Sql Server If Table Exists Drop
Checking if a Table Exists in SQL Server
There are several ways to check if a table exists in SQL Server. We will cover four commonly used methods in this section.
1. Using the sys.tables Catalog View to Check Table Existence:
The sys.tables catalog view is a system view that contains metadata about tables in the current database. You can use a simple SELECT statement along with the WHERE clause to check if a table exists in this view.
“`sql
IF EXISTS (SELECT 1 FROM sys.tables WHERE name = ‘YourTableName’)
BEGIN
— Table exists, do something
END
“`
2. Using the IF EXISTS Statement with the sys.objects Catalog View:
The sys.objects catalog view contains metadata about all objects in the current database, including tables. You can use the IF EXISTS statement along with the OBJECT_ID function to check if a table exists.
“`sql
IF EXISTS (SELECT 1 FROM sys.objects WHERE object_id = OBJECT_ID(N’dbo.YourTableName’) AND type = N’U’)
BEGIN
— Table exists, do something
END
“`
3. Using the INFORMATION_SCHEMA.TABLES View to Check Table Existence:
The INFORMATION_SCHEMA.TABLES view provides information about tables in the current database. Similar to the previous methods, you can use a SELECT statement along with the WHERE clause to check table existence.
“`sql
IF EXISTS (SELECT 1 FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_NAME = ‘YourTableName’)
BEGIN
— Table exists, do something
END
“`
4. Using the sys.sysobjects System Catalog View to Check Table Existence:
The sys.sysobjects system catalog view is another way to check if a table exists in SQL Server. You can use a SELECT statement with the WHERE clause to check table existence.
“`sql
IF EXISTS (SELECT 1 FROM sys.sysobjects WHERE name = ‘YourTableName’ AND xtype = ‘U’)
BEGIN
— Table exists, do something
END
“`
Dropping a Table if It Exists Using the DROP TABLE Statement:
Once you have determined that a table exists, you can use the DROP TABLE statement to remove it from the database. The DROP TABLE statement completely deletes the table and all its associated objects, such as indexes, constraints, and triggers.
“`sql
IF EXISTS (SELECT 1 FROM sys.tables WHERE name = ‘YourTableName’)
BEGIN
DROP TABLE YourTableName
END
“`
Using the sp_MSforeachtable System Stored Procedure to Drop Tables:
The sp_MSforeachtable system stored procedure allows you to perform an operation on each table in the current database. You can utilize this procedure to drop all tables in one go, regardless of whether they exist or not.
“`sql
EXEC sp_MSforeachtable ‘DROP TABLE ?’
“`
Handling Errors and Exceptions when Dropping a Table if It Exists:
When dropping a table, it is important to handle any potential errors or exceptions that may occur. The following example demonstrates how to use the TRY…CATCH block to catch and handle any errors that occur during the table dropping process.
“`sql
BEGIN TRY
IF EXISTS (SELECT 1 FROM sys.tables WHERE name = ‘YourTableName’)
BEGIN
DROP TABLE YourTableName
END
END TRY
BEGIN CATCH
— Handle errors or exceptions here
SELECT ERROR_MESSAGE() AS ErrorMessage
END CATCH
“`
FAQs:
Q: What is the purpose of checking if a table exists before dropping it?
A: Checking if a table exists before dropping it helps avoid errors that may occur if the table does not exist. It allows for more reliable and error-proof code.
Q: Can I drop multiple tables at once?
A: Yes, you can use the sp_MSforeachtable stored procedure to drop multiple tables in one go.
Q: Is it possible to drop a table if it exists in multiple databases?
A: Yes, you can modify the SQL statements to include the database name to drop a table if it exists in a specific database.
Q: How can I drop a table if it exists in Oracle, PostgreSQL, or MySQL?
A: The syntax and methods for dropping tables may vary across different database systems. It is recommended to consult the respective database documentation for the correct syntax.
In conclusion, checking if a table exists before dropping it is an essential task in SQL Server. By using the different methods discussed in this article, you can ensure the smooth execution of your SQL scripts and avoid errors when dropping tables. Whether you prefer using system views, catalog views, or system stored procedures, SQL Server provides several options to handle this operation reliably and efficiently. Remember to handle errors and exceptions appropriately to maintain the integrity of your database.
Drop If Exists In Sql Server 2016 | Sql Drop If Exists
Keywords searched by users: sql server if table exists drop DROP TABLE IF EXISTS, DROP TABLE IF EXISTS SQL Server, DROP TABLE if EXISTS SQL, Drop table if exists oracle, DROP TABLE IF EXISTS postgres, DROP TABLE IF EXISTS MySQL, Drop table if exists student cascade, Execute immediate drop table if exists
Categories: Top 28 Sql Server If Table Exists Drop
See more here: nhanvietluanvan.com
Drop Table If Exists
Introduction:
In the world of database management, there are times when it becomes necessary to remove tables from a database. This is where the DROP TABLE command comes into play. However, before performing this action, it is crucial to ensure that the table exists to prevent any unforeseen errors. The DROP TABLE IF EXISTS statement comes to the rescue in such scenarios. In this article, we will explore the concept of DROP TABLE IF EXISTS in-depth, discussing its significance, syntax, and usage. Additionally, we will answer some frequently asked questions related to this topic.
Understanding DROP TABLE IF EXISTS:
The DROP TABLE IF EXISTS statement is a highly useful feature offered by the MySQL database. It allows you to delete a table from a database if it exists, without triggering any errors if the table is not present. This statement ensures that your database remains intact, preventing any accidental removal of tables or disruption of data.
Syntax of DROP TABLE IF EXISTS:
The syntax for using DROP TABLE IF EXISTS is straightforward. The basic structure of this statement is as follows:
DROP TABLE IF EXISTS table_name;
Example:
Consider a scenario where you have a database named “mydb” with a table named “customers” that you wish to delete. The SQL statement to accomplish this using DROP TABLE IF EXISTS would be:
DROP TABLE IF EXISTS mydb.customers;
Now, let us delve into the specifics and intricacies of DROP TABLE IF EXISTS in order to better understand its applications.
Usage of DROP TABLE IF EXISTS:
1. Preventing errors:
The primary purpose of using DROP TABLE IF EXISTS is to avoid errors that occur when attempting to delete a non-existent table. Without this statement, executing a DROP command on a table that does not exist would lead to an error. However, with DROP TABLE IF EXISTS, the statement ensures that no error will be thrown if the table is not found, providing a seamless experience while managing databases.
2. Automating database management tasks:
Another significant application of DROP TABLE IF EXISTS is the automation of database management tasks. Suppose you have an automated script that runs periodically to update your database schema. By including DROP TABLE IF EXISTS before creating or modifying tables, you can guarantee that your script will execute successfully even if the targeted tables have been removed or are not yet present.
3. Safer database operations:
DROP TABLE IF EXISTS provides a safety net during the execution of complex database operations. For instance, when writing scripts to import data from external sources, you may need to delete and recreate tables to ensure the data is in the appropriate format. The use of DROP TABLE IF EXISTS within such workflows can prevent accidental data loss or corruption by avoiding table deletion errors.
Frequently Asked Questions (FAQs):
Q1: What happens if I execute the DROP TABLE statement without using the IF EXISTS clause?
A1: When executing DROP TABLE without IF EXISTS, an error will occur if the table being deleted does not exist in the database. Using the IF EXISTS clause prevents such errors and allows for smoother database management.
Q2: Can I drop multiple tables using the DROP TABLE IF EXISTS statement?
A2: Yes, you can drop multiple tables in a single statement. Simply list the table names separated by commas. For example, DROP TABLE IF EXISTS table1, table2, table3;
Q3: Is there any performance impact on using DROP TABLE IF EXISTS?
A3: No, there is no significant performance impact when using the IF EXISTS clause. It simply adds an extra step to check if the table exists before performing the deletion.
Q4: Can the DROP TABLE IF EXISTS statement delete tables from different databases?
A4: Yes, it is possible to use DROP TABLE IF EXISTS to delete tables from different databases. Simply specify the database name and table name separated by a dot, such as database_name.table_name.
Conclusion:
In conclusion, the DROP TABLE IF EXISTS statement is a valuable tool in MySQL for managing tables within databases. It ensures that database operations run smoothly by preventing errors related to non-existent tables. Whether it is for automating tasks, ensuring safe database operations, or simply safeguarding against accidental deletions, DROP TABLE IF EXISTS is an indispensable feature for efficient and error-free database management.
Drop Table If Exists Sql Server
In SQL Server, a table is used to store data in a structured format and is composed of rows and columns. However, there may be situations where you need to remove a table from your database, either to replace it with a new one or to free up space. Traditionally, to drop a table, you would use the DROP TABLE statement. However, if the table does not exist, this would throw an error, disrupting the execution flow. This is where DROP TABLE IF EXISTS comes to the rescue.
The syntax for DROP TABLE IF EXISTS statement is straightforward:
“`
DROP TABLE IF EXISTS table_name;
“`
Where `table_name` refers to the name of the table you want to drop. If the table exists, it will be dropped; otherwise, nothing will happen. This statement simplifies your code, as you don’t need to write additional logic to first check if the table exists before dropping it. It ensures that your script continues without any disruption, even if the table is absent.
One of the key advantages of using DROP TABLE IF EXISTS is its ease of use and efficiency. You don’t need to write complex conditional statements or check the existence of a table using system views or other functions. The statement handles this check automatically, making your code more concise and readable. Additionally, it reduces the likelihood of errors caused by attempting to drop non-existent tables.
Another advantage is the time saved when performing repetitive tasks. When managing databases with a large number of tables or when performing routine operations such as creating and dropping temporary tables, you can easily incorporate DROP TABLE IF EXISTS into your scripts. It allows you to focus on the actual task at hand, without needing to worry about handling exceptions or writing additional logic to handle potential errors.
It’s worth noting that DROP TABLE IF EXISTS is available from SQL Server 2016 onwards. If you’re using an older version, you should consider alternative approaches, such as checking the existence of a table using system views like `sys.tables` and then conditionally dropping it.
FAQs:
Q: Does the DROP TABLE IF EXISTS statement delete the table along with its data?
A: Yes, just like the regular DROP TABLE statement, DROP TABLE IF EXISTS removes the table and its associated data from the database.
Q: What happens if the table I want to drop has constraints or dependencies?
A: DROP TABLE IF EXISTS will take care of dropping the table along with its constraints and dependencies, if any. It ensures that the table is removed completely from the database.
Q: Can I use DROP TABLE IF EXISTS to drop multiple tables at once?
A: No, the DROP TABLE IF EXISTS statement cannot be used to drop multiple tables in a single statement. You need to execute separate DROP TABLE statements for each table you want to remove.
Q: Will using DROP TABLE IF EXISTS affect other tables or objects in the database?
A: No, the statement only affects the specified table. It does not have any impact on other tables, views, stored procedures, or any other objects present in the database.
Q: How can I verify the execution result of the DROP TABLE IF EXISTS statement?
A: You can check the Messages tab in SQL Server Management Studio (SSMS) to view the execution result. If the table was dropped, you will see a confirmation message; otherwise, no message will be displayed.
In conclusion, DROP TABLE IF EXISTS is a handy SQL Server statement that simplifies the task of dropping tables from a database. It provides a straightforward syntax, eliminates the need for additional checks, and saves time when working with repetitive operations. Whether you’re a developer or a database administrator, this statement can help you efficiently manage your database tables.
Images related to the topic sql server if table exists drop
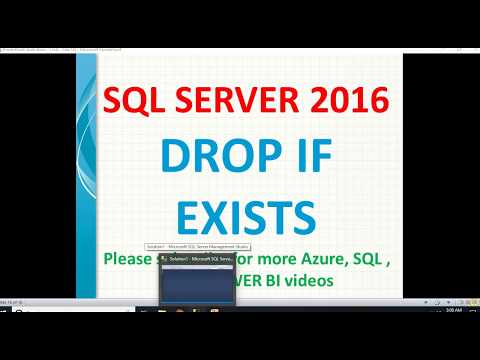
Found 48 images related to sql server if table exists drop theme
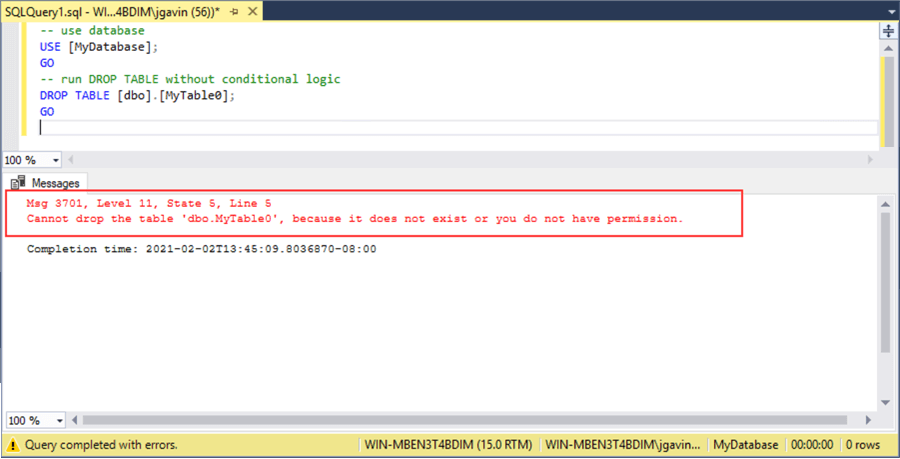
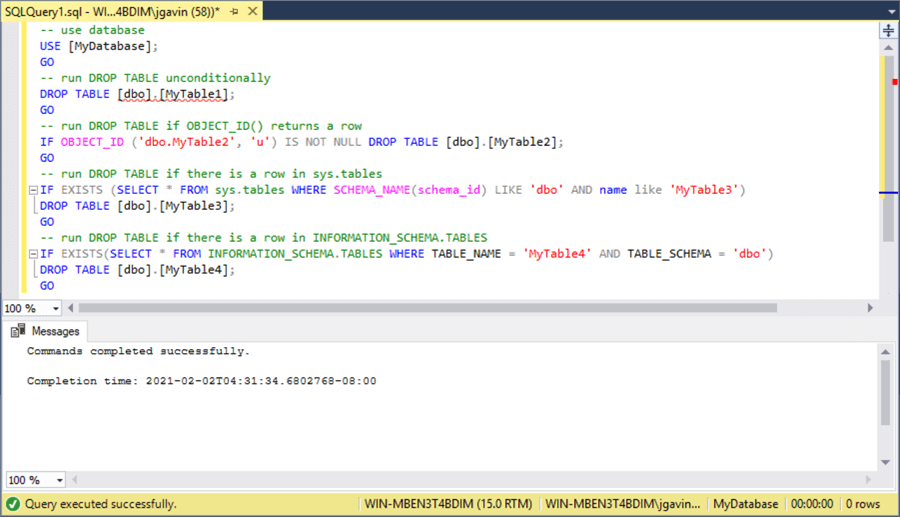

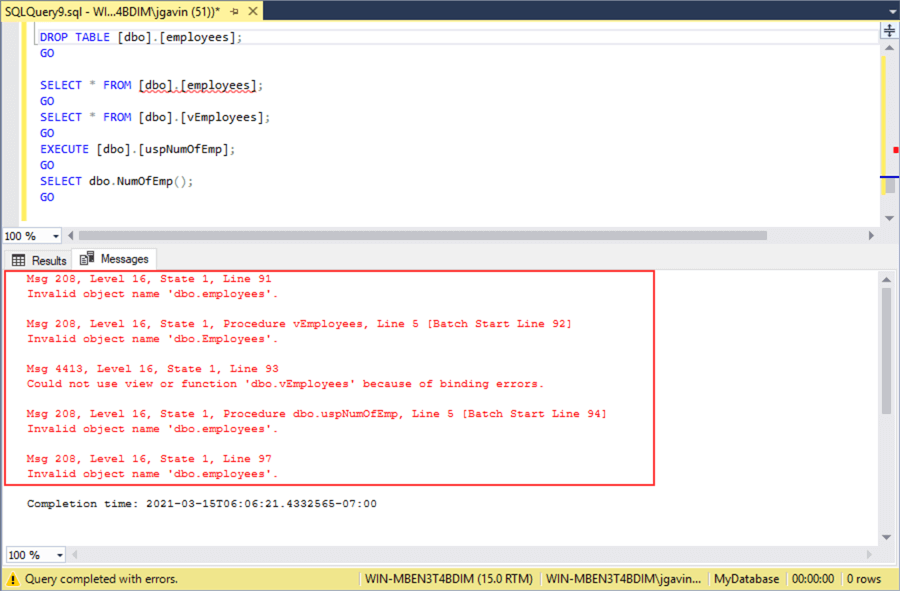

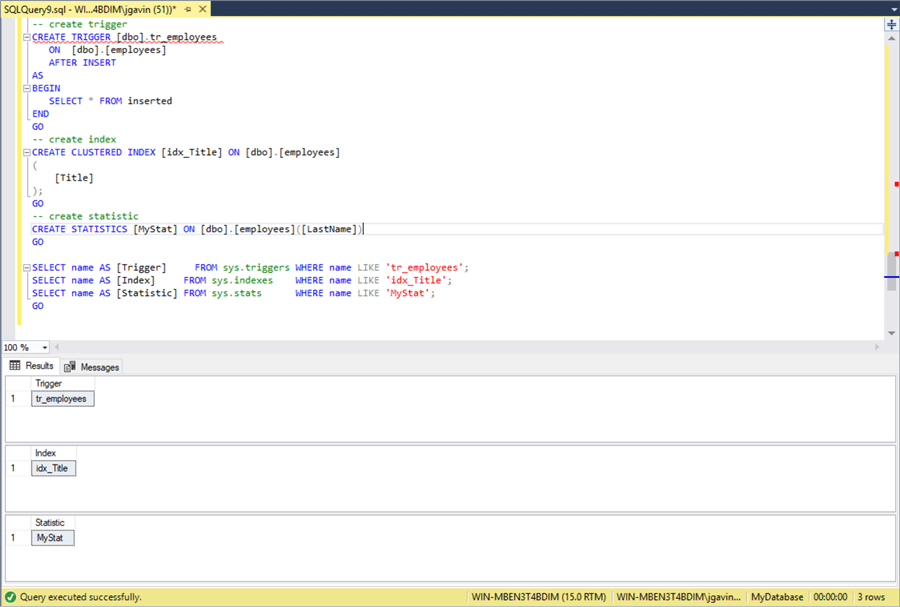
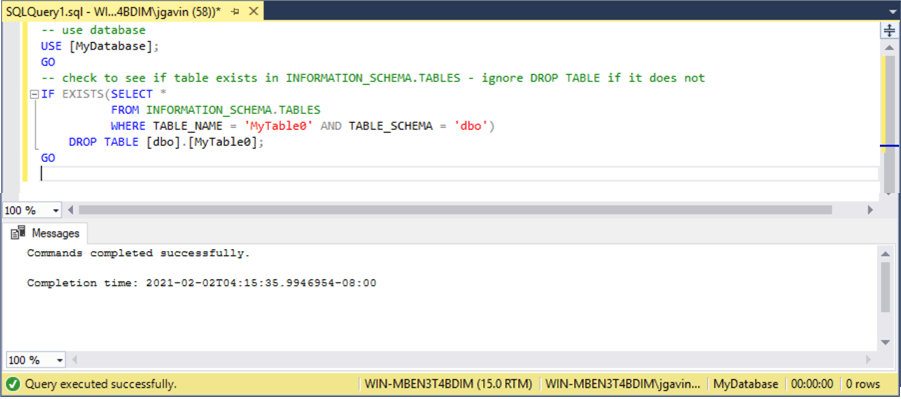
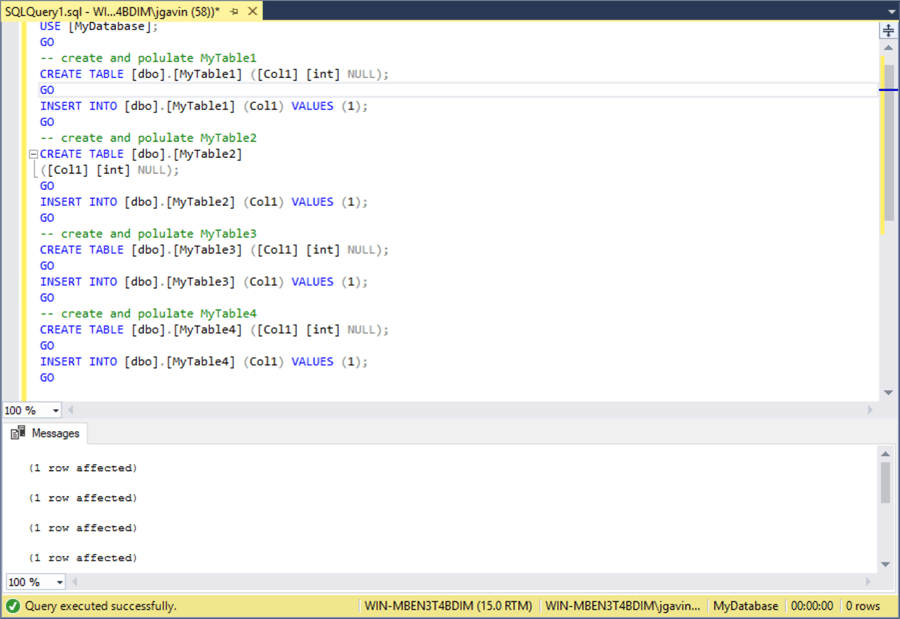




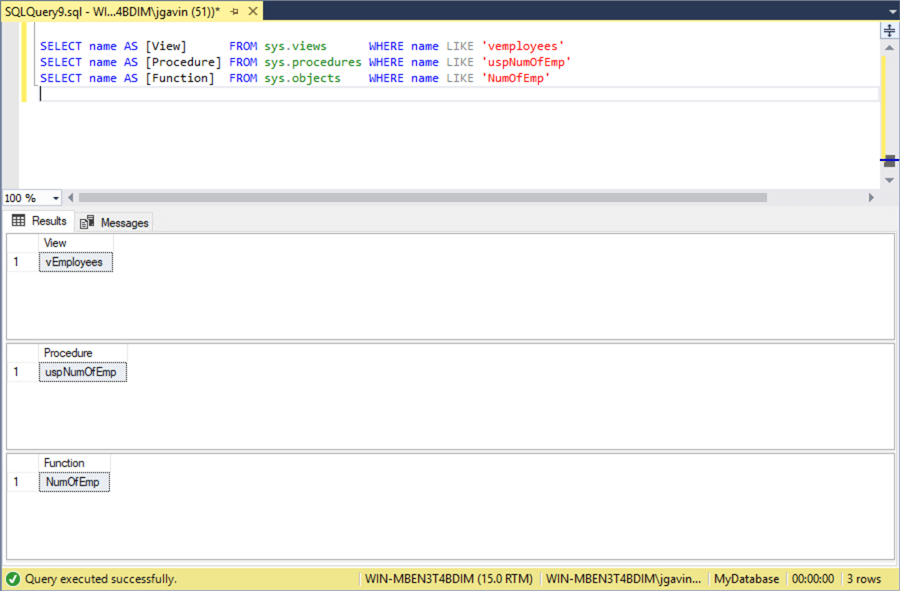

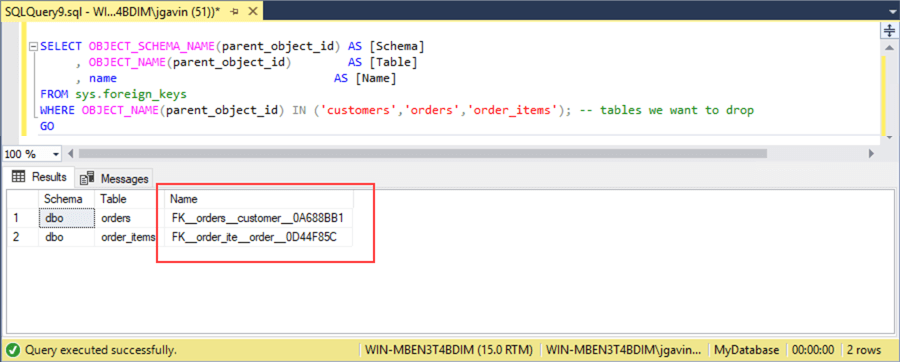


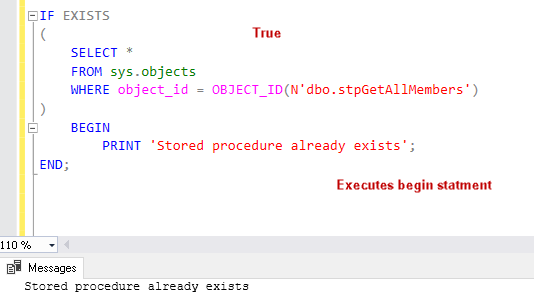




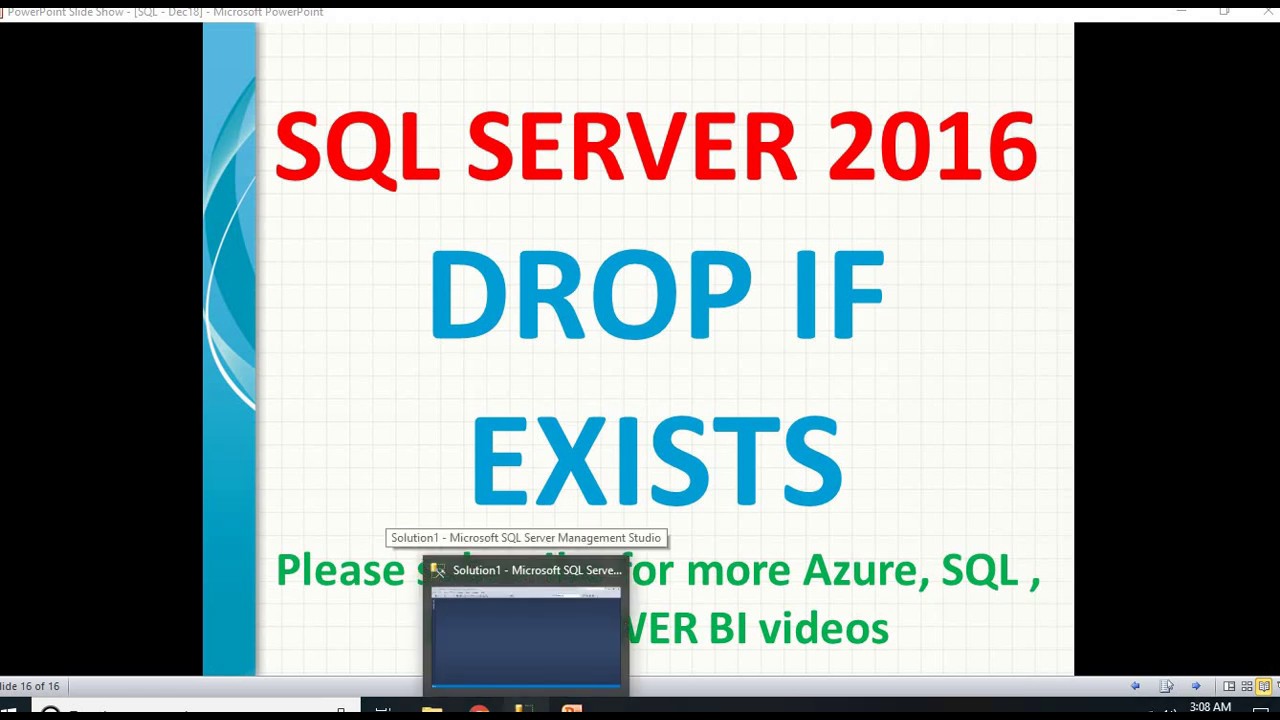
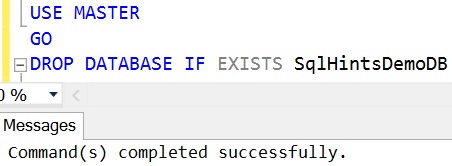
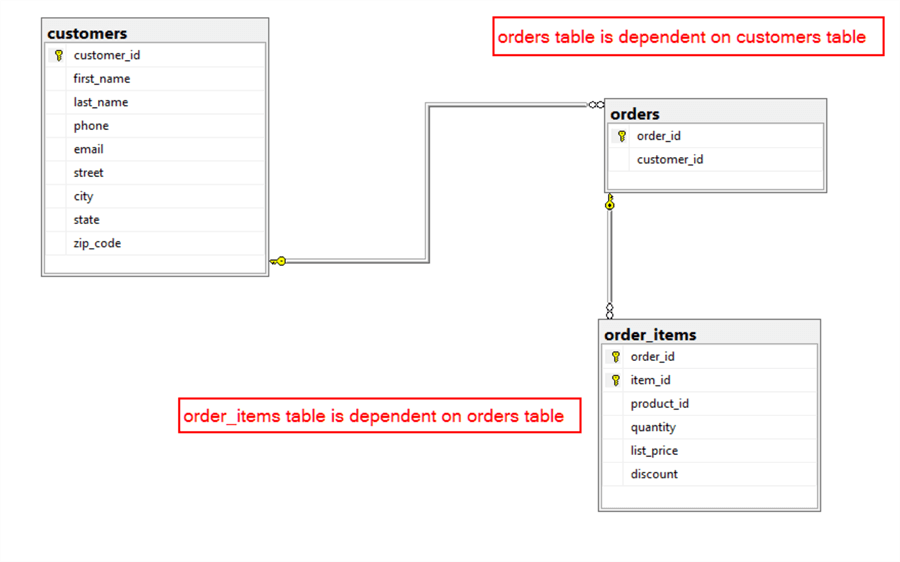

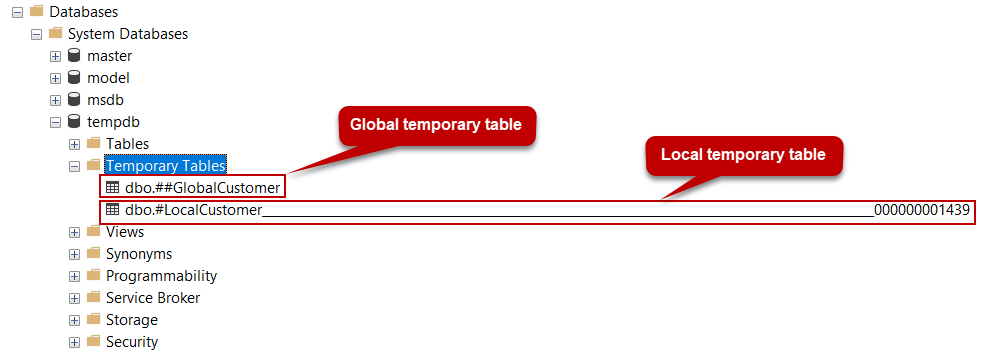
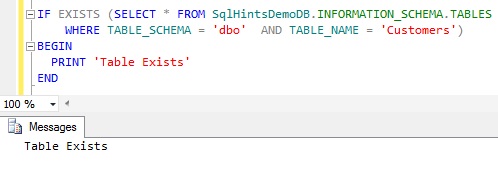
![SQL Server CREATE, ALTER, DROP Table [T-SQL Examples] Sql Server Create, Alter, Drop Table [T-Sql Examples]](https://www.guru99.com/images/1/030819_0814_SQLServerTa1.png)
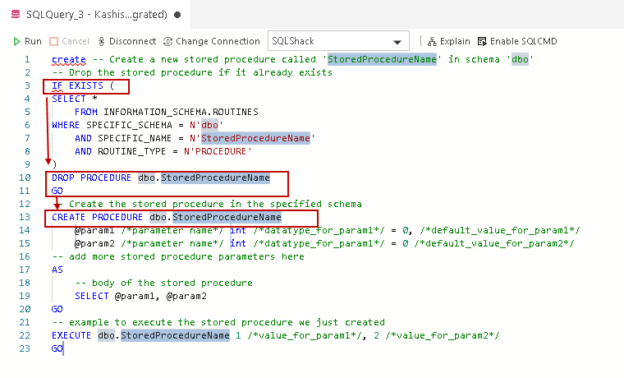

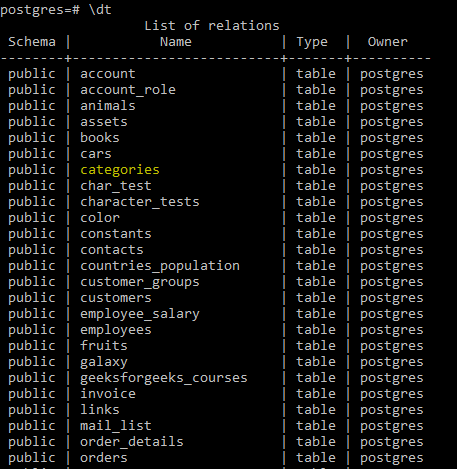
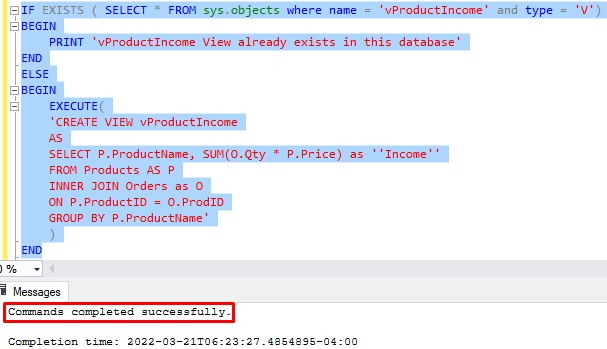







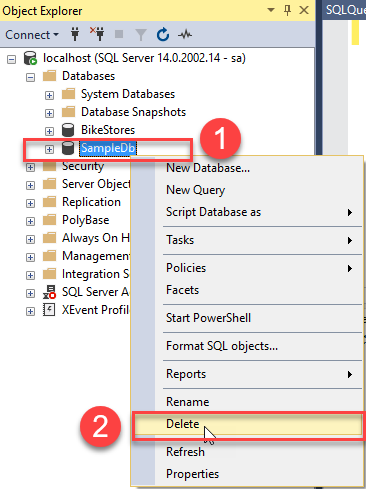
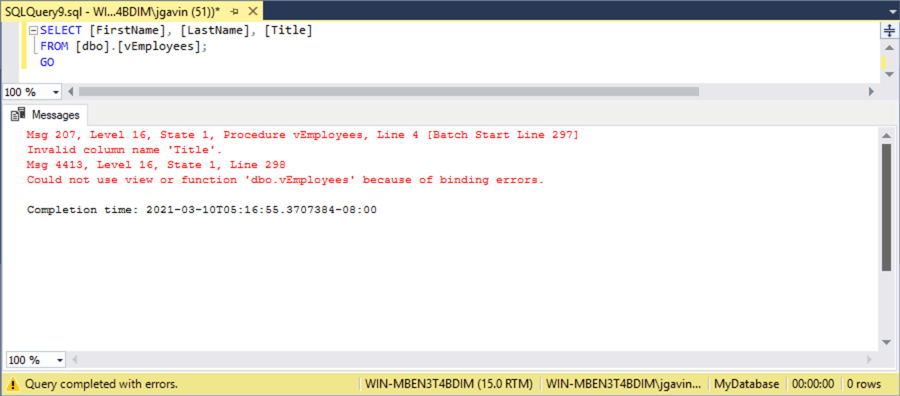

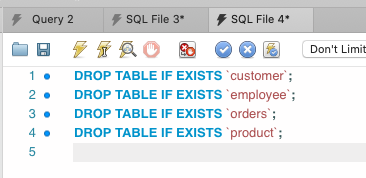


![Delete table in SQL / DROP TABLE in SQL [Practical Examples] | GoLinuxCloud Delete Table In Sql / Drop Table In Sql [Practical Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/8-1-1.png)
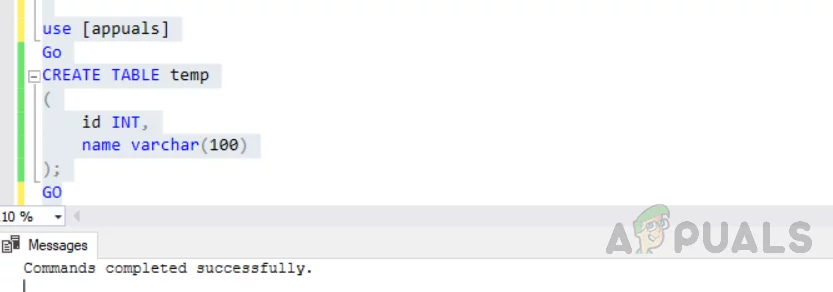
Article link: sql server if table exists drop.
Learn more about the topic sql server if table exists drop.
- SQL Server DROP TABLE IF EXISTS Examples
- sql server – How to drop a table if it exists? – Stack Overflow
- 4 Ways to Check if a Table Exists Before Dropping it in SQL …
- SQL Server If Exists Drop Table – Linux Hint
- SQL – DROP TABLE IF EXISTS Statement – Tutorial Kart
- Drop table if exists – SQL Server
- Drop Table if Exists in SQL Server – MSSQL DBA Blog
See more: https://nhanvietluanvan.com/luat-hoc/