Can Only Be Default-Imported Using The Esmoduleinterop Flag
In JavaScript, when working with modules, there are times when we need to import a default export from a module. By default, the import statement in JavaScript is designed to import named exports, not default exports. However, there is a way to change this behavior and enable default imports using the esmoduleinterop flag.
Understanding the esmoduleinterop flag in JavaScript
The esmoduleinterop flag is a TypeScript compiler option that allows default imports to be used in JavaScript. When this flag is set to true, TypeScript will treat all imports as if they were using the import default syntax.
Enabling default imports with the esmoduleinterop flag
To enable default imports with the esmoduleinterop flag, you need to set the flag to true in your tsconfig.json file. Here is an example of how to configure the flag:
{
“compilerOptions”: {
“esModuleInterop”: true
}
}
Once the flag is set to true, you will be able to use the import default syntax to import default exports from modules.
Examples of default imports using the esmoduleinterop flag
Let’s consider an example where we have a module named “math.js” that exports a default function called “add”.
math.js file:
export default function add(a, b) {
return a + b;
}
To import the default export from the “math.js” module, we can use the following syntax:
import add from ‘./math.js’;
Now, we can use the “add” function in our code:
console.log(add(2, 3)); // Output: 5
Common issues when using the esmoduleinterop flag for default imports
When using the esmoduleinterop flag, there are some common issues that you may encounter. Here are a few examples and how to address them:
1. “React’ refers to a UMD global, but the current file is a module consider adding an import instead”:
This error occurs when trying to import a default export from a UMD-style module. To fix this issue, you can try adding an import statement instead of using the esmoduleinterop flag.
2. “Exports is not defined in ES module scope”:
This error occurs when trying to use exports in a file that is being treated as a module. To resolve this issue, you can remove any references to exports and make sure to use the export default syntax for exporting.
3. “Cannot use import statement outside a module”:
This error occurs when trying to use the import statement in a file that is not being treated as a module. To fix this issue, you need to make sure that the file has the module type, either by using the .mjs extension or by setting the type to “module” in your package.json file.
Advantages and disadvantages of using the esmoduleinterop flag for default imports
There are several advantages of using the esmoduleinterop flag for default imports:
1. Simplified syntax: The esmoduleinterop flag allows you to use the import default syntax, which can make your code more concise and easier to read.
2. Consistency: By enabling default imports, you can use the same syntax for both named and default exports, leading to more consistent code.
3. Compatibility: The esmoduleinterop flag can help resolve compatibility issues when working with modules that have default exports.
However, there are also some disadvantages to consider when using the esmoduleinterop flag:
1. Potential conflicts: Enabling default imports with the esmoduleinterop flag may lead to conflicts with other modules that use different import syntax.
2. Learning curve: Developers who are not familiar with the import default syntax may need to learn and adjust to the new syntax.
Best practices for utilizing the esmoduleinterop flag for default imports
To make the most out of the esmoduleinterop flag, here are some best practices to follow:
1. Use default exports sparingly: While default imports can be convenient, it is generally recommended to use named exports whenever possible, as they provide more clarity and maintainability.
2. Document your code: If you decide to use default imports with the esmoduleinterop flag, make sure to document the import syntax and any potential conflicts to assist other developers who may work on the codebase.
3. Test thoroughly: Default imports and the esmoduleinterop flag may introduce new potential issues. Therefore, it’s important to thoroughly test your code to ensure it behaves as expected.
FAQs
Q: Can only be default imported using the esmoduleinterop flag react?
A: No, the esmoduleinterop flag is not specific to React. It is a TypeScript compiler option that can be used for default imports in JavaScript in general.
Q: What is allowSyntheticDefaultImports?
A: allowSyntheticDefaultImports is another TypeScript compiler option that allows default imports to be used even if the module does not have a default export.
Q: What is Jest esmoduleinterop?
A: Jest esmoduleinterop is a configuration option in Jest, a popular JavaScript testing framework. When set to true, it enables default imports using the esmoduleinterop flag.
Q: What is Ts1259 error?
A: Ts1259 is a TypeScript error that occurs when trying to import a module using the import default syntax without enabling the esmoduleinterop flag.
Q: How to configure the esmoduleinterop flag in tsconfig.json?
A: To configure the esmoduleinterop flag, set “esModuleInterop” to true in the “compilerOptions” section of your tsconfig.json file.
Q: Why am I getting the “Exports is not defined in ES module scope” error?
A: This error occurs when trying to use the exports object in a file that is being treated as a module. To resolve this issue, use the export default syntax for exporting and remove any references to the exports object.
Q: How to fix the “Cannot use import statement outside a module” error?
A: This error occurs when trying to use the import statement in a file that is not being treated as a module. To fix this issue, make sure that the file has the module type either by using the .mjs extension or by setting the type to “module” in your package.json file.
In conclusion, the esmoduleinterop flag in JavaScript allows default imports to be used. It can simplify the import syntax and provide consistency when working with modules. However, it is important to be aware of potential conflicts and thoroughly test your code when enabling default imports with the esmoduleinterop flag.
Error Ts1259: Module Can Only Be Default-Imported Using The ‘Esmoduleinterop’ Flag
Keywords searched by users: can only be default-imported using the esmoduleinterop flag Can only be default imported using the esmoduleinterop flag react, allowSyntheticDefaultImports, Jest esmoduleinterop, Ts1259, Tsconfig, React’ refers to a UMD global, but the current file is a module consider adding an import instead, Exports is not defined in ES module scope, Cannot use import statement outside a module typescript
Categories: Top 19 Can Only Be Default-Imported Using The Esmoduleinterop Flag
See more here: nhanvietluanvan.com
Can Only Be Default Imported Using The Esmoduleinterop Flag React
React is one of the most popular JavaScript libraries for building user interfaces, known for its simplicity and efficiency. It allows developers to create reusable UI components and manage the state of an application effectively. When using React, you may come across a situation where you need to import certain modules with specific flags. One such flag is the “esmoduleinterop” flag, which is required for default importing certain modules. In this article, we will discuss what the esmoduleinterop flag is, why it is necessary for default importing in React, and provide some FAQs to address common queries related to this topic.
Understanding the esmoduleinterop flag:
The esmoduleinterop flag is a TypeScript compiler option that enables the import of CommonJS modules with a default export. By default, when importing a CommonJS module, TypeScript expects the imported object to be accessed through the “default” property. However, some modules may not follow this convention and instead directly export the module without the “default” property. To handle this scenario, the esmoduleinterop flag must be enabled.
Default importing in React:
In React, default importing is commonly used to import and utilize third-party libraries or packages. Many popular libraries, such as React Router or React Redux, contain default exports that can be imported using the esmoduleinterop flag. Importing these libraries using the default import syntax simplifies the code and improves readability. Without enabling the esmoduleinterop flag, you may encounter errors when attempting to import certain modules.
Why is the esmoduleinterop flag necessary?
The esmoduleinterop flag is necessary because it provides compatibility when importing CommonJS modules that do not follow the default export convention. React applications often rely on third-party libraries, and it’s common for these libraries to export modules using different patterns. By enabling the esmoduleinterop flag, React developers can import these modules seamlessly and avoid unnecessary errors.
The esmoduleinterop flag is particularly useful when integrating React with TypeScript. TypeScript makes use of type definitions to enforce type safety and provide better developer experience. However, when importing a CommonJS module without the esmoduleinterop flag, TypeScript may throw errors related to incorrect types or missing properties. Enabling the flag helps TypeScript understand the module structure and prevents these errors.
FAQs:
Q: How can I enable the esmoduleinterop flag in my React project?
A: To enable the esmoduleinterop flag, you need to modify your TypeScript configuration file (tsconfig.json). Locate the “compilerOptions” object and add “esModuleInterop”: true. This configuration ensures that the flag is enabled during compilation.
Q: Are there any drawbacks to enabling the esmoduleinterop flag?
A: Enabling the esmoduleinterop flag should generally not introduce any drawbacks. However, it is worth noting that this flag modifies the way TypeScript handles module imports. If you have existing code that relies on the previous behavior, you may need to update your imports accordingly.
Q: Can the esmoduleinterop flag be enabled for all modules by default?
A: Yes, you can enable the esmoduleinterop flag for all modules by adding “esModuleInterop”: true to your TypeScript configuration file. However, it is generally recommended to enable the flag only when necessary, as it may affect the performance and cause potential compatibility issues.
Q: Can I manually modify the imported module to include a default property instead of enabling the esmoduleinterop flag?
A: In some cases, you may be able to modify the imported module to include a default property. However, this is not always feasible, especially when using third-party libraries that you cannot modify directly. Enabling the esmoduleinterop flag provides a more flexible and consistent solution.
Q: What are some alternative approaches to importing modules without the esmoduleinterop flag?
A: If you do not wish to enable the esmoduleinterop flag, you can still import modules using the full import syntax. For example, if a module does not follow the default export convention, you can import it as follows: `import * as module from ‘module’`. However, this approach may lead to longer, more complex code and reduce readability.
In conclusion, the esmoduleinterop flag is an essential TypeScript compiler option when working with React. Enabling this flag allows for default importing of CommonJS modules that do not follow the default export convention. By understanding and utilizing the esmoduleinterop flag, React developers can import third-party libraries seamlessly, improve code readability, and prevent potential errors related to incorrect type handling.
Allowsyntheticdefaultimports
The allowSyntheticDefaultImports option is a feature in JavaScript that allows for the use of default imports even if the module does not have a default export. It can be used to simplify the process of importing modules and make code more concise. In this article, we will delve into the details of allowSyntheticDefaultImports, explaining how it works, when to use it, and any potential drawbacks it may have.
How allowSyntheticDefaultImports works
In JavaScript, modules can have named exports and default exports. Named exports allow multiple values or functions to be exported from a module using a specific name. On the other hand, default exports can export a single value or function as the default export of a module.
By default, JavaScript does not support the use of default imports if a module does not have a default export. However, by enabling the allowSyntheticDefaultImports option, this restriction can be bypassed. This means you can import a module as if it has a default export, even if it does not.
To enable allowSyntheticDefaultImports, you need to modify your module bundler or TypeScript configuration. If you’re using a module bundler like Webpack, you can set the “allowSyntheticDefaultImports” option to true. In TypeScript, the “allowSyntheticDefaultImports” flag should be set to true in the tsconfig.json file.
Example Usage
To illustrate how allowSyntheticDefaultImports works, consider the following scenario: you have a module named “utils” that exports several named exports, but no default export.
“`javascript
// utils.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a – b;
“`
Without allowSyntheticDefaultImports, you would typically import the module and use its named exports like this:
“`javascript
import { add, subtract } from ‘./utils’;
console.log(add(2, 3)); // Output: 5
console.log(subtract(5, 2)); // Output: 3
“`
However, if you enable allowSyntheticDefaultImports, you can import the module using a default import syntax, even though there is no default export:
“`javascript
import utils from ‘./utils’;
console.log(utils.add(2, 3)); // Output: 5
console.log(utils.subtract(5, 2)); // Output: 3
“`
This can be particularly handy when working with third-party libraries that do not provide a default export but can still be used as if they do.
When to use allowSyntheticDefaultImports
allowSyntheticDefaultImports is best used in situations where you want to import modules using a default import syntax for simplicity and code readability. It can be especially useful when working with large projects that require multiple imports, as it allows you to condense your code by removing unnecessary curly braces.
Furthermore, allowSyntheticDefaultImports can be beneficial when trying to migrate older codebases to newer JavaScript versions or frameworks. It can help in smoothly transitioning modules that used to have default exports to named exports, without requiring significant code changes.
Drawbacks and considerations
While allowSyntheticDefaultImports offers convenience, it’s important to use it judiciously and understand its potential drawbacks. Here are a few considerations:
1. Compatibility: Not all module systems and bundlers support allowSyntheticDefaultImports. Ensure that your selected tools support this feature before relying on it.
2. Clarity and readability: Default imports can make code more readable and concise, but they can also lead to confusion if the module being imported doesn’t have a default export. Be cautious and double-check if the module you are importing does have a default export or if you’re using allowSyntheticDefaultImports.
3. Dependency analysis: Enabling allowSyntheticDefaultImports may affect how tools analyze and manage dependencies. Some linting tools or build optimizations might not work as expected, leading to potential issues.
FAQs
Q: What happens if I enable allowSyntheticDefaultImports and import a module that does have a default export?
A: If you enable allowSyntheticDefaultImports but import a module that already has a default export, the import will still work as expected. The allowSyntheticDefaultImports option doesn’t affect modules that have a default export; it simply bypasses the requirement for a default export when using default import syntax.
Q: Can I use both named and default exports in a module with allowSyntheticDefaultImports?
A: Yes, you can use both named and default exports in a module even with allowSyntheticDefaultImports. You’ll be able to import named exports using the traditional import syntax, and default exports using the default import syntax.
Q: Are there any performance implications when using allowSyntheticDefaultImports?
A: Enabling allowSyntheticDefaultImports should not have any significant performance implications on its own. However, as with any code optimization, it’s essential to monitor performance and conduct thorough testing to ensure it aligns with your project’s requirements.
In conclusion, allowSyntheticDefaultImports is a powerful feature in JavaScript that allows for default imports even if a module does not have a default export. It can simplify code by bypassing the need for named imports and can be particularly useful when working with third-party libraries or transitioning older code to newer JavaScript versions. However, it’s essential to use this feature responsibly and be aware of its potential limitations and impact on code clarity and tooling compatibility.
Jest Esmoduleinterop
When it comes to testing JavaScript code, Jest has become a highly popular choice among developers. Jest offers a comprehensive and easy-to-use testing framework, allowing developers to write and execute tests effortlessly. One of the features that make Jest so powerful is its ability to seamlessly work with ES Modules, thanks to the esmoduleinterop configuration option.
In this article, we will explore Jest esmoduleinterop in depth, uncovering its benefits, explaining its usage, and addressing common questions through a comprehensive FAQs section.
Understanding ES Modules
ES Modules, also known as ECMAScript Modules, are a standardized way to organize and share JavaScript code across different files. With the introduction of ES6, the JavaScript ecosystem embraced the concept of modules, making it easier to structure and manage large codebases.
By using import and export statements, developers can clearly define which parts of a module are accessible to other parts of the code. This modularity allows for better code organization, improves reusability, and minimizes naming conflicts.
However, integrating ES Modules into existing testing frameworks can sometimes be challenging. Fortunately, Jest comes to the rescue with its esmoduleinterop feature.
Enabling Jest esmoduleinterop
Jest provides an easy way to enable esmoduleinterop by configuring it through the package.json file or the jest.config.js file. By default, Jest uses CommonJS modules for running tests. However, for projects that heavily rely on ES Modules, enabling esmoduleinterop is crucial.
To enable Jest esmoduleinterop via the package.json file, add the following configuration:
“jest”: {
“esModuleInterop”: true
}
Alternatively, you can create a jest.config.js file and include the esmoduleinterop property:
module.exports = {
esModuleInterop: true,
};
Benefits of Jest esmoduleinterop
1. Seamless Integration: Enabling esmoduleinterop eliminates the need for additional setup or complex configurations. Jest automatically handles and executes tests, regardless of whether they are written as CommonJS or ES Modules.
2. Interoperability: With esmoduleinterop, developers can freely use modules written with different module systems, enabling the use of third-party libraries and frameworks without compatibility issues.
3. Cleaner Imports: ES Modules allow developers to import specific functions or objects from modules. Jest esmoduleinterop simplifies the process by ensuring that imported modules work correctly, saving time and promoting better code readability.
4. Forward Compatibility: As ES Modules gain wide adoption in the JavaScript ecosystem, utilizing Jest’s esmoduleinterop ensures forward compatibility of test suits. Migrating from CommonJS to ES Modules becomes much easier, allowing developers to adapt to future changes smoothly.
Frequently Asked Questions (FAQs)
Q1. Is it necessary to enable esmoduleinterop for every Jest project?
A: No, it is not necessary to enable esmoduleinterop for every project. If your project heavily relies on CommonJS modules and has minimal or no ES Module usage, it is unnecessary to enable esmoduleinterop.
Q2. Can I still use CommonJS modules with esmoduleinterop enabled?
A: Yes, Jest allows the use of CommonJS modules even with esmoduleinterop enabled. However, it is recommended to migrate to ES Modules for better compatibility and future-proofing.
Q3. How does Jest handle imports and exports with esmoduleinterop enabled?
A: Jest automatically converts ES Module syntax to CommonJS syntax under the hood when esmoduleinterop is enabled. This allows seamless execution of tests regardless of the module system used.
Q4. Are there any performance implications of using esmoduleinterop?
A: Enabling esmoduleinterop may introduce a slight performance overhead, but it is generally negligible. Jest’s internal mechanisms efficiently handle module conversion, ensuring minimal impact on test execution speed.
Q5. Can I disable esmoduleinterop after enabling it?
A: Yes, you can disable esmoduleinterop by simply removing or setting the property to false in the configuration. However, it is recommended to avoid frequent toggling as it might introduce compatibility issues while running tests.
Q6. Are there any limitations when using esmoduleinterop?
A: While Jest’s esmoduleinterop greatly simplifies testing with ES Modules, some limitations might arise when dealing with complex module systems, such as AMD (Asynchronous Module Definition). In such cases, additional configuration or manual handling might be required.
Conclusion
Jest’s esmoduleinterop feature simplifies testing in JavaScript projects that leverage ES Modules. Enabling esmoduleinterop ensures seamless integration with both CommonJS and ES Modules, promoting interoperability, cleaner imports, and forward compatibility. By understanding the benefits and usage of Jest esmoduleinterop, developers can enhance their testing workflow and save time in managing module systems.
Images related to the topic can only be default-imported using the esmoduleinterop flag
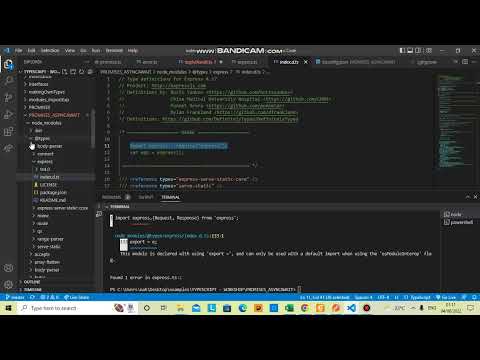
Found 30 images related to can only be default-imported using the esmoduleinterop flag theme

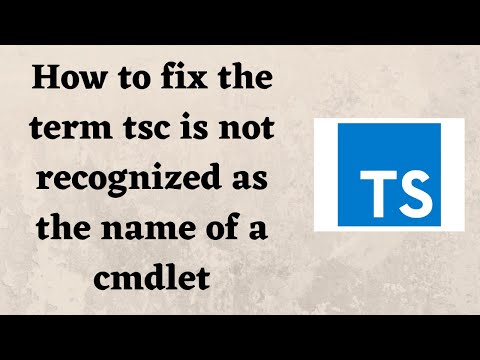



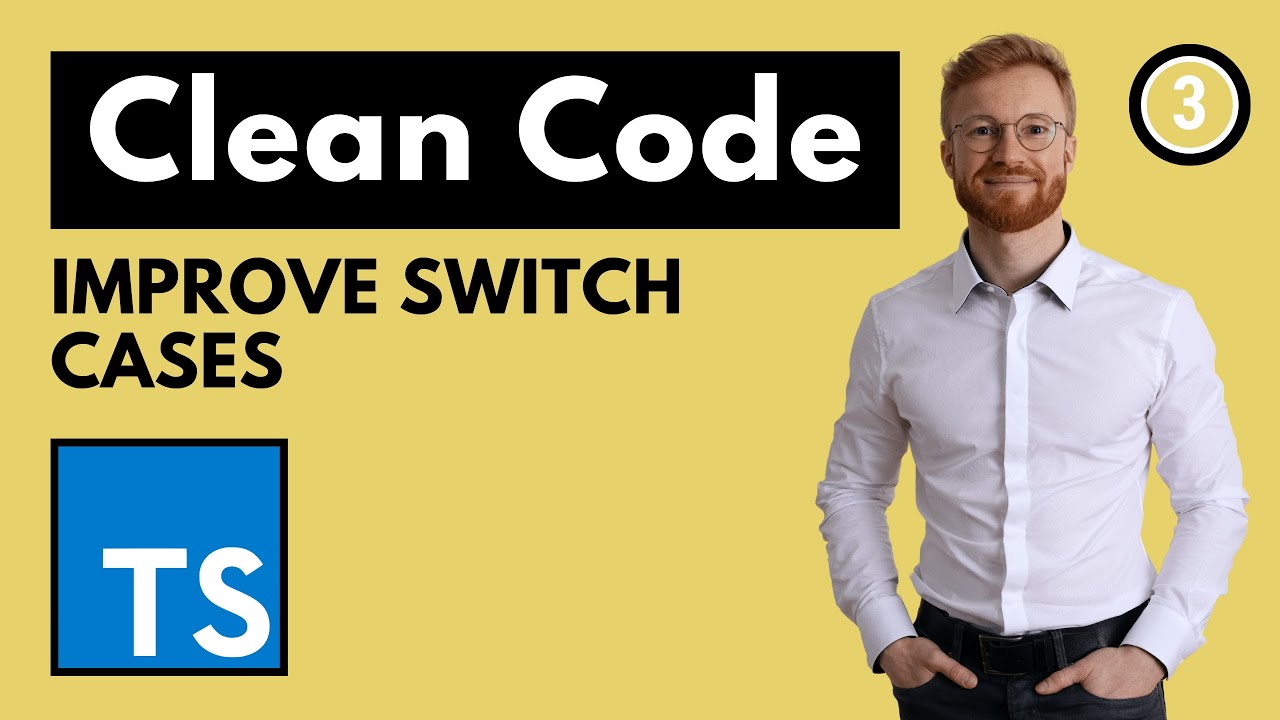
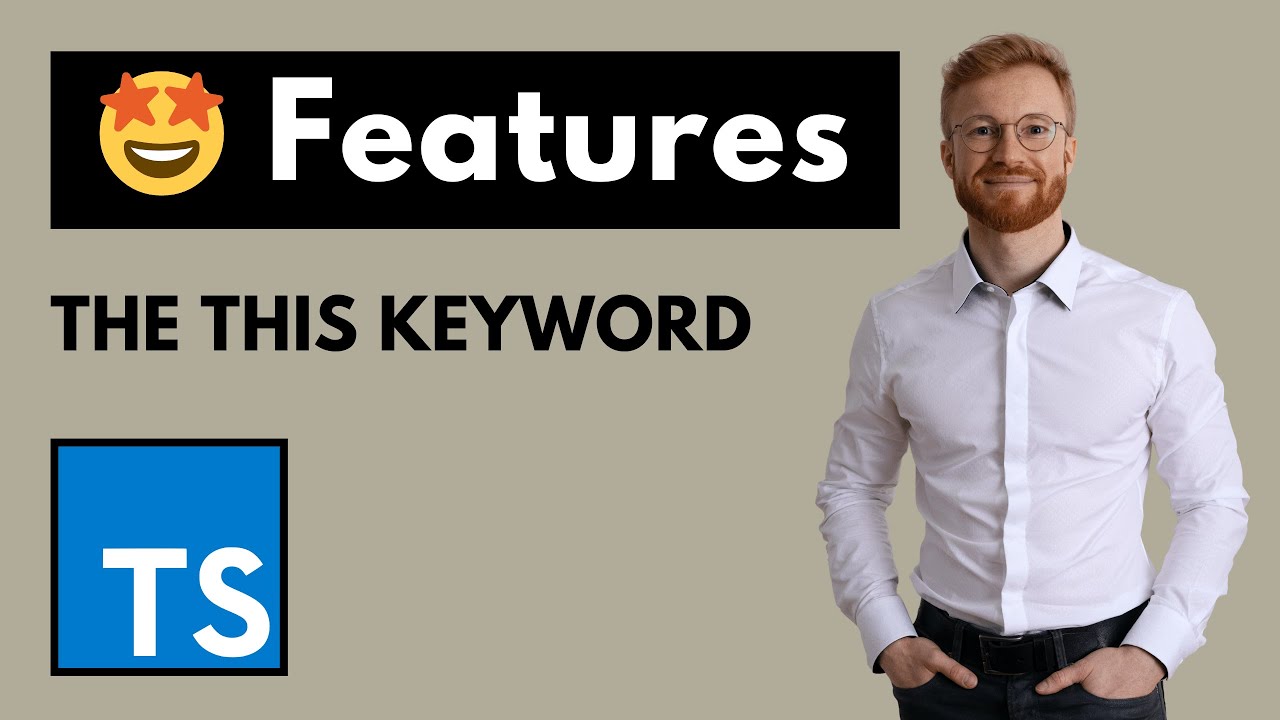
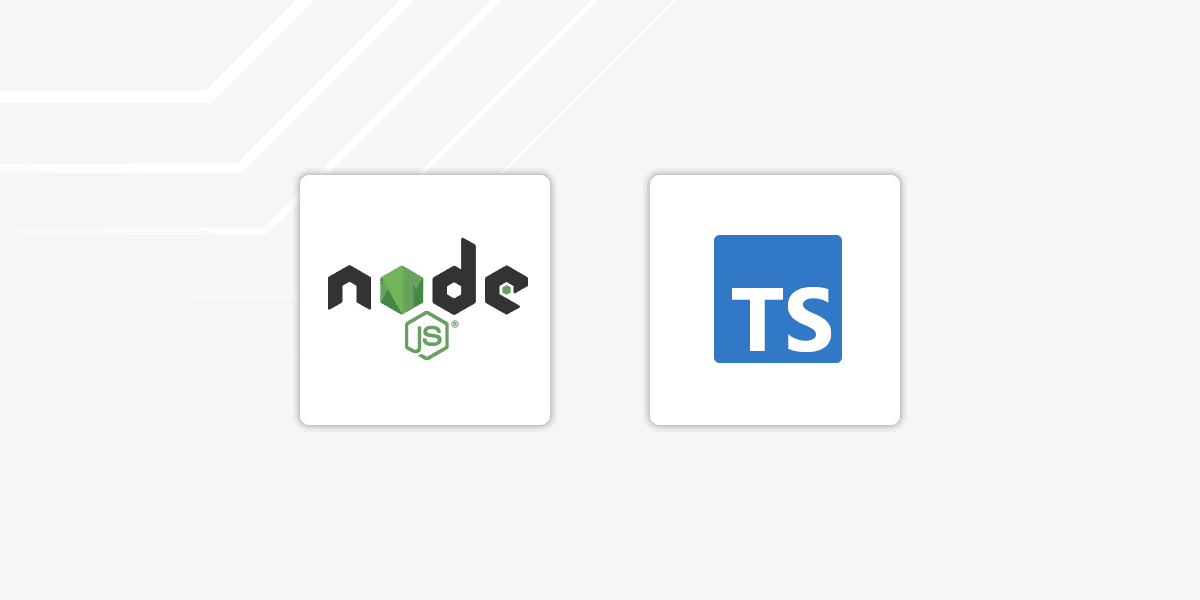
Article link: can only be default-imported using the esmoduleinterop flag.
Learn more about the topic can only be default-imported using the esmoduleinterop flag.
- Module can only be default-imported using esModuleInterop flag
- can only be default-imported using the ‘esModuleInterop’ flag …
- can only be default-imported using the ‘esModuleInterop’ flag
- this module is declared with using ‘export =’, and can only be …
- TSConfig Option: esModuleInterop – TypeScript
- Fix Module can only be default-imported using the … – Medium
- Typescript language server does not use compilerOptions in …
- Compiler Options · typescript
See more: nhanvietluanvan.com/luat-hoc