Single Positional Indexer Is Out Of Bounds
The single positional indexer out of bounds error is a common error that occurs in Python when trying to access an index that is outside the valid range of indexes for a given data structure. This error is typically encountered when working with data structures such as lists, arrays, or data frames in libraries like Pandas. In this article, we will explore the causes of this error, explain how to prevent it, discuss debugging strategies for resolving it, provide examples of code that trigger the error, and offer tips for troubleshooting it.
Explanation of Single Positional Indexer Out of Bounds Error
A single positional indexer out of bounds error occurs when we try to access an index that is not present in the data structure we are working with. This error is raised at runtime and is accompanied by an error message that provides information about the cause of the error. The error message typically includes phrases such as “IndexError positional indexers are out-of-bounds,” “Index 0 is out of bounds for axis 0 with size 0,” “Iloc 1,” and “Iloc Pandas.”
Common Causes of Single Positional Indexer Out of Bounds Error
There are several common reasons why this error may occur:
1. Incorrect index value: One common cause is providing an index value that is larger than the length of the data structure. For example, trying to access element at index 5 in a list with only 3 elements will raise this error.
2. Off-by-one error: In some cases, the error may be due to using a 0-based index system in a code that expects a 1-based index. This can lead to accessing an index that is one position ahead of the intended position.
3. Empty data structure: If the data structure is empty, such as an empty list or an empty data frame, any attempt to access an index will result in this error.
4. Incompatible indexing method: This error can also occur when using indexing methods that are not compatible with the data structure being accessed. For example, trying to use the iloc method on a Pandas Series object instead of a DataFrame will raise this error.
Indexing and Slicing in Python
Indexing and slicing are fundamental operations in Python for accessing elements or portions of a data structure.
Indexing refers to accessing a specific element at a given position in a data structure. In Python, indexing starts at 0 for the first element, with each subsequent element being accessed by incrementing the index by 1.
Slicing, on the other hand, allows us to extract a portion of a data structure by specifying the start and end indices. The slice operator in Python is represented by the colon (:) character.
Both indexing and slicing can be used with different data structures, including lists, arrays, and Pandas data frames.
How to Prevent Single Positional Indexer Out of Bounds Error
To prevent single positional indexer out of bounds error, you can follow these tips and techniques:
1. Validate index values: Always check that the index value you are trying to access is within the valid range. Use conditional statements like if statements to ensure that you are not accessing an index that is out of bounds.
2. Use try-except blocks: Surround your indexing code with a try-except block to catch any IndexError that may occur. This allows you to handle the error gracefully and provide helpful error messages to the user.
3. Check for empty data structures: Before attempting to access an index, check if the data structure is empty. If it is empty, take appropriate actions like displaying a message or skipping the indexing operation altogether.
4. Use the correct indexing method: Make sure you are using the correct indexing method for the data structure you are working with. For example, if you are working with a Pandas DataFrame, use iloc to access elements by their integer position.
Debugging Strategies for Resolving the Error
When encountering the single positional indexer out of bounds error, consider these debugging strategies:
1. Review your index values: Double-check your index values to ensure they are correct and within the valid range. Pay attention to any off-by-one errors or incorrect assumptions about the indexing system.
2. Print intermediate values: Insert print statements in your code to print out intermediate values related to the indexing operation. This can help you identify any unexpected values or inconsistencies that may lead to the error.
3. Step through the code: Use a debugger or step through the code manually to track the execution flow and identify the specific line where the error occurs. This can help you pinpoint the source of the error and understand what is happening.
4. Use logging: Instead of or in addition to print statements, consider using a logging framework to log relevant information about the indexing operation. This can provide more detailed information and can be used for later analysis.
Examples of Code Causing Single Positional Indexer Out of Bounds Error
Here are some examples of code snippets that can trigger the single positional indexer out of bounds error:
Example 1: Accessing an Index Outside the Range
“`
my_list = [1, 2, 3]
print(my_list[5])
“`
Output:
“`
IndexError: list index out of range
“`
Example 2: Using the Incorrect Indexing Method
“`
import pandas as pd
my_series = pd.Series([1, 2, 3])
print(my_series.iloc[1])
“`
Output:
“`
IndexError: single positional indexer is out of bounds
“`
Tips for Troubleshooting Single Positional Indexer Out of Bounds Error
Here are some tips for troubleshooting the single positional indexer out of bounds error:
1. Double-check your indexing code: Review your code and ensure that the indexing operations are correct and conform to the expected format for the data structure you are working with.
2. Examine your data structure: Verify that the data structure you are working with contains the elements or records you expect. If the data structure is empty, you may need to handle this case separately.
3. Check for data transformations: If you are performing any data transformations or manipulations before the indexing operation, ensure that these transformations are executed correctly and do not introduce any errors.
4. Read the documentation: Consult the documentation for the library or module you are using to understand the correct usage of indexing methods and any potential caveats or limitations.
Conclusion
The single positional indexer out of bounds error is a common error that occurs in Python when trying to access an index that is outside the valid range of indexes for a given data structure. In this article, we discussed the causes of this error, explained indexing and slicing in Python, provided tips for preventing and troubleshooting the error, and shared examples of code that trigger the error. By understanding the causes and employing the suggested techniques, you can avoid or effectively resolve this error in your Python code.
How To Fix Index Error: Single Positional Indexer Is Out Of Bounds
What Does Single Positional Indexer Is Out Of Bounds Mean?
When working with data in programming languages like Python with frameworks such as pandas, you may encounter an error message that says “single positional indexer is out of bounds.” This error message is related to indexing and usually occurs when you are trying to access or manipulate data using an invalid index position. In this article, we will explore the meaning of this error message, understand why it occurs, and learn how to resolve it.
Understanding Indexing and Positional Indexer:
Before diving into the error message itself, it is important to understand the concept of indexing and the role of a positional indexer. In programming, indexing allows us to access elements within a data structure, such as a list, array, or pandas DataFrame. Each element in the data structure is assigned a unique index, which acts as its identifier. By specifying the index position, we can easily retrieve or modify the corresponding element.
A positional indexer refers to the index used to access elements by their position, starting from zero. For example, in a list [10, 20, 30, 40], the element 10 can be accessed using the positional index 0, element 20 with index 1, and so on.
What causes the “single positional indexer is out of bounds” error?
The “single positional indexer is out of bounds” error occurs when we try to access an element using an index that is either too high or too low for the data structure. In other words, the index value specified is beyond the valid range of indexes available for that particular data structure.
For instance, let’s consider a pandas DataFrame with 10 rows:
“`python
import pandas as pd
data = {‘Name’: [‘John’, ‘Emma’, ‘Lisa’, ‘Michael’, ‘Anna’, ‘David’, ‘Sarah’, ‘James’, ‘Olivia’, ‘Daniel’],
‘Age’: [25, 29, 36, 42, 31, 28, 40, 37, 24, 33],
‘City’: [‘New York’, ‘London’, ‘Paris’, ‘Tokyo’, ‘Rome’, ‘Berlin’, ‘Sydney’, ‘Toronto’, ‘Moscow’, ‘Dubai’]}
df = pd.DataFrame(data)
“`
If we try to access the 11th row (index position 10), we will encounter the “single positional indexer is out of bounds” error, as the DataFrame only has indexes from 0 to 9.
How to resolve the “single positional indexer is out of bounds” error:
To resolve this error, we need to ensure that the index position we specify falls within the valid range of indexes. Here are some approaches to handle this error:
1. Check the size of your data structure: Before accessing elements using index values, check the size of your data structure. Ensure that your index position doesn’t exceed the total number of elements minus one. For example, in a list with four elements, valid index positions range from 0 to 3.
2. Use built-in methods to check index boundaries: Pandas provides several built-in methods that can help you verify if your index position is within bounds. For instance, you can use the `shape` attribute to determine the dimensions of a DataFrame. If your index position exceeds the range, you can use conditional statements or error handling techniques to handle it appropriately.
3. Be cautious while iterating: When iterating over elements or rows in a data structure, ensure that the loop doesn’t go beyond the valid range of indexes. Use the `len()` function to determine the length of your data structure and constrain the loop accordingly.
4. Check variable assignments: If you’re manipulating indexes programmatically, ensure that your variable assignments for index positions are accurate. It’s possible to mistakenly assign an index that is out of bounds, leading to the error message.
5. Double-check your data source: If your data is coming from an external source, such as a file or API, make sure the data is correctly formatted and aligned with your expectations. It’s possible that your data source contains fewer elements than expected, resulting in an out-of-bounds error.
FAQs:
Q1. Can incorrect slicing also result in a “single positional indexer is out of bounds” error?
A1. Yes, incorrect slicing can also lead to this error. When slicing a data structure using an invalid range, such as using a start or stop value that is out of bounds, you may encounter this error.
Q2. How can I know the valid range of indexes for a data structure?
A2. Many programming languages provide built-in functions or methods to obtain the length or size of a data structure. You can use these methods to determine the valid range of indexes available.
Q3. Can this error occur with other data structures apart from pandas DataFrames?
A3. Yes, this error is not limited to pandas DataFrames. It can occur with any data structure that uses indexing, such as lists, arrays, or dictionaries.
Q4. I am still encountering the error after following the suggested solutions. What should I do?
A4. If you have tried the suggested solutions and the error persists, double-check your code logic and verify that you are not inadvertently modifying the index range in your data structure. Additionally, reviewing any recent changes and understanding the context in which the error occurs may lead to a breakthrough in troubleshooting. If needed, reaching out to the specific programming community for further assistance can be helpful.
In conclusion, the “single positional indexer is out of bounds” error often arises when trying to access data using an invalid index position. By understanding the concept of indexing, being cautious with index boundaries, and verifying variable assignments, you can effectively handle and resolve this error, allowing you to work with your data structure seamlessly.
How Does Iloc Work In Python?
Python is a versatile programming language that offers a wide range of functionalities to manipulate and analyze data. One of the key features in Python is its ability to handle tabular data using libraries such as Pandas. Pandas provides various methods to slice and dice data, and one commonly used method is iLOC.
ILOC, short for integer location, is a method that allows you to select data from a Pandas DataFrame based on the integer index. It provides a powerful way to access specific rows and columns in a DataFrame. The iLOC method takes one or two arguments, which define the row and column labels to be selected.
To understand how iLOC works, let’s consider a simple example. Suppose we have a DataFrame with the following data:
“`
Name Age City
0 John 25 LA
1 Jane 30 NYC
2 Bob 35 SF
3 Mary 40 BOS
“`
To select a specific row using iLOC, you can use the following syntax:
“`python
df.iloc[row_index]
“`
For instance, if we want to select the second row of the DataFrame, we can use:
“`python
df.iloc[1]
“`
This will return:
“`
Name Jane
Age 30
City NYC
Name: 1, dtype: object
“`
Notice that the row is returned as a Series object, with the column labels as the index and the corresponding values. If you prefer to have the row returned as a DataFrame, you can use double square brackets instead:
“`python
df.iloc[[1]]
“`
This will return:
“`
Name Age City
1 Jane 30 NYC
“`
In addition to selecting specific rows, you can also use iLOC to select specific columns. To select a specific column, you should provide a comma-separated list of the column indices. The DataFrame will then be sliced to include only the specified columns. For instance, if we want to select the Name and Age columns, we can use:
“`python
df.iloc[:, [0, 1]]
“`
This will return:
“`
Name Age
0 John 25
1 Jane 30
2 Bob 35
3 Mary 40
“`
Notice that we used a colon `:` to select all rows, and `[0, 1]` to select the first and second columns. Similarly, if you want to select a range of columns, you can use the following syntax:
“`python
df.iloc[:, start_index:end_index]
“`
This will include all rows and the columns from `start_index` to `end_index-1`. For example, to select all rows and columns 1 to 2, you can use:
“`python
df.iloc[:, 1:3]
“`
This will return:
“`
Age City
0 25 LA
1 30 NYC
2 35 SF
3 40 BOS
“`
Lastly, you can also combine row and column selection using iLOC. For instance, if you want to select the first two rows and the last two columns, you can use:
“`python
df.iloc[:2, -2:]
“`
This will return:
“`
Age City
0 25 LA
1 30 NYC
“`
In this example, we used `:2` to select the first two rows, and `-2:` to select the last two columns.
Frequently Asked Questions (FAQs):
Q1: What is the difference between iLOC and LOC in Pandas?
A1: While both iLOC and LOC are used for selecting data from a DataFrame, they differ in the way rows and columns are selected. iLOC uses integer-based indexing, whereas LOC uses label-based indexing. iLOC uses integer indices to select specific rows and columns, while LOC uses labels assigned to the rows and columns.
Q2: Can ILOC be used to update values in a DataFrame?
A2: Yes, iLOC can be used to update values in a DataFrame. For example, to update the value in the second row and third column, you can use the following syntax:
“`python
df.iloc[1, 2] = “Chicago”
“`
This will set the value of the cell at row 1, column 2 to “Chicago”.
Q3: Can I use iLOC with a Boolean condition?
A3: No, iLOC does not support Boolean conditions to filter rows. For such cases, you should use other methods such as boolean indexing or query.
Q4: Can I use negative indices with iLOC?
A4: Yes, you can use negative indices with iLOC. Negative indices start counting from the end of the DataFrame, where -1 represents the last row or column.
Q5: Is iLOC inclusive or exclusive of the endpoint?
A5: The indexing with iLOC is exclusive of the endpoint. For example, if you want to select columns 1 to 3, you should use `df.iloc[:, 1:4]`, where 4 is exclusive.
In conclusion, iLOC is a powerful method in Python’s Pandas library that allows for selecting specific rows and columns from a DataFrame using integer-based indexing. It provides flexibility to slice and dice data, making it a valuable tool for data manipulation and analysis tasks.
Keywords searched by users: single positional indexer is out of bounds IndexError positional indexers are out-of-bounds, Index 0 is out of bounds for axis 0 with size 0, Iloc 1, Iloc Pandas, Too many indexers, Pandas loc and iloc, Get index of row pandas, Assign value to DataFrame
Categories: Top 96 Single Positional Indexer Is Out Of Bounds
See more here: nhanvietluanvan.com
Indexerror Positional Indexers Are Out-Of-Bounds
Introduction:
In programming languages, including Python, IndexError is a common error encountered when trying to access an element from a list, tuple, or other data structure using an index that is out of bounds. An out-of-bounds index refers to an index that exceeds the range of valid indices for a given data structure. In this article, we will focus specifically on IndexError related to positional indexers in English, exploring its causes, implications, and ways to handle it effectively.
Understanding IndexError:
IndexError is a type of exception that occurs when a program attempts to access an element from a sequence (e.g., list, tuple, string) using an index that is outside the valid range. This error is often encountered when iterating over a sequence or trying to access specific elements within it.
In the context of positional indexers in English, IndexError typically arises when dealing with data structures such as lists or strings. English being a language with variable word lengths and structures, these out-of-bounds errors can occur due to differences in indexing styles (e.g., 0-based indexing vs. 1-based indexing) or referencing elements that do not exist within a given context.
Common Causes of IndexError:
1. Zero-Based vs. One-Based Indexing:
In Python and many other programming languages, indexing typically starts from 0. However, some languages and contexts may use 1-based indexing. This discrepancy can lead to confusion, resulting in out-of-bounds errors when accessing elements using the wrong index notation.
2. Length Mismatch:
When working with lists or strings, it is crucial to ensure that the index provided falls within the valid range of the data structure. If a program attempts to access an index that exceeds the length of the sequence, an IndexError will occur. This usually happens when the programmer assumes a different length or forgets to account for zero-based indexing.
3. Incorrect Range Calculation:
Index errors can also arise when calculating and utilizing ranges incorrectly. For example, attempting to iterate from 1 to the length of a list while working with zero-based indexing will result in an IndexError.
Handling IndexError:
1. Debugging:
When encountering an IndexError, it is essential to determine the root cause of the error. Review the code that caused the exception and identify the line(s) where the out-of-bounds access occurs. Use print statements or debugging tools to examine the values of indices, lengths, and other variables involved. This step is crucial for gaining a better understanding of why the error occurred.
2. Verify Indexing Style:
Check if the data structure you are working with follows zero-based or one-based indexing. Adjust your code accordingly to ensure that the correct indexing notation is used. Be cautious when switching between different data structures or programming languages to avoid indexing style conflicts.
3. Bounds Checking:
Before accessing elements within a sequence, perform bounds checking. Ensure that the index lies within the valid range of indices (0 to length – 1 for zero-based indexing). Implement conditional statements or try-except blocks to handle potential out-of-bounds access gracefully. This could involve displaying an error message, modifying the index, or skipping the access attempt altogether.
4. Avoid Off-By-One Errors:
Exercise caution when utilizing for-loops or while-loops that iterate over a range of indices. Ensure that the range is correctly defined, considering the indexing style and the appropriate starting and ending points.
FAQs:
Q: What is the significance of zero-based indexing in Python?
A: Zero-based indexing simplifies language implementation and aligns with many programming practices. It also provides consistency with other popular languages like C and Java, making it easier for programmers to switch between different programming languages.
Q: How can I differentiate between an IndexError and other exceptions?
A: IndexError is a specific type of exception raised when accessing elements with out-of-bounds indices. Use try-except blocks to catch IndexError specifically, so you can handle it differently from other exceptions and improve code reliability.
Q: Can IndexError occur in languages other than English?
A: Yes, IndexError is not limited to a particular language. It can occur in any programming language that supports indexed data structures and where indexing is not handled properly.
Conclusion:
In conclusion, understanding and effectively addressing IndexError related to positional indexers in English is crucial for writing robust code. By paying attention to indexing style, performing appropriate bounds checking, and avoiding off-by-one errors, programmers can mitigate the occurrence of IndexError and improve the overall reliability of their code.
Index 0 Is Out Of Bounds For Axis 0 With Size 0
When working with data analysis, machine learning models, or any form of data manipulation in Python, you may encounter an error message stating “Index 0 is out of bounds for axis 0 with size 0.” This error can be quite frustrating, especially for beginners, as it often seems cryptic and difficult to understand. In this article, we will delve into the meaning of this error message, explore the potential causes, and provide ways to resolve it.
Understanding Indexing and Axes in Python Arrays
In Python, arrays are zero-indexed, meaning that the first element is accessed using index 0, the second element with index 1, and so on. Additionally, NumPy arrays often contain multiple dimensions known as axes. The axis parameter determines along which axis the operations will be performed. By default, axis 0 represents the rows, while axis 1 represents the columns in a 2-dimensional array.
Now, let’s explore the error message itself: “Index 0 is out of bounds for axis 0 with size 0.” This error typically occurs when attempting to access an element in an array that does not exist or when performing operations on an empty array. It signifies that the index specified is greater than the size of the array along the specified axis.
Common Causes of the “Index 0 is Out of Bounds for Axis 0 with Size 0” Error
1. Empty Arrays:
– One of the most common causes of this error is attempting to access elements within an empty array. An empty array has a size of 0 along its axis, which means that no elements are available for indexing. Therefore, any attempt to access these elements will result in the “index out of bounds” error.
2. Incorrect Indexing:
– Another frequent cause of this error is using an incorrect index value. It could happen when mistakenly assuming a different number of elements or dimensions in the array. Incorrect indexing can lead to accessing elements beyond the array’s actual size, causing the error message to appear.
3. Improper Data Manipulation:
– The “Index 0 is out of bounds” error can also occur when performing certain operations on an array that lead to an unexpected reduction in size. For example, if you remove elements from an array without updating the size properly, subsequent indexing attempts can result in an index out of bounds error.
Resolving the “Index 0 is Out of Bounds for Axis 0 with Size 0” Error
1. Check the Dimensions and Size of the Array:
– Start by verifying the dimensions and size of the array you are working with. Ensure that the size along the specified axis is not 0, as this would indicate an empty array.
2. Review Your Indexing:
– Double-check the indexing values you are using. Make sure they are within the valid range of the array’s dimensions. Remember that Python arrays are zero-indexed, so the highest index should be one less than the size of the array.
3. Address Empty Arrays:
– If you determine that an empty array is causing the error, consider reevaluating your data or your approach to ensure that the array contains the necessary elements before attempting any operations.
4. Handle Exceptions:
– To prevent the error from crashing your program, you can implement exception handling. Wrap the code that may trigger the “index out of bounds” error within try-except blocks. This allows you to catch and handle the exception, providing feedback or alternative actions when the error occurs.
Frequently Asked Questions
Q: Does this error only occur with NumPy arrays?
A: No, this error can occur with any type of array in Python, including NumPy arrays. However, the specificity of the error message often indicates that NumPy arrays are involved.
Q: Can I use negative indices to access elements in an array?
A: Yes, negative indices can be used to access elements from the end of the array. For example, -1 represents the last element, -2 represents the second to last element, and so on. However, the “index out of bounds” error can still occur if a negative index surpasses the array’s size.
Q: How can I debug this error when working with large datasets?
A: When dealing with large datasets, it can be challenging to identify the precise cause of the error. In such cases, consider using print statements or debugging tools to track the size, dimensions, and indices of the arrays at various stages of your code.
Q: Are there any other related error messages I should be aware of?
A: Yes, there are several related error messages that you may encounter while working with arrays, such as “IndexError: index x is out of bounds for axis y with size z.” These messages may vary slightly in wording but generally indicate a similar issue of accessing elements outside the bounds of the array.
Conclusion
The “Index 0 is out of bounds for axis 0 with size 0” error message is a common roadblock faced by Python developers working with arrays. By understanding the concept of indexing, the axes of arrays, and the potential causes of this error, you can resolve and prevent it effectively. Always double-check your indexing values, handle exceptions, and review the size and dimensions of the arrays you are working with. With these precautions in place, you can avoid encountering this error and ensure smooth execution of your Python programs.
Iloc 1
Nestled in the northern part of the Philippines, Iloc 1 is a region that boasts not only stunning natural landscapes but also a rich cultural heritage. From its picturesque beaches to its historical landmarks, Iloc 1 offers an unforgettable experience for travelers seeking to immerse themselves in the beauty and charm of this region. In this article, we will delve into the highlights and must-visit destinations of Iloc 1, exploring its natural wonders, cultural attractions, and culinary delights, while also providing insights into practical information that will enhance your journey.
Natural Wonders: The Beauty of Ilocos
One of the most prominent features of Iloc 1 is its breathtaking coastline. With its crystal-clear waters and golden sandy beaches, it is a tropical paradise that rivals the best in the world. Among the must-visit beaches is Saud Beach in Pagudpud, renowned for its pristine shores and perfect waves that attract surfers from around the globe.
For nature enthusiasts, Iloc 1 also offers magnificent landscapes and land formations. The Paoay Sand Dunes, located in Paoay, is a 1500-hectare expanse of rolling sand dunes that provide a unique experience for adventure seekers. Take a thrilling ride on a 4×4 vehicle or try sandboarding on the slopes of the dunes for an adrenaline rush like no other.
Culture and Heritage: A Glimpse into the Past
No visit to Iloc 1 is complete without exploring its rich cultural heritage. The region is home to four UNESCO World Heritage Sites, including the historic town of Vigan. Known for its well-preserved Spanish colonial architecture, Vigan takes you back in time with its cobblestone streets, ancestral houses, and horse-drawn carriages. Take a leisurely stroll along Calle Crisologo, the city’s main street, and immerse yourself in the cultural remnants of the past.
Another significant cultural destination is Bantay Church and Bell Tower in Ilocos Sur. This centuries-old church is not only a place of worship but also an architectural marvel. Climb to the top of the bell tower, and you will be rewarded with a stunning panoramic view of the surrounding countryside.
Culinary Delights: A Taste of Ilocano cuisine
No trip to Iloc 1 would be complete without indulging in the region’s unique culinary offerings. Prepare your taste buds for a delightful adventure as you savor authentic Ilocano dishes. Must-try delicacies include bagnet, crispy deep-fried pork belly, and empanada, a savory pastry filled with meat and vegetables. Another local favorite is the famous longganisa, a flavorful sausage made from locally sourced ingredients.
For a truly immersive food experience, visit the iconic La Preciosa in Vigan, a restaurant that offers traditional Ilocano dishes prepared with love and authenticity. Don’t forget to pair your meal with Ilocano vinegar, a condiment made from sugar cane sap, which adds a tangy twist to every bite.
FAQs:
Q: What is the best time to visit Iloc 1?
A: The best time to visit Iloc 1 is during the dry season, which runs from November to April. During these months, you can enjoy the beautiful beaches and outdoor activities without worrying about heavy rains or typhoons.
Q: How do I get to Iloc 1?
A: The most convenient way to reach Iloc 1 is by air. The region is served by Laoag International Airport, located in Ilocos Norte. Several airlines operate regular flights to and from major cities in the Philippines. From the airport, you can easily hire a car or take a taxi to your desired destination.
Q: Are there accommodations available in Iloc 1?
A: Yes, Iloc 1 offers a wide range of accommodations to suit every budget. From luxury resorts to budget-friendly hotels and cozy guesthouses, there is something for every traveler. In popular tourist areas such as Vigan and Pagudpud, you can find a variety of options that cater to different preferences and requirements.
Q: Are there local tour guides available in Iloc 1?
A: Yes, there are plenty of local tour guides available who can enhance your experience in Iloc 1. They can provide valuable insights into the history, culture, and natural wonders of the region, ensuring that you make the most out of your visit.
In conclusion, Iloc 1 is a destination that offers a perfect blend of natural beauty, cultural heritage, and gastronomic delights. Whether you are a beach lover, a history enthusiast, or a foodie, this region has something to offer everyone. So pack your bags, embark on an unforgettable adventure, and discover the hidden gems of Iloc 1.
Images related to the topic single positional indexer is out of bounds
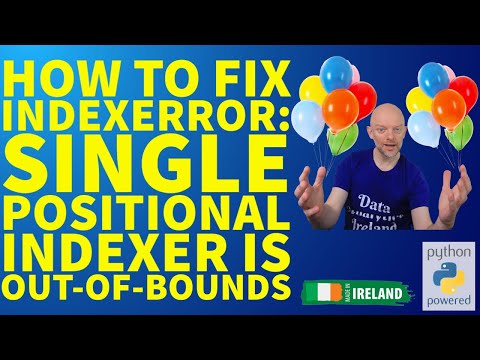
Found 18 images related to single positional indexer is out of bounds theme
.jpg)
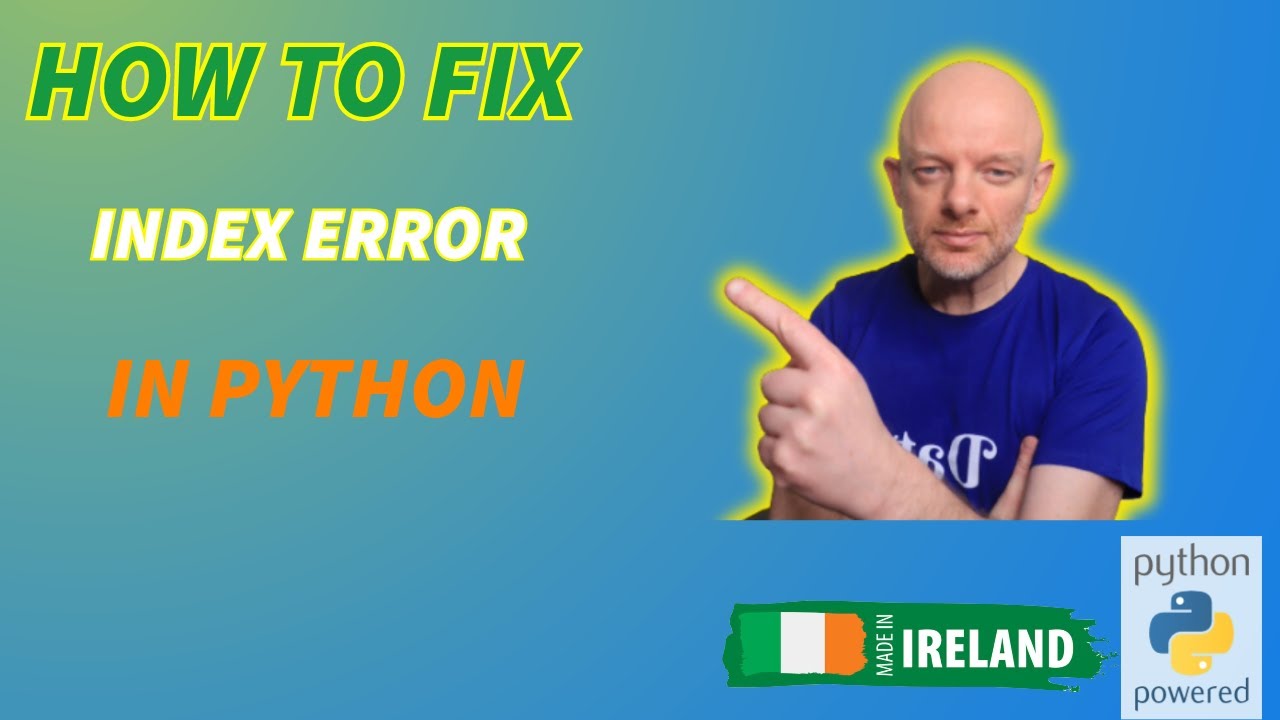
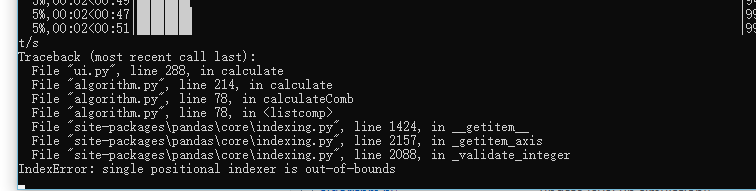



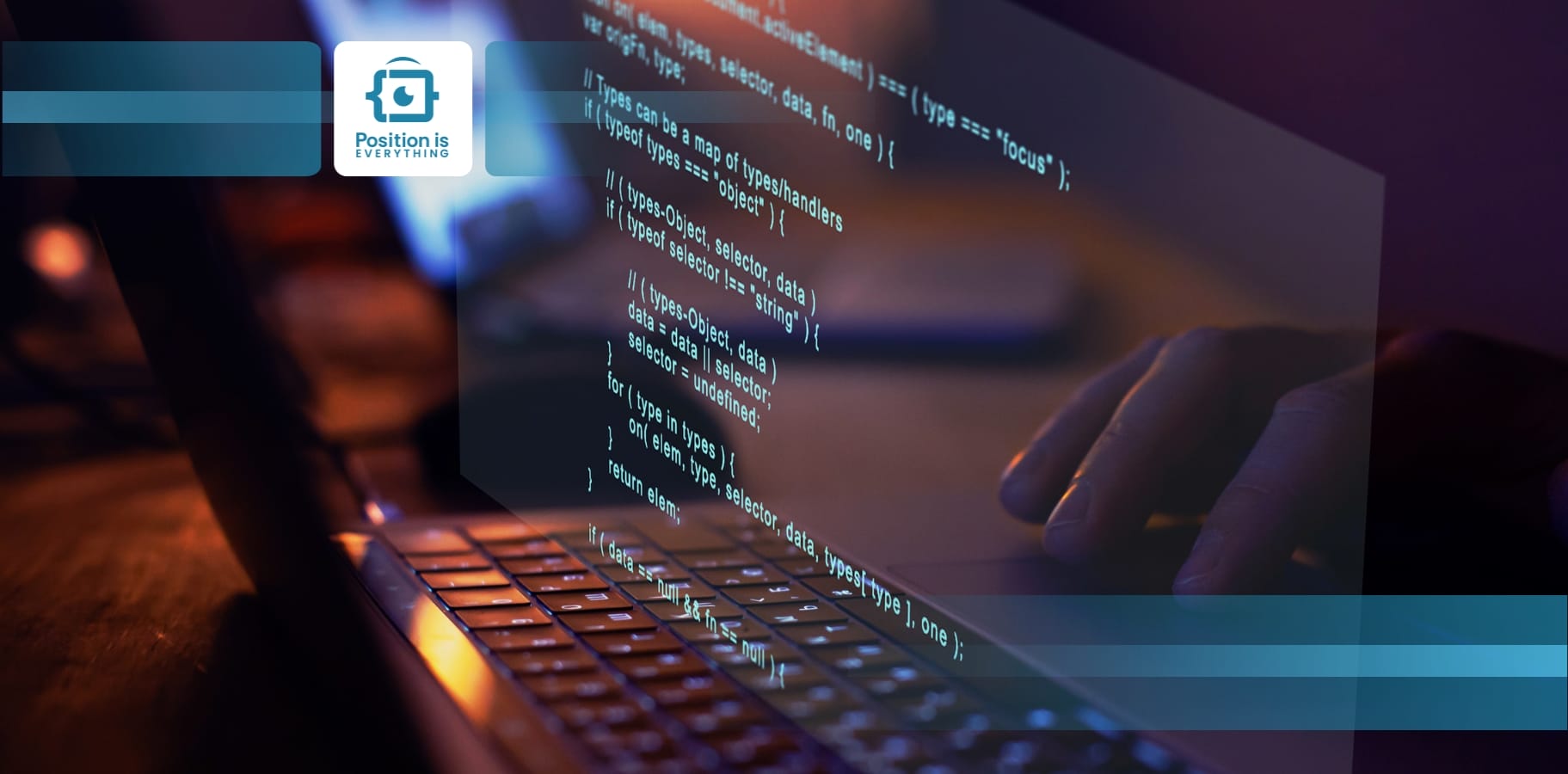
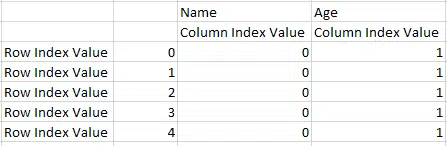
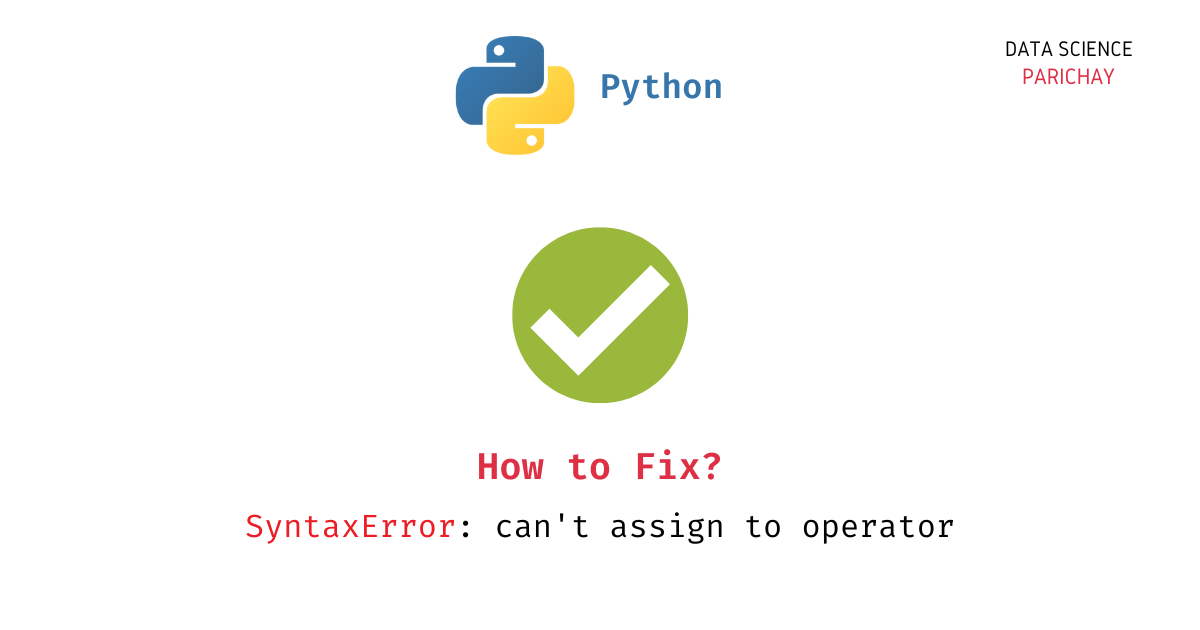
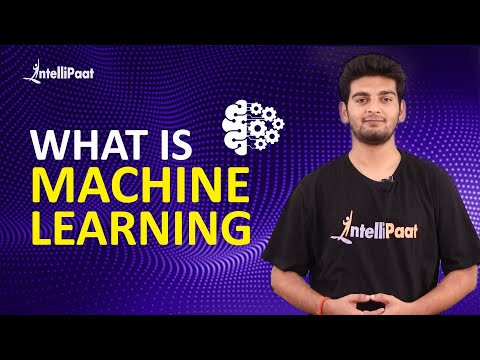

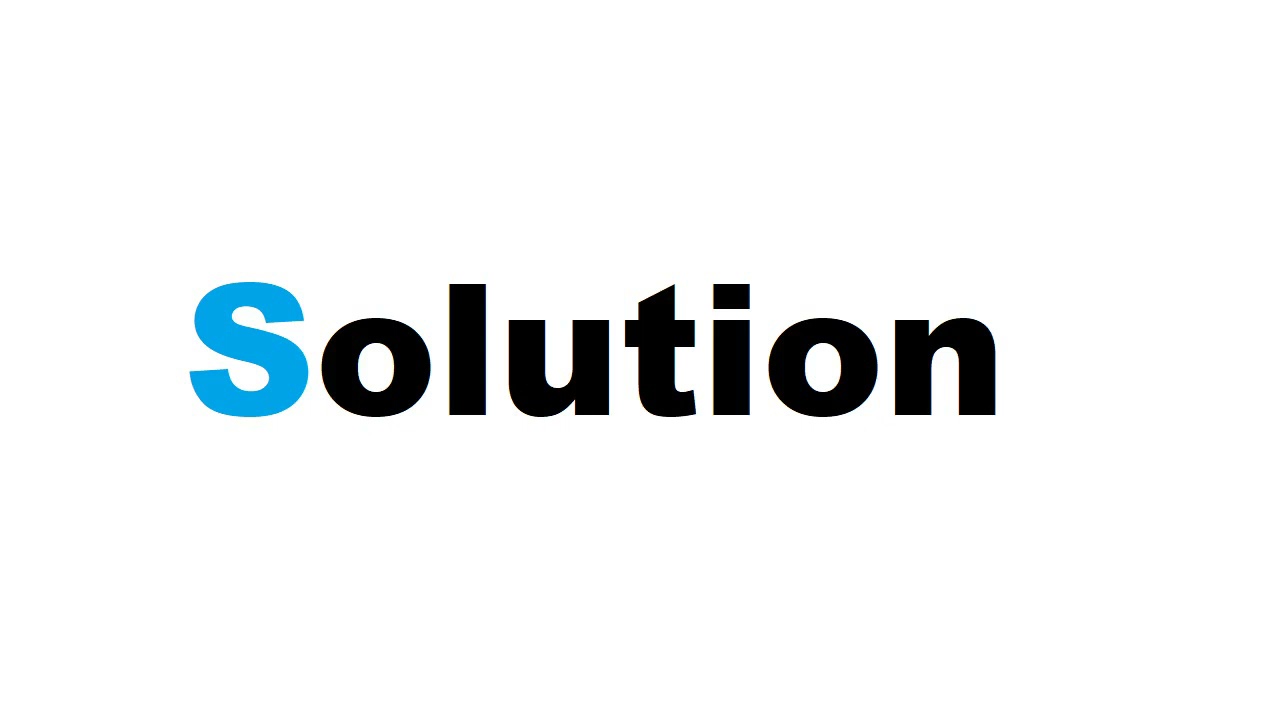
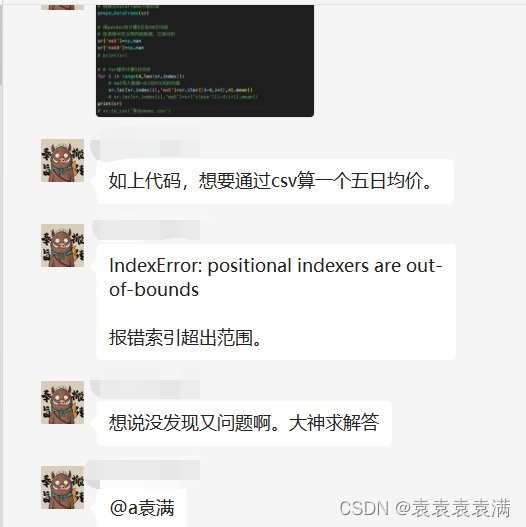


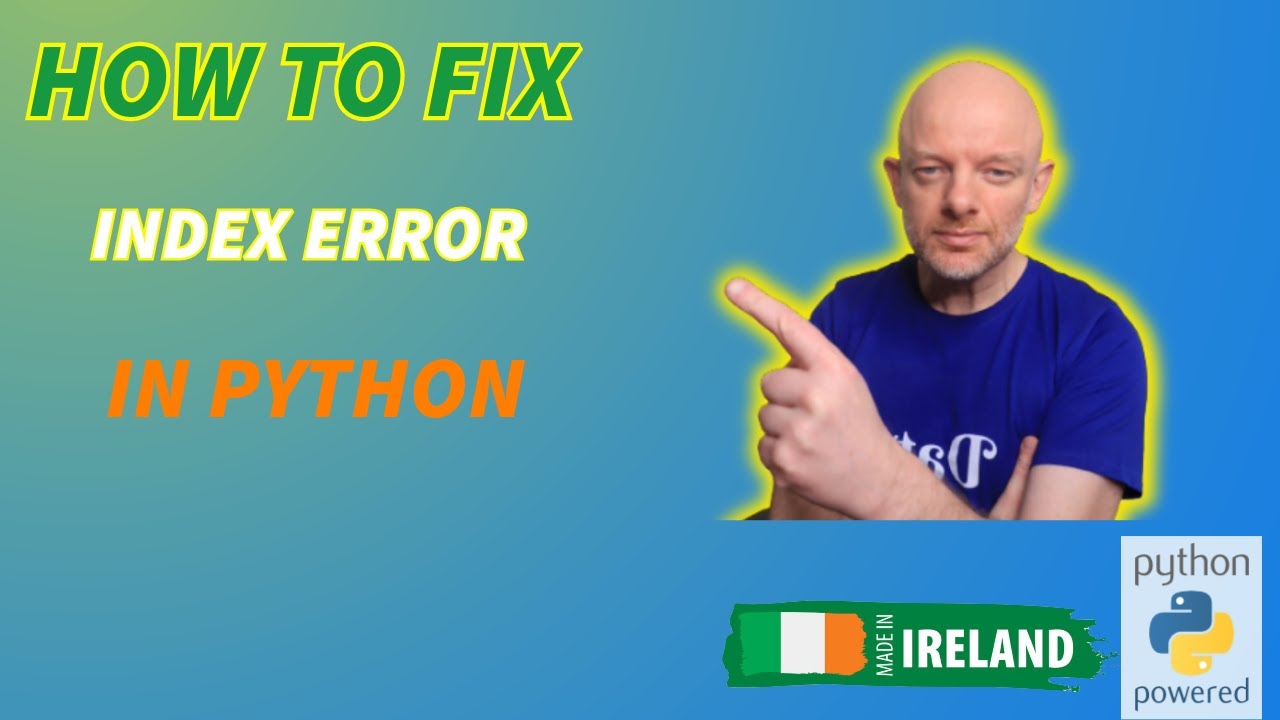
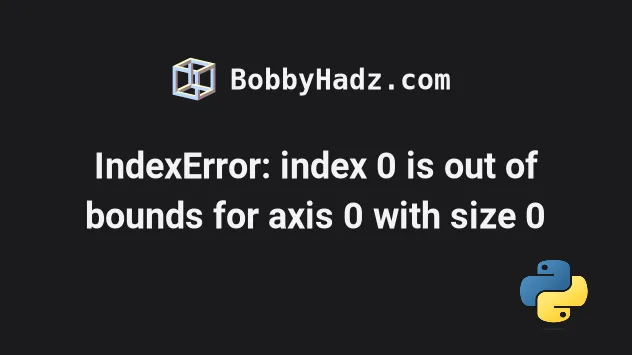
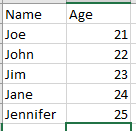
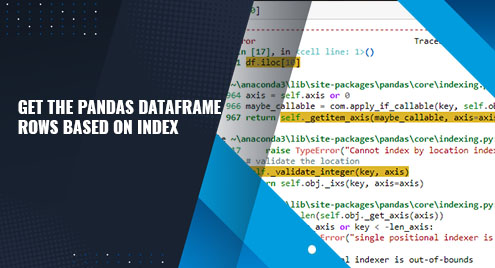
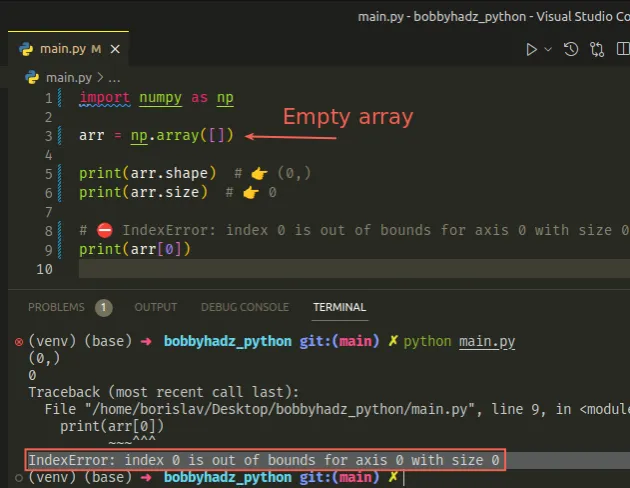
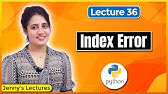
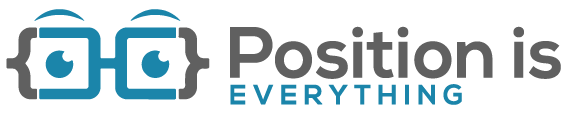

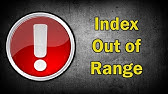

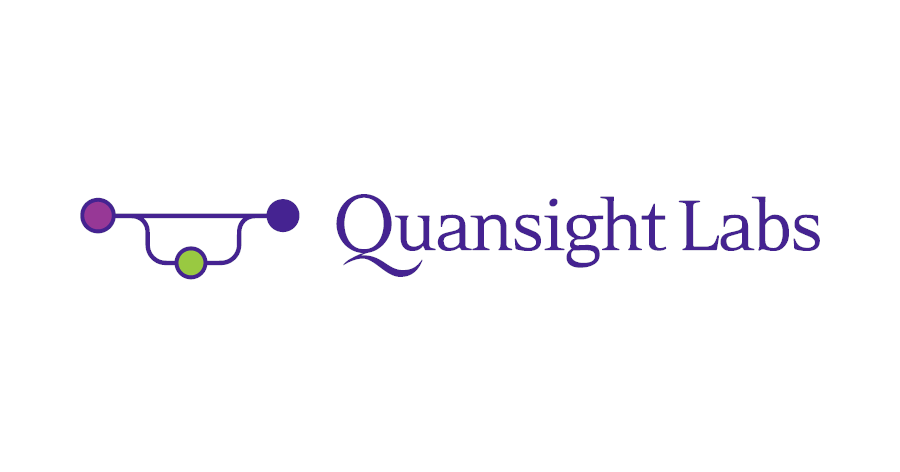


Article link: single positional indexer is out of bounds.
Learn more about the topic single positional indexer is out of bounds.
- iloc giving ‘IndexError: single positional indexer is out-of-bounds’
- indexerror: Single positional indexer is out-of-bounds Error
- iloc giving ‘IndexError: single positional indexer is out-of-bounds’
- iloc() Function in Python – PrepBytes
- IndexError: single positional indexer is out-of-bounds –
- iloc giving \’IndexError: single positional indexer is out-of …
- IndexError: Single positional indexer is out-of-bounds Fix
See more: https://nhanvietluanvan.com/luat-hoc/