A Bytes Like Object Is Required Not Str
In the world of Python programming, the concepts of bytes and str are fundamental to working with data and text. These two data types may seem similar at first glance, but they have distinct differences that every developer should understand. This article will provide a comprehensive overview of bytes and str in Python, exploring their characteristics, use cases, and the important distinctions between them.
Understanding bytes in Python
In Python, bytes is a built-in data type used to represent a sequence of integer values ranging from 0 to 255. It is immutable, meaning that once a bytes object is created, its contents cannot be changed. Bytes objects are commonly used to handle binary data, such as reading and writing from files, network communications, and cryptographic operations.
To define a bytes object in Python, you can use the b prefix followed by single or double quotation marks. For example:
“`python
my_bytes = b’Hello World’
“`
Bytes objects have several characteristics and properties that make them suitable for handling binary data. They are typically stored as a sequence of bytes and can be indexed or sliced like other sequences in Python. Additionally, bytes objects have a fixed length and are represented in memory as a sequence of bytes, which makes them more efficient in terms of storage.
Understanding str in Python
On the other hand, str is the data type used to represent Unicode strings in Python. Unlike bytes objects, str objects are mutable, allowing you to modify their contents. Strings are widely used for working with text-based data, manipulating strings, and performing various string operations.
To define a str object in Python, you can enclose the text within single or double quotation marks. For example:
“`python
my_str = ‘Hello World’
“`
Str objects have similar characteristics to other sequence objects in Python, such as the ability to be indexed and sliced. However, unlike bytes objects, str objects can contain characters from multiple languages and scripts, making them more versatile for handling text data.
Comparison: bytes vs. str
The key differences between bytes and str lie in their storage and representation, encoding and decoding, and the impact on memory consumption and performance.
Bytes objects are stored as a sequence of bytes, which means they consume less memory compared to str objects. On the other hand, str objects store characters using Unicode encoding, which requires more memory to represent a wider range of characters.
Encoding and decoding also differ between bytes and str. Bytes objects can be encoded to str objects using a specified encoding scheme (e.g., UTF-8, ASCII), while str objects can be decoded to bytes using the same encoding scheme. This encoding and decoding process is necessary when working with different data formats or when transmitting data over different network protocols.
In terms of memory consumption and performance, bytes objects are more efficient for handling large amounts of binary data, as they have a fixed length and consume less memory. Str objects, on the other hand, are optimized for working with text-based data and provide a wide range of built-in string manipulation methods.
Working with bytes and str objects
When working with bytes objects in Python, you can create and manipulate them using various methods and operations. Some common methods include `len()`, `index()`, and `count()`. You can also perform bitwise operations and manipulate individual bytes within a bytes object.
Similarly, working with str objects involves creating and manipulating them using string methods and operations, such as `len()`, `concatenation`, `split()`, and `replace()`. Str objects also provide built-in methods for string formatting and manipulation.
Type conversions: bytes to str and vice versa
Converting between bytes and str objects is a common task in Python programming. To convert bytes to str, you can use the `decode()` method, specifying the desired encoding scheme. For example:
“`python
my_bytes = b’Hello World’
my_str = my_bytes.decode(‘utf-8’)
“`
To convert str to bytes, you can use the `encode()` method, again specifying the encoding scheme. For example:
“`python
my_str = ‘Hello World’
my_bytes = my_str.encode(‘utf-8’)
“`
It is important to handle potential errors and encoding/decoding issues when performing these conversions. Some characters may not be representable in certain encoding schemes, leading to loss of data or conversion errors. It is good practice to specify the appropriate encoding scheme based on the data you are working with.
Best practices for using bytes and str in Python
To effectively use bytes and str in Python, it is important to understand your data requirements and choose the appropriate data type accordingly. If you are working with binary data, such as files or network communications, bytes objects are more suitable. For text-based data manipulation, str objects should be used.
When handling encoding and decoding operations, always specify the desired encoding scheme explicitly to ensure consistent results. Avoid relying on default encoding settings, as they can vary across different systems and lead to unexpected behavior.
Additionally, be aware of common pitfalls and performance bottlenecks when working with bytes and str objects. For example, performing string concatenation using the `+` operator can be slower compared to using the `join()` method for str objects. Understanding these nuances can help optimize your code and improve overall performance.
Real-world examples and applications
To further illustrate the use cases of bytes and str in Python, let’s consider a few examples:
– When reading binary data from a file, such as images or audio files, using bytes objects ensures accurate representation of the data.
– In web applications, str objects are commonly used to handle user input and store textual data in databases.
– When working with network protocols, bytes objects are used for sending and receiving data over sockets.
Conclusion
In conclusion, understanding the differences between bytes and str in Python is crucial for effective data handling and manipulation. Bytes are used for binary data, while str is used for text-based data. Each data type has its own characteristics, use cases, and performance considerations. By following best practices and understanding the strengths of each data type, you can write more efficient and robust Python applications.
FAQs:
Q: What does the error message “A bytes-like object is required, not str” mean?
A: This error indicates that a function or operation expected a bytes object but received a str object instead. To resolve this issue, you can convert the str object to bytes using the `encode()` method.
Q: How do I convert a str object to bytes in Python?
A: You can convert a str object to bytes using the `encode()` method, specifying the desired encoding scheme. For example: `my_bytes = my_str.encode(‘utf-8’)`.
Q: How do I convert bytes to str in Python?
A: To convert bytes to str, you can use the `decode()` method, specifying the appropriate encoding scheme. For example: `my_str = my_bytes.decode(‘utf-8’)`.
Q: What should I consider when choosing between bytes and str in Python?
A: When choosing between bytes and str, consider the type of data you are working with. Use bytes for binary data, such as files or network communications, and use str for text-based data manipulation.
Q: How can I optimize performance when working with bytes or str objects?
A: To optimize performance, specify the desired encoding scheme explicitly when encoding or decoding, avoid relying on default encoding settings, and be aware of performance considerations when performing string operations or concatenation.
Q: Are there any limitations or potential errors when converting between bytes and str?
A: Yes, when converting between bytes and str, there can be limitations and potential errors. Some characters may not be representable in certain encoding schemes, leading to loss of data or conversion errors. It is important to handle these issues and choose the appropriate encoding scheme based on your data.
How To Fix Typeerror A Bytes-Like Object Is Required Not ‘Str’
Keywords searched by users: a bytes like object is required not str A bytes like object is required not str csv, A bytes like object is required not str split, P stdin write ls typeerror a bytes like object is required not str, typeerror: a bytes-like object is required, not ‘str’ socket, Decoding to str need a bytes-like object nonetype found, A bytes like object is required not str stable diffusion, Typeerror a bytes like object is required not uploadfile, String to byte Python
Categories: Top 25 A Bytes Like Object Is Required Not Str
See more here: nhanvietluanvan.com
A Bytes Like Object Is Required Not Str Csv
In the world of programming, CSV (Comma Separated Values) files provide a simple way to store tabular data. Whether you are working with spreadsheets, databases, or any other data storage system, CSV files serve as a universal format for easy data exchange. While dealing with CSV files, developers often come across an error stating “A bytes-like object is required, not str”. This error can be quite frustrating, especially for those who are new to programming or working with CSVs. In this article, we will dive deep into the causes of this error, the difference between bytes-like objects and strings, and explore potential solutions to tackle this issue effectively.
Understanding the error: “A bytes-like object is required, not str”
This error message typically occurs when trying to open a CSV file in a way that conflicts with the type of object expected by the function or method being used. In Python, the built-in `csv` module provides functionalities for parsing and writing CSV files. However, this module expects a bytes-like object as input, rather than a regular string object.
Difference between bytes-like objects and strings
In Python, a string is a sequence of characters, represented by the built-in `str` class. Strings are treated as a collection of Unicode characters. On the other hand, a bytes-like object represents a sequence of bytes, similar to a string but with some important differences. Bytes-like objects are represented by the built-in `bytes` or `bytearray` classes and are often used to handle binary data or byte-oriented operations.
CSV files often contain plain text data, encoded in ASCII or UTF-8 formats. While reading or writing CSV files, one needs to be aware of the encoding of the byte stream being used. Improper encoding can lead to errors such as the “A bytes-like object is required, not str” error.
Solutions to the error: “A bytes-like object is required, not str”
1. File opening modes:
When opening a CSV file using Python’s built-in `open` function, ensure that you use the appropriate file opening mode. The “w” mode is used for writing to a file, whereas the “r” mode is used for reading from a file. If you are trying to read a CSV file, use `open(filename, ‘rb’)` instead of `open(filename, ‘r’)`. The “b” in ‘rb’ indicates binary mode, which allows treating the file content as bytes rather than a string.
2. Encoding:
While opening a CSV file, specifying the encoding explicitly can eliminate the “A bytes-like object is required, not str” error. For instance, `open(filename, ‘rb’, encoding=’utf-8′)` ensures that the file is opened in binary mode with the desired encoding. Replace ‘utf-8’ with your specific encoding if necessary.
3. Convert to bytes-like object:
If you already have a string object, you can convert it to a bytes-like object using the `encode` method before passing it to the `csv` module. For example, `my_string.encode(‘utf-8’)` converts a string `my_string` to a bytes-like object with UTF-8 encoding.
FAQs: Fixing the “A bytes-like object is required, not str” error
1. Q: Why does the “A bytes-like object is required, not str” error occur only with CSV files?
A: The error is not specific to CSV files but can occur with any method or function that requires a bytes-like object while dealing with file I/O or binary data.
2. Q: Can I open a CSV file without specifying the encoding?
A: Although Python tries to guess the encoding if not specified, it is recommended to explicitly specify the encoding to avoid potential errors.
3. Q: How do I determine the encoding of a CSV file?
A: The encoding of a CSV file may not always be explicitly mentioned. You can use third-party libraries like `chardet` or examine the file metadata to make an informed guess about the encoding or use various encoding detection algorithms provided by these libraries.
4. Q: How can I convert a bytes-like object back to a string?
A: Use the `decode` method to convert a bytes-like object back to a string. For instance, `my_bytes.decode(‘utf-8’)` decodes a bytes-like object `my_bytes` with UTF-8 encoding.
In conclusion, when encountering the “A bytes-like object is required, not str” error while working with CSV files in Python, remember to ensure proper file opening modes, specify the encoding explicitly, and consider converting strings to bytes-like objects. Having a solid understanding of the difference between strings and bytes-like objects and implementing the suggested solutions will help you overcome this error and continue working with CSV files seamlessly.
A Bytes Like Object Is Required Not Str Split
When working with strings in Python, we often come across situations where we need to split them into smaller parts based on certain delimiters. The str.split() method is commonly used for this purpose. However, there are cases where using a bytes-like object instead of str.split() is necessary or more efficient. In this article, we will explore the concept of bytes-like objects, their advantages over str.split(), and how they can be utilized in various scenarios.
Understanding bytes-like objects
In Python, bytes-like objects are sequences of bytes that represent binary data. They can be created using the bytes() or bytearray() constructors, as well as other functions that support bytes-like objects, such as open(). Bytes-like objects are immutable, meaning their values cannot be changed once set. This immutability can be advantageous in certain situations where data integrity is crucial.
Advantages of bytes-like objects over str.split()
1. Efficient handling of binary data: bytes-like objects are specifically designed for binary data, making them efficient when dealing with such content. They provide a convenient way to manipulate binary data without the overhead associated with string operations.
2. Memory efficiency: bytes-like objects occupy less memory compared to their string counterparts. This is because they represent raw binary data and do not require additional encoding and decoding mechanisms utilized by strings. Consequently, for large datasets or resource-constrained environments, using bytes-like objects can significantly reduce memory footprint.
3. Compatibility with various protocols: Many network protocols, file formats, and data structures operate on bytes rather than strings. By using bytes-like objects, you can directly manipulate and parse protocol-specific binary data. This compatibility is essential when performing tasks like parsing headers, network packet inspection, or reading binary files.
4. Enhanced performance: The internal representation and operations performed on bytes-like objects are optimized for binary data. This intrinsic optimization results in faster execution times for binary-oriented operations, such as searching, pattern matching, and byte-wise transformations.
Utilizing bytes-like objects in various scenarios
1. Parsing byte-oriented protocols: It is common to encounter protocols like TCP/IP, UDP, or HTTP, which rely on binary data structures. By using bytes-like objects, you can efficiently extract relevant fields from such packets. This approach enhances performance and reduces the risk of incorrect data interpretation due to encoding issues.
2. File manipulation: When reading or writing binary files, bytes-like objects provide a straightforward and efficient way to handle and process the data. For example, you can read a file as a bytes object, manipulate it using various byte-wise operations, and then write the modified bytes to the output file.
3. Encoding and decoding data: Some encoding schemes, like base64, work on bytes rather than strings. By utilizing bytes-like objects, you can effortlessly encode or decode data without the need for intermediate string conversions.
FAQs:
Q: Can I convert a string into a bytes-like object?
A: Yes, you can convert a string into a bytes-like object using the encode() method. For example, “Hello, world!”.encode() will give you a bytes-like object representing the string.
Q: Are bytes and bytes-like objects the same thing?
A: No, bytes is a built-in type in Python, and it is a synonym for bytes-like objects. Bytes-like objects are more general and can also include bytearray objects.
Q: What is the difference between bytes and strings?
A: Strings are used for representing textual data in Python, while bytes or bytes-like objects are used for binary data. Strings are immutable sequences of characters, while bytes and bytes-like objects are immutable sequences of bytes.
Q: Can I use bytes-like objects in regular string operations like concatenation or slicing?
A: No, bytes-like objects are not compatible with regular string operations. If you need to manipulate a bytes-like object, you should use bytes-specific methods like slicing with brackets or using the bytearray type if you need mutable bytes.
In conclusion, understanding when to use a bytes-like object instead of str.split() can greatly enhance the efficiency and performance of your Python code, especially when dealing with binary data. By leveraging the advantages of bytes-like objects, you can efficiently handle binary content, reduce memory consumption, ensure compatibility with various protocols, and optimize performance in specific use cases.
P Stdin Write Ls Typeerror A Bytes Like Object Is Required Not Str
Introduction (75 words)
——————————————————
Python is a widely-used programming language known for its simplicity and versatility. However, beginners often encounter perplexing error messages that can hinder their progress. One such error, “TypeError: a bytes-like object is required, not ‘str'”, commonly occurs when using the `Popen` function from the `subprocess` module. This article aims to provide a detailed explanation of this error message, explore its causes, and offer potential solutions to help Python programmers overcome it.
Understanding “TypeError: a bytes-like object is required, not ‘str'” (250 words)
——————————————————
The “TypeError: a bytes-like object is required, not ‘str'” message indicates that a function or method requires a bytes-like object as an argument, instead of a string. This error is most frequently encountered in the context of the `subprocess.Popen` class, where passing a string as an argument raises the exception.
The rationale behind this requirement is related to the concept of character encoding. In Python, strings are represented as Unicode by default, while bytes represent raw binary data. When using the `subprocess.Popen` function, it expects arguments to be in bytes format to avoid conflicts or corruption when dealing with non-textual data.
Often, this error arises when attempting to pass a command to be executed within the shell as a string value, instead of converting it to bytes. To overcome this, the command can be encoded using various string encoding methods such as `.encode()`.
For example, consider the following code snippet:
“`python
import subprocess
command = “ls”
command_bytes = command.encode()
proc = subprocess.Popen(command_bytes, shell=True)
“`
In the above code, the `command` string is encoded into the `command_bytes` variable using the `.encode()` method. This ensures that the argument passed to `Popen` method is in the required bytes-like format, avoiding the “TypeError: a bytes-like object is required, not ‘str'” error.
Other similar scenarios that may cause this issue include working with file I/O streams in binary mode, using certain operating system-specific functions or libraries, or when dealing with network communications that require binary data.
FAQs (529 words)
——————————————————
Q1. Why does Python require bytes-like objects instead of strings in certain cases?
A: The requirement for bytes-like objects instead of strings in certain situations is intended to ensure proper handling of non-textual data, avoiding encoding or decoding issues. This is particularly important when interacting with external processes, binary data, or system calls where the distinction between binary and textual data is critical.
Q2. How can I determine the encoding of the input string before converting it into bytes?
A: Python provides the `sys.getdefaultencoding()` method to retrieve the default string encoding. Additionally, tools like `chardet` and `charset-normalizer` can be used to detect the encoding of a given string.
Q3. Are there any alternative methods to circumvent the bytes-like object requirement?
A: While the best practice is to convert the string to bytes using the `.encode()` method, some Python libraries and modules offer alternative solutions. For instance, the `subprocess.run()` function can accept strings as arguments, automatically handling the encoding internally.
Q4. Why does the `subprocess` module enforce the use of bytes instead of strings?
A: This design choice aims to maintain consistency, as various libraries and system calls may assume or require data in a specific format. The use of bytes instead of strings eliminates any ambiguity related to string encodings and guarantees conformity when interacting with different processes.
Q5. Can I always use `.encode()` to convert strings to bytes without any issues?
A: While `.encode()` is the most common method, it is important to consider the encoding used. Different platforms and systems might have different default encodings. It is good practice to explicitly specify the desired encoding through the `encoding` parameter of the `.encode()` method to ensure consistent behavior.
Q6. What are some commonly-used encodings in Python?
A: Some popular encodings in Python include UTF-8, ASCII, ISO-8859-1 (Latin-1), and UTF-16.
Q7. Can I convert bytes to strings if needed?
A: Yes, Python provides the `.decode()` method to convert bytes-like objects back into strings. Similar to encoding, explicit specification of the decoding scheme is recommended to avoid ambiguities.
Q8. Are there any risks associated with neglecting this error and passing strings instead of bytes?
A: Neglecting this error can result in unexpected behavior, data corruption, or security vulnerabilities due to incorrect assumptions made by other modules or processes.
Conclusion (300 words)
——————————————————
The “TypeError: a bytes-like object is required, not ‘str'” error is a common pitfall encountered by novice Python programmers. This error message is often observed when passing strings as arguments to certain functions or libraries that require bytes-like objects. By understanding the underlying reasons for this requirement, developers can avoid such errors and write clearer, more robust code.
This article has explored the causes of the “TypeError: a bytes-like object is required, not ‘str'” error and provided potential solutions. By converting strings into bytes-like objects using the `.encode()` method, Python programmers can ensure compatibility between strings and functions that demand bytes for proper execution.
Additionally, the FAQs section addressed common queries regarding this error, delving into encoding determinations, alternative methods, implications of neglecting the error, and more.
As you progress in your journey as a Python programmer, mastering this error will enhance your ability to work with subprocesses, binary data, and networking more effectively. Remember to always consider the encoding requirements of the context you are working in, and leverage the wide range of Python’s string manipulation and encoding capabilities to write clean and efficient code.
Images related to the topic a bytes like object is required not str
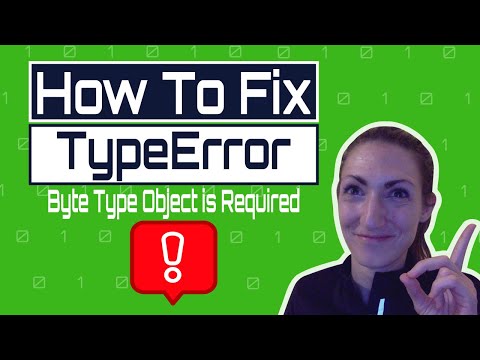
Found 41 images related to a bytes like object is required not str theme
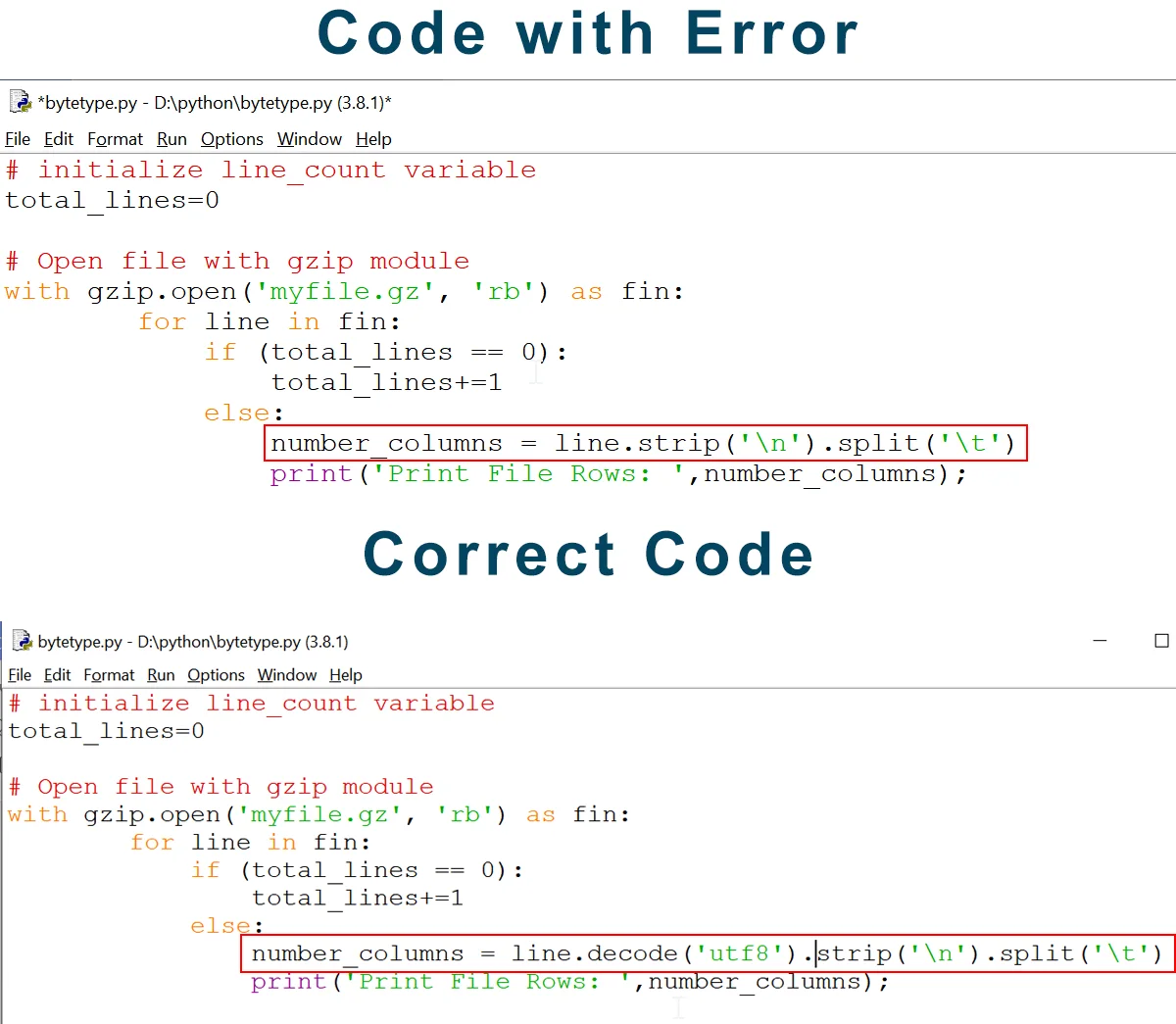
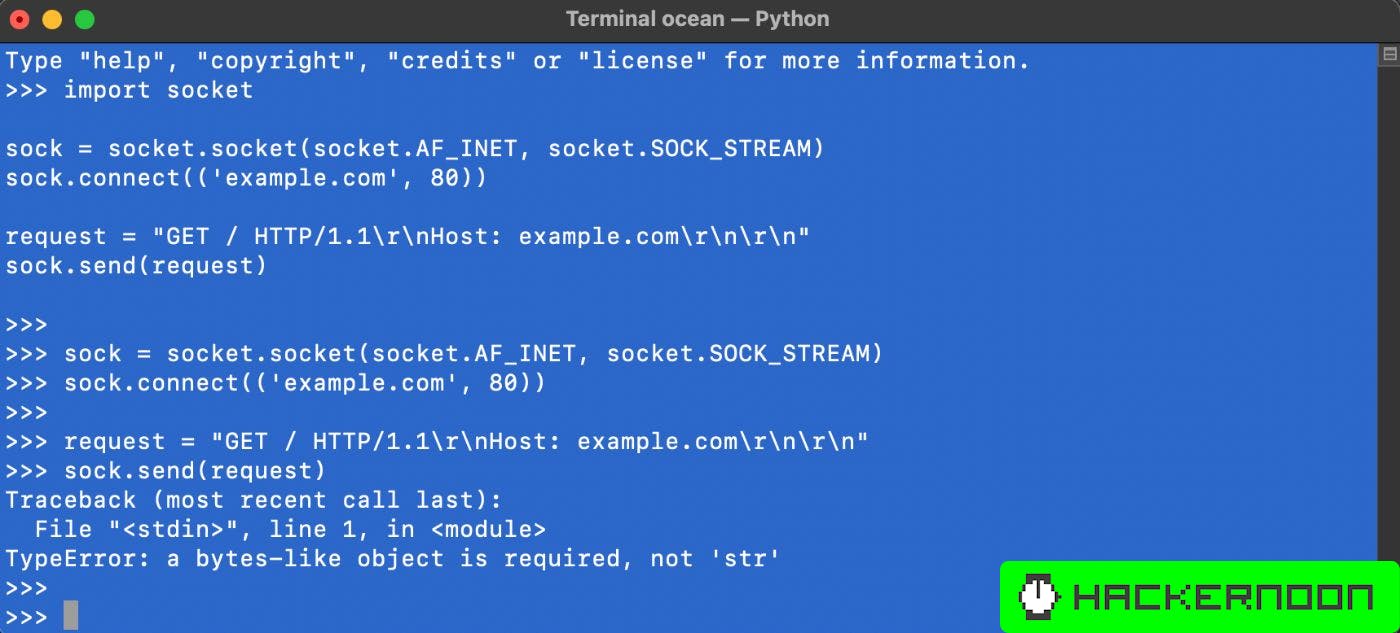
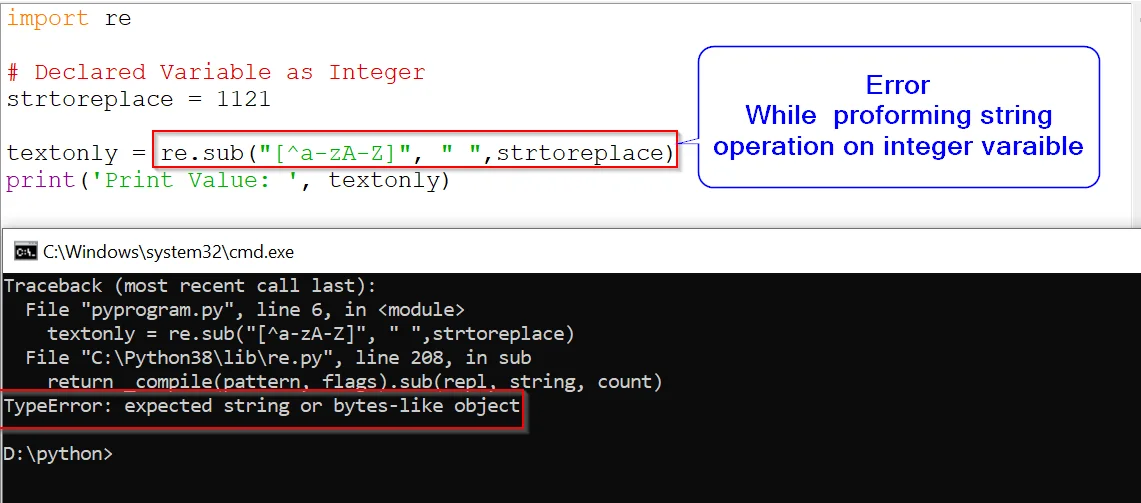

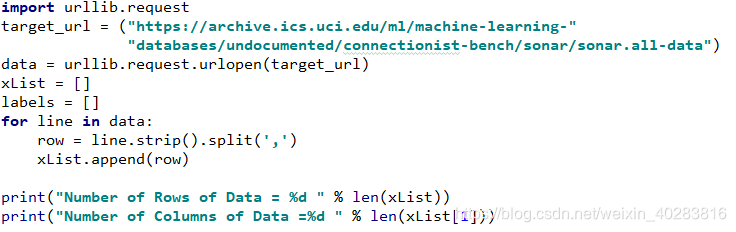
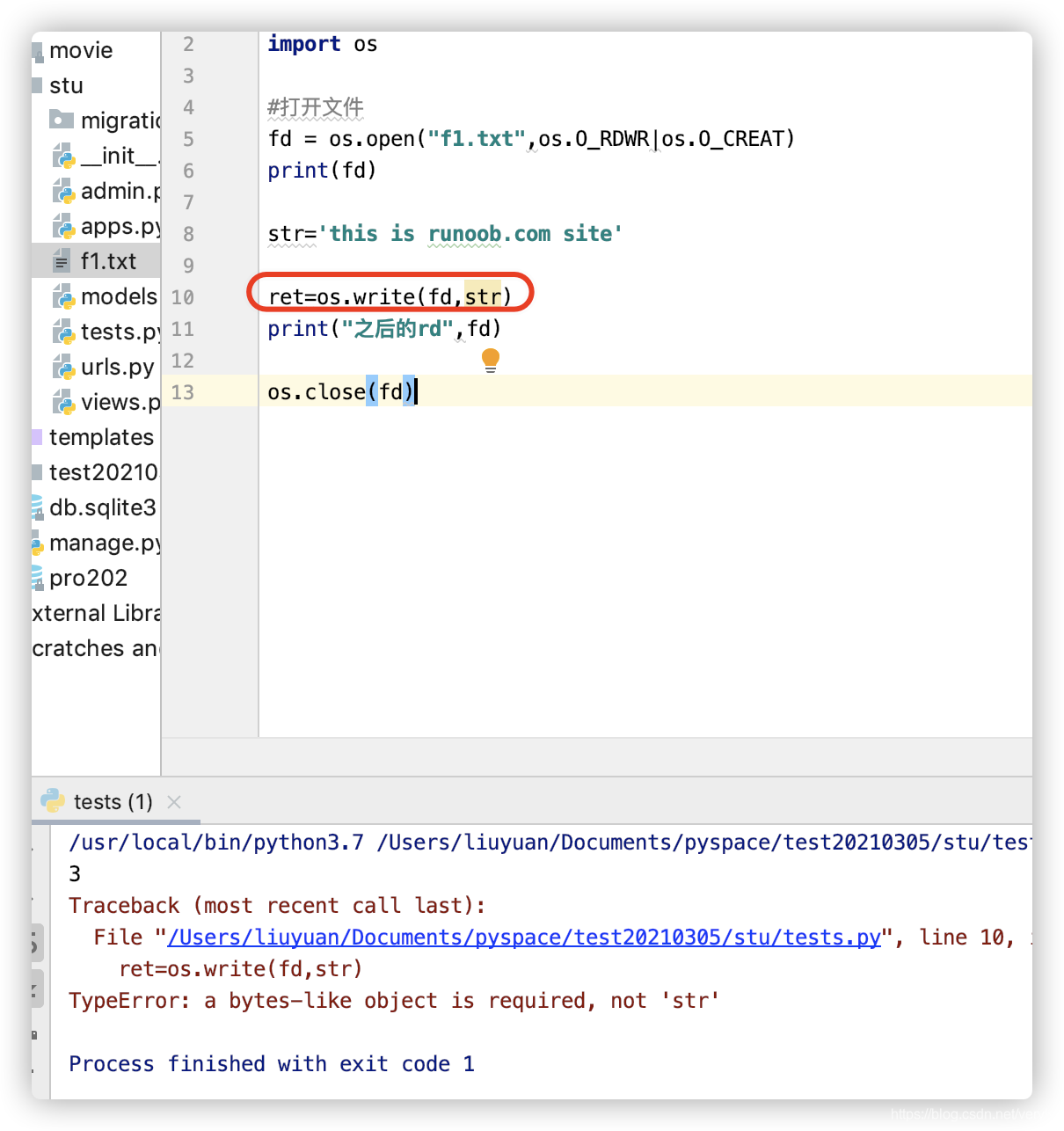

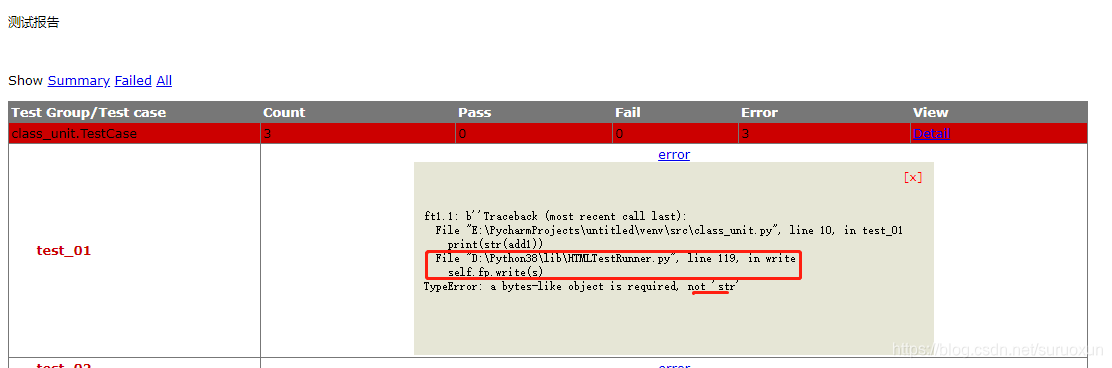

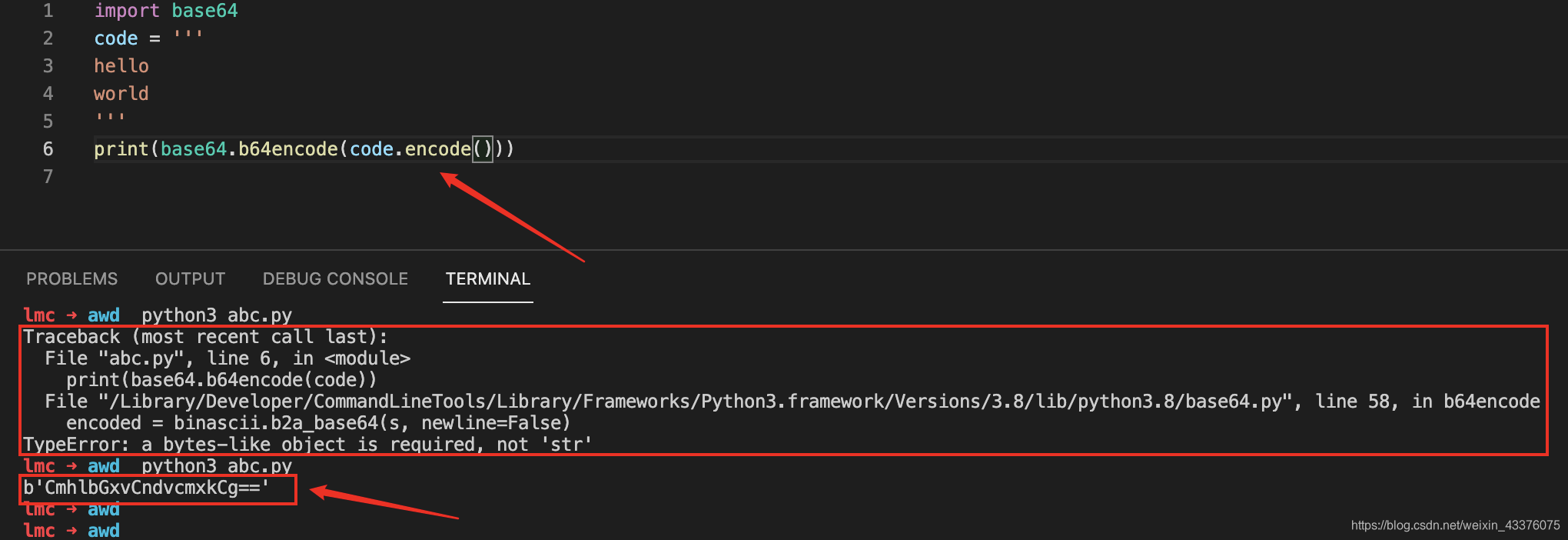
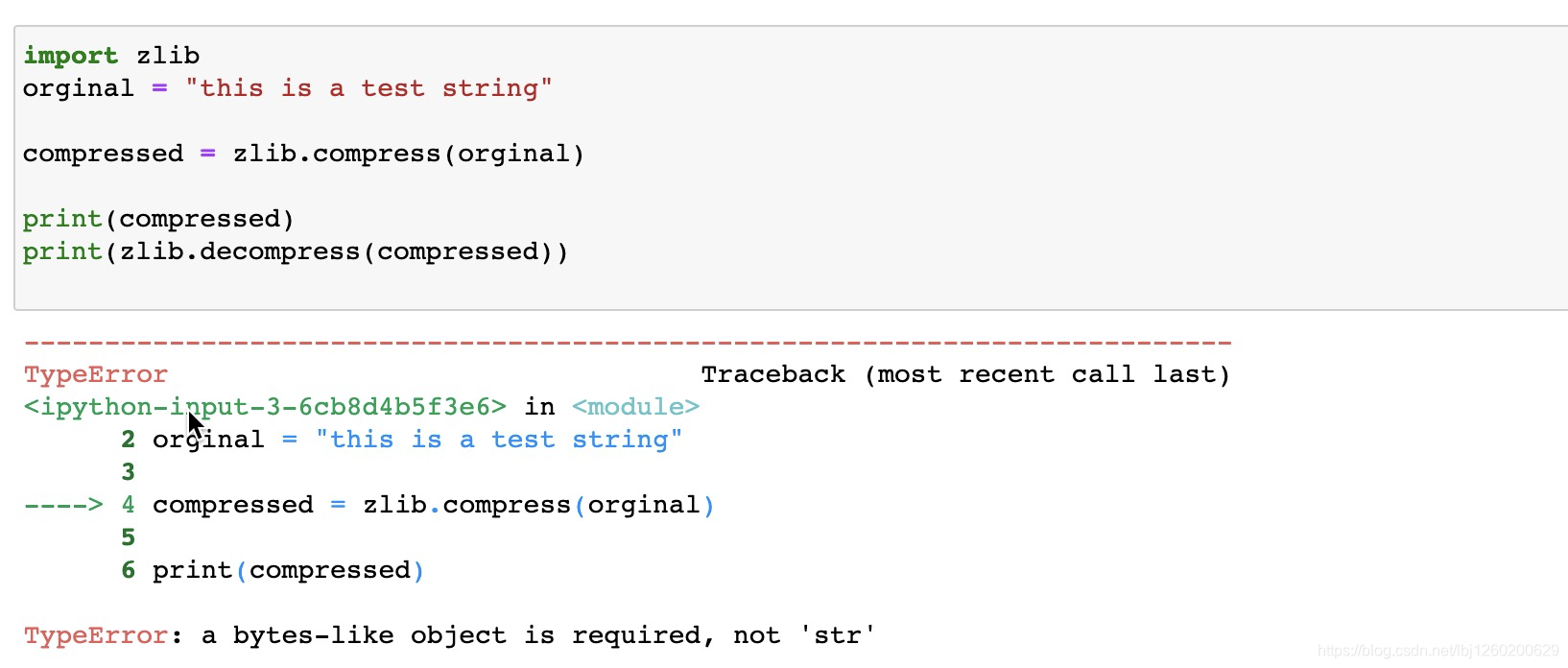

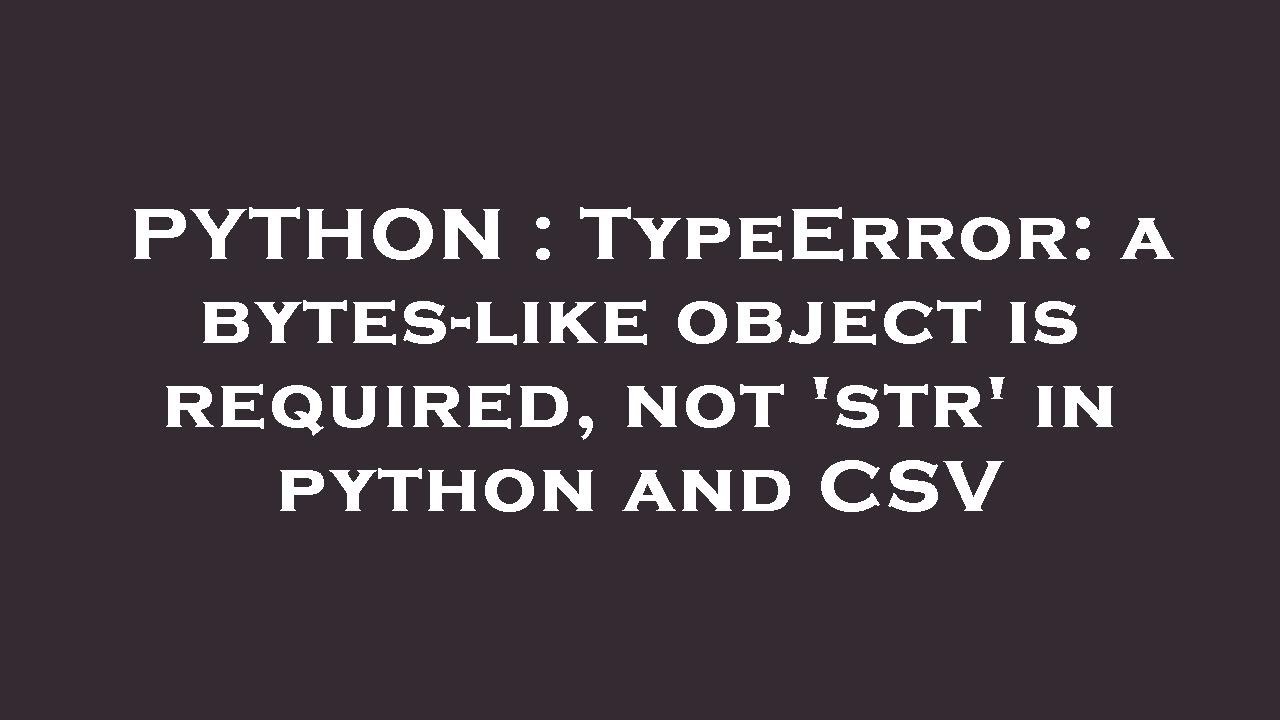
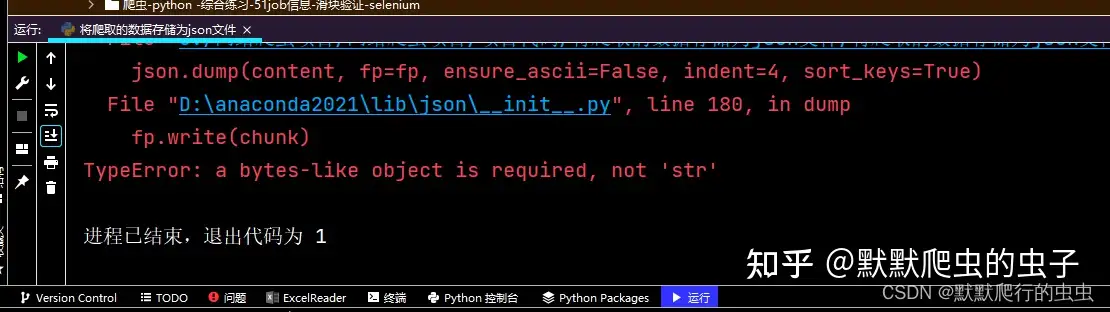
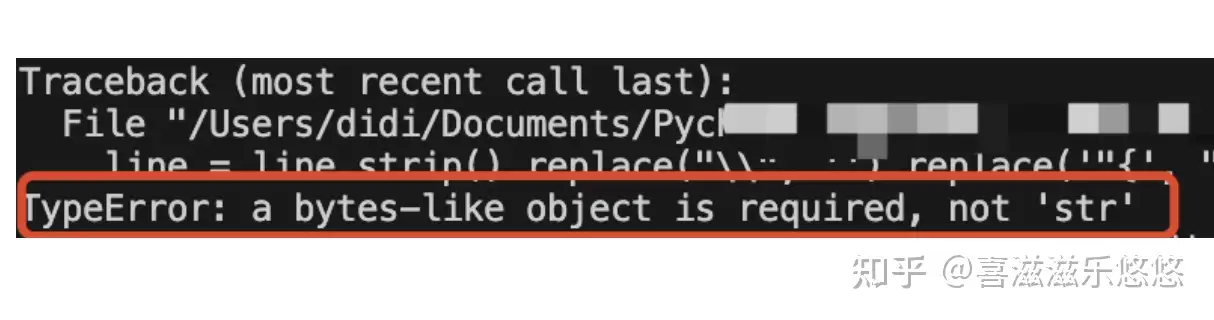

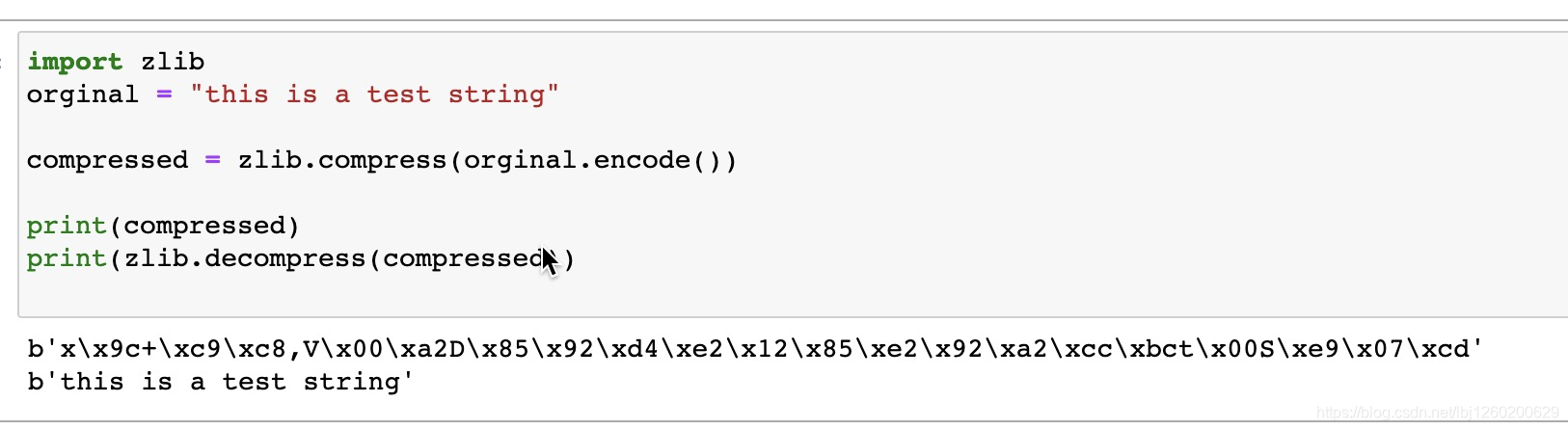
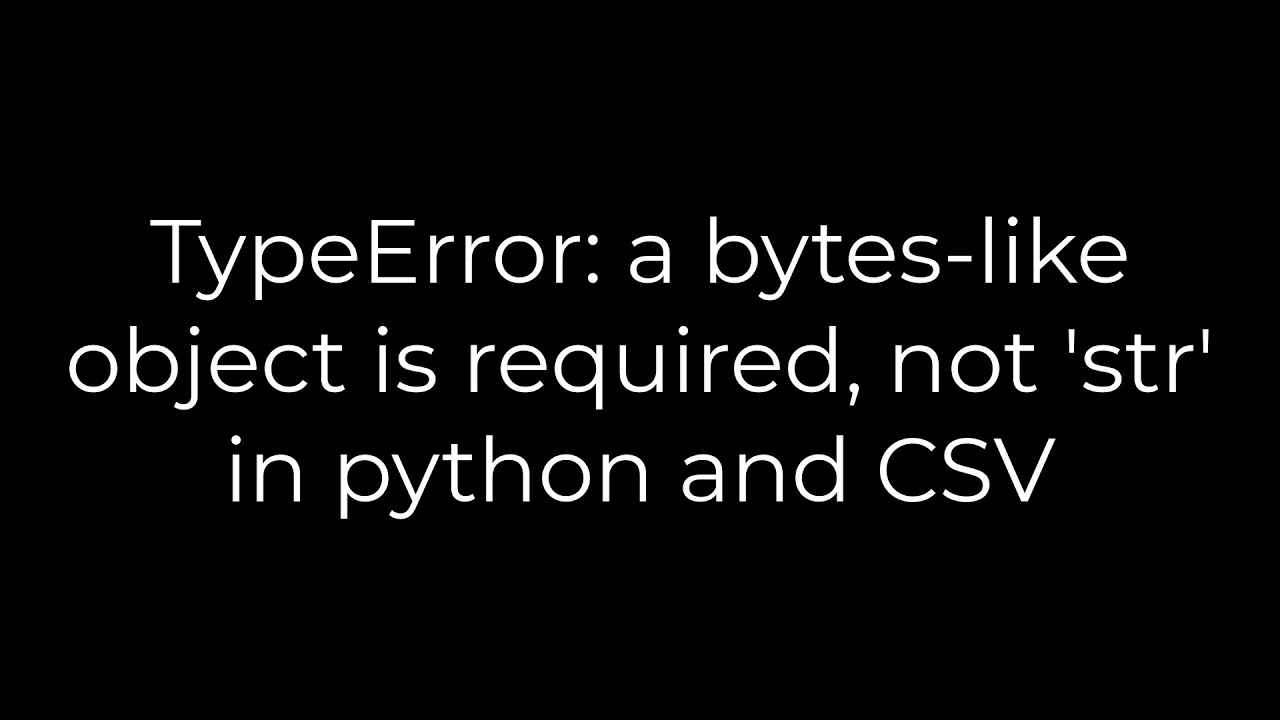
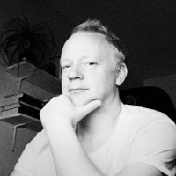
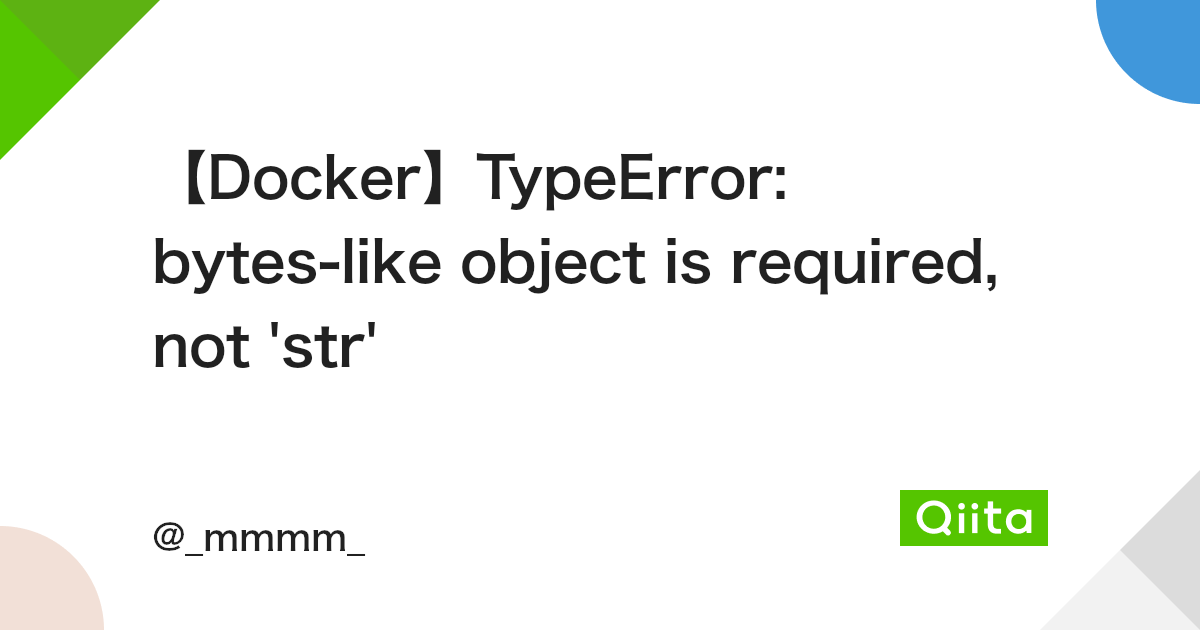
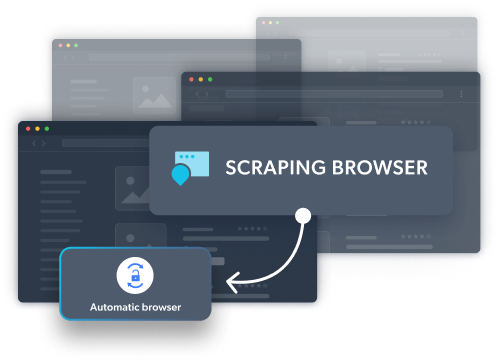
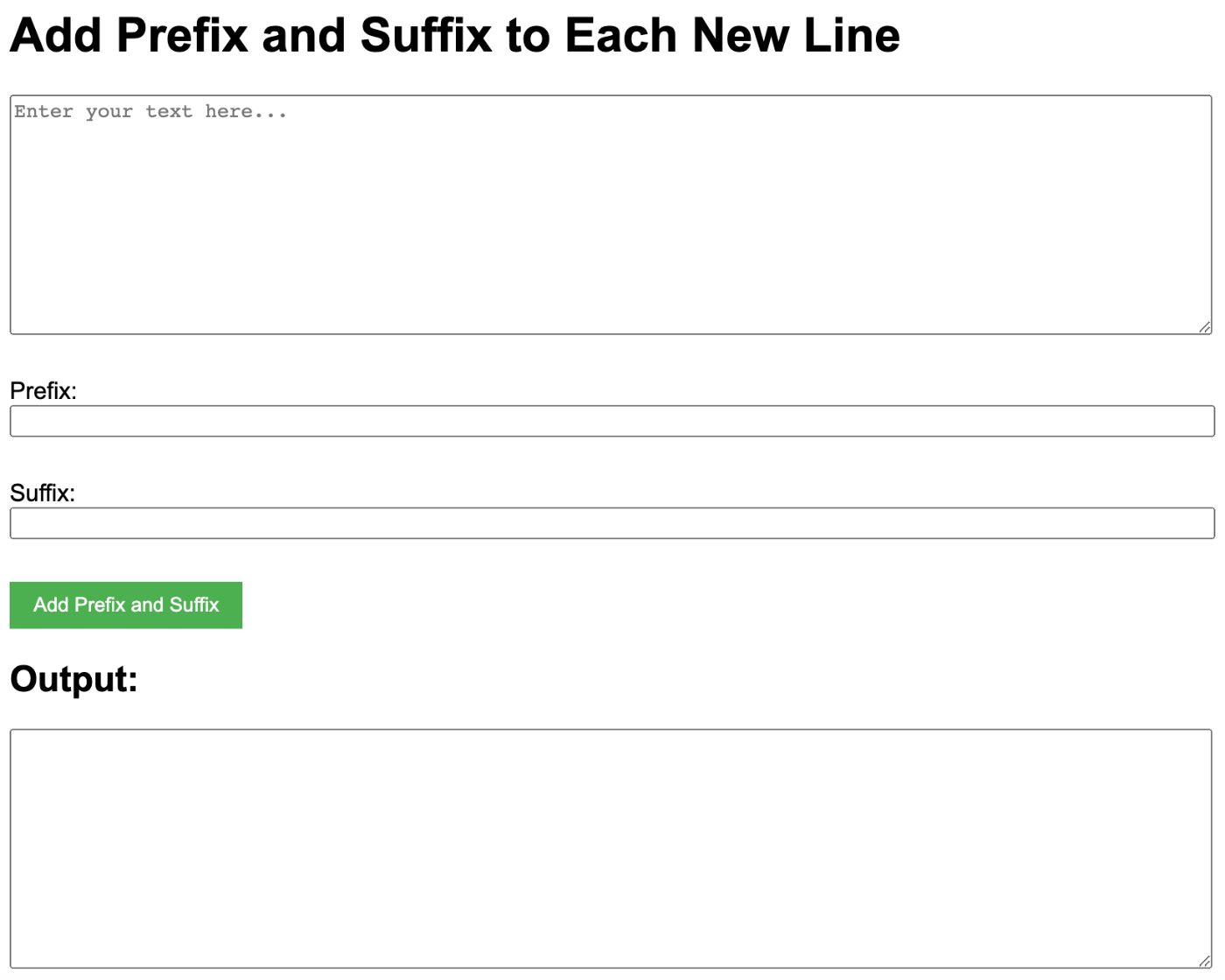
![Typeerror a bytes like object is required not str [SOLVED] Typeerror A Bytes Like Object Is Required Not Str [Solved]](https://itsourcecode.com/wp-content/uploads/2023/04/4.png?ezimgfmt=rs:352x204/rscb35/ngcb34/notWebP)
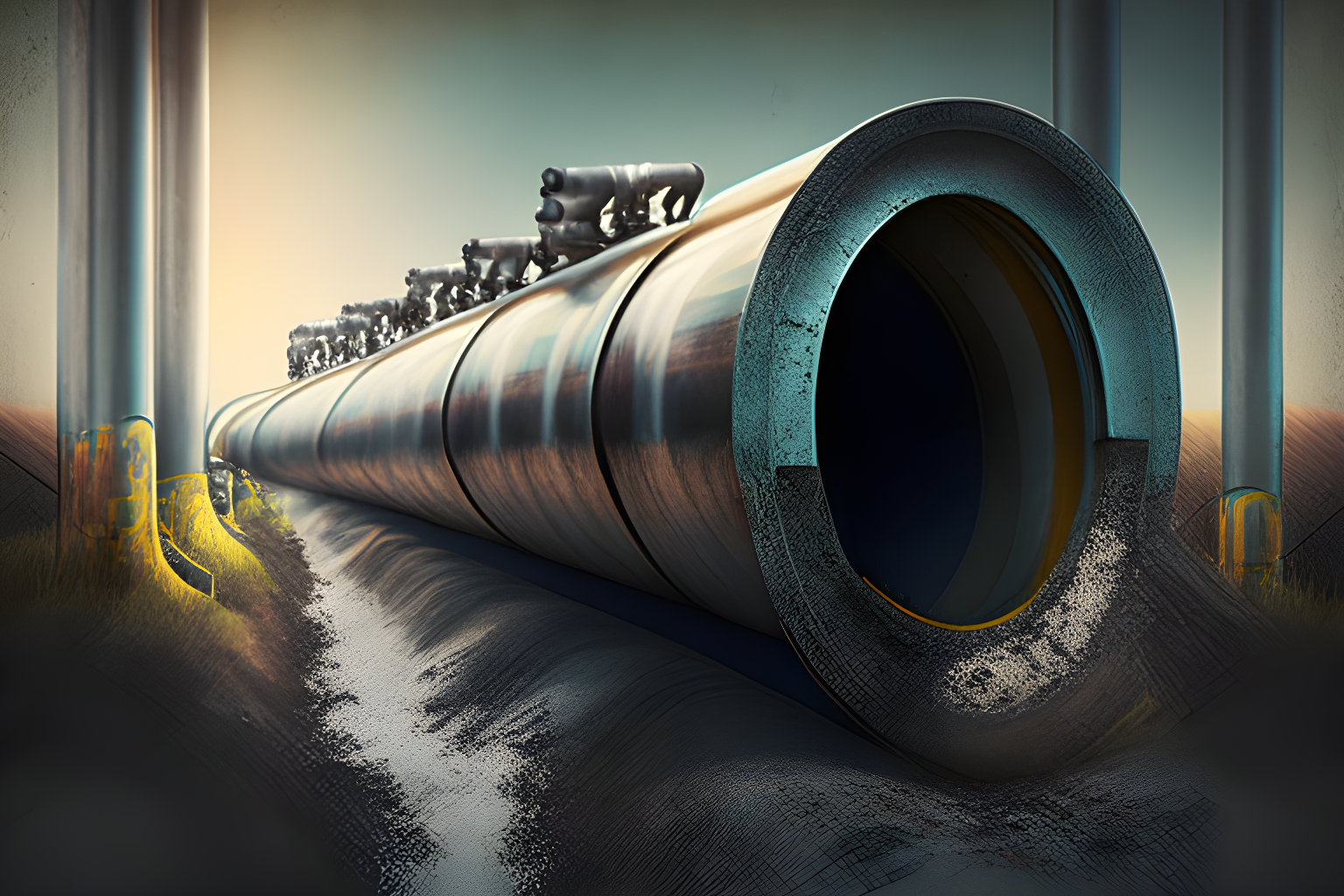



![Solved] Authentication throwing an error - Dash Python - Plotly Community Forum Solved] Authentication Throwing An Error - Dash Python - Plotly Community Forum](https://global.discourse-cdn.com/business7/uploads/plot/original/2X/f/fceb5d365fb0694be0b818d93ca03f6c1ff02bde.png)

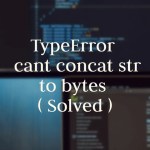
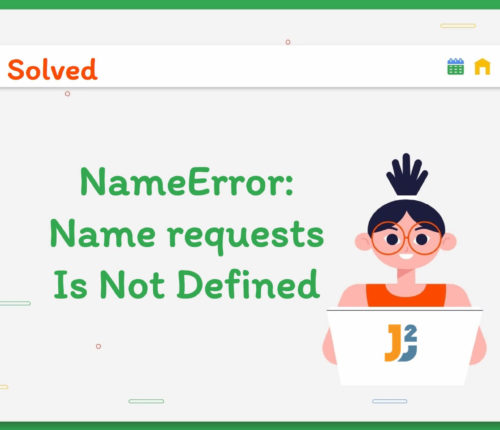
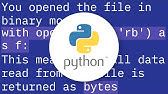
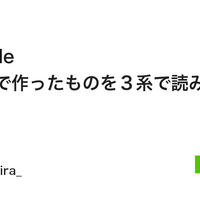
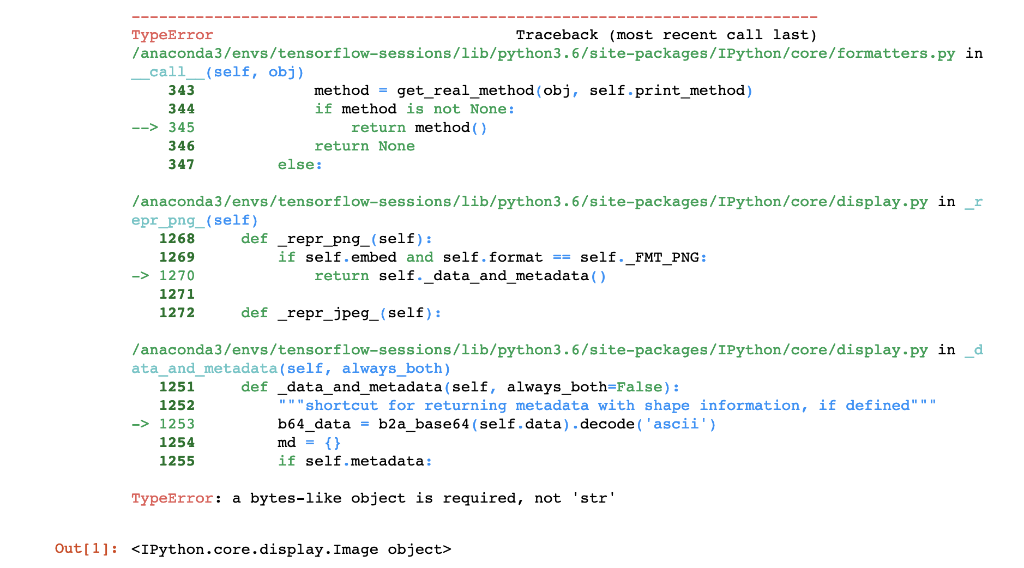
![Python] TypeError: a bytes-like object is required, not 'str' Python] Typeerror: A Bytes-Like Object Is Required, Not 'Str'](https://img1.daumcdn.net/thumb/C176x176/?fname=https://blog.kakaocdn.net/dn/b9sgYH/btrGt1kyhVC/IDAy6PxMUdQdhK06icbcuK/img.png)
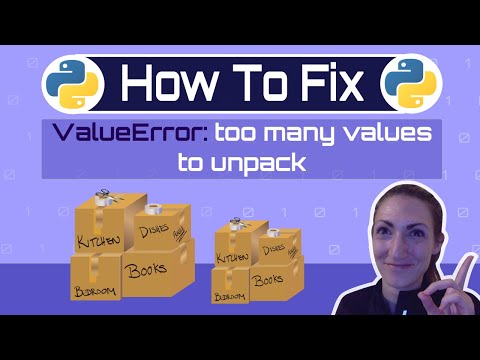


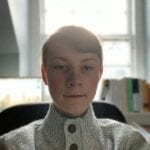
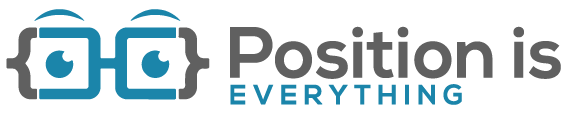

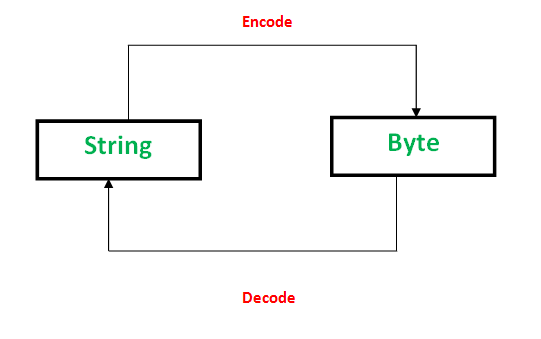
![Fixed] Expected str bytes or os.pathlike object Error - Python Pool Fixed] Expected Str Bytes Or Os.Pathlike Object Error - Python Pool](https://www.pythonpool.com/wp-content/uploads/2023/01/expected-str-bytes-or-os.pathlike-object.webp)
Article link: a bytes like object is required not str.
Learn more about the topic a bytes like object is required not str.
- a bytes-like object is required, not ‘str'” when handling file …
- Fix the typeerror a bytes like object is required not str in Python
- TypeError: a bytes-like object is required, not ‘str’ – bobbyhadz
- Resolving TypeError: A Bytes-like Object is Required, Not ‘str …
- Typeerror a bytes like object is required not str : How to Fix?
- TypeError: a bytes-like object is required, not ‘str’ when writing …
- How to Fix Typeerror a bytes-like object is required not ‘str’
- typeerror: a bytes-like object is required, not ‘str’ – STechies
- Python typeerror: a bytes-like object is required, not ‘str’ Solution
- a bytes-like object is required, not ‘str’ | Generating PDF Problem
See more: nhanvietluanvan.com/luat-hoc