Typeerror ‘Float’ Object Is Not Subscriptable
Python is a powerful and versatile programming language that allows users to perform various operations and manipulations on different data types. However, sometimes, while working with Python, you might encounter an error message that says “TypeError: ‘float’ object is not subscriptable.” This error message indicates that you are attempting to use subscripting on a float object, which is not supported in Python.
1. Understanding the concept of subscripting in Python
Subscripting is the process of accessing elements or items from a sequence type object using square brackets ([]). In Python, subscripting is commonly used with data types such as lists, tuples, and strings to access individual elements or sections of the object. For example, you can use subscripting to access a specific element within a list or extract a substring from a string.
2. Explanation of TypeError: ‘float’ object is not subscriptable
The TypeError: ‘float’ object is not subscriptable occurs when you try to apply subscripting operations on a float object. This error typically arises when you mistakenly use subscripting syntax on an object of type float, which is not iterable or indexable.
3. Attempting subscripting on a float object
Let’s consider an example to understand how this error occurs:
“`python
num = 3.14
print(num[0])
“`
In the above code, we are trying to access the first element of the float object “num” using subscripting with [0]. Since float objects are not subscriptable, Python raises a TypeError: ‘float’ object is not subscriptable.
4. How subscripting is used with different data types in Python
Subscripting is commonly used with various data types in Python. For example:
– Lists: You can access individual elements of a list using their indexes. For instance, `my_list[0]` will access the first element of the list.
– Strings: Subscripting can be used with strings to extract specific characters or substrings. For example, `my_string[0]` will retrieve the first character of the string.
– Tuples: Tuples, similar to lists, can be subscripted to access specific elements.
– Dictionaries: In dictionaries, subscripting is used to retrieve values associated with specific keys.
5. Consequences of subscripting a float object
When you try to subscript a float object in Python, you will encounter the “TypeError: ‘float’ object is not subscriptable” error. This error will prevent the execution of the program and disrupt the expected flow of operations. Therefore, it’s essential to handle this error effectively to ensure smooth program execution.
6. Common mistakes leading to ‘float’ object is not subscriptable error
The most common mistake leading to the “TypeError: ‘float’ object is not subscriptable” error is attempting to access individual elements or sections within a float object using subscripting syntax. Remember that float objects are not iterable or indexable, so any attempt to subscript them will result in an error.
A common mistake may occur when you try to use subscripting on a variable without realizing its data type. For example:
“`python
result = 10.5
print(result[0]) # This will raise a TypeError
“`
In the above code, the programmer mistakenly tries to access the first element of the float object “result,” resulting in a TypeError.
7. Identifying and debugging the error
To identify and debug the “TypeError: ‘float’ object is not subscriptable” error, you can follow these steps:
– Review the error message and identify the specific line of code that raises the error.
– Check if you are attempting to subscript a float object. If yes, remove the subscripting syntax accordingly.
– Ensure that you understand the data types of the objects you are working with. Verify that subscripting is supported for that particular data type.
– Check for any typos or syntax errors in your code. Sometimes, a simple typo can result in errors like this.
8. Handling the ‘float’ object is not subscriptable error
To handle the “TypeError: ‘float’ object is not subscriptable” error, you can use conditional statements or try-except blocks to ensure that subscripting is only used with supported data types.
“`python
result = 10.5
if isinstance(result, (list, tuple, str)):
# Perform subscripting operations here
else:
# Handle the error or perform alternative operations
“`
In the above code, we check if the “result” variable is an instance of a supported data type and then perform subscripting accordingly. If the data type is not supported, you can choose to handle the error gracefully or use alternative approaches to accomplish the desired outcome.
9. Alternative approaches to accomplish the desired outcome
If you encounter the “TypeError: ‘float’ object is not subscriptable” error, you can consider alternative approaches to achieve your desired outcome. For example:
– If you need to access specific digits of a float number, you can convert the float to a string and then use string subscripting to access individual characters.
– If you are working with a list containing float elements and want to perform subscripting operations, ensure that you are using the correct index values.
– If you need to perform mathematical calculations involving floats, ensure that you are using appropriate mathematical operations instead of subscripting.
FAQs:
Q: What other similar error messages can occur in Python related to subscripting?
A: Other similar error messages related to subscripting include “TypeError: ‘type’ object is not subscriptable,” “TypeError: ‘int’ object is not subscriptable,” and “TypeError: ‘object’ is not subscriptable.”
Q: How can I convert a list of float numbers into a list of integers in Python?
A: You can use the map() function along with the int() function to convert a list of float numbers to integers. Here’s an example:
“`python
float_list = [1.5, 2.7, 3.2]
int_list = list(map(int, float_list))
“`
Q: What does the error message “TypeError: ‘property’ object is not subscriptable” mean in Python?
A: This error commonly occurs when you try to apply subscripting operations on a property object instead of the underlying value. Make sure to access the property correctly or use its underlying value for subscripting.
Q: How can I convert a list of strings representing float numbers to a list of float numbers in Python?
A: You can use the map() function along with the float() function to convert a list of string representations of float numbers to actual float numbers. Here’s an example:
“`python
string_list = [“1.5”, “2.7”, “3.2”]
float_list = list(map(float, string_list))
“`
Q: Can I convert a list of integers to a list of float numbers in Python?
A: Yes, you can use the map() function along with the float() function to convert a list of integers to float numbers. Here’s an example:
“`python
int_list = [1, 2, 3]
float_list = list(map(float, int_list))
“`
In conclusion, the “TypeError: ‘float’ object is not subscriptable” error occurs when you try to apply subscripting operations on a float object, which is not supported in Python. It is essential to understand the concept of subscripting, the limitations of float objects, and how to handle this error effectively to ensure smooth program execution. By following the guidelines mentioned in this article, you can overcome this error and accomplish your desired outcome efficiently.
Fix Typeerror Int Or Float Object Is Not Subscriptable – Python
Keywords searched by users: typeerror ‘float’ object is not subscriptable TypeError: ‘type’ object is not subscriptable, Int’ object is not subscriptable, Object is not subscriptable python3, Convert float list to int Python, TypeError property object is not subscriptable, List to float Python
Categories: Top 98 Typeerror ‘Float’ Object Is Not Subscriptable
See more here: nhanvietluanvan.com
Typeerror: ‘Type’ Object Is Not Subscriptable
When working with Python, you might come across a common error message that says “TypeError: ‘type’ object is not subscriptable.” This error is related to subscripting, which is a way of accessing individual elements in an iterable object like a list or a string. In this article, we will explore the meaning of this error, its causes, and possible solutions for resolving it.
What does the error message mean?
The error message itself provides a crucial clue to understanding the issue. It states that a “type” object, rather than a subscriptable object, is being accessed. In Python, a type object refers to the class or type of an object. Subscripting, on the other hand, allows us to access elements within an iterable object using square brackets [].
Causes of the ‘type’ object is not subscriptable error
Several factors can lead to this error message. Let’s discuss some of the common causes:
1. Incorrect usage of parentheses: One common mistake that can trigger this error is misusing parentheses instead of square brackets. For example, attempting to access elements within a list like this: “my_list(0)” instead of “my_list[0]” will raise a ‘type’ object is not subscriptable error.
2. Overwriting built-in Python types: Python has numerous built-in types, such as list, dict, and tuple. If you accidentally overwrite these types, for instance, by assigning a list to a variable named “list,” it can lead to a ‘type’ object is not subscriptable error because “list” now refers to the variable rather than the built-in type.
3. Incorrect assignment of variables: Another possible cause is assigning a type object itself to a variable and then trying to access it using subscript notation. For example, “my_variable = int” and then attempting to access the variable using “my_variable[0]” will cause the error.
4. Invalid usage of functions or methods: Using functions or methods in an inappropriate way can also result in a ‘type’ object is not subscriptable error. It may occur when trying to invoke a function on a class object instead of an instance of that class.
Solutions to the ‘type’ object is not subscriptable error
Now that we understand the potential causes of this error, let’s explore some solutions:
1. Check for incorrect usage of parentheses: Review your code to ensure that you are using square brackets instead of parentheses when accessing items within iterable objects like lists, strings, or tuples.
2. Avoid variable name conflicts: Be cautious while naming your variables to prevent conflicts with Python’s built-in types. Double-check your code for any variables that might have overwritten these types and rename them if necessary.
3. Verify variable assignments: Make sure you are not assigning a type object itself to a variable if you intend to use subscript notation. Ensure that your variables are properly assigned to instances of the types you are working with.
4. Review function or method invocations: If you are encountering the error while using a function or method, ensure that you are calling it correctly. Verify that you are applying it to an instance of a class, rather than the class itself.
Frequently Asked Questions (FAQs)
Q1. Can this error occur with any object in Python?
Yes, this error can occur with any object in Python, but it is most commonly seen when working with lists, strings, or tuples.
Q2. Why is subscripting important in Python?
Subscripting allows us to access individual elements within iterable objects. It is a fundamental concept in Python because it enables us to manipulate and retrieve specific data efficiently.
Q3. Are there any other similar errors I should be aware of?
Yes, there are a few similar errors you may encounter while working with Python, such as “TypeError: ‘int’ object is not iterable” or “TypeError: ‘dict’ object does not support indexing.” These errors have similar causes and can be resolved using similar approaches as discussed in this article.
In conclusion, the ‘type’ object is not subscriptable error is a common mistake faced by Python developers. It occurs when a type object is accessed using subscript notation, which should be used for accessing elements within iterable objects instead. By understanding the causes and following the suggested solutions in this article, you can effectively troubleshoot and resolve this error, ensuring smooth execution of your Python programs.
Int’ Object Is Not Subscriptable
When working with Python programming, you may come across an error message that says “TypeError: ‘int’ object is not subscriptable.” This error can be quite confusing for beginners and even for experienced programmers at times. In this article, we will dive deep into what this error means, why it occurs, and how to resolve it.
Understanding the Error:
The error message “‘int’ object is not subscriptable” usually occurs when you try to access or index an integer as if it were a list or dictionary. In Python, subscripting refers to accessing elements within an iterable by using square brackets and providing an index or key.
For example, consider the following code snippet:
“` python
num = 12345
print(num[2])
“`
Attempting to run this code will result in the ‘int’ object not subscriptable error. This is because integers are not iterable, meaning you cannot access individual elements within them using subscript notation.
Why Does this Error Occur?
The error occurs because subscripting is applicable only to iterable objects like lists, strings, and dictionaries in Python. These data types are inherently designed to allow access and manipulation of individual elements.
When you try to subscript an integer, Python treats it as if you were trying to access an element within it, which is not allowed. Since integers are considered immutable objects, they do not support subscripting operations.
How to Resolve the ‘int’ object not subscriptable Error:
Fortunately, resolving this error is relatively straightforward. To fix it, you need to ensure that you are using subscripting on iterable objects such as lists, strings, or dictionaries, rather than on integers.
Here are a few steps you can take to troubleshoot and resolve the error:
1. Check the variable type:
Before subscripting any object, it’s crucial to ensure that the variable you are working with is of an iterable type. Verify the type of the object using the `type()` function to avoid mistakenly subscripting an integer.
2. Double-check your code:
Review your code and ensure that you are not mistakenly attempting to subscript an integer. It’s easy to overlook this error, especially if you are dealing with multiple variables and data types.
3. Use the correct data type:
If your intention is to manipulate individual elements, consider using a list or a string instead of an integer. Lists and strings are iterable objects that allow subscripting operations.
Now, let’s address some frequently asked questions to further clarify the topic:
FAQs:
Q: Can I subscript an integer value if it is part of a list?
A: Yes, you can subscript an integer if it is part of a list. In Python, you can access individual elements within a list using square brackets and the corresponding index. However, if you try to directly subscript the integer itself, you will still encounter the same ‘int’ object is not subscriptable error.
Q: Is there any other scenario where this error might occur?
A: The ‘int’ object not subscriptable error can also occur when you mistakenly try to access elements within a string using invalid index values. For example, if you provide an index that is larger than the length of the string, Python will throw this error.
Q: What other similar errors might I encounter while working with subscripting?
A: Apart from the ‘int’ object not subscriptable error, you may also come across a few others, such as ‘float’ object is not subscriptable or ‘tuple’ object is not subscriptable. These errors occur when you mistakenly try to subscript a float or a tuple, respectively.
In conclusion, the “‘int’ object is not subscriptable” error in Python is encountered when you try to access or index an integer as if it were a list or dictionary. This error can easily be resolved by ensuring that you are using subscripting operations on iterable objects like lists, strings, or dictionaries. By understanding the error message and following the recommended steps, you can quickly identify and fix this type of error in your Python code.
Object Is Not Subscriptable Python3
When we receive the “object is not subscriptable” error message, it means that we are trying to access an element using square brackets, but the object we are trying to access does not support such operations. Let’s take a closer look at some common scenarios where this error can occur.
One common cause of this error is trying to access an element using indexing or slicing on an object that is not subscriptable. For example, consider the following code snippet:
“`python
name = “John”
print(name[0])
“`
In this case, we are trying to access the first character of the string using indexing. Since strings are subscriptable in Python, this code will work perfectly fine and output ‘J’. However, if we try the same operation on an object that is not subscriptable, such as an integer or float, we will encounter the “object is not subscriptable” error.
Another common cause of this error is mistakenly assuming that a certain object is subscriptable when it’s not. For example, consider the following code snippet:
“`python
person = {
“name”: “John”,
“age”: 25,
“city”: “New York”
}
for i in range(3):
print(person[i])
“`
In this case, we are trying to access the elements of the dictionary using numeric indexes. However, dictionaries in Python are not subscriptable, as they are unordered collections of key-value pairs. Therefore, trying to access dictionary elements using indexing will result in the “object is not subscriptable” error.
Now that we understand the causes of this error, let’s explore some possible solutions. The simplest way to avoid this error is to make sure that the object we are trying to access supports indexing or slicing operations. If we are dealing with a string, list, tuple, or dictionary, then indexing and slicing will work perfectly fine. However, if we are working with an object that is not subscriptable, we need to find an alternative approach.
If the object in question is a dictionary, we can access its values using the respective keys. For example:
“`python
person = {
“name”: “John”,
“age”: 25,
“city”: “New York”
}
print(person[“name”])
“`
By using the correct keys, we can access the values stored in the dictionary without encountering the “object is not subscriptable” error.
If the object is not a dictionary, we should reassess our approach and determine if indexing or slicing is necessary. If not, we can modify our code accordingly. If indexing or slicing is indeed required, we might need to convert the object into a subscriptable form. For example, if we have a non-subscriptable object like a set, we can convert it into a list before accessing its elements. Here’s an example:
“`python
num_set = {1, 2, 3, 4, 5}
num_list = list(num_set)
print(num_list[0])
“`
In this case, we first convert the set into a list using the `list()` function, and then access the first element using indexing. By doing so, we prevent the “object is not subscriptable” error and obtain the desired result.
Now, let’s address some frequently asked questions (FAQs) about the “object is not subscriptable” error:
Q: Why does Python have objects that are not subscriptable?
A: Python is a dynamically-typed language that allows flexibility in data structures. Not all objects support indexing or slicing because they are not designed to be accessed in a sequential manner. Subscriptability depends on the nature and purpose of the object.
Q: How can I identify if an object is subscriptable?
A: To determine if an object is subscriptable or not, you can use the `hasattr()` function. For example, `hasattr(obj, “__getitem__”)` will return `True` if the object is subscriptable.
Q: Can I make an object subscriptable if it’s not by default?
A: Yes, you can make an object subscriptable by implementing the `__getitem__` special method in your custom object. This method allows you to define how the object should behave when indexing or slicing is attempted.
Q: Is there a performance difference between subscriptable and non-subscriptable objects?
A: Generally, subscriptable objects have direct access to their elements, resulting in a faster performance compared to non-subscriptable objects. However, this performance difference might not be noticeable unless dealing with a large number of iterations or a highly optimized code.
In conclusion, the “object is not subscriptable” error occurs when we try to access an element of an object that does not support indexing or slicing. By understanding the causes and applying appropriate solutions, we can overcome this error and ensure smooth execution of our Python programs. Remember to always double-check the subscriptability of an object and adjust your code accordingly to avoid running into this error.
Images related to the topic typeerror ‘float’ object is not subscriptable
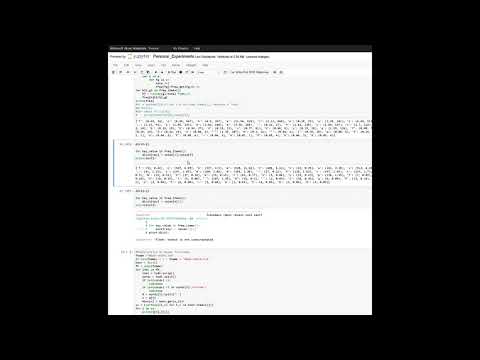
Found 44 images related to typeerror ‘float’ object is not subscriptable theme
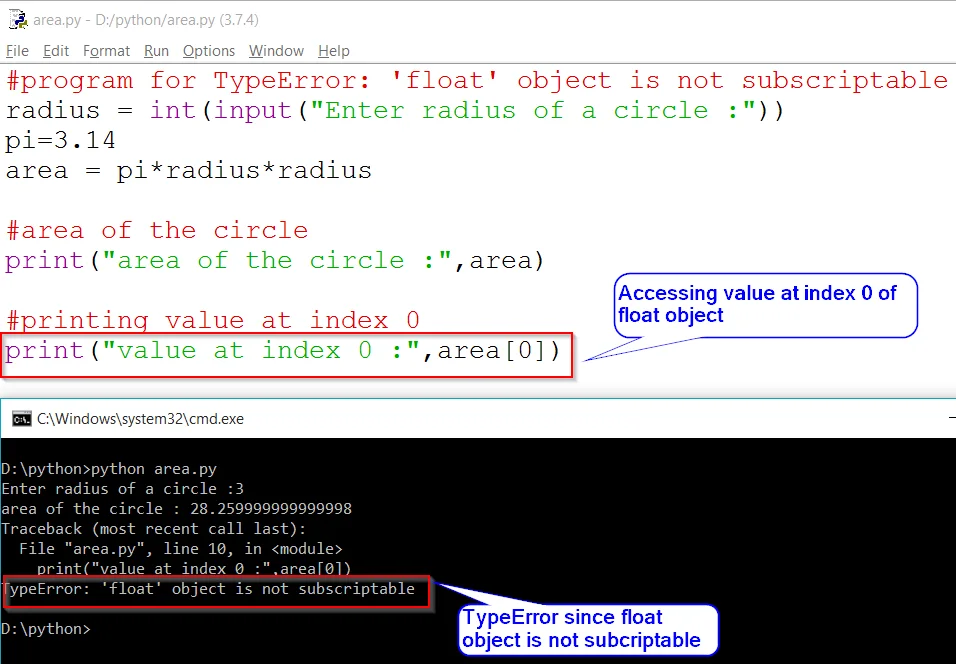
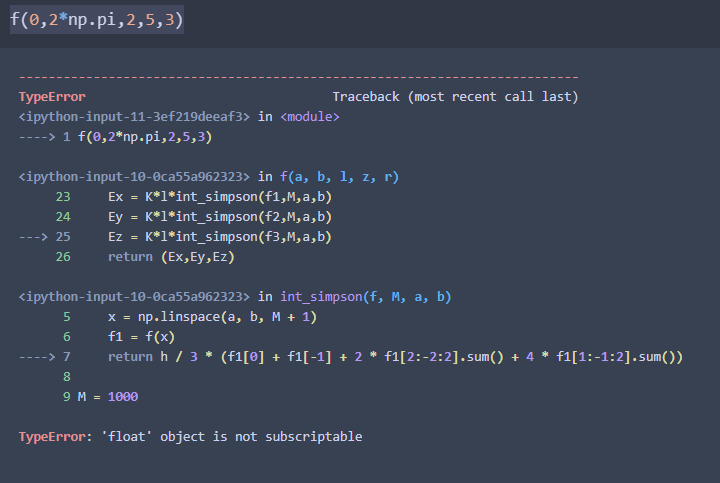

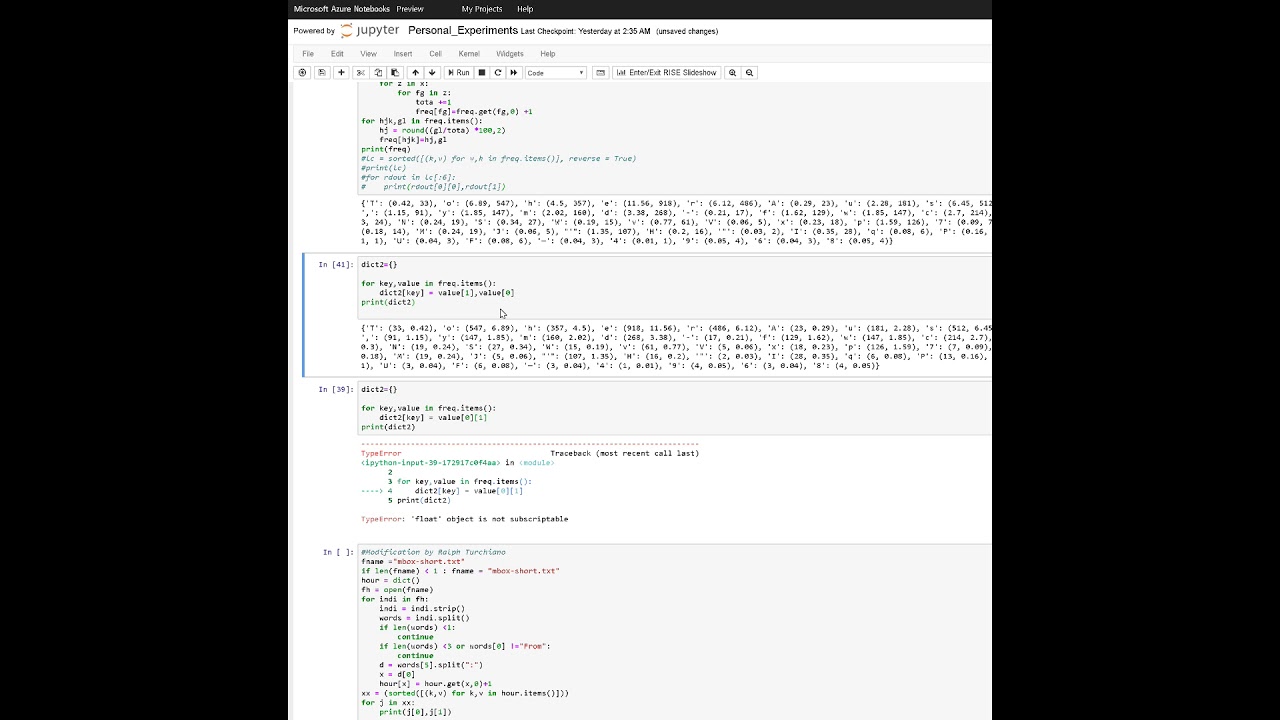

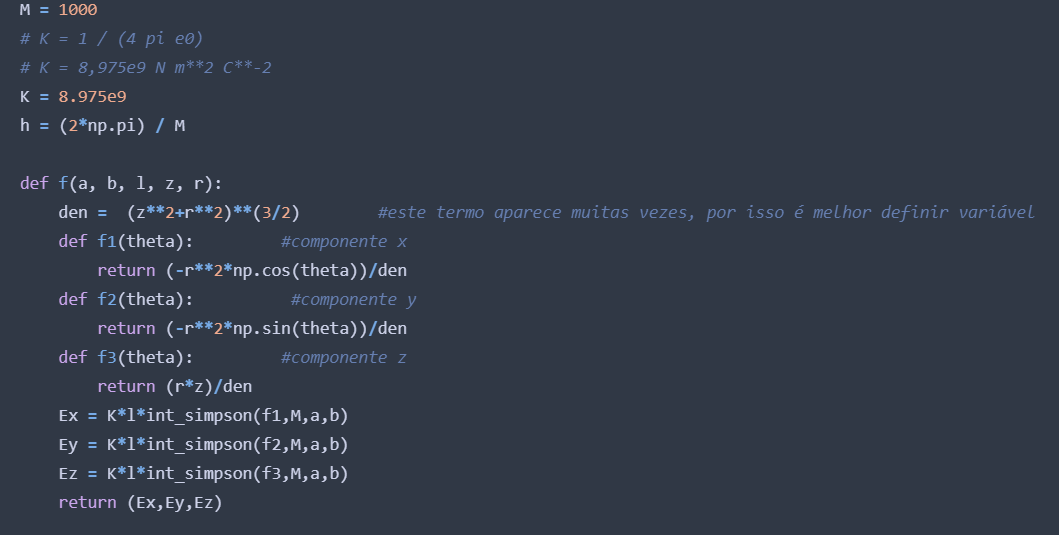
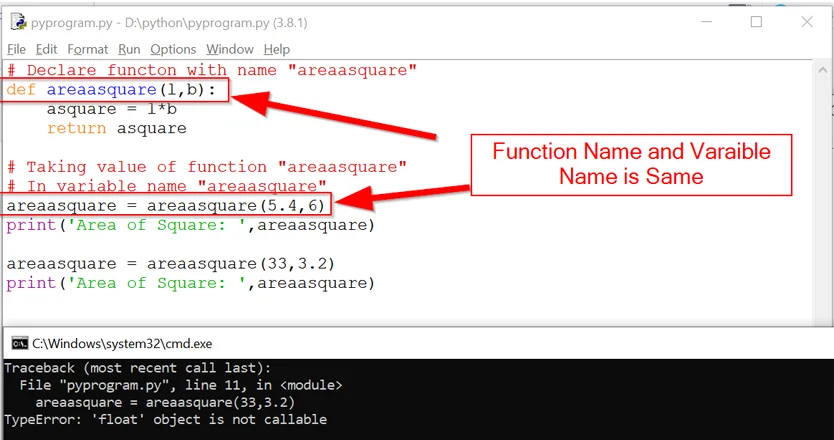
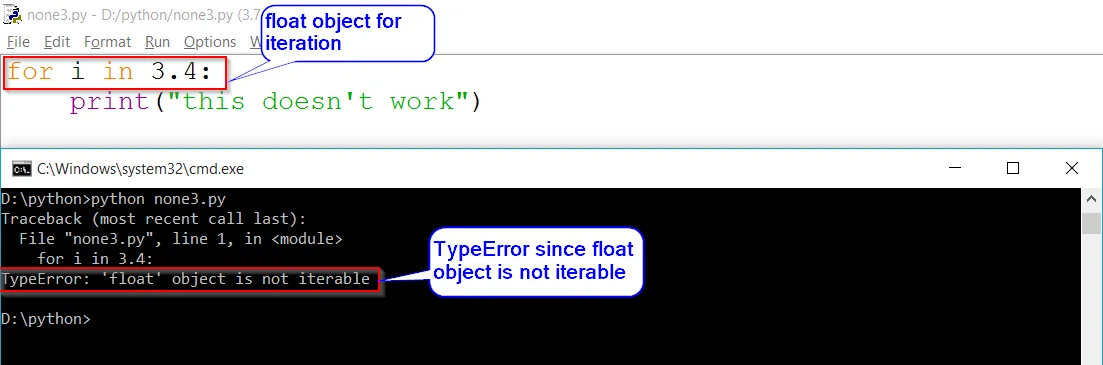
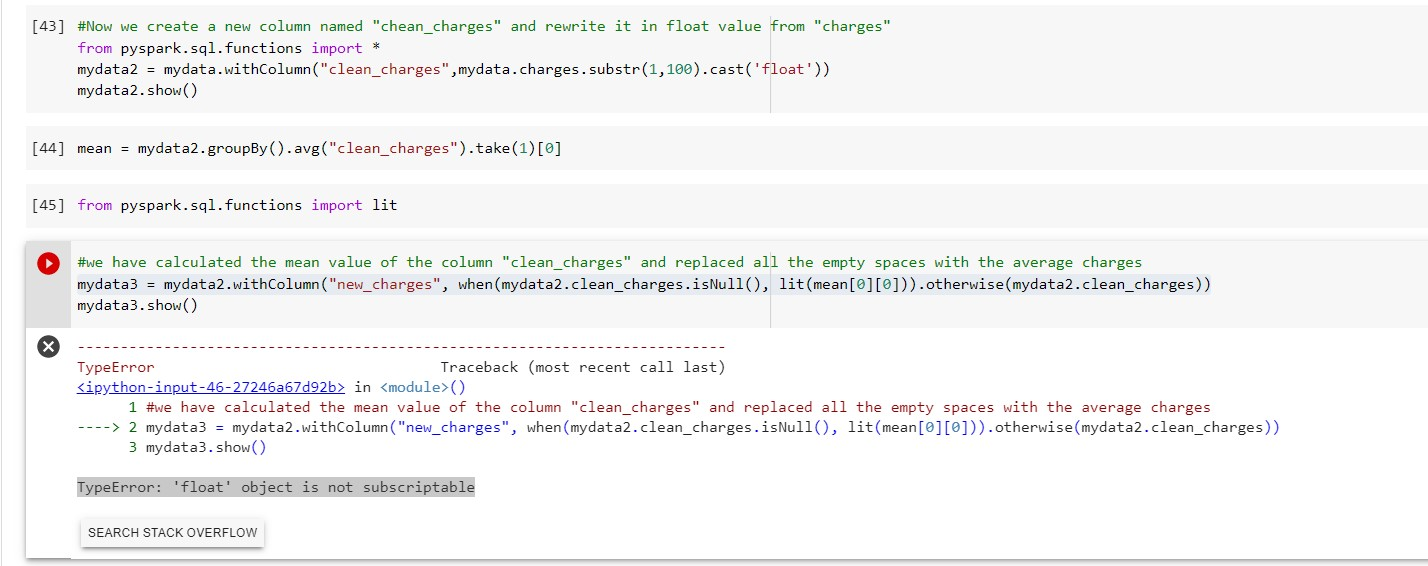
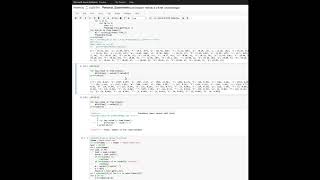

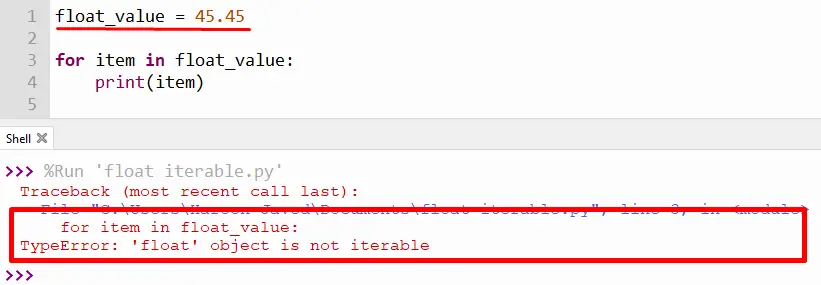
![TypeError: 'int' object is not subscriptable [Solved Python Error] Typeerror: 'Int' Object Is Not Subscriptable [Solved Python Error]](https://www.freecodecamp.org/news/content/images/2022/10/in_not_subable.png)
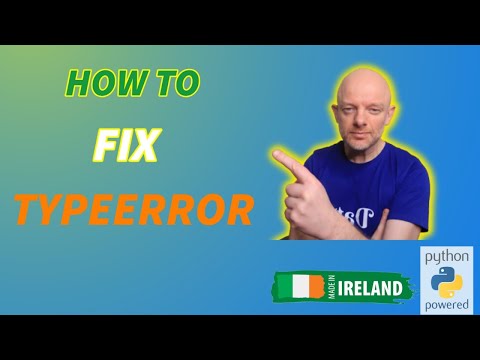
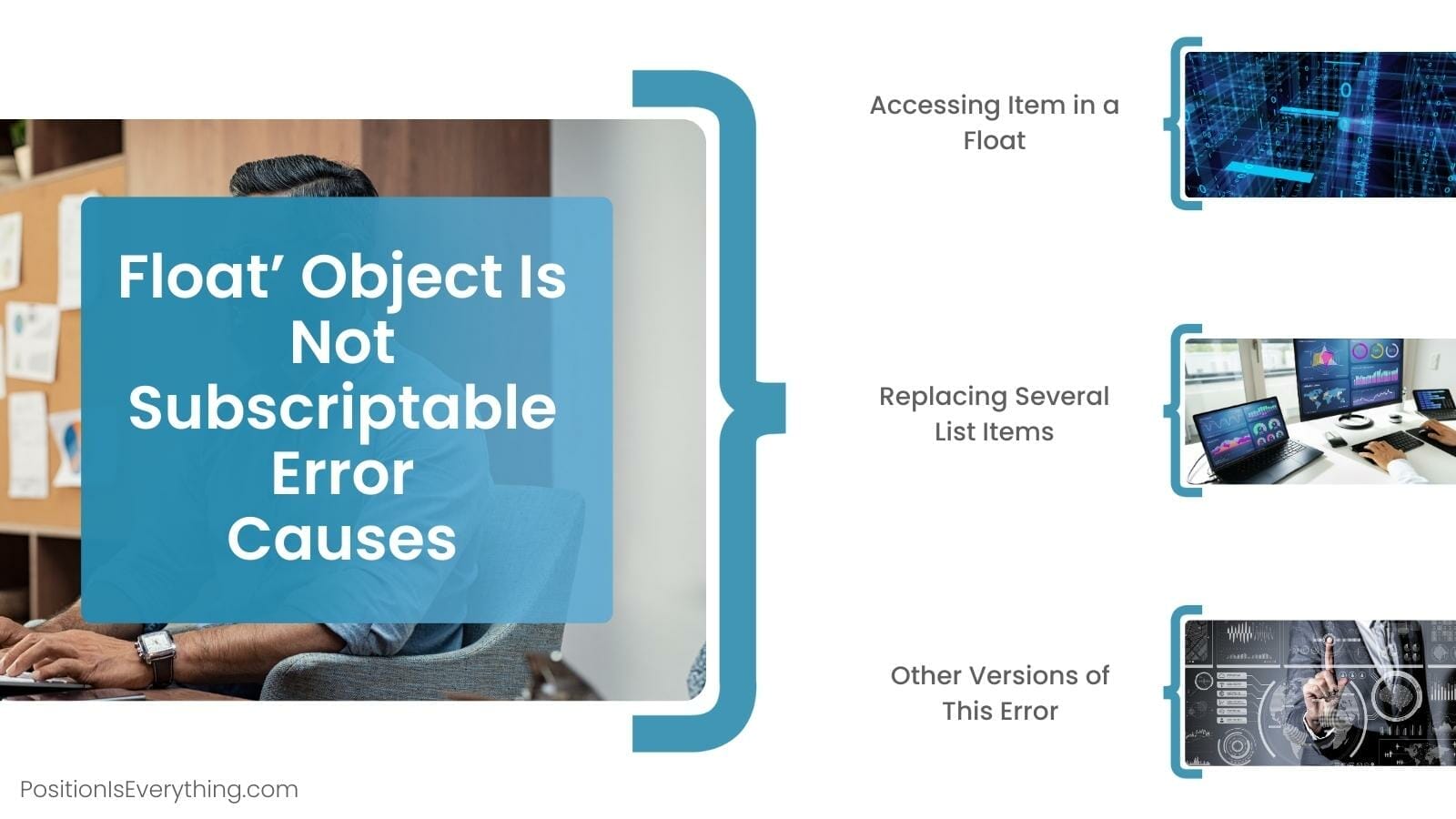
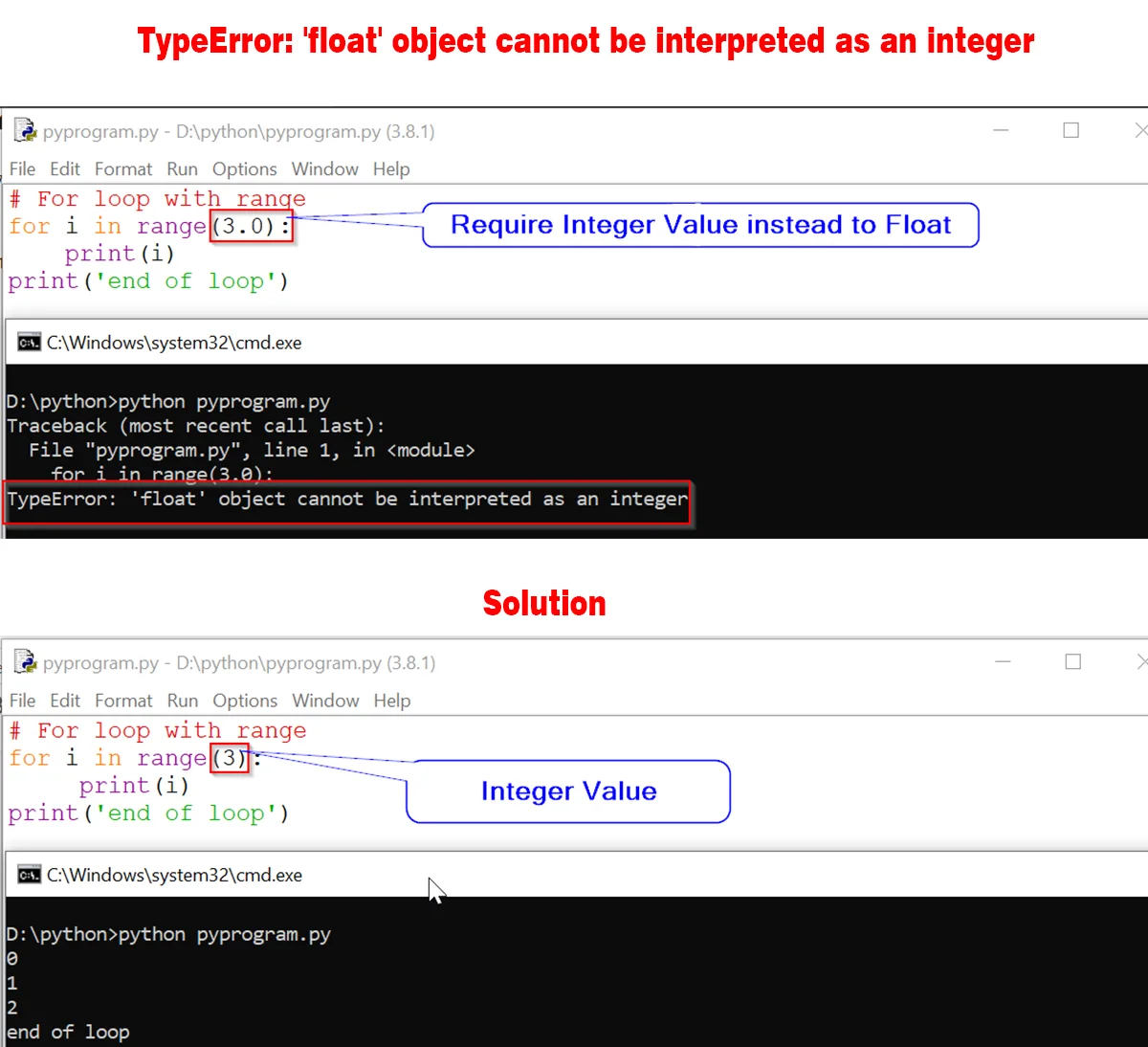
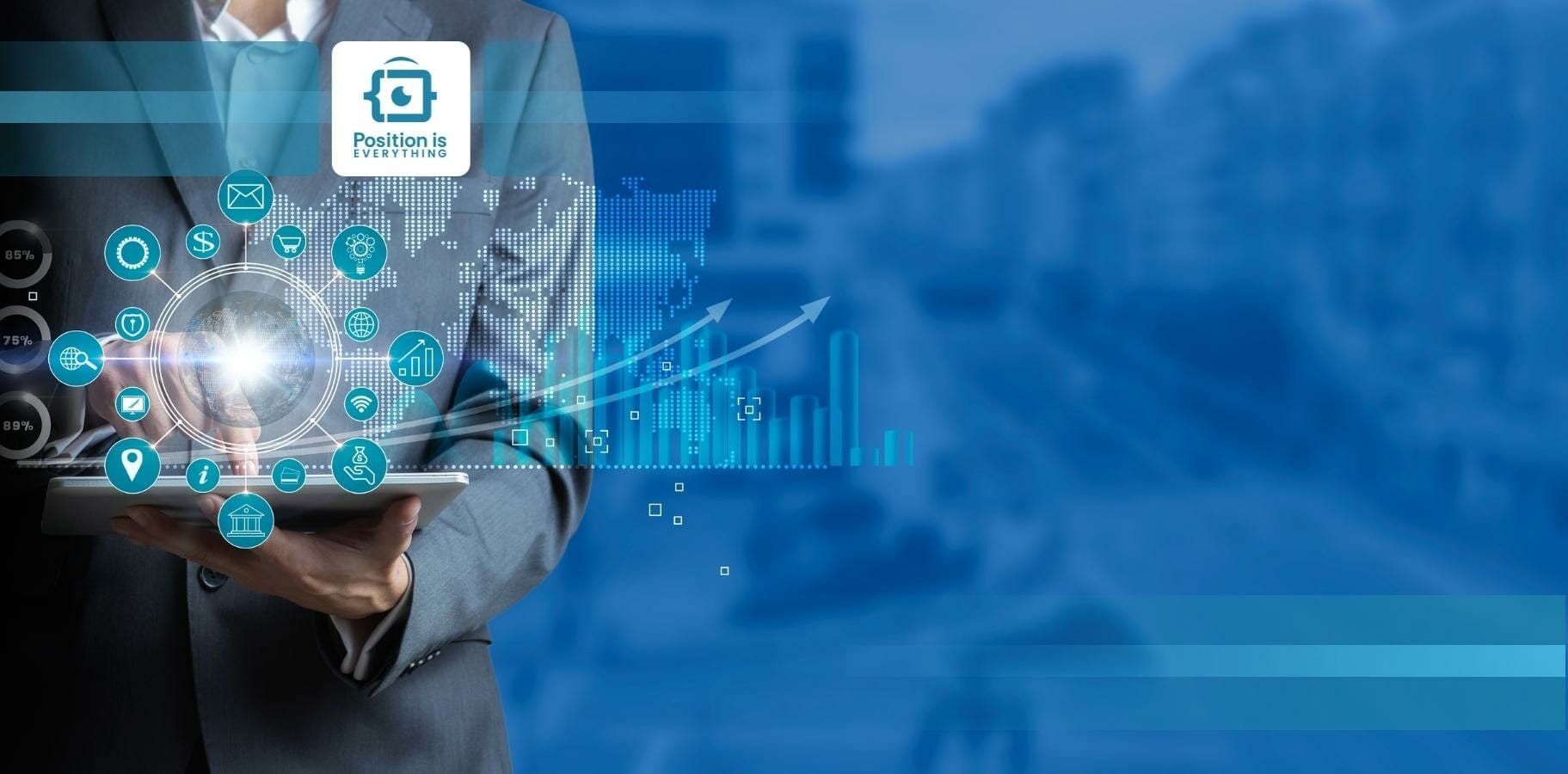
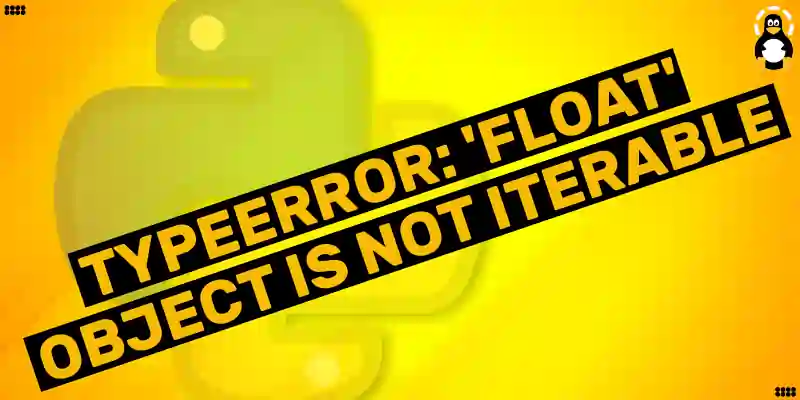
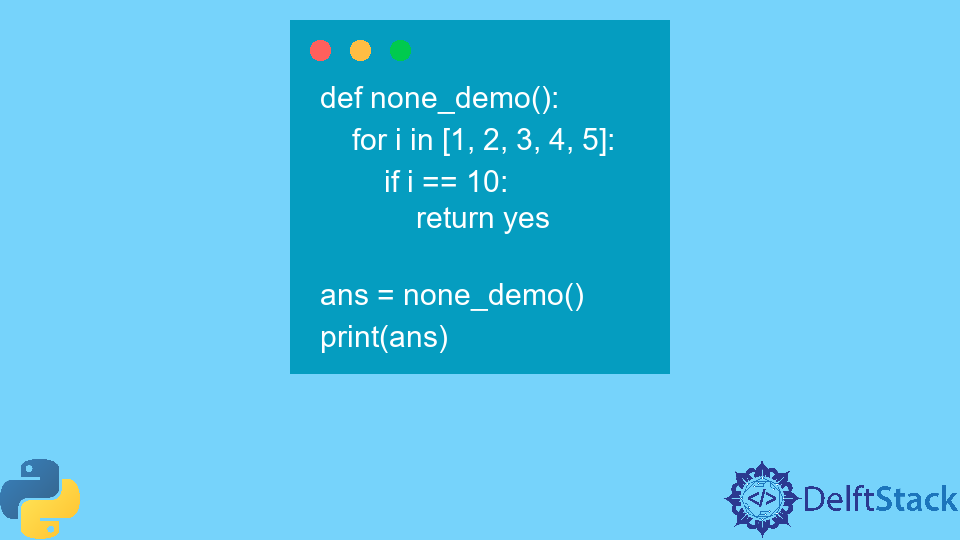
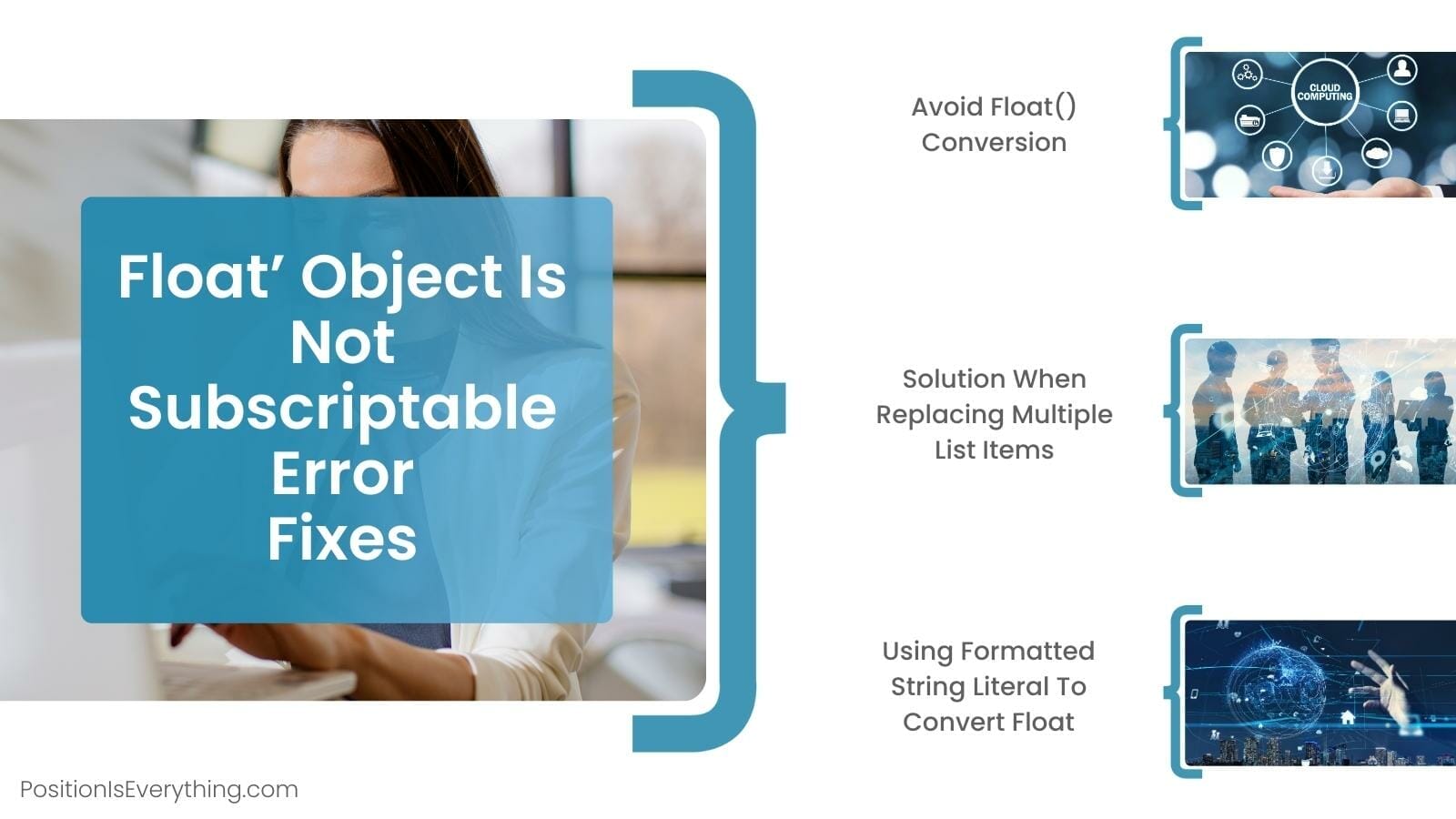


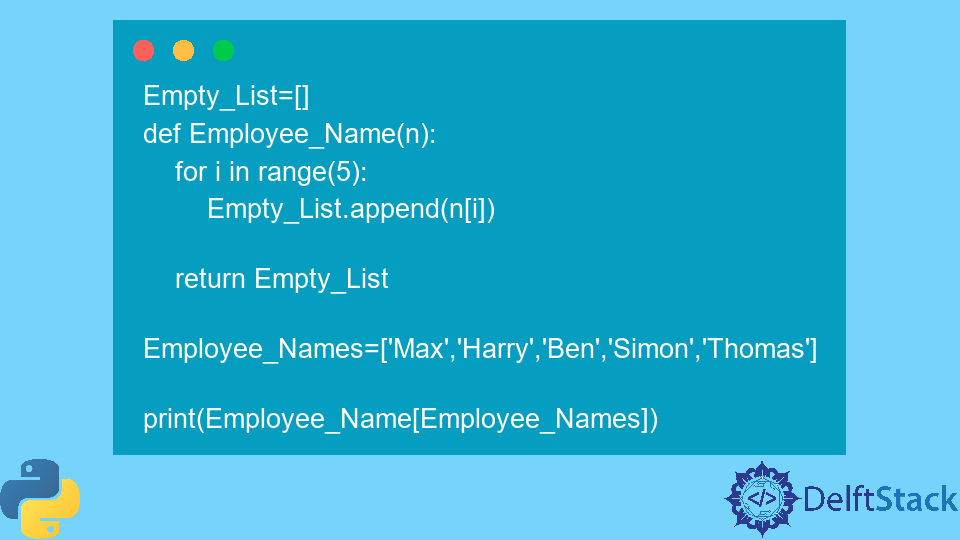
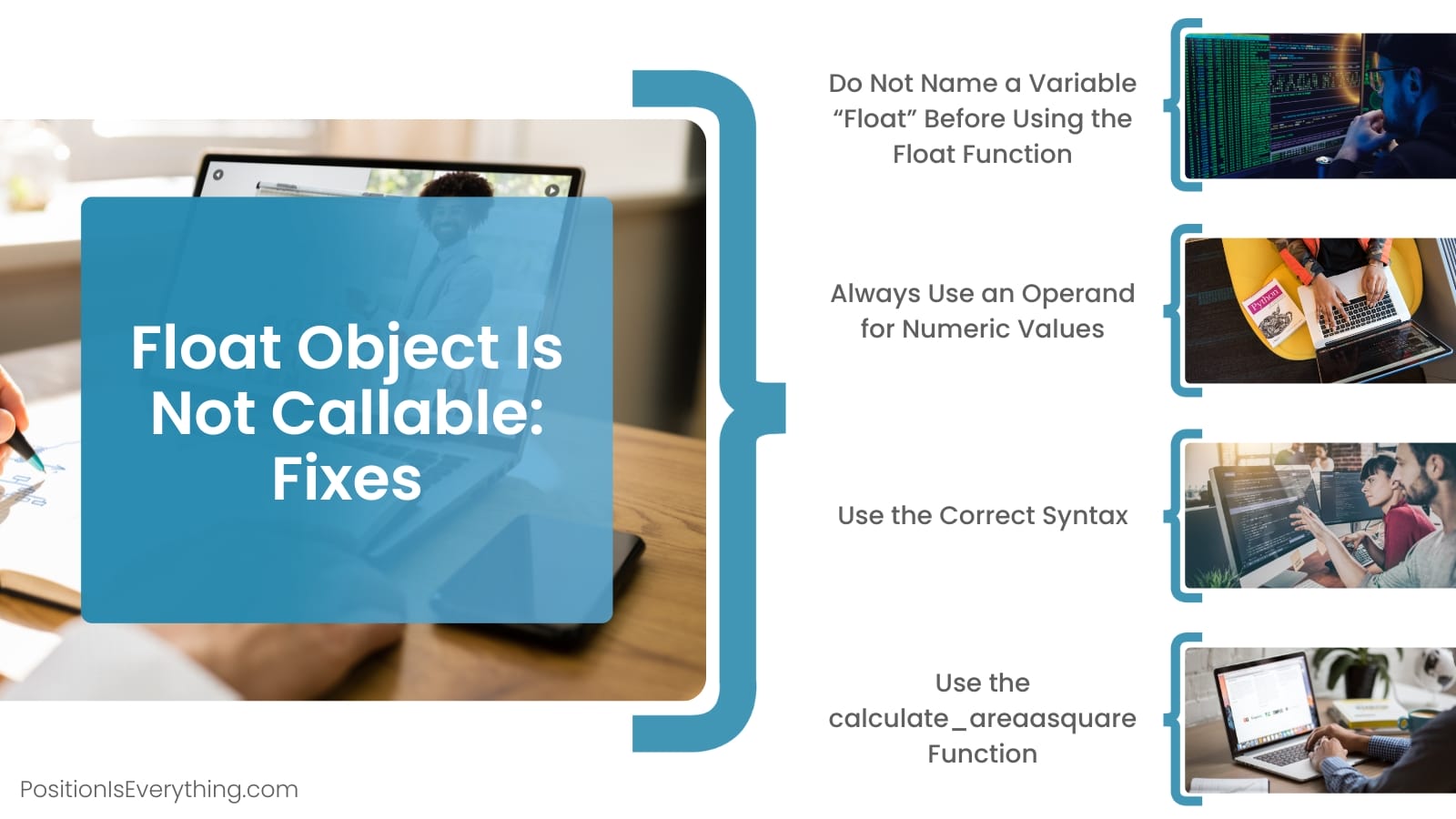
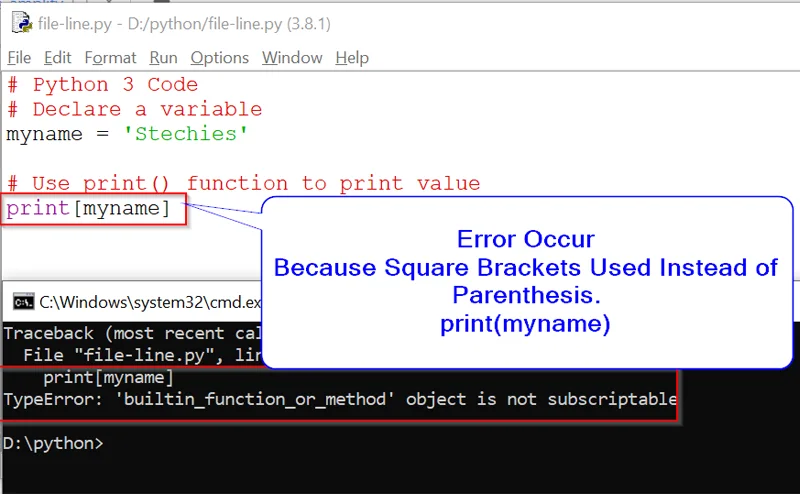
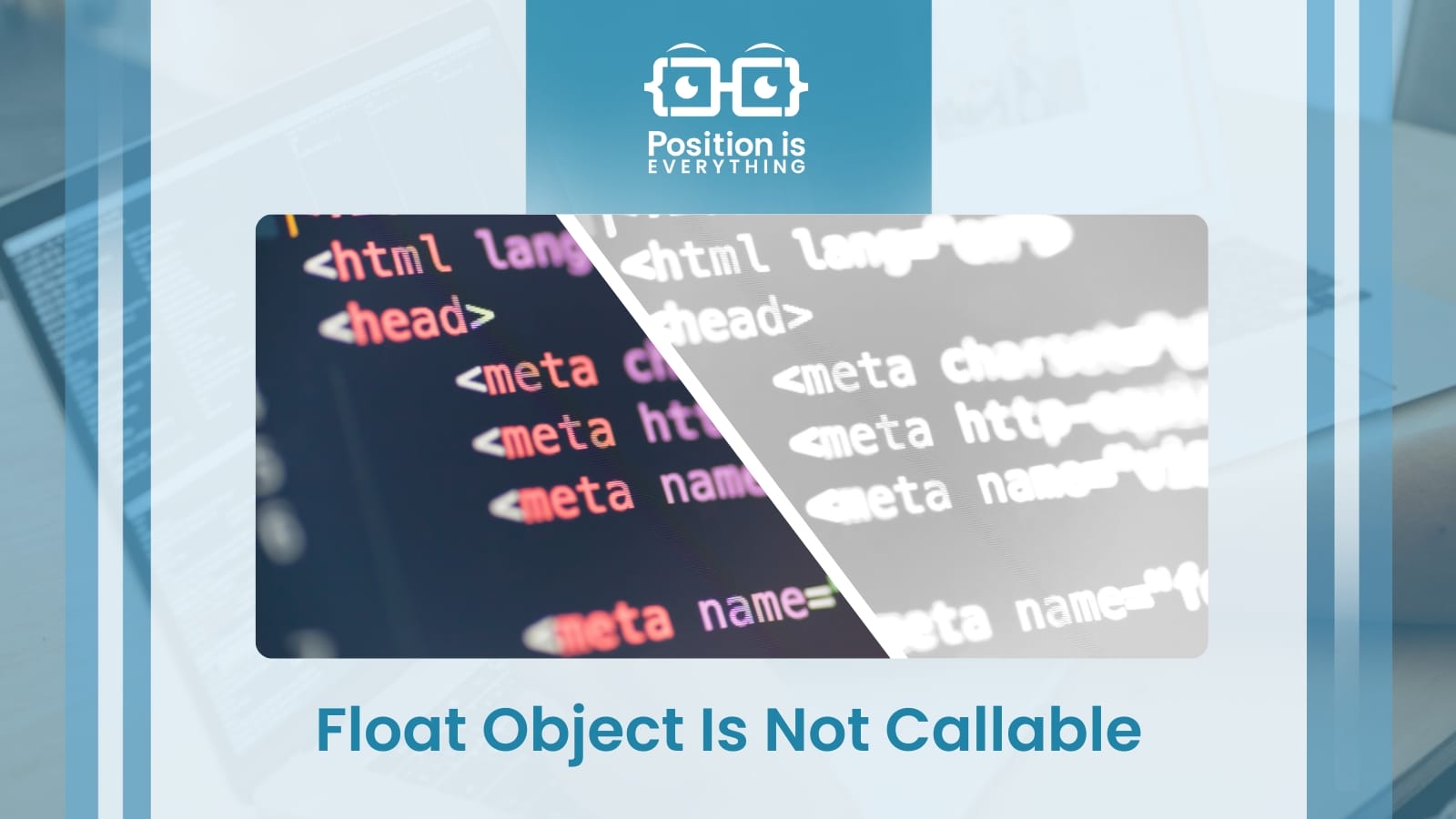

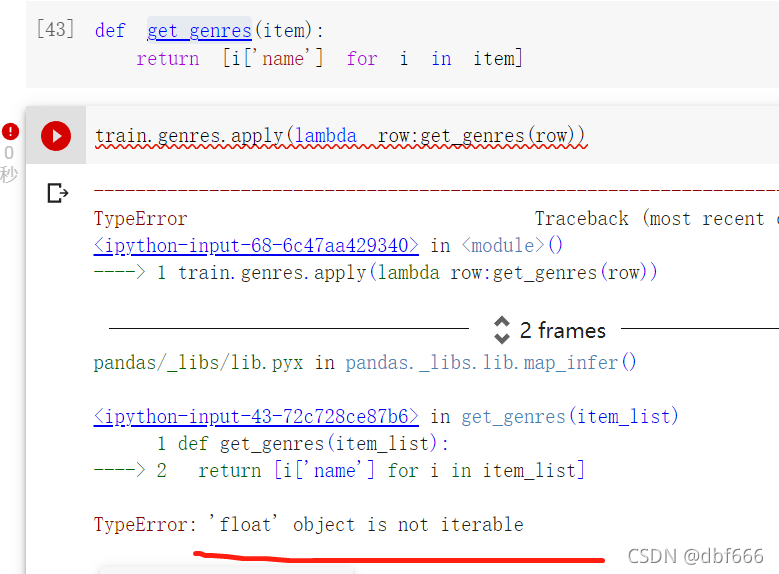


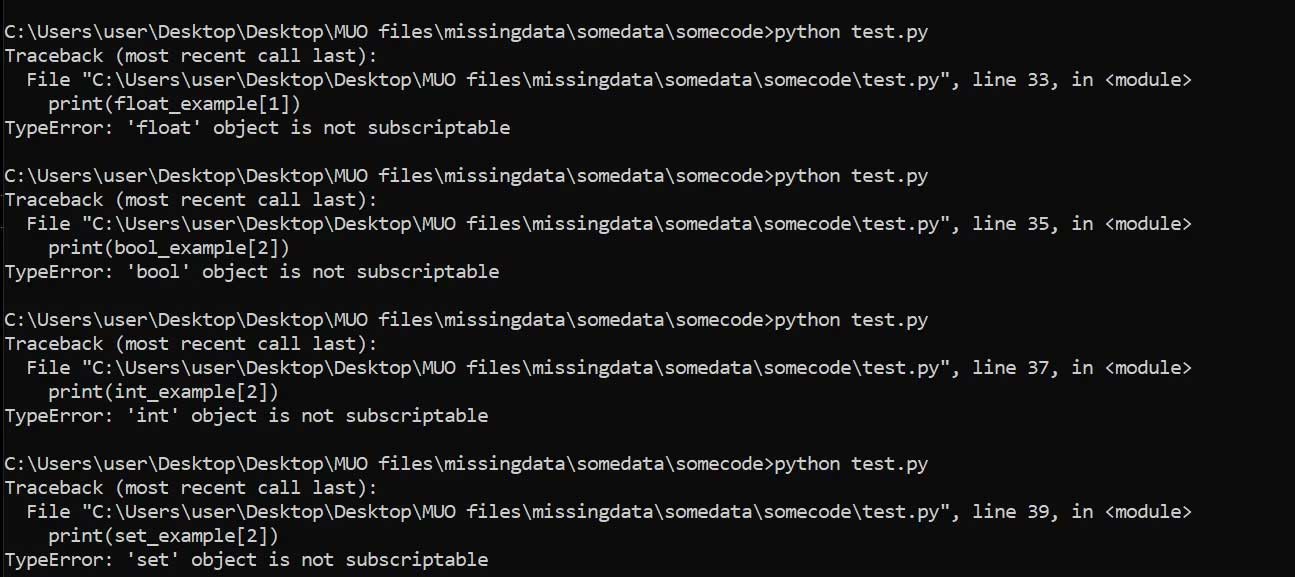

![Solved] TypeError: 'int' Object Is Not Subscriptable in Python – Be on the Right Side of Change Solved] Typeerror: 'Int' Object Is Not Subscriptable In Python – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2021/03/typeError.png)




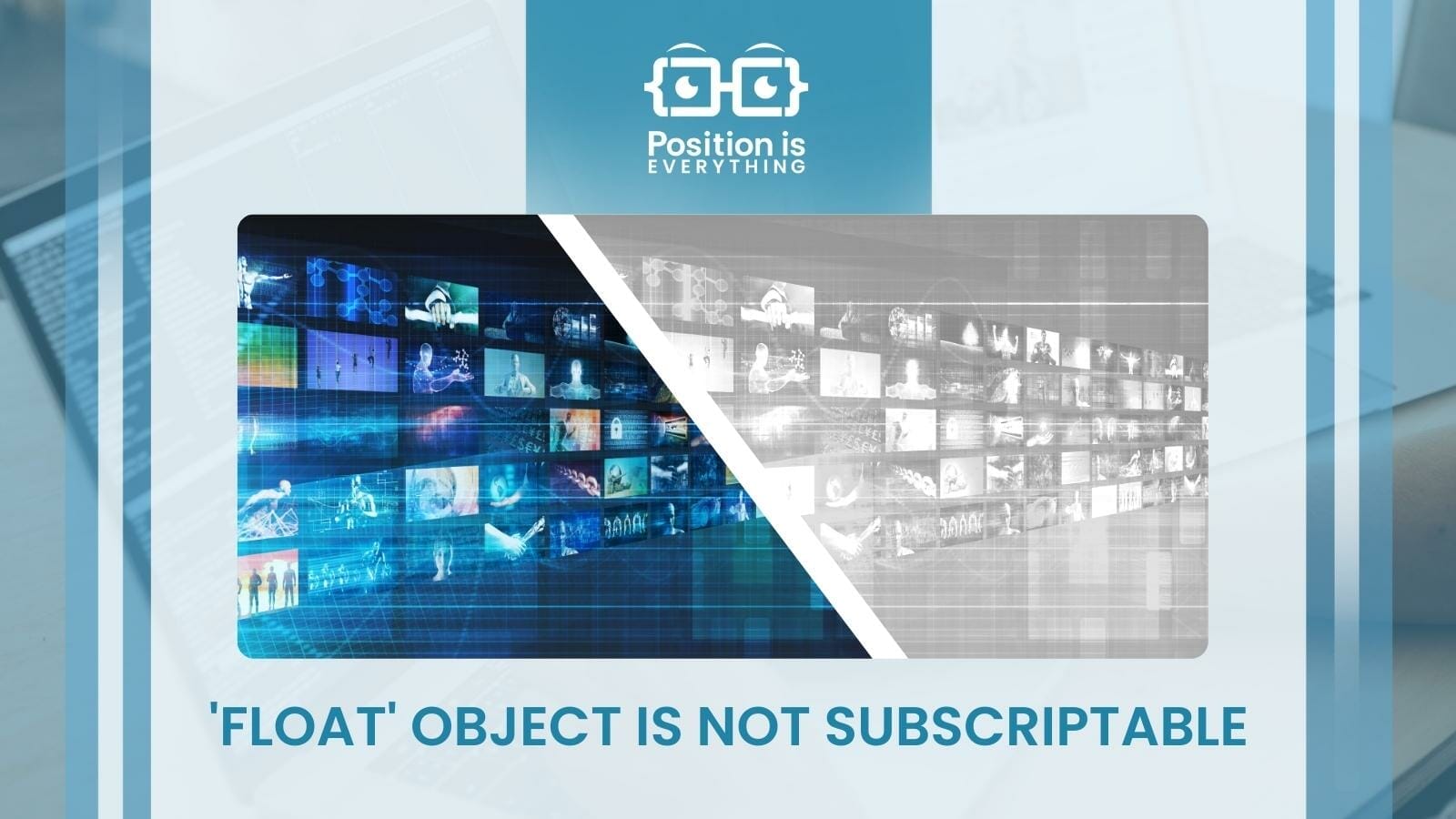


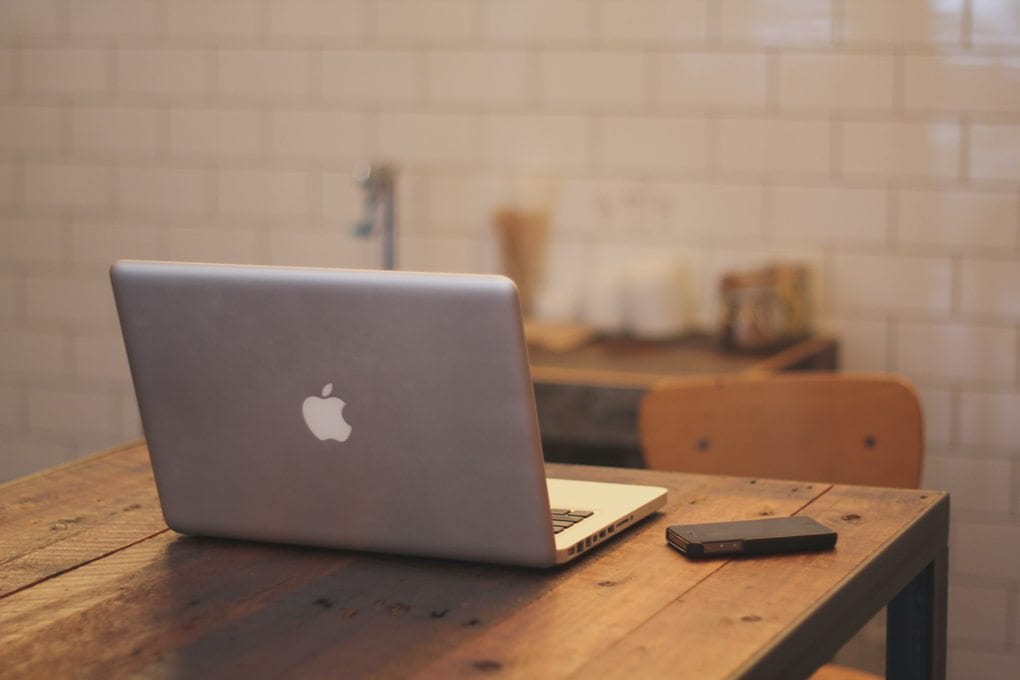
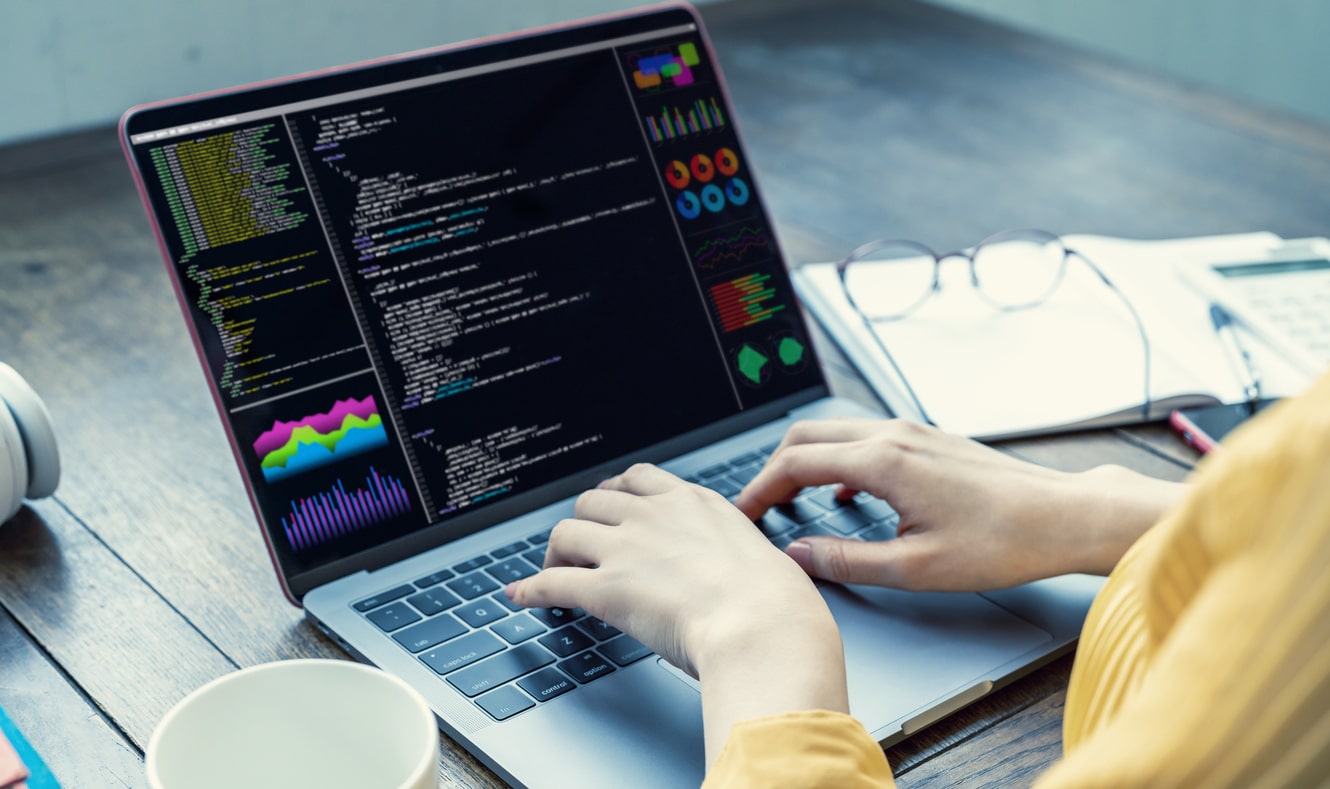
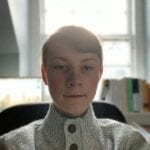
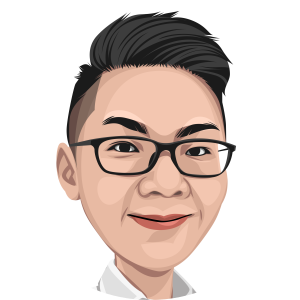
Article link: typeerror ‘float’ object is not subscriptable.
Learn more about the topic typeerror ‘float’ object is not subscriptable.
- TypeError: ‘float’ object is not subscriptable in Python
- TypeError: ‘float’ object is not subscriptable – Stack Overflow
- (Solved) Python TypeError: ‘float’ object is not subscriptable
- TypeError: ‘float’ object is not subscriptable – STechies
- Python typeerror: ‘float’ object is not subscriptable Solution
- Solve Python TypeError: ‘float’ object is not subscriptable
- Typeerror: ‘float’ object is not subscriptable [SOLVED]
- ‘float’ object is not subscriptable: How to fix it in Python
- [Solved] TypeError: ‘float’ object is not subscriptable
- ‘float’ object is not subscriptable python – AI Search Based Chat
See more: nhanvietluanvan.com/luat-hoc