Class Extends Value Undefined Is Not A Constructor Or Null
Class inheritance is a powerful feature in JavaScript that allows us to create new classes based on existing ones. It provides a way to define a class that inherits all the properties and methods of another class, known as the parent or superclass. This inheritance allows for code reuse and promotes a more organized and modular approach to programming.
Defining classes and their purpose in JavaScript
In JavaScript, a class is a blueprint for creating objects. It serves as a template that defines the structure and behavior of objects that will be created based on it. Classes encapsulate data (in the form of properties) and behavior (in the form of methods) into a single entity, making it easier to manage and manipulate.
The purpose of classes in JavaScript is to provide a way to create objects with shared properties and methods. They promote code reusability, maintainability, and readability. Classes also support the concept of inheritance, allowing for the creation of more specialized classes based on existing ones.
Understanding value undefined and its implications
In JavaScript, the value undefined is a primitive data type that represents an uninitialized variable or a variable that has been declared but has not been assigned a value. It is often used to indicate the absence of a value.
When a variable is declared without assigning an initial value to it, its default value is undefined. It can also be explicitly assigned to a variable by the programmer.
The implications of the value undefined are that operations or calculations involving undefined can lead to unexpected or undesirable results. It is important to handle undefined values properly in order to avoid errors and ensure the correct behavior of your code.
Introduction to constructors and their role in JavaScript
In JavaScript, a constructor is a special method that is used to create and initialize objects created from a class. It is called automatically when a new object is instantiated from a class using the “new” keyword.
Constructors are commonly used to set initial values for the properties of an object or perform any necessary setup tasks. They allow for the creation of multiple objects with similar properties and behavior defined by the class.
Common errors related to using undefined as a constructor
One common error related to using undefined as a constructor is the “Class extends value undefined is not a constructor or null” error. This error occurs when trying to extend a class that is undefined or null.
This error is often caused by a mistake in the code that leads to the class being incorrectly referenced or accessed. It can also occur when there are issues with module imports or circular dependencies.
To fix this error, it is important to ensure that the class being extended is properly defined and that all necessary dependencies are correctly imported.
Comparison between undefined and null in JavaScript
In JavaScript, both undefined and null represent the absence of value. However, they are not the same.
Undefined is a primitive value that is assigned to variables that have been declared but have not been assigned a value. It is also the default value for function parameters that have not been provided.
Null, on the other hand, is a special value that represents the deliberate absence of any object value. It is often used to indicate that a variable or object property intentionally does not have a value.
Differences between value undefined and null
The main difference between undefined and null is that undefined is a value assigned to variables that have not been assigned a value, while null is a value that can be assigned intentionally to indicate the absence of an object value.
Undefined is a primitive value, while null is an object value. This means that typeof null returns “object”, while typeof undefined returns “undefined”.
Common scenarios where value undefined is encountered
There are several common scenarios where the value undefined can be encountered in JavaScript:
1. When a variable is declared without assigning a value to it.
2. When a function parameter is not provided.
3. When accessing an object property or array element that does not exist.
4. When a function does not return a value explicitly.
It is important to handle these scenarios properly to avoid unexpected behavior or errors in your code.
Advanced techniques for handling undefined values in JavaScript
There are several advanced techniques for handling undefined values in JavaScript:
1. Using conditional statements (such as if statements or the ternary operator) to check for undefined before performing operations.
2. Using the logical OR operator (||) to provide default values in case of undefined variables or properties.
3. Using optional chaining (?.) to safely access nested properties or methods without throwing an error if an intermediate value is undefined.
4. Using the nullish coalescing operator (??) to provide default values only for null or undefined values, excluding other falsy values like empty strings or 0.
Best practices for avoiding the “is not a constructor” error
To avoid the “Class extends value undefined is not a constructor or null” error, it is important to follow these best practices:
1. Double-check that the class being extended is properly defined and accessible.
2. Ensure that all necessary dependencies are correctly imported.
3. Avoid circular dependencies between modules, as they can cause issues with class definitions.
4. Use a consistent and reliable module system, such as ES6 modules or a bundler like Webpack, to manage dependencies and ensure proper class resolution.
By following these best practices, you can minimize the chances of encountering this error and ensure the smooth execution of your JavaScript code.
**FAQs**
Q: What does the “Class extends value undefined is not a constructor or null” error mean?
A: This error occurs when trying to extend a class that is undefined or null. It typically indicates that there is an issue with accessing or referencing the class being extended.
Q: How can I fix the “Class extends value undefined is not a constructor or null” error?
A: To fix this error, check that the class being extended is properly defined and accessible. Ensure that all necessary dependencies are correctly imported and that there are no circular dependencies between modules.
Q: What is the difference between undefined and null in JavaScript?
A: Undefined is a value assigned to variables that have not been assigned a value, while null is a value that can be assigned intentionally to indicate the absence of an object value. Undefined is a primitive value, while null is an object value.
Q: How can I handle undefined values in JavaScript?
A: There are several techniques for handling undefined values, including using conditional statements, the logical OR operator for default values, optional chaining for safe property access, and the nullish coalescing operator for providing default values only for null or undefined values.
Q: What are constructors in JavaScript?
A: Constructors are special methods in JavaScript that are used to create and initialize objects created from a class. They are called automatically when a new object is instantiated using the “new” keyword and are used to set initial values or perform setup tasks.
Nodejs : How To Fix ‘Class Extends Value Undefined Is Not A Constructor Or Null’ Nodejs
Keywords searched by users: class extends value undefined is not a constructor or null Class extends value undefined is not a constructor or null, Class extends value undefined is not a constructor or null react, Class extends value undefined is not a constructor or null nextjs, Class extends value undefined is not a constructor or null npm, Class extends value undefined is not a constructor or null angular, Class extends value undefined is not a constructor or null nestjs, Stylelint typeerror class extends value undefined is not a constructor or null at object, Class extends value undefined is not a constructor or null stylelint
Categories: Top 49 Class Extends Value Undefined Is Not A Constructor Or Null
See more here: nhanvietluanvan.com
Class Extends Value Undefined Is Not A Constructor Or Null
Understanding the Error:
The error message “Class extends value undefined is not a constructor or null” is thrown when you try to extend a class that is not defined or has been assigned a value of undefined or null. This usually occurs when you try to instantiate an object that is not a valid class or is not accessible within the given scope.
Possible Causes:
1. Misspelled Class Name: One of the common causes of this error is misspelling the class name you are trying to extend. JavaScript is case-sensitive, so even a small typo in the class name can result in this error.
2. Reference Error: If the class you are trying to extend does not exist, the variable referencing it might be undefined. This can occur if you are trying to extend a class before it is declared or if you are trying to extend a class that is in another file that has not been properly imported or included.
3. Overwriting Class with a Different Value: Another possibility is that the original class could have been overwritten with another value, such as a function or null. In this case, when you try to extend the class, you will encounter this error message.
4. Webpack or Babel Configuration Issues: If you are using tools like Webpack or Babel, it is possible that there might be configuration issues causing this error. Incorrect setup or incorrect transformations can result in the class value being undefined or null when trying to extend it.
Resolving the Error:
1. Double-check Class Name: The first step to resolving this error is to verify the class name you are trying to extend. Ensure that it is spelled correctly and matches the actual class name.
2. Verify Scope and Dependencies: If you are extending a class from another file, ensure that the file with the class definition is being imported correctly and is accessible within the current scope. Similarly, make sure that any required dependencies are correctly imported and available.
3. Check Timing of Class Declaration: If you are extending a class that is declared in the same file, ensure that the class is defined before its extension. JavaScript runs code sequentially, so the class must be defined before it is extended.
4. Debugging with Console Logs: Utilize console.log statements to identify the point at which the class value becomes undefined or null. This can help you identify any potential overwritten values or track the flow of the code where the error occurs.
5. Verify Webpack or Babel Configuration: If you are using Webpack or Babel, check your configuration files. Ensure that the correct transformations are applied, and any plugins or loaders are configured properly.
FAQs:
Q1. Can a class be extended before it is declared?
A1. No, JavaScript requires that the class being extended is defined before it is extended. Ensure that the class declaration is above the point of extension.
Q2. Why am I seeing this error if I have properly imported the class?
A2. There might be an issue with the import statement or the file path. Double-check that the file is being imported correctly, and the class is being exported from that file.
Q3. Are there any tools or packages that can help resolve this error?
A3. Development tools like ESLint can help identify misspelled class names or any other potential errors in your code. Additionally, using a debugger can assist in tracking down the source of the error.
Q4. Why am I seeing this error when I haven’t made any changes to the code?
A4. It is possible that another part of the codebase is modifying or overwriting the class value, resulting in this error. Check for any recent changes or any included external scripts or libraries that might cause conflicts.
In conclusion, the “Class extends value undefined is not a constructor or null” error occurs when trying to extend a class that is not defined, has a value of undefined or null, or is not accessible within the current scope. By double-checking class names, verifying import statements, and understanding the timing of class declarations, developers can effectively debug and overcome this error. Remember to check your configuration files if you are using tools like Webpack or Babel, and utilize debugging techniques like console logging to identify the root cause of the error.
Class Extends Value Undefined Is Not A Constructor Or Null React
Reasons behind the error:
1. Incorrect import or missing dependency: One of the primary reasons for this error is an incorrect import or missing dependency. If the class being extended is not properly imported or if the required dependency is not installed, React will throw the “Class extends value undefined is not a constructor or null” error.
2. Circular dependencies: Circular dependencies can also trigger this error. When two or more components have circular references, React may fail to correctly resolve the order of component initialization, resulting in the “Class extends value undefined is not a constructor or null” error.
3. Typos or syntax errors: It is common for developers to mistype or make syntax errors during coding, such as misspelling the class name or using incorrect syntax while extending a class. These typos or syntax errors can lead to the error at hand.
Solutions to resolve the error:
1. Check imports and dependencies: Begin by checking the import statements of the class being extended to ensure they are correct and pointing to the intended file. Also, verify that all required dependencies are properly installed and updated. A missing or incorrect import can produce the mentioned error.
2. Review circular references: In situations where circular dependencies exist, it is crucial to review the code and modify it to remove the circular reference. Consider restructuring the code or separating functionalities to break the circular dependency chain. By eliminating the circular references, React will be able to correctly resolve the component initialization order, avoiding the error.
3. Verify class names and syntax: Pay attention to the spelling and correctness of the class names. Ensure that the class being extended has the correct name and that the syntax used for extending the class is proper. Be thorough in checking for any typos or syntax errors, as even a small mistake can result in the mentioned error.
FAQs:
Q1: What does “Class extends value undefined is not a constructor or null” mean?
A1: This error indicates that the class being extended in React is either undefined or null. It commonly occurs due to incorrect imports, missing dependencies, circular references, or typos/syntax errors.
Q2: How can I fix the “Class extends value undefined is not a constructor or null” error?
A2: To resolve this error, start by checking your imports and dependencies. Ensure that they are correctly imported and installed. If there are circular dependencies, review and modify the code to eliminate the circular references. Finally, double-check class names and syntax for any typos or errors.
Q3: Is there a specific IDE or tool that can help identify such errors?
A3: Yes, there are several popular IDEs and text editors that offer advanced linting and error-checking capabilities for React development. Tools like ESLint, TypeScript, and IDEs such as Visual Studio Code can detect and highlight many common errors, including the “Class extends value undefined is not a constructor or null” error.
Q4: I have verified my syntax and imports, but the error persists. What can I do?
A4: If you have checked all the potential causes and the error still persists, it is recommended to seek help from the React developer community. Post your specific issue on platforms like Stack Overflow, where experienced developers can provide guidance and identify potential problems in your code.
Q5: Can code refactoring help avoid this error altogether?
A5: Yes, proper organization and refactoring of the codebase can significantly reduce the chances of encountering the “Class extends value undefined is not a constructor or null” error. By following best practices, such as separating concerns, maintaining modularity, and avoiding circular dependencies, you can minimize the occurrence of such errors.
In conclusion, the “Class extends value undefined is not a constructor or null” error is a common hurdle faced by React developers. By understanding the potential causes and following the suggested solutions, developers can tackle this error effectively, saving precious time and ensuring the smooth functioning of their React applications. Remember to double-check imports, dependencies, class names, and syntax, while also addressing any circular dependencies in the code. With diligence and attention to detail, this error can be overcome, allowing developers to build robust and error-free React applications.
Images related to the topic class extends value undefined is not a constructor or null
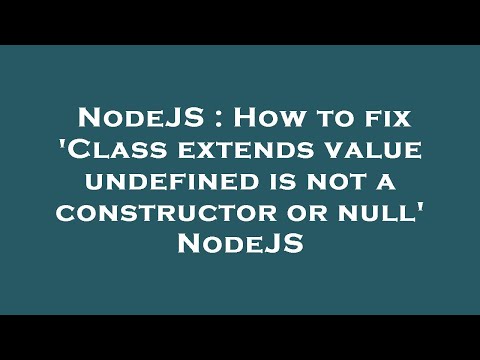
Found 33 images related to class extends value undefined is not a constructor or null theme

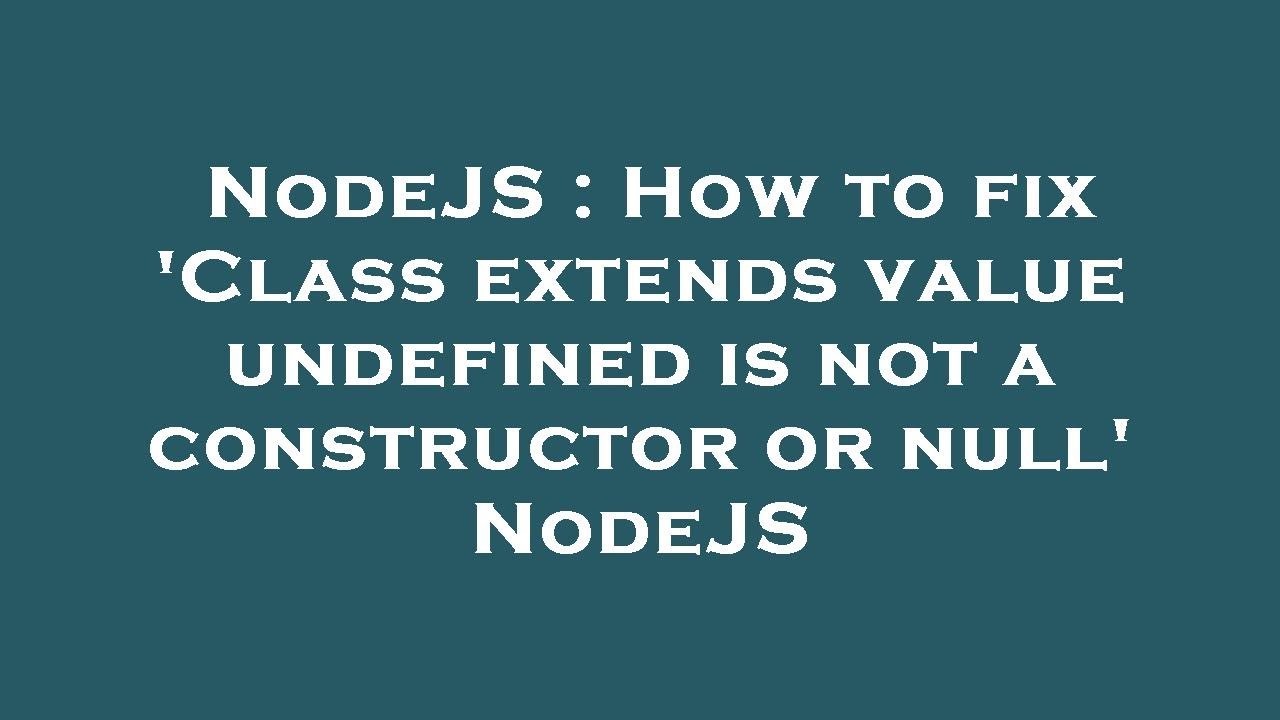
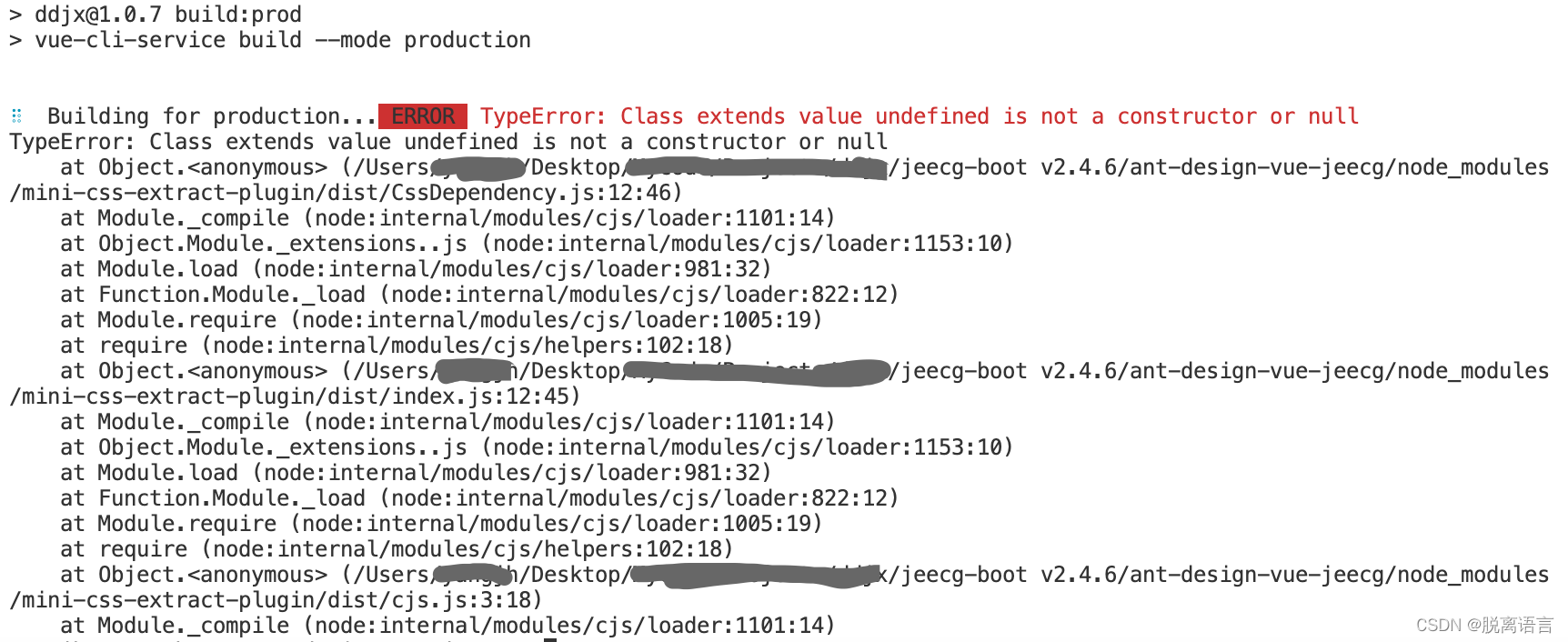





Article link: class extends value undefined is not a constructor or null.
Learn more about the topic class extends value undefined is not a constructor or null.
- Class extends value undefined is not a function or null – Stack …
- Class extends value undefined is not a constructor or null …
- class extends value undefined is not a constructor or null – AI …
- jss – Error : TypeError: Class extends value undefined is not a …
- TypeError: Class extends value undefined is not a constructor …
- CommandOperation “Class extends value undefined is not a …
- TypeError: Class extends value undefined is not a … – Drupal
- Class extends value undefined is not a constructor or null …
- Class extends value #
See more: nhanvietluanvan.com/luat-hoc