An Object Reference Is Required For The Non-Static Field
In Java programming, object references play a crucial role in managing and manipulating objects. An object reference allows us to access the properties and methods of an object, making it an essential concept in object-oriented programming.
An object reference is a variable that holds the memory address of an object. When we create an object using the ‘new’ keyword, memory is allocated to hold the object’s data. The object reference then points to this memory location, providing a way to access and manipulate the object.
The Difference Between Static and Non-Static Fields
In Java, fields are variables within a class that store data. However, there is a fundamental distinction between static and non-static fields.
Static fields, also known as class variables, are associated with the class itself rather than instances (objects) of the class. They are shared among all instances of the class and are accessible without creating an object. Static fields are useful for storing data that is shared among all objects of a class, such as constants or counts.
On the other hand, non-static fields, also known as instance variables, are unique to each instance of a class. They hold data specific to each object and can only be accessed with an object reference. Non-static fields represent the state of an object and are modified individually for each instance.
The Importance of Object References in Java
Object references are essential in Java for various reasons. Firstly, they allow us to access and modify an object’s state by providing a link to the object’s memory location. Without object references, we would not be able to interact with objects directly.
Secondly, object references enable us to pass objects as parameters to methods. By passing an object reference as an argument, the method gains access to the object’s data and can operate on it. This is crucial for creating flexible code that can manipulate different objects.
Lastly, object references facilitate object-oriented programming principles such as inheritance and polymorphism. Through object references, we can create relationships between objects, inherit properties and behaviors from parent classes, and implement interfaces.
Common Errors: Non-Static Fields without Object References
One of the common errors related to object references is the “Object reference is required” error. This error occurs when trying to access a non-static field without an object reference. Since non-static fields are associated with instances of a class, they require an object reference to access them.
For example, consider a class named “Person” with a non-static field called “name.” If we try to access the “name” field without an object reference (e.g., Person.name), the error will occur. To resolve this error, we need to create an object of the “Person” class and use the object reference to access the “name” field.
How to Resolve the “Object Reference is Required” Error
To resolve the “Object reference is required” error, we need to ensure that we have created an object and are using the correct object reference to access the non-static field. Here are some steps to follow:
1. Create an object of the class that contains the non-static field. For example, if the class is named “Person,” create an object: Person person = new Person();
2. Use the object reference (in this case, person) to access the non-static field. For example, to access the “name” field: person.name = “John”;
By following these steps, we provide the required object reference to access the non-static field, resolving the error.
Best Practices for Handling Object References in Java
To handle object references effectively in Java, it is essential to follow some best practices. These practices help ensure proper memory management and avoid potential issues:
1. Initialize object references: Always initialize object references before using them. Failure to do so may result in null references and runtime errors.
2. Understand object lifecycles: Understand the lifecycle of objects and their references. Properly managing object references, such as releasing them when they are no longer needed, can optimize memory usage.
3. Avoid unnecessary references: Avoid unnecessary object references as they can lead to confusion and potential memory leaks. Use object references only when needed.
4. Use descriptive names: Choose meaningful names when creating object references. This improves code readability and makes it easier to understand the purpose of each reference.
Advanced Concepts: Object References in Object-Oriented Programming
Object references play a central role in object-oriented programming (OOP). They enable powerful features such as inheritance, polymorphism, and dynamic method dispatch.
Inheritance allows objects of a subclass to be treated as objects of their superclass. Using object references, we can create a superclass reference that points to a subclass object. This reference can then be used to access both the superclass and subclass methods and fields.
Polymorphism, another key concept in OOP, allows objects of different classes to be treated as objects of a common superclass. Object references enable this behavior by allowing us to use a reference variable of the superclass type to point to objects of different subclasses.
Dynamic method dispatch, also known as runtime polymorphism, is achieved through object references. When a method is called using an object reference, the actual implementation of the method is determined at runtime based on the object type.
By understanding and effectively using object references, we can leverage these advanced concepts to create flexible and extensible code.
FAQs
Q: What does the “Object reference not set to an instance of an object” error mean?
A: This error occurs in languages like C# and Unity when trying to use an object reference that is null or has not been assigned an instance of an object. It often indicates a programming mistake or a failure to initialize the object reference properly.
Q: Can a static field access non-static fields?
A: No, static fields cannot directly access non-static fields. Static fields do not have an associated object instance, so they cannot access or modify the state of specific instances.
Q: Can a non-static method access static fields?
A: Yes, non-static methods can access static fields. Static fields are shared among all instances of a class, so non-static methods can access them without relying on an object reference.
Q: Can a non-static field be accessed without an object reference?
A: No, non-static fields require an object reference to be accessed. They are associated with instances of a class and represent the state of individual objects.
Q: Are static fields recommended in object-oriented programming?
A: Static fields should be used with caution in object-oriented programming. While they can be useful for certain scenarios, overusing static fields can lead to coupling and make code less maintainable. They should be used primarily for constants and shared data.
In conclusion, object references are essential in Java programming and object-oriented programming in general. They allow us to interact with objects, pass them as parameters, and implement advanced features like inheritance and polymorphism. Understanding how to use and manage object references is crucial for writing efficient and maintainable code.
Cs0120: An Object Reference Is Required For The Non-Static Field, Method, Or Property… Easy Fix
How To Use Non Static Field In Static Method Java?
In Java, it is generally considered a good practice to design classes and methods in a way that follows the principles of object-oriented programming. However, there might be situations where we need to access non-static fields within static methods. While this is not a recommended approach, it is still possible to achieve this by using certain workarounds. In this article, we will discuss various ways to utilize non-static fields in static methods in Java, along with their advantages, disadvantages, and potential pitfalls.
Understanding Static and Non-Static Elements in Java:
Before delving into the details, it is essential to understand the difference between static and non-static elements in Java.
Static elements, such as fields and methods, belong to the class itself and are not tied to any specific instance of the class. They can be accessed using the class name directly, without creating an instance of the class. On the other hand, non-static elements are associated with each instance of the class and can only be accessed within an instance context using the reference.
Generally, when we want to access an instance field within a static method, we face a compilation error as the non-static field cannot be referenced from a static context. However, we can overcome this limitation by employing alternative approaches, such as passing instances as parameters or creating new instances.
Using Instances as Parameters:
One of the most straightforward ways to use non-static fields in static methods is by passing instances as parameters. By doing so, we can access the required non-static field within the static method using the provided instance.
Here’s an example to illustrate this approach:
“`java
public class MyClass {
private int nonStaticField;
public static void doSomething(MyClass instance) {
int value = instance.nonStaticField;
// Perform operations with the nonStaticField
}
}
“`
In this example, the static method `doSomething()` accepts an instance of the `MyClass` as a parameter. By using the provided instance, we can access the non-static field `nonStaticField`.
Creating New Instances:
Another approach to utilize non-static fields in static methods is by creating new instances of the class within the static method itself.
Here’s an example to demonstrate this technique:
“`java
public class MyClass {
private int nonStaticField;
public static void doSomething() {
MyClass instance = new MyClass();
int value = instance.nonStaticField;
// Perform operations with the nonStaticField
}
}
“`
In this example, the static method `doSomething()` creates a new instance of `MyClass` within the method itself. By doing this, we can access the non-static field `nonStaticField` using the newly created instance.
Advantages and Disadvantages:
Using non-static fields in static methods can provide flexibility in certain scenarios. It allows us to reuse the logic written for non-static fields within static contexts without duplicating the code or modifying the method access type. However, this approach can also lead to potential pitfalls and is generally considered a deviant from traditional object-oriented programming principles.
Some advantages of using non-static fields in static methods are:
1. Code Reusability: By accessing non-static fields within static methods, we can reuse code written for non-static contexts.
2. Simplicity: In some cases, it might be more straightforward to use non-static fields directly within a static method instead of creating additional objects or modifying method access types.
On the other hand, this approach has certain disadvantages and potential pitfalls:
1. Reduced Testability: Static methods are generally harder to test than non-static methods. By using non-static fields within static methods, we introduce additional dependencies on instances, making the static method less testable.
2. Potential Errors: Accessing non-static fields from static methods can lead to potential errors if the instances passed as parameters or created within the method are not properly initialized or null.
3. Increased Complexity: Using non-static fields within static methods can make the code more convoluted and harder to understand, as it deviates from the conventional object-oriented programming approach.
FAQs:
Q: Can static methods access non-static variables directly?
A: No, static methods cannot access non-static variables directly. They need to use alternative approaches such as passing instances as parameters or creating new instances to access non-static variables.
Q: Why is accessing non-static fields within static methods not recommended?
A: Accessing non-static fields within static methods is not recommended as it deviates from the principles of object-oriented programming and can introduce potential errors, reduce testability, and increase code complexity.
Q: Is it possible to modify non-static fields within static methods?
A: Yes, it is possible to modify non-static fields within static methods, as long as the non-static fields are accessible using the provided instance or a newly created instance.
Q: When should I consider using non-static fields in static methods?
A: Using non-static fields in static methods should be considered when code reusability or simplicity outweighs the potential drawbacks of reduced testability and increased complexity. However, it is generally recommended to follow the principles of object-oriented programming and avoid using non-static fields within static methods whenever possible.
In conclusion, although it is generally not recommended to use non-static fields within static methods in Java, there are workarounds available to achieve this. By passing instances as parameters or creating new instances within the static methods, we can access non-static fields. However, it is important to consider the advantages, disadvantages, and potential pitfalls before opting for this approach.
How To Access Non Static Field In Static Method C#?
In object-oriented programming, there are situations where we encounter scenarios where we need to access a non-static field from a static method. In C#, static methods are class members that can be accessed without creating an instance of the class. On the other hand, non-static fields are specific to each instance of the class. This creates a challenge when it comes to accessing non-static fields from a static method in C#. However, there are a few workarounds that can be used to achieve this. Let’s explore them in detail.
Understanding static methods and non-static fields:
Before delving into the ways of accessing non-static fields from a static method, it is important to have a clear understanding of what static methods and non-static fields actually are.
A static method belongs to a class rather than an instance of that class. They can be invoked directly on the class without the need for creating objects. Static methods do not have access to instance-specific data, including non-static fields. This is because non-static fields exist at an instance level and are specific to each instance of the class.
Non-static fields, on the other hand, are instance-specific variables. They are declared within a class and can have different values for each object of that class. Non-static fields can be accessed only through an instance of the class.
Given this, it becomes necessary to find a way to access non-static fields from within a static method.
Ways to access non-static fields from a static method in C#:
1. Pass an instance of the class as a parameter:
One way to access non-static fields is by passing an instance of the class as a parameter to the static method. This way, the static method can access the non-static fields of that particular instance.
Example:
“`csharp
class MyClass {
private string myField = “Hello”;
public static void DoSomething(MyClass instance) {
Console.WriteLine(instance.myField);
}
}
“`
Usage:
“`csharp
MyClass obj = new MyClass();
MyClass.DoSomething(obj);
“`
2. Declare the non-static field as static:
If it is absolutely necessary to access a non-static field from a static method, another option is to declare the non-static field as static. By doing so, the field becomes a static member of the class and can be accessed from any static method.
Example:
“`csharp
class MyClass {
private static string myField = “Hello”;
public static void DoSomething() {
Console.WriteLine(myField);
}
}
“`
Usage:
“`csharp
MyClass.DoSomething();
“`
3. Create an instance of the class within the static method:
In scenarios where it is not possible to pass an instance of the class as a parameter or declare the non-static field as static, an alternative approach is to create an instance of the class within the static method itself. This enables access to the non-static fields of that newly created instance.
Example:
“`csharp
class MyClass {
private string myField = “Hello”;
public static void DoSomething() {
MyClass instance = new MyClass();
Console.WriteLine(instance.myField);
}
}
“`
Usage:
“`csharp
MyClass.DoSomething();
“`
Frequently Asked Questions (FAQs):
Q: Why can’t we directly access non-static fields from a static method in C#?
A: Static methods do not have access to instance-specific data, including non-static fields, because they are class-level members and not tied to any specific instance of the class.
Q: Can we change a non-static field’s value from within a static method?
A: No, we cannot directly modify the value of a non-static field from a static method. However, if the non-static field is changed to static, it becomes modifiable by the static method.
Q: What are some alternatives to accessing non-static fields from a static method?
A: Pass an instance of the class as a parameter, declare the non-static field as static, or create an instance of the class within the static method.
Q: Are there any best practices when it comes to accessing non-static fields from a static method?
A: It is generally recommended to limit the usage of accessing non-static fields from a static method, as it can lead to potential design issues and violate object-oriented principles. Instead, try reevaluating the design to find a more appropriate way to accomplish the desired functionality.
In conclusion, accessing non-static fields from a static method in C# can be challenging since static methods do not have direct access to non-static fields. However, by implementing the approaches mentioned above, one can overcome this limitation and access non-static fields when necessary. It is important to choose the appropriate approach based on the specific requirements of the program and the design principles being followed.
Keywords searched by users: an object reference is required for the non-static field lỗi an object reference is required for the non-static field method or property, An object reference is required for the non static field method or property Unity, An object reference is required for the non static field method or property in asp net, Object reference not set to an instance of an object, Object reference not set to an instance of an object C#, A field initializer cannot reference the non-static field, method, or property, Object reference not set to an instance of an object unity, Not set to an instance of an object unity
Categories: Top 76 An Object Reference Is Required For The Non-Static Field
See more here: nhanvietluanvan.com
Lỗi An Object Reference Is Required For The Non-Static Field Method Or Property
When working with C# programming language, developers may come across the error message: “An object reference is required for the non-static field, method, or property.” This error can be quite puzzling for beginners and can hinder the progress of their coding journey. In this article, we will delve into the depths of this error, explain its causes and provide solutions to resolve it.
An object reference is essentially a pointer to an instance of a class. In C#, objects are created based on classes, which are essentially blueprints for creating instances of objects. While static fields, methods, and properties can be accessed directly through the class itself, non-static members require an instance of that class to be accessed.
When encountering the “Object Reference is Required” error, it implies that you are attempting to access a non-static field, method, or property without providing an object reference. Let’s explore the different scenarios where this error can occur:
1. Accessing non-static members directly from a static context:
In C#, static members are accessible at the class level, whereas non-static members require an instance of the class. If you try to access non-static members directly from a static context (e.g., a static method or property), the error will be thrown. To resolve this, create an instance of the object and access the member through the instance.
2. Forgetting to instantiate an object before accessing its members:
Before you can access the non-static members of a class, you need to create an instance of that class. If you try to access a member of an object without instantiating it first, the “Object Reference is Required” error will occur. To fix this, instantiate the object using the `new` keyword before accessing its members.
3. Null object references:
In some cases, the error can occur when you try to access the members of an object that has not been properly initialized or has been set to null. When a variable is set to null, it means it doesn’t point to any object in memory. Therefore, attempting to access its members will result in the error. Ensure that the object is properly instantiated and not set to null before accessing its members.
4. Passing an invalid argument to a method or property:
If a method or property requires a parameter of a specific object type, passing an invalid argument can lead to the “Object Reference is Required” error. Double-check the type of the object being passed and ensure it matches the expected type.
FAQs:
Q: Can a static method access non-static members?
A: No, static methods cannot directly access non-static members. As static members belong to the class, they don’t have direct access to the instance level members. However, it is possible to access non-static members by creating an instance of the class within the static method.
Q: How can I resolve the “Object Reference is Required” error?
A: To resolve this error, ensure that you are accessing non-static members through an instance of the class. If you are working in a static context, create an instance of the object and access the members through it. Ensure that the object is properly instantiated and not set to null.
Q: What is the difference between a static and non-static member?
A: Static members belong to the class itself, while non-static members belong to instances or objects created based on that class. Static members can be accessed directly through the class without creating an object, whereas non-static members require an object reference to be accessed.
Q: Why do I get the “Object Reference is Required” error when my object is not null?
A: Even if the reference to an object exists, the error can still occur if you attempt to access a non-static member without using the proper object reference. Ensure that you are not trying to access non-static members in a static context or forgetting to create an instance of the object.
Q: Is it possible to access non-static members without creating an instance of the class?
A: No, non-static members can only be accessed through an instance of the class. Creating an instance of the class allows you to access its non-static members.
In conclusion, the “Object Reference is Required” error in C# occurs when non-static members are accessed without providing an object reference. By thoroughly understanding the causes and solutions to this error, developers can avoid frustration and ensure smooth coding experiences. Remember to create instances of the class, check for null references, and access non-static members through the proper object reference.
An Object Reference Is Required For The Non Static Field Method Or Property Unity
Unity is a popular game development engine used by professionals and hobbyists alike. It provides a comprehensive set of tools and features that enable developers to create immersive and interactive experiences for various platforms. However, like any software, Unity has its quirks, and one of the common errors that developers encounter is “An object reference is required for the non-static field, method, or property Unity.” In this article, we will explore what this error means, how it can be triggered, and how to resolve it.
What does “An object reference is required for the non-static field, method, or property Unity” mean?
This error message typically occurs when a non-static class member, such as a field, method, or property, is accessed without an instance reference. In other words, Unity is informing you that you are trying to access a variable or function that belongs to an instance of a class but have not provided that instance.
How is this error triggered?
There are several scenarios in which this error can be triggered. Let’s explore a few common scenarios:
1. Accessing a non-static field without an instance reference:
In Unity, classes can have non-static fields, which means these fields belong to a specific instance of the class. If you try to access these fields without an instance reference, Unity will throw the “An object reference is required for the non-static field” error.
2. Calling a non-static method without an instance reference:
Similar to fields, classes can also have non-static methods. When you try to call a non-static method without an instance reference, Unity will generate the error message.
3. Accessing a non-static property without an instance reference:
Properties in Unity are also susceptible to this error. If you attempt to access or modify a non-static property without an instance reference, Unity will flag the issue.
How to resolve the error?
To resolve the “An object reference is required for the non-static field, method, or property Unity” error, you need to ensure that you provide the appropriate instance reference when accessing the non-static member. Here are a few solutions to consider:
1. Create an instance of the class:
If you are trying to access a non-static member from another script or class, you can create an instance of that class and use it to access the desired member. By creating an instance, you provide a valid object reference, resolving the error.
2. Make the member static:
Alternatively, you can consider making the member you are trying to access static. By making it static, you no longer need an instance reference to access the member, and Unity will not generate the error.
3. Ensure that the member is accessible:
Unity generates this error when you try to access a non-static member that is either private or protected. To resolve this, ensure that the member you are trying to access is declared with appropriate access modifiers, such as public or internal.
Frequently Asked Questions (FAQs):
Q: Why is it necessary to provide an object reference for non-static members?
A: In the object-oriented paradigm, non-static members are associated with specific instances of a class. To access or modify these members, you must provide a valid object reference that refers to the instance where the members are located.
Q: Can I only access non-static members within Unity’s scripts?
A: No, you can access non-static members within any Unity script or class, as long as you provide a valid object reference. However, you cannot access non-static members from static methods directly.
Q: How can I find which line of code triggered this error?
A: Unity’s console window usually provides a stack trace when this error occurs. Look for the line in the stack trace that corresponds to your script or class where the error originated. This will help you identify the location of the issue.
Q: Can I use a static member to access a non-static member?
A: Yes, you can use a static member to access a non-static member, as long as you provide a valid object reference for the instance from which you want to access the non-static member.
Q: Are there any performance implications in using non-static members?
A: Non-static members require the creation of an instance of a class, which can consume memory and have a slight performance impact compared to static members. However, the difference is typically negligible unless you are dealing with a massive number of instances.
In conclusion, the “An object reference is required for the non-static field, method, or property Unity” error is a common occurrence in Unity development. Understanding the causes of this error and how to resolve it is crucial for smooth development. By ensuring that you provide the appropriate object reference, modifying member accessibility, or considering static members where applicable, you can tackle this error and continue building amazing games and experiences using Unity.
An Object Reference Is Required For The Non Static Field Method Or Property In Asp Net
ASP.NET is a widely used web development framework that allows programmers to build dynamic web applications easily. However, like any other programming language, it has its own set of rules and guidelines that developers need to follow in order to avoid errors and ensure smooth execution of their code. One such error that developers often come across in ASP.NET is the “An object reference is required for the non-static field, method, or property” error. In this article, we will explore this error in depth, understand its causes, and provide some troubleshooting tips to fix it.
Understanding the Error:
The “An object reference is required for the non-static field, method, or property” error occurs when a non-static member (field, method, or property) is accessed without an object reference. In other words, the code is trying to access a non-static member without creating an instance of the class containing that member. Since non-static members are associated with instances of a class, they cannot be accessed directly without an instance. This error usually occurs when developers try to access a non-static member from a static context, such as a static method.
Causes of the Error:
1. Accessing Non-Static Members from a Static Context:
As mentioned earlier, the most common cause of this error is trying to access a non-static member from a static context. In ASP.NET, this can happen when a developer tries to access an instance variable or method from within a static method. Since static methods are not associated with any instance, they cannot access non-static members directly.
2. Null Reference or Uninitialized Object:
Another cause of this error is when developers try to access a non-static member on an object that is null or uninitialized. In such cases, there is no object reference available, and therefore the error occurs. It is important to ensure that any object being used to access non-static members is properly initialized before accessing them.
3. Incorrect Scoping:
Sometimes, this error can occur due to incorrect scoping of the object reference. If the object reference is declared inside a different scope or block, it may not be accessible from the current scope, leading to this error.
Fixing the Error:
Here are some troubleshooting tips to fix the “An object reference is required for the non-static field, method, or property” error in ASP.NET:
1. Create an Instance:
If you are trying to access a non-static member from a static method, you need to create an instance of the class containing that member and then access it through the instance.
2. Check for Null References:
Ensure that the object being used to access the non-static member is not null. If it is null, initialize it properly before accessing any of its members.
3. Check Scoping:
Verify the scoping of the object reference and make sure it is accessible from the current scope. If necessary, move the declaration of the object reference to a higher or more accessible scope.
4. Make the Member Static (if applicable):
If the non-static member does not rely on any instance-specific data, consider making it a static member. This way, it can be accessed directly from a static context without the need for an object reference.
5. Use an Instance Method (if applicable):
If the non-static member needs to be accessed from a static method, consider converting the static method into an instance method or creating an instance method that encapsulates the functionality required by the static method.
Frequently Asked Questions:
1. Can you explain the difference between a static and non-static member in ASP.NET?
Static members are associated with the class itself and can be accessed directly without creating an instance of that class. Non-static members, on the other hand, are associated with instances of the class and require an object reference to be accessed.
2. Why do I get this error when accessing a non-static member from a static method?
Static methods are not associated with any instance, so they cannot access non-static members directly. You need to create an instance of the class containing the non-static member and access it through that instance.
3. How can I check if an object reference is null or uninitialized?
Before accessing a non-static member on an object, you can use an if statement to check if the object reference is null. If it is null, properly initialize the object before accessing its members.
4. Can I make all my non-static members static to avoid this error?
While it is possible to make all non-static members static, it is not recommended unless necessary. Non-static members are often used to maintain instance-specific data and functionality, which cannot be achieved with static members.
In conclusion, the “An object reference is required for the non-static field, method, or property” error is a common error faced by ASP.NET developers. Understanding its causes and applying the suggested troubleshooting tips can help in resolving this error effectively. It is important to remember that static members cannot access non-static members directly and proper object references need to be created and initialized before accessing non-static members.
Images related to the topic an object reference is required for the non-static field
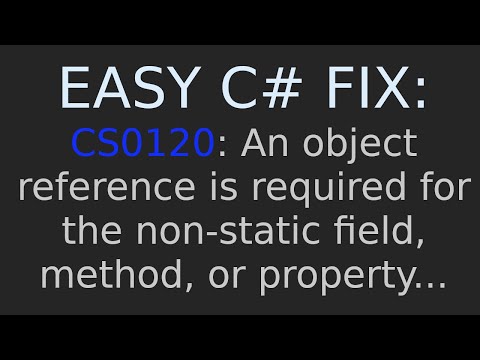
Found 32 images related to an object reference is required for the non-static field theme
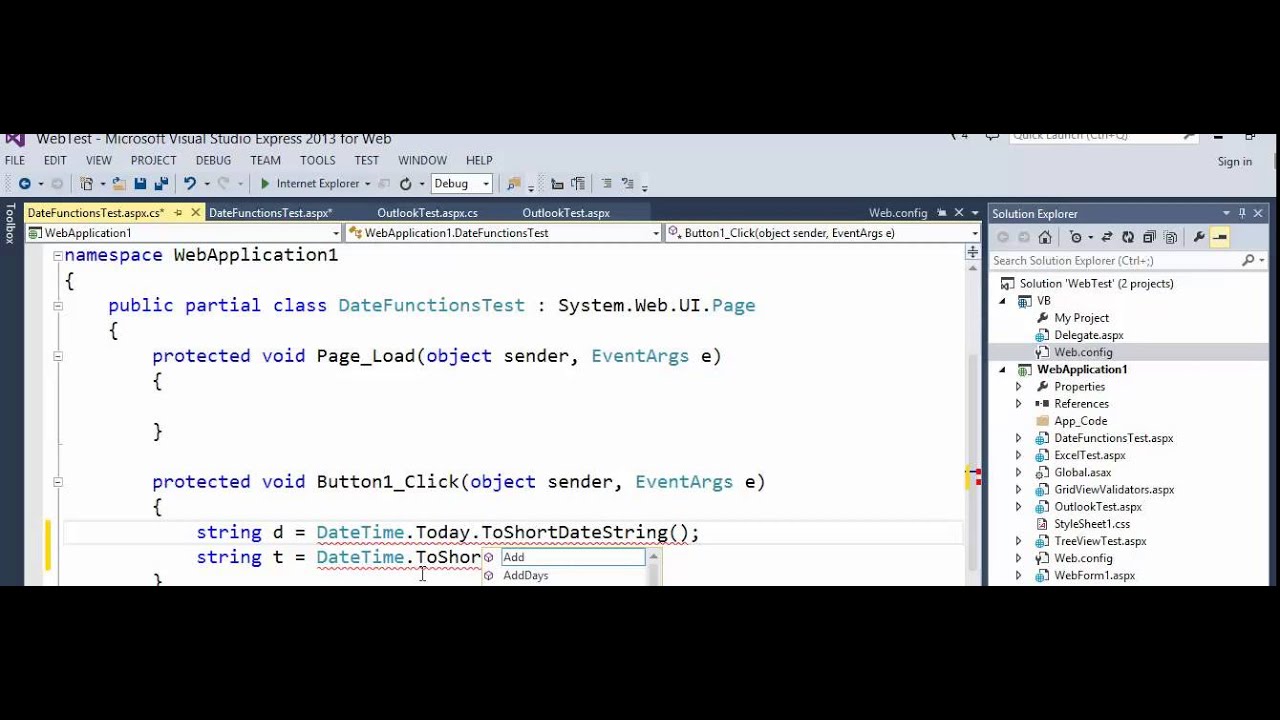






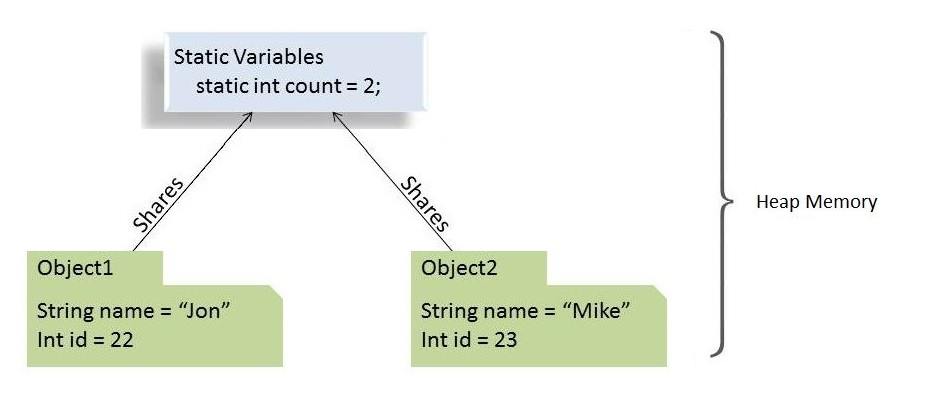


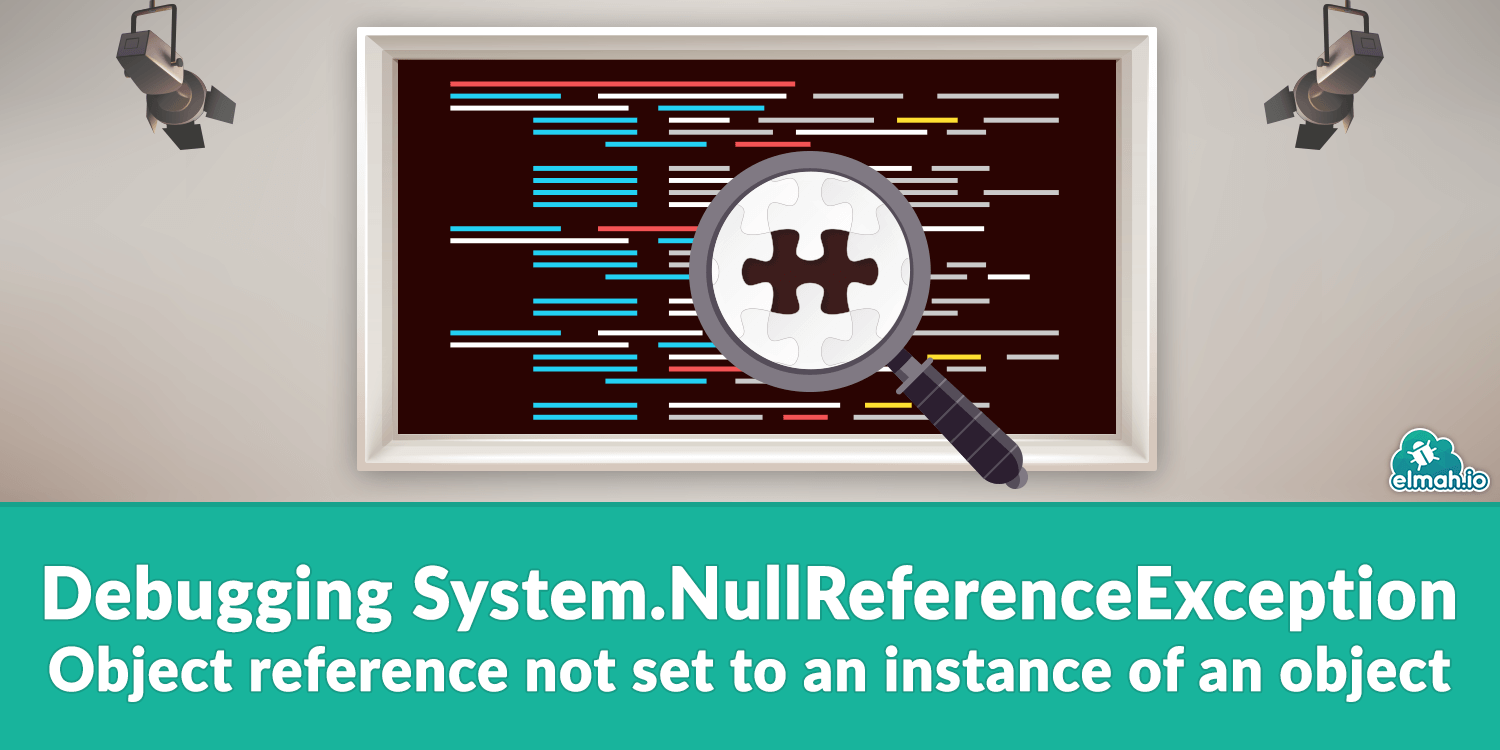
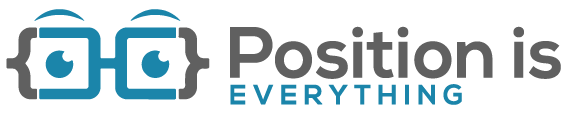
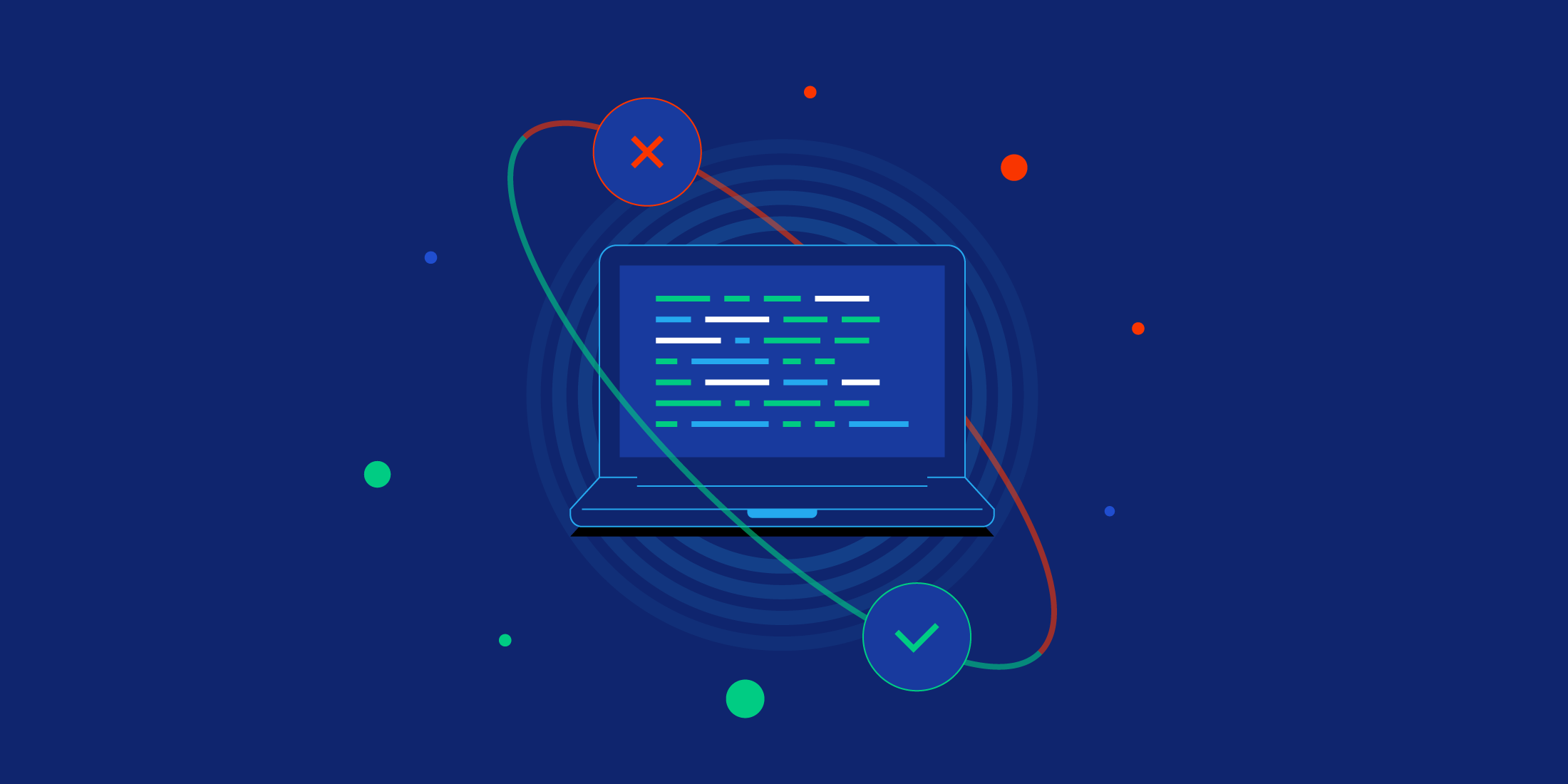
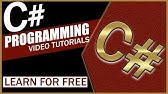
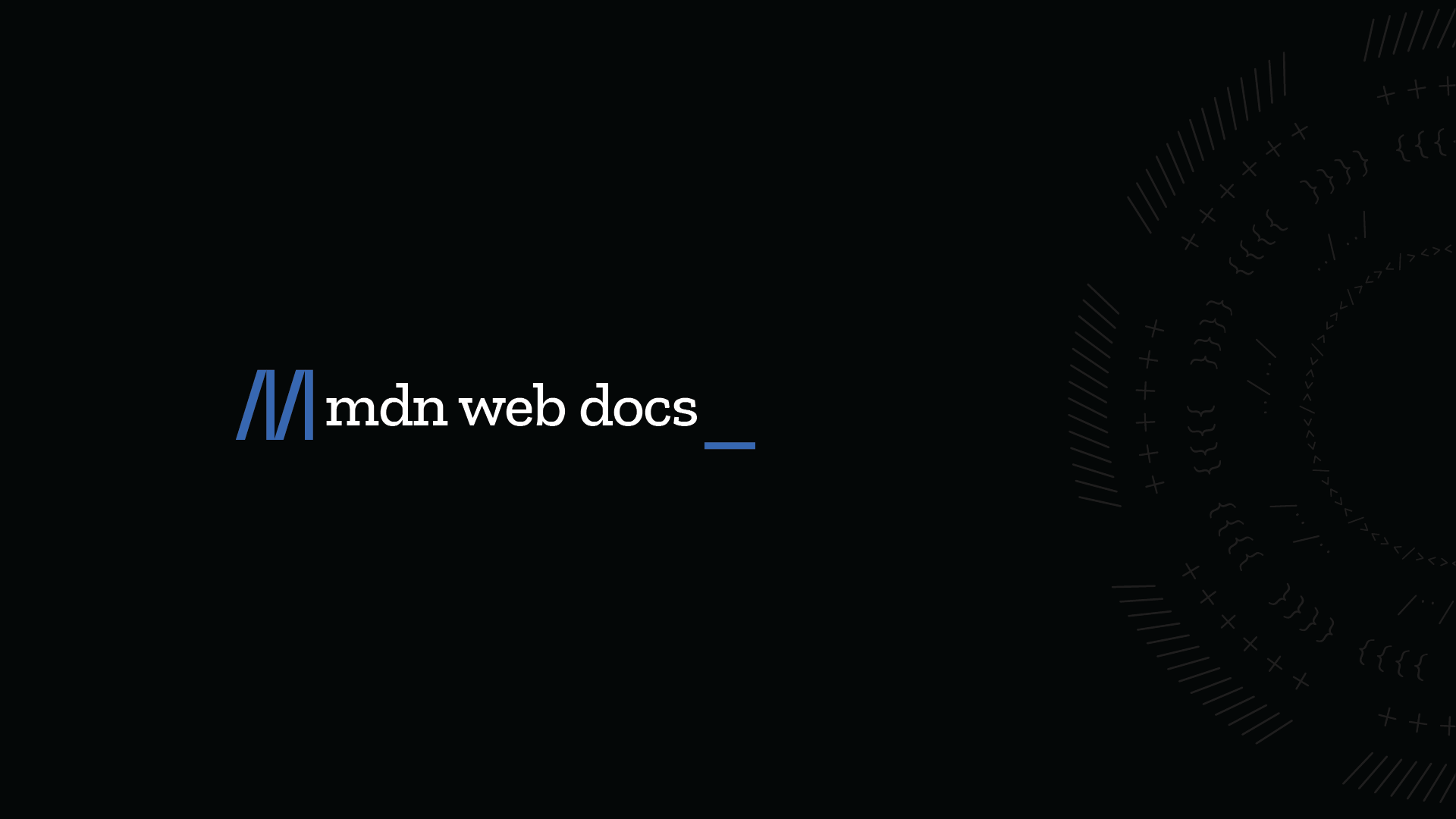
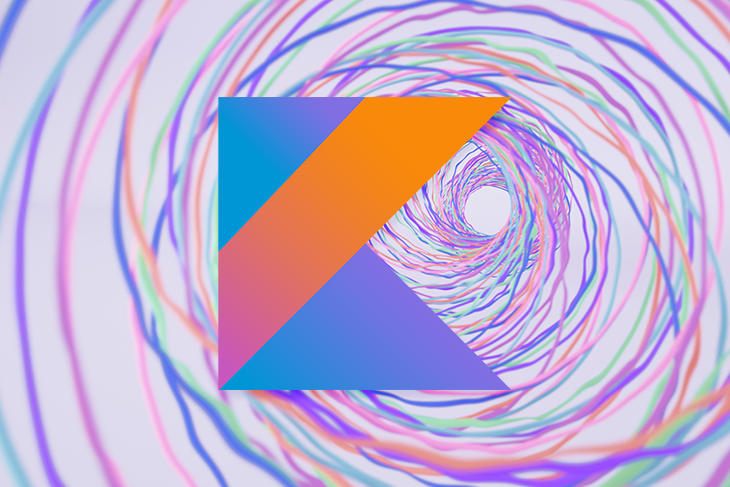
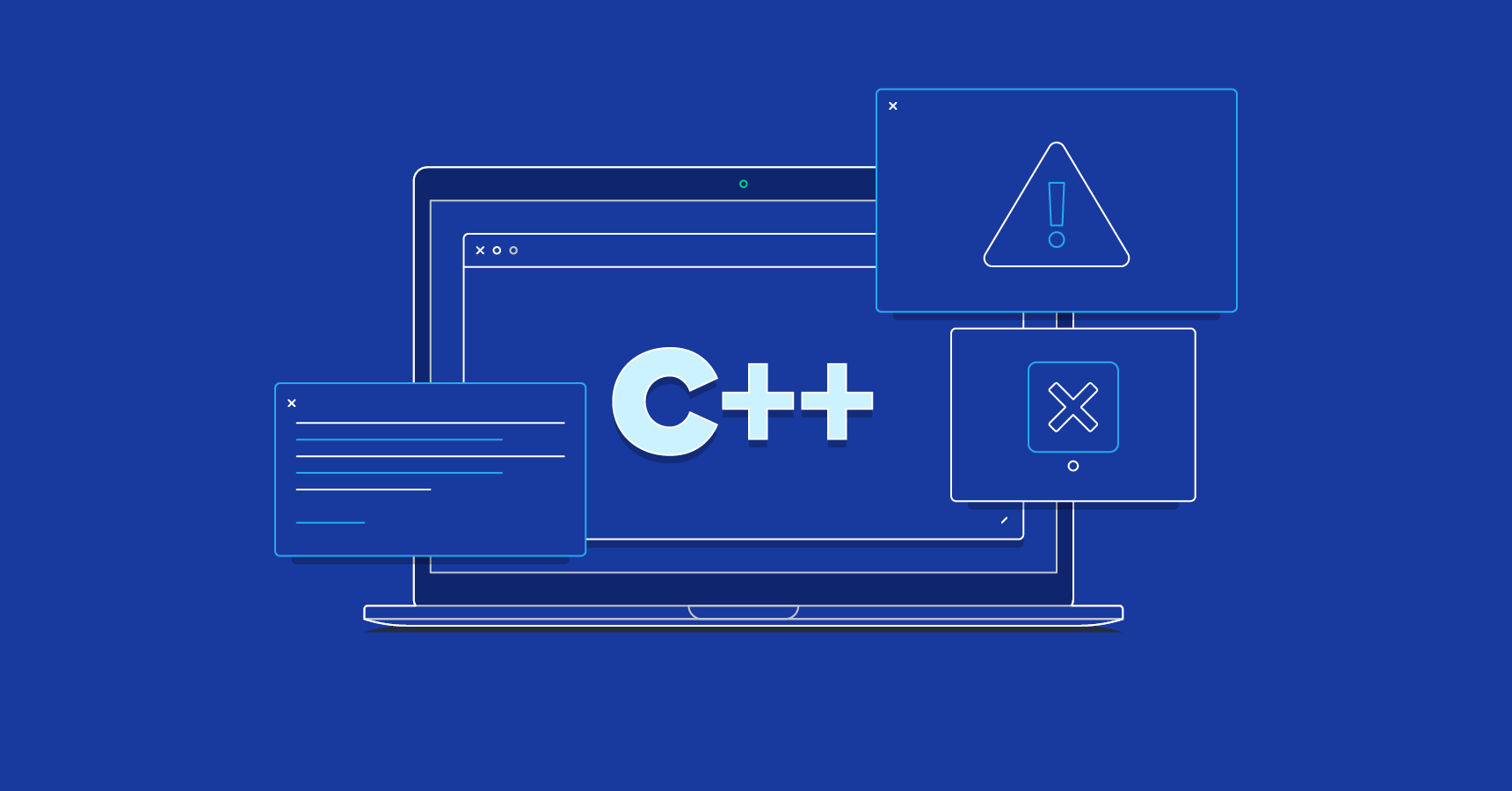

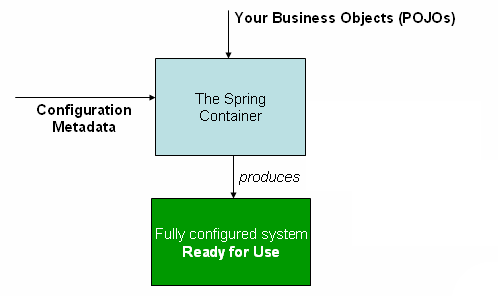
Article link: an object reference is required for the non-static field.
Learn more about the topic an object reference is required for the non-static field.
- An Object Reference Is Required for the Non-static Field: Fix It …
- C# Error: How to Resolve an object reference is required for …
- An object reference is required for the nonstatic field, method …
- Static Variable in Java with Examples – Scaler Topics
- Static vs Non-Static Members in C# with Examples – Dot Net Tutorials
- Difference between Static and Non-Static fields of a class.
- Difference between Static and Non-Static Variables in Java
- Compiler Error CS0120 | Microsoft Learn
- error An object reference is required for the non-static field …
- An object reference is required for the non-static … – Unity Forum
- Error CS0120 An object reference is required for the non-static …
- An object reference is required to access a non-static field
See more: blog https://nhanvietluanvan.com/luat-hoc