Write To Text File In C#
In C#, writing data to a text file is a common task in many applications. Whether you need to store logs, save user preferences, or generate reports, being able to write data to a text file is essential. Fortunately, C# provides a simple and efficient way to accomplish this.
This article will guide you through the process of writing data to a text file in C#. We will cover importing the required namespaces, creating or opening a text file, writing data to the file, appending data to an existing file, closing the file, handling exceptions, and best practices for writing to a text file.
Importing the Required Namespaces
Before we can start writing to a text file, we need to import the necessary namespaces in our C# code. The primary namespace we need is the System.IO namespace, which provides classes and methods for working with files and directories. To import this namespace, add the following line at the beginning of your C# file:
“`
using System.IO;
“`
Creating or Opening a Text File
To write data to a text file, we first need to create or open an existing text file. We can do this using the StreamWriter class. The StreamWriter class provides methods to write text to a file synchronously or asynchronously. Let’s see how to create a new text file and open it for writing:
“`
// Specify the file path
string filePath = “C:\\example.txt”;
// Create a new text file and open it for writing
StreamWriter writer = new StreamWriter(filePath);
“`
In the above code, we specified the file path as “C:\\example.txt”. You can change the file path to the desired location on your system. The StreamWriter constructor takes the file path as an argument and creates a new text file at the specified location. If the file already exists, it will be overwritten.
Writing Data to the Text File
Once we have opened the text file for writing, we can start writing data to it. The StreamWriter class provides several methods for writing text. The most commonly used method is the Write method, which writes a string to the file. We can also use the WriteLine method to write a string followed by a newline character. Here’s an example of writing data to a text file:
“`
// Write a string to the text file
writer.Write(“Hello World!”);
// Write a string followed by a newline character
writer.WriteLine(“This is a new line”);
“`
In the above code, we first use the Write method to write the string “Hello World!” to the text file. Then, we use the WriteLine method to write the string “This is a new line” followed by a newline character. The newline character ensures that each line of text is written on a separate line in the file.
Appending Data to an Existing Text File
In some cases, you may need to append data to an existing text file instead of overwriting it. To achieve this, we can open the file in append mode by passing an additional argument to the StreamWriter constructor. Here’s an example:
“`
// Open the text file in append mode
StreamWriter writer = new StreamWriter(filePath, true);
// Append data to the file
writer.WriteLine(“This line will be appended”);
“`
In the above code, we passed `true` as the second argument to the StreamWriter constructor, which opens the file in append mode. This ensures that any data we write will be added to the end of the file, rather than overwriting it.
Closing the Text File
Once we have finished writing data to the text file, it is important to close the file to release any system resources associated with it. We can do this by calling the Close method on the StreamWriter object. Here’s an example:
“`
// Close the text file
writer.Close();
“`
By closing the file, we ensure that any data that has been written is flushed from the memory and permanently saved to the disk. Failing to close the file can result in data loss or other errors.
Handling Exceptions
When working with file operations, it is important to handle exceptions that may occur. File-related operations can encounter various exceptions, such as when the file is inaccessible, the disk is full, or the file path is invalid. To handle exceptions, we can use try-catch blocks. Here’s an example:
“`
try
{
// Perform file operations here
}
catch (IOException e)
{
// Handle the exception here
Console.WriteLine(“An error occurred: ” + e.Message);
}
“`
In the above code, we have enclosed the file operations within a try block. If any exception occurs during the execution of the try block, it will be caught in the catch block. In this example, we catch the IOException, which is a commonly thrown exception by file-related operations. Inside the catch block, we can handle the exception gracefully, such as displaying an error message to the user.
Best Practices for Writing to a Text File in C#
When writing data to a text file in C#, it is important to follow some best practices to ensure efficient and reliable code:
1. Use using statement: To ensure that system resources are properly cleaned up, it is recommended to use the using statement when working with the StreamWriter class. The using statement automatically calls the Dispose method on the StreamWriter object, which releases any resources used by the object.
“`
using (StreamWriter writer = new StreamWriter(filePath))
{
// Perform file operations here
}
“`
2. Buffering: By default, the StreamWriter class uses buffering for better performance. However, if you want to ensure that every write operation is immediately saved to the file, you can disable buffering by passing `false` as the second argument to the StreamWriter constructor.
“`
using (StreamWriter writer = new StreamWriter(filePath, false))
{
// Perform file operations here
}
“`
3. Encodings: The StreamWriter class allows you to specify the text encoding when writing to a text file. By default, it uses UTF-8 encoding, which supports a wide range of characters. If you need to write text in a different encoding, you can pass the desired encoding as an argument to the StreamWriter constructor.
“`
using (StreamWriter writer = new StreamWriter(filePath, Encoding.ASCII))
{
// Perform file operations here
}
“`
FAQs
Q: How can I read a file in C?
A: To read a file in C, you can use the fopen function to open the file, and then use the fscanf or fgets function to read the file line by line.
Q: How can I read a text file in C?
A: Reading a text file in C is similar to reading any file. You can use the fopen function to open the text file, and then use the fscanf or fgets function to read the text file line by line.
Q: How can I write a file in C?
A: To write a file in C, you can use the fopen function to open the file in write mode, and then use the fprintf or fputs function to write data to the file.
Q: How can I read a file in C++?
A: In C++, you can read a file using the ifstream class. You can open the file using the open method, and then use the getline or >> operators to read the file line by line or data by data.
Q: What is a file in C?
A: In C, a file is a collection of data stored on a secondary storage device, such as a hard disk. It can be a text file or a binary file, containing text or binary data, respectively.
Q: How can I open a file in C?
A: To open a file in C, you can use the fopen function, which takes two arguments: the file path and the mode. The mode specifies whether you want to open the file for reading, writing, or appending, among other options.
Q: What is fscanf() in C?
A: fscanf is a function in C that reads formatted data from a file. It works similarly to the scanf function, but instead of reading input from the standard input (keyboard), it reads input from a file.
Q: How can I write a line to a file in C#?
A: To write a line to a file in C#, you can use the StreamWriter class’s WriteLine method. Simply create an instance of the StreamWriter class, open the file for writing, and then use the WriteLine method to write a string followed by a newline character.
In conclusion, writing data to a text file in C# is a straightforward process. By importing the required namespaces, creating or opening a text file, writing data to the file, appending data to an existing file, closing the file, handling exceptions, and following best practices, you can efficiently and reliably write data to a text file in your C# applications.
#29: C File Handling | C Programming For Beginners
How To Write In A Text File In C?
Have you ever wondered how to write data into a text file using the C programming language? Writing data into a text file is a fundamental task for many programming applications. Whether you are creating a log file, storing user information, or simply saving data for later use, knowing how to write in a text file in C is an essential skill. In this article, we will guide you through the process of writing data into a text file using C, step by step.
1. Opening a Text File:
To start writing in a text file, you must first open it. The `fopen()` function is used for this purpose. It takes two arguments: the name of the file you want to open and the mode in which you want to open it. For writing to a file, you need to pass the mode as `”w”`, which stands for “write”. Here is an example of opening a text file named “textfile.txt” for writing:
“`c
#include
int main() {
FILE *filePointer;
filePointer = fopen(“textfile.txt”, “w”);
// Further code for writing into the file
fclose(filePointer); // Always remember to close the file
return 0;
}
“`
2. Writing Data into the File:
Now that you have opened the text file, you can use the `fprintf()` function to write data into it. `fprintf()` is formatted output, similar to `printf()`, but it writes its output to a specified file instead of the standard output. It takes a file pointer argument and a format string along with any necessary data to be written. Here is an example of writing a simple string into the file:
“`c
#include
int main() {
FILE *filePointer;
filePointer = fopen(“textfile.txt”, “w”);
fprintf(filePointer, “Hello, World!\n”);
fclose(filePointer);
return 0;
}
“`
3. Writing Multiple Lines:
To write multiple lines of data, you can simply call `fprintf()` multiple times, each time with the desired content. Each call to `fprintf()` will append the data to the existing file content. Here is an example of writing multiple lines of data into a text file:
“`c
#include
int main() {
FILE *filePointer;
filePointer = fopen(“textfile.txt”, “w”);
fprintf(filePointer, “Line 1\n”);
fprintf(filePointer, “Line 2\n”);
fprintf(filePointer, “Line 3\n”);
fclose(filePointer);
return 0;
}
“`
4. Appending Data to an Existing File:
If you want to add data to an existing file without overwriting its previous contents, you can use the file opening mode `”a”` instead of `”w”`. The `”a”` mode stands for “append”. Here is an example:
“`c
#include
int main() {
FILE *filePointer;
filePointer = fopen(“textfile.txt”, “a”);
fprintf(filePointer, “New line appended!\n”);
fclose(filePointer);
return 0;
}
“`
5. Error Handling:
While writing to a text file, it is important to check for errors that may occur during the process. You can do this by checking if the file pointer is `NULL` after calling `fopen()`, which indicates that the file was not opened successfully. Additionally, you can also use the `ferror()` function to check if any error occurred during the write operation. It returns a non-zero value if an error occurred. Here is an example:
“`c
#include
int main() {
FILE *filePointer;
filePointer = fopen(“textfile.txt”, “w”);
if (filePointer == NULL) {
printf(“Error opening the file.\n”);
return 1;
}
if (fprintf(filePointer, “Hello, World!\n”) < 0) { printf("Error writing to the file.\n"); return 1; } fclose(filePointer); return 0; } ``` FAQs: Q: Can I write data in binary format instead of text format? A: Yes, you can write data in binary format by opening the file in binary mode. Instead of using `"w"` or `"a"` mode, you would use `"wb"` or `"ab"` respectively. Q: How can I write numbers in a text file? A: Numbers can be written using the `%d`, `%f`, or `%lf` format specifiers in the `fprintf()` function. These specifiers are similar to those used in `printf()`. Q: Can I write data in a specific location within the file? A: By default, writing data using `fprintf()` appends it to the end of the file. If you want to write data at a specific offset, you would need to use more advanced file handling techniques using functions such as `fseek()` or `fwrite()`. Q: How can I ensure that my data is saved even if the program terminates unexpectedly? A: You can call `fflush(filePointer)` after each write operation to buffer the data immediately onto the disk. Additionally, closing the file with `fclose()` ensures that all buffers are flushed. Q: Are there any size limitations for writing data to a text file? A: The size of data you can write to a text file is limited by the available disk space and the data type you are writing. There are no specific limitations imposed solely by the C programming language. In conclusion, writing data into a text file using C involves opening the file, using `fprintf()` to write data, and ensuring error handling. With these basics covered, you can now confidently write data to text files using C for a wide range of applications. Practice and experimentation will help you master this essential skill. Happy coding!
How To Write Number To Text File In C?
When working with C programming language, it is common to encounter scenarios where you need to write numbers to a text file. Whether it is for data storage or printing purposes, knowing how to write numbers to a text file can be an essential skill. In this article, we will delve into the various methods and techniques involved in writing numbers to a text file in C, providing you with a comprehensive understanding of the process.
Writing Numbers to a Text File in C
Before diving into the details, let’s familiarize ourselves with the basic steps required to write numbers to a text file:
1. Open the text file: The first step is to open the file in write mode (or append mode, if you want to add the numbers to an existing file). You can achieve this by using the `fopen()` function and specifying the appropriate mode (`w` for write mode, `a` for append mode).
2. Write the numbers: Now that the file is open, you can use the `fprintf()` function to write the numbers to the file. This function is specifically designed to write formatted output to a file, making it suitable for our purpose. You can specify the format of the numbers using format specifiers such as `%d` (for integers), `%f` (for floating-point numbers), or `%ld` (for long integers), among others.
3. Close the file: Once you’ve finished writing the numbers, it is crucial to close the file using the `fclose()` function. This ensures that any changes made to the file are saved and clears up system resources.
Example: Writing Numbers to a Text File
Let’s take a closer look at an example that demonstrates how to write numbers to a text file in C:
“`c
#include
int main() {
FILE *file;
int number = 42;
file = fopen(“numbers.txt”, “w”);
if (file == NULL) {
printf(“Error opening the file.”);
return 1;
}
fprintf(file, “%d”, number);
fclose(file);
return 0;
}
“`
In this example, we open a file named “numbers.txt” in write mode, assign a value of 42 to the variable `number`, and write it to the file using the `fprintf()` function. Finally, we close the file using `fclose()`. This simple code snippet outlines the basic mechanism involved in writing numbers to a text file in C.
FAQs
Q1: Can I write multiple numbers to a text file at once?
Yes, you can write multiple numbers to a text file at once. Simply use multiple `fprintf()` statements, each corresponding to a different number. For instance, to write two numbers to a file, you could write:
“`c
fprintf(file, “%d “, number1);
fprintf(file, “%d”, number2);
“`
Q2: How can I write floating-point numbers to a text file?
To write floating-point numbers, you can use the `%f` format specifier in conjunction with `fprintf()`. For example:
“`c
float pi = 3.14159;
fprintf(file, “%f”, pi);
“`
Q3: Is it possible to store numbers as strings in the text file?
Yes, you can store numbers as strings in the text file by converting them using the `sprintf()` function. This function is similar to `fprintf()`, but instead of writing to a file, it writes to a character array. Here’s an example:
“`c
char str[10];
int number = 42;
sprintf(str, “%d”, number);
fprintf(file, “%s”, str);
“`
Q4: How can I append numbers to an existing text file?
To append numbers to an existing text file, use the “a” mode while opening the file instead of “w”. This way, the file’s contents won’t be overwritten, and the numbers will be added to the existing content. Here’s an example:
“`c
file = fopen(“numbers.txt”, “a”);
“`
Q5: Can I write numbers to a text file with specific formatting?
Absolutely! In addition to format specifiers like `%d` for integers and `%f` for floating-point numbers, you can use additional flags, such as precision and width, to control formatting. For instance:
“`c
float pi = 3.14159;
fprintf(file, “%.2f”, pi); // Writes 3.14 to the file
“`
Conclusion
Writing numbers to a text file in C involves opening the file, using `fprintf()` to write the desired format, and closing the file. Remember to handle any potential errors, such as the failure to open the file. By understanding these core principles and using the appropriate format specifiers, you can successfully write numbers to a text file. Happy coding!
Keywords searched by users: write to text file in c# Read file in C, Read text file in C, Write file in C, Read file C++, File in C, Open file in C, Fscanf() in C, Write line to file in c
Categories: Top 75 Write To Text File In C#
See more here: nhanvietluanvan.com
Read File In C
In computer programming, reading data from a file is a common task that developers often encounter. The C programming language provides several methods to read files efficiently and handle the data effectively. In this article, we will explore the various techniques and functions available in C for reading files, along with their usage and best practices.
Understanding File I/O in C:
Before delving into reading files in C, it is essential to have a basic understanding of file input/output (I/O) in the C programming language. In C, file I/O operations are handled using pointers to the FILE structure, which holds information about the opened file, such as its name, file position indicator, and status flag.
To perform any file I/O operation, we need to include the stdio.h header file, which contains definitions for various I/O related functions and FILE structure.
Reading a Text File Line by Line:
One of the common requirements while reading a file is to read it line by line. This can be achieved by using the fgets() function, which reads a line of text from the specified file and stores it into the provided buffer.
Here’s an example of reading a text file line by line using fgets():
“`c
#include
int main() {
FILE* file = fopen(“file.txt”, “r”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
char buffer[256];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf(“%s”, buffer);
}
fclose(file);
return 0;
}
“`
In the above example, we open the file using the fopen() function in read mode (“r”). We handle the case where the file fails to open by checking if the file pointer is NULL. Then, we define a buffer with a sufficient size to store each line’s content. Finally, we loop through the file using fgets() until the end of file is reached, printing each line to the console.
Reading a Binary File:
Unlike reading a text file line by line, reading binary files requires different techniques. Binary files contain non-textual data, such as images, audio, or video files. To read such files, we use the fread() function, which reads a specified number of bytes from the file into a buffer.
Here’s an example of reading a binary file in C:
“`c
#include
int main() {
FILE* file = fopen(“image.jpg”, “rb”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
fseek(file, 0, SEEK_END);
long fileSize = ftell(file);
rewind(file);
char* buffer = (char*)malloc(fileSize);
fread(buffer, sizeof(char), fileSize, file);
// Process the binary data
fclose(file);
return 0;
}
“`
In this example, we open the binary file in read and binary mode (“rb”). We determine the file’s size by positioning the file pointer to the end of the file using fseek(). The ftell() function helps us retrieve the file’s size, which is then used to allocate memory for reading binary data. We read the binary data into the buffer using fread(). Finally, we can process the binary data as required.
Frequently Asked Questions:
Q1. How do I check if a file exists before reading it in C?
Ans: To check if a file exists before reading it in C, you can use the access() function provided by the C library. The access() function returns 0 if the file exists and can be accessed, or -1 if the file does not exist or access is denied. You can use it as follows:
“`c
if (access(“file.txt”, F_OK) != -1) {
// File exists, perform read operations
} else {
printf(“File does not exist!”);
}
“`
Q2. What is the difference between text and binary file reading in C?
Ans: Text file reading in C involves treating the file as a sequence of lines, while binary file reading deals with reading the file byte by byte or in chunks. Text files are human-readable and can be opened in any text editor, while binary files may contain non-textual data and require specific processing.
Q3. How do I read specific data types from a binary file in C?
Ans: To read specific data types from a binary file in C, you can use the fread() function by specifying the data type’s size and count. For example, to read an integer from a binary file, you can use:
“`c
int value;
fread(&value, sizeof(int), 1, file);
“`
Here, we read one integer from the file into the ‘value’ variable.
Conclusion:
Reading files is an essential part of many programming tasks, and C provides adequate functions and techniques to accomplish this efficiently. By understanding the concepts explained above and applying them based on your requirements, you will be able to read files effectively in C. Whether you are dealing with text files or binary files, C offers functions like fgets() and fread() that make the process straightforward and flexible.
Remember to handle error conditions appropriately, ensure file existence before reading, and free any dynamically allocated memory to prevent memory leaks. With these practices in mind, you can confidently tackle file reading tasks using C, optimizing your programs’ functionality and performance.
Read Text File In C
Reading a text file in the C programming language is a common task for many developers. It allows us to access the content of a file and process it within our program. In this article, we will explore the various methods to read a text file in C, including different modes, file pointers, and error handling. We will also provide practical examples and address some frequently asked questions to help you understand the read text file concept thoroughly.
Reading a Text File:
The most popular method to read a text file in C is by using the `fopen()` function to open the file and the `fscanf()` function to read the file’s content. Here is a basic example of how to read a text file in C:
“`c
#include
int main() {
FILE *file = fopen(“example.txt”, “r”);
if (file == NULL) {
printf(“Could not open the file.”);
return 1;
}
char content[100];
while (fscanf(file, “%s”, content) != EOF) {
printf(“%s\n”, content);
}
fclose(file);
return 0;
}
“`
Explanation:
1. We start by including the `
2. Next, we declare a file pointer named `file` that will be used to access the text file.
3. We use the `fopen()` function to open the “example.txt” file in read mode (specified by “r”). If the file cannot be opened, we handle the error by printf-ing an error message and returning 1.
4. We declare a character array named `content` with a size of 100 to store the contents of the file.
5. Using a while loop, we repeatedly use the `fscanf()` function to read strings from the file until `EOF` (end of file) is reached. Each string is then printed using the `printf()` function.
6. Finally, we close the file using the `fclose()` function to release the resources associated with it.
Different File Modes:
The file mode parameter passed to the `fopen()` function determines how the file is accessed. The following modes are commonly used:
– `”r”`: Read mode – Opens the file for reading. The file must exist; otherwise, an error will occur.
– `”w”`: Write mode – Creates a new file for writing. If the file already exists, it is truncated to zero length.
– `”a”`: Append mode – Opens a file for writing in append mode. If the file doesn’t exist, it is created. Writing to the file always occurs at the end, regardless of the file pointer’s position.
– `”r+”`: Read and write mode – Opens a file for both reading and writing.
– `”w+”`: Read and write mode – Creates a new file for both reading and writing. If the file already exists, it is truncated to zero length.
– `”a+”`: Read and append mode – Opens a file for reading and appending. If the file doesn’t exist, it is created.
File Pointers and Seek Functions:
When reading a text file, we often need to move the file pointer to a specific position. The `fseek()` function allows us to set the file pointer’s position based on the current position or an absolute position in the file. Here’s an example of using `fseek()` to read specific lines from a file:
“`c
#include
int main() {
FILE *file = fopen(“example.txt”, “r”);
if (file == NULL) {
printf(“Could not open the file.”);
return 1;
}
char line[100];
int lineNumber = 1;
while (fgets(line, sizeof(line), file) != NULL) {
if (lineNumber >= 10 && lineNumber <= 20) {
printf("%s", line);
}
lineNumber++;
}
fclose(file);
return 0;
}
```
In this example, we use the `fgets()` function to read a line of text from the file. We check if the `lineNumber` variable falls within the desired range (10 to 20), and if so, we print the line.
Error Handling:
Error handling is crucial when dealing with file operations. It is essential to check if the file pointers are valid before using them. If a file cannot be opened or read, it is necessary to handle the error gracefully and inform the user. This can be achieved using conditional statements, as shown in the previous examples, to check if the file pointers are NULL.
FAQs:
Q: What happens if the file cannot be opened?
A: If the file cannot be opened, the `fopen()` function returns NULL, indicating an error. It is essential to handle this scenario and display an appropriate error message.
Q: How do I read a text file character by character?
A: Instead of using `fscanf()` or `fgets()`, you can use the `fgetc()` function to read characters from a text file one by one. It returns the next character from the file or `EOF` if the end of the file is reached.
Q: How can I read a binary file in C?
A: To read a binary file, you need to open it in binary mode ("rb") using the `fopen()` function. Then, you can use functions like `fread()` to read binary data from the file.
Q: Can I read a text file from a different directory?
A: Yes, you can read a text file from a different directory by providing the full or relative file path when opening the file using `fopen()`.
Conclusion:
In this article, we have covered the various aspects of reading a text file in C. We explored the `fopen()` function, different file modes, file pointers, seek functions, error handling, and provided examples for practical understanding. Reading a text file is a fundamental operation in C programming and knowing how to perform it correctly is crucial for any programmer.
Write File In C
Introduction:
File handling is an essential aspect of programming and is crucial for storing and retrieving data in various formats. In the C programming language, the ability to write files is essential to create data files or store program outputs. This article aims to provide a comprehensive guide on how to write files using C, covering everything from file opening to data writing, and including vital tips and practices. Additionally, a Frequently Asked Questions (FAQs) section is included at the end to address common queries developers may have.
Step 1: Opening a File
To begin writing a file in C, you must first open it. The `fopen()` function is used for this purpose and requires two arguments: the file path and the open mode. The open modes determine the file’s functionality, such as reading, writing, or both. Popular open modes include “r” (read-only), “w” (write-only), and “a” (append). Example usage is as follows:
“`c
FILE *filePointer;
filePointer = fopen(“myfile.txt”, “w”);
“`
Step 2: Writing Data to the File
Once the file is open in write mode, you can utilize several functions to write data. One of the most basic functions is `fputc()`, which writes a single character at a time. For writing strings, the `fputs()` function is used. Here’s an example showcasing both methods:
“`c
FILE *filePointer;
filePointer = fopen(“myfile.txt”, “w”);
fputc(‘H’, filePointer);
fputs(“ello, World!”, filePointer);
“`
Furthermore, for formatted output, the `fprintf()` function is utilized. It allows writing data in various formats, such as integers, floats, characters, and strings. Here’s an example demonstrating the usage of `fprintf()`:
“`c
FILE *filePointer;
filePointer = fopen(“myfile.txt”, “w”);
fprintf(filePointer, “My name is %s and I am %d years old.”, “John”, 25);
“`
Step 3: Closing the File
After writing data to a file, it is crucial to close it properly. The `fclose()` function is used to close a file pointer, indicating that writing operations have completed. Failing to close the file may lead to data loss or corruption. Here’s an example of how to close a file:
“`c
FILE *filePointer;
filePointer = fopen(“myfile.txt”, “w”);
// Write operations
fclose(filePointer);
“`
Best Practices:
– Always check if the file pointer returned by `fopen()` is not NULL. If it is, handle the error accordingly.
– When working with strings, ensure that the string ends with a null character (`’\0’`) to signify the end of the string.
– Avoid repeatedly opening and closing files for continuous write operations. Instead, open the file once and write data using multiple `fprintf()` calls.
– Double-check the open mode while opening files. Be mindful of whether you want to overwrite an existing file or append new data.
– Handle exceptions by using appropriate error-checking mechanisms when working with files. For instance, if file opening fails, display an error message and terminate the program gracefully.
FAQs:
Q1. How do I append data to an existing file using C?
Ans. To append data to an existing file, open it using the “a” mode instead of “w”. This way, new data will be added to the end of the file without overwriting the existing content.
Q2. How can I write data to a binary file?
Ans. To write data to a binary file, use the “wb” mode while opening the file. Additionally, instead of `fputc()` or `fputs()`, use `fwrite()` to write binary data directly to the file.
Q3. What happens if I forget to close a file after writing?
Ans. Failing to close a file after writing may lead to data loss, corrupted files, or resource leaks, as the operating system does not immediately release the file’s resources. To prevent such issues, always close the file using `fclose()`.
Q4. How can I check if writing to a file was successful?
Ans. To verify that the writing operation was successful, check the return value of the writing function (`fputc()`, `fputs()`, or `fprintf()`). If the return value matches the number of characters written, the operation was successful.
Conclusion:
Writing files in C is an essential skill for any programmer. By following the steps outlined in this article and adhering to best practices, you can efficiently write data to files, ensuring accurate and reliable file handling. Remember to open and close files appropriately, choose the correct open mode for your requirements, and handle errors diligently for a robust file writing implementation.
Images related to the topic write to text file in c#
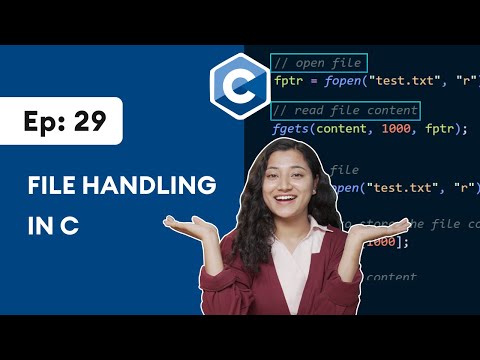
Article link: write to text file in c#.
Learn more about the topic write to text file in c#.
- C Write Text File – Learn C Programming from Scratch
- C Files I/O: Opening, Reading, Writing and Closing a file
- Write to .txt file? – Stack Overflow
- C – File I/O – Tutorialspoint
- C Programming – File Input/Output
- C – File Handling – Read and Write Integers – C Programming
- C Files I/O: Opening, Reading, Writing and Closing a file
- Reading & Writing to Text Files in C Programming – Study.com
- C Programming – File Input/Output
- Write Numerical or Text Data to File in C Programming …
- How to write to a text file in C# – C# Corner
- Read and write to text file in C – OpenGenus IQ
See more: https://nhanvietluanvan.com/luat-hoc