allow you to implement optional functionality in your functions. You can define parameters that provide additional options or modify the behavior of the function when needed.
Advanced Techniques for Default Parameters
In addition to simple default parameter values, Python provides advanced techniques to enhance their usage. Let’s explore some of these techniques:
1. Using mutable objects as default values: Default parameter values are evaluated only once when a function is defined, which can lead to unexpected behavior when mutable objects are involved. Consider the following example:
“`python
def append_to_list(value, my_list=[]):
my_list.append(value)
return my_list
“`
At first glance, you might expect that calling `append_to_list(1)` and then `append_to_list(2)` would return `[1, 2]`. However, Python remembers and reuses the same list object that was created when the function was defined. As a result, calling `append_to_list(2)` would actually return `[1, 2, 2]`, as the default list object is modified. To mitigate this issue, a common practice is to use `None` as the default parameter value and then assign the actual default value inside the function:
“`python
def append_to_list(value, my_list=None):
if my_list is None:
my_list = []
my_list.append(value)
return my_list
“`
This ensures that a new list is created each time the function is called, eliminating any potential issues with mutable default objects.
2. Usage of complex default values: Default parameter values in Python are not limited to simple data types like numbers or strings. We can also use more complex objects as default values. Take a look at this example:
“`python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def create_person(name, age=Person(“John Doe”, 25)):
return Person(name, age)
“`
In this case, the `create_person` function has a default `age` parameter, which is an instance of the `Person` class. If no `age` argument is provided, the default person object is used. However, if an `age` argument is passed, it overrides the default value.
FAQs
Q1: Can I specify default parameters for some arguments but not others?
A1: Yes, you can specify default parameters for any number of arguments. Just remember that the arguments with default values should appear after the ones without default values in the function’s parameter list.
Q2: Can I change the default parameter values at runtime?
A2: No, the default parameter values are evaluated only once when the function is defined. If you need to change the default values, you can redefine the function.
Q3: What is the best practice for using default parameters?
A3: Default parameters should be used judiciously and only when they enhance the clarity and usability of your functions. Choose default values that make sense for most use cases and avoid mutable default objects to prevent any unexpected behavior.
Q4: Can I mix positional and keyword arguments when using default parameters?
A4: Yes, you can mix positional and keyword arguments. Positional arguments are matched based on their order, while keyword arguments are matched based on their names. Mixing them allows greater flexibility when calling functions.
Conclusion
Default parameters in Python allow you to define default values for function parameters, providing flexibility and simplifying function calls. By using default parameters effectively, you can enhance the readability of your code and enable optional functionality. However, be mindful of potential pitfalls with mutable default objects and choose default values wisely. Understanding and utilizing default parameters can greatly improve your ability to write efficient and reusable functions in Python.
Got Multiple Values For Argument
In Python, the number of function arguments is typically fixed and defined when the function is declared. However, there may be situations where we need to pass a varying number of arguments to a function. Python provides a flexible way to handle such cases using two special syntaxes: *args and **kwargs.
The *args syntax allows a function to accept any number of positional arguments. These arguments are packed into a tuple, which can then be accessed within the function using the args variable. On the other hand, the **kwargs syntax allows a function to accept any number of keyword arguments. These arguments are packed into a dictionary, which can be accessed within the function using the kwargs variable.
Now, let’s take a look at an example where the “Got multiple values for argument” error can occur:
“`python
def calculate_sum(a, b, *args):
total = a + b
for num in args:
total += num
return total
numbers = [1, 2, 3, 4, 5]
result = calculate_sum(*numbers)
print(result)
“`
In this example, we have a function called `calculate_sum` that takes two positional arguments `a` and `b` and also accepts any number of additional arguments using the *args syntax. We have a list of numbers and are attempting to pass them as arguments using the unpacking operator (*).
However, running this code will result in the following error message:
“`
TypeError: calculate_sum() got multiple values for argument ‘a’
“`
This error occurs because Python is interpreting the first element of the `numbers` list as the value for the `a` argument and the remaining elements as additional arguments, which is not the intended behavior.
To resolve this error, we need to modify the function call to explicitly specify the names of the arguments being passed:
“`python
result = calculate_sum(*numbers[:2], *numbers[2:])
“`
In this updated code, we use slicing to split the `numbers` list into two parts: the first two elements as values for `a` and `b`, and the remaining elements as additional arguments using the * operator. This way, the function call is properly structured, and the error is avoided.
FAQs (Frequently Asked Questions):
Q: How can I fix the “Got multiple values for argument” error in Python?
A: To fix this error, you need to ensure that the function call is structured correctly, especially when using *args or **kwargs syntax. Explicitly specify the names of the arguments being passed to avoid Python interpreting them incorrectly.
Q: Can I pass a list as an argument to a function in Python?
A: Yes, you can pass a list as an argument by using the unpacking operator (*) to unpack the elements of the list as separate arguments. However, be cautious when using *args because it treats all elements as separate positional arguments.
Q: What should I do if I need to pass a varying number of arguments to a function?
A: In Python, you can utilize *args or **kwargs syntax to handle a varying number of arguments. Use *args when you want to pass a varying number of positional arguments and **kwargs for keyword arguments. By doing this, you can provide flexibility while calling the function and avoid “Got multiple values for argument” errors.
Q: Is there a limit to the number of arguments I can pass to a function in Python?
A: In theory, there is no fixed limit to the number of arguments you can pass to a function. However, keep in mind that passing a large number of arguments may affect the performance and readability of your code. It is advisable to consider alternative approaches, such as using data structures like lists or dictionaries, when dealing with a large number of arguments.
In conclusion, encountering the “Got multiple values for argument” error in Python is a common challenge for programmers. By understanding the concept of multiple values for argument, utilizing the *args and **kwargs syntax, and ensuring proper structuring of function calls, you can overcome this error efficiently. Remember to be mindful of the argument order and type in order to avoid such errors and write clean, error-free code.
Images related to the topic syntaxerror: non-default argument follows default argument
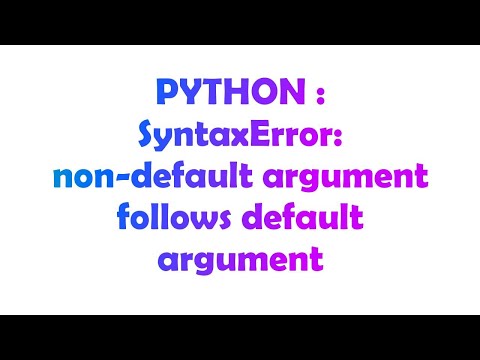
Found 43 images related to syntaxerror: non-default argument follows default argument theme
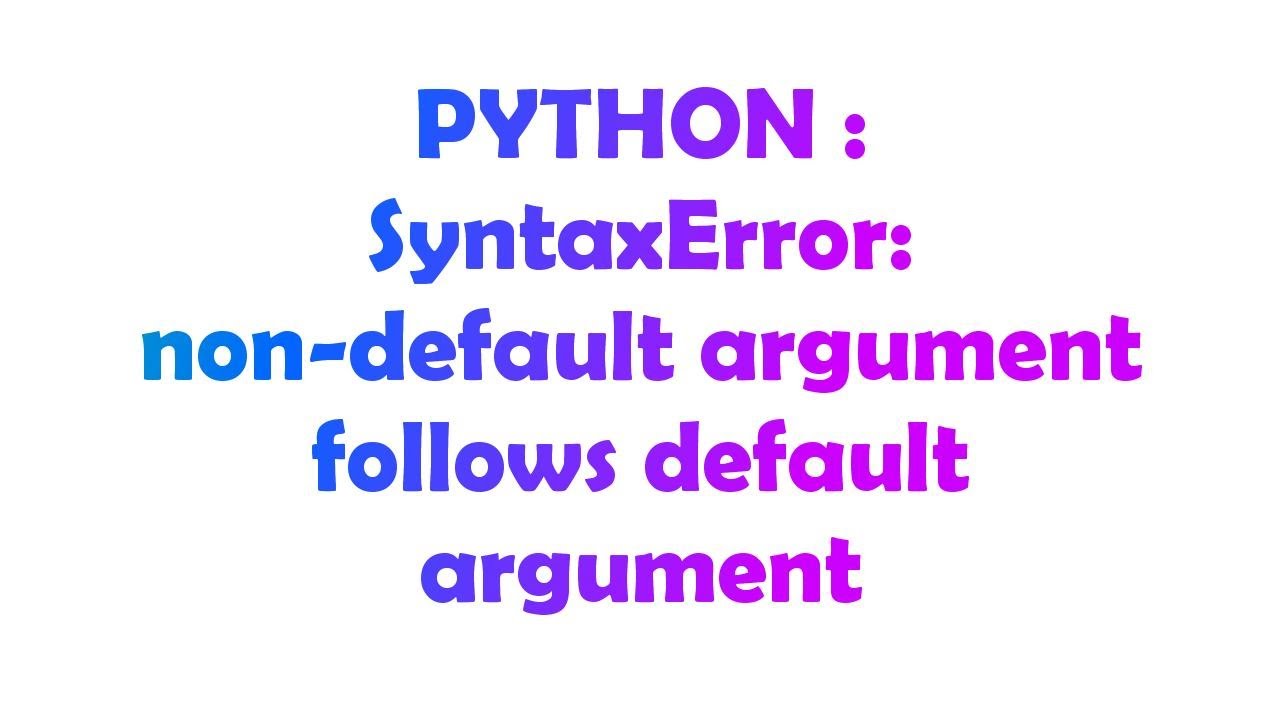
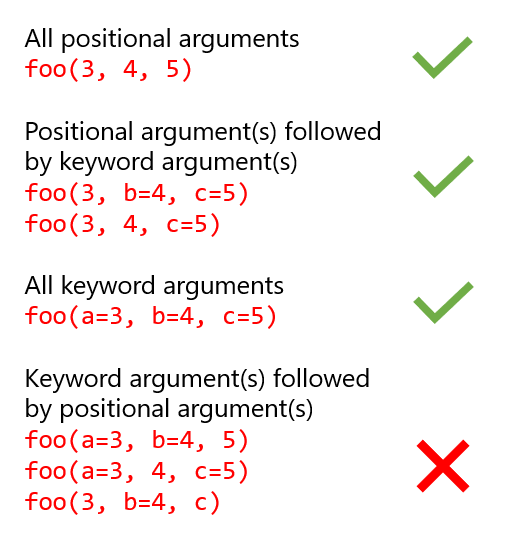

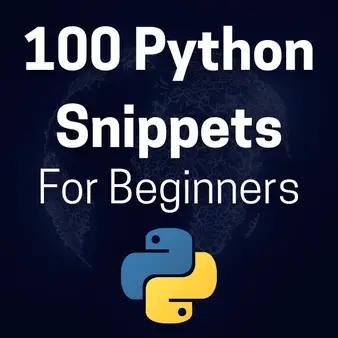
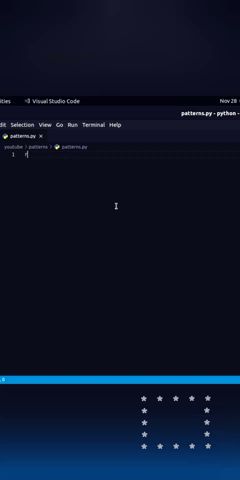
![Solved] SyntaxError: non-default argument follows default argument - Clay-Technology World Solved] Syntaxerror: Non-Default Argument Follows Default Argument - Clay-Technology World](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2021/03/cropped-output.png?fit=350%2C350&ssl=1)
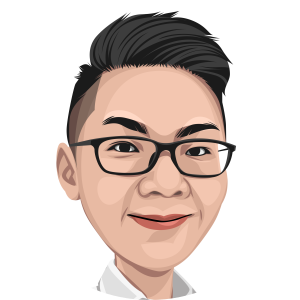
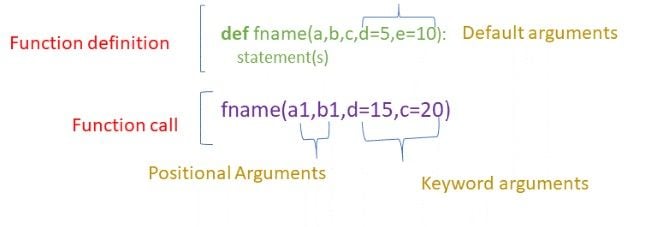
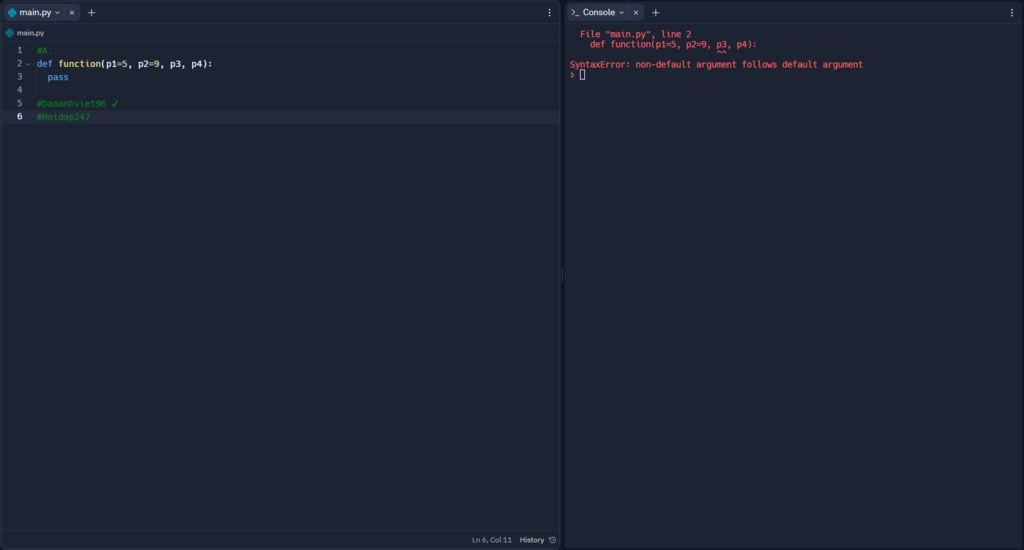
![Solved][Python] SyntaxError: non-default argument follows default argument Solved][Python] Syntaxerror: Non-Default Argument Follows Default Argument](https://img1.daumcdn.net/thumb/C176x176/?fname=https://blog.kakaocdn.net/dn/cz06uj/btqEspU1TLH/vttwhkkmaU5iJynx83z991/img.png)
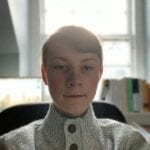
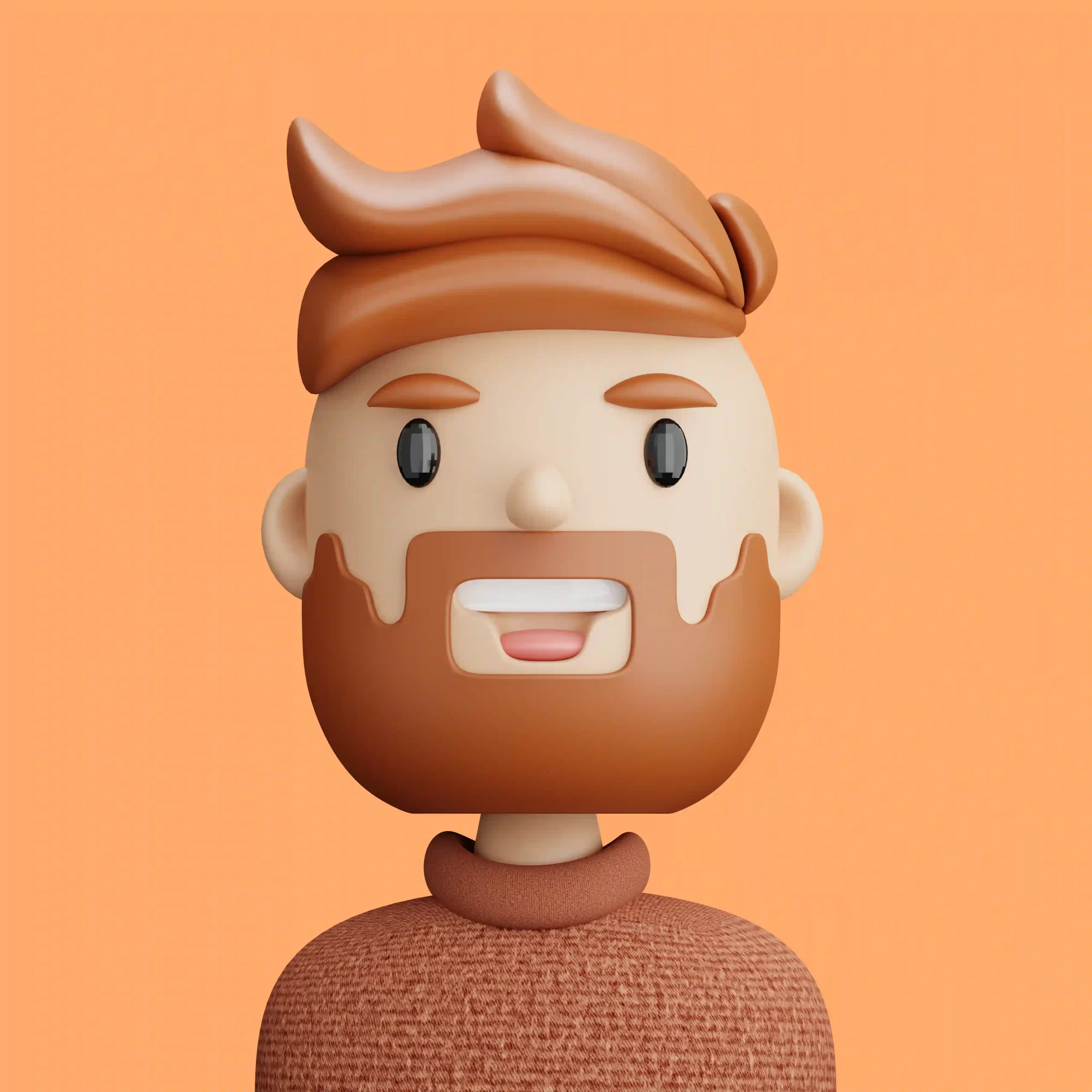
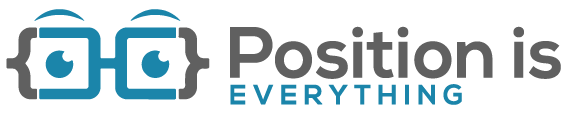

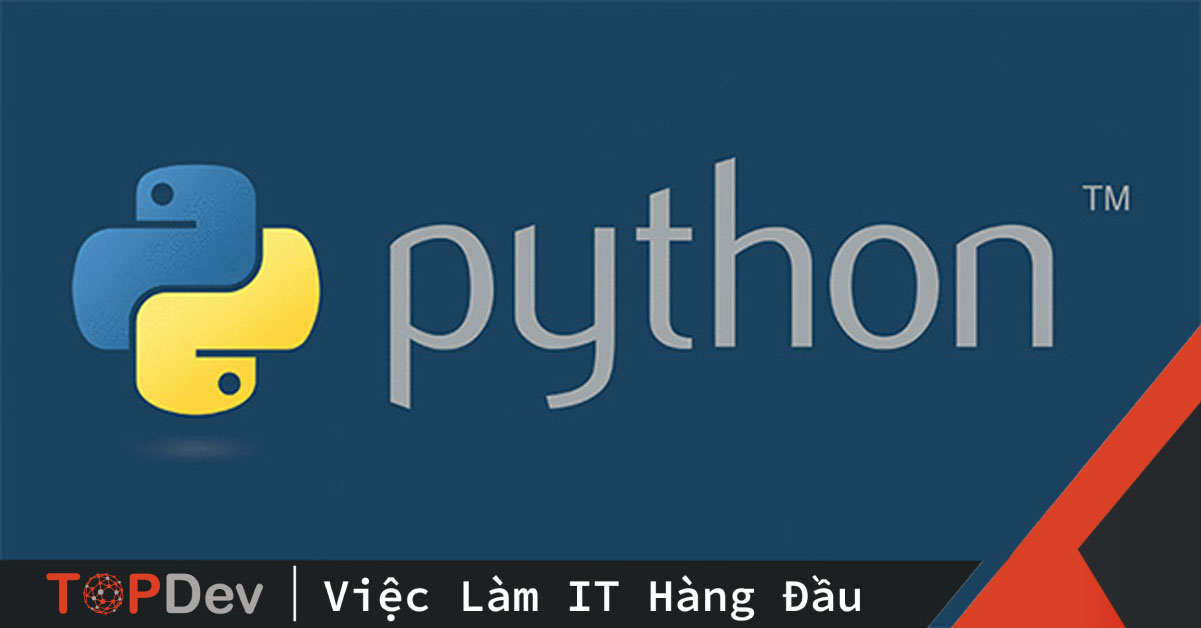


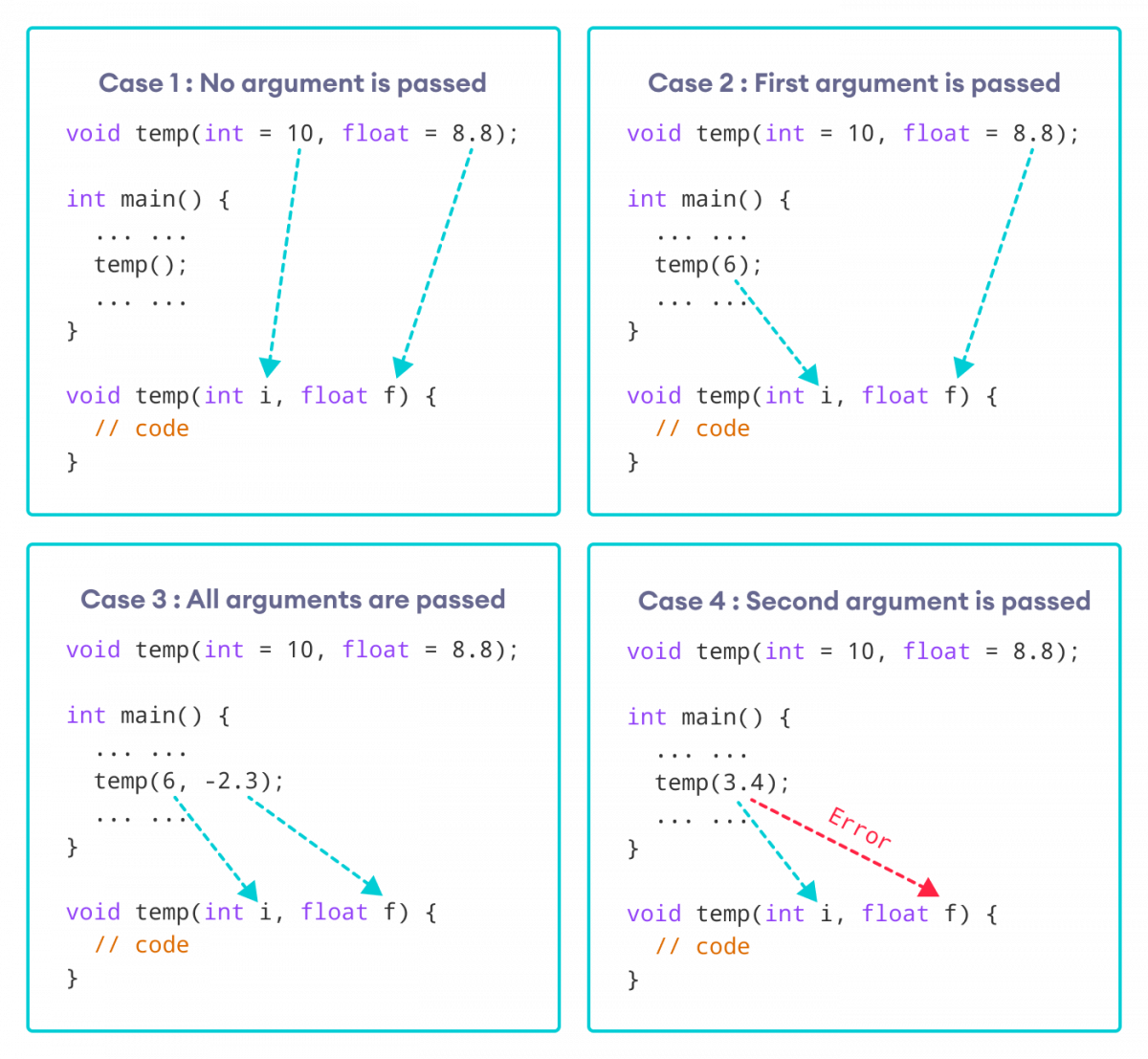
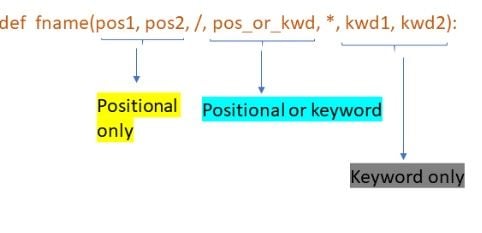
Article link: syntaxerror: non-default argument follows default argument.
Learn more about the topic syntaxerror: non-default argument follows default argument.
- How to Resolve the “SyntaxError: Non-Default Argument …
- SyntaxError: non-default argument follows … – bobbyhadz
- SyntaxError: non-default argument follows default argument
- Python SyntaxError: non-default argument follows default
- 4 Ways to Fix Python SyntaxError: Non-Default Argument …
- [Solved]: non-default argument follows default argument
- [Solved] SyntaxError: non-default argument follows default …
- “SyntaxError: non-default argument follows default … – GitHub
- Issue 47054: “SyntaxError: non-default argument follows …
See more: nhanvietluanvan.com/luat-hoc