Attributeerror: Nonetype Object Has No Attribute Append
Understanding the AttributeError
In Python, the AttributeError is a common error that occurs when you try to access an attribute or method of an object that does not exist or is not compatible with the object’s type. This error can be frustrating, especially when it involves the ‘append’ method and a NoneType object.
The AttributeError specifically mentions that a NoneType object has no attribute ‘append’. This means that you are trying to call the ‘append’ method on a variable that is assigned the value of None, which is a special data type in Python that represents the absence of a value.
Possible Causes of the AttributeError
The AttributeError can occur due to several reasons. Some of the common causes include:
1. Uninitialized variable: If you have not initialized a variable before trying to call the ‘append’ method on it, it will be assigned the value of None by default.
2. Incorrect function or method call: You might be calling a function or method that does not exist or is not compatible with the object that you are using.
3. Overwriting the object: In some cases, you might accidentally overwrite the object assigned to a variable with the value of None, causing the ‘append’ method to be unavailable.
Working with NoneType Objects
To understand the AttributeError involving a NoneType object, it is crucial to have a basic understanding of how NoneType objects work in Python.
A NoneType object represents the absence of a value or the lack of a value that can be assigned to a variable. It is commonly used to indicate that a function or method has no output or that a variable has not been initialized.
In Python, you can check if an object is of type None by using the ‘is’ operator. For example:
“`
variable is None
“`
This expression will return True if the variable is assigned the value of None, indicating that it is a NoneType object.
The ‘append()’ Method and Lists
Lists are an essential data structure in Python that allows you to store and manipulate multiple values. The ‘append()’ method is a built-in method of lists that allows you to add an element to the end of a list.
For example:
“`
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
“`
In this example, the ‘append()’ method is called on the ‘my_list’ variable, adding the value 4 to the end of the list.
Resolving the AttributeError with ‘append()’
To resolve the AttributeError involving the ‘append()’ method and a NoneType object, you need to ensure that the variable on which you are calling the ‘append()’ method is actually a list and not None.
One common mistake that leads to this error is forgetting to initialize the list before calling the ‘append()’ method on it. To fix this, you can initialize an empty list before using the ‘append()’ method. For example:
“`
my_list = []
my_list.append(1)
print(my_list) # Output: [1]
“`
In this example, an empty list is created before calling the ‘append()’ method, allowing the method to add the value 1 to the list.
Common Mistakes and Pitfalls
There are a few common mistakes and pitfalls that can lead to the AttributeError involving the ‘append()’ method and NoneType objects. Some of these include:
1. Forgetting to initialize a list before calling ‘append()’: As mentioned earlier, if you do not initialize a list before calling the ‘append()’ method, you will encounter the AttributeError.
2. Overwriting a list with ‘None’: If you accidentally assign the value of None to a list variable, you will not be able to use the ‘append()’ method on it. Make sure to avoid overwriting lists with None.
3. Calling ‘append()’ on a non-list object: Ensure that you are calling the ‘append()’ method on an actual list object and not any other data type.
Best Practices to Avoid the AttributeError
To avoid encountering the AttributeError involving the ‘append()’ method and NoneType objects, it is essential to follow best practices while coding. Some of these practices include:
1. Initialize lists before using them. Make sure to create an empty list or assign an existing list to a variable before calling the ‘append()’ method on it.
2. Double-check object types. Before calling the ‘append()’ method, verify that you are working with a list object and not any other data type.
3. Use proper variable naming conventions. Avoid naming variables in a way that can cause confusion or lead to accidental overwriting.
4. Test and debug your code regularly. Regularly test your code and use debugging tools to identify and fix any issues, including AttributeError.
FAQs
1. What is the ‘append()’ method in Python?
The ‘append()’ method is a built-in method of lists in Python. It allows you to add an element to the end of a list.
2. What does the AttributeError “NoneType object has no attribute ‘append'” mean?
This error message indicates that you are trying to call the ‘append()’ method on a variable that is assigned the value of None, which is a NoneType object. NoneType objects do not have the ‘append()’ method.
3. How can I fix the AttributeError involving ‘append()’ and NoneType objects?
Make sure to initialize a list before calling the ‘append()’ method on it. This ensures that the variable is not assigned the value of None. Additionally, double-check that you are calling ‘append()’ on an actual list object and not any other data type.
4. What are some best practices to avoid AttributeError?
To avoid the AttributeError, follow best practices such as initializing lists before using them, verifying object types, using proper variable naming conventions, and regularly testing and debugging your code.
In conclusion, the AttributeError involving the ‘append()’ method and NoneType objects can be resolved by properly initializing lists and double-checking object types. By following the best practices and avoiding common mistakes, you can minimize the occurrence of this error in your Python code.
Python Attributeerror: ‘Nonetype’ Object Has No Attribute ‘Append’
Can You Append A Nonetype?
When working with Python, you may come across the term “NoneType” at some point. NoneType is a special built-in data type in Python that represents the absence of a value. It is often used as a placeholder or to signify the lack of a concrete value. It is inherently different from other data types like integers, strings, or lists, as it doesn’t have any methods or attributes. One common question that arises is whether it is possible to append a NoneType. In this article, we will explore this topic in depth and understand the intricacies involved.
Understanding NoneType
To comprehend the concept of appending a NoneType, it is essential to have a good understanding of what NoneType represents. In Python, NoneType is the type of the keyword “None,” which is used to define a null, empty, or undefined value. While commonly used in cases where a function or variable returns nothing, None can also be assigned explicitly to variables. Its primary purpose is to indicate the absence of a value, similar to the null value in other programming languages.
NoneType, as the name suggests, is a type on its own, and it cannot be modified or extended beyond its core purpose. It does not possess any methods or attributes that allow it to be manipulated or appended like other data types.
Appending NoneType
Since NoneType does not have any methods or attributes, attempting to append a NoneType to a list or any other mutable data structure will result in a TypeError. When trying to append a NoneType, Python raises an exception because NoneType objects are not iterable or mutable.
To better understand this, consider the following example:
“`python
my_list = [1, 2, 3]
my_list.append(None)
print(my_list)
“`
The code snippet above attempts to append a NoneType to an existing list called `my_list`. However, instead of appending None to the list, it raises a TypeError, stating that NoneType object is not iterable.
Output:
“`
Traceback (most recent call last):
File “main.py”, line 2, in
my_list.append(None)
TypeError: ‘NoneType’ object is not iterable
“`
In this case, Python is informing us that NoneType objects cannot be iterated over and, therefore, cannot be appended to a list. Instead, its purpose is to represent nonexistent or undefined values.
Workarounds and Considerations
Although it is not possible to directly append a NoneType to a list, there are several workarounds and considerations to keep in mind depending on your specific use case.
1. Conditional Appending: One approach is to use conditional statements to determine whether to append a value or None to a list. For example:
“`python
my_list = [1, 2, 3]
value = None
if value is not None:
my_list.append(value)
print(my_list)
“`
By using an if statement, the code checks whether the value is not None before appending it to the list. This approach ensures that the code only attempts to append non-None values.
2. Compatibility with Other Data Types: When dealing with different data types, such as strings or integers, it is crucial to ensure that they are compatible with NoneType. For instance, attempting to append a string representation of None (`”None”`) instead of the actual None object can lead to confusion or unexpected behaviors.
3. Error Handling: When working with functions or methods that potentially return None, it is essential to have proper error handling mechanisms in place. This ensures that if None is returned, it is handled appropriately and does not disrupt the flow of the program.
Frequently Asked Questions (FAQs):
Q: Can I append a value to a NoneType directly?
A: No, it is not possible to append a value to a NoneType directly. NoneType does not possess any methods or attributes to support appending or mutation.
Q: What happens if I try to append None to a list without error handling?
A: If you attempt to append None without proper error handling, Python will raise a TypeError, indicating that NoneType is not iterable.
Q: Why is NoneType necessary in Python?
A: NoneType provides a standard way to represent the absence or lack of a value. It helps in signaling special cases or missing values, thereby enhancing code readability and maintainability.
Q: Can I use None in operations or comparisons?
A: Yes, None can be used in operations and comparisons. However, it is crucial to handle it appropriately, as operations involving None may result in unexpected behaviors or errors.
In conclusion, it is not possible to append a NoneType directly. NoneType represents the absence of a value and is different from other data types that possess methods or attributes to support appending or mutation. Understanding the purpose and limitations of NoneType is vital when working with Python to ensure the correct handling of null values. By leveraging conditional statements, proper error handling, and considering compatibility with other data types, you can effectively deal with NoneType as a programmer.
What Is Append In Python With Example?
Python is a high-level, versatile programming language widely used for various applications. One of the fundamental concepts in Python is the concept of lists. Lists in Python are used to store collections of items, and they have built-in functions or methods to manipulate them. One such essential method is append().
The append() method in Python is used to add an element at the end of an existing list. It modifies the original list instead of creating a new one. By using the append() method, we can easily extend the size of a list or add items dynamically.
Syntax of append():
The syntax to use append() is straightforward:
list_name.append(element)
Here, list_name refers to the name of the list to which we want to add an item, and element is the item we want to add. The element can be of any data type, including integers, floats, strings, or even another list.
Example 1:
To understand the append() method better, let’s consider a simple example. Suppose we have a list called ‘fruits’ that contains three elements: ‘apple’, ‘banana’, and ‘orange’. We can add a new fruit, ‘mango’, to the list using the append() method as follows:
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits.append(‘mango’)
print(fruits)
Output:
[‘apple’, ‘banana’, ‘orange’, ‘mango’]
In the code above, we first initialize the list ‘fruits’ with three elements. Then, we use the append() method to add the element ‘mango’ at the end of the list. Finally, we print the updated list, which now includes the new fruit ‘mango’.
Example 2:
The append() method can also work with different data types. Let’s say we have a list called ‘numbers’ that contains two elements: 10 and 20. We can add a new element, 30, to the list using the append() method, as shown below:
numbers = [10, 20]
numbers.append(30)
print(numbers)
Output:
[10, 20, 30]
In the code above, we first initialize the list ‘numbers’ with two elements. Then, we use the append() method to add the element 30 at the end of the list. Finally, we print the updated list, which now includes the new element 30.
FAQs:
Q1. Can I append multiple elements to a list at once using the append() method?
A1. No, the append() method can only add one element at a time. If you want to add multiple elements, you can either call the append() method multiple times or use other list manipulation techniques, such as concatenation or extend().
Q2. What happens if I try to append a list to another list using the append() method?
A2. When you append a list to another list using the append() method, Python treats the appended list as a single item. It does not flatten the appended list or add its individual elements. To add all the elements of one list to another list, you can use the extend() method instead.
Q3. Is the append() method specific to Python lists only?
A3. Yes, the append() method is specific to Python lists. It cannot be used with other data types, such as tuples or dictionaries.
Q4. Can I append an item to a list at a specific position using the append() method?
A4. No, the append() method always adds an item at the end of the list. If you want to insert an item at a specific position, you can use the insert() method or other list manipulation techniques.
In conclusion, the append() method in Python is a powerful tool that allows us to add an element at the end of a list. It provides a simple and efficient way to dynamically extend the size of a list or add items as needed. By understanding how to use the append() method and its syntax, you can effectively manipulate lists in Python and build more robust programs.
Keywords searched by users: attributeerror: nonetype object has no attribute append Command append Python, Extend and append in Python, Python create empty list and append in loop, NoneType to list, Python add list to list, Append item to list Python, NoneType’ object is not iterable, Append multiple elements Python
Categories: Top 78 Attributeerror: Nonetype Object Has No Attribute Append
See more here: nhanvietluanvan.com
Command Append Python
The append command in Python is designed to add elements to the end of a list, expanding its size dynamically. This operation modifies the original list, making it a mutable data type. Whether you are working with a pre-defined list or creating an empty list, the append command provides an efficient solution to add new elements or data points as your program executes.
Syntax-wise, the append command is straightforward to use. It has the following structure:
“`python
list_name.append(item)
“`
Here, `list_name` refers to the name of the list variable you are working with, while `item` represents the element you want to append at the end of the list. It is important to note that the item being appended can be of any data type, including strings, integers, floating-point numbers, or even other lists.
One of the main advantages of using the append command is that it allows for dynamic, on-the-fly expansion of lists. This can be especially useful in scenarios where the size or content of the list is determined during the runtime of a program. By appending elements to the list, you can keep track of data points without having to allocate memory or define a fixed size for the list beforehand.
Let’s dive into an example to better understand the append command. Consider the following code snippet:
“`python
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits.append(‘kiwi’)
print(fruits)
“`
In this example, we start with a pre-defined list of fruits. Using the append command, we add a new fruit, ‘kiwi’, to the existing list. Running the code will yield the following output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘kiwi’]
“`
As you can see, the append command successfully added the ‘kiwi’ element to the original list, extending its size dynamically.
There are a few crucial points to keep in mind while working with the append command in Python. First, it modifies the original list, so every time you use the append command, the list itself is updated. Therefore, if you need to preserve the original list, you may consider creating a copy or using other list methods to avoid unintentional modifications.
Additionally, it is worth noting that the append command only adds the specified element to the end of the list. If you need to insert an element at a specific position or perform a more complex operation, other list methods, like insert or extend, might be a better fit.
Now, let’s address some frequently asked questions (FAQs) related to the append command in Python:
Q1: Can I append multiple elements at once using the append command?
A1: No, the append command only supports the addition of a single element at a time. However, if you have multiple elements to add, you can use a loop or list comprehension to append them iteratively.
Q2: Can I append a list to another list using the append command?
A2: No, the append command does not support directly appending one list to another. To achieve that, you can use the extend method, which takes an iterable as an argument and adds its elements to the end of the list.
Q3: Is there any performance difference between appending elements using the append command versus using the “+” operator?
A3: Yes, there is a performance difference. The append command modifies the original list in place, while the “+” operator creates a new list by concatenating two existing lists. For large lists, the append command is generally faster and more memory-efficient.
In conclusion, the append command in Python is a powerful tool when it comes to dynamically expanding lists. Its simplicity and flexibility make it an essential method to add elements to a list during program execution. Understanding how to use the append command correctly and its limitations will help you write efficient and clear Python code while maintaining the integrity of your lists.
Extend And Append In Python
Before diving into the details, it is important to understand the concept of lists in Python. A list is an ordered collection of items, enclosed in square brackets ([ ]). It can store elements of different data types, including integers, floats, strings, or even other lists. Lists are mutable, meaning they can be modified after they are created, making them a flexible and powerful tool for data manipulation.
Now, let’s focus on the extend method. The extend method is used to add multiple elements to the end of an existing list. It takes an iterable (such as a list, tuple, or string) as its argument and appends each element from the iterable to the original list. The original list is modified in place, and the method returns None.
Consider the following code snippet as an example:
“`
my_list = [1, 2, 3]
new_elements = [4, 5, 6]
my_list.extend(new_elements)
print(my_list)
“`
The output of this code will be: [1, 2, 3, 4, 5, 6]. As you can see, the extend method adds the elements from `new_elements` to `my_list`, resulting in the extended list.
On the other hand, the append method is used to add a single element to the end of a list. It takes a single argument, and that argument becomes the new element of the list. Similar to the extend method, the append method modifies the original list in place and returns None.
Let’s look at an example to illustrate the usage of the append method:
“`
my_list = [1, 2, 3]
new_element = 4
my_list.append(new_element)
print(my_list)
“`
The output will be: [1, 2, 3, 4]. In this case, the append method adds the new element, `4`, to the end of the list `my_list`.
To summarize the key difference between extend and append: extend adds multiple elements to the end of a list, while append adds a single element.
Now, let’s discuss some common scenarios where extend and append can be useful.
– Use extend when you have another list or iterable and want to combine its elements with an existing list. For example, when working with data sets or when merging multiple lists together, the extend method can be handy.
“`
data1 = [1, 2, 3]
data2 = [4, 5, 6]
data1.extend(data2)
print(data1)
“`
The output will be: [1, 2, 3, 4, 5, 6].
– Use append when you want to add a single element to the end of a list. This can be useful when you are dynamically building a list or when you want to add elements one at a time.
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits.append(‘mango’)
print(fruits)
“`
The output will be: [‘apple’, ‘banana’, ‘orange’, ‘mango’].
Frequently Asked Questions (FAQs):
Q: Can I use extend to add elements of different data types to a list?
A: Yes, extend can be used to add elements of different data types. Since lists in Python can store elements of different types, the extend method simply adds the new elements to the end of the list.
Q: Can the append method add multiple elements at once?
A: No, the append method can only add a single element at a time. If you need to add multiple elements, you can either use a loop to iterate over the elements and append them one by one or use the extend method.
Q: Does extend and append modify the original list or create a new list?
A: Both extend and append methods modify the original list in place. The methods do not create a new list.
Q: Which method is more efficient, extend or append?
A: The efficiency of extend and append depends on the specific use case. In general, append is slightly more efficient as it only needs to add a single element. However, the difference in efficiency is usually negligible unless dealing with extremely large lists.
Q: Can I use extend and append together in the same list?
A: Yes, you can certainly use both methods in combination. It depends on your requirements and the data you are working with. In some cases, using a combination of extend and append can be a convenient way to build or modify a list.
In conclusion, extend and append are two important methods for manipulating lists in Python. While they have similar functionality, their key difference lies in the way elements are added to the list. Understanding when and how to use extend and append can greatly enhance your ability to work with lists in Python.
Python Create Empty List And Append In Loop
Creating an empty list in Python is a straightforward task. You can simply assign an empty pair of square brackets to a variable. For example:
“`python
my_list = []
“`
Now that we have an empty list, let’s move on to appending items to it in a loop. To do this, we can use the `append()` method, which adds an item to the end of the list.
Let’s say we want to create a list of integers from 1 to 10. We can do this using a simple for loop and the `append()` method. Here’s an example:
“`python
my_list = [] # Create an empty list
for i in range(1, 11): # Loop from 1 to 10
my_list.append(i) # Append each number to the list
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
“`
In the above code snippet, the range function generates numbers from 1 to 10. The for loop iterates over each number in the range, and the `append()` method adds the current number to the list `my_list`. Finally, we print the resulting list.
Appending items in a loop is a powerful technique that allows you to dynamically add elements to a list based on conditions or calculations.
But what if we want to append items to a list based on user input? We can easily achieve this by utilizing a while loop. Here’s an example:
“`python
my_list = [] # Create an empty list
while True:
user_input = input(“Enter a number (or ‘q’ to quit): “)
if user_input == ‘q’: # Break the loop if ‘q’ is entered
break
my_list.append(int(user_input)) # Convert the input to an integer and append to the list
print(my_list)
“`
In this code snippet, we create an infinite while loop using `while True`. Inside the loop, we ask the user to enter a number. If the user enters ‘q’, we break out of the loop. Otherwise, we convert the user input to an integer and append it to the list `my_list`. Finally, we print the list.
Appending items to a list in a loop allows you to capture and store a dynamic set of inputs, which can be extremely useful in a wide range of scenarios.
Now, let’s address some frequently asked questions about creating an empty list and appending items in a loop using Python.
**FAQs**
**Q1**: Can a list be created with elements from different data types?
**A1**: Absolutely! Python lists are versatile and can hold elements of different data types. For example, you can have a list with integers, strings, or even other lists.
**Q2**: Is it possible to append multiple items to a list at once?
**A2**: Yes, it is possible. You can use the `extend()` method to add multiple items to a list in one go. For example:
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
“`
**Q3**: Can I append another list to an existing list?
**A3**: Yes, you can append one list to another using the `+` operator or the `extend()` method. Here’s an example using the `extend()` method:
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
“`
**Q4**: Is it possible to append items in a specific position in a list?
**A4**: Yes, using the `insert()` method you can insert an item at a specific position in a list. The method takes two arguments: the index position and the item to be inserted. For example:
“`python
my_list = [1, 2, 3, 5]
my_list.insert(3, 4)
print(my_list) # Output: [1, 2, 3, 4, 5]
“`
**Q5**: What happens if I append an entire list to itself?
**A5**: Appending a list to itself will result in a circular reference and infinite recursion. Python detects this and throws an error.
In conclusion, creating an empty list and appending items to it in a loop is a fundamental technique in Python. It allows you to dynamically construct lists based on various conditions, user inputs, or calculations. By mastering this technique, you expand your ability to handle data effectively and efficiently in Python.
Images related to the topic attributeerror: nonetype object has no attribute append
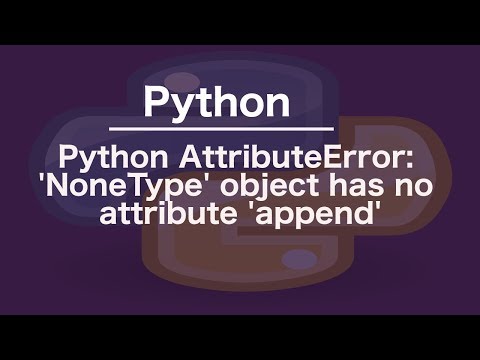
Found 33 images related to attributeerror: nonetype object has no attribute append theme


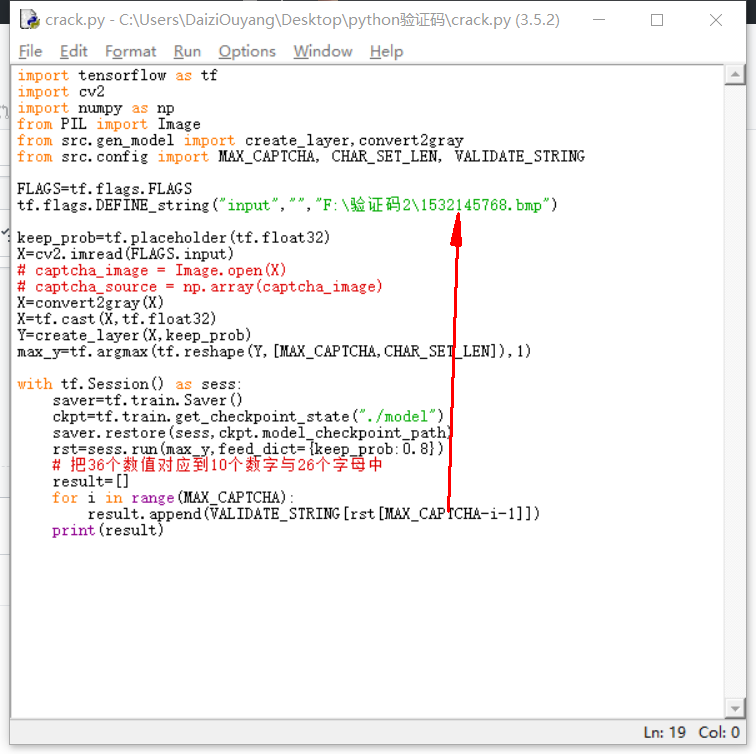
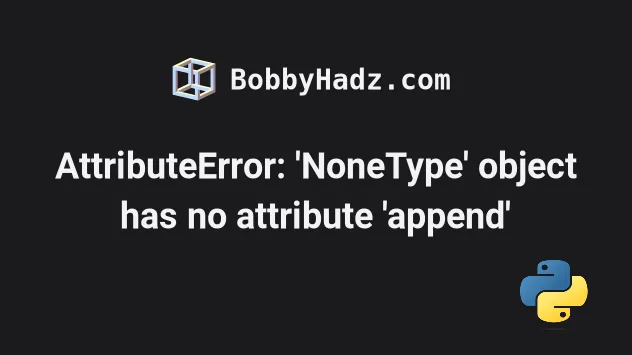
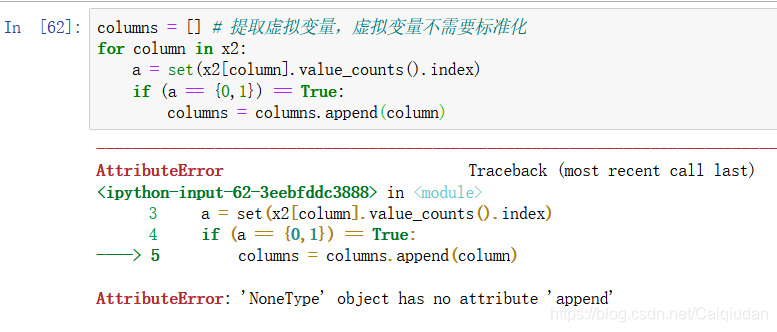
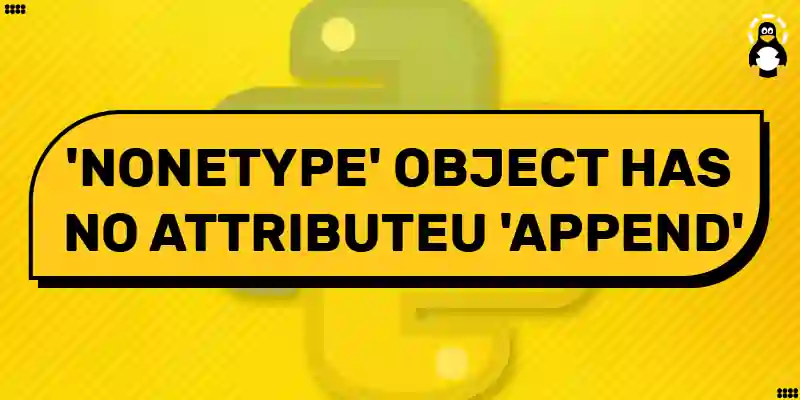
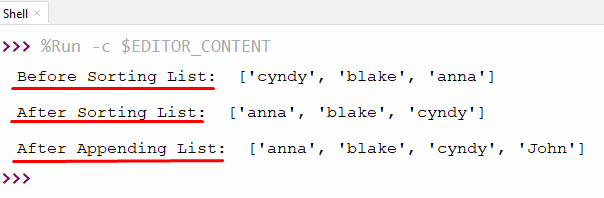
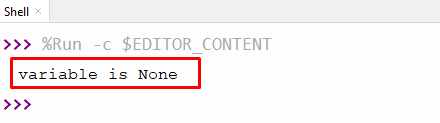


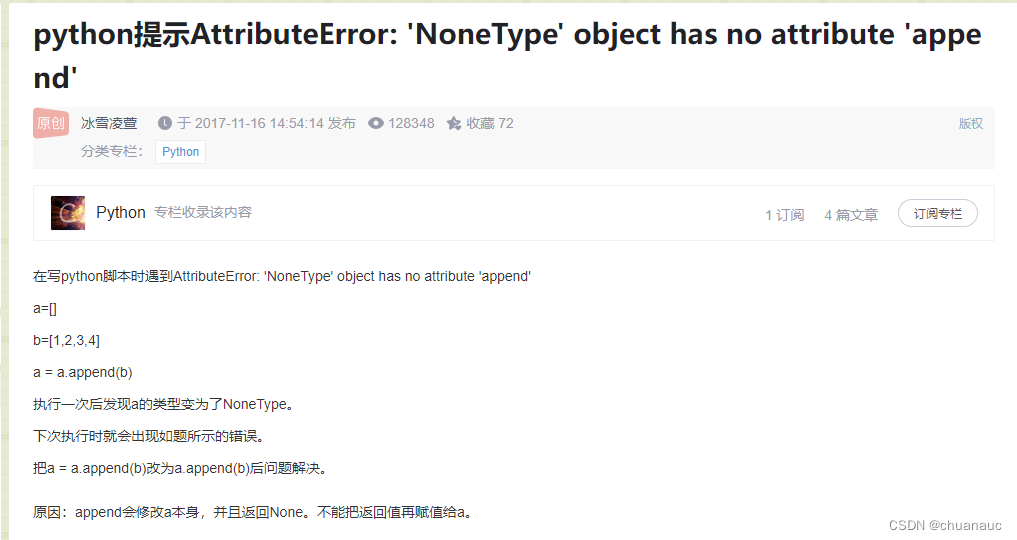
![Solved] AttributeError: 'module' object has no attribute in 3minutes - YouTube Solved] Attributeerror: 'Module' Object Has No Attribute In 3Minutes - Youtube](https://i.ytimg.com/vi/LLmv7oiqjQ4/maxresdefault.jpg)

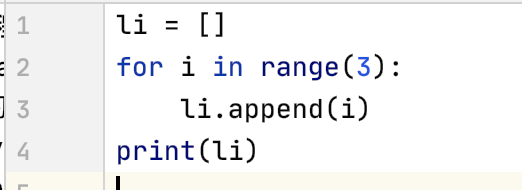
![Attributeerror: 'str' object has no attribute 'append' [SOLVED] Attributeerror: 'Str' Object Has No Attribute 'Append' [Solved]](https://itsourcecode.com/wp-content/uploads/2023/03/attributeerror-str-object-has-no-attribute-append.png)

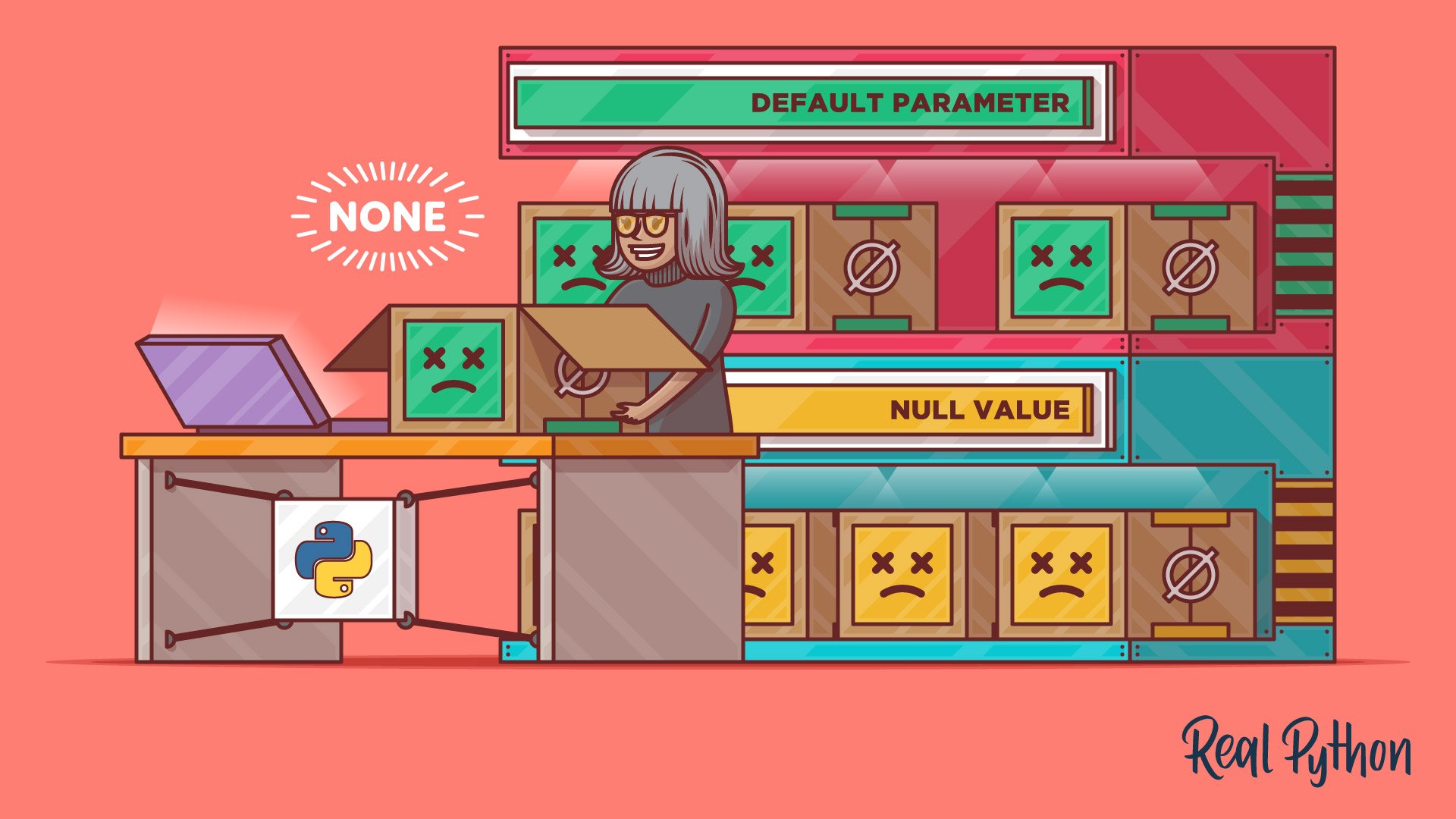

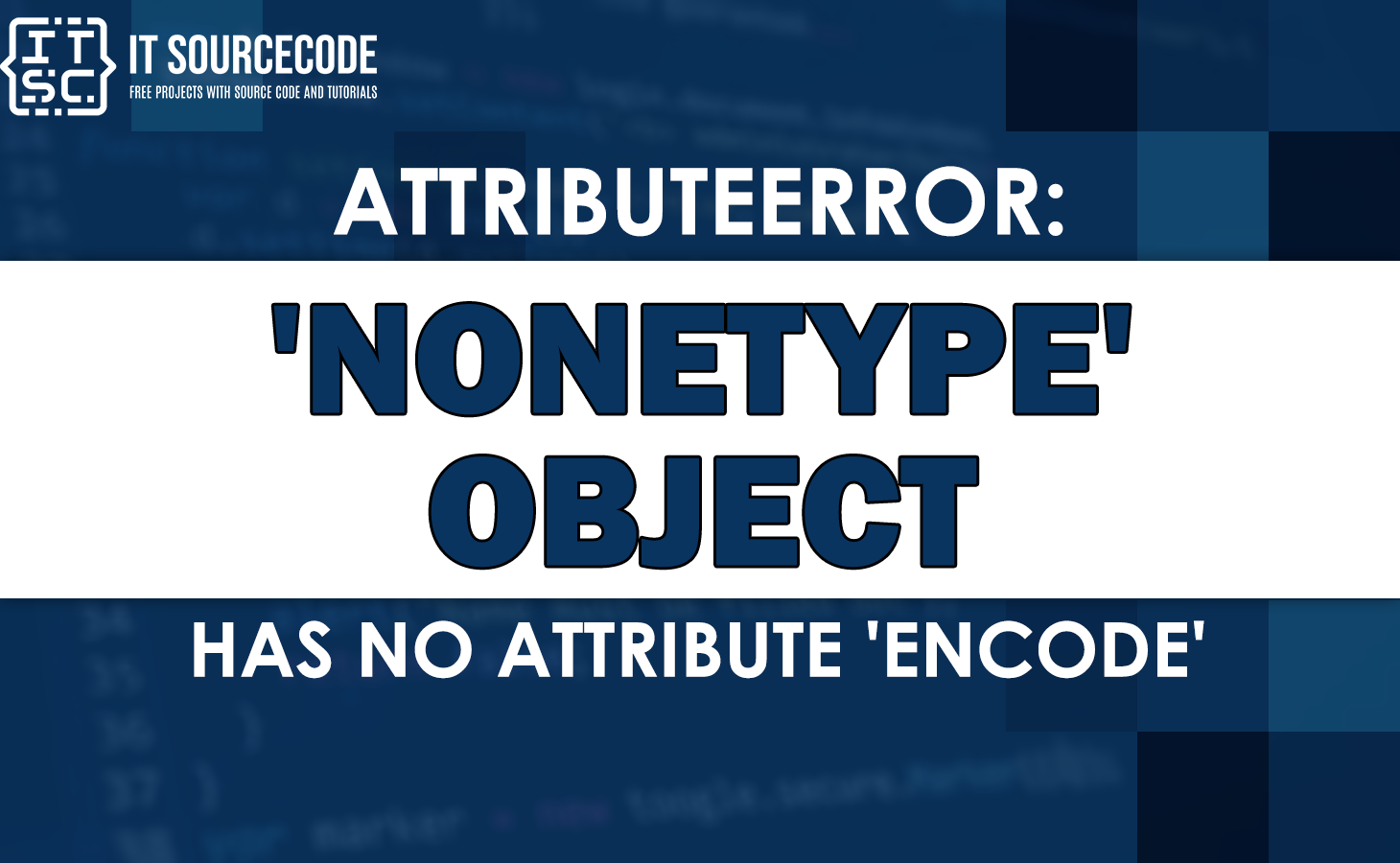
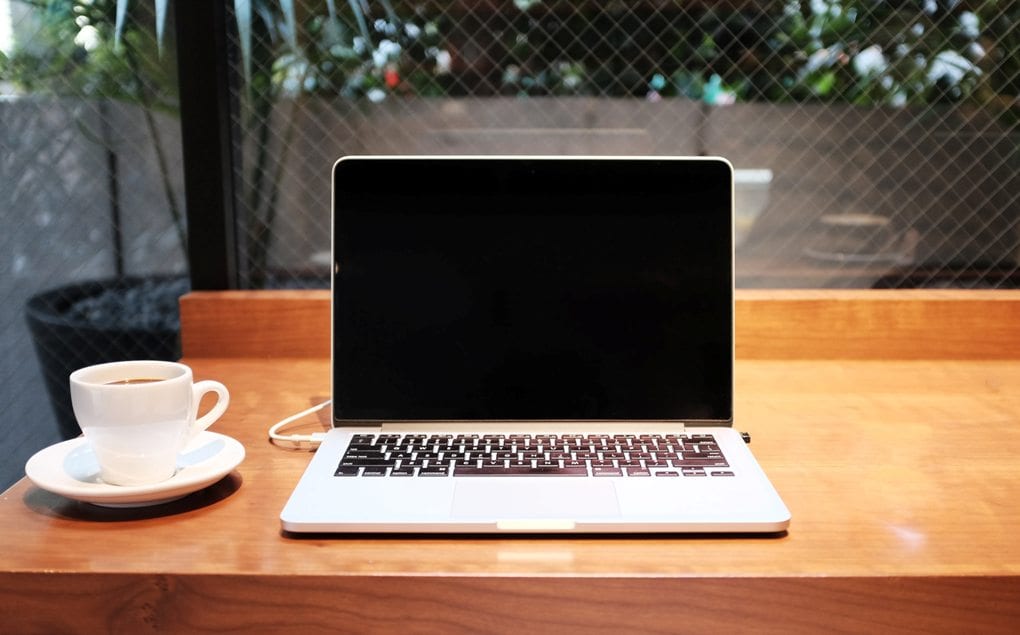

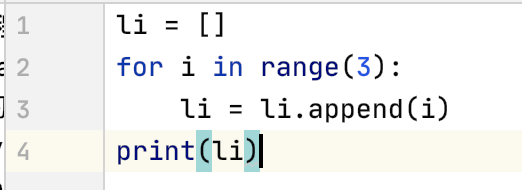



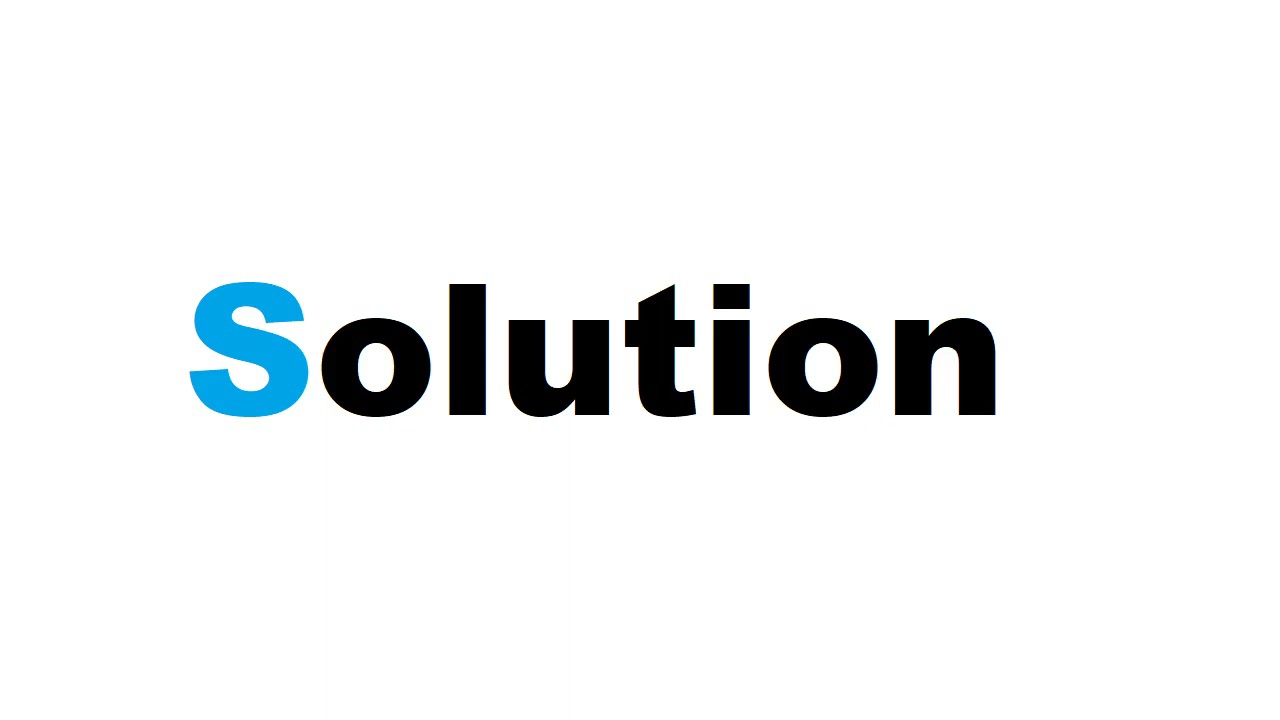

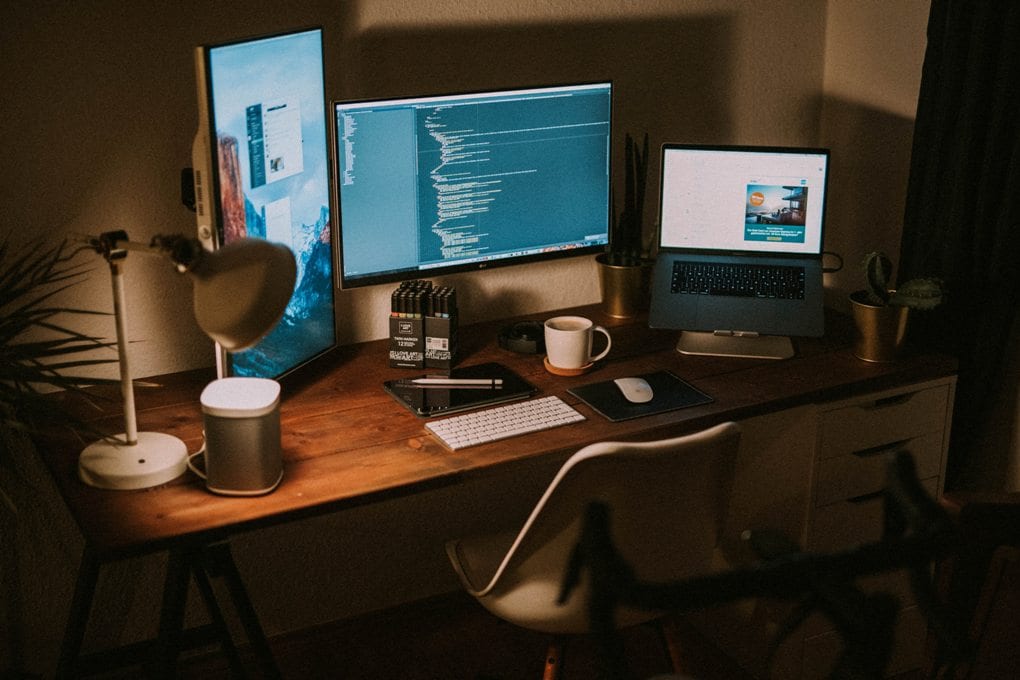
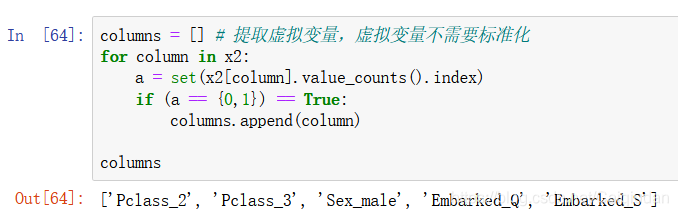


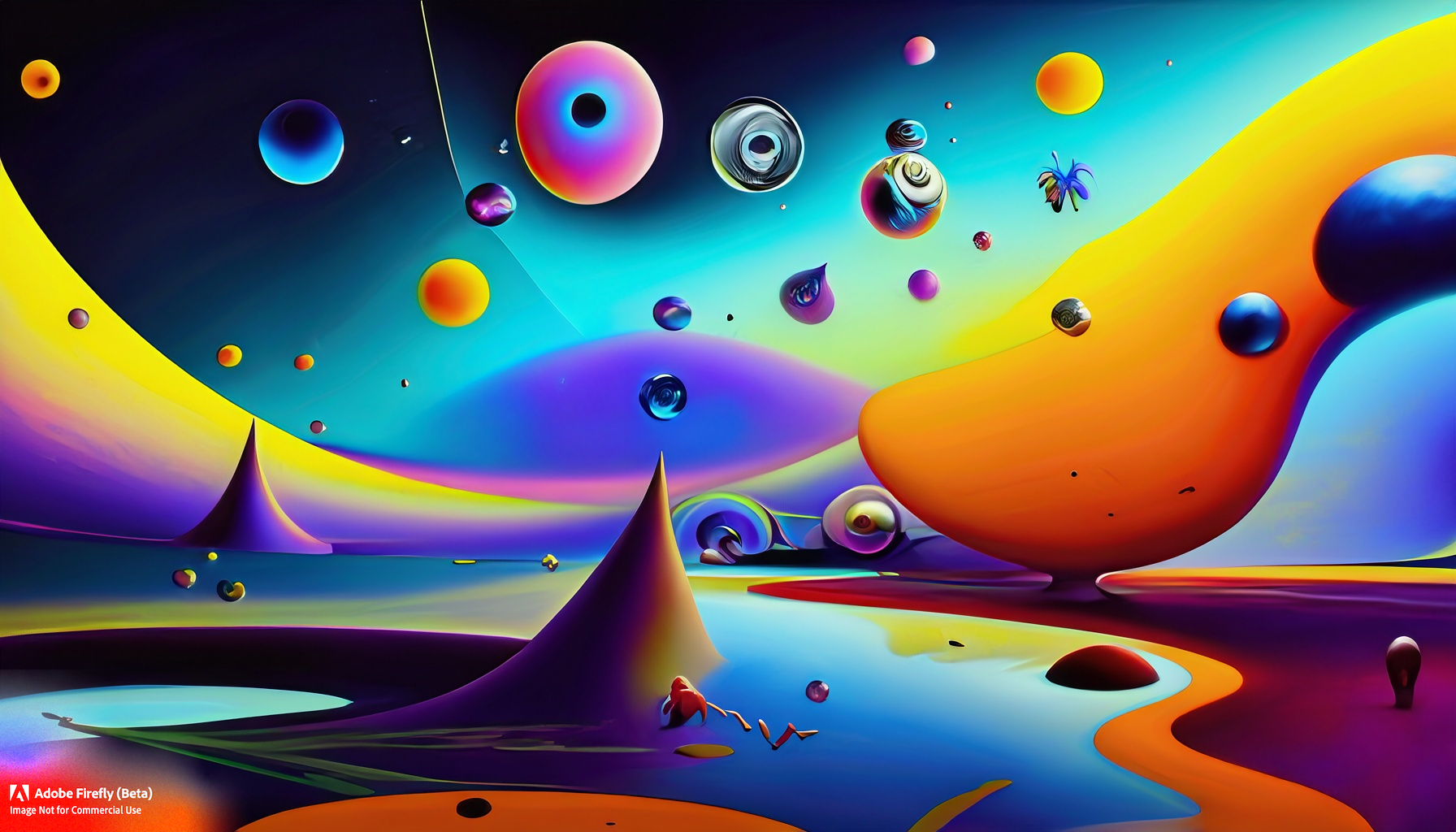


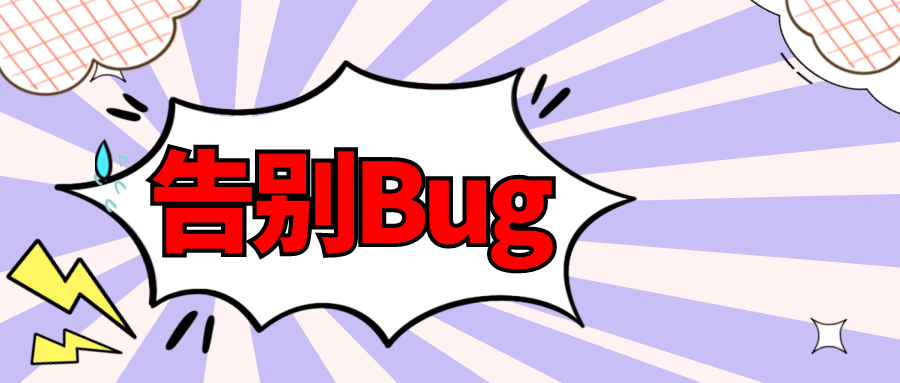
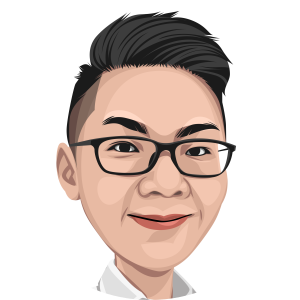


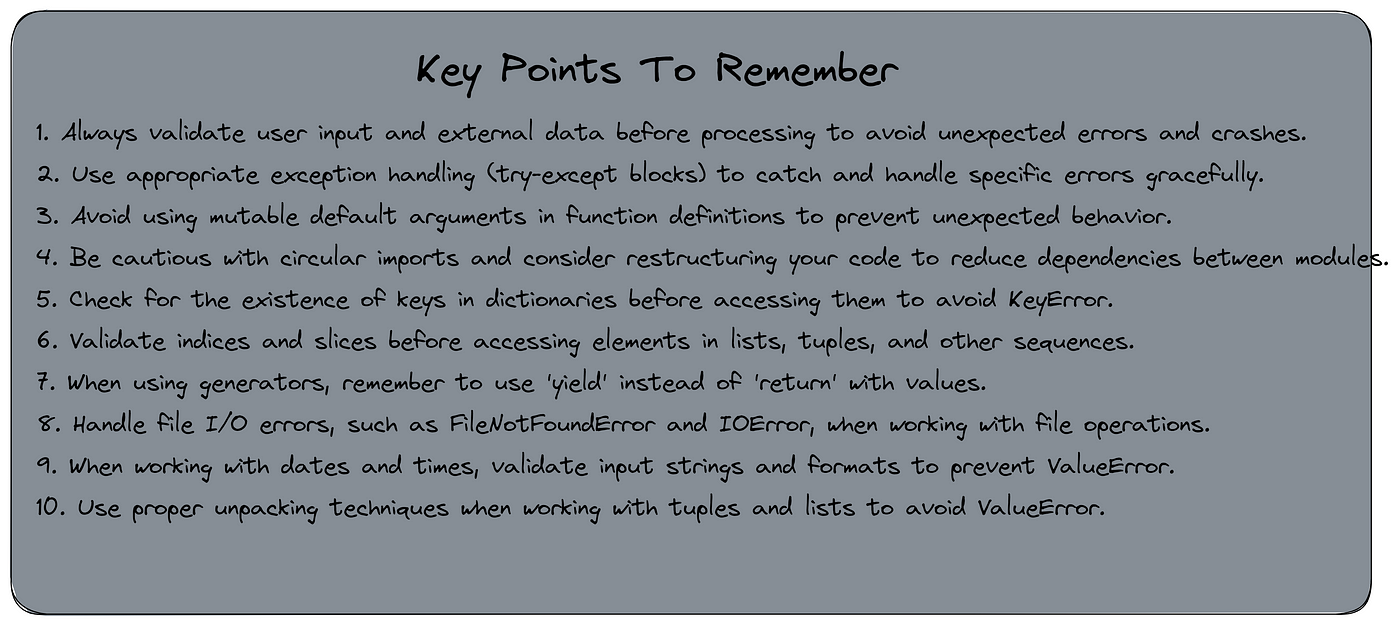
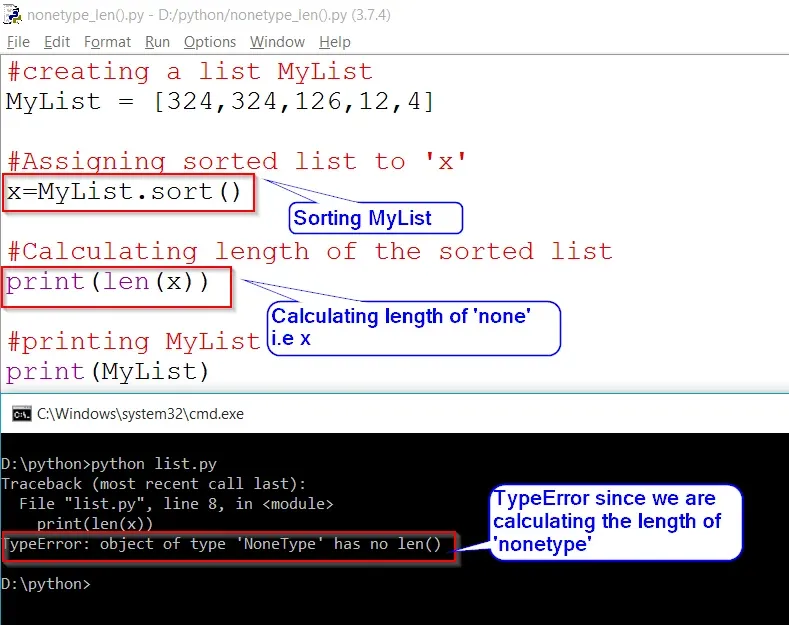
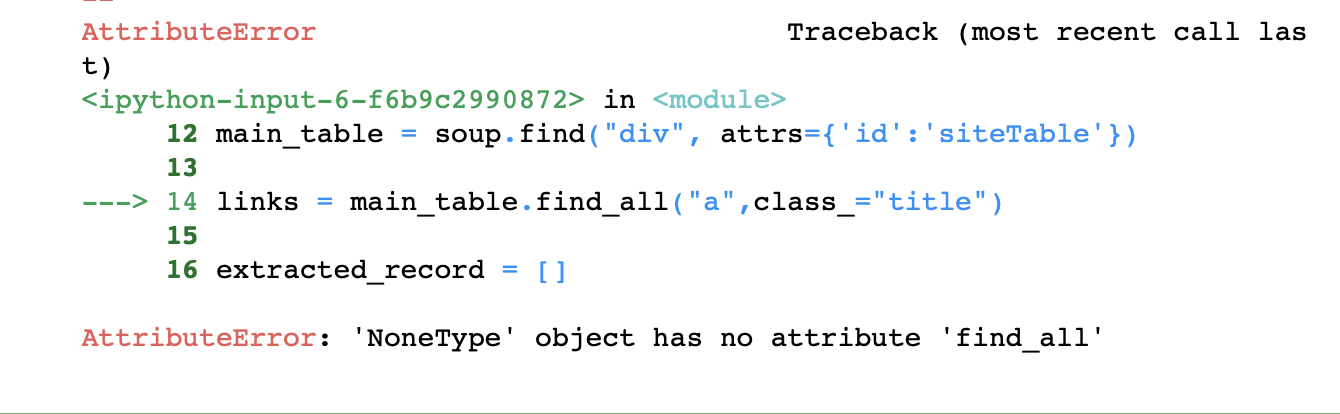

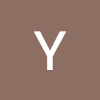


Article link: attributeerror: nonetype object has no attribute append.
Learn more about the topic attributeerror: nonetype object has no attribute append.
- AttributeError: ‘NoneType’ object has no attribute ‘append’
- appending list but error ‘NoneType’ object has no attribute …
- TypeError: ‘NoneType’ object has no attribute ‘append’
- AttributeError: ‘NoneType’ object has no attribute ‘append’
- AttributeError: ‘NoneType’ object has no attribute ‘append’
- Python’s .append(): Add Items to Your Lists in Place
- append() and extend() in Python – GeeksforGeeks
- Append in Python – How to Append to a List or an Array – freeCodeCamp
- AttributeError: ‘NoneType’ object has no attribute ‘append’
- AttributeError: ‘NoneType’ object has no attribute ‘append’
- 18/18 AttributeError: ‘NoneType’ object has no attribute ‘append’
- ‘NoneType’ object has no attribute ‘append’ Solution – velog
- Attributeerror nonetype object has no attribute append [Solved]
- NoneType Object Has No Attribute Append in Python