Single Positional Indexer Is Out-Of-Bounds
The “single positional indexer is out-of-bounds” error is a common issue encountered by many programmers, particularly when working with data analysis tools like Pandas in Python. This error occurs when you attempt to access an element in a sequence using an index that is outside the range of valid indices for that sequence. In simpler terms, you are trying to access an element in a list, array, or DataFrame using an index that does not exist.
For example, consider the following code snippet:
“`python
import pandas as pd
data = [1, 2, 3]
df = pd.DataFrame(data)
print(df[3])
“`
In this case, we have created a DataFrame with a single column containing the values [1, 2, 3]. However, when we try to access the element at index 3 using `df[3]`, we encounter the “single positional indexer is out-of-bounds” error. This is because the valid indices for this DataFrame range from 0 to 2, and there is no element at index 3.
Common Causes for the “Single Positional Indexer is Out-of-Bounds” Error
There are several common causes for this error, including:
1. Incorrect index values: This error can occur if you mistakenly provide an incorrect index value when trying to access an element in a sequence. It is important to ensure that the index you provide is within the valid range.
2. Missing data: If you are working with incomplete or missing data, it is possible that the index you are trying to access is simply not present in the dataset.
3. Empty sequences: Another potential cause for this error is when you attempt to access an element in an empty sequence. In such cases, the valid index range is from 0 to 0, resulting in the “Index 0 is out of bounds for axis 0 with size 0” error.
Handling Missing or Incorrect Index Values
To handle missing or incorrect index values, you can employ various error-handling techniques. One common approach is to use conditional statements to check if the index is valid before accessing the element. For example:
“`python
import pandas as pd
data = [1, 2, 3]
df = pd.DataFrame(data)
index = 3
if index >= 0 and index < len(df): value = df[index] print(value) else: print("Invalid index") ``` By checking if the index is within the valid range, you can avoid the "single positional indexer is out-of-bounds" error and handle the situation gracefully. Error Handling Techniques for the "Out-of-Bounds" Error When dealing with the "out-of-bounds" error, it is necessary to implement error handling techniques to catch and handle such exceptions. In Python, you can use `try` and `except` blocks to catch the `IndexError` and prevent the program from crashing. For example: ```python import pandas as pd data = [1, 2, 3] df = pd.DataFrame(data) try: value = df[3] print(value) except IndexError: print("Invalid index") ``` By using a `try-except` block, if an `IndexError` occurs, the program will execute the code within the `except` block, allowing you to handle the error appropriately. Resolving the Error by Adjusting the Index or Utilizing Appropriate Data Structures To resolve the "single positional indexer is out-of-bounds" error, you can adjust the index to a valid value within the range of the sequence you are working with. Alternatively, you can utilize appropriate data structures and functions provided by libraries like Pandas to handle index-related operations more effectively. For instance, if you want to retrieve the index of a specific row in a Pandas DataFrame, you can use the `iloc` function: ```python import pandas as pd data = [1, 2, 3] df = pd.DataFrame(data) index = df.iloc[1] print(index) ``` In this example, we use `df.iloc[1]` to retrieve the index of the second row in the DataFrame, which is 1. By utilizing the appropriate tools and functions, you can avoid the "out-of-bounds" error and perform your desired operations successfully. Preventive Measures: Validating and Sanitizing Index Values To prevent encountering the "single positional indexer is out-of-bounds" error, it is important to validate and sanitize index values before using them to access elements in a sequence. This involves ensuring that the index value is within the valid range and adjusting it if necessary. One approach to validating index values is to use conditional statements to check if the index is within the range of the sequence. Additionally, you can utilize built-in functions and methods provided by libraries like Pandas to perform range checks and validate the index values efficiently. Potential Implications and Consequences of the Error The "single positional indexer is out-of-bounds" error can have several implications and consequences in your program. If this error goes unnoticed or is not handled properly, it can lead to unexpected program behavior and incorrect results. Additionally, it can cause your program to crash or terminate abruptly, negatively impacting the user experience. Furthermore, when working with large datasets or complex data analysis tasks, such errors can cause delays and hinder progress. Therefore, it is crucial to understand the causes of this error and implement appropriate measures to handle and prevent it. Best Practices for Avoiding the "Single Positional Indexer is Out-of-Bounds" Error To avoid encountering the "single positional indexer is out-of-bounds" error, it is important to follow some best practices: 1. Validate index values: Always validate index values before using them to access elements in a sequence. Perform range checks and ensure the index is within the valid range. 2. Utilize appropriate data structures and libraries: Use suitable data structures and libraries that provide functions and methods to handle index-related operations efficiently. Libraries like Pandas offer powerful tools that can simplify and enhance your data analysis tasks. 3. Implement error handling techniques: Use `try` and `except` blocks to catch and handle `IndexError` exceptions. This will prevent your program from crashing and allow you to handle such errors gracefully. 4. Thorough testing: Conduct thorough testing of your code, especially when working with large datasets or complex operations involving indices. Test various scenarios and edge cases to ensure your code handles them correctly. By following these best practices and implementing proper error handling techniques, you can minimize the occurrence of the "single positional indexer is out-of-bounds" error and ensure the stability and reliability of your code. FAQs Q: What does the "single positional indexer is out-of-bounds" error mean? A: This error occurs when you try to access an element in a sequence using an index that is outside the range of valid indices for that sequence. Q: What are the common causes of this error? A: Common causes include incorrect index values, missing data, and attempting to access elements in empty sequences. Q: How can I handle missing or incorrect index values? A: Use conditional statements to validate index values before accessing elements. Additionally, you can implement error handling techniques like `try` and `except` blocks to catch and handle `IndexError` exceptions. Q: How can I resolve the error? A: Adjust the index to a valid value within the range of the sequence or utilize appropriate data structures and functions provided by libraries like Pandas. Q: What are the potential implications of this error? A: If not handled properly, this error can lead to unexpected program behavior, incorrect results, and program crashes. Q: What are some best practices to avoid this error? A: Validate index values, utilize appropriate data structures and libraries, implement error handling techniques, and conduct thorough testing of your code.
How To Fix Index Error: Single Positional Indexer Is Out Of Bounds
What Does Single Positional Indexer Is Out Of Bounds Mean?
If you have ever encountered the error message “Single positional indexer is out of bounds” while working with data in Python, you might have wondered what it actually means. This error usually occurs when you are trying to access a value from a series or dataframe using an index that is out of range. In this article, we will delve into this error message, its possible causes, and the steps to resolve it.
Understanding the Error Message:
The error message “Single positional indexer is out of bounds” signifies that the index you are trying to access does not exist within the range of available indices. In simpler terms, you are attempting to access a position in a series or dataframe that does not have a valid index associated with it.
Causes of the Error:
There could be several reasons behind encountering this particular error message. Let’s explore some common scenarios:
1. Incorrect Indexing Range:
One possible cause is specifying an index value that is beyond the range of indices present in the series or dataframe. For example, if you have a series with 5 elements and you try to access the 6th element, you will encounter this error.
2. Zero-based Indexing:
Another common cause is the confusion between zero-based indexing and positional indexing. In Python, indexing starts from 0, meaning the first element of a series or dataframe has an index of 0, the second element is at index 1, and so on. If you mistakenly use a position-based index starting from 1 instead of 0, this error will occur.
3. Missing Values:
Sometimes, the error might occur if the series or dataframe has missing values, resulting in a gap in the index range. Accessing these missing indices will lead to the “Single positional indexer is out of bounds” error.
4. Mismatched Indexes:
If you are trying to merge or perform operations on multiple series or dataframes with misaligned indexes, this error can occur. It is crucial to ensure that the indices are compatible before attempting any index-based operations.
How to Resolve the Error:
Now that we understand the possible causes, let’s look at some ways to resolve the “Single positional indexer is out of bounds” error:
1. Check Index Range:
Double-check the range of indices available in the series or dataframe. Ensure that the index you are trying to access falls within this range. Remember that indexing starts from 0 in Python, so adjust accordingly.
2. Handle Missing Values:
If there are missing values in the series or dataframe, it is vital to handle them appropriately. You can either fill the missing values using techniques like forward-fill, backward-fill, or interpolation, or you can drop the missing values altogether.
3. Align Indexes:
When working with multiple series or dataframes, ensure that their indexes align correctly. Use the “reindex” function to align the indexes before performing any operations.
4. Verify Index Types:
Make sure that the index type is consistent throughout the series or dataframe. If you come across index types that are incompatible, consider converting them to a compatible type for seamless operations.
FAQs:
Q1. I’m receiving the error even if my index seems valid, what could be the issue?
It is possible that despite having a valid index range, there might be a mismatch between the index labels and their corresponding values. Recheck if the indices are correctly associated with their respective values.
Q2. Can this error occur when using non-integer indexes?
Yes, this error can occur with non-integer indexes as well. Any type of index, be it integers, strings, or dates, can lead to this error if the index value you are trying to access is out of bounds.
Q3. I’m still unable to fix the error. What should I do?
If you have exhausted all the options mentioned above and are still unable to resolve the error, it might be helpful to consult online forums or seek assistance from experienced programmers. Sharing your code and the specific error message can provide further insights into the issue.
In conclusion, the “Single positional indexer is out of bounds” error occurs when you try to access an index that does not exist within the range of available indices. This can result from various causes, such as incorrect indexing range, confusion between zero-based and positional indexing, missing values, or mismatched indexes. By following the recommended steps to resolve the error, you should be able to overcome this obstacle and continue working with your data effectively.
How Does Iloc Work In Python?
Python is a powerful and popular programming language known for its simplicity and ease of use. It provides a wide range of tools and functions to manipulate and analyze data efficiently. One such tool is the ILOC function, which stands for index location and allows users to access specific rows and columns within a DataFrame or a Series object. In this article, we will explore the intricacies of ILOC in Python, understand its syntax and usage, and delve into some common FAQs related to this topic.
Understanding ILOC:
ILOC is used in Python to select specific rows and columns within a DataFrame or a Series object. It provides a way to access data based on its integer position, disregarding any row labels or column names. This method is particularly useful when dealing with large datasets or when we don’t want to rely on labels for data retrieval.
Syntax of ILOC:
The syntax for ILOC in Python is as follows:
`iloc[row, column]`
The `row` parameter denotes the row(s) we want to select, while the `column` parameter represents the column(s) we want to select. Both parameters can take either a single integer value or a range of integers separated by a colon.
Basic Usage of ILOC:
To demonstrate the basic usage of ILOC, consider the following example:
“`
import pandas as pd
data = {‘name’: [‘John’, ‘Alice’, ‘Bob’, ‘Emily’],
‘age’: [25, 30, 35, 40],
‘city’: [‘New York’, ‘London’, ‘Paris’, ‘Sydney’]}
df = pd.DataFrame(data)
# Accessing a single row using ILOC
row1 = df.iloc[1]
print(row1)
# Accessing a single value using ILOC
value = df.iloc[2, 1]
print(value)
# Accessing a range of rows and columns using ILOC
subset = df.iloc[0:2, 1:3]
print(subset)
“`
In this example, we create a DataFrame using a Python dictionary. We then use ILOC to access different rows and columns within the DataFrame. The first use of ILOC selects the second row, the second use selects a specific value at the intersection of the third row and the second column, and the third use selects a subset of rows and columns based on their respective indices.
Advanced Usage of ILOC:
ILOC provides advanced functionalities to retrieve more complex data from a DataFrame or a Series object. It allows users to access data using boolean conditions, integer indexing, and even combination with other indexing methods. Let’s explore some of these advanced concepts:
1. Using boolean conditions:
ILOC can be combined with boolean conditions to select specific data based on certain criteria. For example:
“`python
# Selecting rows based on a condition
rows = df.iloc[df[‘age’] > 30]
# Selecting specific columns based on a condition
columns = df.iloc[:, df.columns.get_loc(‘age’)]
“`
In the first line, rows are selected where the age is greater than 30. The second line selects the ‘age’ column using its name.
2. Integer indexing:
ILOC allows users to select data using integer indices directly. This can be used to retrieve data based on specific positions. For example:
“`python
# Selecting rows using integer indices
specified_rows = df.iloc[[0, 2, 3]]
# Selecting columns using integer indices
specified_columns = df.iloc[:, [0, 2]]
“`
In the first line, the rows at positions 0, 2, and 3 are selected, while in the second line, columns at positions 0 and 2 are selected.
Frequently Asked Questions (FAQs):
Q1. Can ILOC work with both DataFrames and Series objects?
Yes, ILOC can be used with both DataFrames and Series objects. It allows users to select specific rows and columns within a DataFrame, and it enables the selection of specific values within a Series.
Q2. How is ILOC different from LOC?
While ILOC works with integer positions, LOC allows data retrieval based on labels rather than integer positions. LOC is used when we want to access data based on column or row labels.
Q3. Can I use ILOC to modify data in a DataFrame?
No, ILOC is primarily used for data retrieval and does not modify the actual data in a DataFrame. If you want to modify data, you can use the ILOC function in combination with other methods like `at` or `iat`.
Q4. Is it possible to use ILOC with multidimensional arrays?
No, ILOC is specifically designed for DataFrames and Series objects. However, you can convert your multidimensional array into a DataFrame and then use ILOC on it.
Q5. Can I use negative indices with ILOC?
No, ILOC only works with positive integers. Negative indexing is not supported with this method.
In conclusion, ILOC is a powerful tool in the Python programming language that allows users to retrieve specific rows and columns from a DataFrame or a Series based on their integer positions. It offers a flexible syntax and can be combined with other indexing techniques to perform complex data manipulations. By understanding the syntax and usage of ILOC, you can effectively leverage this functionality to analyze and manipulate data in Python.
Keywords searched by users: single positional indexer is out-of-bounds IndexError positional indexers are out-of-bounds, Index 0 is out of bounds for axis 0 with size 0, Iloc 1, Iloc Pandas, Too many indexers, Pandas loc and iloc, Get index of row pandas, Assign value to DataFrame
Categories: Top 90 Single Positional Indexer Is Out-Of-Bounds
See more here: nhanvietluanvan.com
Indexerror Positional Indexers Are Out-Of-Bounds
In the realm of programming, errors occur frequently, and one such error is the IndexError: positional indexers are out-of-bounds. This error message is commonly encountered by developers, especially when working with data structures that support indexing. In this article, we will delve into the details of this error, its causes, and potential solutions, providing a comprehensive understanding of this topic.
Understanding IndexError: positional indexers are out-of-bounds
The IndexError: positional indexers are out-of-bounds is an error message raised when attempting to access an element in a data structure using an index that surpasses the boundaries of the structure. In simpler terms, it occurs when the index provided to retrieve an element from a list, array, or similar data structure is outside the permissible range.
Causes of the IndexError
This error commonly arises due to a few key reasons:
1. Out-of-range index: The most common cause is trying to access an index that exceeds the range of the data structure. For example, if you have a list with five elements, indexed from 0 to 4, accessing index 5 or any higher index will result in an IndexError.
2. Misunderstanding zero-based indexing: Many programming languages, including Python, utilize zero-based indexing, where the first element is accessed using index 0. Consequently, attempting to access an element with an index of -1 or a negative value will trigger an IndexError.
3. Incorrect data structure: If the data structure itself does not support indexing or does not follow conventional indexing rules, an IndexError is likely to occur. For instance, dictionaries in Python are not accessed using numerical indices but rather keys.
Understanding the error message
When encountering the IndexError, Python provides a helpful error message that provides insight into the issue. The error message typically includes the exact line of code where the error occurred, the type of error (IndexError), and a description indicating that positional indexers are out-of-bounds. This message serves as a useful starting point for troubleshooting.
Handling and preventing IndexError
To handle the IndexError: positional indexers are out-of-bounds error, several strategies can be employed.
1. Double-check the indices: The primary step should always be to review and validate the indices being utilized. Ensure they fall within the correct range for the given data structure. Be cautious of off-by-one errors, as they can often cause these issues.
2. Verify the length of the data structure: If you are unsure of the actual length or number of elements in the data structure, acquire this information explicitly. This can be achieved using built-in functions or methods provided by the programming language. By comparing indices against the correct data structure length, you can avoid IndexError.
3. Understand the data structure’s indexing rules: Different data structures have distinct indexing rules, and it is crucial to comprehend them correctly. For example, lists typically use numerical indices, whereas dictionaries utilize keys. Familiarize yourself with the specific indexing rules to ensure proper usage.
4. Implement error handling mechanisms: To prevent abrupt crashes, it is advisable to include exception handling mechanisms in your code. By wrapping index-related operations in a try-except block, you can catch the IndexError and handle it gracefully without stopping program execution abruptly.
5. Debugging and testing: Debugging tools and techniques can be highly effective in identifying and resolving IndexError occurrences. Employ debuggers, breakpoints, and testing frameworks to pinpoint the exact location and cause of the error. This systematic approach can save considerable time and effort.
FAQs:
1. Why do positional indexers go out-of-bounds?
Positional indexers go out-of-bounds when the index value used to access an element exceeds the permissible range of the data structure. This can commonly occur due to confusion with zero-based indexing, incorrect length calculations, or misunderstanding the indexing rules of a particular data structure.
2. Can an IndexError appear with any programming language?
No, the IndexError is specific to programming languages that support indexing of data structures. Most high-level programming languages, such as Python, Java, and C++, utilize indexing, making the IndexError a common occurrence in these languages.
3. How do I fix an IndexError?
To fix an IndexError, you should verify that the indices being used fall within the appropriate range for the data structure. Additionally, understand the indexing rules of the data structure and consider implementing error handling mechanisms to gracefully handle this error.
4. Are there programming tools to help detect and resolve IndexError?
Yes, modern programming tools offer various features to assist in detecting and resolving IndexError. Integrated development environments (IDEs), debuggers, and testing frameworks are extensively used to identify the precise location and cause of the error. These tools enable developers to address the issue efficiently.
In conclusion, the IndexError: positional indexers are out-of-bounds error is a common stumbling block for programmers working with indexed data structures. By understanding its causes and following the recommended solutions discussed in this article, developers can effectively handle and prevent this error. Being diligent with indices, understanding the data structure’s indexing rules, and implementing appropriate error handling mechanisms can significantly reduce the occurrence of this error, leading to more robust and reliable code.
Index 0 Is Out Of Bounds For Axis 0 With Size 0
Understanding the Error:
When we encounter the “Index 0 is out of bounds for axis 0 with size 0” error, it means that we are trying to access or reference an element in a data structure that does not exist. Specifically, the error indicates that we are attempting to access the element at index 0, but the size of the data structure is 0, which means it is empty and does not contain any elements.
Possible Causes:
1. Empty Data Structure: The most common cause of this error is when we try to access an element in an empty data structure. This can happen when we forget to populate the structure with elements, or if there is an error in our code that is preventing the insertion of elements.
2. Incorrect Indexing: Another cause of this error is incorrect indexing of the data structure. In Python, indexing starts from 0, so if we try to access an element at index 0 in a data structure, but mistakenly assume it starts from index 1, we will encounter this error.
3. Incorrect Dimension: This error can also occur when working with multi-dimensional data structures, such as matrices or arrays. If we attempt to access an element at index 0 on an axis that does not exist, or if the size of the dimension is 0, this error will be raised.
Resolving the Error:
To resolve the “Index 0 is out of bounds for axis 0 with size 0” error, we need to address the root cause. Here are some steps to follow:
1. Verify Data Structure: Double-check if the data structure is empty. If it is, ensure that you have included the necessary code to populate it with elements. If not, revise your code to add elements before attempting to access them.
2. Review Indexing: Check your indexing logic. Remember that indices in Python start from 0, so if you mistakenly assume they start from 1, revise your code accordingly. Ensure that you are using the correct index values to access elements in the data structure.
3. Dimension Issues: If you are working with multi-dimensional data structures, make sure you are accessing elements within valid dimensions. Check the size of each dimension and ensure it is not 0. If necessary, adjust the code to correctly specify the appropriate indices for accessing elements within the desired dimensions.
Frequently Asked Questions (FAQs):
Q1. Why am I getting the “Index 0 is out of bounds for axis 0 with size 0” error?
A1. This error occurs when you are trying to access an element at index 0 in a data structure that is empty or has a size of 0. Review your code to ensure that the data structure is populated with elements before attempting to access them.
Q2. How can I fix the “Index 0 is out of bounds for axis 0 with size 0” error?
A2. To resolve this error, you need to check if the data structure you are trying to access has elements. If it is empty, add elements to it before accessing them. Additionally, review your indexing approach to ensure you are using the correct indices to access the desired elements.
Q3. I am getting this error while working with a multi-dimensional data structure. How can I resolve it?
A3. When dealing with multi-dimensional data structures like matrices or arrays, you must consider the size of each dimension. If the size of a dimension is 0 or the dimension itself does not exist, you will encounter this error. Make sure you are accessing elements within valid dimensions and adjust your code accordingly.
Q4. Can this error occur in programming languages other than Python?
A4. While the specific error message “Index 0 is out of bounds for axis 0 with size 0” is unique to Python and certain Python libraries like NumPy, similar error messages related to out-of-bounds access or empty data structures can occur in other programming languages as well.
Closing Thoughts:
The “Index 0 is out of bounds for axis 0 with size 0” error message can be frustrating to encounter, but by understanding its causes and following the steps outlined in this article, you can effectively resolve the issue. Remember to thoroughly examine your code, ensure the data structure is properly populated, and double-check your indexing approach to access the desired elements accurately.
Iloc 1
Located in the northernmost part of Luzon Island in the Philippines lies the incredible province of Ilocos Norte. Known for its rich history, vibrant culture, breathtaking landscapes, and warm hospitality, Ilocos Norte has become a beloved destination for both local and international travelers. Among the many wonders that this province offers, one stands out prominently — Iloc 1, the iconic wind farm of Ilocos Norte.
Iloc 1, also referred to as the Bangui Windmills, is a mesmerizing sight that dominates the coastline of Bangui Bay. With a total of 20 colossal turbines lined up along the shore, it is the first-ever wind farm in the Philippines, inaugurated in 2005. Each windmill stands at an impressive height of 70 meters and has three towering propeller-like blades, stretching out to a diameter of 41 meters. The farm’s towers are engineered to face the prevailing wind direction, which allows them to harness the maximum amount of wind energy.
The windmills of Iloc 1 are not only a marvel of modern engineering but also a symbol of sustainability and clean energy. These impressive structures generate an enormous amount of electricity, providing approximately 40% of the power supply for Ilocos Norte. With its capacity to generate 33-megawatts of clean, renewable energy, the farm plays a significant role in reducing the province’s dependence on fossil fuel-based power sources and combating climate change.
Beyond its contribution to green energy, Iloc 1 has become an iconic landmark that attracts countless tourists every year. The windmills have transformed the previously barren coastal area into an awe-inspiring spectacle. The vast expanse of the wind farm against the backdrop of blue skies and the majestic Bangui Bay creates a truly mesmerizing view. Visitors often find themselves captivated by the sight, snapping photos, and marveling at the grandeur of these towering structures.
Exploring Iloc 1 allows visitors to not only admire the windmills from a distance but to get up close and personal with these giants of the sky. A road has been specially constructed that runs parallel to the wind farm, allowing tourists to have an unobstructed view of the turbines. Walking along this path while feeling the mighty gusts of wind and hearing the gentle hum of the spinning blades is an experience like no other. It enables visitors to truly appreciate the monumental scale of Iloc 1 and the leaps humanity has taken in renewable energy technology.
In recent years, Iloc 1 has become an incredibly popular destination for both local and international tourists. Many travelers flock to this wind farm to witness the natural beauty and grace of these mammoth structures. The wind farm also serves as an educational hub, offering visitors the opportunity to learn about renewable energy and the importance of sustainable practices.
To cater to the increasing number of visitors, several amenities have been developed in the area surrounding Iloc 1. These include souvenir shops, restaurants serving local delicacies, and picnic areas where families and friends can gather to enjoy the stunning view while having a sumptuous meal. Additionally, various guides and tour operators offer services to help visitors explore and understand the entire wind farm complex better.
FAQs:
Q: When is the best time to visit Iloc 1?
A: The wind farm is open year-round, but the best time to visit is during the dry season, which runs from November to April. During these months, visitors can enjoy clear skies and favorable weather conditions.
Q: How can I reach Iloc 1?
A: Iloc 1 is easily accessible by land. From Laoag City, the capital of Ilocos Norte, it takes approximately an hour’s drive to reach the wind farm. Public transportation such as buses and vans are available, or visitors can also opt to hire a private vehicle.
Q: Are there any fees to enter the wind farm?
A: There are no entrance fees to visit Iloc 1. However, visitors are encouraged to support the local economy by purchasing souvenirs, food, and services from the nearby shops and restaurants.
Q: Can I take photos at Iloc 1?
A: Yes, photography is allowed and even encouraged. The wind farm provides endless opportunities for stunning photos. Just make sure to be respectful and not interfere with the operation of the windmills.
Q: Are there any other attractions nearby?
A: Yes, there are several other attractions near Iloc 1, including Kapurpurawan Rock Formation, Pagudpud Beach, and the historic Paoay Church. These sites are worth exploring while visiting the province of Ilocos Norte.
In conclusion, Iloc 1, with its remarkable windmills and commitment to clean energy, has become an emblem of pride for the Ilocano people and a must-visit destination for travelers. Not only does it showcase the beauty of renewable energy, but it also offers an unforgettable experience, immersing visitors in the magnificence of these colossal wind turbines. So, if you find yourself in the northern part of the Philippines, make sure to include Iloc 1 on your itinerary and witness the grandeur of this engineering marvel.
Images related to the topic single positional indexer is out-of-bounds
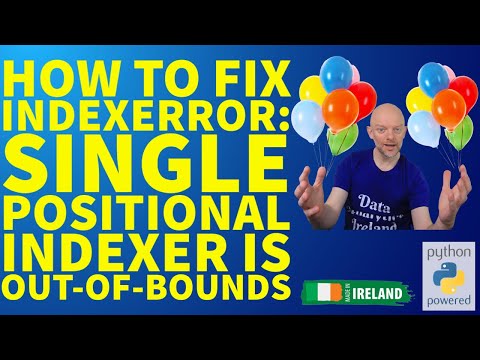
Found 22 images related to single positional indexer is out-of-bounds theme
.jpg)
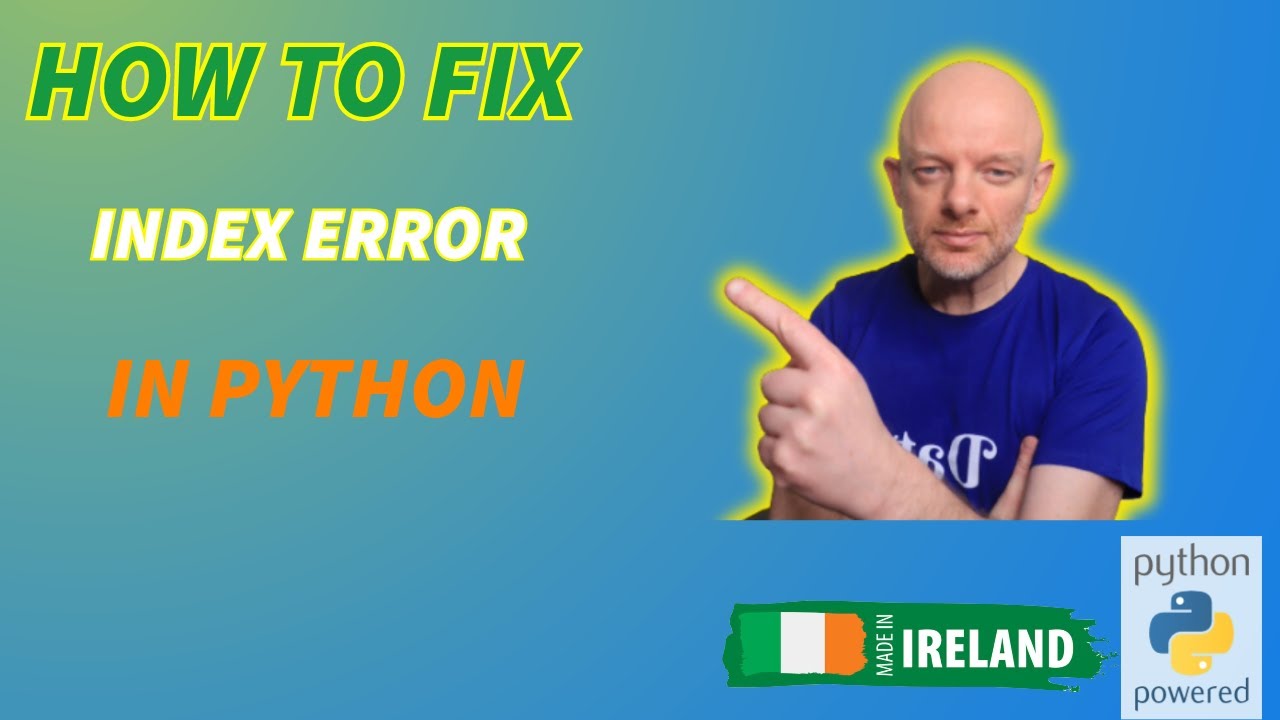
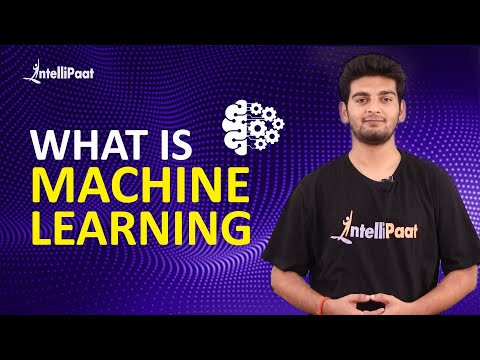
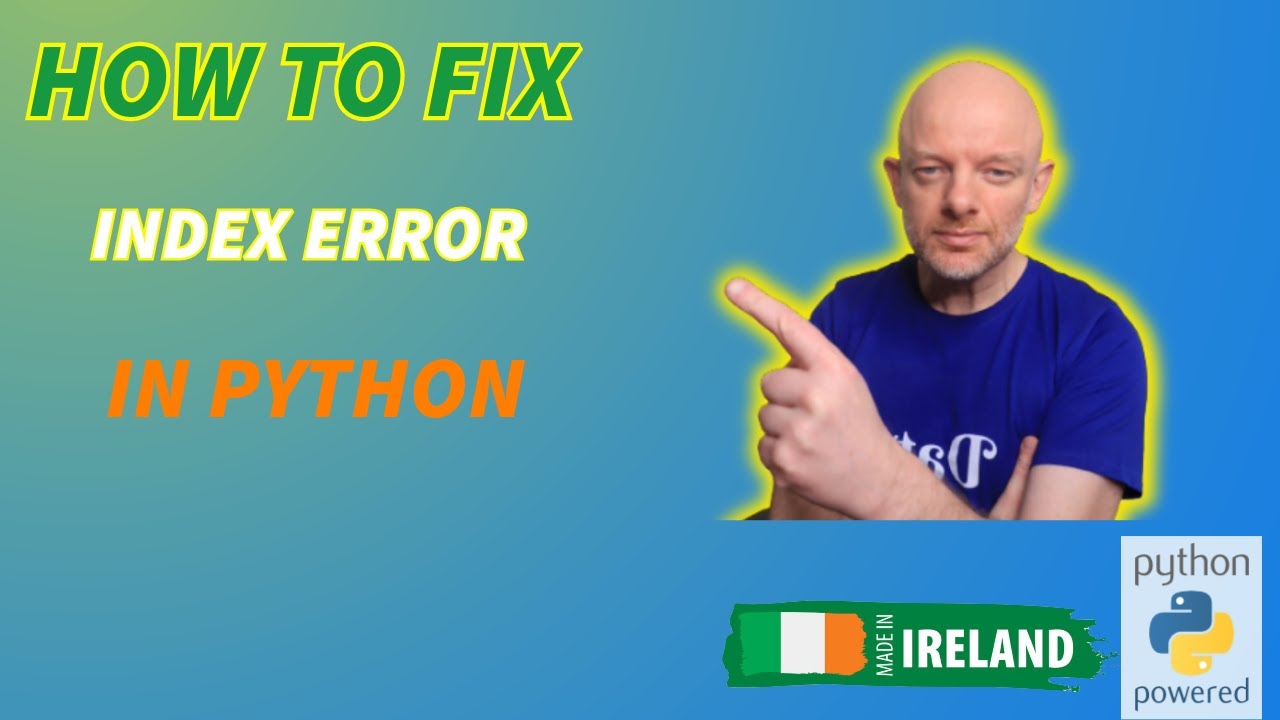
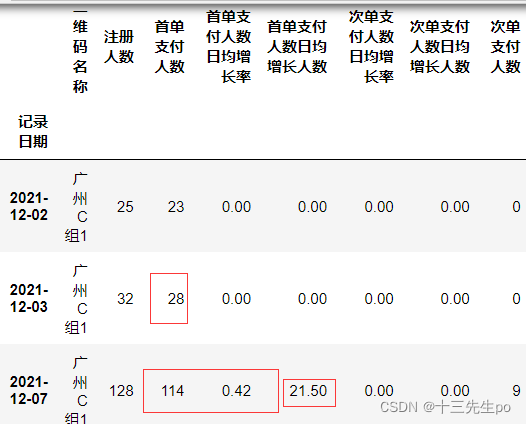
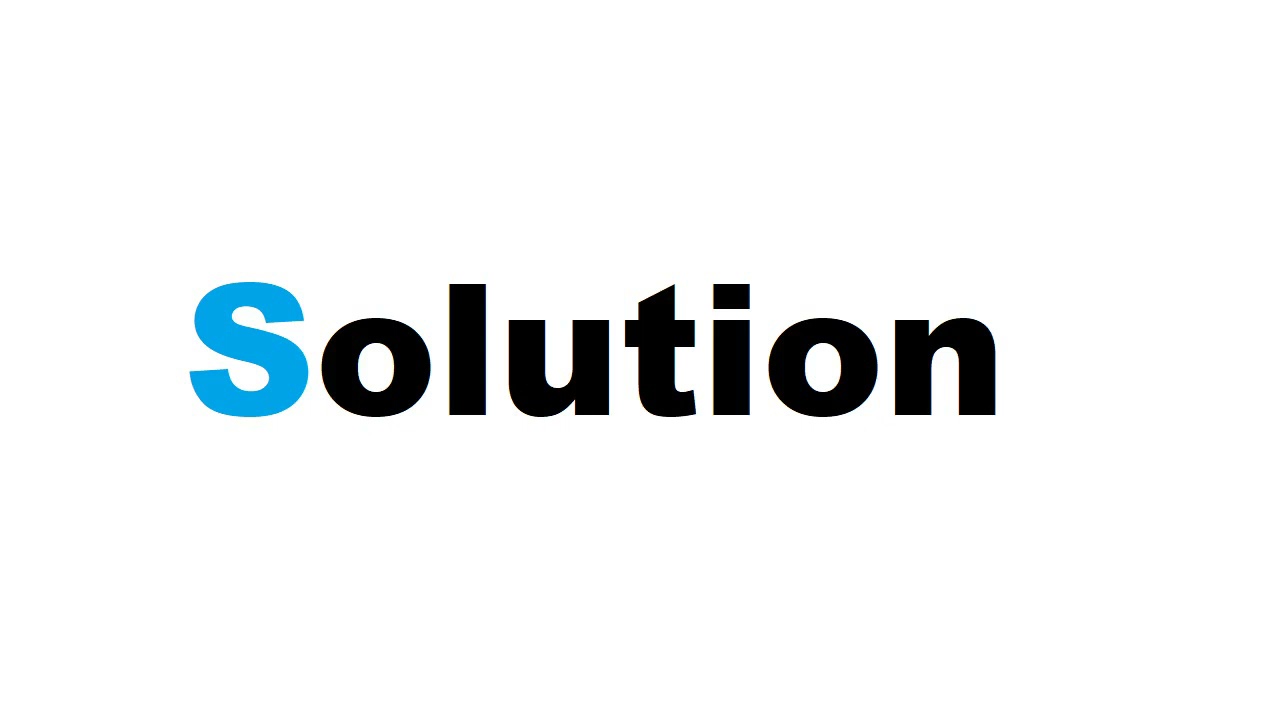
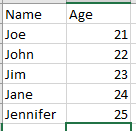


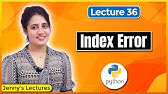
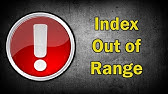

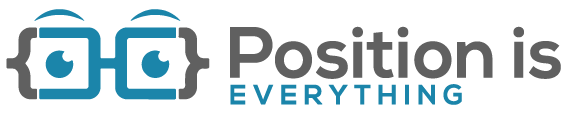
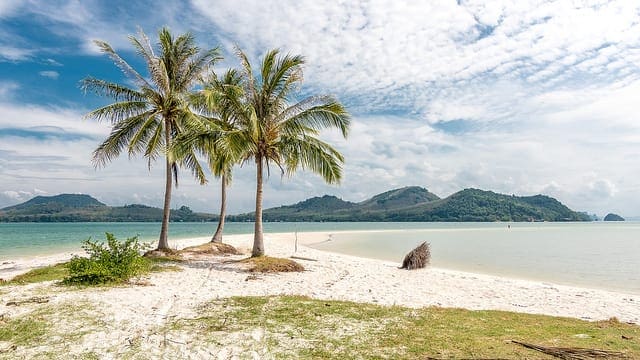


Article link: single positional indexer is out-of-bounds.
Learn more about the topic single positional indexer is out-of-bounds.
- iloc giving ‘IndexError: single positional indexer is out-of-bounds’
- indexerror: Single positional indexer is out-of-bounds Error
- iloc giving ‘IndexError: single positional indexer is out-of-bounds’
- iloc() Function in Python – PrepBytes
- IndexError: single positional indexer is out-of-bounds –
- iloc giving \’IndexError: single positional indexer is out-of …
- IndexError: Single positional indexer is out-of-bounds Fix
See more: https://nhanvietluanvan.com/luat-hoc/