Valueerror: I/O Operation On Closed File.
While working with files in Python, you may come across an error message stating “ValueError: I/O operation on closed file.” This error occurs when your code attempts to perform an input/output operation, such as reading from or writing to a file, that has already been closed. In this article, we will delve into the causes, troubleshooting techniques, best practices, and common mistakes related to this error to help you understand and resolve it effectively.
Causes and Scenarios Leading to a Closed File Error
There are several scenarios that can result in a closed file error. Let’s explore some common causes:
1. Forgetting to Close or Reopen the File: If you attempt to perform operations on a file that has been closed, the error arises. It is crucial to properly manage file operations and ensure that you close and reopen the file as needed.
2. Premature File Closure: If your code closes the file prematurely, i.e., before you finish reading from or writing to it, subsequent attempts to access the file will result in a closed file error. To avoid this, ensure that you close the file only after you have completed all necessary operations.
3. Incorrect File Modes: Opening files with incorrect modes can lead to closed file errors. For instance, if you attempt to write to a file opened in read-only mode, it will generate an error when you try to write to it after reading.
Troubleshooting Techniques for Resolving a Closed File Error
To resolve the closed file error, consider applying the following troubleshooting techniques:
1. Check for File Closure: Before performing any I/O operations on a file, verify that the file is open. You can use the `closed` attribute of the file object to determine if the file is closed. If it is closed, reopen it using the `open()` function.
2. Properly Manage File Opening and Closing: Ensure that you are opening and closing files at the appropriate times. Open the file only when required and close it as soon as you finish working with it. This prevents premature closures and subsequent closed file errors.
3. Verify File Modes: Double-check the file modes to ensure they are correct. If you intend to perform both reading and writing operations, open the file in the appropriate mode (e.g., “r+” for reading and writing).
Best Practices to Prevent Closed File Errors
To minimize the occurrence of closed file errors, follow these best practices:
1. Use Context Managers: Utilize the `with` statement when working with files. It automatically takes care of opening and closing the file, even in exceptional scenarios, ensuring that it is properly managed.
2. Double-check File Operations: Always verify that the file is open before performing any I/O operations. This prevents any attempts to access closed files.
3. Implement Error Handling: Incorporate proper error handling mechanisms in your code to catch and handle any exceptions related to file operations. This allows you to gracefully handle errors and prevent them from causing closed file issues.
Common Mistakes that can Result in a Closed File Error
Now that we understand the causes and troubleshooting techniques, let’s highlight some common mistakes that can lead to closed file errors:
1. Forgetting to Reopen the File: Failing to reopen a file after closing it can result in I/O operation errors on a closed file. Always ensure you reopen the file if required for any subsequent operations.
2. Incorrect Indentation: Incorrect indentation can inadvertently close files before you complete your desired operations. Pay close attention to indentation levels, especially when using loops or conditional statements.
Advanced Tips for Handling Closed File Errors Efficiently
Here are some advanced tips to further assist you in handling closed file errors efficiently:
1. Properly Dispose of File References: When you are done with a file, ensure that you properly dispose of the file reference to release system resources. This can be done by setting the file object to `None` or using the `del` statement.
2. Incorporate Logging: Implement a logging mechanism to record file operations, including opening, closing, and any potential exceptions. The logs can help in identifying the root cause of closed file errors and expedite their resolution.
3. Debugging: In case you encounter complex closed file errors, employ a debugger to analyze the state of your code and identify any problematic areas. Debuggers can provide valuable insights into the execution flow and facilitate error diagnosis.
FAQs
Q1. How can I check if a file is open or closed in Python?
To check if a file is open or closed, you can use the `closed` attribute of the file object. It returns `True` if the file is closed and `False` if it is open.
Q2. What does “ValueError: read of closed file” mean in Python?
The “ValueError: read of closed file” indicates that your code is attempting to read from a file that has already been closed. It commonly occurs when you forget to reopen the file after closing it or attempt to access a prematurely closed file.
Q3. Can a file be reopened after closing it?
Yes, a file can be reopened after closing it. However, you need to make sure you open it again using the `open()` function before attempting any I/O operations.
Q4. Is it necessary to close a file after reading it in Python?
In Python, it is considered good practice to close a file after you finish reading from or writing to it. By closing the file, you release system resources and ensure that it can be accessed by other processes.
Q5. How do I append data to a CSV file without opening and closing it repeatedly?
To append data to a CSV file without repeatedly opening and closing it, you can open the file in the append `’a’` mode. This allows you to write data to the end of the existing file without overwriting the contents.
In conclusion, understanding, troubleshooting, and preventing closed file errors is essential when working with files in Python. By following the techniques and best practices outlined in this article, you can handle these errors efficiently and ensure smooth file operations in your Python programs.
Python :Valueerror : I/O Operation On Closed File(5Solution)
Keywords searched by users: valueerror: i/o operation on closed file. ValueError: read of closed file, Close file Python, Open and close file Python, Writeline to file Python, Append CSV files Python, Check if file is open python, Io unsupportedoperation not writable json python, Python read CSV file
Categories: Top 74 Valueerror: I/O Operation On Closed File.
See more here: nhanvietluanvan.com
Valueerror: Read Of Closed File
What causes the ValueError: read of closed file error?
The most common cause of this error is attempting to read data from a file object that has already been closed. In Python, files are opened using the `open()` function which returns a file object. This file object is responsible for reading data from or writing data to the file. After the necessary operations on the file are completed, it is essential to explicitly close the file using the `close()` method. Failure to close the file can lead to memory leaks and unpredictable behavior.
When a file is closed, the association between the file object and the actual file is terminated. Hence, any subsequent attempt to read data from the file object results in the ValueError: read of closed file error. It is crucial to remember that once a file is closed, no further I/O operations should be performed on it.
Common scenarios triggering the ValueError: read of closed file error:
1. Forgetting to close a file: A common mistake made by programmers is forgetting to close a file after performing I/O operations. If data is read or written to a file, but not closed properly, subsequent attempts to read the file again will result in the ValueError: read of closed file error.
2. Attempting to read from a closed file object: Another scenario is when a file has been closed, but the program accidentally attempts to read from it. This can occur if the code execution flow is not properly structured or if there are logical errors in the program.
3. Sharing file objects between multiple functions: When multiple functions are involved in handling file I/O, it can be easy to overlook the closing of the file object. If one function closes the file, but another function tries to read from it later, the ValueError: read of closed file error is likely to occur.
How to handle the ValueError: read of closed file error:
1. Ensure proper file handling practices: Always remember to close a file explicitly using the `close()` method when you are done reading or writing to it. This is considered good programming practice and helps avoid the ValueError: read of closed file error.
2. Use context managers: Python provides a more elegant way of ensuring file closure by using context managers. The `with` statement can be used when working with files, and it automatically takes care of closing the file after the block of code is executed. This approach eliminates the need for explicitly calling the `close()` method.
Here’s an example of using the `with` statement to read data from a file:
“`python
with open(‘file.txt’, ‘r’) as file:
data = file.read()
# Process the data
“`
3. Debug your code execution flow: If you encounter the ValueError: read of closed file error, carefully analyze your code’s execution flow. Ensure that the file is not closed prematurely or attempts to read from a closed file object are not made. Using print statements or a debugger can help identify problematic areas in your code.
4. Reopen the file if needed: In some scenarios, you might need to read from a file multiple times throughout your program’s execution. In such cases, instead of closing the file after each read operation, consider reopening the file before reading the data again. This ensures that the file object is always open when you need to read from it.
FAQs:
Q1. Can I still write to a closed file?
No, once the file is closed, both reading from and writing to the file are prohibited. You will encounter the ValueError: read of closed file error if you attempt to write to a closed file object.
Q2. Is it necessary to close files opened in ‘read’ mode?
While it is necessary to close files opened in ‘write’ or ‘append’ mode to commit the changes to the file, it is good practice to close files opened in ‘read’ mode as well. Closing a file releases system resources and ensures proper memory management.
Q3. Can I reuse a closed file object after reopening it?
No, once a file object is closed, it cannot be reused directly. You need to create a new file object by reopening the file if you want to perform further I/O operations on the same file.
Q4. How do I handle the ValueError: read of closed file error when using external libraries?
When using external libraries, you should refer to their documentation to understand their file handling practices. Most well-designed libraries handle file closure automatically, reducing the chances of encountering the ValueError: read of closed file error. If you encounter such an error while using a specific library, it is recommended to seek help from the library’s support channels or forums.
In conclusion, the ValueError: read of closed file error is a common issue faced by Python programmers while working with file I/O operations. By following proper file handling practices, avoiding premature file closures, and effectively using context managers, this error can be easily mitigated. Remember to always close files when you are done with them, and be mindful of the execution flow to prevent attempts to read from a closed file object.
Close File Python
Introduction
In Python, file handling is an essential skill for any programmer. Understanding how to open, read, write, and close files is crucial in creating programs that interact with external data sources. Among these file operations, knowing how to properly close a file after use is often overlooked but is equally important. This article aims to explore the concept of closing files in Python, its significance, best practices, and common FAQs surrounding this topic.
Understanding the Importance
Closing a file is vital to ensure system resources are freed and the file itself is properly saved and organized. When a file is opened, it occupies system resources, such as memory, which are limited in capacity. Consequently, if a file is not closed correctly, it can lead to resource leakage and may eventually cause system instability or crashes.
Additionally, closing a file guarantees that all pending write operations are completed and the changes made are saved. Without proper closure, data may be lost, and subsequent operations on the file could yield unexpected results.
How to Close Files
In Python, it is necessary to follow specific steps to close a file correctly. These steps typically involve opening the file, performing necessary operations, and finally calling the close() method. The close() method is responsible for deallocating system resources used by the file and ensuring that all buffers are flushed.
To close a file, start by using the open() function to create a file object. This function accepts two arguments: the filename and the mode in which we want to open the file (e.g., read, write, append). Next, perform your desired operations on the file, such as reading or writing data. Once finished, call the close() method on the file object to properly close the file.
Best Practices for Closing Files
While the close() method is essential, the following best practices should be considered while closing files in Python:
1. Always close the file explicitly: Though Python provides automatic garbage collection, it is good practice to explicitly close files to ensure resources are released promptly.
2. Use the with statement: Python’s with statement is an elegant construct that automates file closure. It takes care of closing the file automatically, even if an exception occurs. The with statement ensures that the file is properly closed regardless of the code execution path.
3. Read and write efficiently: When reading or writing large files, it is recommended to process the content in chunks instead of loading the entire file into memory. This approach minimizes memory consumption and prevents potential memory leaks.
FAQs (Frequently Asked Questions)
Q: What happens if I forget to close a file in Python?
A: Forgetting to close a file may result in resource leakage, hogging memory, and potential system crashes. Additionally, any changes made to the file may not be saved if the file is not closed correctly.
Q: Can I reopen a closed file in Python?
A: Yes, you can reopen a file even after it has been closed. However, it is essential to note that reopening a file does not revert any changes made before closing it. Thus, closed files should be reopened for further operations with caution.
Q: Can I skip closing a file if the program terminates?
A: While the operating system automatically closes files when a program terminates, this practice is not recommended. Explicitly closing files ensures immediate resource release and prevents any unexpected behavior caused by relying solely on the OS to handle it.
Q: Is it possible to check if a file is closed in Python?
A: Python does not provide a direct method to check if a file is closed. However, you can employ error handling techniques, like catching the ValueError or IOError that may be raised if attempting to use a closed file.
Q: What happens if a file is closed multiple times?
A: Closing an already closed file does not throw an error in Python. It is a safe practice to close a file multiple times, as subsequent calls to the close() method have no effect.
Conclusion
Closing files in Python is a crucial step in file handling and management. By adhering to best practices and utilizing the close() method or the with statement, programmers can ensure proper resource release, prevent memory leaks, and avoid unexpected results. Always remember to close files explicitly and promptly after finishing operations to maintain system stability and data integrity.
Images related to the topic valueerror: i/o operation on closed file.
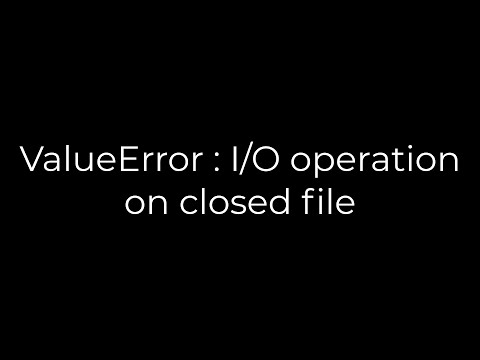
Found 29 images related to valueerror: i/o operation on closed file. theme

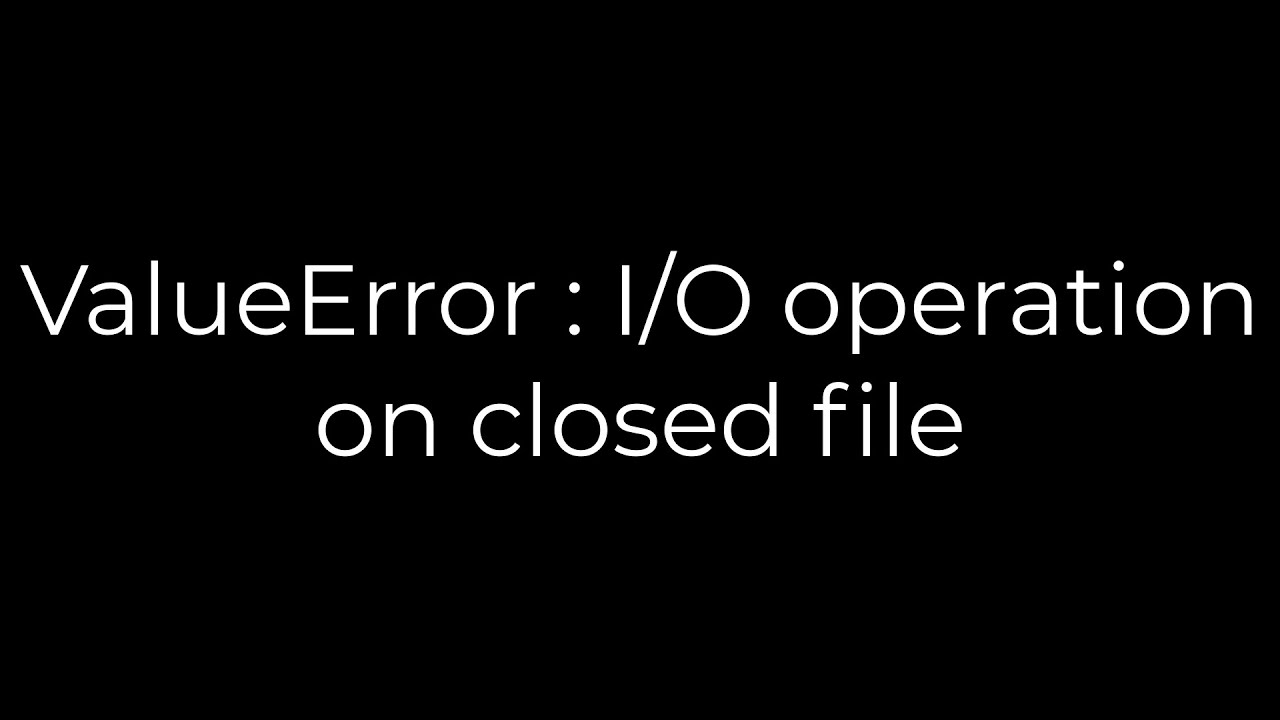


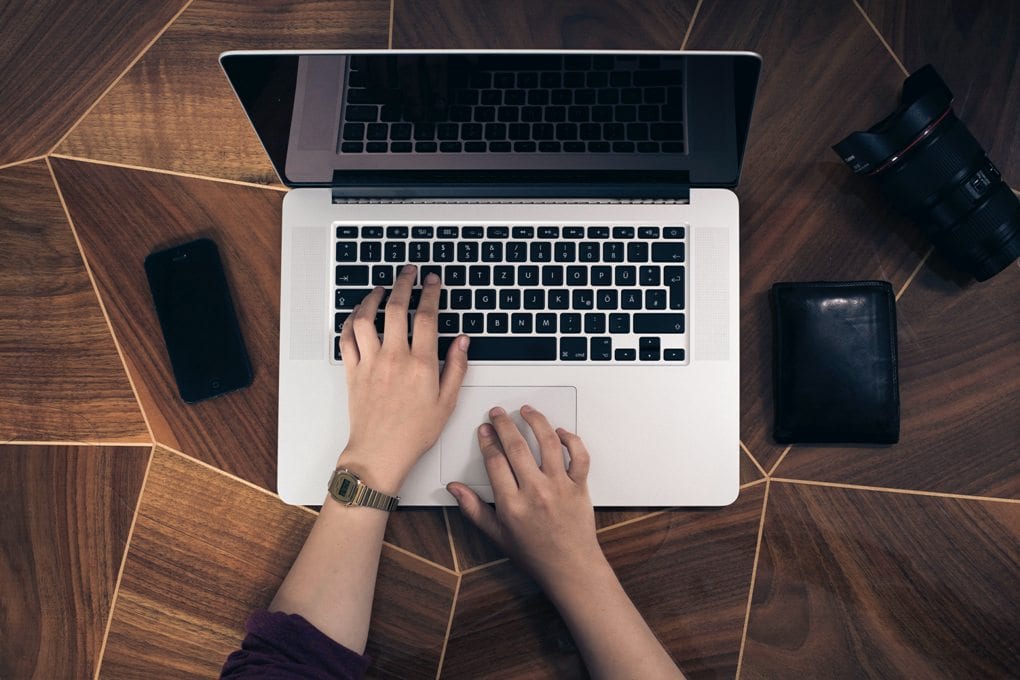
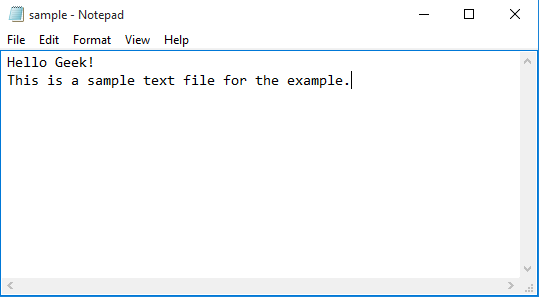

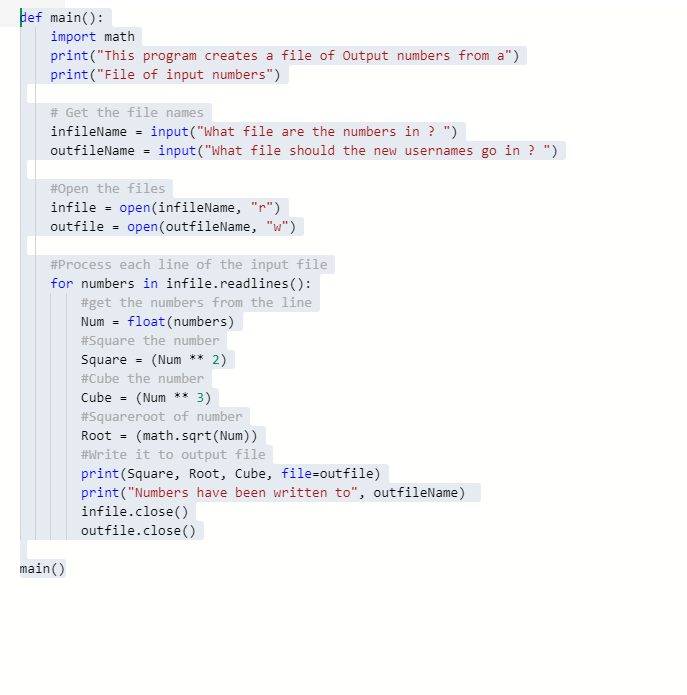

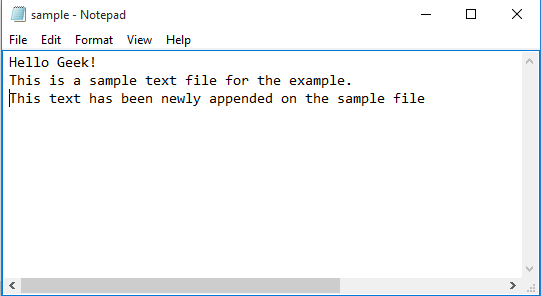

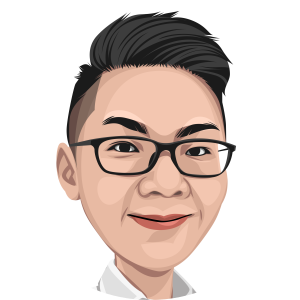
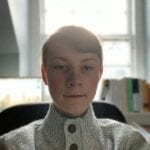
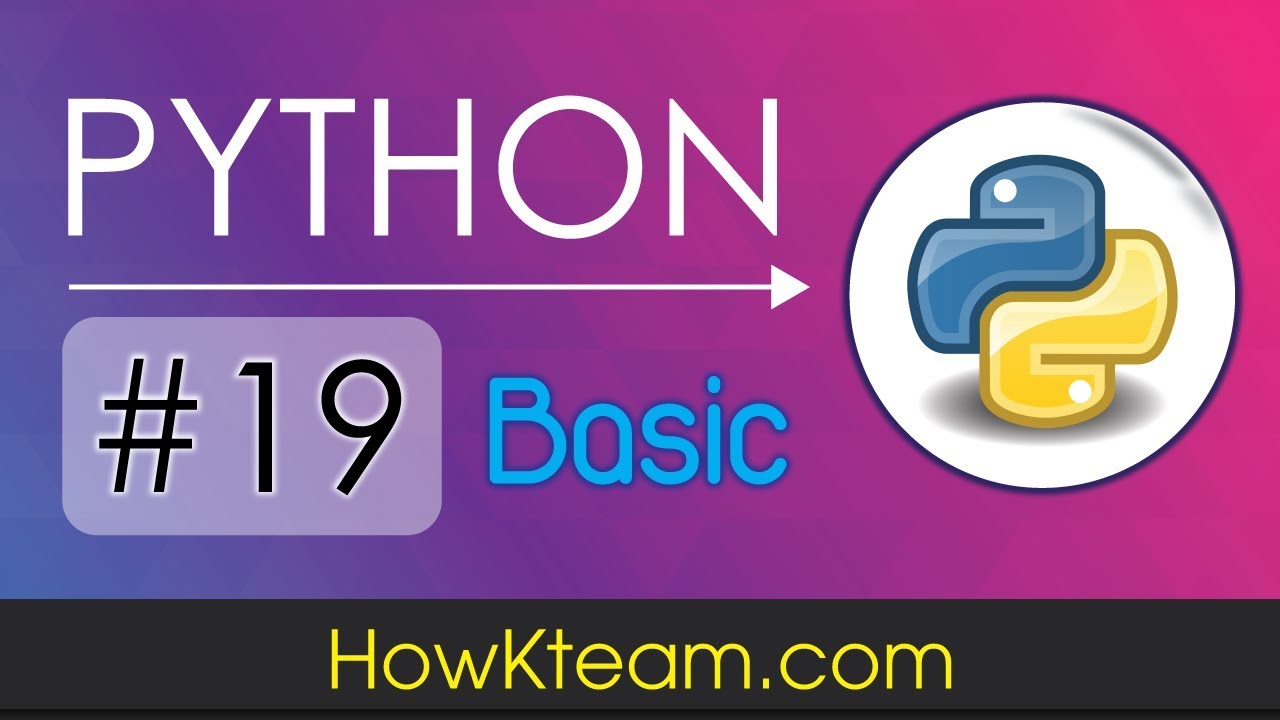

Article link: valueerror: i/o operation on closed file..
Learn more about the topic valueerror: i/o operation on closed file..
- ValueError: I/O operation on closed file in Python [Solved]
- ValueError : I/O operation on closed file – Stack Overflow
- How to fix “ValueError: I/O operation on closed file” in Python
- Python ValueError: I/O operation on closed file Solution
- How to fix ValueError: I/O operation on closed file in Python
- ValueError : I/O operation on closed file ( Solved )
- [Solved] ValueError: I/O operation on closed file. – Python Pool
- ValueError: I/O operations on closed file – STechies
- How to Fix ValueError: i/o operation on closed file – AppDividend
- Solve the ValueError: I/O Operation on Closed File in Python
See more: https://nhanvietluanvan.com/luat-hoc