Typeerror: ‘Float’ Object Is Not Subscriptable
When working with Python, you may come across various error messages that can cause confusion and frustration. One such error message is “TypeError: ‘float’ object is not subscriptable.” This error occurs when you try to perform a subscript operation, such as accessing an element of a list or a character of a string, on a float object.
Understanding Subscriptable and Non-Subscriptable Objects
Before delving into the details of the error message, it is important to understand the concept of subscriptable and non-subscriptable objects in Python. Subscriptable refers to objects that can be accessed using square brackets [] to retrieve specific elements. Examples of subscriptable objects include lists, strings, tuples, and dictionaries. On the other hand, non-subscriptable objects are those that cannot be accessed using square brackets. These objects include integers, floats, and boolean values.
Overview of Python Float Data Type and its Limitations
In Python, the float is a built-in data type used to represent real numbers with decimal points. Floats are commonly used for mathematical calculations involving fractions or decimal values. However, unlike subscriptable objects, floats cannot be indexed or sliced because they are considered non-subscriptable.
Common Situations Leading to the ‘float’ object not being Subscriptable
There can be several scenarios where you might mistakenly attempt to subscript a float object. One common situation is when you have a list containing mixed data types, including floats and other subscriptable objects like strings or integers. If you try to access the elements of this list using square brackets, you will encounter the ‘float’ object is not subscriptable error.
Working with Subscriptable Objects in Python
When dealing with subscriptable objects, it is important to remember their characteristics. Lists, for example, are ordered collections of items that can be modified, indexed, and sliced. To access a specific element of a list, you can use square brackets along with the index of the desired item. Similarly, strings can be treated as arrays of characters, allowing you to access individual characters using index positions.
Explanation of the Error Occurring When Trying to Perform Subscript Operation on a Float
The ‘float’ object is not subscriptable error occurs because floats do not support subscripting. Since floats are non-subscriptable, trying to access their elements using square brackets leads to the error. This error message serves as a reminder that not all objects in Python can be subscripted and that you need to be cautious when handling different data types.
Possible Causes of Mistakenly Treating a Float as a Subscriptable Object
There can be various reasons why someone might mistakenly treat a float as a subscriptable object. One reason could be a typographical error or a misunderstanding of the data type being used. It is crucial to understand the characteristics of each data type and their limitations to avoid such mistakes.
Tips to Avoid or Debug the ‘float’ object not being Subscriptable Error
To prevent encountering the ‘float’ object is not subscriptable error, you should ensure that you are working with the correct data types. Double-check your code and confirm that the object you are trying to access using subscript notation is actually subscriptable, such as a list or a string. If you need to perform subscript operations on elements that involve floats, consider converting the float to a subscriptable object, such as a string or an integer.
Alternatives to Subscripting a Float Object in Python
If you find yourself needing to access specific parts or elements of a float, you can use alternative approaches instead of subscripting. One option is to convert the float to another data type, such as an integer, by using the int() function. This allows you to perform subscript operations on the integer representation. Additionally, you can convert the float to a string and access its characters using subscript notation.
Exploring the Implications of the Error and its Potential Impact on Code Functionality
The ‘float’ object is not subscriptable error could have various implications on your code’s functionality. If you mistakenly treat a float as a subscriptable object and try to access its elements using square brackets, your code will raise an error and fail to execute as expected. This error can also lead to unexpected behavior or produce incorrect results in your program.
FAQs
Q: What other related error messages are commonly encountered?
A: Some related error messages include “TypeError: ‘type’ object is not subscriptable,” “int’ object is not subscriptable,” “Object is not subscriptable python3,” “Convert float list to int Python,” “TypeError property object is not subscriptable,” and “List to float Pythontypeerror: ‘float’ object is not subscriptable.”
Q: How can I convert a float list to an integer list in Python?
A: To convert a float list to an integer list, you can iterate through each element of the float list, convert it to an integer using the int() function, and append the converted value to a new list.
Q: What should I do if I encounter the ‘float’ object is not subscriptable error?
A: You should carefully review your code and verify if you are trying to subscript a float object. If so, consider converting the float to a subscriptable data type, such as an integer or a string, to achieve the desired outcome.
Q: Are floats the only non-subscriptable objects in Python?
A: No, there are other non-subscriptable objects in Python, such as integers and boolean values. It is essential to understand the subscriptability of each data type to avoid encountering errors like this one.
In conclusion, the ‘float’ object is not subscriptable error occurs when trying to perform a subscript operation on a float object. Understanding the difference between subscriptable and non-subscriptable objects, as well as the limitations of the float data type, is crucial in avoiding this error. By being aware of common situations that lead to this error and following best practices, you can prevent unexpected issues in your Python code. Remember to always double-check the data types you are working with and consider alternative approaches when subscripting a float object.
Fix Typeerror Int Or Float Object Is Not Subscriptable – Python
Keywords searched by users: typeerror: ‘float’ object is not subscriptable TypeError: ‘type’ object is not subscriptable, Int’ object is not subscriptable, Object is not subscriptable python3, Convert float list to int Python, TypeError property object is not subscriptable, List to float Python
Categories: Top 19 Typeerror: ‘Float’ Object Is Not Subscriptable
See more here: nhanvietluanvan.com
Typeerror: ‘Type’ Object Is Not Subscriptable
Introduction:
If you are new to programming or even if you have some experience, you might have come across an error message like “TypeError: ‘type’ object is not subscriptable”. This is a common error encountered by Python developers, especially when working with collections like lists, tuples, or dictionaries. In this article, we will explore what this error means, its causes, and how to fix it.
Understanding the error:
Let’s begin by understanding the error message itself: “TypeError: ‘type’ object is not subscriptable”. In Python, a subscriptable object is one that can be accessed using square brackets [], such as lists, tuples, or dictionaries. This error occurs when we try to access an object that is not subscriptable, which means it cannot be indexed or sliced using square brackets.
Causes of the error:
1. Incorrect variable assignment:
One possible cause of this error is incorrectly assigning a variable to a type instead of an instance of that type. For example:
“`python
my_list = list # Incorrect
my_list = [1, 2, 3] # Correct
“`
In the first line of code, we are assigning the type ‘list’ to the variable ‘my_list’, which makes it a “type” object. Since “type” objects are not subscriptable, any attempt to access elements using square brackets will result in the TypeError.
2. Using parentheses instead of square brackets:
Another common mistake that leads to this error is using parentheses () instead of square brackets [] to access elements of a collection. For example:
“`python
my_list = [1, 2, 3]
print(my_list(0)) # Incorrect
print(my_list[0]) # Correct
“`
The first print statement uses parentheses to access the element at index 0 instead of square brackets. This will result in a TypeError since parentheses are used for function calls rather than subscripting.
3. Accessing non-subscriptable objects/methods:
Sometimes, this error occurs when we try to access non-subscriptable attributes or methods of an object. For instance:
“`python
my_string = “Hello, World!”
print(my_string[0]) # Correct
print(my_string.upper[0]) # Incorrect
“`
In the second print statement, we are trying to access the “upper” method of the string object using square brackets, which is invalid. Method invocations require parentheses, not square brackets.
How to fix the error:
Now that we have examined the causes of the ‘TypeError: ‘type’ object is not subscriptable’ error, let’s explore some strategies to fix it:
1. Check variable assignments:
Double-check that you have assigned an instance of a subscriptable object to your variable. Assigning a type instead of an instance will lead to this error. Make sure to use the correct syntax when assigning a list, tuple, or dictionary to a variable.
2. Use square brackets:
Ensure that you are using square brackets, not parentheses, when accessing elements of a collection. Square brackets are reserved for indexing and slicing operations in Python.
3. Verify object attributes and methods:
Make sure that you are not trying to access non-subscriptable attributes or methods. Some objects may have non-subscriptable methods or attributes that require different syntax. Refer to the documentation or online resources to understand the correct usage.
4. Debug step by step:
If you are unsure about the cause of the error, consider using print statements to debug your code step by step. Print the values and types of relevant variables to identify any inconsistencies or incorrect assignments.
5. Ask for help:
If you have tried all the above steps and are still struggling to fix the error, don’t hesitate to ask for help from fellow developers or online communities. Often, a fresh pair of eyes can spot the mistake easily.
Frequently Asked Questions (FAQs):
Q1. Why am I getting the ‘TypeError: ‘type’ object is not subscriptable’ error?
– This error occurs when you try to access a non-subscriptable object or incorrectly assign a variable to a type instead of an instance.
Q2. How can I solve the ‘TypeError: ‘type’ object is not subscriptable’ error?
– Double-check your variable assignments, use square brackets for indexing, and ensure you are not accessing non-subscriptable objects.
Q3. How can I debug this error?
– Use print statements to check the values and types of relevant variables. This will help identify any inconsistencies or incorrect assignments.
Q4. Are there any specific cases where this error might occur?
– Yes, this error is commonly encountered when working with collections like lists, tuples, or dictionaries. However, it can occur in other scenarios as well, such as accessing non-subscriptable attributes or methods of objects.
Q5. Can I access elements of a string using square brackets?
– Yes, strings in Python can be accessed using square brackets. Each character of a string can be accessed by its index, starting from 0.
Conclusion:
Understanding and fixing the ‘TypeError: ‘type’ object is not subscriptable’ error is crucial for any Python developer. By comprehending the causes discussed in this article and applying the suggested solutions, you can overcome this error and save valuable time when debugging your code. Remember to check variable assignments, use square brackets for indexing, and ensure you are not accessing non-subscriptable objects or methods. Keep practicing and learning, and soon this error will pose no challenge to you. Happy coding!
Int’ Object Is Not Subscriptable
Introduction:
Python, being a versatile and powerful programming language, offers a wide range of built-in data types to work with. Integers, denoted by ‘int’ in Python, are one of the fundamental data types used to represent whole numbers. However, encountering the error message “TypeError: ‘int’ object is not subscriptable” can be perplexing for inexperienced programmers. In this article, we will dive deep into understanding this error, explore its causes, and provide actionable steps to resolve it.
Understanding the Error:
The term ‘subscriptable’ refers to the capability of an object to be accessed using square brackets for indexing or slicing. In Python, several built-in data types, including lists, tuples, and strings, are subscriptable. However, integers, being immutable objects, lack this capability. Hence, the error message “TypeError: ‘int’ object is not subscriptable” is generated when an attempt is made to access or modify an integer using square brackets.
Causes of the Error:
1. Incorrect Syntax: The most common cause of this error is incorrect usage of square brackets with an integer value. For example, trying to access a specific element from an integer directly with square brackets will raise this error.
2. Nested Data Structures: Another cause of encountering this error is when an integer is mistakenly used as an index for a nested data structure, such as a list of lists or a dictionary. Trying to access an element within a nested structure using an integer index will result in the ‘int’ object not subscriptable error.
3. Misunderstanding Variable Type: Sometimes, this error can occur due to a misunderstanding of variable types. If a variable is initially assigned an integer value but later assumed to be a subscriptable data type, such as a list, this error may arise during runtime.
How to Fix the Error:
1. Verify Syntax: The first step in resolving this error is to carefully analyze the line of code where the error is being raised. Ensure that square brackets are not being used with an integer object. If they are, consider modifying the code logic to use the appropriate data type.
2. Check Nested Data Structures: If the error occurs within a nested data structure, examine the code to ensure that the index or key being used is not an integer. Make sure to use a valid index or key based on the type of data structure being accessed.
3. Review Variable Assignments: In case the error arises due to variable type misunderstanding, double-check the initial assignment of the variable. Ensure that it is compatible with the operation being performed. If necessary, redefine the variable with the appropriate data type.
FAQs:
Q1. Can integers be used as indices in Python?
A1. Yes, integers can be used as indices in certain subscriptable data types like lists and strings. However, integers themselves are not subscriptable.
Q2. How do I access specific digits within an integer in Python?
A2. Python provides various methods for manipulating integers. To access specific digits within an integer, convert it to a string using the str() function, and then use indexing or slicing techniques to extract the desired digits.
Q3. Why can’t I use an integer as a dictionary key?
A3. Dictionary keys must be hashable, and since integers are immutable objects, they fulfill this requirement. However, they cannot be directly used as subscriptable keys.
Q4. Is there any case where the ‘int’ object is subscriptable error can be ignored?
A4. No, this error should not be ignored. Ignoring this error may lead to unpredictable behavior or incorrect results in your program. It is best to identify and fix the root cause of the error.
Q5. Are there any alternative data types that are subscriptable and can replace integers?
A5. Yes, there are several subscriptable data types available in Python, such as lists, tuples, and strings. These can be used to store and manipulate collections of data instead of relying solely on integers.
Conclusion:
Understanding the “TypeError: ‘int’ object is not subscriptable” error is crucial for Python programmers to write efficient and bug-free code. By grasping the reasons behind this error and following the provided solutions, you can overcome this obstacle and continue to enhance your coding skills. Remember to verify your syntax, check nested data structures, and review variable assignments to ensure compatibility and avoid further occurrence of this error.
Images related to the topic typeerror: ‘float’ object is not subscriptable
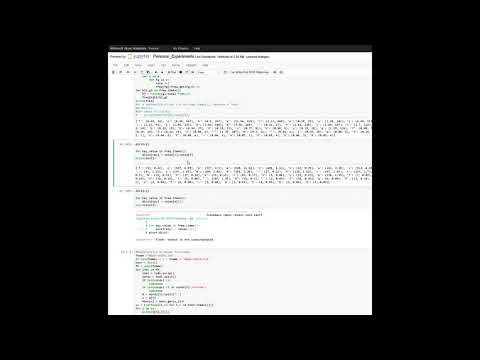
Found 14 images related to typeerror: ‘float’ object is not subscriptable theme
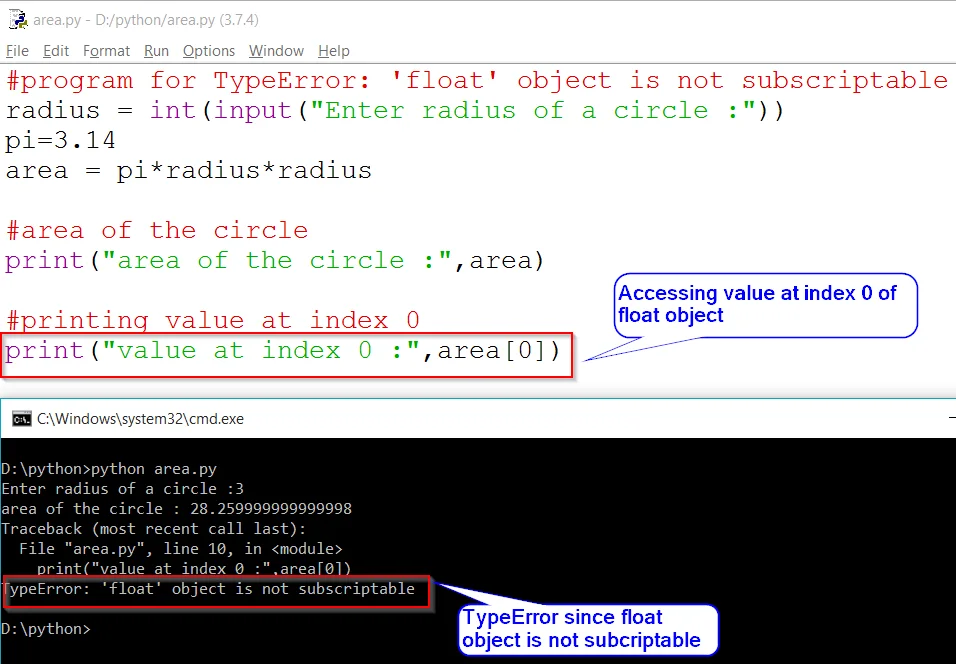
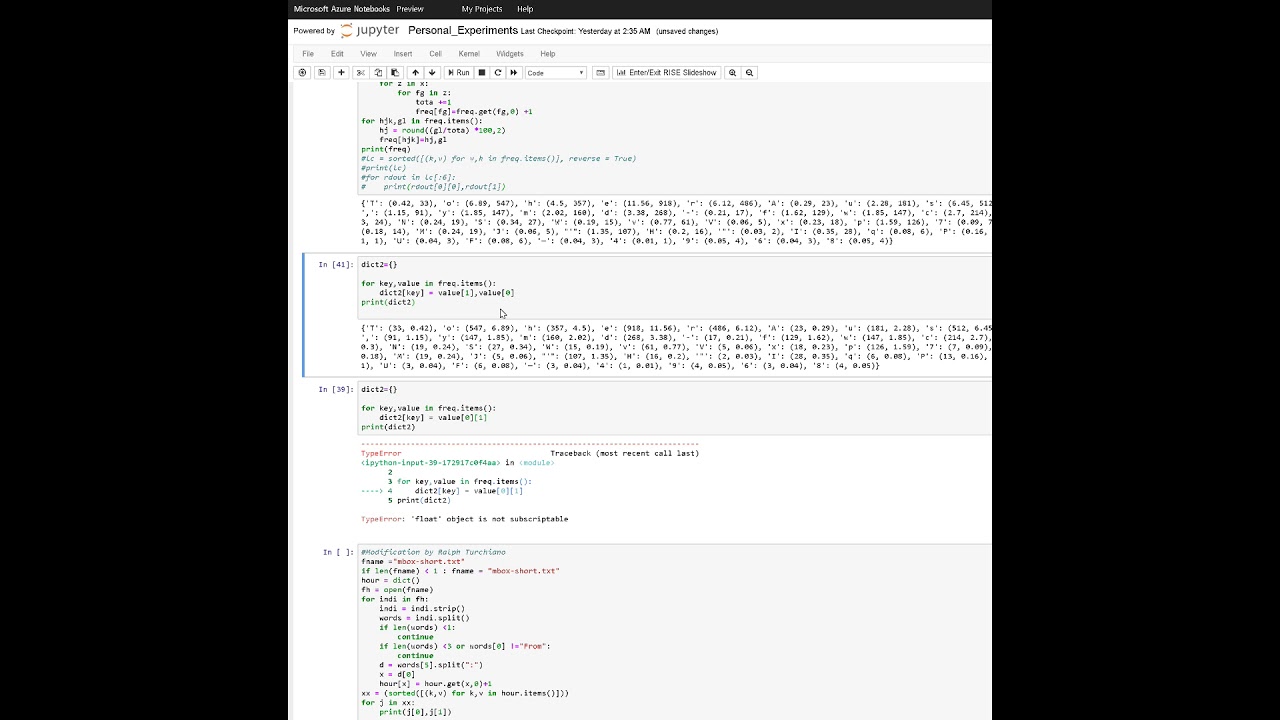
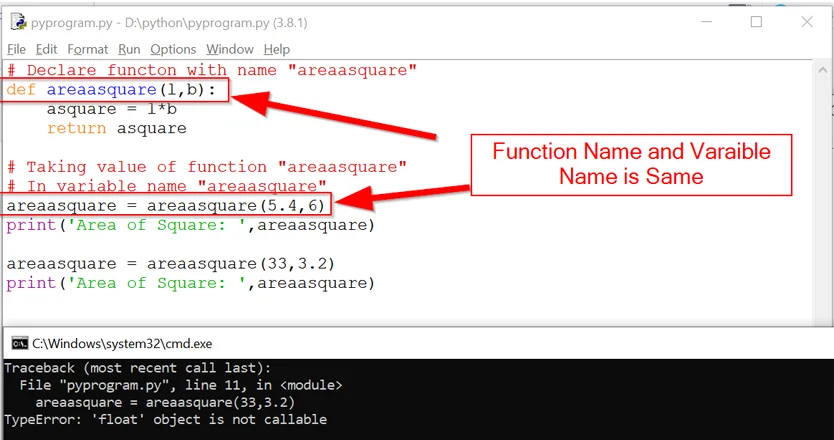
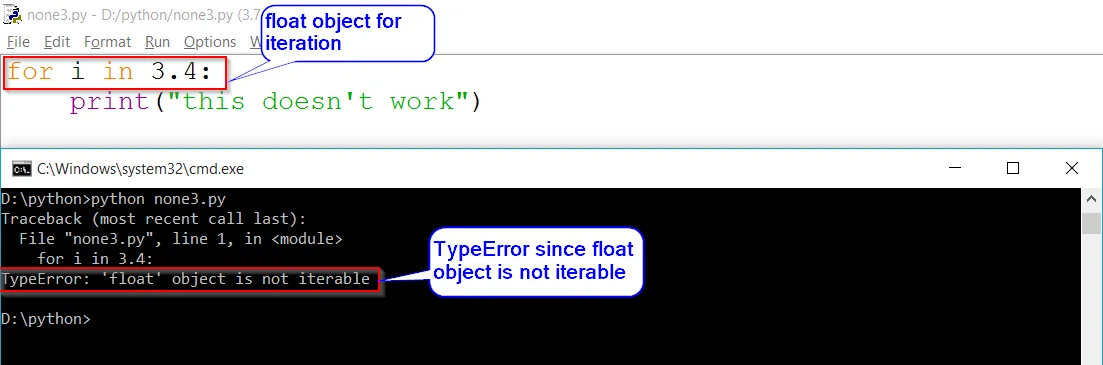
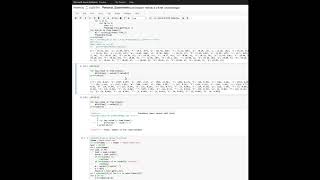
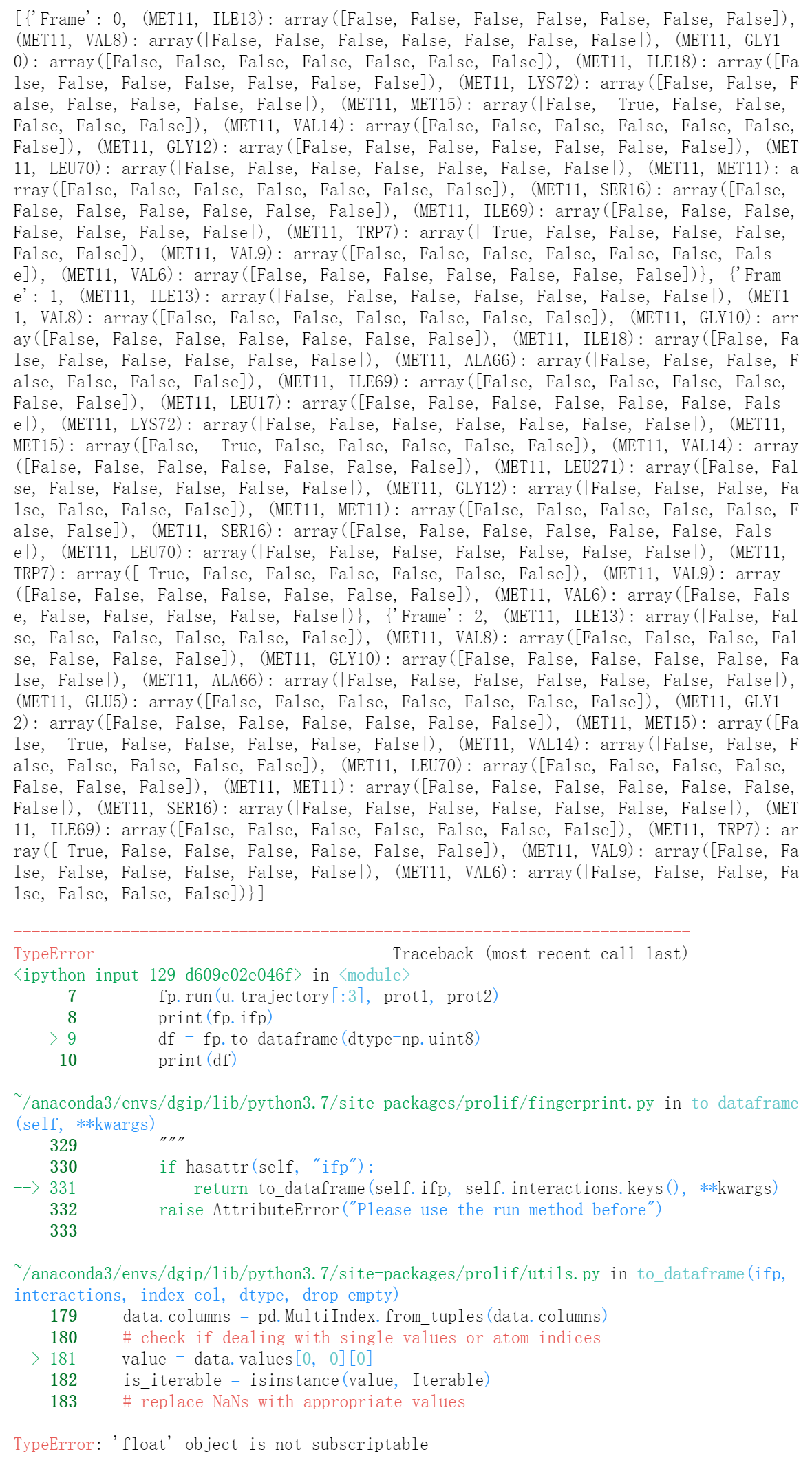
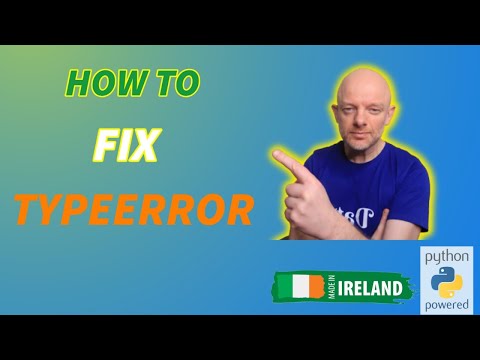
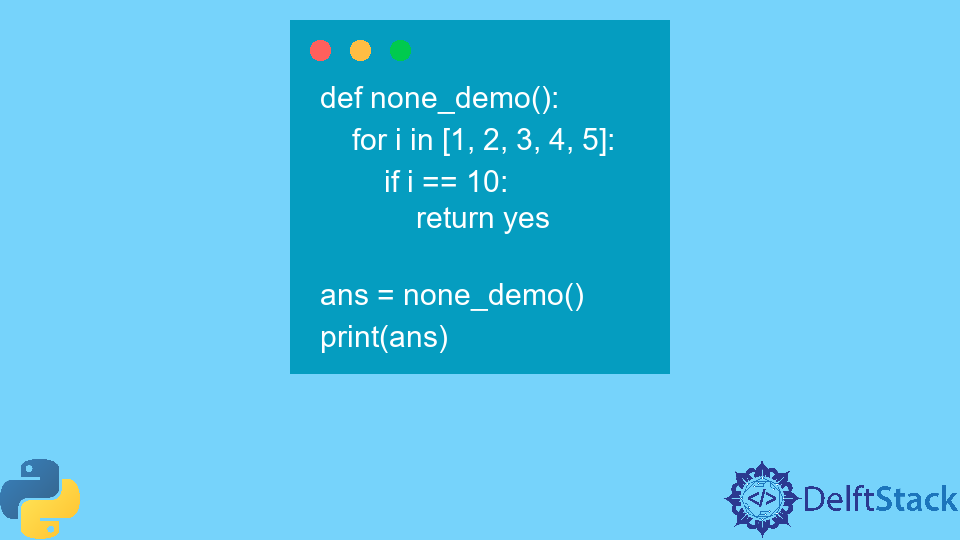


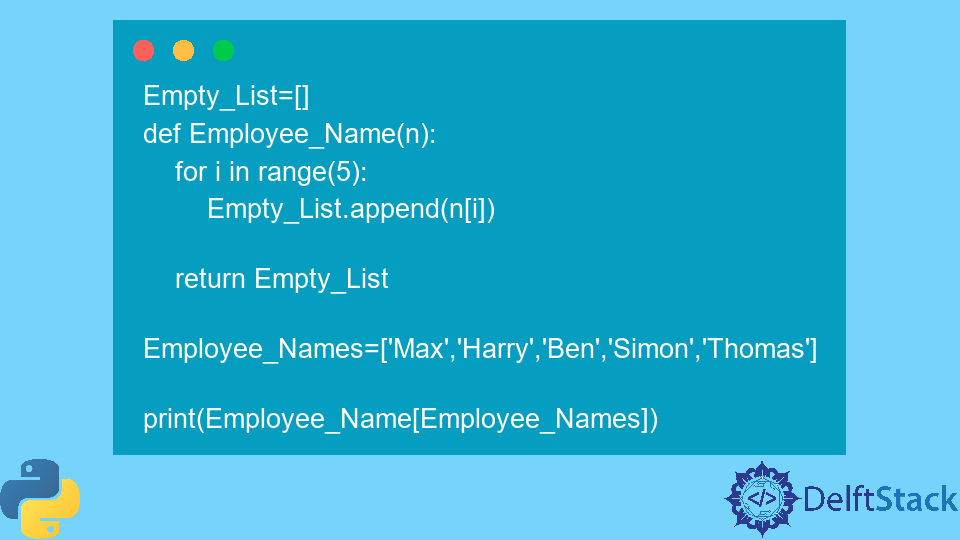
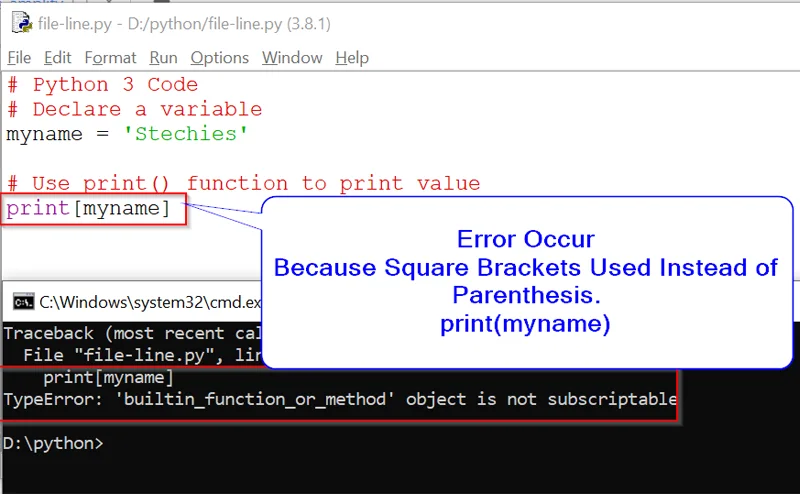
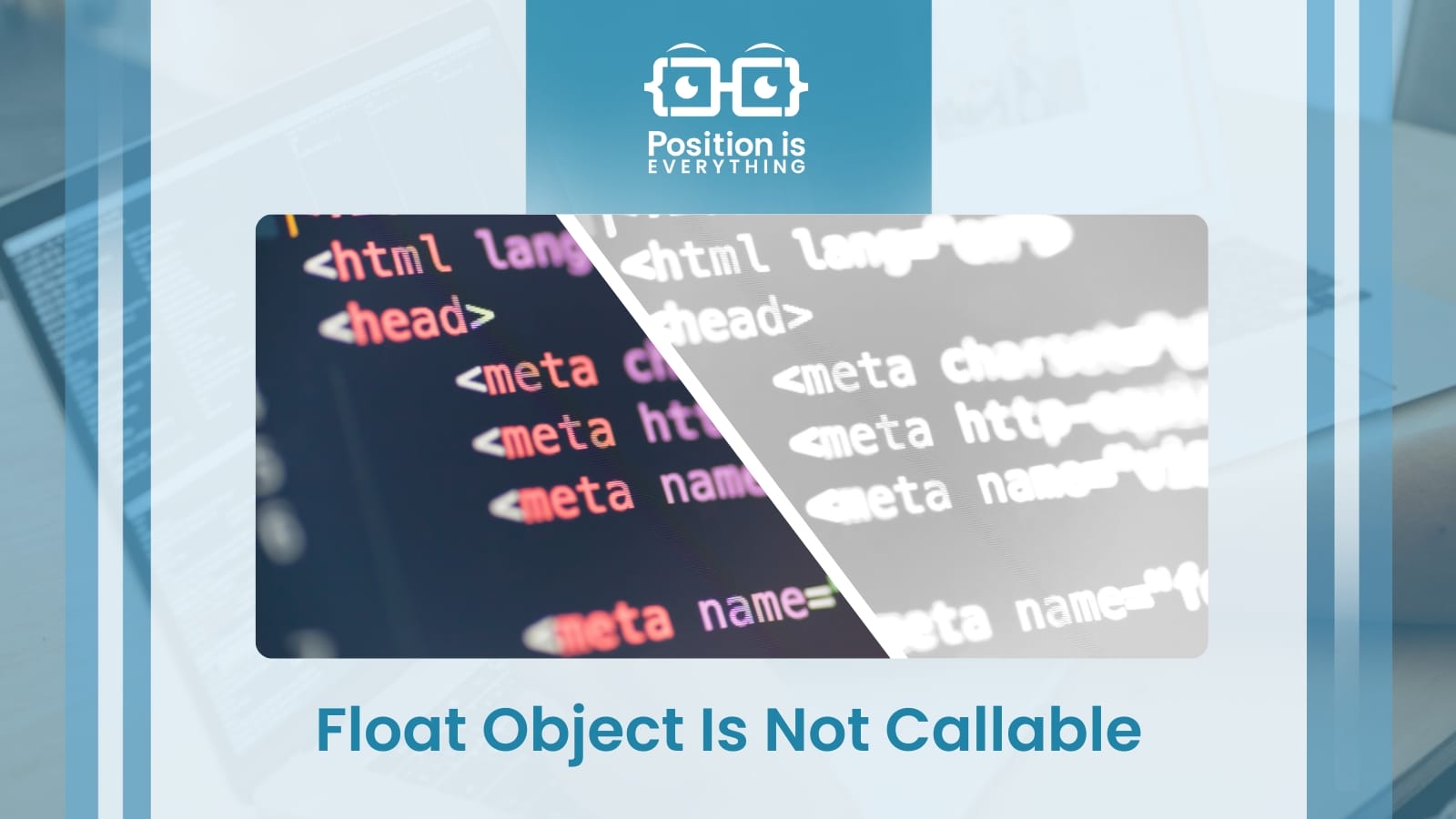

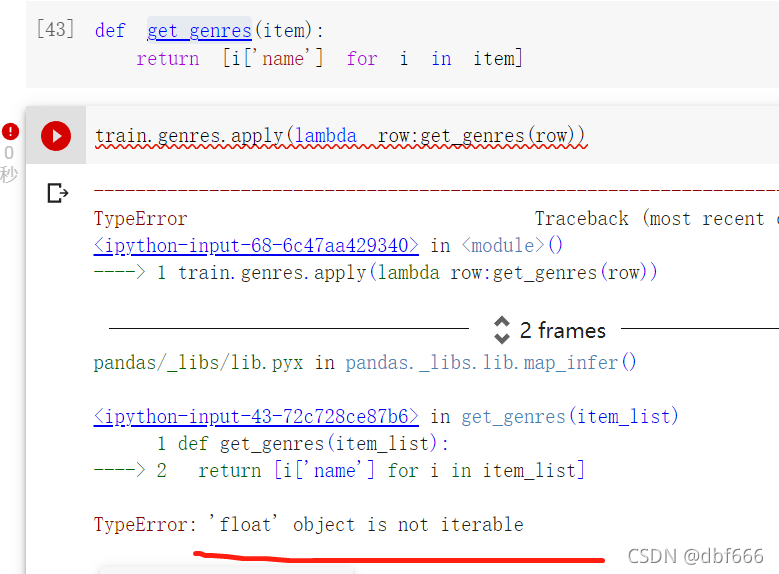
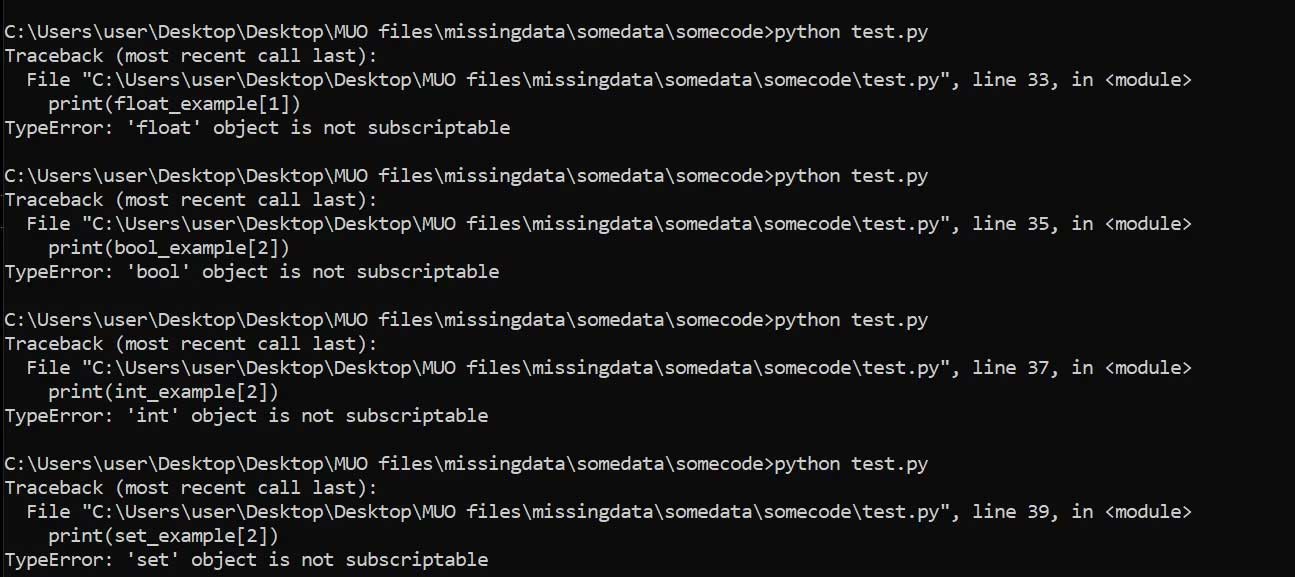

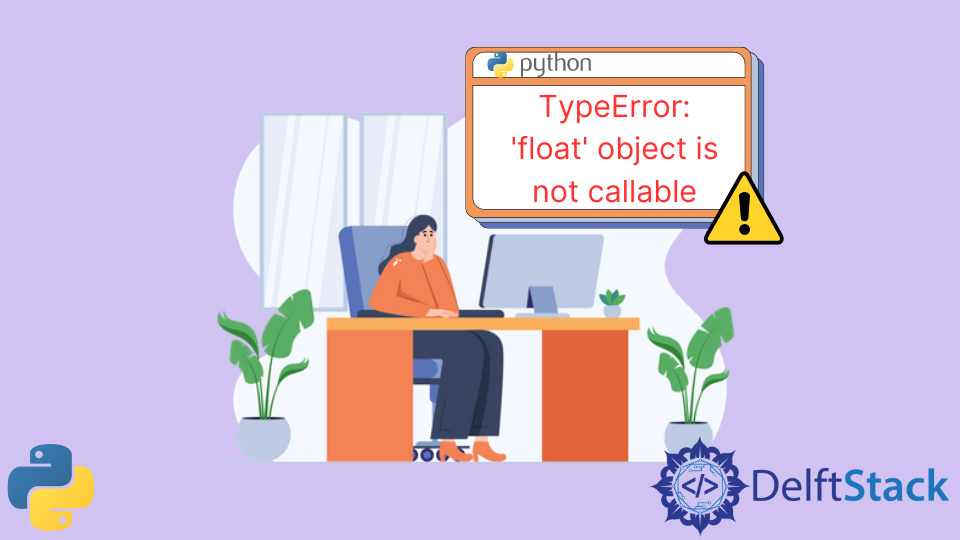
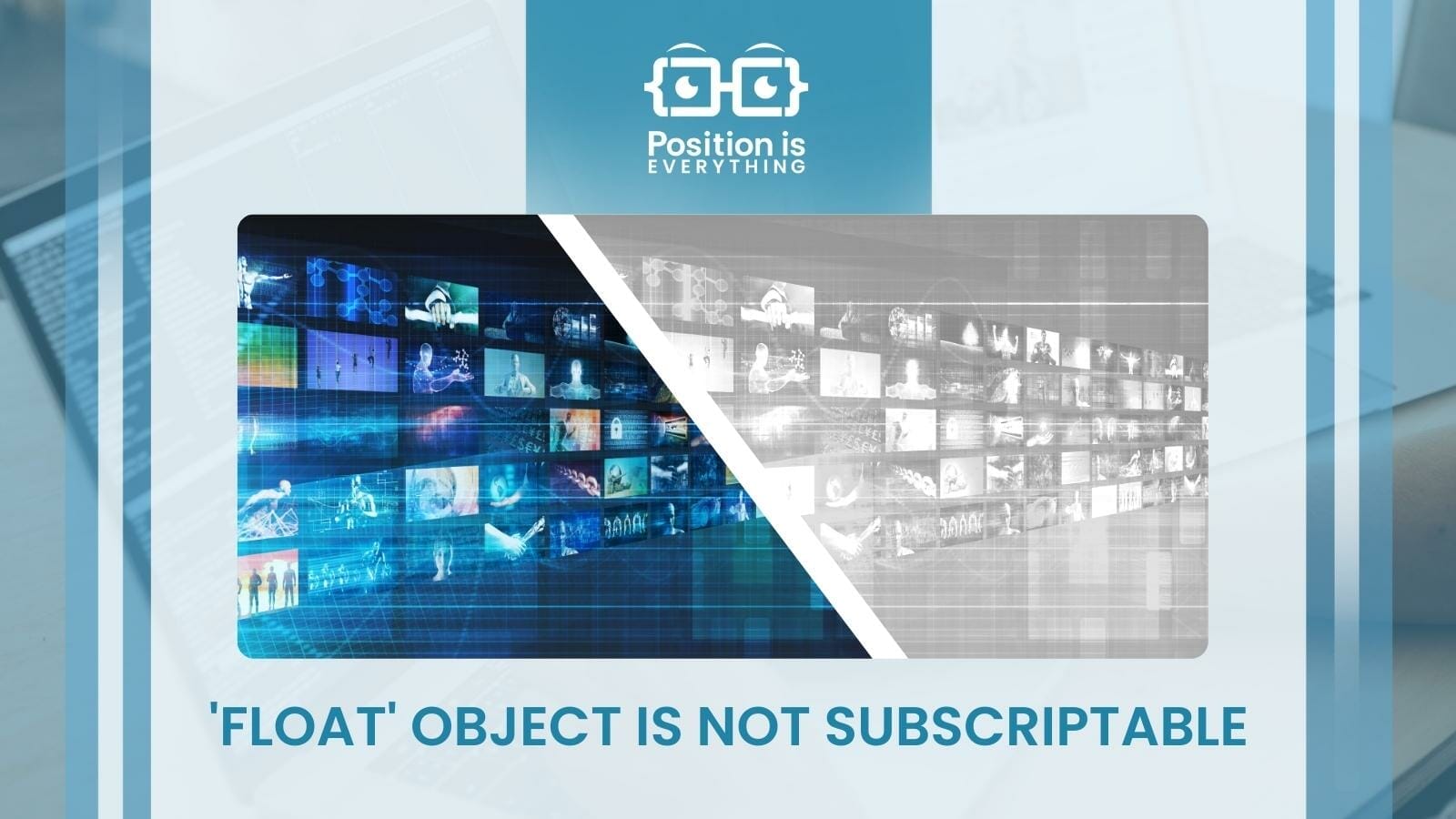


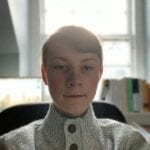


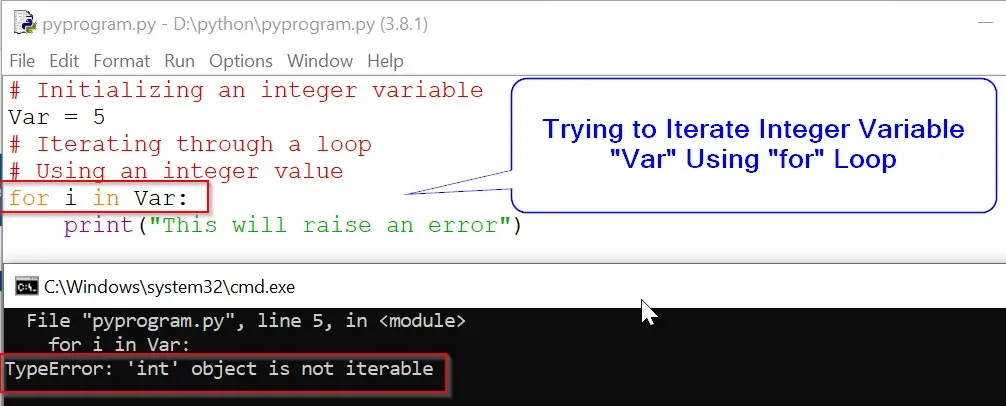

![Solved] TypeError: 'NoneType' Object is Not Subscriptable - Python Pool Solved] Typeerror: 'Nonetype' Object Is Not Subscriptable - Python Pool](https://www.pythonpool.com/wp-content/uploads/2022/04/TypeError-str-object-is-not-callable-1.webp)
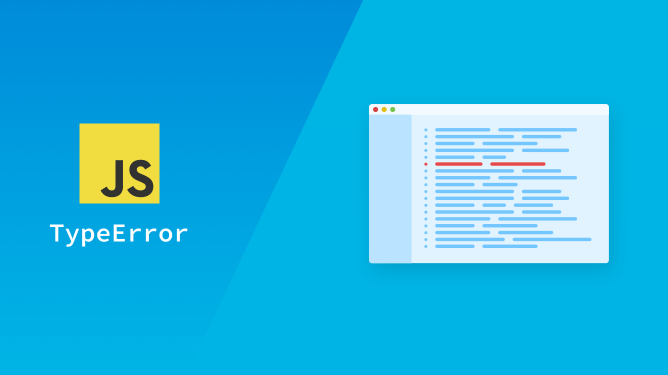

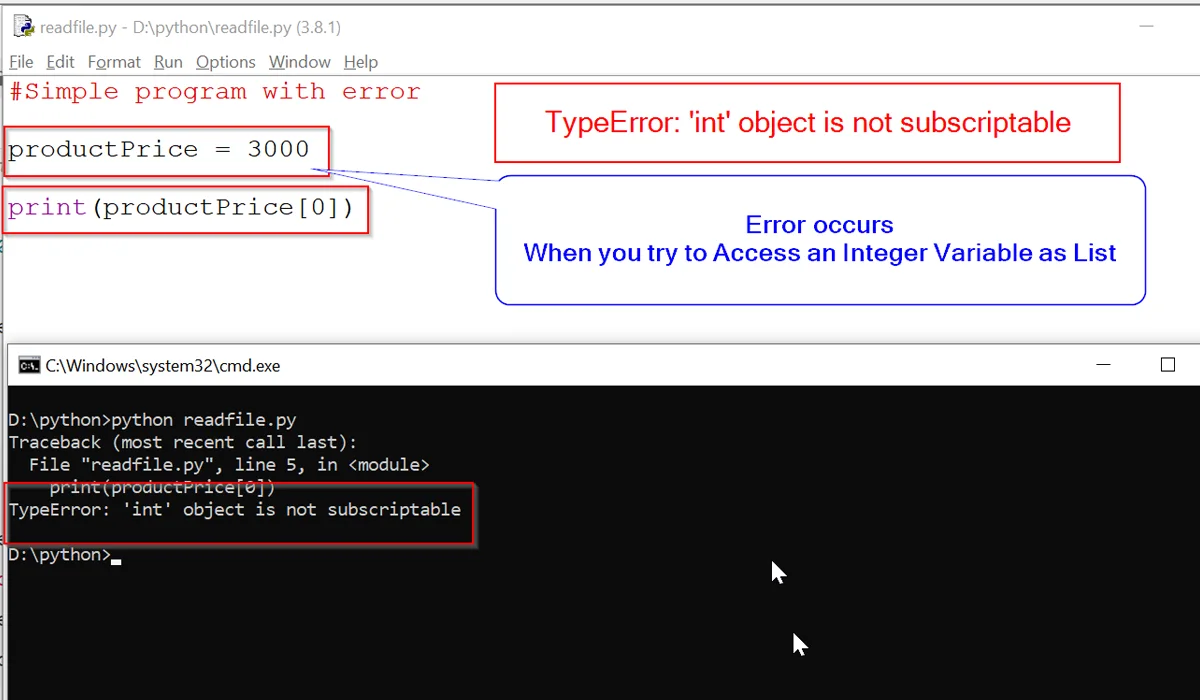

_fix-typeerror-int-or-float-object-is-not-subscriptable-python.jpg)
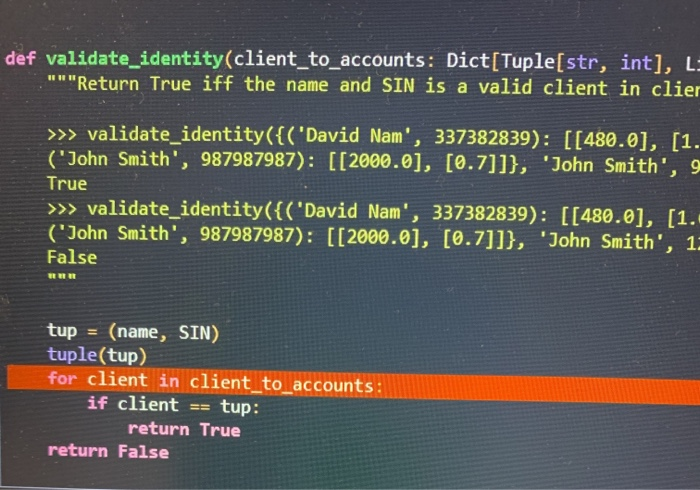
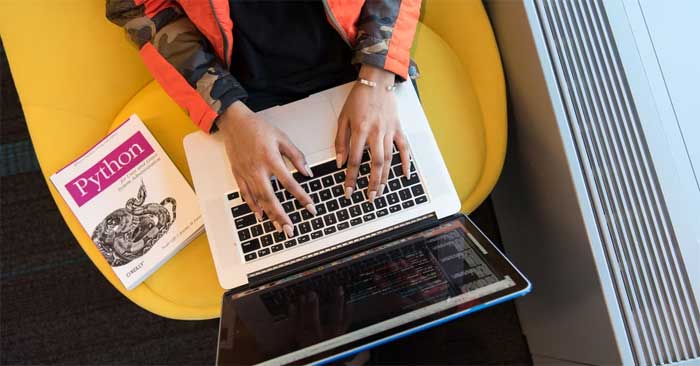
![Typeerror: 'float' object is not subscriptable [SOLVED] Typeerror: 'Float' Object Is Not Subscriptable [Solved]](https://itsourcecode.com/wp-content/uploads/2021/01/IT-SOURCECODE_ICON-07.jpg)

![Solved] TypeError: 'float' object is not subscriptable - Technolads Solved] Typeerror: 'Float' Object Is Not Subscriptable - Technolads](https://technolads.com/wp-content/uploads/2020/12/cropped-Site_Name-2.png)

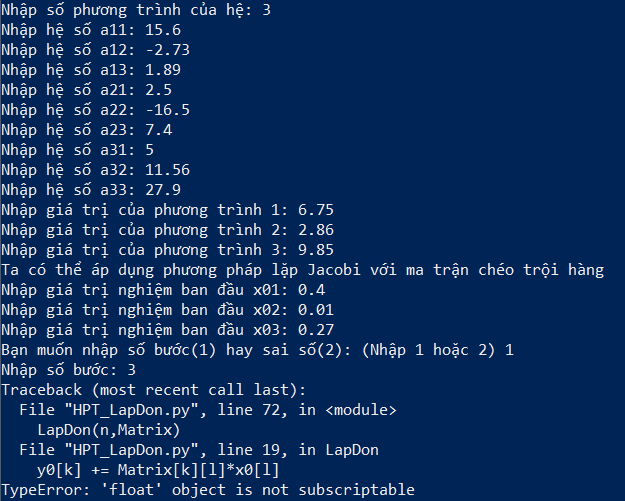
_typeerror-39set39-object-is-not-subscriptable.jpg)
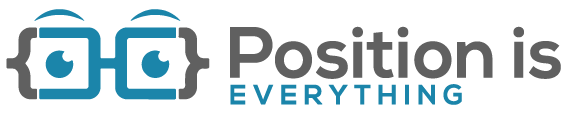
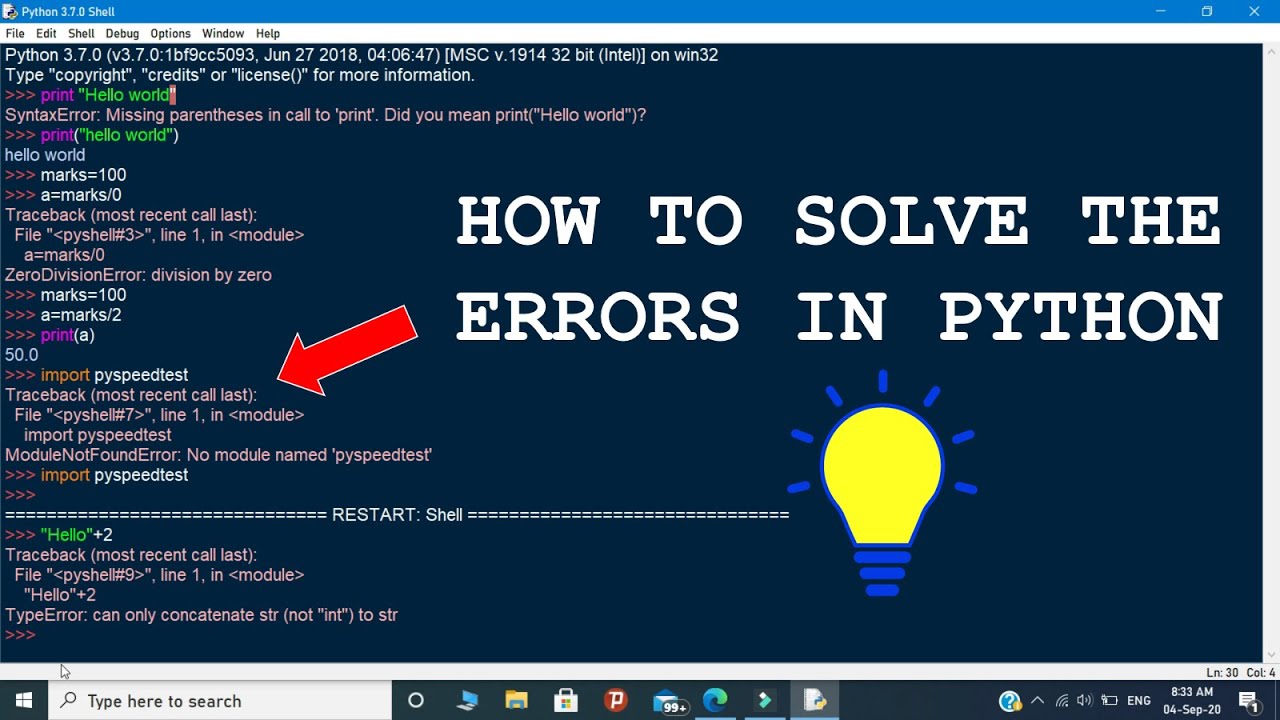
_typeerror-39type39-object-is-not-subscriptable-during-reading-data.jpg)
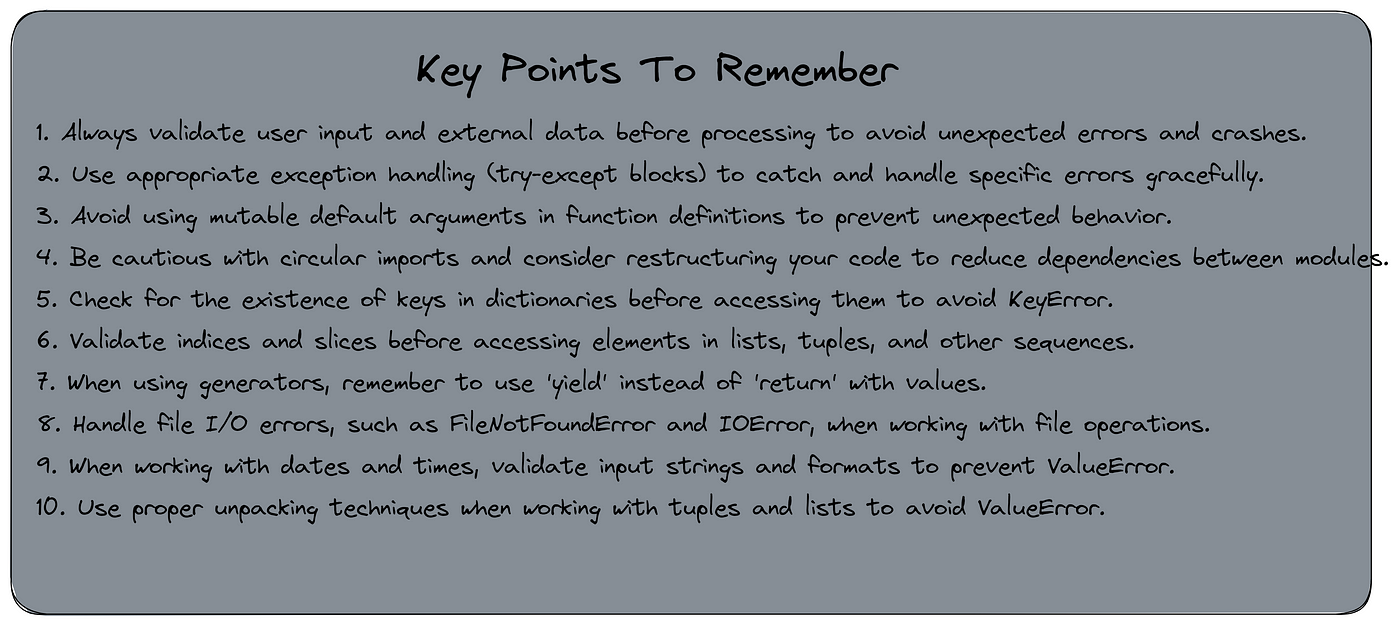
Article link: typeerror: ‘float’ object is not subscriptable.
Learn more about the topic typeerror: ‘float’ object is not subscriptable.
- TypeError: ‘float’ object is not subscriptable in Python
- TypeError: ‘float’ object is not subscriptable – Stack Overflow
- (Solved) Python TypeError: ‘float’ object is not subscriptable
- TypeError: ‘float’ object is not subscriptable – STechies
- Python typeerror: ‘float’ object is not subscriptable Solution
- Solve Python TypeError: ‘float’ object is not subscriptable
- Typeerror: ‘float’ object is not subscriptable [SOLVED]
- ‘float’ object is not subscriptable: How to fix it in Python
- [Solved] TypeError: ‘float’ object is not subscriptable
- ‘float’ object is not subscriptable python – AI Search Based Chat
See more: nhanvietluanvan.com/luat-hoc