Websocket Sendasync Example C#
WebSocket is a communication protocol that enables full-duplex communication between a client and a server over a single, long-lived connection. Unlike traditional HTTP polling methods, WebSocket allows real-time data transfer, making it more efficient for web applications that require instant updates.
WebSocket SendAsync Method
The SendAsync method is a key feature of the WebSocket protocol. It allows asynchronous sending of messages from the client to the server, improving performance and responsiveness in web applications. By using SendAsync, developers can send data without blocking the main application thread, ensuring a smooth user experience.
Establishing a WebSocket Connection
To establish a WebSocket connection, both the client and the server must follow the WebSocket protocol. The process involves performing a handshake, where the client sends an Upgrade request and the server responds with an Upgrade response. This handshake ensures compatibility between the client and the server for WebSocket communication.
Creating a WebSocket Client in C#
In C#, you can create a WebSocket client using the System.Net.WebSockets namespace. By initializing a ClientWebSocket object and connecting it to a WebSocket server, you can establish a WebSocket connection and send messages using the SendAsync method.
Sending a Message
To send a message using the WebSocket SendAsync method in C#, you need to convert the message to a byte array and wrap it in a WebSocketMessage object. Using the Encoding class, you can convert the message to bytes and then call the SendAsync method to send the message over the WebSocket connection.
Handling SendAsync Completion
After calling the SendAsync method, it’s important to handle the completion of the operation. The SendAsync method returns a Task object that can be awaited or used with other asynchronous programming techniques to handle the successful or failed sending of the message. Implementing error handling and retry mechanisms can help manage message sending failures.
Handling WebSocket Exceptions
Exception handling is crucial when using the WebSocket SendAsync method. Network issues, server errors, or other unforeseen circumstances can lead to exceptions. By implementing proper exception handling, you can gracefully handle these situations and provide appropriate feedback to the user.
WebSocket SendAsync Best Practices
To ensure efficient and reliable message sending using the WebSocket protocol, it is recommended to follow some best practices. These include managing WebSocket connections and their lifecycles effectively, optimizing message serialization and deserialization, using message compression techniques for large payloads, and monitoring network latency and performance to identify bottlenecks or issues.
WebSocket SendAsync Alternatives
While the SendAsync method offers convenient asynchronous message sending over a WebSocket connection, there might be situations where alternative approaches are preferred. Some alternatives include using the Send method for synchronous message sending or utilizing third-party libraries or frameworks that provide higher-level abstractions for WebSocket communication.
FAQs
1. How does WebSocket differ from traditional HTTP polling methods?
WebSocket enables full-duplex communication between a client and a server, allowing real-time data transfer over a single, long-lived connection. This is more efficient than traditional HTTP polling methods that involve repeated requests from the client to the server to check for updates.
2. Can a WebSocket connection be established with any server?
No, both the client and the server must adhere to the WebSocket protocol to establish a WebSocket connection. This involves performing a handshake to ensure compatibility between the client and the server.
3. Can I send messages synchronously using WebSocket?
Yes, besides the SendAsync method, WebSocket also provides a Send method for synchronous message sending. However, asynchronous sending using SendAsync is generally recommended for better performance and responsiveness in web applications.
4. How can I handle exceptions that occur during WebSocket communication?
It’s important to implement exception handling when using the WebSocket SendAsync method. Network issues, server errors, or other unforeseen circumstances can lead to exceptions. By handling exceptions, you can gracefully handle these situations and provide appropriate feedback to the user.
5. Are there any best practices for sending messages using WebSocket?
To ensure efficient and reliable message sending, it is recommended to manage WebSocket connections effectively, optimize message serialization and deserialization, use compression techniques for large payloads, and monitor network latency and performance to identify and resolve any issues.
6. Are there alternatives to using the SendAsync method?
Yes, while SendAsync is a convenient method for sending messages asynchronously over a WebSocket connection, there might be situations where alternative approaches are preferred. Some alternatives include using the Send method for synchronous message sending or utilizing third-party libraries or frameworks that provide higher-level abstractions for WebSocket communication.
By following these guidelines and best practices, developers can effectively utilize the WebSocket SendAsync method in C# and ensure efficient and responsive communication between clients and servers.
How To Use Websockets – Javascript Tutorial For Beginners
Keywords searched by users: websocket sendasync example c# clientwebsocket c# example, websocket in asp net mvc, c# websocket server send message to client, signalr websocket example, c# websocket send json, c# secure websocket client example, httpcontext websockets acceptwebsocketasync, websocket receiveasync buffer size
Categories: Top 21 Websocket Sendasync Example C#
See more here: nhanvietluanvan.com
Clientwebsocket C# Example
Introduction to ClientWebSocket:
WebSocket is a communication protocol that provides full-duplex communication over a single TCP connection, allowing data to be sent and received simultaneously. Unlike traditional HTTP, WebSocket provides a persistent connection, reducing overhead and enabling real-time communication between a client and a server.
ClientWebSocket is a class available in C# that simplifies the WebSocket client implementation. It is part of the System.Net.WebSockets namespace and supports both .NET Framework and .NET Core.
Usage of ClientWebSocket:
1. Creating a ClientWebSocket object:
To start using ClientWebSocket, you must instantiate a new object of the ClientWebSocket class. This can be achieved using the following code snippet:
ClientWebSocket clientWebSocket = new ClientWebSocket();
2. Connecting to a WebSocket server:
After creating the ClientWebSocket object, you need to connect it to the desired WebSocket server. This is done through the ConnectAsync method provided by the ClientWebSocket class. The code snippet below demonstrates the connection process:
Uri serverUri = new Uri(“wss://example.com”);
await clientWebSocket.ConnectAsync(serverUri, CancellationToken.None);
3. Sending and receiving data:
Once the connection has been established, you can send and receive data over the WebSocket channel. The SendAsync and ReceiveAsync methods of the ClientWebSocket class are used for this purpose. Here’s an example that shows how to send and receive messages:
// Sending a message
string message = “Hello Server!”;
byte[] messageBytes = Encoding.UTF8.GetBytes(message);
await clientWebSocket.SendAsync(new ArraySegment
// Receiving a message
var buffer = new ArraySegment
WebSocketReceiveResult result = await clientWebSocket.ReceiveAsync(buffer, CancellationToken.None);
string receivedMessage = Encoding.UTF8.GetString(buffer.Array, buffer.Offset, result.Count);
4. Closing the WebSocket connection:
Once you have finished using the WebSocket channel, it’s important to close the connection to release system resources. This can be done by calling the CloseAsync method of the ClientWebSocket class. Here is an example:
await clientWebSocket.CloseAsync(WebSocketCloseStatus.NormalClosure, “Connection closing”, CancellationToken.None);
Benefits of using ClientWebSocket:
1. Real-time communication: ClientWebSocket provides a simple and efficient way to implement real-time communication between a client and a server.
2. Efficiency: The WebSocket protocol eliminates the need for HTTP requests/response headers for every message exchange, resulting in reduced network overhead.
3. Bi-directional communication: WebSocket allows both the client and server to send messages to each other simultaneously, enabling efficient two-way communication.
4. Wider browser support: Although WebSocket is primarily used in browser-based applications, ClientWebSocket extends its capabilities to desktop and server-side applications developed in C#.
FAQs:
Q1. Can I use ClientWebSocket in both .NET Framework and .NET Core applications?
A1. Yes, ClientWebSocket is supported in both .NET Framework and .NET Core.
Q2. Are there any limitations to the size of data that can be sent/received using ClientWebSocket?
A2. The maximum message size that can be sent or received depends on the configuration of the WebSocket server. Typically, most servers support message sizes up to 4KB.
Q3. Can I use ClientWebSocket to connect to WebSocket servers secured with SSL/TLS?
A3. Yes, ClientWebSocket supports connecting to WebSocket servers secured with SSL/TLS. Simply use the “wss://” protocol scheme when specifying the server URI.
Q4. Is it possible to handle WebSocket errors using ClientWebSocket?
A4. Yes, ClientWebSocket provides error-handling capabilities through exception handling. Common exceptions include WebSocketException, OperationCanceledException, and InvalidOperationException.
Q5. Can ClientWebSocket be used in multi-threaded environments?
A5. ClientWebSocket is not thread-safe by default. If you need to use it in a multi-threaded environment, appropriate synchronization mechanisms, such as locks, should be used to ensure thread safety.
In conclusion, ClientWebSocket is a versatile and powerful feature available in C# that simplifies the implementation of WebSocket clients. It enables real-time, bi-directional communication between a client and a server, allowing for efficient and responsive applications. By following the aforementioned steps and taking advantage of its benefits, developers can easily integrate WebSocket functionality into their applications and provide seamless, real-time communication.
Websocket In Asp Net Mvc
WebSocket is a communication protocol that provides full-duplex communication channels over a single TCP connection, enabling real-time data transfer between a client and a server. In the context of ASP.NET MVC, WebSocket allows developers to build interactive web applications that can push data from the server to the client instantly, eliminating the need for constant client polling.
This article aims to provide a comprehensive guide to using WebSocket in ASP.NET MVC. We will cover its benefits, its implementation within the ASP.NET MVC framework, and provide answers to frequently asked questions (FAQs) regarding its usage.
Benefits of WebSocket in ASP.NET MVC:
WebSocket provides several advantages over traditional HTTP polling or long-polling techniques:
1. Real-time data transfer: With WebSocket, data can be pushed from the server to the client instantly, allowing for real-time updates and eliminating the need for constant client requests.
2. Decreased server load: Unlike traditional polling techniques, WebSocket establishes a persistent connection, enabling the server to push data only when necessary, saving server resources and reducing network latency.
3. Increased scalability: WebSocket allows servers to handle a larger number of simultaneous connections more efficiently, making it an excellent choice for applications that require frequent communication between the server and clients.
Implementing WebSocket in ASP.NET MVC:
ASP.NET MVC provides great support for implementing WebSocket functionality. The steps outlined below will guide you through the process of setting up a WebSocket connection in an ASP.NET MVC application.
Step 1: Install the necessary packages
To begin, open your ASP.NET MVC project in Visual Studio and make sure you have the required packages installed. WebSocket is supported natively in ASP.NET Core, so no additional packages are needed. However, if you are using ASP.NET MVC 5 or earlier versions, you will need to install the `Microsoft.WebSockets` NuGet package.
Step 2: Add WebSocket support to your project
Next, add a new controller to your project that will handle WebSocket connections. This controller will inherit from the `WebSocketHandler` class provided by ASP.NET MVC. Override the necessary methods to handle incoming messages and establish communication with clients.
Step 3: Configure routing
WebSocket connections require a separate route configuration to differentiate them from standard HTTP requests. Add a route for WebSocket requests in the `RouteConfig.cs` file by mapping the WebSocket controller to a specific URL pattern. For example, you can use the `/ws` route to handle WebSocket connections: `routes.MapRoute(“WebSocket”, “/ws”, new { controller = “WebSocket”, action = “Index” });`.
Step 4: Client-side implementation
On the client-side, you can establish a WebSocket connection using JavaScript. Create a new WebSocket instance, passing the URL of the WebSocket controller defined in the previous step. Attach event listeners to handle the various states of the WebSocket connection, such as `onopen`, `onmessage`, `onclose`, or `onerror`. These events allow you to communicate with the server and handle incoming data.
WebSocket FAQs:
Q1: Is WebSocket supported in all web browsers?
A: Yes, most modern web browsers support WebSocket. However, it’s essential to check browser compatibility, especially if you are developing applications for older versions.
Q2: Can WebSocket be used with SSL/TLS encrypted connections?
A: Absolutely! WebSocket connections can be secured using the same SSL/TLS encryption utilized by standard HTTPS connections. This ensures data integrity and confidentiality.
Q3: Can I use WebSocket with ASP.NET Web Forms?
A: Unfortunately, WebSocket is not supported natively in ASP.NET Web Forms. However, third-party libraries are available to enable WebSocket functionality within Web Forms applications.
Q4: Are there any limitations to using WebSocket in ASP.NET MVC?
A: WebSocket is an excellent tool for real-time communication, but it might not be the best solution for all scenarios. It’s important to consider factors like browser support, scalability, and other architectural requirements before adopting WebSocket in your project.
Q5: How does WebSocket differ from SignalR?
A: SignalR is a high-level real-time communication library built on top of WebSocket. While WebSocket provides the underlying transport, SignalR simplifies the development process with additional features like automatic reconnection and real-time messaging.
In conclusion, WebSocket in ASP.NET MVC enables developers to build interactive and real-time web applications efficiently. By implementing WebSocket functionality within the ASP.NET MVC framework, you can take advantage of its numerous benefits, including real-time data transfer, decreased server load, and increased scalability. However, it’s crucial to consider browser compatibility, architectural requirements, and the potential need for additional libraries like SignalR before implementing WebSocket in your project.
Images related to the topic websocket sendasync example c#
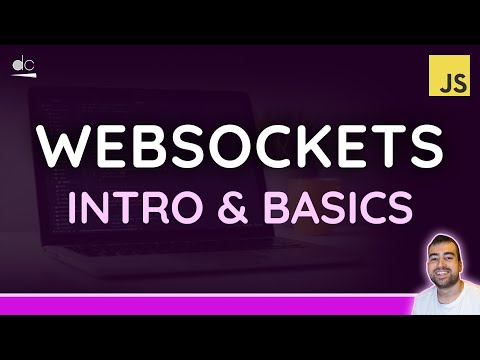
Article link: websocket sendasync example c#.
Learn more about the topic websocket sendasync example c#.
- c# 4.0 – How to send/receive messages through a web socket …
- C# (CSharp) System.Net.WebSockets WebSocket.SendAsync …
- Simple WebSocket client and server application using .NET
- C# WebSocket – working with websockets in C# – ZetCode
- websocket-sharp – GitHub Pages
See more: nhanvietluanvan.com/luat-hoc