Typeerror Expected String Or Bytes Like Object
What is a TypeError?
In programming, a TypeError is a specific type of error that occurs when an operation or function is performed on an object of an inappropriate or incompatible type. It indicates that the program is trying to use or manipulate data in a way that is not supported by the object’s type.
Common causes of TypeErrors
TypeErrors can occur due to various reasons, including:
1. Incorrect variable types: Using variables of the wrong type in an operation or function can lead to a TypeError. For example, trying to concatenate a string with an integer will result in this error.
2. Incompatible object types: Performing operations or passing arguments on objects that are not compatible can also trigger a TypeError. For instance, trying to multiply a string with a list would raise this error.
3. Mistakes in function calls: Providing the wrong number or type of arguments to a function can cause a TypeError. Each function expects specific arguments, and if they are not provided correctly, a TypeError will be raised.
A Look at “Expected string or bytes-like object”
One common type of TypeError message developers encounter is “Expected string or bytes-like object.” This error message typically occurs when the program expects a string or bytes-like object as an argument, but receives something different.
Reasons for the occurrence of this specific type of TypeError
There are several reasons why the “Expected string or bytes-like object” TypeError may occur:
1. Trying to convert an unsupported object to string or bytes: When attempting to convert an object that does not have the necessary methods or attributes to be converted to a string or bytes, this error can occur. For example, trying to convert a complex number to a string would result in this TypeError.
2. Passing an incompatible object as an argument to a string or bytes method/function: Some methods or functions in Python expect string or bytes-like objects as arguments. When an incompatible object is passed, such as a list or a number, the “Expected string or bytes-like object” TypeError is raised.
3. Incorrect usage of string or bytes methods/functions: Incorrectly using string or bytes methods/functions can also trigger this TypeError. This could happen if the method or function is used on an object that is not a string or bytes-like object. For example, applying the `.join()` method on an integer would lead to this error.
Common Scenarios Leading to “Expected string or bytes-like object” TypeError
1. Trying to convert an unsupported object to string or bytes:
“`
complex_number = 3 + 2j
str(complex_number) # Raises “Expected string or bytes-like object” TypeError
“`
2. Passing an incompatible object as an argument to a string or bytes method/function:
“`
number = 5
bytes(number) # Raises “Expected string or bytes-like object” TypeError
“`
3. Incorrect usage of string or bytes methods/functions:
“`
age = 25
age.join([‘My’, ‘is’, ‘years old’]) # Raises “Expected string or bytes-like object” TypeError
“`
Handling the “Expected string or bytes-like object” TypeError
When encountering the “Expected string or bytes-like object” TypeError, there are several steps you can take to handle it effectively:
1. Identifying the specific line causing the error: Carefully examine the error message to determine the line of code that is causing the TypeError. This will help you pinpoint the exact issue and resolve it.
2. Ensuring the variable is of the expected type: Make sure the variable being used in the operation or function is of the correct type. If needed, convert the variable to the appropriate type before using it.
3. Making necessary conversions before passing arguments: If the error occurs when passing arguments to a string or bytes method/function, ensure that the arguments are of the expected type. Convert them beforehand if required.
4. Checking for potential data encoding issues: If the error occurs when working with bytes-like objects, check if there are any encoding issues. Ensure that the data is properly encoded before performing any operations.
Potential Pitfalls and Best Practices
To handle the “Expected string or bytes-like object” TypeError effectively, consider the following best practices:
1. Understand the expected argument type: Before using any method or function, familiarize yourself with the expected argument type. Ensure that the provided argument matches the expected type to avoid TypeErrors.
2. Utilize type-checking techniques: Use techniques such as type annotations or type hints to indicate the expected types of variables and function arguments. This can help identify potential TypeErrors before runtime.
3. Employ defensive programming: Write code that can handle unexpected scenarios gracefully. Use if statements or try-except blocks to catch and handle specific TypeErrors, providing meaningful error messages or fallback solutions.
4. Considerations for troubleshooting and debugging: When encountering TypeErrors, utilize debugging tools and techniques to identify the root cause of the error. Tools like print statements and debuggers can help track the flow of the program and identify incorrect variable types or faulty logic.
Other Related TypeErrors and How to Handle Them
In addition to the “Expected string or bytes-like object” TypeError, there are several other related TypeErrors that developers may encounter. Here are a few examples and suggestions on how to handle them:
1. TypeErrors related to incorrect argument types: Ensure that the arguments provided to functions or methods match the expected types. Convert variables or use conditional statements to handle different input types gracefully.
2. Handling TypeErrors caused by unsupported operations: When performing operations on objects, make sure that the objects support the specific operation. Use conditional statements or try-except blocks to handle unsupported operations and provide appropriate fallbacks.
3. Sorting out TypeErrors due to mismatched data types: Verify that the data types being used in the program are compatible with each other. Perform necessary type conversions when needed to avoid incompatibilities and TypeErrors.
Resources for Further Learning
If you want to delve deeper into handling TypeErrors and related topics, consider exploring the following resources:
– Online documentation and resources: Consult the official documentation for the programming language you are using. It often provides detailed explanations of error messages and common pitfalls.
– Popular forums and developer communities: Participate in online forums and communities like Stack Overflow to seek guidance from experienced developers. They may have encountered similar issues and can offer valuable insights and solutions.
– Relevant books and tutorials: Look for books and tutorials that focus on troubleshooting programming errors, including TypeErrors. These resources provide step-by-step explanations and practical exercises to enhance your skills in handling such errors effectively.
Conclusion
Expected String Or Bytes Like Object | Python Programming
What Is A Bytes-Like Object?
In the world of programming and computer science, the concept of a bytes-like object plays a vital role. It is an essential data type used to represent a sequence of bytes. Understanding what a bytes-like object is and how it operates is crucial for developers, as it allows them to handle and manipulate binary data efficiently. In this article, we delve into the depths of bytes-like objects, exploring their characteristics, uses, and how they relate to other data types.
Characteristics of a bytes-like object
A bytes-like object is an immutable sequence of integers each representing a single byte. The object can contain values ranging from 0 to 255, inclusive, and is typically written as a sequence of two-digit hexadecimal numbers. For instance, a bytes-like object might look like this: b’\x61\x62\x63′. Each value within the object represents the ASCII value of a respective character, allowing programmers to work with textual data in various programming languages.
One significant characteristic of a bytes-like object is its immutability. Once created, it cannot be modified. This immutability ensures the integrity and consistency of the data throughout the program’s execution. However, it’s important to note that bytes-like objects do allow for the creation of modified versions through methods like slicing and concatenation. These methods create new objects without altering the original one.
Another aspect worth mentioning is the memory optimization of bytes-like objects. In many programming languages, bytes-like objects are stored as a sequence of bytes, each requiring one byte of memory. This efficient memory usage makes them ideal for working with large amounts of binary data or handling network protocols.
Uses of bytes-like objects
Bytes-like objects have a wide range of applications in programming. They are commonly utilized when dealing with file I/O operations, network protocols, cryptographic operations, and encoding and decoding of textual data. Here are a few areas where bytes-like objects are commonly utilized:
1. File I/O: When reading or writing binary files, bytes-like objects are often used to handle the data efficiently. These objects allow for smooth input/output operations, ensuring data integrity.
2. Networking: Bytes-like objects are essential when working with network protocols like TCP and UDP. They enable the transmission and reception of binary data packets across networks.
3. Cryptography: Cryptographic algorithms often operate on binary data. Bytes-like objects are used as inputs and outputs for these algorithms to ensure secure communication.
4. Text encoding/decoding: Bytes-like objects can be encoded into various character encodings like UTF-8 or ASCII to represent textual data. Conversely, they can be decoded back into bytes when needed.
Relationship with other data types
Bytes-like objects bear resemblance to other data types such as strings and byte arrays, often leading to confusion. Here’s a breakdown of their relationships:
1. Strings: While strings and bytes-like objects can both represent textual data, they differ in their characteristics and uses. Strings are typically mutable in nature, allowing modifications, and often used to represent human-readable text. Conversely, bytes-like objects are immutable and primarily used to store binary data or represent textual data in non-human-readable formats.
2. Byte Arrays: Byte arrays share similarities with bytes-like objects as both represent sequences of bytes. However, byte arrays are mutable, allowing direct modification of individual bytes, whereas bytes-like objects do not permit modifications.
FAQs:
Q: Can I convert a bytes-like object to a string?
A: Yes, bytes-like objects can be converted to strings using the appropriate decoding methods, such as UTF-8 decoding.
Q: How can I modify a bytes-like object?
A: The immutability of bytes-like objects means they cannot be modified directly. However, you can create modified versions using slicing, concatenation, or by converting them to a mutable byte array.
Q: What is the advantage of using bytes-like objects over strings for binary data?
A: Bytes-like objects provide an efficient, memory-optimized way of handling binary data. They also offer flexibility when working with network protocols, file I/O operations, and cryptographic algorithms.
Q: Is a bytes-like object the same as a byte string?
A: Yes, a bytes-like object is often referred to as a byte string or binary string, as it represents a sequence of bytes.
Q: Can I convert a bytes-like object to an integer?
A: Yes, you can convert a bytes-like object to an integer using appropriate conversion functions, such as the int.from_bytes() method.
In conclusion, understanding what a bytes-like object is and how it operates is crucial for any programmer working with binary data. These objects provide an immutable and memory-efficient way of representing sequences of bytes, enabling efficient manipulation of binary data. By grasping the characteristics, uses, and relationships of bytes-like objects, developers can effectively utilize them in various programming tasks, from file I/O to networking and cryptography.
What Is Bytes Python?
Python is a versatile programming language that offers various data types to handle different kinds of information. One of these data types is called bytes. In Python, bytes represent a sequence of integer values between 0 and 255. These values can be used to store raw binary data, such as encoded text or images, in memory or on disk.
Bytes objects are similar to strings, but they are immutable, meaning that their contents cannot be modified once they are created. This immutability allows bytes to be used as keys in dictionaries and elements in sets, which are requirements for these data structures in Python.
Bytes objects can be defined in several ways. The most common method is by using the b prefix followed by the sequence of integer values enclosed in parentheses or square brackets. For example:
“`python
data = b’Hello, World!’
“`
The above code creates a bytes object called data, which contains the ASCII representation of the string “Hello, World!”. Each character of the string is represented by its corresponding integer value, ranging from 0 to 127 in the ASCII table.
The len() function can be used to determine the size of a bytes object in bytes. For instance:
“`python
data = b’Hello, World!’
size = len(data)
print(size) # Output: 13
“`
In the example above, the len() function returns the size of the bytes object data, which is 13 bytes.
Bytes objects also support indexing and slicing operations, just like strings. The individual elements of a bytes object can be accessed using square brackets. For example:
“`python
data = b’Hello, World!’
print(data[0]) # Output: 72
print(data[-1]) # Output: 33
print(data[7:12]) # Output: b’World’
“`
In the code snippet above, data[0] retrieves the integer value at index 0, which corresponds to the ASCII code of the character ‘H’. data[-1] returns the integer value at the last index, which corresponds to the ASCII code of the exclamation mark. Lastly, data[7:12] retrieves a portion of the bytes object containing the ASCII representation of the string “World”.
Bytes objects can also be created using the built-in bytes() constructor. This constructor takes an iterable of integers as its argument and returns a bytes object containing their binary representation. For example:
“`python
data = bytes([72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33])
print(data) # Output: b’Hello, World!’
“`
In the above code, the bytes() constructor is used to create the bytes object data, which contains the ASCII representation of the string “Hello, World!”.
Bytes objects can also be converted to strings using the decode() method. This method takes an optional encoding argument, which specifies the character encoding to be used. The default encoding is UTF-8. For example:
“`python
data = b’Hello, World!’
string = data.decode()
print(string) # Output: Hello, World!
“`
In the code above, the decode() method is called on the bytes object data, which converts it into a string representation.
FAQs about bytes Python
Q: Can I modify the contents of a bytes object?
A: No, bytes objects are immutable. Once created, their contents cannot be modified. If you need to modify the data, you should create a new bytes object with the desired changes.
Q: How can I convert a string to bytes?
A: You can convert a string to bytes using the encode() method. This method takes an optional encoding argument, which specifies the character encoding to be used. The default encoding is UTF-8. For example: `data = ‘Hello, World!’.encode()`
Q: How can I check if a bytes object contains a specific value?
A: You can use the `in` operator to check if a specific value exists in a bytes object. For example: `print(b’Hello’ in data)`
Q: What is the difference between bytes and bytearray in Python?
A: The main difference is that bytes objects are immutable, while bytearrays are mutable. This means that bytearrays can be modified after they are created, whereas bytes objects cannot.
Q: Can I perform arithmetic operations on bytes objects?
A: No, bytes objects do not support arithmetic operations like strings do. If you need to manipulate binary data, you can convert the bytes object to an integer and perform the operations on the integer representation.
In conclusion, bytes objects in Python are a useful data type for working with binary data, such as encoded text or images. They are immutable and provide various methods and operations to interact with their contents. Understanding how to create, manipulate, and convert bytes objects can greatly expand your arsenal of tools when working with binary data in Python.
Keywords searched by users: typeerror expected string or bytes like object Expected string or bytes like object re findall, Expected string or bytes like object django, A bytes-like object is required, not ‘str, Regex match string Python, Re sub trong Python, Cannot use a string pattern on a bytes-like object, Decoding to str need a bytes-like object nonetype found, Regex extract Python
Categories: Top 60 Typeerror Expected String Or Bytes Like Object
See more here: nhanvietluanvan.com
Expected String Or Bytes Like Object Re Findall
The re.findall method is part of the re (regular expressions) module in Python, which provides a wide range of functions to support pattern matching and text manipulation. The method returns all non-overlapping matches of a pattern within a target string as a list. However, it is important to note that the target string should be either a regular string object or a bytes-like object, such as a byte string or a bytearray.
To understand how re.findall works, let’s take a look at a simple example. Suppose we have a string called “text” that contains the following text:
“This is a sample text with some numbers like 123 and 456.”
Now, let’s say we want to extract all the numbers from this text. We can achieve this using the re.findall method as follows:
“`python
import re
text = “This is a sample text with some numbers like 123 and 456.”
numbers = re.findall(r’\d+’, text)
print(numbers)
“`
Output:
“`
[‘123’, ‘456’]
“`
In the example above, we import the re module and define our target string “text” that contains the mentioned text. We then use the re.findall method with the pattern `\d+`, which matches one or more digits. The result is a list of all the numbers found in the text.
The re.findall method can be used with a wide range of patterns. Some commonly-used patterns include:
– `\d+` – matches one or more digits
– `\w+` – matches one or more alphanumeric characters (word characters)
– `\s+` – matches one or more whitespace characters
– `.+` – matches one or more of any character, except a newline
Moreover, re.findall also supports the usage of capturing groups, allowing us to extract specific parts of a match. For example:
“`python
import re
text = “Hello, my name is John Doe. I live in New York.”
names = re.findall(r’My name is (\w+)’, text)
print(names)
“`
Output:
“`
[‘John’]
“`
In this example, we capture the name using the capturing group `(\w+)` inside the pattern. This way, we obtain the desired output by extracting only the name.
Now, let’s address some frequently asked questions about expected string or bytes-like object re.findall:
**Q: Can re.findall match multiple patterns at once?**
A: No, re.findall matches only a single pattern at a time. However, you can combine multiple patterns into a single pattern using the `|` (pipe) operator to search for multiple patterns simultaneously.
**Q: Can re.findall be case-insensitive?**
A: Yes, re.findall supports case-insensitive matching by passing the `re.IGNORECASE` flag as a second argument to re.findall.
**Q: What happens if re.findall doesn’t find any matches?**
A: If re.findall doesn’t find any matches, it returns an empty list.
**Q: Are regular expressions efficient for large amounts of text?**
A: Regular expressions can be efficient, but they may become slower as the complexity of the pattern increases or the text size grows significantly. In such cases, you might want to consider optimizing your regular expression pattern or exploring alternative methods.
**Q: Are there any limitations to re.findall with respect to the pattern complexity?**
A: The complexity of the pattern used in re.findall can impact performance. Patterns containing nested quantifiers, backreferences, or lookaheads/lookbehinds may have performance implications. It’s important to consider the complexity of the pattern and evaluate performance accordingly.
In conclusion, expected string or bytes-like object re.findall is a powerful tool in Python for searching patterns within strings or byte-like objects. It provides flexibility and robustness for extracting valuable information or validating the presence of patterns within large amounts of textual data. Understanding how to use this method effectively can greatly enhance text processing capabilities in Python.
Expected String Or Bytes Like Object Django
Django is a popular web framework that follows the Model-View-Controller (MVC) architectural pattern. It is written in Python, making it highly scalable and versatile for web development projects. When working with Django, you might come across an error message stating “TypeError: expected string or bytes-like object.” In this article, we will explore the concept of expected string or bytes-like object in Django, what causes this error, and how to solve it.
Understanding the Error
The error message “TypeError: expected string or bytes-like object” typically occurs when Django expects a string or bytes object but receives a different data type. This error can be seen in various scenarios, such as when handling form data, file uploads, manipulating URLs, or working with external libraries.
Common Causes of the Error
There are several common causes for this error in Django applications:
1. Form Data: One common source of the error is when handling form data. Django provides a Form class that simplifies form handling. If the request.POST data that comes from the form is not a string or bytes-like object, the error will occur. This can happen if the form is not properly validated or if the data is not properly encoded.
2. File Uploads: Another area where this error can occur is when handling file uploads. Django provides the FileField and ImageField classes to handle file uploads in models. If the file being uploaded does not conform to the expected string or bytes-like object, the error will be raised. This can happen if the file is corrupted, doesn’t exist, or is not properly encoded.
3. URL Manipulation: Django allows for dynamic URL routing and manipulation through its URL patterns. If the URL passed to Django does not conform to the expected string or bytes-like object, the error can occur. This can happen if the URL is malformed, contains unsupported characters, or is not properly encoded.
4. External Libraries: Django often interacts with external libraries and modules to enhance functionality. If the external library receives an unexpected data type, it might raise the “TypeError: expected string or bytes-like object” error. This can happen if the data being passed to the library is not properly formatted or encoded.
Solving the Error
To resolve the “TypeError: expected string or bytes-like object” error in Django, it is essential to identify the source of the problem and apply the appropriate solution. Here are some common solutions:
1. Form Data: When handling form data, ensure that the form is properly validated before attempting to access the data. Use Django’s built-in validators to ensure that the data conforms to the expected format. Additionally, check that the form data is properly encoded using UTF-8 or the appropriate encoding.
2. File Uploads: When dealing with file uploads, verify that the uploaded file is valid and not corrupted. Check if the file exists before attempting to manipulate it. Also, ensure that the FileField or ImageField in the model is properly declared, including max_length and upload_to attributes.
3. URL Manipulation: When working with URLs, make sure that the URLs are properly formed and encoded. Use Django’s built-in URL encoding and decoding functions to handle special characters properly. If the URL contains different data types, convert them to strings or bytes before passing them to Django.
4. External Libraries: When using external libraries or modules, always refer to their documentation. Validate the data being passed to the library to ensure it is of the expected format. If necessary, format or encode the data in the required manner before passing it to the library.
FAQs
Q1. Why am I getting the “TypeError: expected string or bytes-like object” error in Django?
A1. This error occurs when Django expects a string or bytes-like object but receives a different data type. It can happen when handling form data, file uploads, manipulating URLs, or working with external libraries.
Q2. How can I fix the error in handling form data?
A2. To fix the error in handling form data, ensure that the form is properly validated before accessing the data. Use Django’s validators and ensure the data is properly encoded.
Q3. What should I do if I encounter the error during file uploads?
A3. When handling file uploads, check if the uploaded file is valid and not corrupted. Also, verify that the FileField or ImageField in the model is properly declared.
Q4. How can I resolve the URL manipulation error?
A4. To resolve the URL manipulation error, ensure that the URLs are properly formed and encoded. Use Django’s URL encoding and decoding functions and convert data types to strings or bytes when necessary.
Q5. What steps should I follow when working with external libraries?
A5. When using external libraries, refer to their documentation and validate the data being passed to them. Format or encode the data as required by the library.
In conclusion, the “TypeError: expected string or bytes-like object” error can occur in various scenarios when working with Django. It is crucial to identify the source of the error and apply the appropriate solution. By following the guidelines provided in this article, you can resolve this error and ensure smooth execution of your Django application.
Images related to the topic typeerror expected string or bytes like object
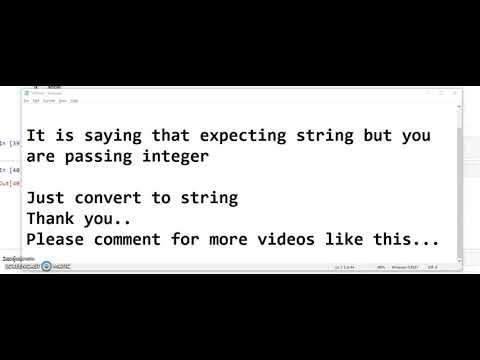
Found 22 images related to typeerror expected string or bytes like object theme
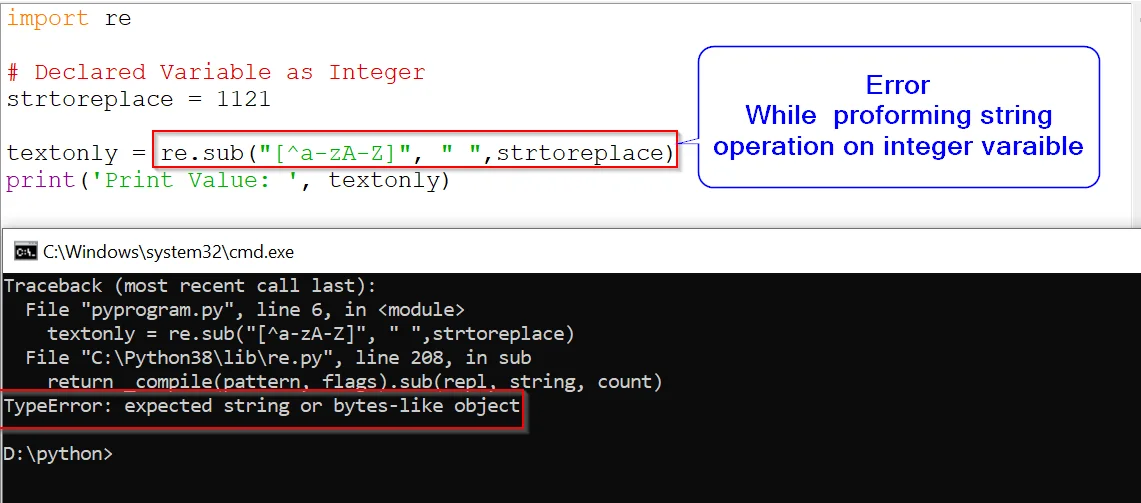
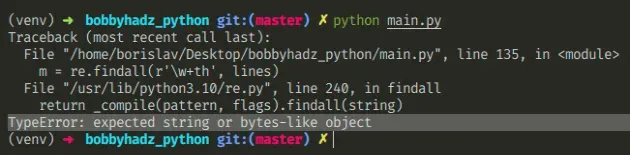
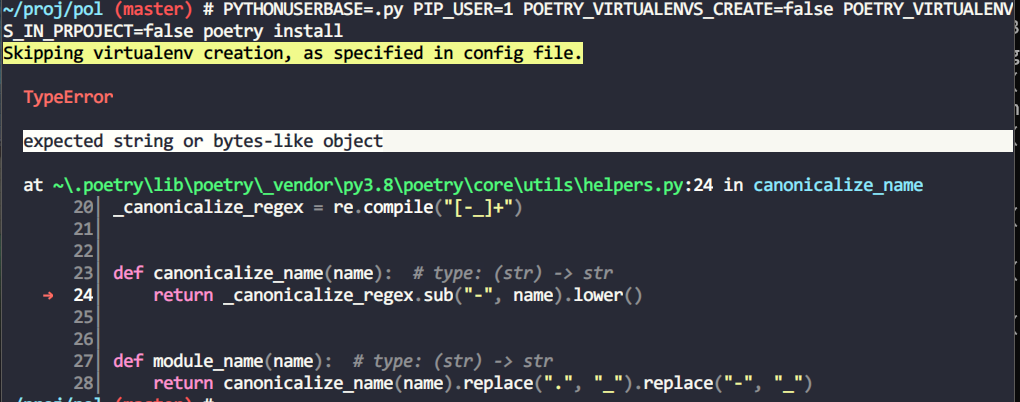
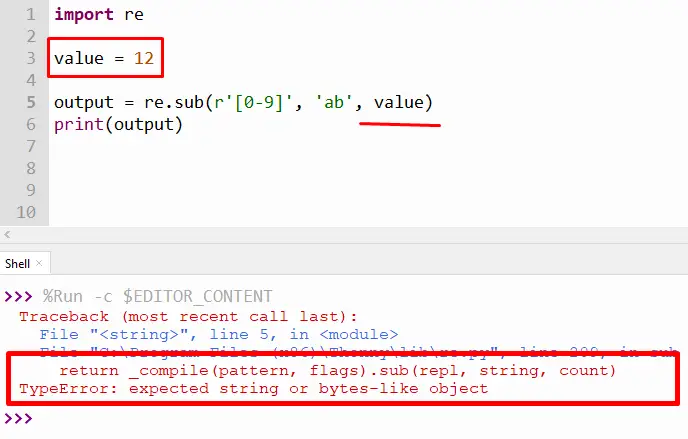

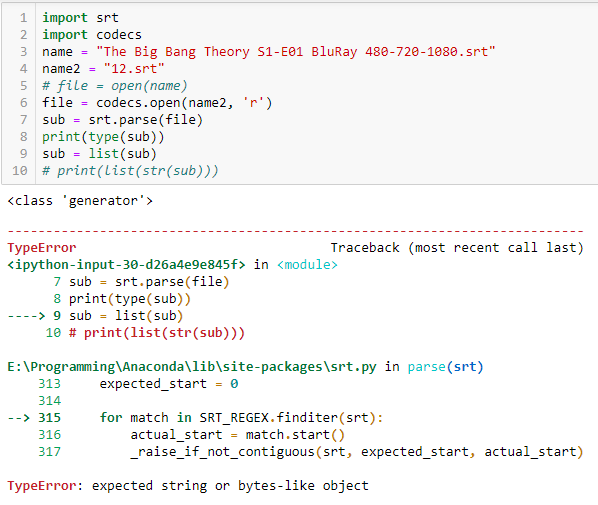
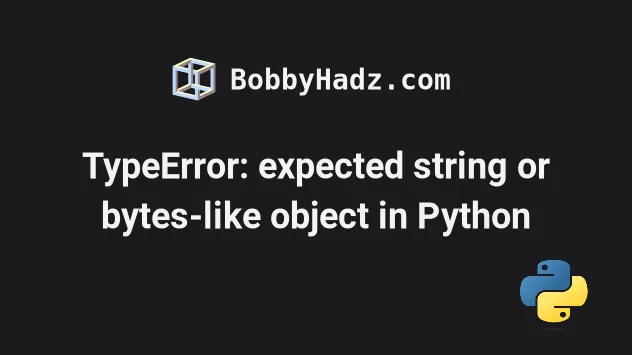
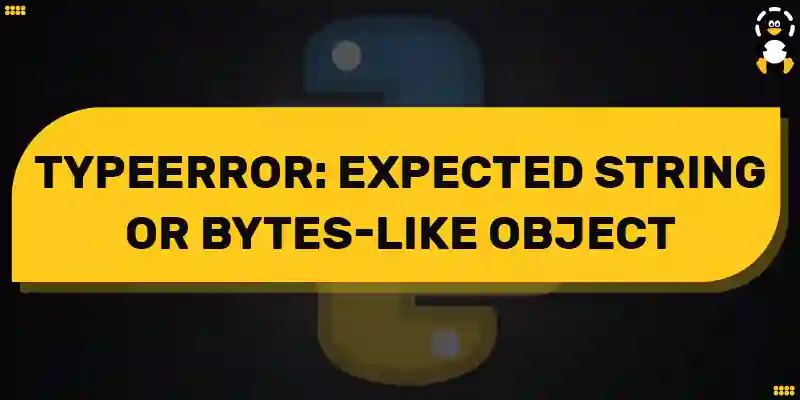
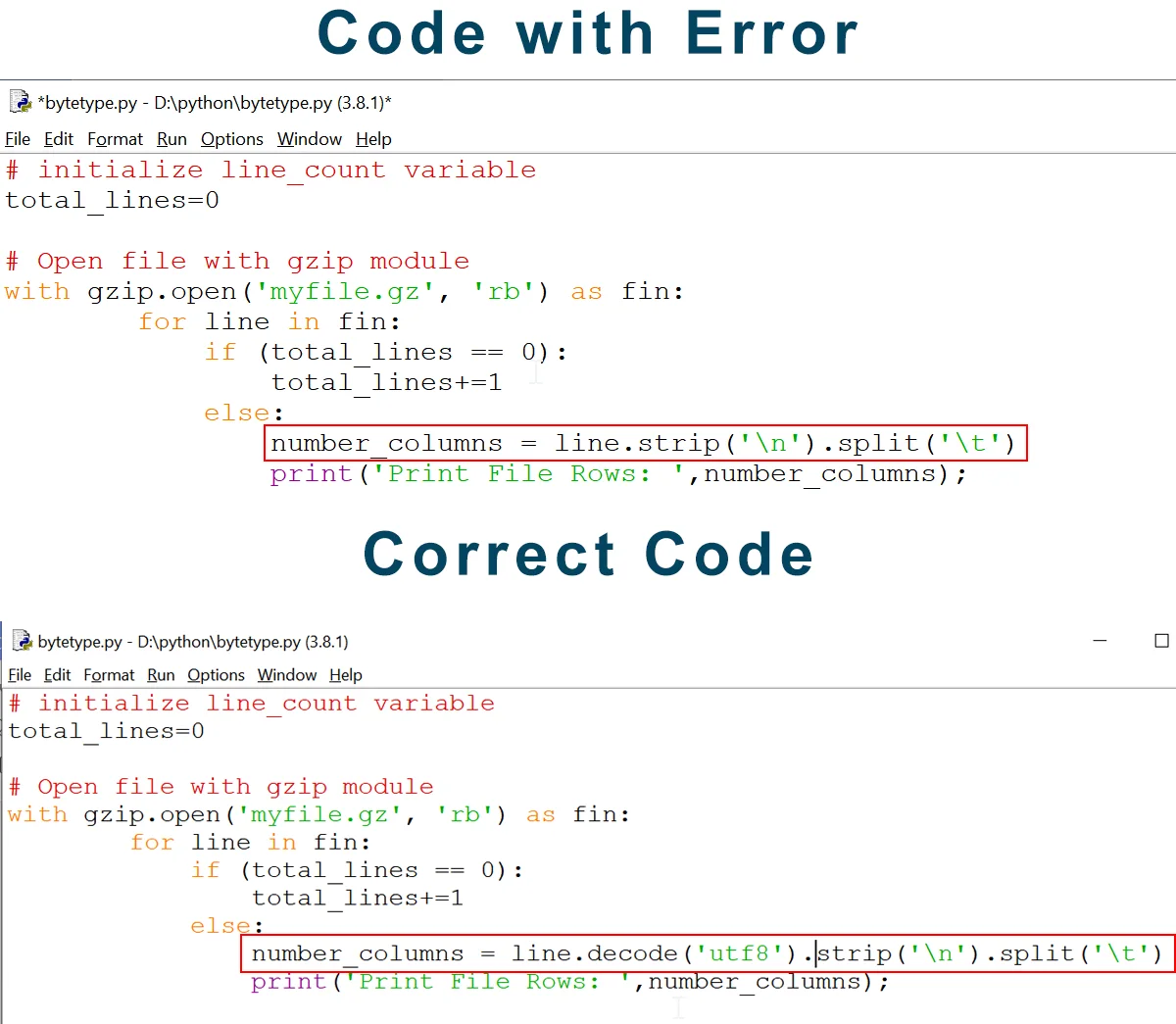
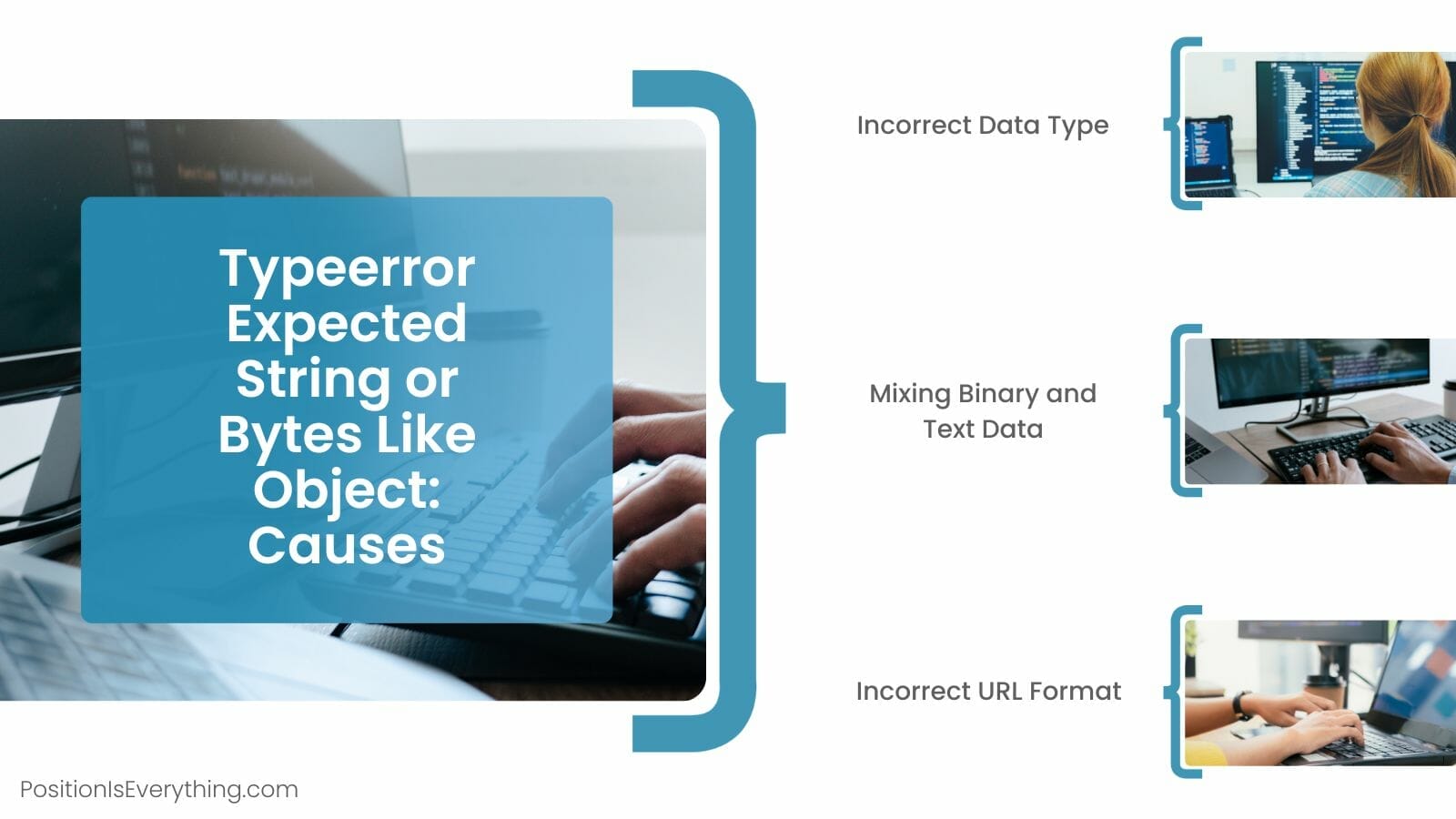
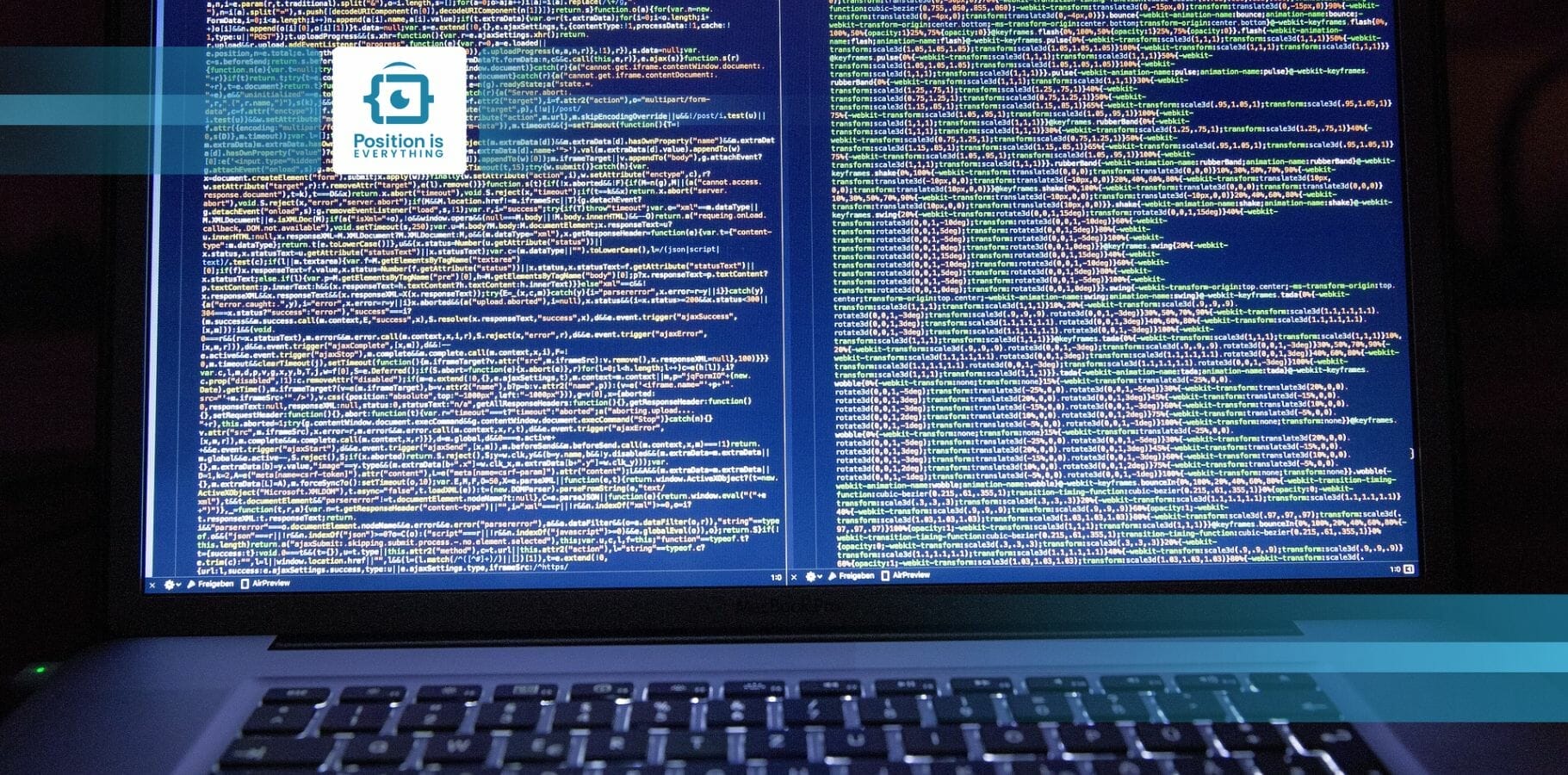
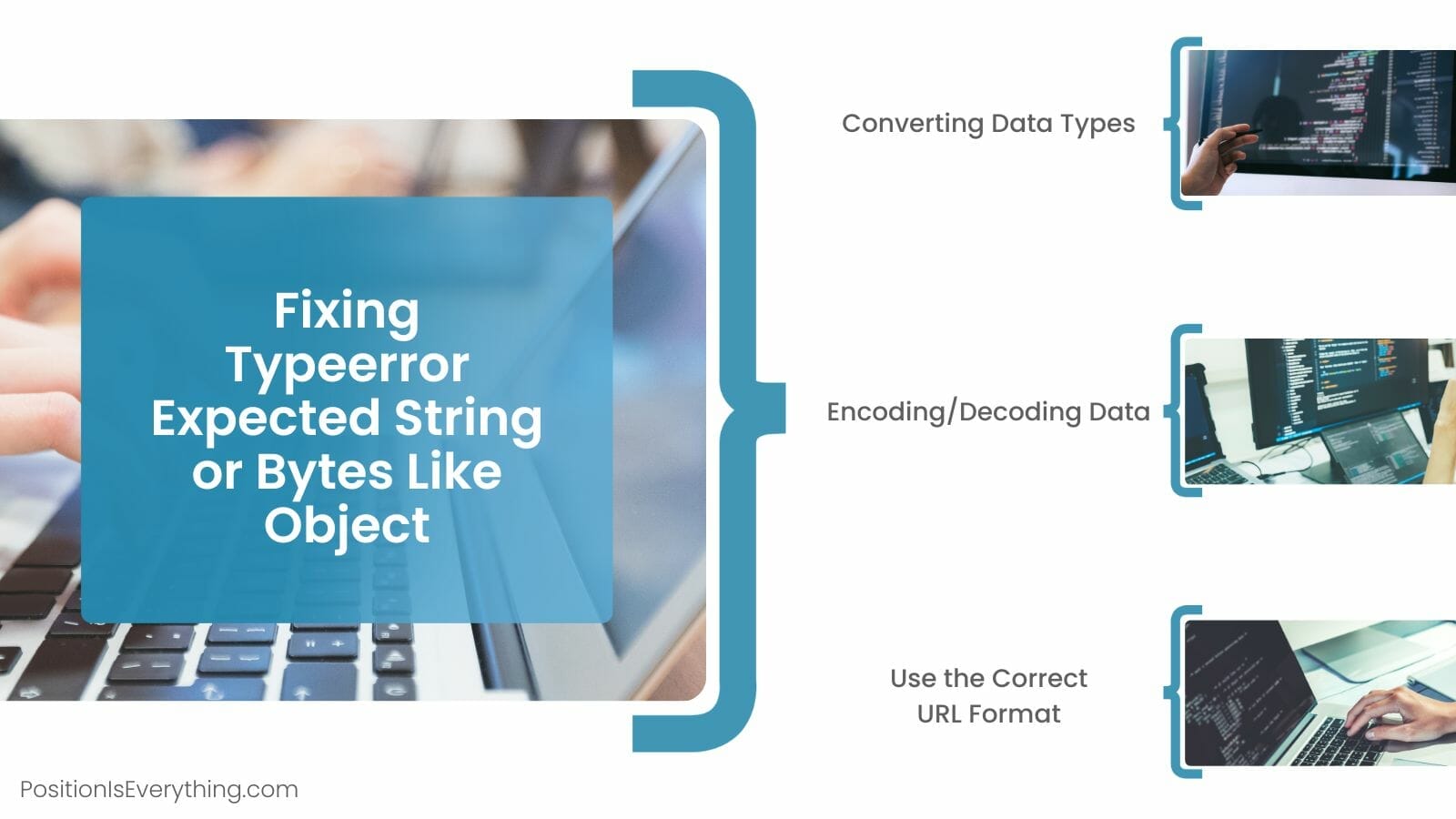

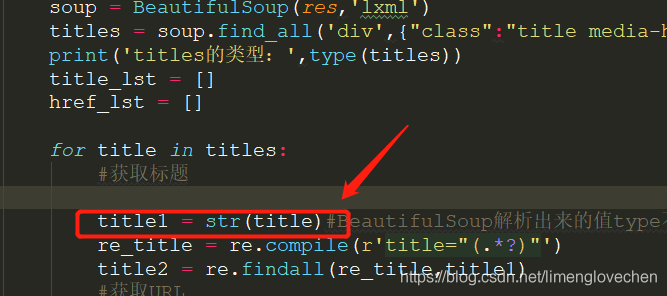
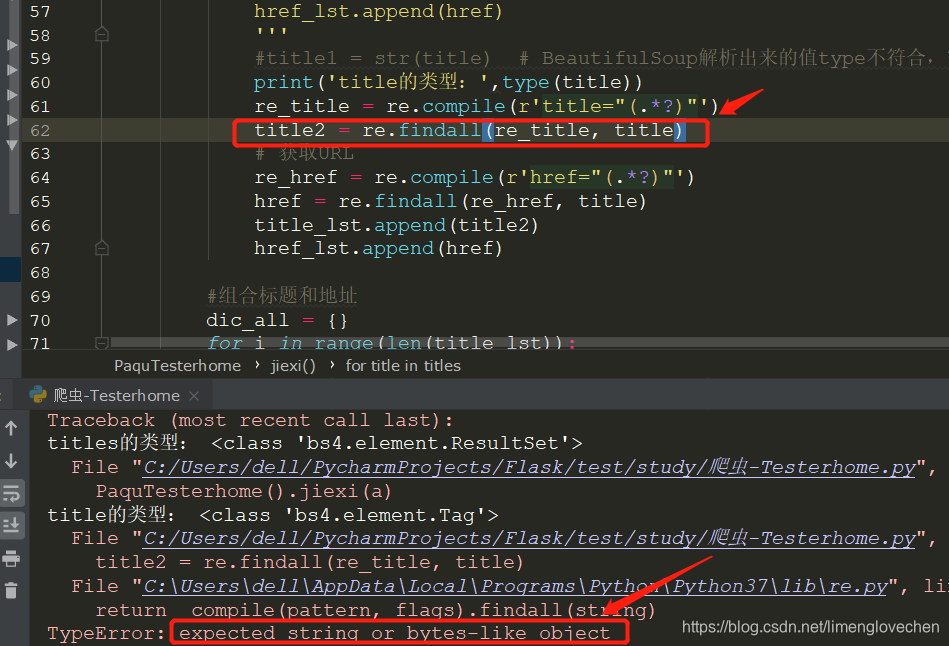
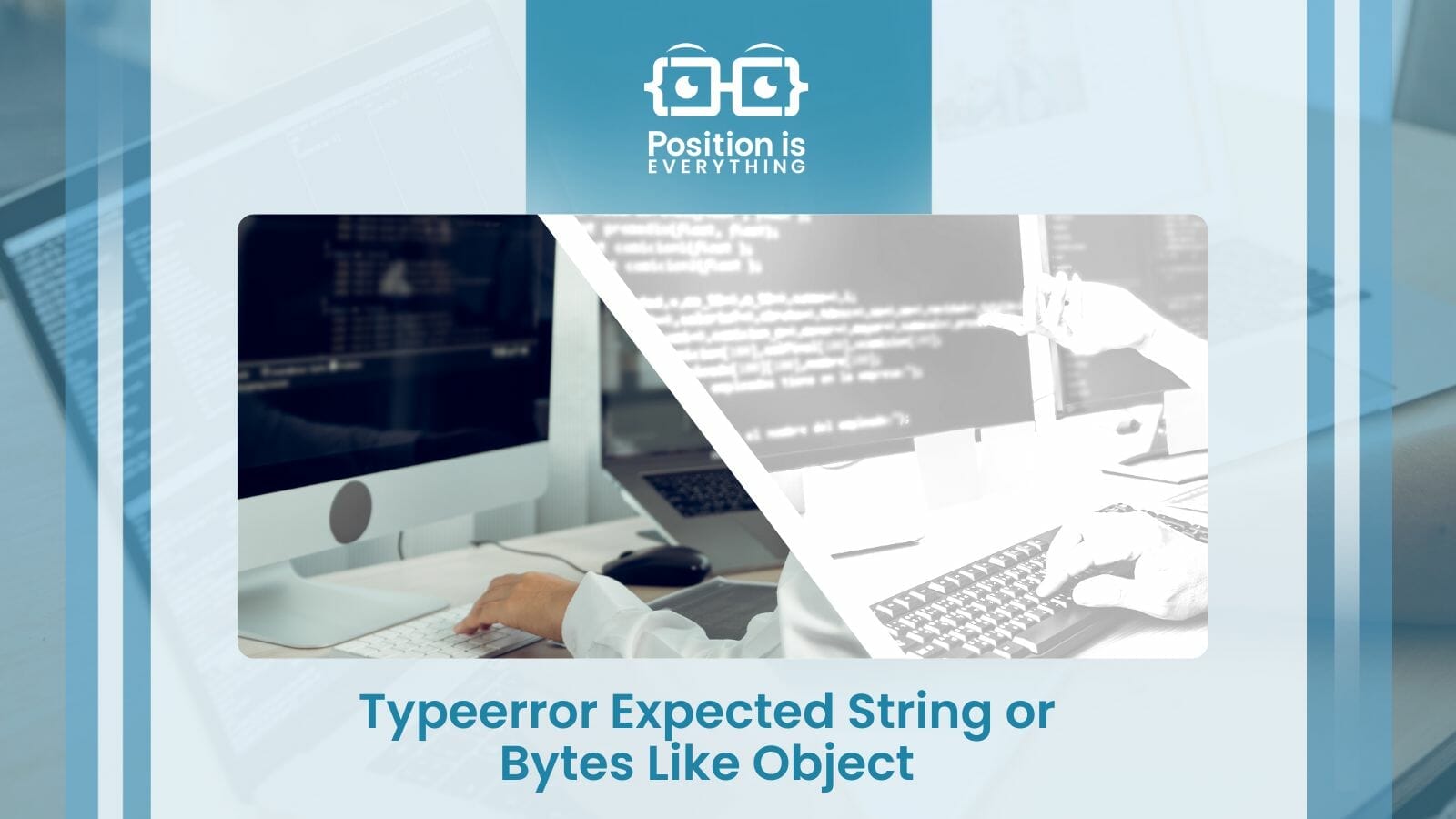
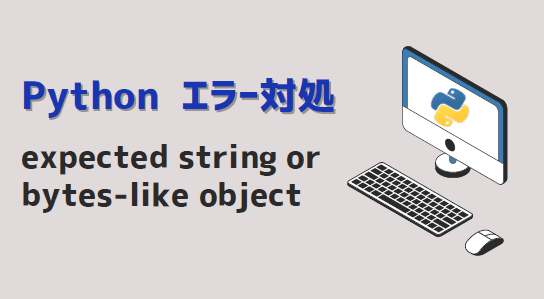
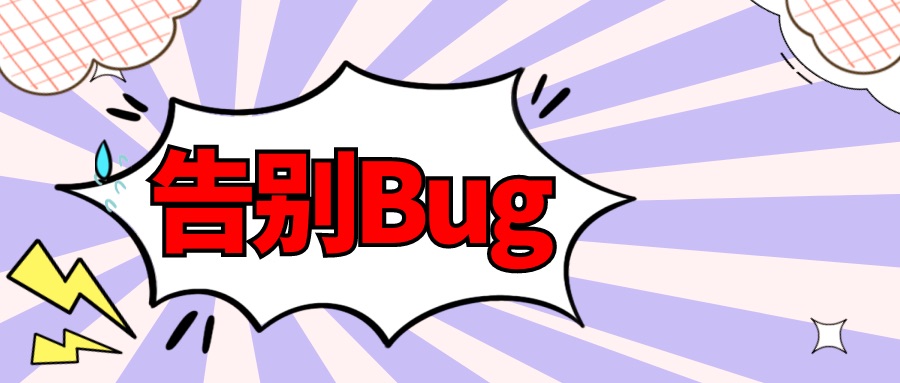
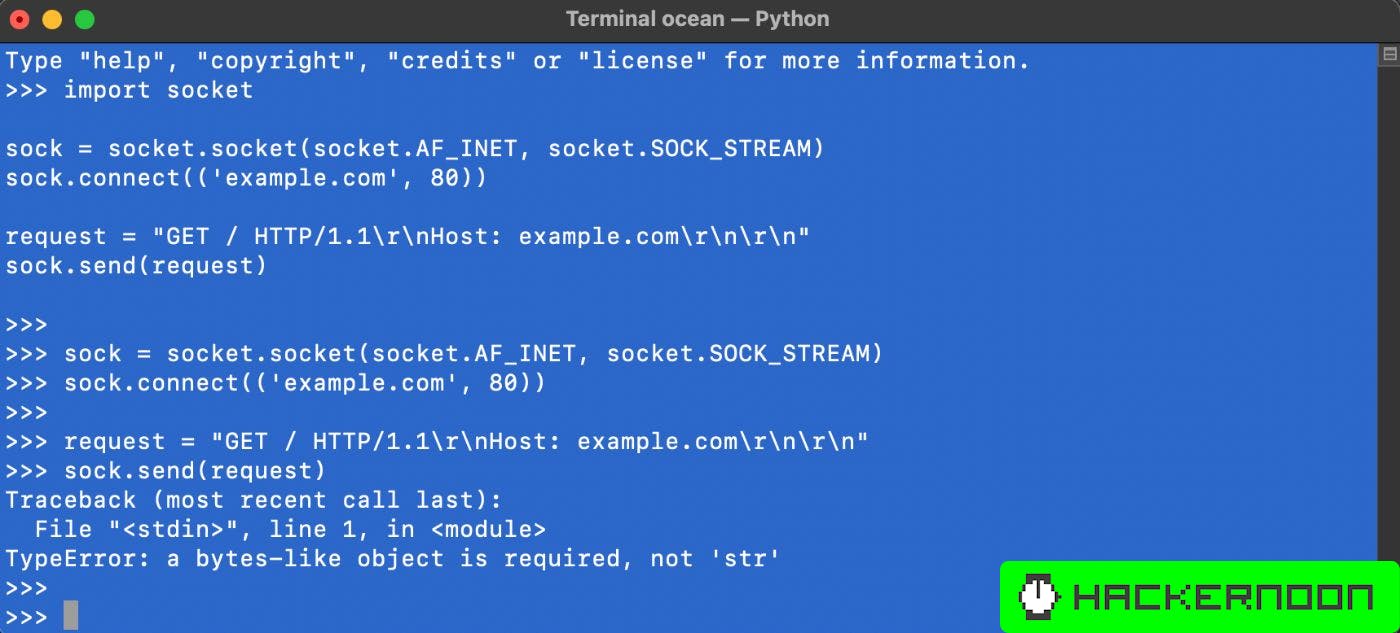
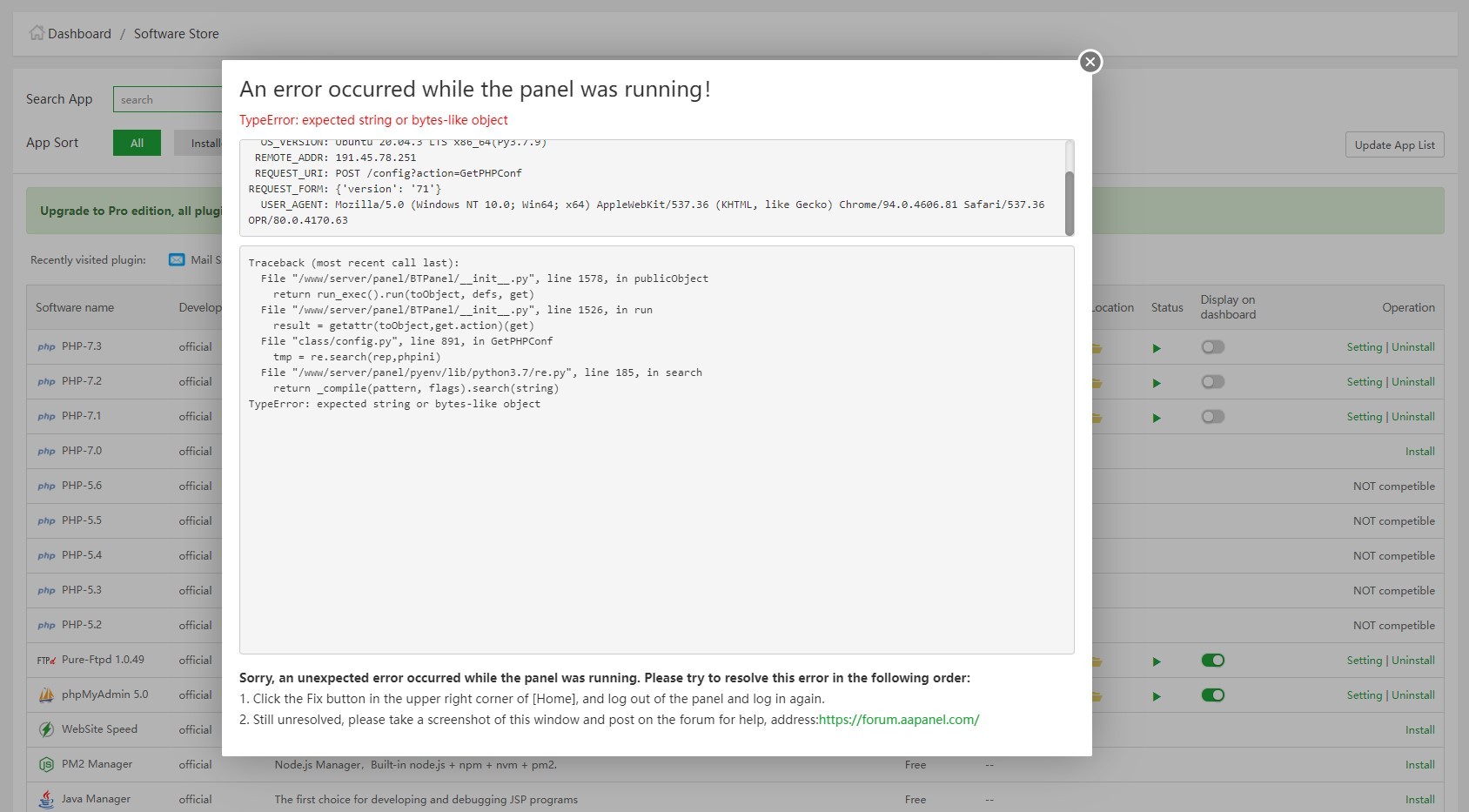

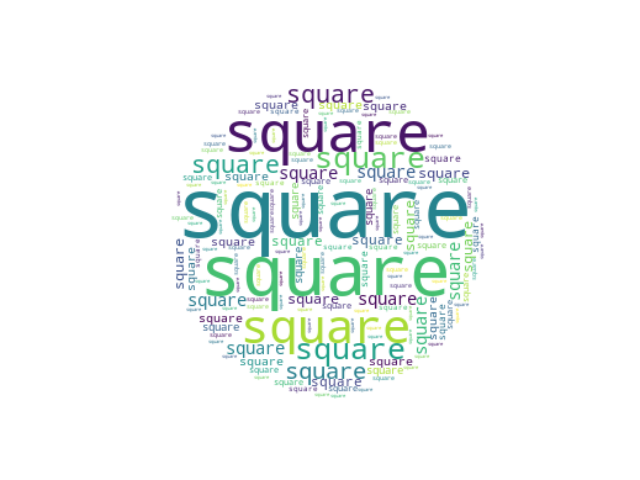
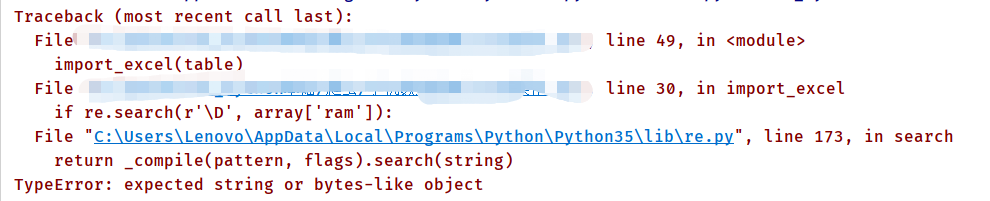

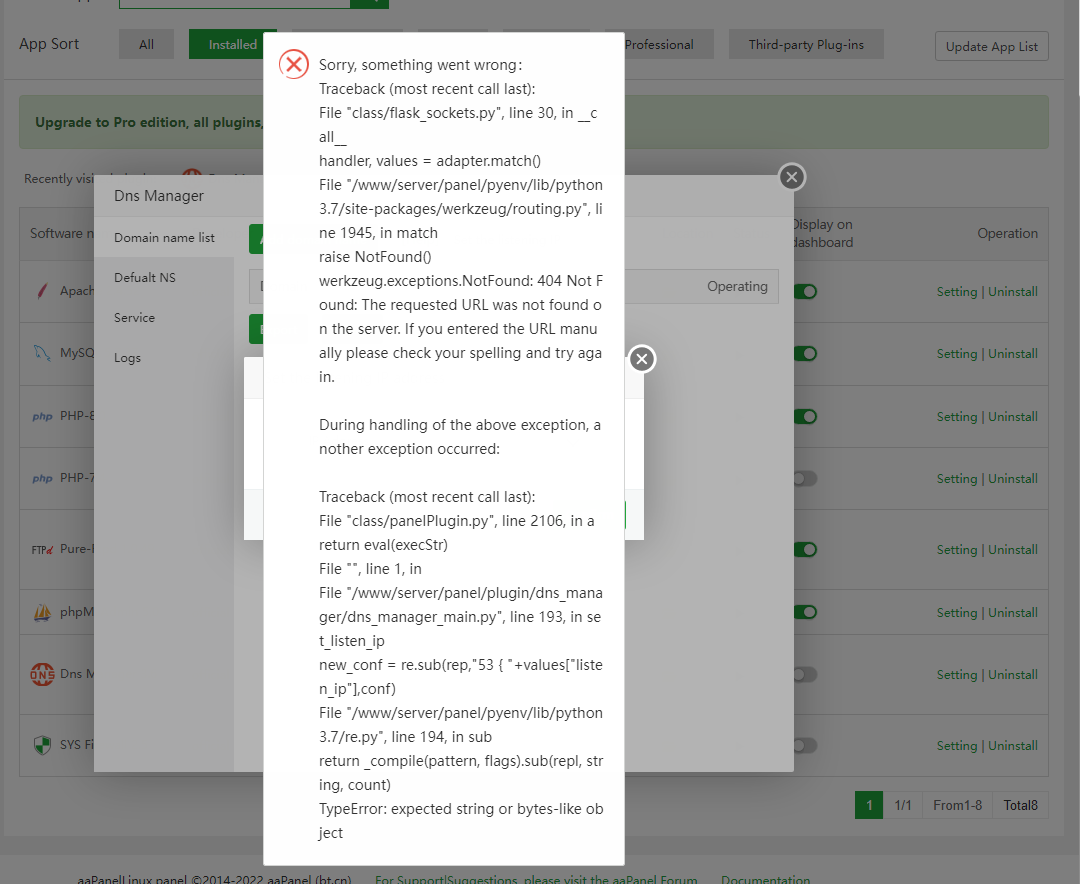
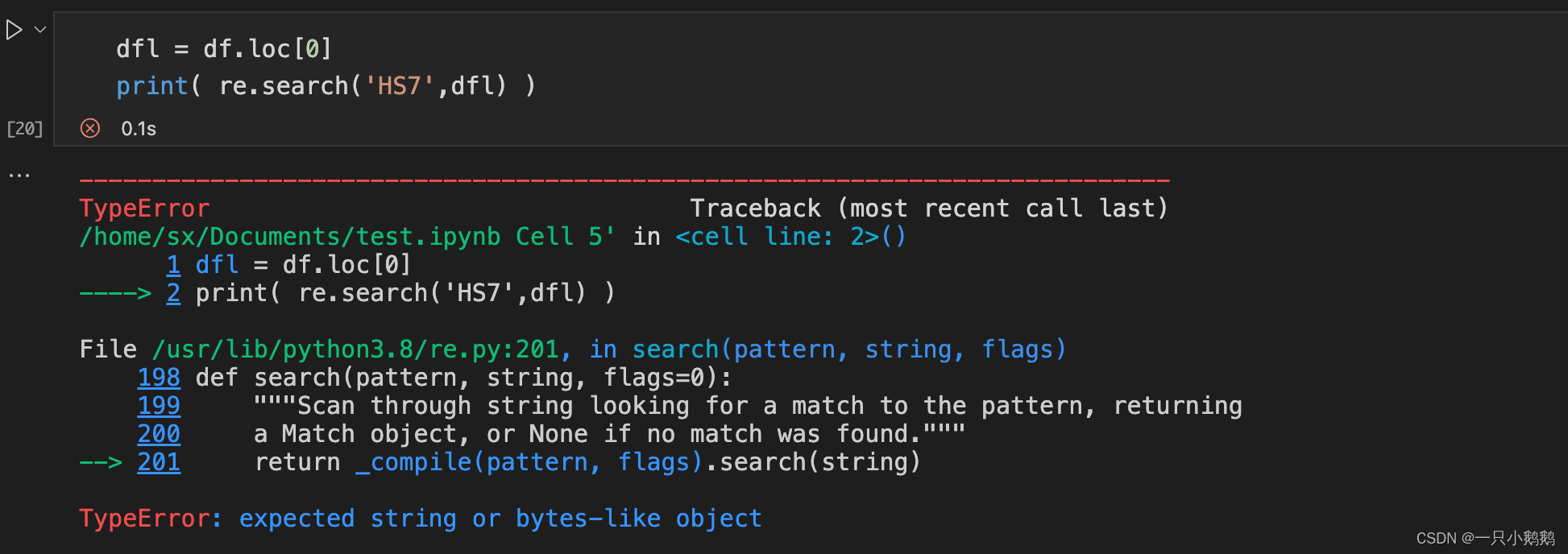

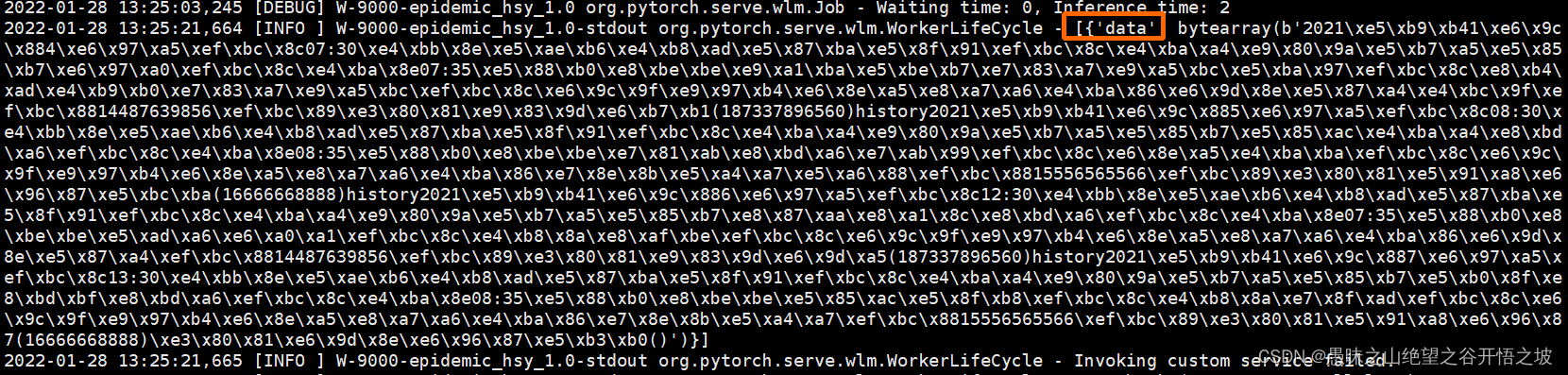
![Typeerror expected str bytes or os.pathlike object not list [FIXED] Typeerror Expected Str Bytes Or Os.Pathlike Object Not List [Fixed]](https://itsourcecode.com/wp-content/uploads/2023/04/typeerror-expected-str-bytes-or-os-pathlike-object-not-list.png)

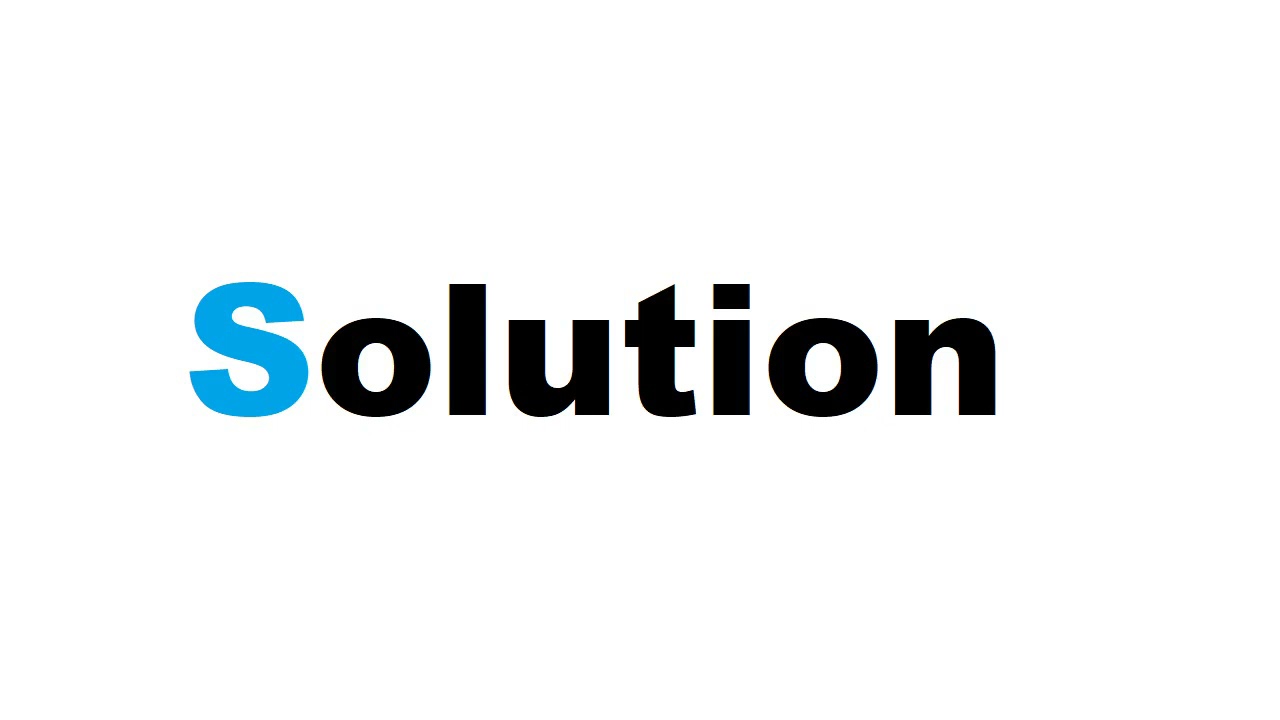


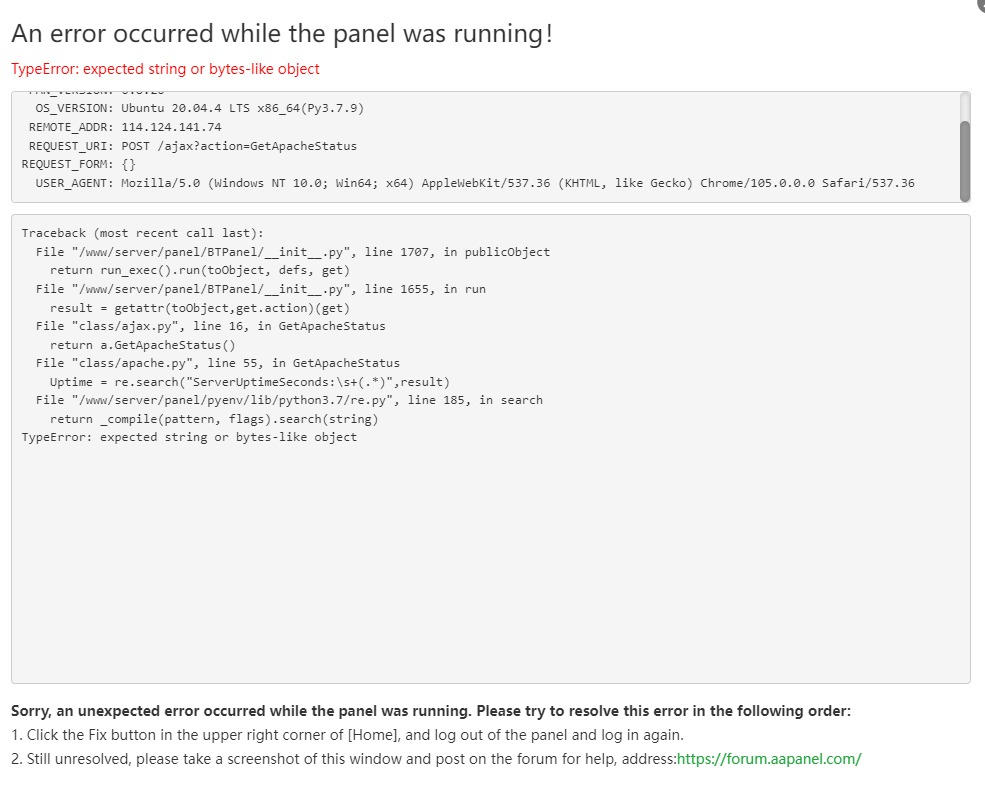


![Fixed] Expected str bytes or os.pathlike object Error - Python Pool Fixed] Expected Str Bytes Or Os.Pathlike Object Error - Python Pool](https://www.pythonpool.com/wp-content/uploads/2023/01/expected-str-bytes-or-os.pathlike-object.webp)

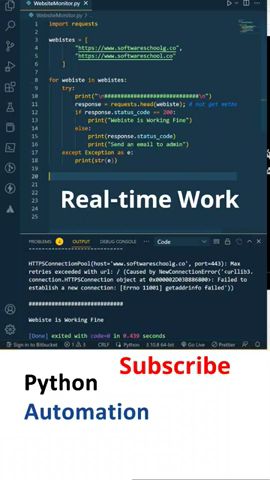
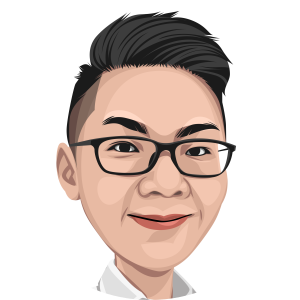

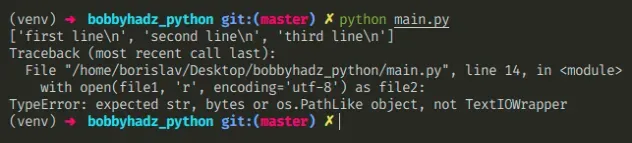
![Python Error] TypeError: expected string or bytes-like object Python Error] Typeerror: Expected String Or Bytes-Like Object](https://img1.daumcdn.net/thumb/C176x176/?fname=https://blog.kakaocdn.net/dn/GANO7/btq0fkMS2nK/oNdOqvOPpkfeJvkPEopV0K/img.png)
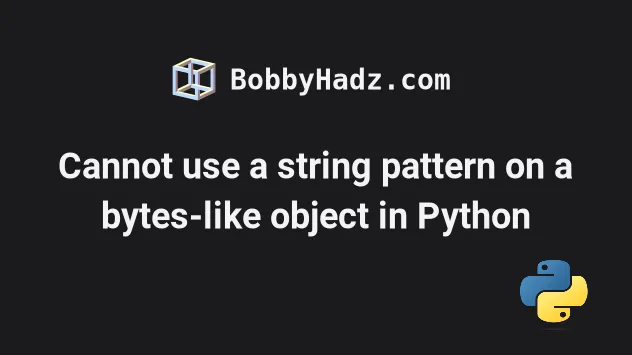
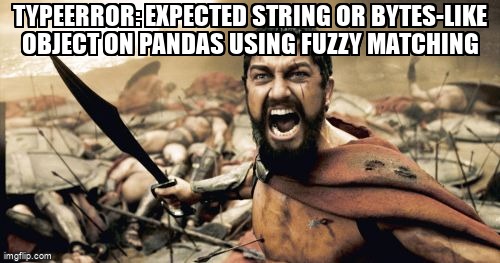
Article link: typeerror expected string or bytes like object.
Learn more about the topic typeerror expected string or bytes like object.
- TypeError: expected string or bytes-like object in Python
- How to Fix: Typeerror: expected string or bytes-like object
- Solve Python TypeError: expected string or bytes-like object
- re.sub erroring with “Expected string or bytes-like object”
- TypeError expected string or bytes-like object – STechies
- Resolving TypeError: A Bytes-like Object is Required, Not ‘str’ in Python
- Why is Python bytes() function used? – Toppr
- TypeError: expected string or bytes-like object in Python
- How to Fix TypeError: expected string or bytes-like object
- Typeerror: expected string or bytes-like object [SOLVED]
See more: nhanvietluanvan.com/luat-hoc