Golang Struct Name Convention
Golang (or Go) is a statically-typed language that aims to be efficient, concise, and scalable. It has gained significant popularity among developers due to its simplicity and performance. When it comes to writing clean and maintainable Go code, adhering to structured naming conventions is crucial. In this article, we will discuss the best practices for naming conventions specifically related to structs (structured types) in the Go programming language.
1. Naming Conventions for Struct Types
In Go, struct types represent user-defined composite types that are composed of named fields. To ensure readability and clarity, it is recommended to follow these guidelines for naming struct types:
– Use descriptive names that reflect the purpose or concept of the struct.
– Use camel case notation, starting with an uppercase letter (e.g., EmployeeDetails, OrderItem).
– Keep the struct names concise, but avoid unnecessary abbreviations.
Example:
type EmployeeDetails struct {
ID int
Name string
Salary float64
}
2. Naming Conventions for Struct Fields
Struct fields represent the properties of a struct type. The naming conventions for struct fields are similar to those of variables in Go:
– Use descriptive names that accurately convey the purpose or meaning of the field.
– Follow camel case notation, starting with a lowercase letter.
– Avoid unnecessary abbreviations, unless they are widely understood or widely used in the domain.
Example:
type EmployeeDetails struct {
id int
name string
salary float64
}
3. Naming Conventions for Struct Methods
Struct methods provide behavior for the struct types. Naming conventions for struct methods follow the standard Go naming conventions for functions:
– Use descriptive names that clearly articulate the functionality provided by the method.
– Use camel case notation, starting with an uppercase letter.
– Include the receiver type name as the first parameter, using an abbreviated form if necessary.
Example:
func (e EmployeeDetails) GetSalaryIncreasePercentage() float64 {
// Method implementation
}
4. Unexported Fields and Naming Conventions
In Go, the visibility of struct fields and types is controlled by capitalization. Unexported fields (accessible only within the same package) are typically used for encapsulation purposes. The usual convention for naming unexported fields is to start them with a lowercase letter.
Example:
type EmployeeDetails struct {
id int // unexported field
Name string // exported field
salary float64 // unexported field
}
5. Embedded Structs and Naming Conventions
Go allows embedding one struct type into another to achieve composition. When naming embedded structs, it’s generally recommended to use the same rules as for regular struct types.
Example:
type EmployeeDetails struct {
PersonDetails
// other fields
}
type PersonDetails struct {
Name string
Age int
}
6. Naming Conventions for Struct Interfaces
In Go, interfaces represent a contract or set of methods that a certain type can implement. Naming interfaces follows the same conventions as naming regular types.
– Use descriptive names that convey the behavior or functionality described by the interface.
– Use camel case notation, starting with an uppercase letter.
Example:
type Printer interface {
Print()
}
7. Naming Conventions for Struct Variables
Naming conventions for struct variables should generally follow the same conventions as for regular variables in Go:
– Use descriptive names that reflect the purpose of the variable.
– Use camel case notation, starting with a lowercase letter.
– Avoid excessive abbreviation, unless widely understood or widely used in the domain.
Example:
func main() {
employeeDetails := EmployeeDetails{
id: 1,
name: “John Doe”,
salary: 5000,
}
}
FAQs:
Q1. Should struct names always begin with an uppercase letter?
A1. Yes, struct names should start with an uppercase letter to follow the convention for exported (public) types in Go.
Q2. Should I use an unexported field within the same package?
A2. Yes, unexported fields are typically used for encapsulation purposes within the same package.
Q3. Can I use the same name for a struct and an interface?
A3. While it is possible to use the same name for a struct and an interface, it is generally recommended to use distinct names to avoid confusion and improve readability.
Q4. What is the naming convention for global variables in Go?
A4. Global variables in Go should be named using camel case notation, starting with an uppercase letter.
Q5. Can a function return a struct type?
A5. Yes, a function in Go can return a struct type. It is a common practice and contributes to the clarity and flexibility of the codebase.
Q6. Is capitalization mandatory for struct fields?
A6. Capitalization of struct fields affects their visibility. Exported fields (starting with an uppercase letter) are accessible outside the package, while unexported fields (starting with a lowercase letter) are only accessible within the same package.
In conclusion, adhering to consistent and informative naming conventions is crucial for writing clean and maintainable Go code. By following the best practices for naming struct types, fields, methods, interfaces, and variables discussed in this article, you can improve the readability and maintainability of your Go codebase.
Golang: 10+ Unique Concepts/Conventions That Beginners Should Know About!
Keywords searched by users: golang struct name convention golang naming convention, golang struct name lowercase, golang struct fields, golang interface and struct same name, golang function name convention, golang return struct, golang struct capitalization, golang global variable naming convention
Categories: Top 30 Golang Struct Name Convention
See more here: nhanvietluanvan.com
Golang Naming Convention
1. Why is Naming Convention Important?
Before delving into the specifics of Go naming conventions, it is essential to understand why adhering to proper naming conventions is crucial in the first place. Naming conventions provide a standardized way of naming variables, functions, packages, and other code entities, making code easier to read and comprehend. Furthermore, by following a consistent naming convention, different developers working on the same project can easily understand each other’s code, leading to increased collaboration and efficiency. Lastly, adhering to a naming convention ensures that code looks visually consistent, which reduces cognitive load and makes debugging and maintenance easier.
2. Basic Naming Rules in Go
Go has a set of basic naming rules that should be followed by developers to maintain code consistency. These rules are straightforward, making it easy for developers to understand and apply them. Here are some key points to remember:
– Use meaningful names that accurately describe the purpose or functionality of the code entity. Avoid using ambiguous names or single-letter names.
– Use camel case for function and variable names. Camel case is a convention where the first letter of each word is capitalized, except the first word which starts with a lowercase letter. For instance, use “myFunction” instead of “MyFunction” or “myfunction.”
– Capitalize acronyms and abbreviations only if they are the first word of the name. For example, use “HTTPServer” instead of “httpServer” or “httpserver.”
– Avoid using package names that conflict with standard library packages or popular third-party libraries. This can help prevent naming conflicts and confusion.
3. Naming Variables
Variable naming has a significant impact on the readability and maintainability of the code. In Go, variables should be named in a concise yet meaningful way. Here are some guidelines for naming variables in Go:
– Use lowercase letters for most variables, following the camel case convention. For example, use “firstName” instead of “First_Name” or “first_name.”
– For global variables, use uppercase letters to indicate their visibility scope. For instance, use “MaxAttempts” instead of “maxAttempts” or “MAXATTEMPTS.”
4. Naming Functions
Function names in Go should be descriptive, indicative of their purpose, and follow the camel case convention. Here are a few guidelines to follow while naming functions:
– Use verbs or verb phrases to name functions, indicating the action they perform. For example, use “calculateSum” instead of “sumCalculation.”
– Avoid prefixing functions with redundant verbs like “get” or “retrieve.” Instead, focus on the main action the function performs. For instance, use “getUser” instead of “fetchUser” or “retrieveUser.”
5. Naming Packages
The naming of packages carries significant importance in Go as it impacts import statements and package organization. When naming packages, consider the following conventions:
– Use lowercase letters for package names.
– Choose short and concise package names that reflect their purpose. Package names should be descriptive, but not overly verbose.
– Avoid using single-character package names, unless the package is exceptionally small and has a very specific purpose.
FAQs:
Q1. Can I use underscores in variable and function names in Go?
No, Go does not support the use of underscores in variable and function names. Instead, it is recommended to use camel case convention for naming variables and functions.
Q2. Are there any standardized prefixes or suffixes for package names in Go?
No, Go does not enforce any standardized prefixes or suffixes for package names. However, it is a common practice to use short and concise package names that are indicative of their functionality or purpose.
Q3. Should I use Hungarian notation when naming variables in Go?
No, Hungarian notation is generally not recommended in Go. Instead, prefer using meaningful and descriptive names that accurately convey the purpose of the variable.
Q4. Is there a maximum length for Go identifiers?
Technically, Go does not impose a fixed maximum length for identifiers. However, it is considered a best practice to keep identifiers reasonably short and concise for improved readability.
In conclusion, following a consistent naming convention is crucial when programming in Go. Adhering to Go’s naming conventions ensures code readability, maintainability, and facilitates collaboration among developers. By carefully choosing names for variables, functions, and packages, developers can significantly enhance the overall quality of their code. Remember to keep the code concise, descriptive, and visually consistent to reap the full benefits of Go’s naming conventions.
Golang Struct Name Lowercase
When working with Go, one of the first things you encounter is its unique naming convention for structs. Unlike other programming languages, Go prefers struct names to start with a lowercase letter. This unconventional approach may puzzle newcomers, but it carries its own set of advantages and adheres to Go’s philosophy of simplicity and clarity. In this article, we will delve into the reasoning behind Golang struct name lowercase and discuss best practices for naming structs in your Go code.
Understanding the Lowercase Convention:
The Go programming language emphasizes simplicity and readability in its code. The designers of Go aimed to eliminate syntactic clutters and highlight the most essential elements of a program. By adopting lowercase struct names, Go promotes a uniform and concise naming convention that stays true to its principles.
Benefits of Lowercase Struct Names:
1. Unifies Naming Style:
Having lowercase struct names helps in ensuring a uniform naming style throughout the Go codebase. By adhering to a consistent convention, it becomes easier for developers to identify and differentiate between structs, variables, and functions.
2. Enhances Readability:
Go prioritizes code readability, and lowercase struct names contribute to this goal. With struct names in lowercase, it becomes instantly clear that we are dealing with a type definition and not a function or variable. It eliminates any ambiguity and helps to maintain clarity within the codebase, making it easier for other developers to understand and maintain the code.
3. Reduces Visual Noise:
By using lowercase struct names, Go avoids introducing unnecessary visual noise into the code. Lowercase struct names are shorter and less distracting, allowing developers to focus on more crucial aspects of the code. This decision aligns with Go’s philosophy of minimalism and encourages programmers to keep their code as clear and concise as possible.
Naming Conventions for Structs:
While Go encourages lowercase struct names, there are still some guidelines to follow when naming them:
1. Capitalize acronyms:
If the struct name consists of an acronym, capitalize all the letters. For example, HTTPHandler is preferred over HttpHandler.
2. Use descriptive names:
Choose clear and meaningful names that accurately represent the purpose and behavior of the struct. Avoid generic names like “data” or “info” and strive for descriptive names that reflect the specific role of the struct.
3. Use camel case for multi-word names:
If your struct name includes multiple words, use camel case. For example, userData, employeeDetails, or customerRecord.
4. Avoid excessive verbosity:
While descriptive names are essential, avoid excessive verbosity that may hinder code comprehension. Strike a balance between descriptive and concise names to ensure readability without unnecessary fluff.
Now that we have explored the rationale and best practices behind Golang struct name lowercase, let’s address some commonly asked questions:
FAQs – Frequently Asked Questions:
Q1. Can we use uppercase struct names in Go?
A1. Although you can technically use uppercase struct names in Go, it is not in line with the language’s naming convention and is discouraged. It is best to stick to the lowercase convention to maintain consistency and readability.
Q2. Are there any performance benefits to using lowercase struct names?
A2. The use of lowercase struct names does not have any direct impact on the performance of the code. It mainly focuses on readability and maintainability.
Q3. How should I name exported structs?
A3. Exported structs that need to be accessible from other packages should be named with an uppercase letter. This allows them to be exported and imported by other files.
Q4. Do the lowercase struct names apply to methods as well?
A4. No, the lowercase struct name convention only applies to the struct definition itself. Methods associated with the struct can still be named using camel case, following the convention of uppercase for exported methods and lowercase for unexported methods.
Q5. Can I use lowercase struct names in other programming languages?
A5. The use of lowercase struct names is specific to Go and its unique design choices. Other programming languages may have different conventions and standards for struct naming, so it is essential to follow the conventions of each language you work with.
In conclusion, the Golang struct name lowercase convention may initially appear unusual to those coming from other programming languages. However, by adhering to this naming convention, Go promotes code consistency, readability, and minimalism. By understanding and following the established guidelines for struct naming, you can create clean and maintainable code that aligns with the core principles of the Go programming language.
Images related to the topic golang struct name convention
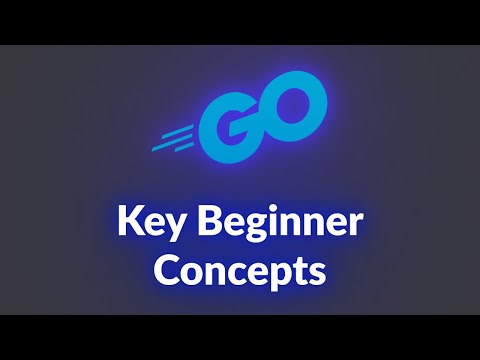
Found 41 images related to golang struct name convention theme
![Structs and Embedding in Go — Golang Zero to Hero Full Course [08] - YouTube Structs And Embedding In Go — Golang Zero To Hero Full Course [08] - Youtube](https://i.ytimg.com/vi/rguduxb71qM/maxresdefault.jpg)
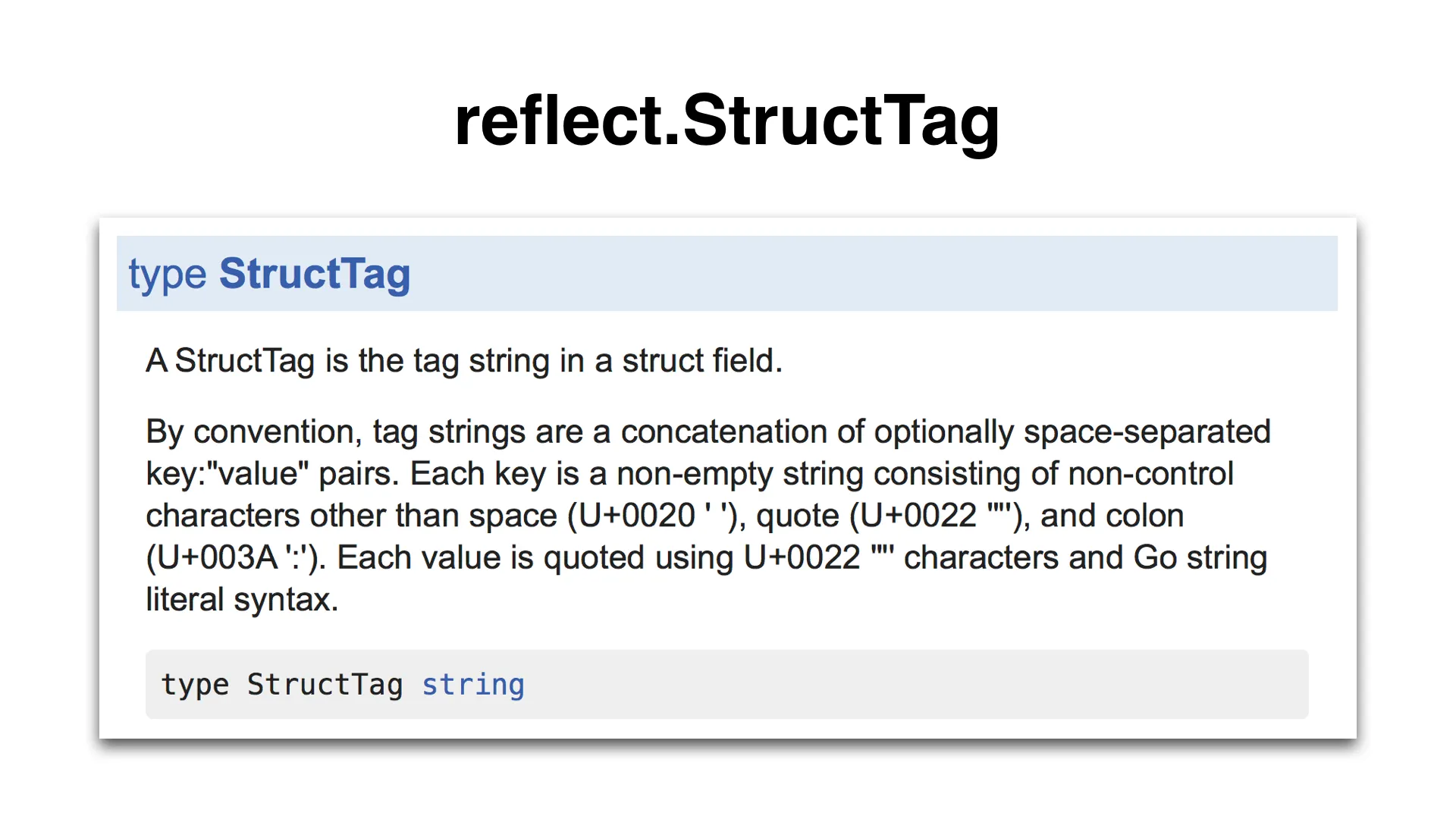

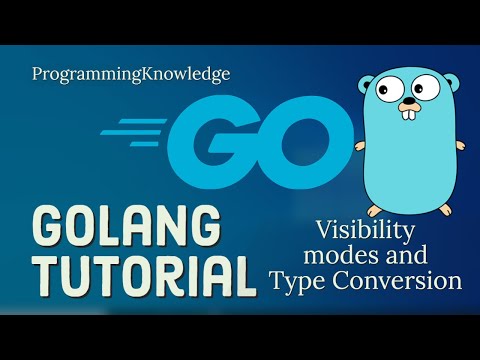


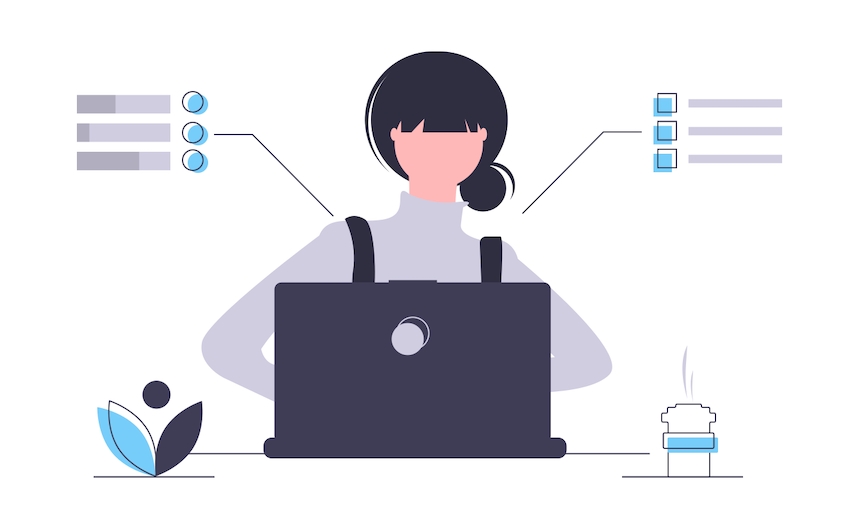
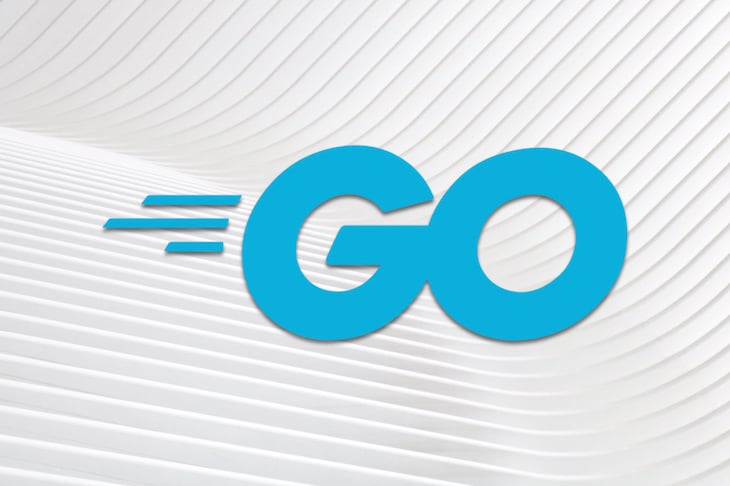
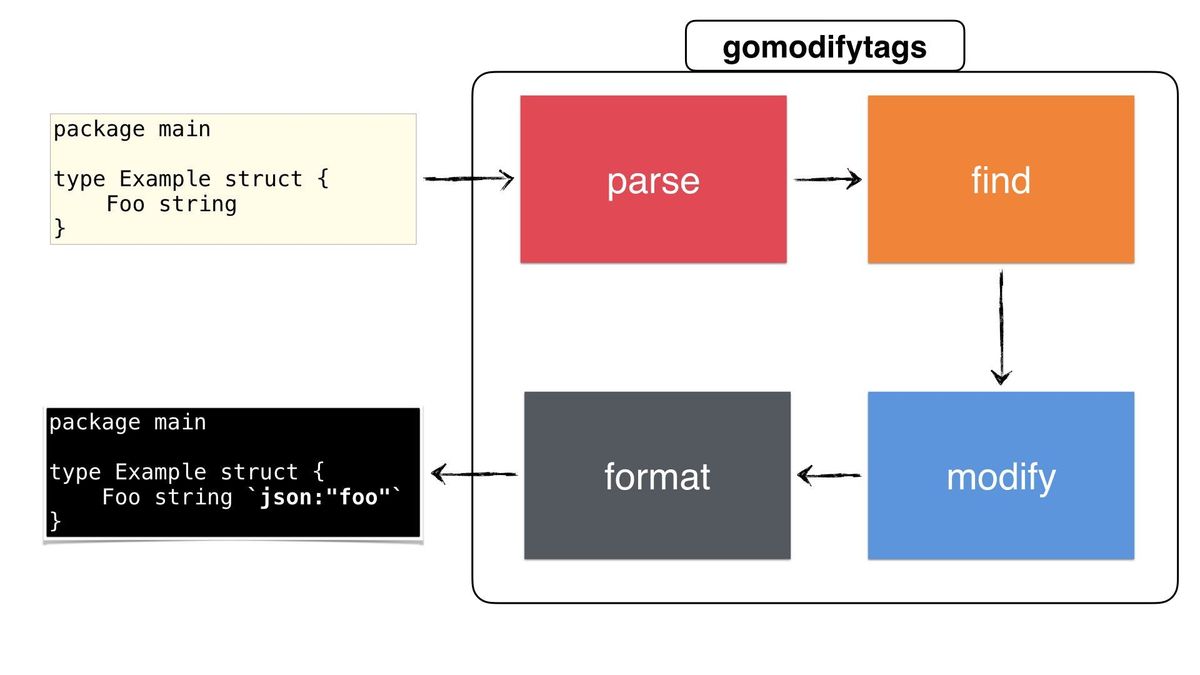
Article link: golang struct name convention.
Learn more about the topic golang struct name convention.
- What are Golang Struct Field Naming Conventions?
- Naming Conventions in Go: Short but Descriptive | by Dhia
- Go Development | Compass by Nimble
- Golang Naming Conventions – Dev Genius
- What are Golang Struct Field Naming Conventions?
- What is Blank Identifier(underscore) in Golang? – GeeksforGeeks
- Naming conventions – Getting Help – Go Forum
- Effective Go – The Go Programming Language
- How to name structs, functions, variables etc ? : r/golang – Reddit
- Golang type naming convention – Google Groups
- Go – What are Golang Struct Field Naming Conventions
- What are Golang Struct Field Naming Conventions?
- GoLang Naming Rules and Conventions | by Kassim Damilola
See more: nhanvietluanvan.com/luat-hoc