Golang Xml To Struct
XML (eXtensible Markup Language) is a popular data format used for representing structured information. It follows a set of rules for encoding documents in a format that is both human-readable and machine-readable. XML consists of a hierarchical structure, with nested elements and attributes, allowing for the representation of complex data models.
XML documents are made up of tags, elements, attributes, and content. Tags are used to define the beginning and end of an element and are enclosed in angle brackets (< and >). Elements are the basic building blocks of an XML document and can contain other elements or have attributes. Attributes provide additional information about an element and are defined within the start tag. Content refers to the data contained within an element.
Introduction to Golang’s XML Package
Golang, also known as Go, is a powerful programming language that was developed by Google. It is known for its simplicity, efficiency, and ease of use. The language comes with a standard library that includes a comprehensive XML package that provides functionalities for parsing and generating XML documents.
The XML package in Golang offers a set of functions and types for handling XML data. It allows developers to parse XML documents and convert them into Go data structures, and vice versa. The package provides a straightforward and intuitive approach to working with XML in Golang, making it easier for developers to work with XML data.
Parsing XML in Golang
The XML package in Golang provides the xml.Unmarshal function for parsing XML data. This function takes an XML document as input and converts it into a Go data structure. The parsing process involves mapping the XML elements and attributes to corresponding fields and values in the Go structure.
To parse XML in Golang, you first need to define the structure of the data you expect to receive in the XML document. This structure is known as a struct in Golang. The struct should have fields that correspond to the XML elements and attributes. Once the struct is defined, you can use the xml.Unmarshal function to parse the XML data and populate the struct with the values from the XML document.
Defining Structs for XML Unmarshalling
In Golang, structs are used to define custom data types that consist of a collection of fields. When parsing XML data, you need to define a struct that represents the structure of the XML document. Each field in the struct should correspond to an XML element or attribute.
For example, consider the following XML document:
“`
“`
To parse this XML document, you can define a struct in Golang as follows:
“`
type User struct {
Name string `xml:”name”`
Age int `xml:”age”`
}
“`
In this example, the User struct has two fields, Name and Age, which correspond to the name and age elements in the XML document. The `xml` struct tag is used to specify the mapping between the XML elements and the struct fields.
Mapping XML Elements to Struct Fields
The xml.Unmarshal function in Golang uses reflection to map XML elements to struct fields. It automatically matches the XML element names with the corresponding struct field names. If the names match exactly, the value of the XML element is assigned to the struct field.
In cases where the XML element name and struct field name do not match, you can use struct tags to specify the mapping explicitly. The `xml` struct tag allows you to specify the XML element name using the `xml:”elementName”` syntax.
Working with XML Attributes
XML elements can also have attributes, which provide additional information about the element. To map XML attributes to struct fields, you can use struct tags with the `attr` directive. The `attr` directive specifies that the corresponding field should be populated with the value of the XML attribute.
For example, consider the following XML document:
“`
“`
To map the roles attribute to a struct field, you can define the User struct as follows:
“`
type User struct {
Name string `xml:”name”`
Age int `xml:”age”`
Roles string `xml:”roles,attr”`
}
“`
In this example, the Roles field is tagged with `xml:”roles,attr”`, which specifies that it should be populated with the value of the roles attribute.
Handling Nested XML Structures
XML documents can have nested structures, where elements can contain other elements. To handle nested XML structures in Golang, you can define nested structs within the main struct. Each nested struct should represent the structure of the corresponding nested XML element.
For example, consider the following XML document:
“`
“`
To parse this XML document, you can define the User struct with a nested Address struct:
“`
type User struct {
Name string `xml:”name”`
Age int `xml:”age”`
Address Address `xml:”address”`
}
type Address struct {
Street string `xml:”street”`
City string `xml:”city”`
}
“`
In this example, the Address struct represents the structure of the address element. The Name, Age, and Address fields are then populated with the corresponding values from the XML document.
Dealing with Optional XML Elements
XML documents may contain optional elements, which are not present in all instances of the document. In Golang, you can handle optional XML elements by using pointers for the corresponding struct fields.
For example, consider the following XML document:
“`
“`
To handle the optional address element, you can define the User struct as follows:
“`
type User struct {
Name string `xml:”name”`
Age int `xml:”age”`
Address *Address `xml:”address”`
}
“`
In this example, the Address field is a pointer to the Address struct. If the address element is present in the XML document, the Address field will be populated with the values from the XML document. If the address element is not present, the Address field will be set to nil.
Handling XML Namespace in Golang
XML documents can include namespaces, which are used to avoid naming conflicts in XML elements and attributes. When parsing XML documents with namespaces in Golang, you need to take the namespaces into account and specify their mappings in the struct tags.
For example, consider the following XML document with a namespace:
“`
“`
To handle the namespace in Golang, you can define the User struct as follows:
“`
type User struct {
Name string `xml:”http://example.com name”`
Age int `xml:”http://example.com age”`
}
“`
In this example, the `xml` struct tag includes the namespace URI before the element name to specify the mapping between the XML element and the struct field.
Error Handling and Validation in XML Parsing
When parsing XML data in Golang, it is important to handle any potential errors that may occur during the parsing process. The xml.Unmarshal function returns an error value that can be checked to determine whether the parsing was successful or not.
In addition to error handling, it is also recommended to perform validation on the XML data after parsing. This can be done by checking the struct fields for any required or specific values and performing any necessary business logic checks.
FAQs
Q: Can I convert XML to a Go struct?
A: Yes, you can convert XML to a Go struct using the xml.Unmarshal function in the xml package of Golang. This function parses the XML data and maps it to the fields of the Go struct.
Q: How do I convert a Go struct to JSON?
A: You can convert a Go struct to JSON using the json.Marshal function in the encoding/json package of Golang. This function serializes the Go struct into a JSON-encoded byte array.
Q: Can Golang generate XML?
A: Yes, Golang can generate XML using the xml.Marshal function in the xml package. This function converts a Go struct into XML data by mapping the struct fields to XML elements and attributes.
Q: How do I parse XML in Golang?
A: You can parse XML in Golang using the xml.Unmarshal function in the xml package. This function takes an XML document as input and converts it into a Go data structure.
Q: How do I handle optional XML elements in Golang?
A: Optional XML elements can be handled in Golang by using pointers for the corresponding struct fields. If the optional element is present in the XML document, the field will be populated with the values from the XML. If the element is not present, the field will be set to nil.
Q: How do I handle XML namespaces in Golang?
A: XML namespaces in Golang can be handled by specifying their mappings in the struct tags using the `xml:”namespaceURI elementName”` syntax. This allows Golang to correctly map the XML elements and attributes to the struct fields.
Parsing Xml – Go Lang Practical Programming Tutorial P.11
Keywords searched by users: golang xml to struct Xml to struct golang, Golang struct to json, Golang XML, Golang generate xml, JSON to struct Golang, Golang XML parser
Categories: Top 28 Golang Xml To Struct
See more here: nhanvietluanvan.com
Xml To Struct Golang
XML (eXtensible Markup Language) is a widely used data format for representing structured information. In the world of programming, XML files are often encountered when working with APIs or parsing data from various sources. Dealing with XML data can be challenging, as it requires parsing and extracting relevant information. In Golang, a powerful programming language, the XML to struct conversion can simplify working with XML data tremendously. In this article, we will dive into the intricacies of XML to struct conversion in Golang, explore useful libraries, and provide answers to frequently asked questions.
Understanding XML to Struct Conversion:
XML follows a hierarchical structure, where each element may contain child elements and attributes. Converting XML data to struct in Golang allows developers to access and manipulate XML data as native Go objects. This conversion simplifies data extraction, validation, and further processing. Golang’s XML package provides functionalities to parse XML data and convert it into struct-like representations.
Golang’s XML Package:
The XML package in Golang provides a set of functions and types to work with XML data. The key component is the `encoding/xml` package, which offers XML parsing and struct conversion capabilities. The primary types involved in this conversion process are `xml.Unmarshaler` and `xml.Marshaler`. These interfaces allow users to define custom marshaling and unmarshaling methods for their structs.
Steps for XML to Struct Conversion in Golang:
To convert XML data into struct representations, follow the steps outlined below:
1. Define the Struct: Create a struct that mirrors the desired XML structure. Use tags to map the XML element names to struct fields. For example:
“`go
type Book struct {
Title string `xml:”title”`
Author string `xml:”author”`
Year int `xml:”year”`
}
“`
2. Import the Required Packages: Import the `”encoding/xml”` package to use XML parsing and marshaling functions, and `”io/ioutil”` to read the XML file.
3. Read XML Data: Read the XML data from a file or API response using the `ioutil.ReadFile()` function, which returns a byte slice or an error.
4. Unmarshal XML Data: Use the `xml.Unmarshal()` function to unmarshal the XML data into the struct. Provide the byte slice and a pointer to the struct as arguments. If successful, the values in the XML will be populated into the struct.
“`go
xmlData, err := ioutil.ReadFile(“books.xml”)
if err != nil {
log.Fatal(err)
}
var books []Book
err = xml.Unmarshal(xmlData, &books)
if err != nil {
log.Fatal(err)
}
“`
Now, the XML data can be accessed through the `books` slice, which holds instances of the `Book` struct.
5. Access XML Data: Accessing data with the converted struct is effortless. Use struct field names to manipulate or retrieve XML data. For example:
“`go
fmt.Println(“Title:”, books[0].Title)
fmt.Println(“Author:”, books[0].Author)
“`
Useful Golang Libraries for XML to Struct Conversion:
While Golang’s standard library provides sufficient tools for XML parsing and struct conversion, several external libraries offer even more extended capabilities. Some popular libraries include:
1. `xmlpath`: This library provides a simple and intuitive way to navigate XML documents using XPath expressions. It is handy for handling complex XML structures.
2. `go-xmlsec`: If your XML data contains digital signatures, this library helps with signature verification and manipulation.
Frequently Asked Questions:
Q1. Can I handle XML namespaces during struct conversion?
Yes, Golang’s XML package supports XML namespaces. By defining additional struct fields with appropriate tags, the namespace-related data can be accessed effortlessly. For example:
“`go
type Book struct {
Title string `xml:”title”`
Author string `xml:”author”`
Year int `xml:”year”`
Namespace string `xml:”title>namespace,attr”`
Description string `xml:”description,attr”`
}
“`
Q2. What happens if there is invalid XML data or the struct doesn’t match the XML structure?
If the XML data is invalid or mismatches the struct structure, Golang’s XML package will return an error during the unmarshaling process. Handling these errors is essential to avoid runtime issues.
Q3. Can I convert struct data back to XML?
Yes, Golang’s XML package provides the `Marshal()` function to convert struct data back to XML. Use `xml.Marshal()` or `xml.MarshalIndent()` to generate the XML representation.
Conclusion:
Working with XML data requires efficient parsing, extraction, and manipulation techniques. Golang’s XML package, combined with struct conversion, simplifies these tasks significantly. In this article, we explored the XML to struct conversion process in Golang, delved into the required steps, and introduced some useful libraries for handling XML data. By mastering XML to struct conversion, developers can seamlessly work with XML data and exploit its full potential.
Golang Struct To Json
Introduction to Golang Struct to JSON Transformation
Structures (or structs) in Golang provide a way to define custom data types that encapsulate different fields. These structures are often used to organize and model data in a more structured format. One common requirement when working with structures is the need to convert them to JSON (JavaScript Object Notation) format, which is a lightweight data interchange format widely used in web development. In this article, we will explore various techniques and best practices for converting Golang structs to JSON.
Understanding JSON Serialization
Serialization is the process of converting an object’s state into a format that can be easily sent over a network or stored persistently. JSON serialization involves transforming data structures, such as Golang structs, into a JSON representation. This allows the data to be easily parsed and reconstructed at the receiving end. Golang’s standard library provides built-in support for JSON serialization and deserialization using the `encoding/json` package.
Struct Tagging in Golang
Before diving into struct-to-JSON conversion, it’s essential to touch upon struct tagging in Golang. Struct tags are annotations that can be added to struct fields, allowing developers to provide metadata or instructions to other components, such as the JSON encoder. Struct tags are generally defined within backticks (`json:”tag”`) immediately preceding each field declaration.
Struct tags are widely used to customize the behavior of the JSON encoder during serialization. For instance, they can be used to specify the JSON field name, omit fields from JSON serialization, or instruct the encoder on how to handle custom types. By default, the key for JSON representation is the name of the struct field, but struct tags can override this behavior.
Golang Struct to JSON Conversion
To convert a Golang struct to JSON, we use a combination of struct tagging and the `encoding/json` package’s `Marshal()` function. Let’s consider an example where we have a struct representing an Employee:
“`go
type Employee struct {
ID int `json:”employeeId”`
FirstName string `json:”firstName”`
LastName string `json:”lastName”`
Age int `json:”age”`
}
“`
The struct tags here specify the desired JSON field names for each struct field. In this scenario, we can encode an `Employee` instance to JSON using the `Marshal()` function:
“`go
employee := Employee{
ID: 123,
FirstName: “John”,
LastName: “Doe”,
Age: 35,
}
json, err := json.Marshal(employee)
if err != nil {
log.Fatal(err)
}
fmt.Println(string(json))
“`
The output will be:
“`json
{
“employeeId”: 123,
“firstName”: “John”,
“lastName”: “Doe”,
“age”: 35
}
“`
The `json.Marshal()` function automatically detects the struct tags and serializes the struct fields according to the specified JSON field names. Note that fields must be exported (start with an uppercase letter) to be accessible during JSON encoding.
Nested Structs and Embedded Types
In complex data structures, structs can be nested or contain embedded types. Golang supports these scenarios without any additional complexity. Consider the following example:
“`go
type Address struct {
City string `json:”city”`
State string `json:”state”`
}
type Employee struct {
ID int `json:”employeeId”`
FirstName string `json:”firstName”`
LastName string `json:”lastName”`
Age int `json:”age”`
Address Address
}
“`
In this case, the `Employee` struct contains an embedded `Address` struct. When serializing an `Employee` instance to JSON, the `Address` struct will be automatically converted as well. The resulting JSON will have nested fields representing the embedded structs:
“`json
{
“employeeId”: 123,
“firstName”: “John”,
“lastName”: “Doe”,
“age”: 35,
“address”: {
“city”: “New York”,
“state”: “NY”
}
}
“`
Structs can also embed anonymous fields or types. In this case, the embedded fields will become part of the struct’s JSON representation directly, without any enclosing object. The field names of the embedded struct/type will be used for JSON keys:
“`go
type Employee struct {
ID int `json:”employeeId”`
FirstName string `json:”firstName”`
LastName string `json:”lastName”`
Age int `json:”age”`
Address
}
“`
The `Address` struct is embedded anonymously within the `Employee` struct. When serialized to JSON, the resulting output will be:
“`json
{
“employeeId”: 123,
“firstName”: “John”,
“lastName”: “Doe”,
“age”: 35,
“city”: “New York”,
“state”: “NY”
}
“`
FAQ Section
Q1. Can I specify custom JSON names for a Golang struct’s fields?
A1. Yes, struct tags can be used to override the default JSON field names. By specifying tags like `json:”name”`, you can change how the fields are represented in the JSON.
Q2. How can I exclude certain fields from JSON serialization?
A2. By specifying `json:”-“` as the struct tag for a field, you can instruct the JSON encoder to exclude that field during serialization.
Q3. Can structs with circular references be converted to JSON?
A3. No, JSON does not support circular references. Attempting to encode a struct with circular references will result in a stack overflow error.
Q4. How can I handle custom types while converting structs to JSON?
A4. Golang provides a flexible way to handle custom types. You can implement the `MarshalJSON()` method for a struct to define custom JSON serialization logic for that type.
Q5. How can I control the indentation and formatting of the resulting JSON?
A5. The `json.MarshalIndent()` function can be used instead of `json.Marshal()` to produce formatted JSON output with indentation.
Conclusion
Converting Golang structs to JSON is a straightforward process, thanks to Golang’s robust built-in JSON support and struct tagging capabilities. By properly utilizing struct tags and following the guidelines outlined in this article, you can effortlessly transform your structs into JSON representations. JSON serialization is a crucial requirement for various web development tasks and API integrations, and Golang’s simplicity makes it a suitable choice for implementing such functionality.
Remember to thoroughly understand the JSON encoding and decoding methods provided by Golang, as well as the struct tag options available. This knowledge will empower you to handle various scenarios, including nested structs, custom types, and excluding certain fields from serialization. With these skills, you’ll be well-equipped to work with Golang structs and JSON effectively in your projects.
Images related to the topic golang xml to struct
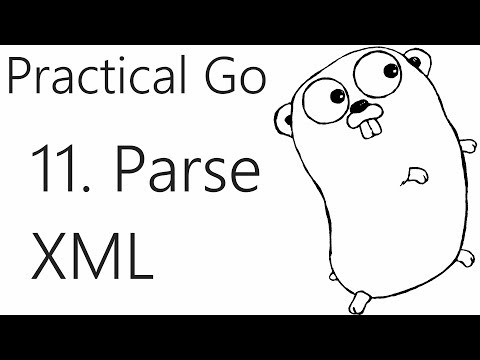
Found 19 images related to golang xml to struct theme
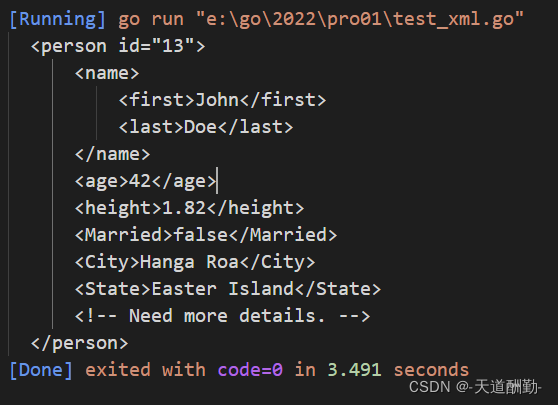
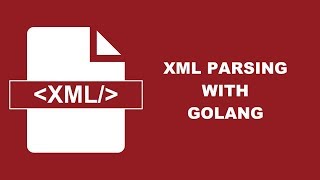


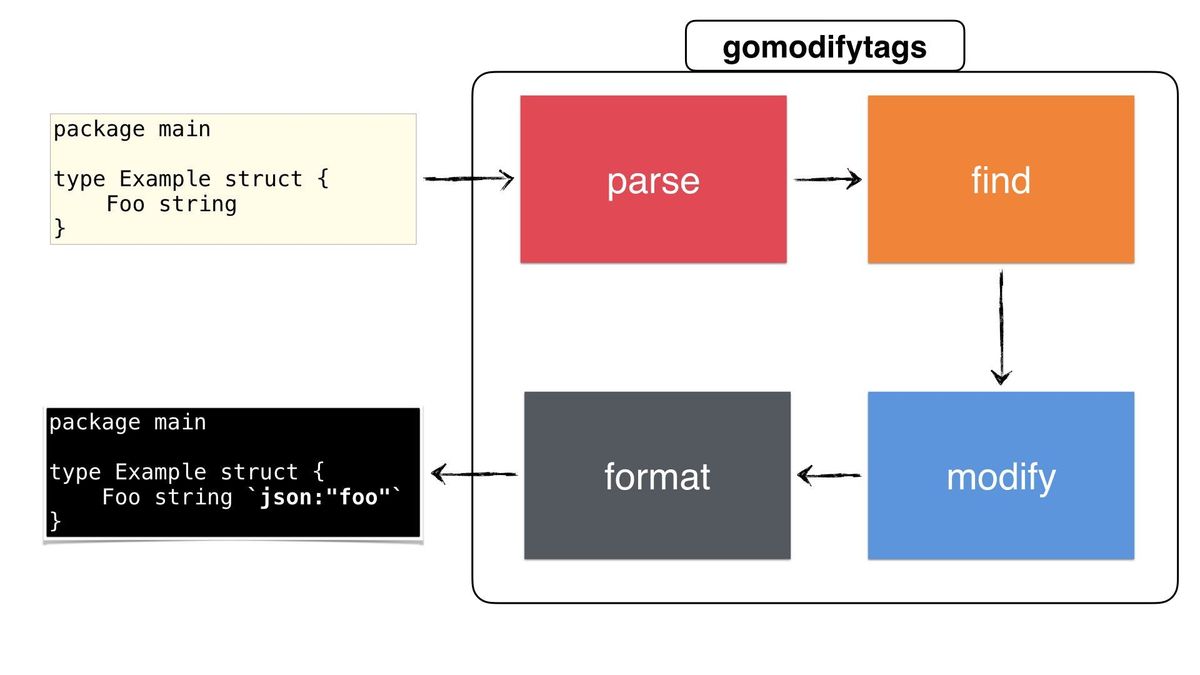


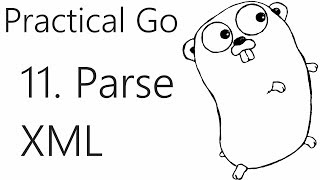
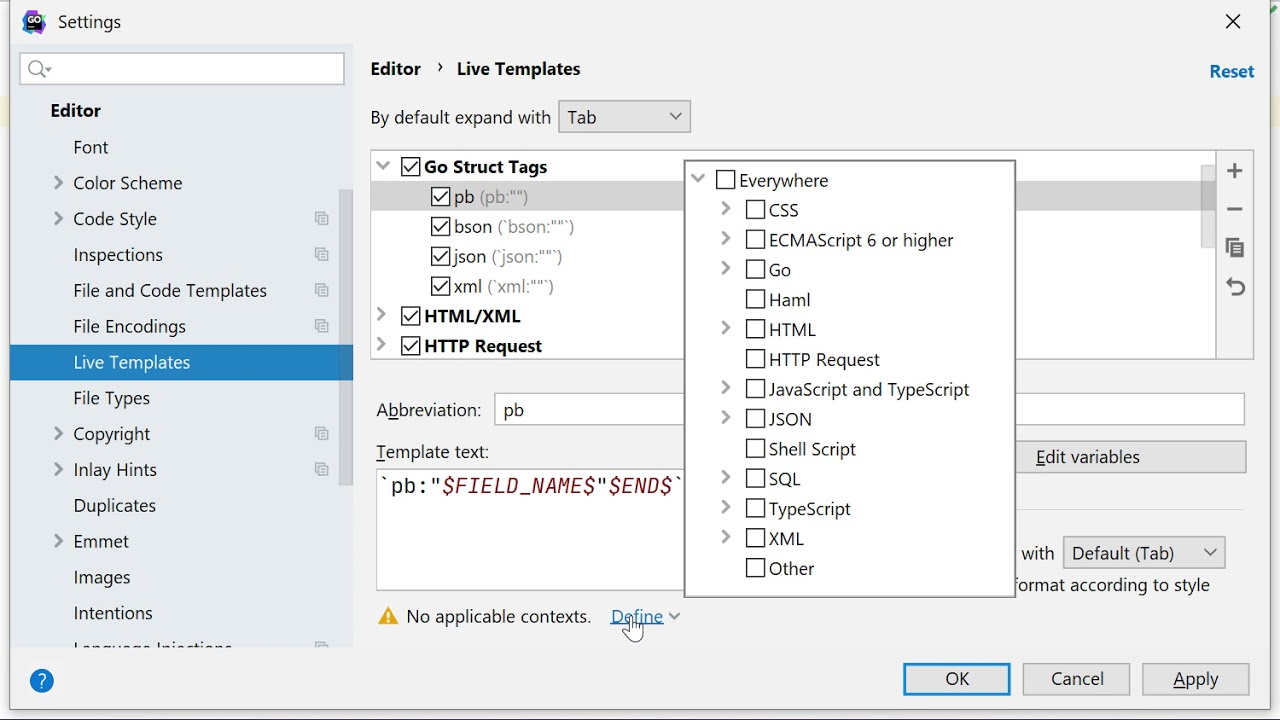
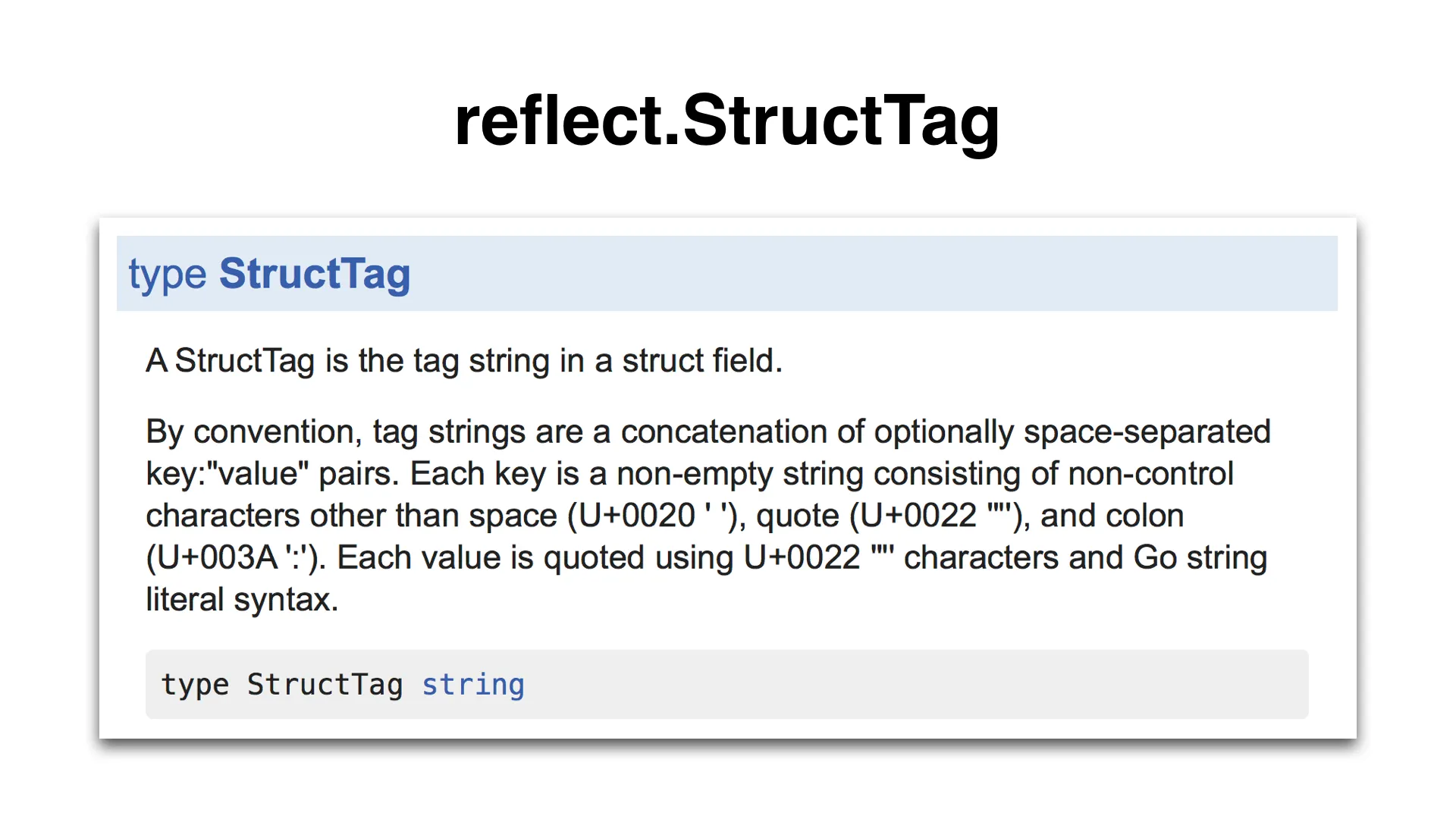
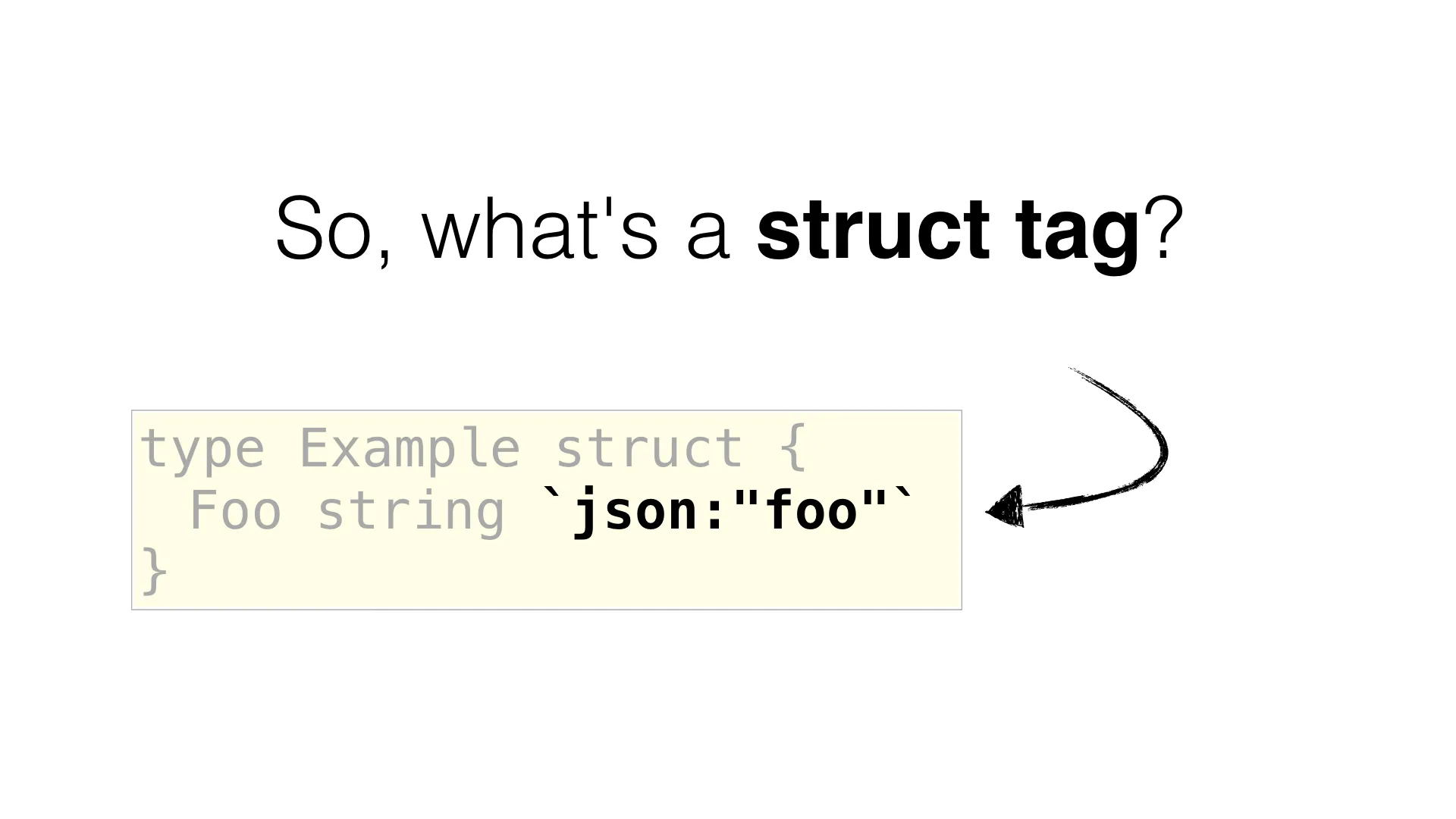

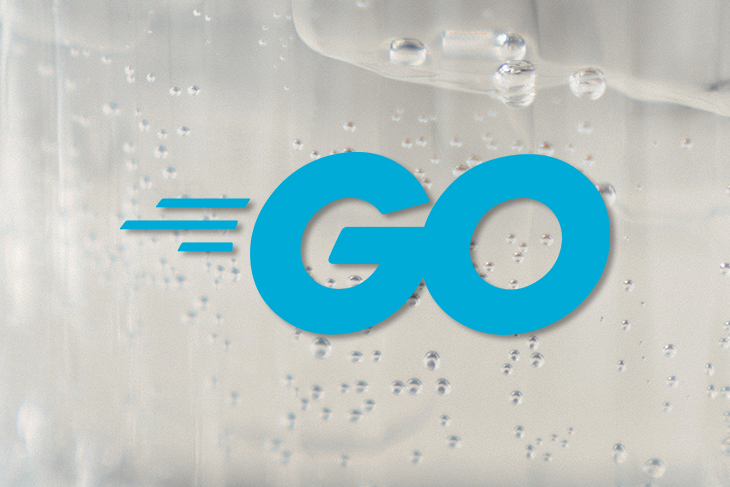



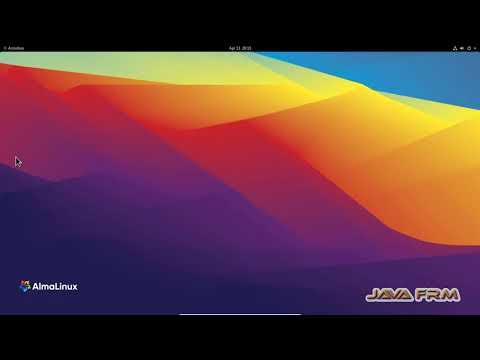
Article link: golang xml to struct.
Learn more about the topic golang xml to struct.
- XML to Go – Krzysztof Kowalczyk
- Golang XML to Struct [closed] – Stack Overflow
- miku/zek: Generate a Go struct from XML. – GitHub
- Golang program to read XML file into struct
- Online XML to Golang Struct Definition Code Tool
- How can I convert xml to struct – Getting Help – Go Forum
- XML – Go by Example
- Best XML to Go Converter – JSON Formatter
See more: nhanvietluanvan.com/luat-hoc