Typescript Double Exclamation Mark
In TypeScript, the double exclamation mark (!!) is known as the non-null assertion operator. It is used to assert that a value is not null or undefined, even if the TypeScript compiler cannot verify its existence at compile-time. This operator is used to tell the compiler that a certain value will always be present, allowing developers to access properties or methods without the need for explicit null or undefined checks.
The purpose of the double exclamation mark in TypeScript
The main purpose of the double exclamation mark in TypeScript is to provide developers with a way to assert that a value is not null or undefined, even if the compiler is unable to prove it at compile-time. This can be useful in scenarios where the developer is confident that a value will be present and wants to avoid unnecessary null or undefined checks.
Usage examples of the double exclamation mark in TypeScript
Here are a few usage examples of the double exclamation mark in TypeScript:
1. Accessing properties or methods:
“`typescript
const element = document.querySelector(‘.element’)!;
element.classList.add(‘active’);
“`
2. Initializing variables inside a function:
“`typescript
function fetchData(): string {
let data: string;
// Code to fetch data and assign it to the ‘data’ variable
return data!;
}
“`
3. Using with optional chaining:
“`typescript
const user = getUser()!;
const email = user?.email!;
“`
Difference between the double exclamation mark and other operators in TypeScript
The double exclamation mark operator is often misunderstood and mistaken for the negation operator or the triple exclamation mark operator. However, it is important to note that the double exclamation mark serves a completely different purpose.
The negation operator (!) is used for boolean negation, whereas the double exclamation mark is used for asserting non-nullability.
The triple exclamation mark (!!!) is not a valid operator in TypeScript and does not have any specific meaning. It is often a typing mistake and should be avoided.
Common misconceptions about the double exclamation mark in TypeScript
One of the common misconceptions about the double exclamation mark in TypeScript is that it guarantees non-nullability of a value. However, it is important to note that the double exclamation mark is only a way to assert non-nullability, and it does not actually prevent a value from being null or undefined at runtime.
Another misconception is that the use of the double exclamation mark eliminates the need for proper null or undefined checks. While the operator can help in certain scenarios, it should not be relied upon as a substitute for proper error handling and defensive programming practices.
Best practices when using the double exclamation mark in TypeScript
When using the double exclamation mark in TypeScript, it is important to follow some best practices:
1. Use the double exclamation mark sparingly and only when you are certain that a value will always be present. Overusing the operator can lead to code that is more error-prone and harder to maintain.
2. Combine the double exclamation mark with proper error handling and defensive programming practices. It is always a good practice to perform null or undefined checks, especially when dealing with external data sources or asynchronous operations.
3. Document the use of the double exclamation mark in your codebase to make it clear that the assertion is intentional and not a mistake. This helps other developers understand the reasoning behind the non-null assertions.
Benefits of using the double exclamation mark in TypeScript
The use of the double exclamation mark in TypeScript offers a few benefits to developers:
1. It allows for more concise and readable code by eliminating the need for explicit null or undefined checks in certain scenarios. This can lead to cleaner code and improved code maintainability.
2. It allows developers to express their intent more clearly by asserting non-nullability. This can make the code more self-explanatory and reduce the chances of introducing bugs related to null or undefined values.
3. It enables the TypeScript compiler to perform type inference more accurately in certain scenarios, as it can deduce that a value is not null or undefined after the assertion.
Potential pitfalls of using the double exclamation mark in TypeScript
While the double exclamation mark can be a useful tool in certain scenarios, there are also potential pitfalls to be aware of:
1. It can lead to runtime errors if the asserted value turns out to be null or undefined. This can happen if the assertion is incorrect or if the underlying data changes unexpectedly.
2. It can mask underlying issues with null or undefined values by allowing code execution to continue even when a value is missing. This can make debugging and error tracking more challenging.
3. It can create a false sense of security if used excessively or without proper error handling practices. It is important to remember that the double exclamation mark is not a foolproof way to ensure non-nullability and should always be used in conjunction with appropriate checks and error handling.
FAQs:
Q: Can I use the double exclamation mark with any type of value?
A: The double exclamation mark can be used with any type of value, not just objects or variables. However, it is important to note that using the operator with a value of a type that can be nullable or undefined can lead to runtime errors.
Q: Are there any alternatives to the double exclamation mark for asserting non-nullability?
A: Yes, TypeScript provides alternative ways to assert non-nullability, such as the as operator and type assertions. These options can be used in scenarios where the use of the double exclamation mark is not appropriate or preferred.
Q: Does the double exclamation mark change the type of a value?
A: No, the double exclamation mark does not change the type of a value. It is purely a compiler-level assertion that informs TypeScript that a value is not null or undefined.
Q: Can the double exclamation mark be used in JavaScript?
A: No, the double exclamation mark is a TypeScript-specific operator and is not part of the JavaScript language. However, there are similar operators in JavaScript, such as the exclamation mark (!) used for boolean negation.
Q: Is it good practice to use the double exclamation mark with all variables?
A: No, it is not recommended to use the double exclamation mark with all variables. It should only be used when there is a strong assurance that a value will always be present. Overusing the operator can lead to code that is harder to maintain and debug.
What Are Double Shots In Javascript
What Does 2 Exclamation Mark Mean In Typescript?
TypeScript is a strongly typed superset of JavaScript, commonly used for building scalable and robust applications. One of the features that often confuses developers new to TypeScript is the use of exclamation marks. In TypeScript, a single exclamation mark “!” is called the non-null assertion operator, but what does it mean when we see two exclamation marks “!!” in the code? In this article, we will delve into this topic and explore the significance of using two exclamation marks in TypeScript.
Understanding the Non-null Assertion Operator (!)
Before diving into the use of two exclamation marks, it is crucial to grasp the concept of the non-null assertion operator, denoted by a single exclamation mark. In TypeScript, variables have types that can be either explicitly defined or inferred by the compiler. When a variable is defined without an explicit type annotation or without an initial value, its type is considered to be “undefined” or “null.”
The non-null assertion operator “!” is used to explicitly inform the TypeScript compiler that a variable is guaranteed to be non-null and can be safely accessed. By using this operator, developers are effectively telling TypeScript to temporarily suspend the nullability checks and trust that the variable will not be null or undefined.
The Double Exclamation Marks (!!)
Now that we understand the non-null assertion operator, we can proceed to explore the meaning of using two exclamation marks. In TypeScript, the double exclamation marks “!!” are used to convert a value to its corresponding boolean representation explicitly. This operation is often referred to as the “double-negation trick” or “double-bang” in JavaScript. In other words, “!!” casts a truthy or falsy value to its boolean equivalent.
For example, consider the following code snippet:
“`typescript
const value: string = “Hello TypeScript”;
const booleanValue: boolean = !!value;
console.log(booleanValue); // Output: true
“`
In this example, the variable `value` is assigned a string value. By using `!!value`, we convert the truthy string value to its boolean representation, resulting in `booleanValue` being assigned the value `true`. This can be useful when we need to explicitly check if a value is truthy or falsy.
Common Use Cases
The double exclamation marks are often used in conditional statements and expressions where boolean values are expected. Here are some common use cases:
1. Conditionals: When evaluating conditions that rely on the truthiness or falsiness of a value, the double exclamation marks can be used to ensure that the value is interpreted correctly. For example:
“`typescript
const myVariable = “Some value”;
if (!!myVariable) {
console.log(“Variable is truthy”);
} else {
console.log(“Variable is falsy”);
}
“`
2. Type Conversion: By using double exclamation marks, we can explicitly convert a value to its boolean equivalent. This can be handy when dealing with various data types and checking for their existence. For instance:
“`typescript
const someValue: any = null;
const isValid: boolean = !!someValue;
console.log(isValid); // Output: false
“`
Frequently Asked Questions (FAQs)
Q1. Why do we need to use two exclamation marks to convert a value to a boolean? Can’t we use a single exclamation mark?
The single exclamation mark (!) in TypeScript is reserved for the non-null assertion operator. Therefore, using a single exclamation mark would indicate a non-null assertion rather than a conversion to a boolean.
Q2. What happens if we apply the double exclamation marks to a boolean value?
If the value is already a boolean, applying double exclamation marks would have no effect, as the value is already in the required boolean form. The double exclamation marks are primarily used to convert truthy or falsy values to boolean.
Q3. Are there any performance implications when using double exclamation marks?
No, there are no performance implications associated with the use of double exclamation marks. The operation of converting a value to a boolean using “!!” is a simple and lightweight process.
Q4. Can we achieve the same result using the Boolean() function?
Yes, using the Boolean() function is an alternative approach to achieve the same result as using double exclamation marks. However, the double exclamation marks are often preferred due to their brevity and readability.
Q5. Are there any potential pitfalls to be aware of when using double exclamation marks?
One potential pitfall is when working with numbers. In JavaScript, the number 0 is considered falsy, but any nonzero number is considered truthy. By using “!!” on a numeric value, we effectively convert any nonzero value to boolean true.
Conclusion
In TypeScript, the double exclamation marks “!!” are used to convert a value to its corresponding boolean representation. This operation can be handy when working with conditions, type conversion, and determining the truthiness or falsiness of a value. By understanding the non-null assertion operator and the concept of the double exclamation marks, developers can confidently leverage these features to write cleaner and more expressive code in TypeScript.
Can You Use Double Exclamation Mark?
In the world of punctuation, the exclamation mark is known for its ability to add emphasis and excitement to a sentence. But have you ever wondered if it’s acceptable to go beyond using just one? Yes, we’re talking about the double exclamation mark. Can you use it? Is it considered correct in English grammar? Let’s delve into this interesting topic and find out.
The use of a single exclamation mark already carries a significant amount of emphasis. It effectively conveys surprise, excitement, or strong emotions. However, some situations call for an even greater level of intensity. This is where the double exclamation mark comes into play.
Using two exclamation marks consecutively is indeed possible in English writing, but it is important to understand when and how to use them appropriately. While it may seem tempting to sprinkle your text with these powerful punctuation marks, overusing them can diminish their impact and make your writing appear amateurish or even unprofessional. Therefore, discretion is key.
So, when is it appropriate to use a double exclamation mark? The most common situation is in informal or casual writing, such as personal emails, text messages, or social media posts. Sometimes, one exclamation mark may not fully express the extreme level of excitement or intensity that you want to convey. Adding a second one can help emphasize your emotions and ensure that the recipient truly understands the energy behind your message.
Another scenario where double exclamation marks can be used effectively is in creative or expressive writing. Poets and authors often utilize them to convey heightened emotions or to create a sense of urgency within their narratives and dialogues. In this context, they can enhance the overall impact and resonate with the reader on a deeper level.
It’s worth mentioning that in more formal writing, such as academic papers, business documents, or professional correspondences, the use of double exclamation marks is generally discouraged. These contexts require a more restrained and objective tone, where an excessive number of exclamation marks may appear unprofessional or even inappropriate. If you are unsure about whether to use them, it is generally safer to err on the side of caution and stick to a single exclamation mark.
To help clarify any lingering doubts about using double exclamation marks, let’s address some frequently asked questions:
1. Can I use multiple exclamation marks for even greater emphasis?
While it is technically possible to use three or more exclamation marks consecutively, it is generally not recommended. Using multiple exclamation marks can make your writing appear overly enthusiastic or even childish. Stick to one or two to ensure your message is appropriately emphasized.
2. Should I use double exclamation marks in professional emails?
Unless you have an established closer relationship with the recipients or the content requires it, it is best to avoid using double exclamation marks in professional emails. Stick to a single exclamation mark or find alternative ways to convey your excitement or urgency in a more formal manner.
3. Can I use double exclamation marks in social media captions or comments?
Social media platforms, with their relaxed and casual environment, are more forgiving when it comes to using double exclamation marks. They are a great place to utilize this punctuation mark to amplify your excitement and grab attention. Just remember to use them sparingly to avoid diluting their effect.
In conclusion, the use of double exclamation marks is permissible in certain contexts of English writing, such as informal communication or creative writing. They can add extra emphasis, excitement, or urgency to your message when used appropriately. However, in formal or professional settings, it is best to limit yourself to a single exclamation mark, as overusing them can undermine the credibility of your writing. In the end, mastering the art of exclamation mark usage comes down to understanding your audience, purpose, and maintaining a balanced approach to punctuation. So go forth, use those double exclamation marks wisely, and let your writing pop with enthusiasm!
Keywords searched by users: typescript double exclamation mark Double exclamation mark JavaScript, Exclamation mark in TypeScript, Triple exclamation mark JavaScript, JavaScript double, Exclamation mark JavaScript, non-null assertion operator, After variable typescript, Question mark in TypeScript
Categories: Top 28 Typescript Double Exclamation Mark
See more here: nhanvietluanvan.com
Double Exclamation Mark Javascript
JavaScript is a versatile programming language that offers a plethora of features and functionalities to developers. One such feature is the double exclamation mark, also known as the “not-not” operator. This operator is often overlooked or misunderstood, but it can be a powerful tool in certain situations. In this article, we will delve into the details of the double exclamation mark in JavaScript, its usage, and its benefits.
Understanding the Double Exclamation Mark
The double exclamation mark is a unary operator that converts a value to its Boolean equivalent. It does this by applying the logical NOT operator (!) twice in succession. As a result, the final outcome is either true or false, representing the truthiness or falsiness of the original value.
Syntax and Usage
To apply the double exclamation mark operator, place it before the value you want to convert. For instance:
“`
let value = 0;
let result = !!value;
console.log(result); // Output: false
“`
In the example above, the value of 0 is first converted to false using the logical NOT operator. Then, the second NOT operator is applied, resulting in the ultimate value of false. This technique is particularly useful when you need to quickly convert any value to its truthiness or falsiness in a concise manner.
Benefits of the Double Exclamation Mark
The double exclamation mark can be advantageous in several scenarios. Let’s explore some of its key benefits:
1. Converting to Boolean: One of the primary use cases of the double exclamation mark is converting a value to its Boolean equivalent. It evaluates whether a value is truthy or falsy in a concise way without using conditional statements. For example:
“`
let value = “Hello”;
let isTruthy = !!value;
console.log(isTruthy); // Output: true
“`
In this case, the value “Hello” is a non-empty string, which is truthy. By applying the double exclamation mark, we obtain the Boolean value true.
2. Short-circuiting: The double exclamation mark can be employed to short-circuit conditional expressions. By converting a value to its Boolean form, you can control the execution of subsequent statements based on the outcome. Consider the following example:
“`
function printMessage(message) {
message && console.log(message);
}
let myMessage = “Hello, world!”;
printMessage(!!myMessage); // Output: Hello, world!
“`
In this case, the printMessage function logs the message only if it evaluates to true. By using the double exclamation mark, we ensure that the message is only printed when it has a valid value. This technique is especially useful when dealing with optional parameters.
3. Type coercion: JavaScript is known for its dynamic typing, which means variables can change their types during runtime. The double exclamation mark helps in type coercion by explicitly converting a value to a Boolean type. It allows you to enforce certain behaviors based on the truthiness or falsiness of a value.
FAQs
Q1. Can the double exclamation mark be used on non-Boolean values?
Yes, the double exclamation mark can be used on any type of value, not just Booleans. It will convert the value to its Boolean equivalent. For example:
“`
let value = “JavaScript”;
let isTruthy = !!value;
console.log(isTruthy); // Output: true
“`
Q2. How does the double exclamation mark handle undefined or null values?
Undefined and null values are converted to false when the double exclamation mark is applied. For instance:
“`
let value1 = undefined;
let value2 = null;
console.log(!!value1); // Output: false
console.log(!!value2); // Output: false
“`
Q3. Can the double exclamation mark be used multiple times in a row?
Technically, you can chain the double exclamation mark multiple times, but it only returns true or false. The second application of the operator does not provide any additional benefit. It is usually unnecessary and redundant.
Q4. Is there any performance impact of using the double exclamation mark?
The double exclamation mark has negligible performance impact. However, it should be used judiciously, especially in performance-critical scenarios, as it adds an extra computational step.
In conclusion, the double exclamation mark in JavaScript provides a convenient way to convert values to their Boolean equivalents. Its simple syntax and various use cases make it a valuable tool in the JavaScript developer’s toolbox. Whether you need to convert values, short-circuit conditional expressions, or enforce type coercion, the double exclamation mark can help simplify your code and improve its readability.
Exclamation Mark In Typescript
Introduction:
In the world of programming languages, TypeScript has gained immense popularity for its ability to add static typing to JavaScript, providing enhanced tooling and efficient development environments. One distinctive feature in TypeScript is the exclamation mark or the “!” character. This symbol holds great significance and its usage can impact the behavior of the code. In this article, we will explore the meaning of the exclamation mark in TypeScript, its various applications, and clarify common misconceptions surrounding its usage.
Understanding the Exclamation Mark:
The exclamation mark, known as the “non-null assertion operator,” is used to assert that a value of a variable will never be null or undefined. It serves as a way to tell the TypeScript compiler to exclude these specific types from consideration, essentially deferring the responsibility of handling null checks to the developer.
Usage Scenarios:
1. Asserting Non-Nullability:
The primary use of the exclamation mark is to assert that a variable will always have a non-null value. For example:
“`typescript
let name: string | null = getNameFromAPI();
const formattedName: string = name!;
“`
In this scenario, the “name!” expression implies that the developer knows that “name” will never be null or undefined. However, it is important to exercise caution while using the exclamation mark, as incorrect assertions can lead to runtime errors.
2. Accessing Optional Properties:
In TypeScript, one can define properties of an object as either required or optional by appending a question mark after the property name. When working with optional properties, the exclamation mark can be used to assure the compiler that the property will indeed have a value. For instance:
“`typescript
interface User {
name: string;
age?: number;
}
function getUserAge(user: User): number {
return user.age!;
}
“`
Here, the exclamation mark tells the compiler that the “age” property will be present, even though it is defined as optional in the interface.
3. Handling DOM Manipulation:
When interacting with the DOM in TypeScript, there are instances where accessing elements may return nullable values, such as the getElementById method. By utilizing the exclamation mark, we can ensure the compiler that the element will always be found, enabling straightforward code manipulation. Example:
“`typescript
const element = document.getElementById(“myElement”)!;
element.classList.add(“highlighted”);
“`
In this case, the exclamation mark signifies the programmer’s confidence that there is an element with the ID “myElement” in the DOM.
Common Misconceptions and FAQs:
Q1: Does using the exclamation mark guarantee that null or undefined errors won’t occur?
A1: No, the exclamation mark is primarily a tool for the developer to indicate non-nullability to the TypeScript compiler. It does not eliminate the possibility of null or undefined errors if used incorrectly.
Q2: Should I use the exclamation mark everywhere to indicate non-nullability?
A2: It is generally recommended to use the exclamation mark sparingly and only when the developer has concrete knowledge that a variable or property will never be null or undefined. Overusing it may lead to potential runtime errors or hide underlying issues.
Q3: Can the exclamation mark be used with custom types?
A3: Yes, the exclamation mark can be used with custom types as long as the type declaration allows for null or undefined values. The operator’s purpose remains the same across all TypeScript types.
Q4: Are there alternatives to the exclamation mark?
A4: Yes, TypeScript provides alternative approaches to handle nullable values, such as optional chaining (?.) and the “nullish coalescing operator” (??). These alternatives can offer more controlled null checks.
Conclusion:
The exclamation mark in TypeScript acts as a powerful tool for asserting non-nullability and ensuring confident coding. However, it must be used judiciously, with careful consideration of the code context. By understanding its meaning and proper usage scenarios, developers can effectively leverage the exclamation mark to streamline their TypeScript code and enhance its readability and maintainability.
Triple Exclamation Mark Javascript
Understanding Triple Exclamation Mark JavaScript:
In JavaScript, the triple exclamation mark (!!!) is a succinct way of converting a value into a boolean. This operator is known as the logical NOT operator and is used to reverse the boolean value of an expression. However, when used three times consecutively, it performs a double negation, resulting in converting any value into a boolean representation.
Usage and Benefits:
The primary usage of the triple exclamation mark JavaScript is to obtain the boolean representation of a value. This can be particularly handy when you need to determine whether a value is truthy or falsy. By relying on this shorthand notation, developers can streamline their code and achieve more concise conditional statements.
One of the key benefits of triple exclamation mark JavaScript is its ability to handle different types of input. It gracefully handles various data types, including strings, numbers, booleans, objects, and arrays. It transforms these types into a boolean value without raising any errors, providing a flexible and convenient approach to convert values.
Consider the following example:
“`
let value = ‘Hello’;
console.log(!!value); // Output: true
“`
In the above code snippet, the value variable is a string type. By applying the triple exclamation mark operator, the value is converted into its boolean representation. In this case, the output will be true since the string is not empty or a falsy value.
Pitfalls and Considerations:
While the triple exclamation mark JavaScript offers simplicity and brevity, it is important to be aware of certain considerations to avoid potential pitfalls.
One such consideration is the interpretation of empty or falsy values. The triple exclamation mark operator converts several values, such as null, undefined, empty strings, and zeros, into false. However, it converts non-empty strings, non-zero numbers, and objects into true. This behavior can sometimes be counterintuitive and lead to unexpected results if not properly taken into account.
Additionally, using triple exclamation marks excessively or arbitrarily may result in code that is hard to read and understand. It is recommended to use this feature judiciously and only when necessary, as excessive use can make the code less maintainable and harder to comprehend for other developers.
FAQs:
Q: Does the triple exclamation mark JavaScript work with arrays?
A: Yes, the triple exclamation mark operator can convert arrays into boolean values. An empty array will be considered falsy, while a non-empty array will be considered truthy.
Q: What happens if I apply the triple exclamation mark operator on an object?
A: The triple exclamation mark operator will always convert an object into true, regardless of its contents or properties.
Q: Can I use the triple exclamation mark multiple times on a single value?
A: Although you can chain multiple exclamation marks together (e.g., !!!!), it is unnecessary and redundant. A single triple exclamation mark (!!!) is sufficient to convert any value into its boolean representation.
Q: Is triple exclamation mark JavaScript widely supported in all browsers?
A: Yes, the triple exclamation mark JavaScript is supported in all modern browsers, including Chrome, Firefox, Safari, and Edge. However, it is always recommended to test your code across multiple browsers to ensure compatibility.
Conclusion:
Triple exclamation mark JavaScript is a concise and powerful feature that allows developers to quickly convert values into their boolean representation. By using this shorthand notation, developers can enjoy the benefits of simplicity and brevity, reducing the amount of code needed for conditional statements. However, it is crucial to be aware of its behavior with empty or falsy values and use it judiciously to maintain code readability. Overall, triple exclamation mark JavaScript is a useful addition to a developer’s toolkit when handling boolean conversions.
Images related to the topic typescript double exclamation mark
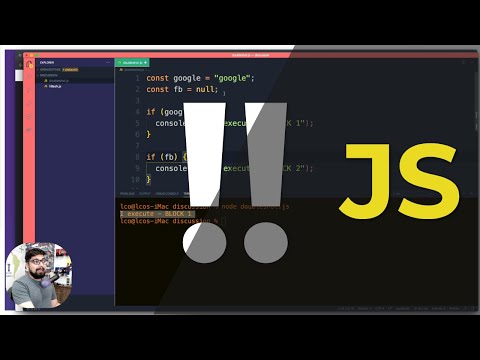
Found 29 images related to typescript double exclamation mark theme
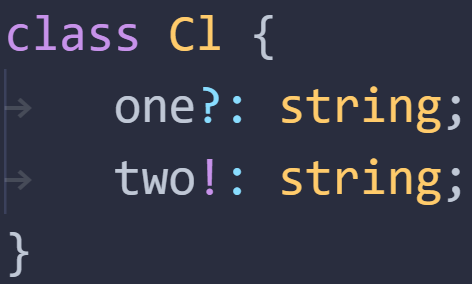
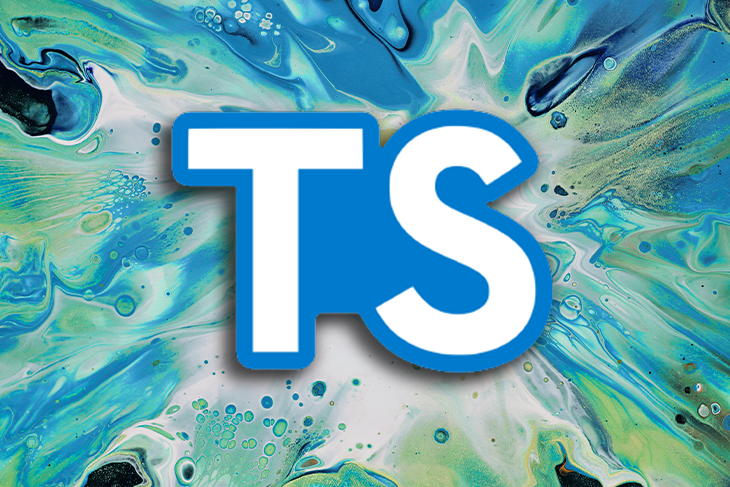
![How to convert a string to boolean in typescript [using 4 different ways] - SPGuides How To Convert A String To Boolean In Typescript [Using 4 Different Ways] - Spguides](https://i0.wp.com/www.spguides.com/wp-content/uploads/2023/02/convert-a-string-to-a-boolean-using-a-ternary-operator-to-check-the-string-equals-true.png)
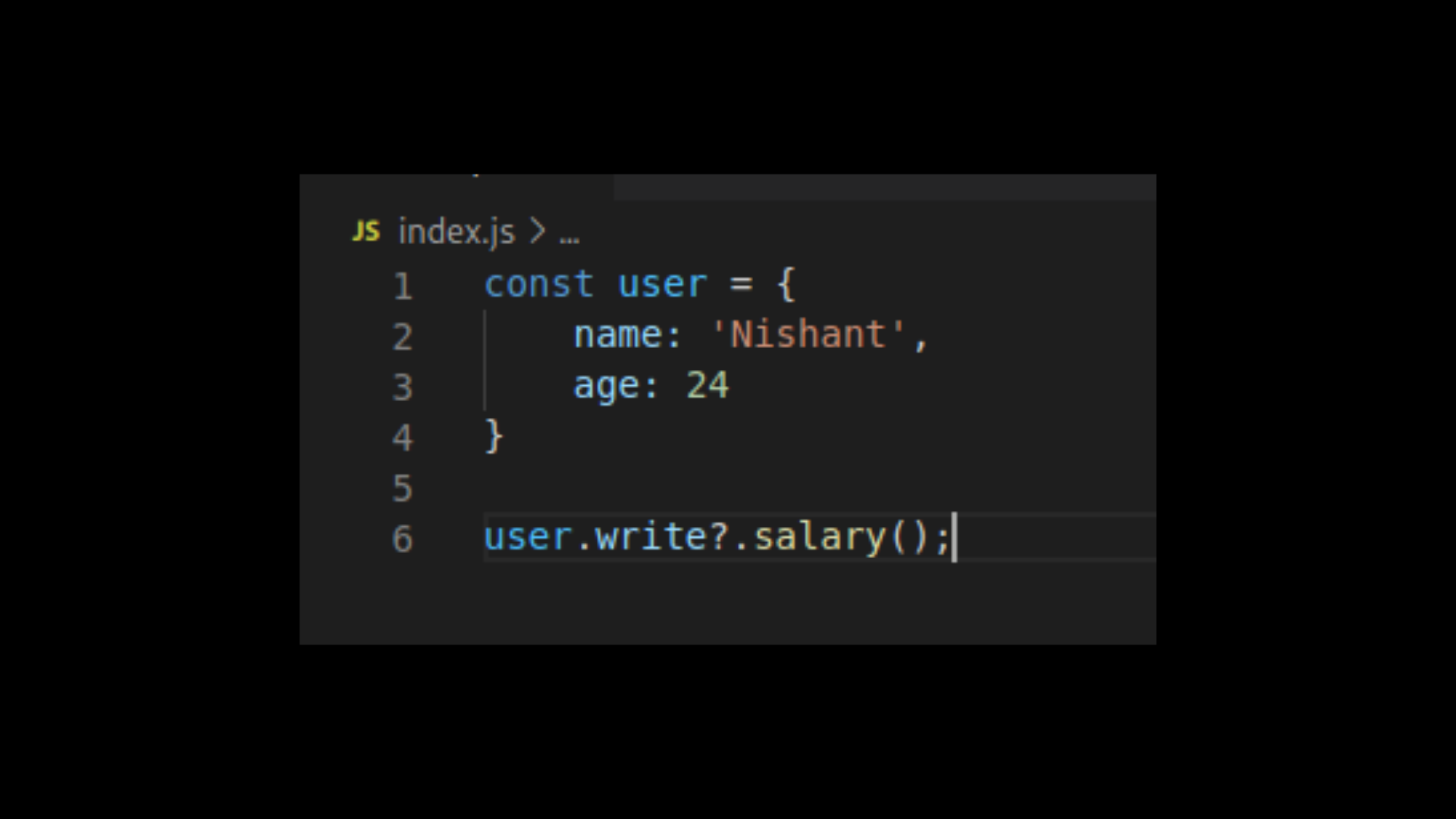
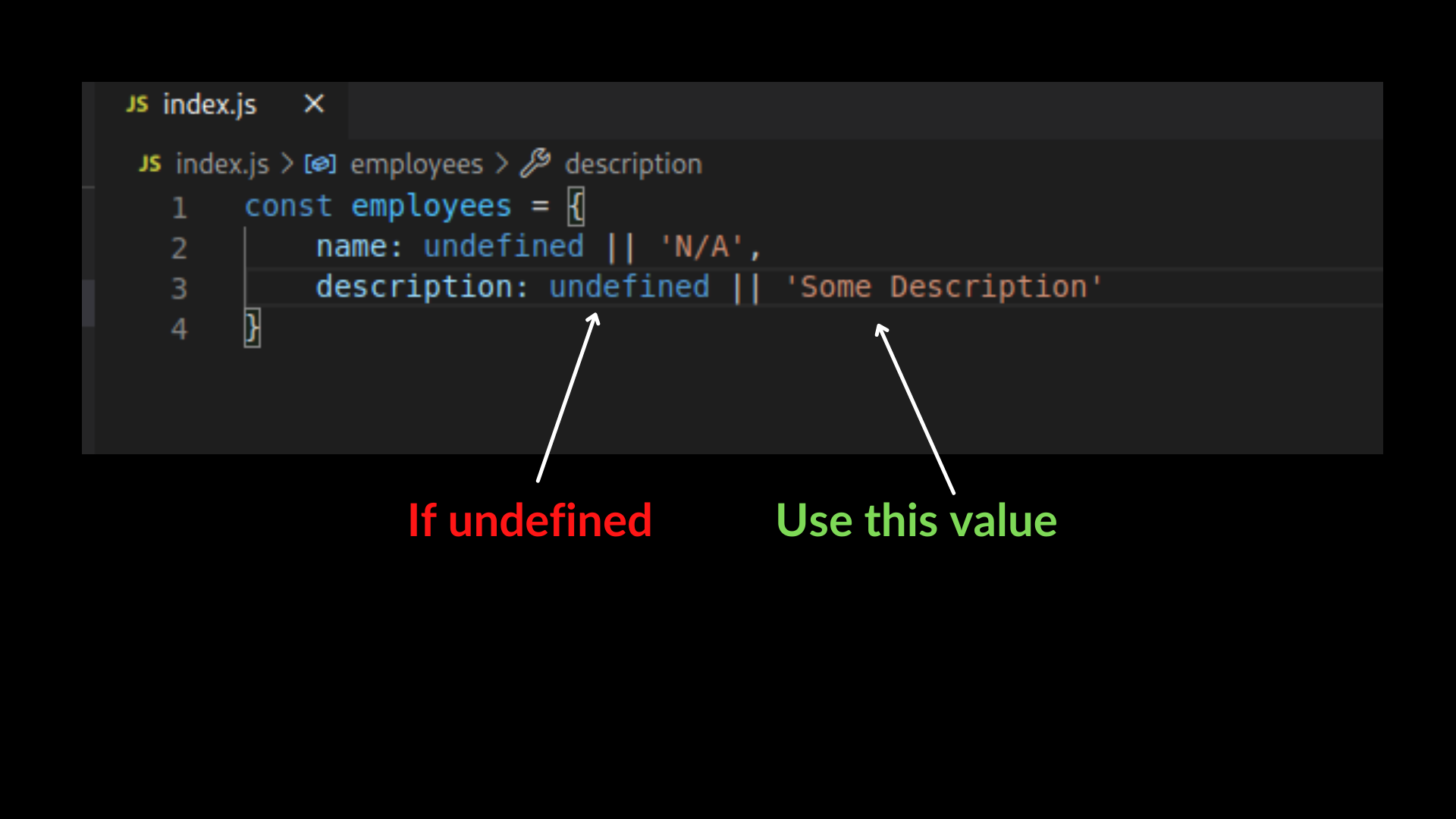
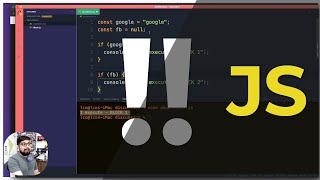

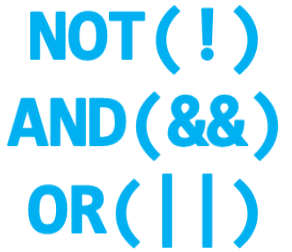
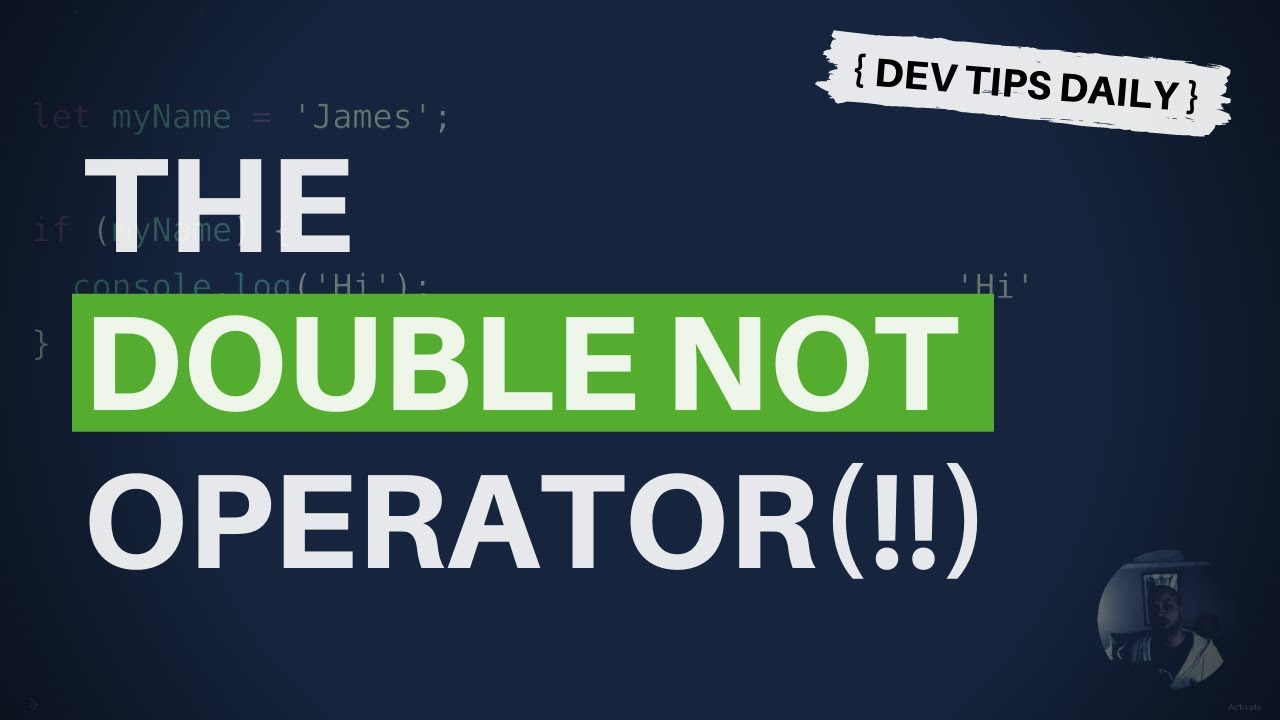
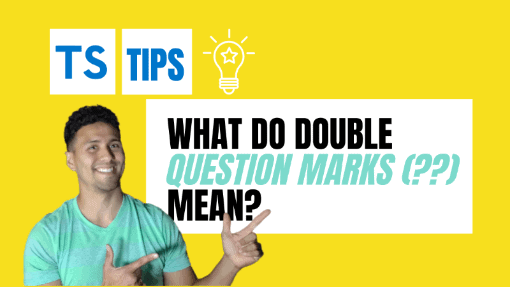
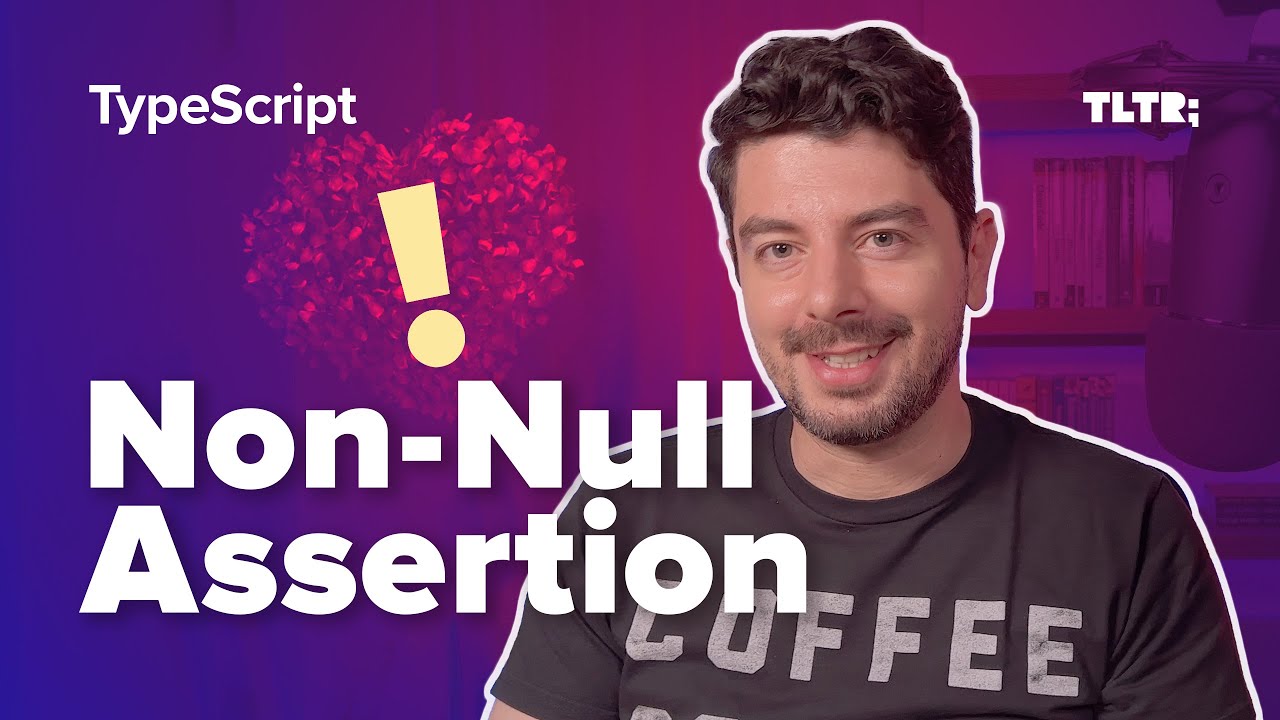
Article link: typescript double exclamation mark.
Learn more about the topic typescript double exclamation mark.
- What does the double exclamation !! operator mean? [duplicate]
- Understanding the exclamation mark in TypeScript
- Understanding the exclamation mark in TypeScript
- The Exclamation Point – TIP Sheets – Butte College
- JavaScript | How Double Bangs (Exclamation Marks) !! Work
- ‼️ Double Exclamation Mark Emoji – Emojipedia
- The Double Exclamation Operator (!!) in JavaScript
- JavaScript | How Double Bangs (Exclamation Marks) !! Work
- What’s the double exclamation sign for in JavaScript? – Medium
- What’s the double exclamation mark for in JavaScript?
- JavaScript double exclamation mark explained (with examples)
- Double Exclamation Operator Example in JavaScript – Linux Hint
- Typescript double “notnot” !! operator | by Aleksei Jegorov
See more: https://nhanvietluanvan.com/luat-hoc