C Sharp Empty Array
In C#, an empty array refers to an array that does not contain any elements. It is a useful concept when you need to create an array without any initial values. This article will guide you through various methods of defining an empty array in C#.
Initializing an Empty Array
The simplest way to create an empty array in C# is by using the `new` keyword with the array’s specified type and length. Here’s an example:
“`csharp
int[] numbers = new int[0];
“`
In the above code snippet, we have initialized an empty array of integers called `numbers` with a length of zero. This signifies that the array is empty and does not contain any elements.
Creating an Empty Array of a Specific Type
In C#, arrays can store elements of any data type, such as integers, strings, or custom class objects. To create an empty array of a specific type, you need to specify the desired type within the square brackets. Here are a couple of examples:
“`csharp
string[] names = new string[0];
decimal[] prices = new decimal[0];
“`
In the first example, an empty array of strings called `names` is created, while the second example creates an empty array of decimals called `prices`.
Using the `Array` Class to Create an Empty Array
C# provides a built-in class called `Array`, which offers several utility methods for working with arrays. One of those methods is `Empty`, which enables you to create an empty array without explicitly specifying the type and length. Here’s an example:
“`csharp
int[] myArray = Array.Empty
“`
In the above code snippet, an empty array of integers called `myArray` is created using the `Array.Empty
Dynamic Initialization of an Empty Array
In some scenarios, you may not know the exact length of the array beforehand. In such cases, you can dynamically initialize an empty array using the `new` keyword without specifying the length. Here’s an example:
“`csharp
int[] dynamicArray = new int[] { };
“`
In the above code snippet, an empty array of integers called `dynamicArray` is created without specifying the length. The compiler automatically determines the length based on the number of elements provided within the curly braces, which is zero in this case.
Checking if an Array is Empty in C#
To check whether an array is empty or not in C#, you can utilize the `Length` property of the array. The `Length` property returns the number of elements in the array. If the `Length` is zero, it means the array is empty. Here’s an example:
“`csharp
int[] myArray = new int[0];
if (myArray.Length == 0)
{
Console.WriteLine(“The array is empty.”);
}
else
{
Console.WriteLine(“The array is not empty.”);
}
“`
In the above code snippet, we check if the `Length` property of `myArray` is zero to determine if the array is empty or not. If it is zero, a message “The array is empty” will be printed; otherwise, “The array is not empty” will be printed.
Performing Operations on an Empty Array
While an empty array may not have any elements, you can still perform operations on it. For instance, you can assign values to the array, access its length, or iterate over it. However, it is important to note that performing operations on an empty array might not produce meaningful results because there are no elements.
FAQs about Empty Arrays in C#
Q: How can I return an empty array in C#?
A: To return an empty array in C#, you can simply create a new array with a length of zero using the `new` keyword. For example: `return new int[0];`
Q: How can I create an empty array in C#?
A: You can create an empty array in C# by using the `new` keyword and specifying the array’s type and length as zero. For example: `int[] myArray = new int[0];`
Q: How can I empty an array in C#?
A: In C#, you cannot directly empty an existing array. However, you can create a new empty array and assign it to the existing array variable. For example: `myArray = new int[0];`
Q: What is the difference between an empty array and a new array in C#?
A: An empty array refers to an array without any elements, whereas a new array can have elements initialized with default values for the specific type. For example, an empty array of integers has a length of zero, while a new array of integers may have elements with default values of zero.
Q: How can I check if an array is empty in C#?
A: You can check if an array is empty or not by using the `Length` property of the array. If the `Length` is zero, it means the array is empty. For example: `if (myArray.Length == 0)`
Q: How can I convert an object to an empty array in C#?
A: To convert an object to an empty array in C#, you need to create a new array of the desired type and assign it to the object variable. For example: `myObject = new ObjectType[0];`
In conclusion, defining an empty array in C# allows you to create an array without any initial values. Whether you choose to use the `new` keyword, the `Array` class, or dynamic initialization, handling empty arrays requires understanding the concept of array length and exploring the specific requirements of your applications. Remember that empty arrays can still perform various operations, but the absence of elements may limit their meaningfulness.
How To Program In C# – Arrays (E05)
What Is Array Empty () In C#?
In C#, arrays are used to store multiple values of the same type. Sometimes, we may need to check whether an array is empty or not. In order to accomplish this, C# provides a built-in method called `Empty()` that allows us to determine whether an array is empty or not.
The `Empty()` method is a static method of the `Array` class in C#. It returns a new array instance with a length of zero and the same element type as the input array. This method is useful when we want to create an empty array for a specific type without allocating any memory space.
To illustrate the usage of `Empty()` method, let’s consider a scenario where we have an integer array named `numbers` and we want to check if it is empty or not:
“`csharp
int[] numbers = new int[0];
if (numbers.Length == 0)
{
Console.WriteLine(“The array is empty”);
}
else
{
Console.WriteLine(“The array is not empty”);
}
“`
In the above example, we create an empty `numbers` array with a length of zero. By using the `Length` property, we check if the array is empty or not. Since the length of the array is zero, the condition evaluates to true and “The array is empty” message is printed.
The `Empty()` method can also be used to initialize an empty array with a specific type. For instance, if we want to create an empty string array, we can use the following code:
“`csharp
string[] names = Array.Empty
“`
In this example, `Array.Empty
It’s important to note that the `Empty()` method always returns an empty array with a length of zero. This means that the returned array will always have a fixed size and cannot be resized. If you need to dynamically add or remove elements from an array, you should consider using other methods such as `List
FAQs:
Q: How is the `Empty()` method different from assigning a length of zero while creating an array?
A: While both methods create an array with a length of zero, the `Empty()` method returns a new array instance, whereas assigning a length of zero while creating an array initializes it with a length of zero. The main difference lies in the fact that the `Empty()` method allows you to create an empty array of a specific type without allocating any memory space.
Q: Can I use the `Empty()` method to check if a multidimensional array is empty?
A: No, the `Empty()` method can only be used with single-dimensional arrays. To check if a multidimensional array is empty, you can check if the `Length` property of the array is equal to zero.
Q: How does the `Empty()` method affect memory allocation?
A: The `Empty()` method does not allocate any memory space for the returned array since it has a length of zero. It simply returns a new array instance that requires no memory allocation.
Q: Can I assign values to an empty array created using the `Empty()` method?
A: No, an empty array created using the `Empty()` method cannot be assigned any values since it does not have any elements. If you need to assign values to an array, you should consider creating an array with a specific length and then assigning the values.
Q: Is the `Empty()` method available in all versions of C#?
A: The `Empty()` method is available in C# 6.0 and later versions. If you’re using an older version of C#, you may need to use other methods to check if an array is empty.
In conclusion, the `Empty()` method in C# provides a convenient way to check if an array is empty by returning a new array instance with a length of zero. It is a useful method when you need to create an empty array of a specific type without allocating any memory space. Remember that the returned array will always have a length of zero and cannot be resized.
How To Make An Empty Array In C Sharp?
Arrays play a crucial role in programming, serving as a data structure that allows the storage and manipulation of multiple elements of the same data type. In C#, creating an empty array is a common task, as it provides a foundation for dynamically adding and removing elements as needed. In this article, we will explore different ways to make an empty array in C# and discuss their differences and applications.
Creating an Empty Array using the ‘new’ Keyword
The simplest and most common way to create an empty array in C# is by using the ‘new’ keyword. The ‘new’ keyword is followed by the data type of the array and the desired size enclosed in square brackets. Here’s an example:
“`csharp
int[] numbers = new int[0];
“`
In this example, we declare an array of integers named ‘numbers’ with a size of 0. This results in an empty array that can be populated later.
Using the ‘Array.Empty
Starting from C# 6.0, the ‘Array.Empty
“`csharp
int[] numbers = Array.Empty
“`
By calling the ‘Array.Empty
Creating an Empty Array with ‘default(T)’
Another way to initialize an empty array is by utilizing the ‘default’ keyword. The ‘default’ keyword returns the default value for the specified type. By creating an array with a size of 0 and assigning each element as the default value, we can achieve an empty array. Here’s an example:
“`csharp
int[] numbers = Enumerable.Repeat(default(int), 0).ToArray();
“`
In this example, we use the ‘Enumerable.Repeat()’ method to repeat the default value of an integer (which is 0 in this case) zero times. Then, we convert it to an array using the ‘ToArray()’ method. The result is an empty array ready for further customization.
Frequently Asked Questions (FAQs):
Q: Are empty arrays useful in C# programming?
A: Yes, empty arrays serve as a foundation for dynamic data manipulation. They allow for efficient addition, removal, and modification of elements as needed.
Q: Can I change the size of an empty array?
A: Technically, the size of an array declared as empty remains fixed. However, you can create a new array with a different size and copy the elements from the existing empty array if needed.
Q: What is the difference between an empty array and a null array?
A: An empty array is an array with a size of 0, while a null array has no reference assigned to it. It is essential to distinguish between the two to avoid unnecessary null reference exceptions.
Q: Can I add elements to an empty array?
A: Yes, you can add elements to an empty array dynamically using methods like ‘Array.Resize()’ or by creating a new array with a larger size and copying elements from the existing array.
Q: How do I check if an array is empty or not?
A: You can check if an array is empty by using its ‘Length’ property. If the length is equal to 0, it indicates that the array is empty.
Conclusion
Creating an empty array in C# is a fundamental task that allows for dynamic storage and manipulation of data. By using the ‘new’ keyword, the ‘Array.Empty
Keywords searched by users: c sharp empty array Return empty array C#, Create empty array C#, How to empty array in c#, Empty array C#, C# array empty vs new, Array format c#, Object to array C#, Check array empty C#
Categories: Top 71 C Sharp Empty Array
See more here: nhanvietluanvan.com
Return Empty Array C#
In programming, there are often situations where we need to return an array that is empty. Whether it is due to a specific condition being met or simply for convenience, returning an empty array can be a common requirement. In this article, we will explore how to return an empty array in C# and discuss various scenarios where it can be beneficial.
Returning an empty array in C# is quite straightforward. The first step is declaring the array with the appropriate data type:
“`
int[] emptyArray = new int[0];
“`
In the above code snippet, we declare an empty integer array. Setting the array length to 0 ensures that it is empty from the start. We can now return this empty array from a method or use it as needed.
One common scenario where returning an empty array is beneficial is when dealing with filtering operations. For example, suppose we have a method that filters out even numbers from an input array. There might be cases where the input array has no even numbers at all. In these situations, instead of returning a null value, it would be more convenient and error-proof to return an empty array. This way, the calling code can still safely iterate over the result, without worrying about null-reference exceptions.
Another scenario where returning an empty array can be handy is in error handling. Let’s say we have a method that retrieves a list of products from a database based on certain criteria. If the specified criteria do not match any products, returning an empty array instead of null allows the calling code to handle the situation accordingly. It provides a clear indication that there are no matching products, without having to deal with unnecessary null checks.
Frequently Asked Questions (FAQs):
1. What is the advantage of returning an empty array over null?
Returning an empty array over null provides several advantages. It eliminates the need for null checks, reducing the chance of null-reference exceptions. It also allows for easier iteration over the empty array, making code more concise and readable. Furthermore, it provides a clear indication that no elements are present, avoiding any confusion or ambiguity.
2. Can we return an empty array of any data type?
Yes, we can return an empty array of any data type. Whether it is an array of integers, strings, objects, or any other data type, the concept remains the same. Simply declare an array with a length of 0 to indicate that it is empty.
3. Is there any performance impact when returning an empty array?
Returning an empty array has little to no impact on the performance of the application. Since it does not involve any memory allocation or deallocation, the overhead is minimal. In fact, it can even improve performance in some cases by avoiding unnecessary null checks.
4. How should empty arrays be handled by the calling code?
When receiving an empty array, the calling code should be aware that there are no elements present. It should avoid assuming that the array is null and handle it appropriately. For example, it could display a message indicating that no results were found or provide alternative actions for the user.
5. Is it possible to modify an empty array?
No, once an array is declared and instantiated as an empty array, its length cannot be changed. To modify the array, you would need to create a new array with the desired length and copy the elements from the existing array.
In conclusion, returning an empty array in C# is a handy technique to handle scenarios where the absence of elements is expected. It allows for smoother error handling, avoids null-reference exceptions, and provides a clear indication of the absence of results. By following the simple declaration of an array with a length of 0, we can easily return an empty array of any data type. So next time you encounter a situation that requires an empty array, consider using this approach to enhance the clarity and ease of your code.
Create Empty Array C#
Arrays are an essential data structure used in programming languages to store and manipulate collections of elements. In C#, arrays are widely used for their simplicity, flexibility, and efficiency. When working with arrays, you may often encounter situations where you need to initialize an empty array before populating it with values. In this article, we will explore different methods to create empty arrays in C# and provide a comprehensive understanding of their usage.
Method 1: Using the new Operator
The simplest and most common way to create an empty array in C# is by using the new operator. This method involves specifying the array type and size during declaration. Here’s an example:
“`
int[] myArray = new int[0];
“`
In the above code snippet, we create an empty array of integers named `myArray`. The size of the array is set to 0, indicating that no elements are initially stored. This method is useful when you have a fixed upper limit for the number of elements in the array.
Method 2: Using the Array.Empty
Introduced in C# 6.0, the Array.Empty
“`
int[] myArray = Array.Empty
“`
In this code example, we create an empty integer array called `myArray` using the Array.Empty
Method 3: Using the Enumerable.Empty
Another way to create an empty array in C# is by using the Enumerable.Empty
“`
int[] myArray = Enumerable.Empty
“`
In the above code snippet, we first create an empty integer sequence using Enumerable.Empty
FAQs
Q: Can I resize an empty array in C#?
A: No, the size of an array in C# cannot be changed once it is created. However, you can create a new array with the desired size and copy the elements from the original array into the new one if resizing is required.
Q: Are all elements in an empty array initialized to default values?
A: Yes, when you create an empty array in C#, all elements are initialized to their default values. For instance, an empty integer array will have all elements set to 0, while an empty string array will have all elements set to null.
Q: Can I access elements in an empty array?
A: No, attempting to access elements in an empty array will result in an IndexOutOfRangeException. Since an empty array contains no elements, there are no valid indexes to access.
Q: Can I add elements to an empty array?
A: No, an empty array has a fixed size that cannot be modified. To add elements to an existing array, you would need to create a new array with a larger size and copy the elements from the original array into the new one.
Q: When should I use each method to create an empty array?
A: The choice of method depends on the specific use case. If you have a fixed size and type for the array, using the new operator is a straightforward approach. If the size is unknown at compile time, prefer Array.Empty
In conclusion, creating empty arrays in C# is a common requirement when working with collections of elements. Understanding the various methods available, such as using the new operator, Array.Empty
Images related to the topic c sharp empty array
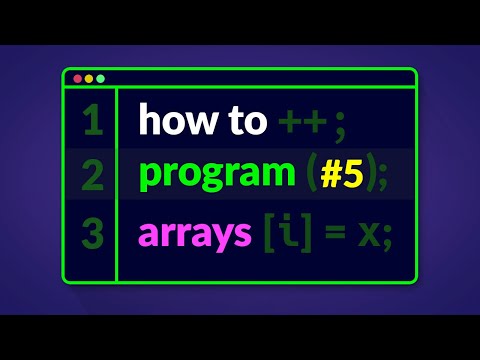
Found 41 images related to c sharp empty array theme

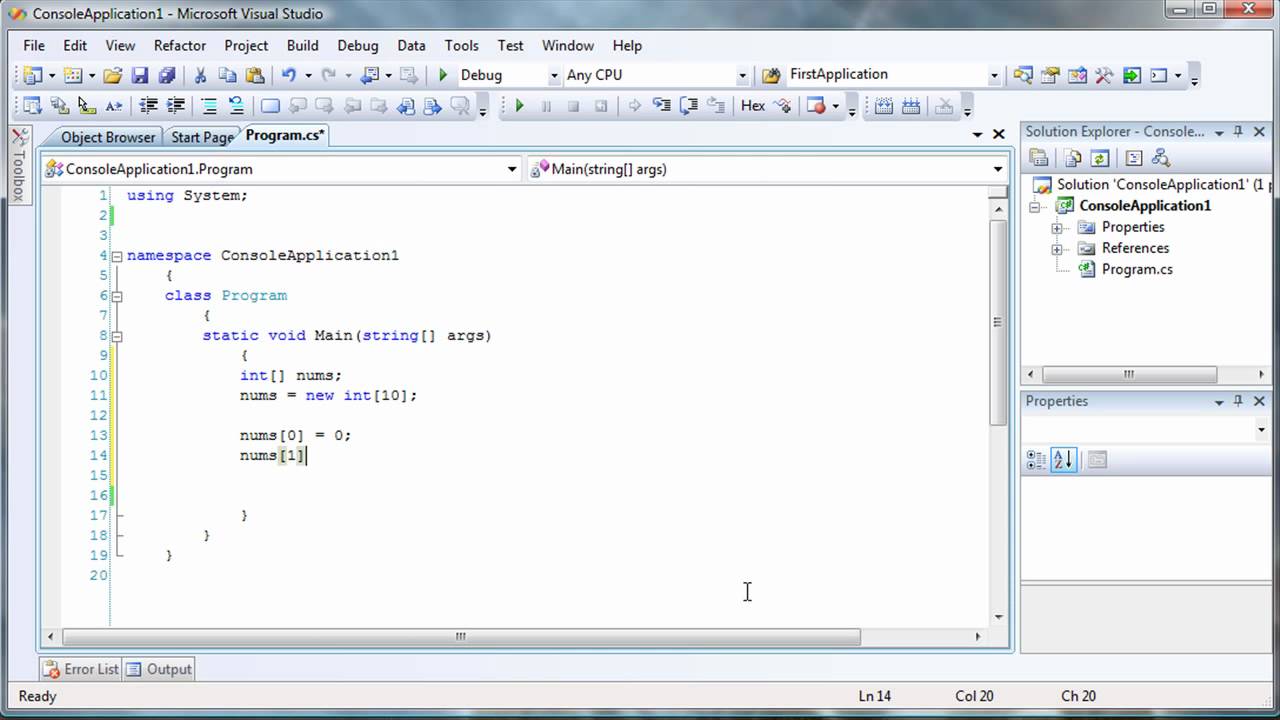


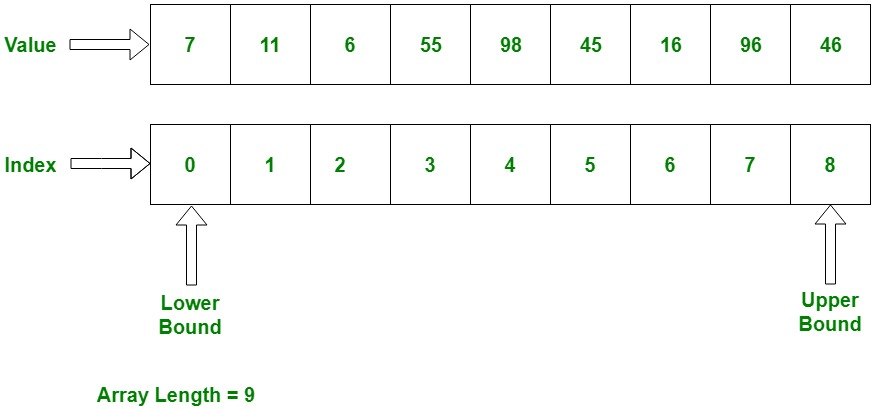


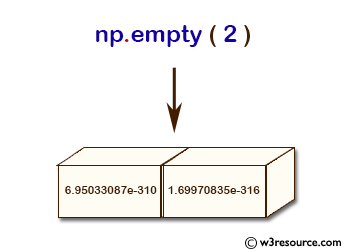
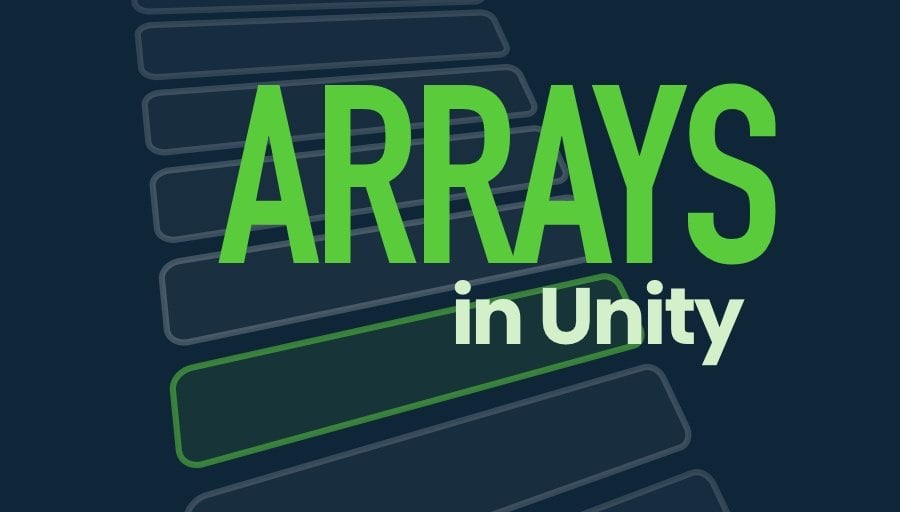
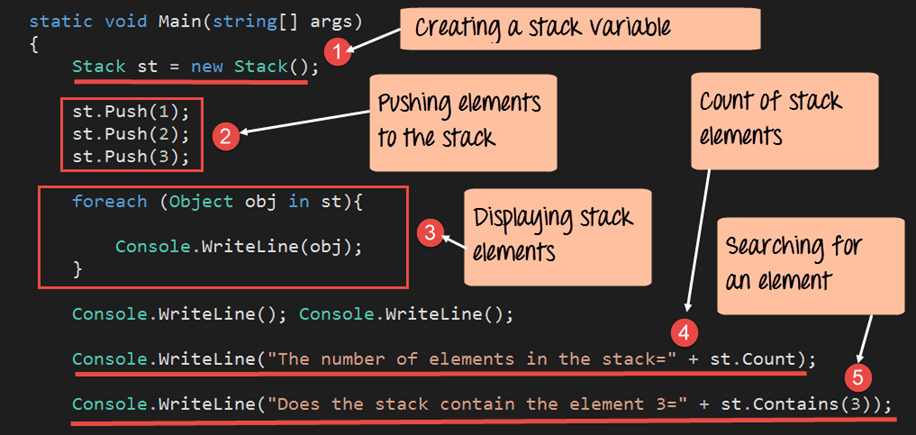
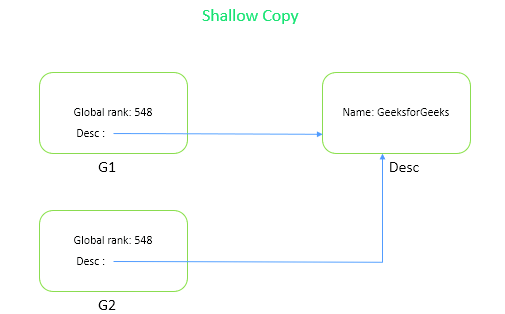
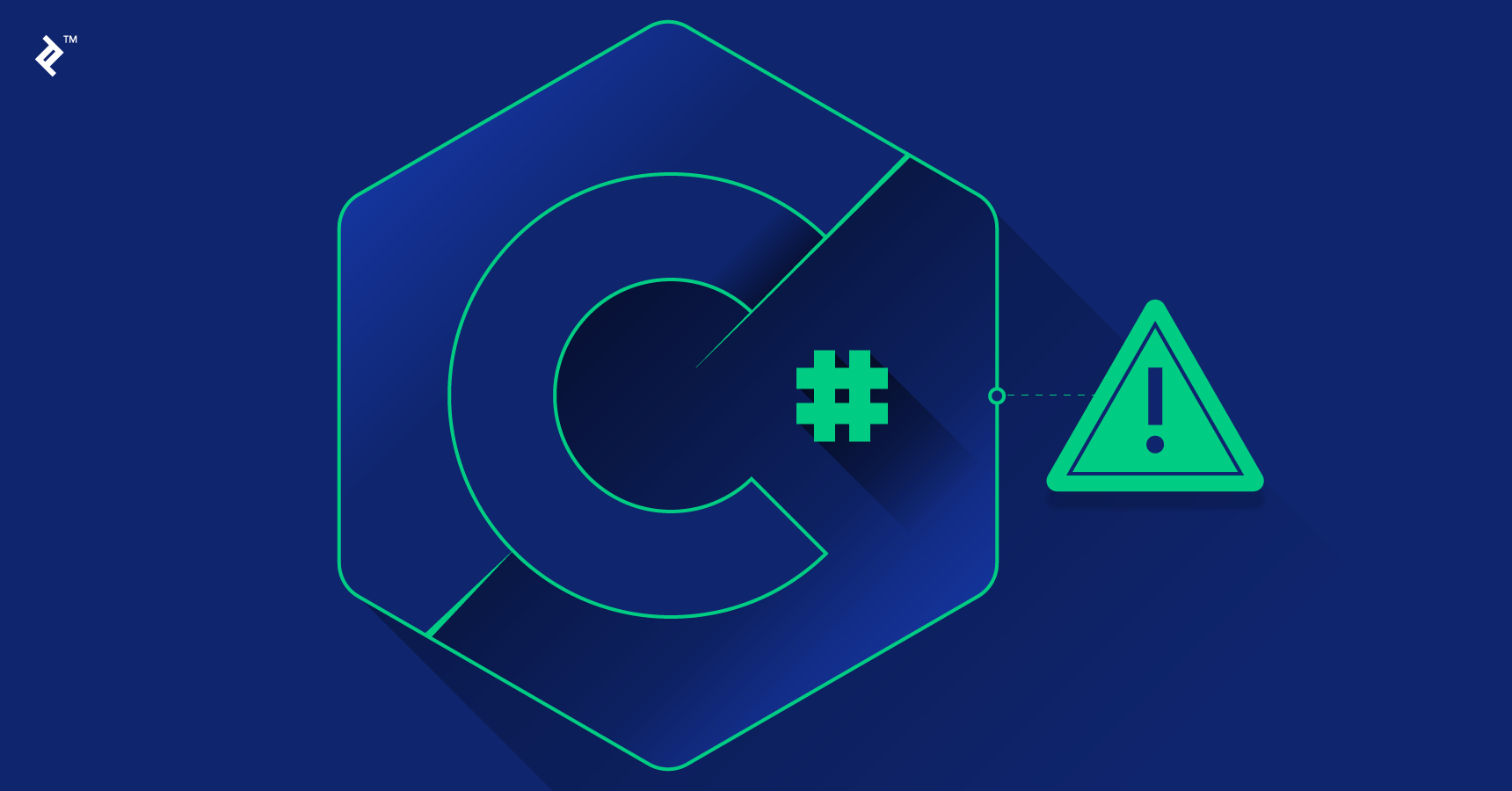

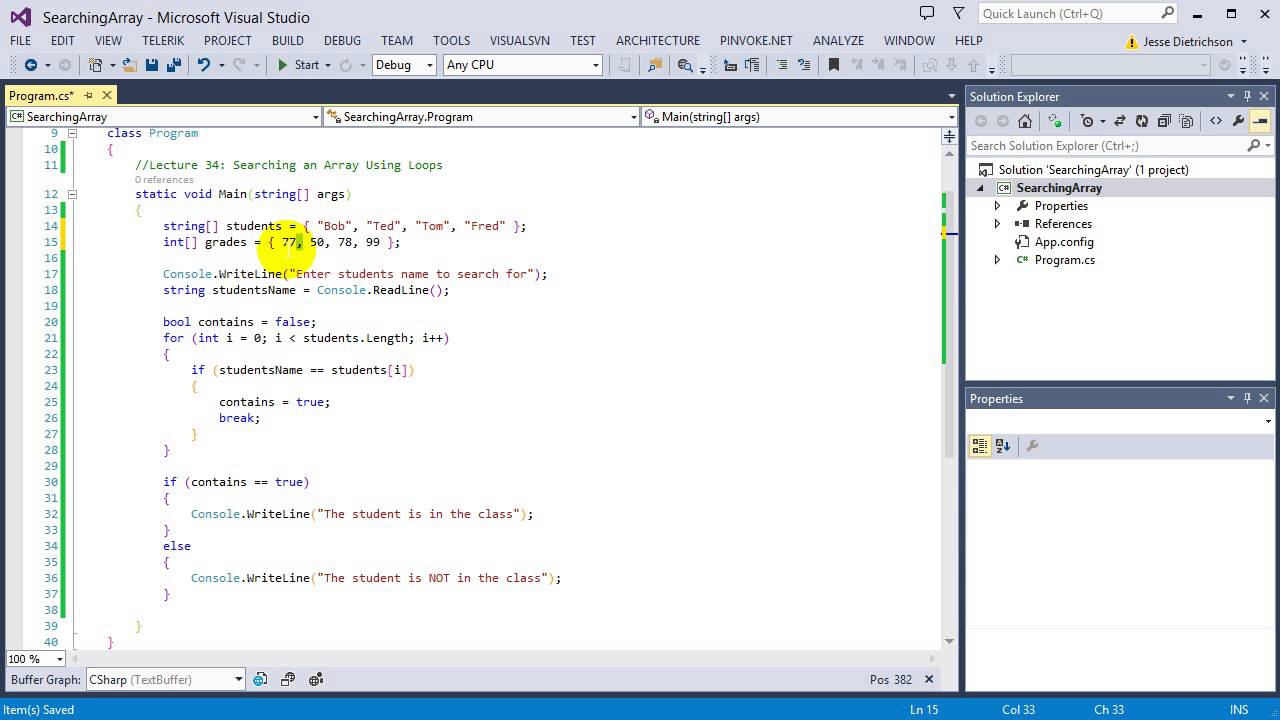



![Queue Implementation Using Array: Your One-Stop Solution [Updated] Queue Implementation Using Array: Your One-Stop Solution [Updated]](https://www.simplilearn.com/ice9/free_resources_article_thumb/Queue_Impl_arr/C%2B%2B_code-Queue_Implementation.png)
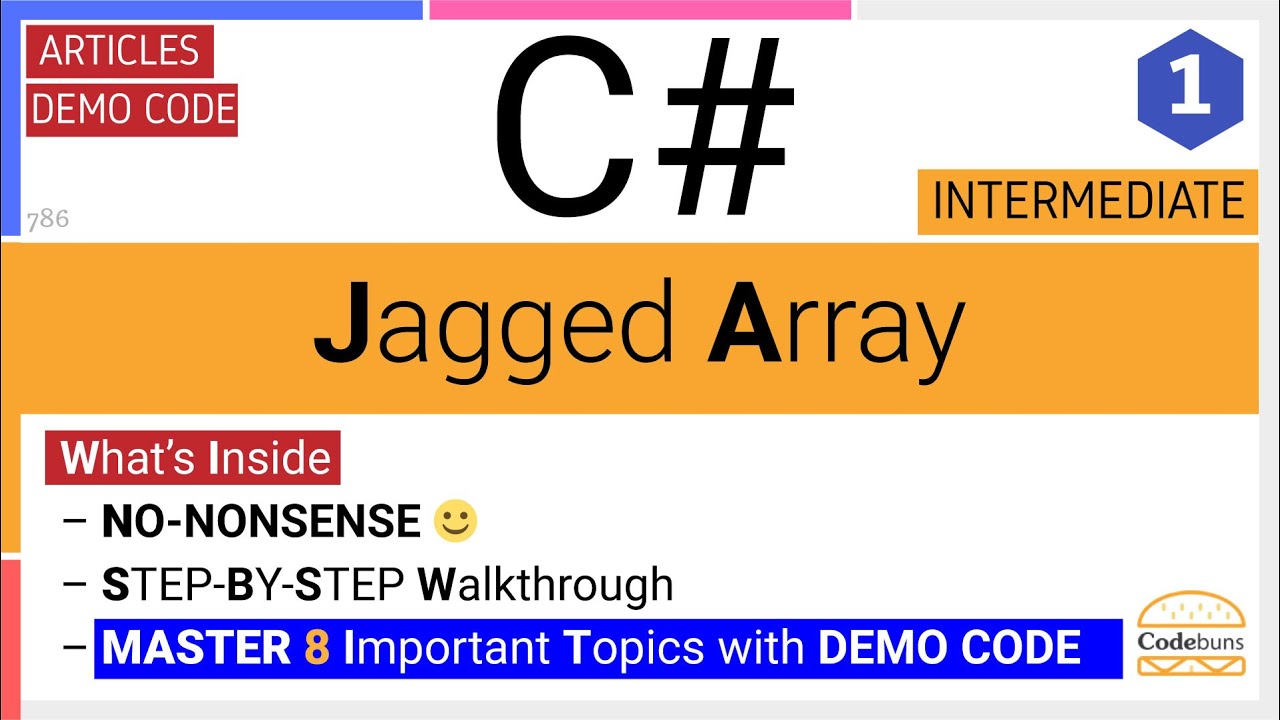
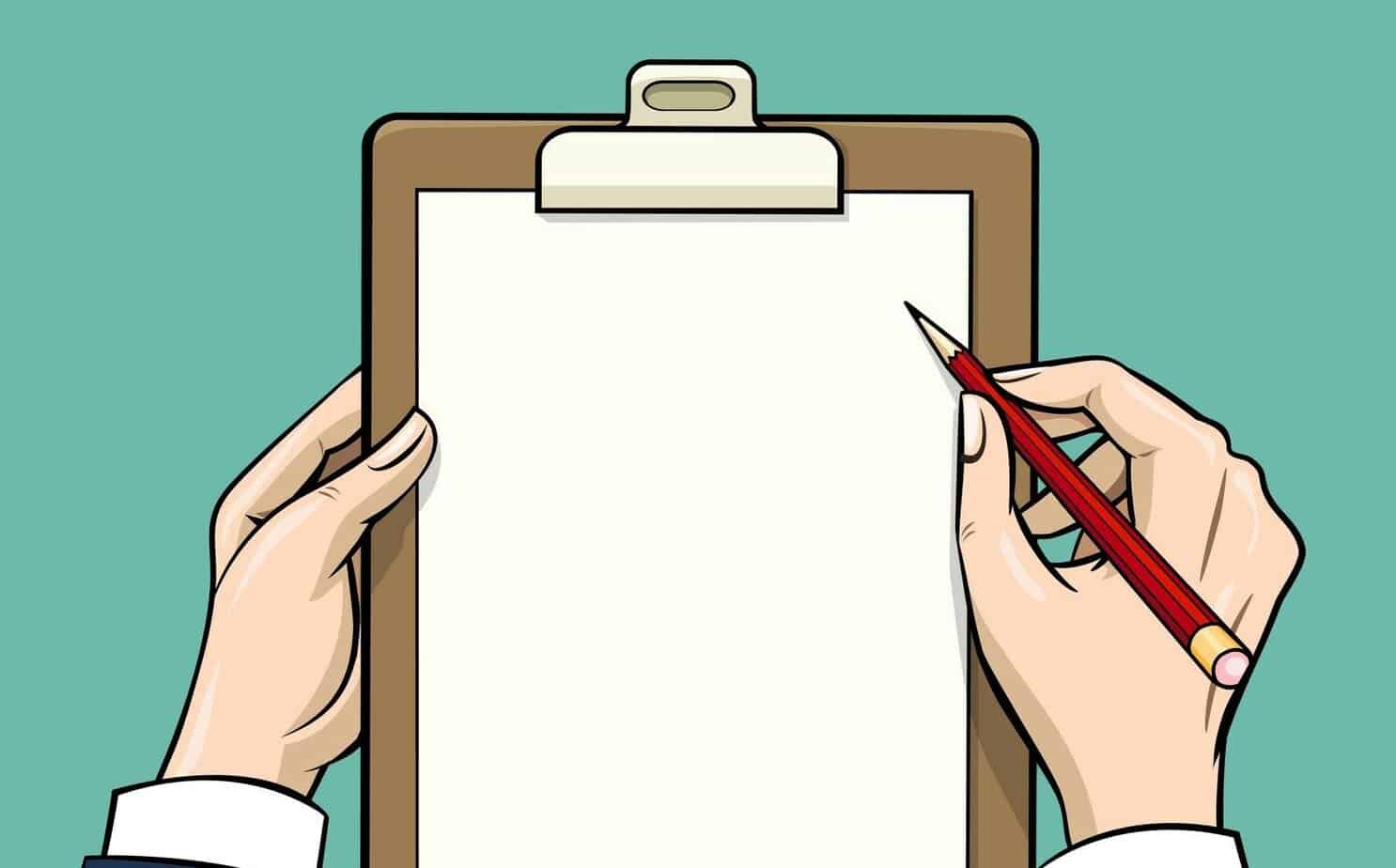

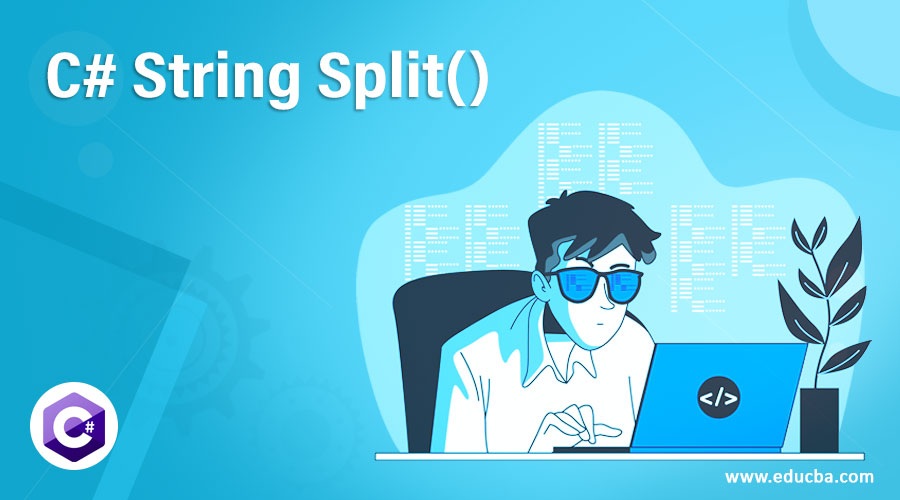
![Queue Implementation Using Array: Your One-Stop Solution [Updated] Queue Implementation Using Array: Your One-Stop Solution [Updated]](https://www.simplilearn.com/ice9/free_resources_article_thumb/C%2B%2B_code2-Queue_Implementation_Using_Array.png)
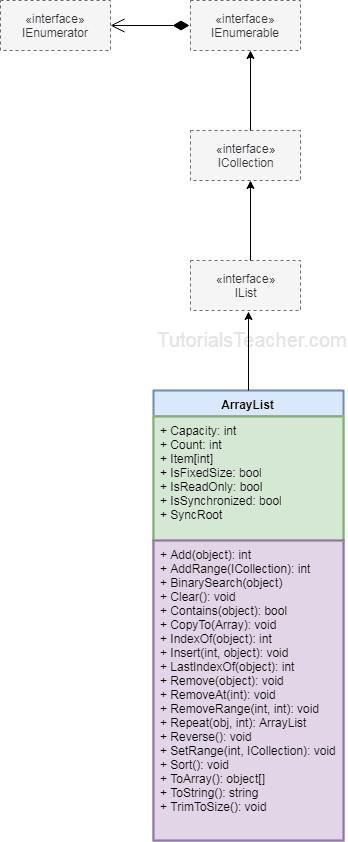

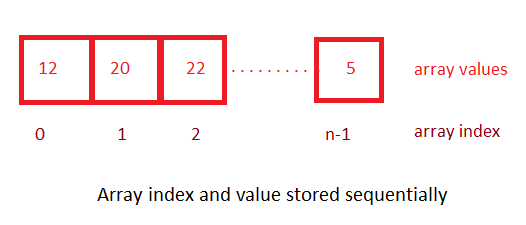
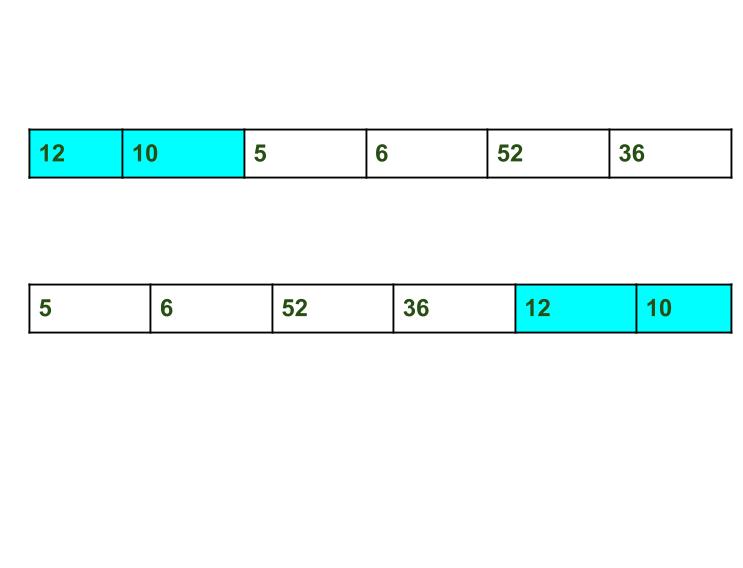

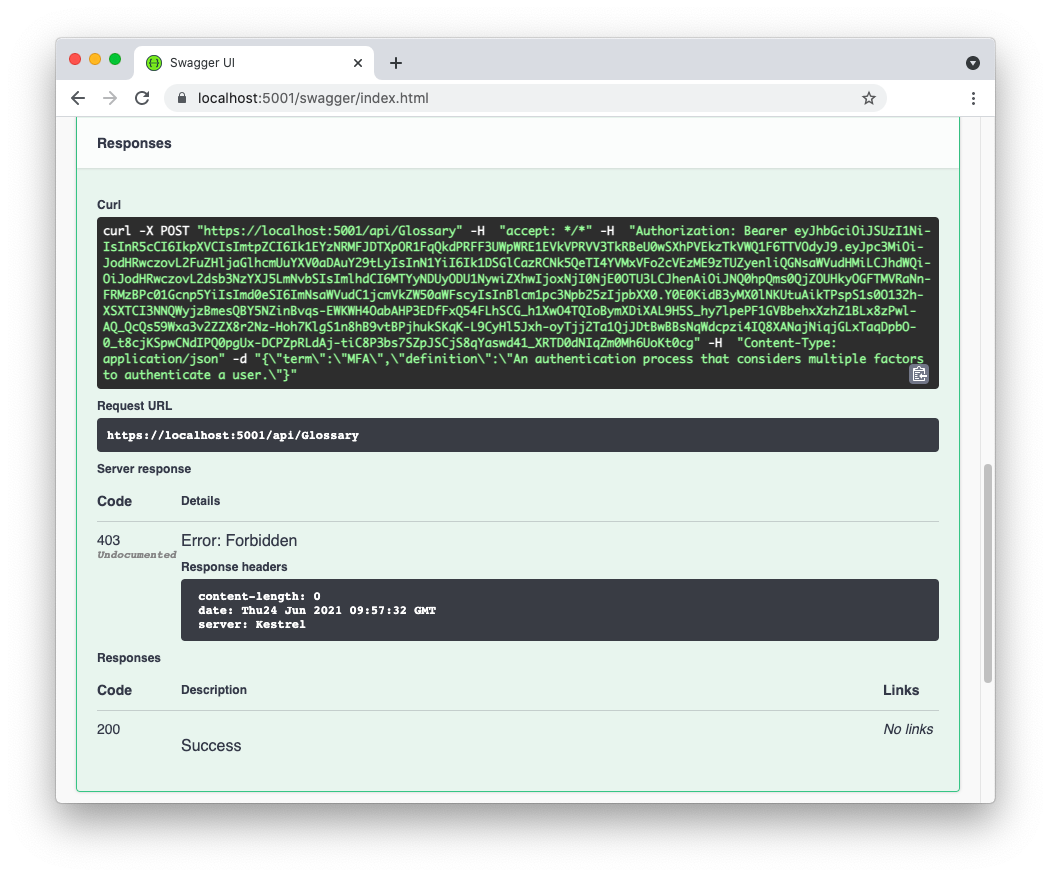
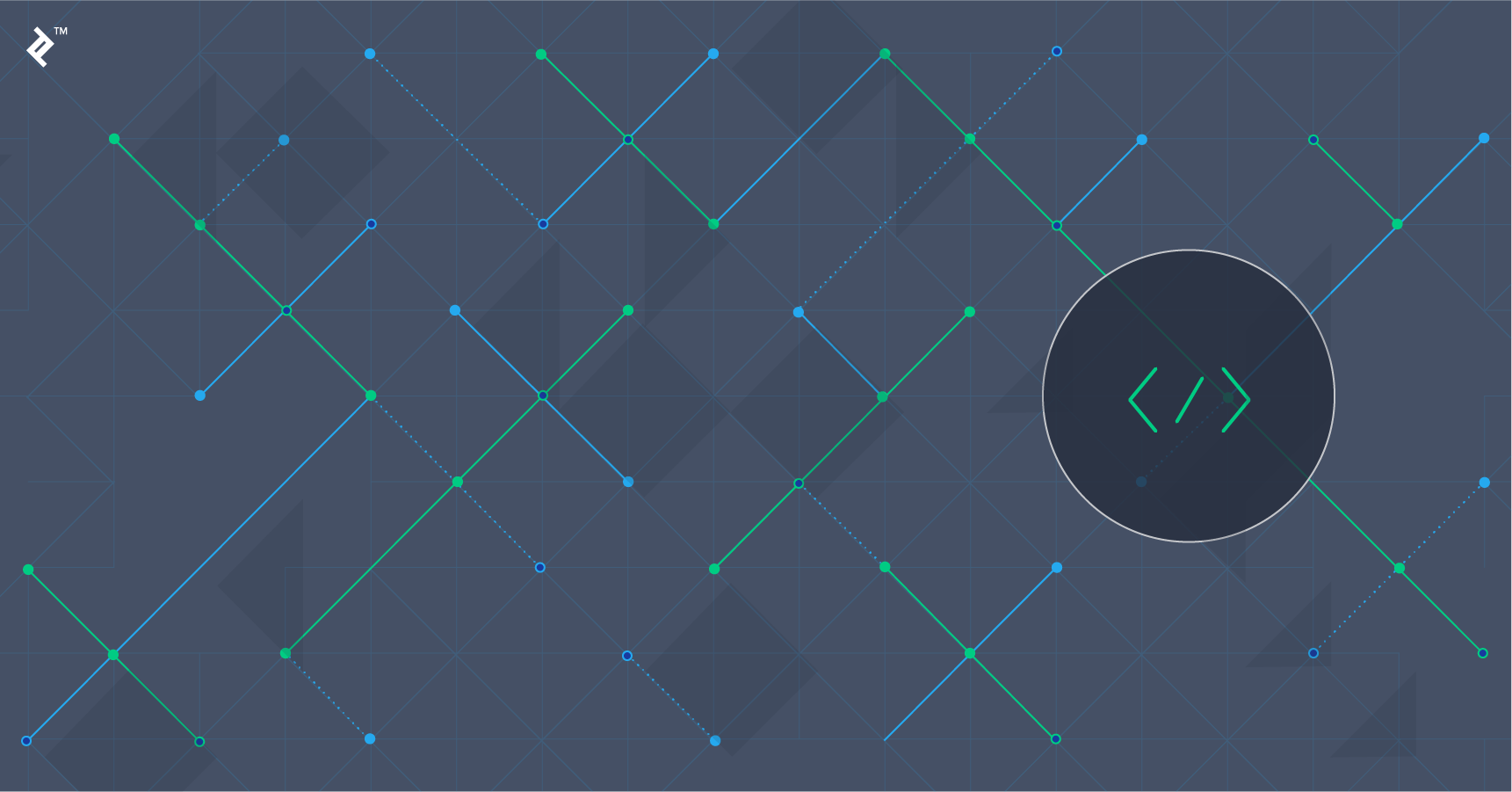

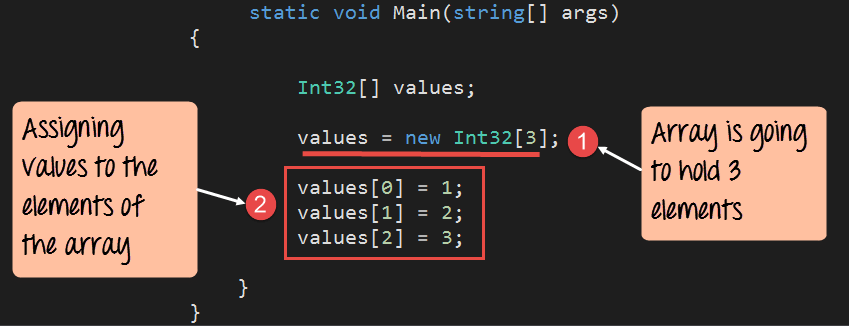
Article link: c sharp empty array.
Learn more about the topic c sharp empty array.
- How do I initialize an empty array in C#? – Stack Overflow
- Declare and initialize an empty array in C# | Techie Delight
- How To Check If An Array Is Empty In C# – C# Corner
- How do you empty an array in C – Tutorialspoint
- Declare and initialize an empty array in C# | Techie Delight
- C# Null Array – Dot Net Perls
- How to Declare an Empty Array in C# – Code Maze
- Array.Empty
Method (System) | Microsoft Learn - How do you empty an array in C – Tutorialspoint
- How To Check If An Array Is Empty In C# – C# Corner
- How to Create an Empty Array in C# ? – TutorialKart
- How to Declare an Empty Array in C# – Linux Hint
- Initialize an Empty Array in C# | Delft Stack
- C# Array Property, Return Empty Array – Dot Net Perls
See more: blog https://nhanvietluanvan.com/luat-hoc