Add Empty Column To Dataframe Pandas
## Create a DataFrame Using pandas
Before diving into adding an empty column, let’s first understand how to create a DataFrame using pandas. A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. Pandas provides various methods to create a DataFrame. Here’s an example to create a simple DataFrame:
“`python
import pandas as pd
# Creating a DataFrame using a Python dictionary
data = {
‘Name’: [‘John’, ‘Emma’, ‘Ben’, ‘Sara’],
‘Age’: [25, 32, 47, 28],
‘City’: [‘New York’, ‘London’, ‘Paris’, ‘Tokyo’]
}
df = pd.DataFrame(data)
print(df)
“`
Output:
“`
Name Age City
0 John 25 New York
1 Emma 32 London
2 Ben 47 Paris
3 Sara 28 Tokyo
“`
## Select Specific Columns from the DataFrame
To perform specific operations on columns, we sometimes need to select specific columns from the DataFrame. Pandas provides several ways to select specific columns. Here are a few examples:
“`python
# Selecting a single column
name_col = df[‘Name’]
# Selecting multiple columns
name_age_cols = df[[‘Name’, ‘Age’]]
# Selecting columns using index positions
name_age_cols_index = df.iloc[:, 0:2]
# Selecting columns using column names and index positions
name_age_cols_mix = df.loc[:, [‘Name’, ‘Age’]]
“`
## Add an Empty Column to the DataFrame
To add an empty column to a DataFrame, we can assign an empty list, `None`, or use the `pd.NA` value. Let’s see how to add an empty column using these techniques:
“`python
# Adding an empty column using assignment
df[‘Salary’] = []
# Adding an empty column using None
df[‘Address’] = None
# Adding an empty column using pd.NA
df[‘Phone’] = pd.NA
print(df)
“`
Output:
“`
Name Age City Salary Address Phone
0 John 25 New York [] None
1 Emma 32 London [] None
2 Ben 47 Paris [] None
3 Sara 28 Tokyo [] None
“`
## Add Data to the Empty Column
Once we have added an empty column, we might want to populate it with data later. We can achieve this by assigning values to the empty column. Here’s an example:
“`python
# Adding data to the empty column
df[‘Salary’] = [5000, 7000, 6000, 9000]
df[‘Address’] = [‘123 Main St’, ‘456 Elm St’, ‘789 Oak St’, ‘321 Pine St’]
df[‘Phone’] = [‘123-456-7890’, ‘987-654-3210’, ‘555-123-4567’, ‘999-888-7777’]
print(df)
“`
Output:
“`
Name Age City Salary Address Phone
0 John 25 New York 5000 123 Main St 123-456-7890
1 Emma 32 London 7000 456 Elm St 987-654-3210
2 Ben 47 Paris 6000 789 Oak St 555-123-4567
3 Sara 28 Tokyo 9000 321 Pine St 999-888-7777
“`
## Insert the Empty Column at a Specific Position
By default, when we add an empty column, it is inserted at the end of the DataFrame. However, we can also insert it at a specific position using the `insert()` method. The `insert()` method takes the position as the first argument and the column name as the second argument. Here’s an example:
“`python
# Inserting an empty column at position 2
df.insert(2, ‘Department’, None)
print(df)
“`
Output:
“`
Name Age Department City Salary Address Phone
0 John 25 None New York 5000 123 Main St 123-456-7890
1 Emma 32 None London 7000 456 Elm St 987-654-3210
2 Ben 47 None Paris 6000 789 Oak St 555-123-4567
3 Sara 28 None Tokyo 9000 321 Pine St 999-888-7777
“`
## Drop the Empty Column from the DataFrame
If we want to remove the empty column from the DataFrame, we can use the `drop()` method. The `drop()` method takes the column name as the argument and removes the column from the DataFrame. Here’s an example:
“`python
# Dropping the empty column ‘Department’
df = df.drop(‘Department’, axis=1)
print(df)
“`
Output:
“`
Name Age City Salary Address Phone
0 John 25 New York 5000 123 Main St 123-456-7890
1 Emma 32 London 7000 456 Elm St 987-654-3210
2 Ben 47 Paris 6000 789 Oak St 555-123-4567
3 Sara 28 Tokyo 9000 321 Pine St 999-888-7777
“`
## Update the Empty Column with New Data
To update the empty column with new data, we can assign values to the column as we did before. Here’s an example:
“`python
# Updating the ‘Salary’ column
df[‘Salary’] = [5500, 7200, 6100, 9200]
print(df)
“`
Output:
“`
Name Age City Salary Address Phone
0 John 25 New York 5500 123 Main St 123-456-7890
1 Emma 32 London 7200 456 Elm St 987-654-3210
2 Ben 47 Paris 6100 789 Oak St 555-123-4567
3 Sara 28 Tokyo 9200 321 Pine St 999-888-7777
“`
## FAQs
### Q1: How can I drop a column from a DataFrame in pandas?
To drop a column from a DataFrame, you can use the `drop()` method with the `axis=1` parameter. For example: `df = df.drop(‘ColumnName’, axis=1)`.
### Q2: What is the difference between None and pd.NA when adding an empty column?
`None` is the Python object representing null values, while `pd.NA` is a scalar missing value introduced in pandas 1.0. `pd.NA` is designed to be used consistently across different data types.
### Q3: How can I replace a string in a specific column of a DataFrame in pandas?
To replace a string in a specific column of a DataFrame, you can use the `replace()` method. For example: `df[‘Column’] = df[‘Column’].replace(‘old_string’, ‘new_string’)`.
### Q4: How can I add a new row to a DataFrame in pandas?
To add a new row to a DataFrame, you can use the `append()` method or create a new DataFrame and concatenate it using `pd.concat()`. For example: `df.append({‘Column1’: value1, ‘Column2’: value2}, ignore_index=True)`.
### Q5: How can I assign a value to a specific cell in a DataFrame in pandas?
To assign a value to a specific cell in a DataFrame, you can use the indexing notation. For example: `df.at[row_index, ‘Column’] = value`.
### Q6: How can I create a column filled with zeros in a DataFrame in pandas?
To create a column filled with zeros in a DataFrame, you can use the assignment notation with the list of zeros. For example: `df[‘Column’] = [0] * len(df)`.
### Q7: How can I get a specific column from a DataFrame in pandas?
To get a specific column from a DataFrame, you can use the indexing notation. For example: `df[‘Column’]`.
### Q8: How can I assign a value to an entire DataFrame in pandas?
To assign a value to an entire DataFrame, you can use the assignment notation. For example: `df = value` or `df[:] = value`.
In this article, we covered how to add an empty column to a DataFrame in pandas, insert it at a specific position, add data to it, drop the column, update the column with new data, and performed related operations such as dropping columns, adding columns, replacing strings in columns, adding rows, assigning values to cells, creating zero columns, getting columns, and assigning values to entire DataFrames. Utilize these techniques to enhance your data analysis tasks efficiently using pandas.
Pandas Add Empty Column In The Dataframe|Pandas Python|Pandas Add Column To Dataframe
How To Add Column To Dataframe Pandas?
Pandas is a powerful data manipulation and analysis library in Python. It provides numerous functionalities to work with structured data, including the ability to add columns to a DataFrame. In this article, we will explore different ways to add a column to a DataFrame using pandas.
Adding a column to a DataFrame can be useful when you want to perform additional calculations, combine multiple columns, or insert new data. Pandas provides multiple approaches to achieve this, so let’s dive into each method.
Method 1: Using Assignment Operator
One of the simplest ways to add a column to a DataFrame is by using the assignment operator (=). Suppose we have a DataFrame called df and we want to add a new column called ‘new_col’ with values [1,2,3,4]:
“` python
import pandas as pd
df = pd.DataFrame({‘old_col’: [‘A’, ‘B’, ‘C’, ‘D’]})
df[‘new_col’] = [1, 2, 3, 4]
“`
The above code will add a column named ‘new_col’ to the existing DataFrame. The values [1, 2, 3, 4] will be assigned to each respective row.
Method 2: Using DataFrame.insert()
The DataFrame.insert() method allows you to insert a column at a specific position. This method takes three arguments – loc, column, and value. The loc parameter specifies the position where you want to insert the column (0 for the first position), column is the name of the column, and value contains the values to be inserted. Here’s an example:
“` python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3, 4], ‘B’: [5, 6, 7, 8]})
df.insert(loc=1, column=’new_col’, value=[9, 10, 11, 12])
“`
In the above code, the column ‘new_col’ is inserted as the second column in the DataFrame. The values [9, 10, 11, 12] will be assigned to the respective rows.
Method 3: Using DataFrame.assign()
The DataFrame.assign() method is used to create a new DataFrame with additional columns. It takes named arguments where the name of the argument is the column name, and the value is the new data. Here’s an example:
“` python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3, 4]})
df = df.assign(new_col=[5, 6, 7, 8])
“`
In this code, we create a new DataFrame called df with a column named ‘new_col’ and assign it the values [5, 6, 7, 8].
Method 4: Using DataFrame.assign() with function
The assign() method can also be used with functions to perform calculations or transformations on existing columns. Here’s an example:
“` python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3, 4]})
df = df.assign(new_col = lambda x: x[‘A’] * 2)
“`
In this code, we create a new column ‘new_col’ and assign the double of each value in column ‘A’. The lambda function takes each row as input and applies the desired transformation.
Method 5: Using Concatenation
Another way to add a column to a DataFrame is by concatenating two DataFrames. This can be useful when you have a separate DataFrame or Series that you want to merge with the existing DataFrame. Here’s an example:
“` python
import pandas as pd
df1 = pd.DataFrame({‘A’: [1, 2, 3, 4]})
df2 = pd.DataFrame({‘B’: [5, 6, 7, 8]})
df = pd.concat([df1, df2], axis=1)
“`
In this code, we create two separate DataFrames (df1 and df2) and then concatenate them using the pd.concat() function. The resulting DataFrame (df) will have both columns ‘A’ and ‘B’.
FAQs:
Q1: Can I add multiple columns to a DataFrame simultaneously?
Yes, you can add multiple columns to a DataFrame simultaneously using any of the methods mentioned earlier. Simply provide multiple column names and values within square brackets.
Q2: How can I add a column with default values?
If you want to add a column with default values, you can use any of the methods mentioned earlier and pass a list of default values as the value parameter.
Q3: Can I add a column at a specific index position?
Yes, you can add a column at a specific index position using the DataFrame.insert() method. Specify the desired position using the loc parameter when calling the insert() method.
Q4: Can I add columns from a different DataFrame?
Yes, you can add columns from a different DataFrame by concatenating the two DataFrames using the pd.concat() function, as shown in Method 5.
In conclusion, pandas provides several flexible methods to add columns to a DataFrame. Whether you need to insert a column at a specific position, assign values using a function, or combine multiple DataFrames, pandas has got you covered. Experiment with these methods to enhance your data manipulation capabilities using pandas.
How To Fill Empty Column In Pandas?
Introduction
Pandas is a popular data manipulation tool for Python, providing valuable functionalities for data analysis and cleaning. One common task is filling empty columns, which can arise due to missing values or incomplete data. In this article, we will explore various techniques to effectively fill empty columns in Pandas, ensuring accurate and reliable data analysis.
I. Understanding Empty Columns in Pandas
An empty column in Pandas refers to a column in a DataFrame that contains missing or null values. These values can negatively impact data analysis and modeling, often requiring us to handle them appropriately. By filling empty columns, we can address missing data and ensure comprehensive analysis.
II. Checking for Empty Columns
Before we dive into techniques for filling empty columns, it is crucial to identify whether any columns are empty. Pandas provides several functions to assess missing values in a DataFrame. The ‘isna()’ or ‘isnull()’ function returns a boolean DataFrame indicating where values are missing. We can then use the ‘any()’ function to check if any columns contain missing values.
III. Techniques to Fill Empty Columns
1. Filling with a Constant Value:
One simple approach is to fill empty columns with a constant value. We can use the ‘fill’ function, replacing all missing values in the column with a specific value. This technique is useful when the missing values do not carry significant information.
2. Forward Filling:
Forward filling, also known as the ‘ffill’ method, involves filling empty columns with the most recent non-null value in the column. This technique is particularly helpful when dealing with time-series data or sequential data. Forward filling retains the previous valid value to maintain the temporal order.
3. Backward Filling:
Opposite to forward filling, backward filling, or the ‘bfill’ method, fills empty columns with the next non-null value. Similar to forward filling, this method is beneficial for temporal or sequential data analysis. Backward filling utilizes the next valid value to maintain the temporal ordering within the column.
4. Interpolation:
Interpolation refers to filling empty columns by estimating values based on existing data. Pandas offers various interpolation techniques, such as linear, polynomial, or spline interpolation. These methods provide estimations between valid values, generating a smooth transition within the column.
5. Group-Specific Filling:
In some scenarios, filling empty columns with group-specific values might be necessary. Using the ‘groupby’ function, we can group data based on selected columns and fill missing values with statistics specific to each group. This technique is useful in data analysis scenarios where group properties play a significant role.
IV. Handling Data of Different Types
When dealing with diverse data types, it is crucial to choose appropriate techniques to fill empty columns. For numerical values, using statistical measures like mean, median, or mode can be effective. On the other hand, for categorical data, utilizing the most frequent category or even creating a new category for missing values might be appropriate.
V. Performance Considerations
Handling large datasets with many empty columns can impact performance. In such cases, it is advisable to use faster and memory-efficient techniques. For instance, the ‘fillna’ function in Pandas allows specifying the method as an argument, controlling how to fill empty columns. Additionally, replacing missing values in place, using the ‘inplace’ parameter, can optimize memory usage.
FAQs
Q1: What is the difference between forward filling and backward filling?
A1: Forward filling replaces missing values in empty columns with the most recent non-null value, while backward filling utilizes the next non-null value to fill missing values. These techniques maintain the temporal order within the column.
Q2: Can I fill empty columns with custom values rather than statistical measures?
A2: Yes, you can fill empty columns with custom constant values using the ‘fillna’ function in Pandas. This approach is suitable when missing values do not carry significant information.
Q3: How can I handle empty columns with different data types?
A3: For numerical data, statistical measures like mean, median, or mode can be used to fill missing values. For categorical data, utilizing the most frequent category or creating a new category for missing values is appropriate.
Q4: What if I have large datasets with many empty columns?
A4: When dealing with large datasets, consider using memory-efficient techniques like specifying the filling method in the ‘fillna’ function or using the ‘inplace’ parameter to optimize memory usage.
Conclusion:
Filling empty columns in Pandas is a crucial step in preparing data for analysis. By employing appropriate techniques such as constant value filling, forward filling, backward filling, interpolation, or group-specific filling, we can mitigate the impact of missing values on data analysis. Furthermore, considering the data types and performance optimizations can enhance efficiency when handling large datasets. Pandas provides a wide range of methods and functions to address the challenge of empty columns, ensuring accurate and reliable data analysis and modeling.
Keywords searched by users: add empty column to dataframe pandas Drop column pandas, Add column pandas, Pandas replace string in column, Add row to DataFrame, Add value to row pandas, Create zero column pandas, Get column in pandas, Assign value to DataFrame
Categories: Top 12 Add Empty Column To Dataframe Pandas
See more here: nhanvietluanvan.com
Drop Column Pandas
Introduction:
In data analysis and manipulation, pandas is a widely-used Python library that offers a powerful and flexible toolset. One common task involves dropping columns from a DataFrame. Whether you want to get rid of unnecessary or irrelevant data, or simply remove columns that are not required for your analysis, the drop column function in pandas allows for efficient and straightforward removal of specific columns from a DataFrame. In this article, we will explore the various ways to drop columns using the pandas library, along with examples and best practices.
Removing columns using the drop() method:
The drop() method in pandas provides a convenient way to remove one or more columns from a DataFrame. It requires the column name(s) to be specified as the argument(s), and an optional parameter, axis, to determine whether the operation should be performed along the columns (axis=1) or rows (axis=0) of the DataFrame.
Syntax:
DataFrame.drop(labels, axis=1, inplace=False)
By default, the drop() method returns a new DataFrame with the specified column(s) removed. However, if the inplace parameter is set to True, the operation will be performed directly on the original DataFrame, altering it permanently.
Example 1: Removing a single column
Let’s say we have a DataFrame called df, with columns A, B, and C. To remove column C, we can use the drop() method as follows:
“`
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]})
df.drop(‘C’, axis=1, inplace=True)
print(df)
“`
Output:
“`
A B
0 1 4
1 2 5
2 3 6
“`
In this example, the column ‘C’ is dropped from the DataFrame, resulting in a modified DataFrame with only columns ‘A’ and ‘B’.
Example 2: Removing multiple columns
To drop multiple columns simultaneously, you can pass a list of column names to the drop() method. Let’s consider the following DataFrame with columns ‘A’, ‘B’, ‘C’, and ‘D’:
“`
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9],
‘D’: [10, 11, 12]})
df.drop([‘B’, ‘D’], axis=1, inplace=True)
print(df)
“`
Output:
“`
A C
0 1 7
1 2 8
2 3 9
“`
In this example, the columns ‘B’ and ‘D’ are dropped, resulting in a modified DataFrame with only columns ‘A’ and ‘C’.
Dropping columns based on conditions:
In addition to dropping columns by specifying their names, pandas also allows you to remove columns based on certain conditions. This can be achieved using boolean indexing.
Example 3: Removing columns based on conditions
Suppose we have a DataFrame with columns ‘A’, ‘B’, and ‘C’, with values ranging from 0 to 9:
“`
import pandas as pd
df = pd.DataFrame({‘A’: range(10),
‘B’: range(10, 20),
‘C’: range(20, 30)})
df.drop(df.columns[df.sum() < 30], axis=1, inplace=True) print(df) ``` Output: ``` C 0 20 1 21 2 22 3 23 4 24 5 25 6 26 7 27 8 28 9 29 ``` In this example, we drop the columns where the sum of their elements is less than 30. Only the column 'C' satisfies this condition, so all other columns are dropped. FAQs: Q1: Can I drop columns based on their index position rather than their names? Yes, you can drop columns using their index position instead of names by specifying the integer positions of the columns you want to drop. Q2: Will the drop() method affect the original DataFrame if the inplace parameter is set to False? No, if you set the inplace parameter to False (the default value), the drop() method will return a new DataFrame without modifying the original DataFrame. Q3: Can I drop multiple columns using a mixed approach of column names and index positions? Yes, you can combine column names and index positions in the same list passed to the drop() method. However, this approach can quickly become complex and less readable, so it is recommended to stick with either names or index positions for better code clarity. Q4: How can I drop columns when their names contain spaces or special characters? Columns with spaces or special characters can be dropped using the same drop() method. Simply provide the exact column name or a list of names as arguments, including the spaces or special characters. Conclusion: The drop column functionality in pandas provides a flexible and efficient way to remove unnecessary columns from a DataFrame. By using the drop() method, you can remove columns based on their names, index positions, or even specific conditions. Understanding how to effectively drop columns in pandas empowers data analysts and helps streamline data preparation and analysis workflows.
Add Column Pandas
(Word count: 1102)
Introduction:
Pandas is a powerful data manipulation tool built on top of the Python programming language. It provides easy-to-use data structures and data analysis tools that make working with structured data efficient and intuitive. One crucial operation when working with data is adding a new column to an existing DataFrame. In this article, we will explore how to add a column in pandas and some common use cases for this operation.
Adding a Column in Pandas:
In pandas, a DataFrame is a two-dimensional data structure that consists of rows and columns. Adding a new column to an existing DataFrame allows us to store and manipulate additional data related to the existing dataset. There are several ways to add a column in pandas, depending on the requirements and complexity of the data transformation.
Method 1: Direct Assignment
The simplest way to add a new column to a pandas DataFrame is by directly assigning values to it. We can use either a scalar value to assign the same value to all rows or an iterable object (e.g., a list, a NumPy array) to assign different values to each row. For example:
“`python
import pandas as pd
# Create a DataFrame
data = {‘Name’: [‘John’, ‘Emma’, ‘Mike’],
‘Age’: [25, 28, 32]}
df = pd.DataFrame(data)
# Add a new column
df[‘City’] = [‘London’, ‘New York’, ‘Sydney’]
print(df)
“`
Output:
“`
Name Age City
0 John 25 London
1 Emma 28 New York
2 Mike 32 Sydney
“`
Method 2: Using the `assign()` method
Pandas provides the `assign()` method, which allows us to add a new column while creating a new DataFrame. This method returns a new DataFrame with the added column, without modifying the original DataFrame. It is useful when we want to keep the original DataFrame unaltered. For example:
“`python
import pandas as pd
# Create a DataFrame
data = {‘Name’: [‘John’, ‘Emma’, ‘Mike’],
‘Age’: [25, 28, 32]}
df = pd.DataFrame(data)
# Add a new column using assign()
df_new = df.assign(City=[‘London’, ‘New York’, ‘Sydney’])
print(df_new)
“`
Output:
“`
Name Age City
0 John 25 London
1 Emma 28 New York
2 Mike 32 Sydney
“`
Method 3: Applying a Function across Rows or Columns
Sometimes, we may need to compute values for a new column based on existing columns. Pandas provides the `apply()` method, which allows us to apply a custom function across rows or columns of a DataFrame. This method is particularly useful when we want to perform complex transformations on the data. For example, let’s say we want to add a new column that contains the full names of individuals based on their first and last names:
“`python
import pandas as pd
# Create a DataFrame
data = {‘First Name’: [‘John’, ‘Emma’, ‘Mike’],
‘Last Name’: [‘Doe’, ‘Smith’, ‘Johnson’]}
df = pd.DataFrame(data)
# Create a function to concatenate first and last names
def get_full_name(row):
return row[‘First Name’] + ‘ ‘ + row[‘Last Name’]
# Apply the function and add a new column
df[‘Full Name’] = df.apply(get_full_name, axis=1)
print(df)
“`
Output:
“`
First Name Last Name Full Name
0 John Doe John Doe
1 Emma Smith Emma Smith
2 Mike Johnson Mike Johnson
“`
Frequently Asked Questions (FAQs):
Q1: Can I add a column based on conditions in pandas?
Ans: Yes, you can add a column based on certain conditions in pandas. You can use the `numpy.where()` function or the `DataFrame.loc` accessor to select rows based on conditions and assign values to the new column accordingly.
Q2: Can I add multiple columns at once in pandas?
Ans: Yes, you can add multiple columns at once in pandas. You can directly assign a list of values or an array to multiple columns using the direct assignment method. Alternatively, you can use the `assign()` method to add multiple columns simultaneously.
Q3: How do I add a column with default values in pandas?
Ans: To add a column with default values in pandas, you can assign the same default value to all rows during the column addition process. By specifying the default value as a scalar, it gets assigned to all rows of the added column.
Q4: Does adding a column modify the original DataFrame?
Ans: When using direct assignment or the `assign()` method, a new column is added to the DataFrame without modifying the original DataFrame. However, when using the `apply()` method, the original DataFrame is modified as the computed values are added as a new column.
Conclusion:
Adding a column to a pandas DataFrame is a common operation that allows us to enrich and manipulate our data for further analysis. Pandas provides multiple methods to add a column, catering to different use cases and data transformation requirements. Whether it’s direct assignment, using the `assign()` method, or applying custom functions, pandas makes the process efficient and intuitive.
Pandas Replace String In Column
## Understanding the Problem
Before delving into the methods of replacing strings in pandas, it’s essential to grasp the problem at hand. Let’s consider a typical scenario where you have a dataset with a column containing strings, and you want to replace a specific substring or string within that column with another value. For instance, you might have a column that contains names of countries, but some entries have typographical errors or inconsistent spellings. To address this, you could replace those incorrect strings with the correct ones.
## Pandas’ Replace Function
To tackle string replacement in pandas, the `replace()` function comes in handy. This function allows you to replace specified strings within a column with a desired value. The basic syntax for using `replace()` is as follows:
“`python
df[‘column_name’] = df[‘column_name’].replace(‘old_string’, ‘new_string’)
“`
Using this syntax, you can replace all occurrences of the ‘old_string’ with the ‘new_string’ in the specified column (in this case, ‘column_name’). It’s important to note that the `replace()` function only replaces exact matches, so it is case-sensitive.
## Replacing Substrings
Sometimes, you may need to replace only a part of the string within a column. Pandas provides the `str.replace()` method to accomplish this. Using `str.replace()`, you can replace sub-strings within a column with a specified value. This method is particularly useful when you want to modify only certain portions of the strings in your data.
“`python
df[‘column_name’] = df[‘column_name’].str.replace(‘old_substring’, ‘new_substring’)
“`
By using `str.replace()`, all instances of the ‘old_substring’ within the ‘column_name’ will be replaced with ‘new_substring’. Similar to the `replace()` function, this method is also case-sensitive.
## Regular Expressions for Advanced Replacement
In more complex scenarios, you might need to perform advanced replacements based on patterns rather than exact matches. For this purpose, pandas’ `replace()` function supports regular expressions. Regular expressions (regex) provide a powerful way to define patterns and match text within strings.
To utilize regex for replacement, set the `regex` parameter to `True` in the `replace()` function. Here’s an example:
“`python
df[‘column_name’] = df[‘column_name’].replace(r’pattern’, ‘replacement’, regex=True)
“`
In this example, the `r’pattern’` represents the regular expression pattern you want to match within the ‘column_name’, and ‘replacement’ specifies the value to replace those matched patterns with. By activating the `regex` flag, pandas will interpret the ‘pattern’ as a regular expression.
Regex opens up a wide array of possibilities, allowing you to perform complex replacements based on defined patterns. For instance, you can simultaneously replace all occurrences of multiple strings with different replacements in a single go.
## Handling NaN and Missing Values
When working with data, it’s common to encounter missing or NaN (Not a Number) values. When performing string replacements in pandas, by default, NaN values remain as NaN in the resulting dataframe. However, you can explicitly define how to handle missing values during replace operations using `fillna()` function.
“`python
df[‘column_name’] = df[‘column_name’].replace(‘old_string’, ‘new_string’).fillna(‘default_value’)
“`
In this example, any missing values resulting from the replacements will be filled with ‘default_value’. This step ensures that missing values are accounted for appropriately in your data.
## FAQs
**Q: Can I replace strings across multiple columns simultaneously?**
A: Yes, you can replace strings across multiple columns simultaneously by applying `replace()` function to each column separately.
**Q: How can I perform case-insensitive replacements?**
A: By using regex, you can set the `re.IGNORECASE` flag to perform case-insensitive replacements. For example,
“`python
df[‘column_name’] = df[‘column_name’].replace(r’pattern’, ‘replacement’, regex=True, flags=re.IGNORECASE)
“`
**Q: Does string replacement modify the original dataframe?**
A: Yes, using the syntax `df[‘column_name’] = df[‘column_name’].replace(…)`, the original dataframe is modified. If you want to keep the original dataframe untouched, consider creating a copy before applying string replacements.
## Conclusion
Pandas provides efficient and flexible methods to replace strings in columns. Whether you want to replace exact matches, substrings, or employ regular expressions for advanced replacements, pandas has got you covered. Understanding these methods will enable you to perform accurate, consistent, and error-free data manipulations. So, the next time you need to replace strings within a column in pandas, remember the functions we covered here and apply them to suit your specific requirements.
Images related to the topic add empty column to dataframe pandas
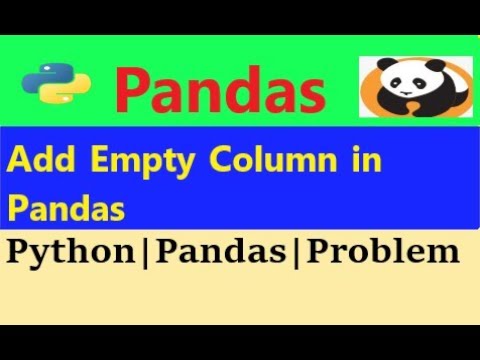
Found 31 images related to add empty column to dataframe pandas theme
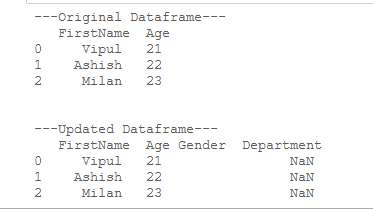
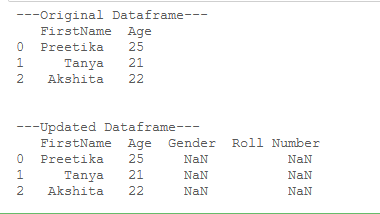
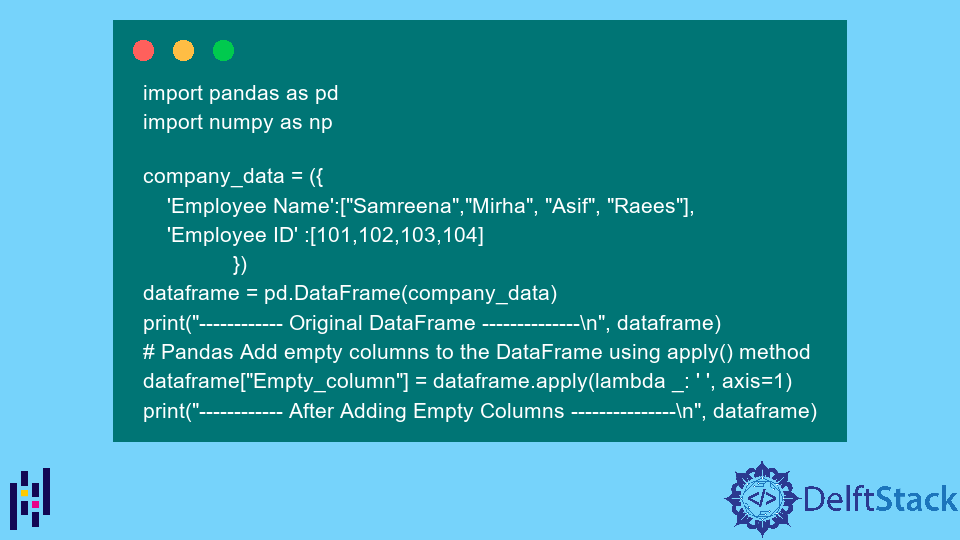

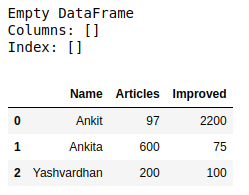
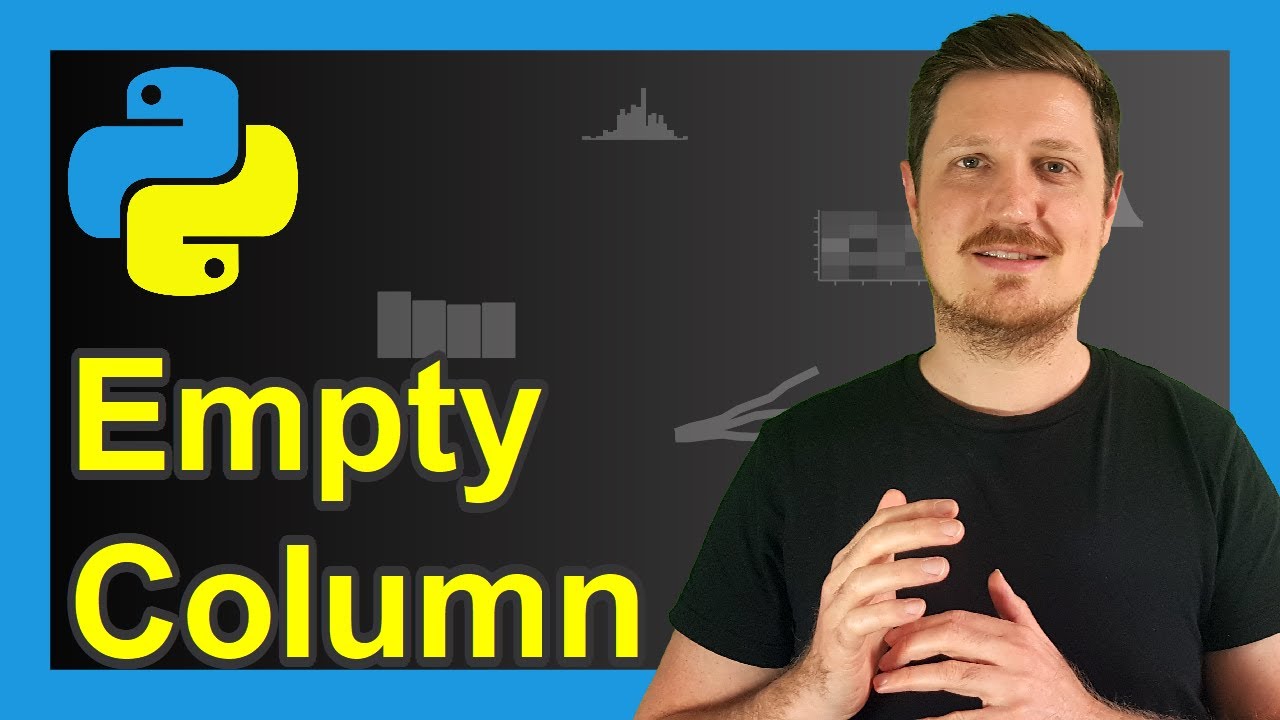



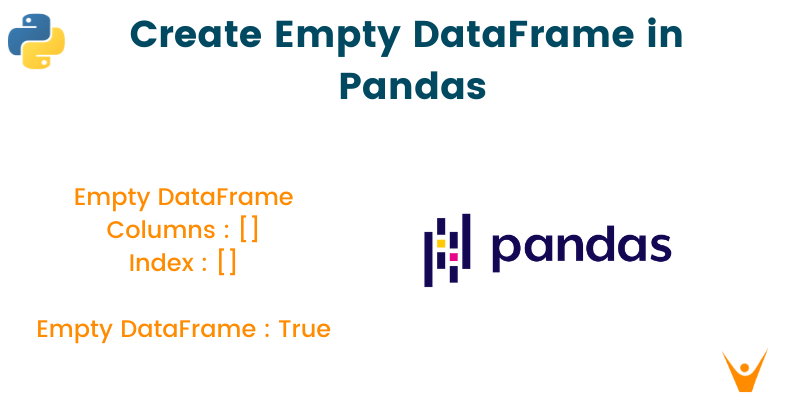

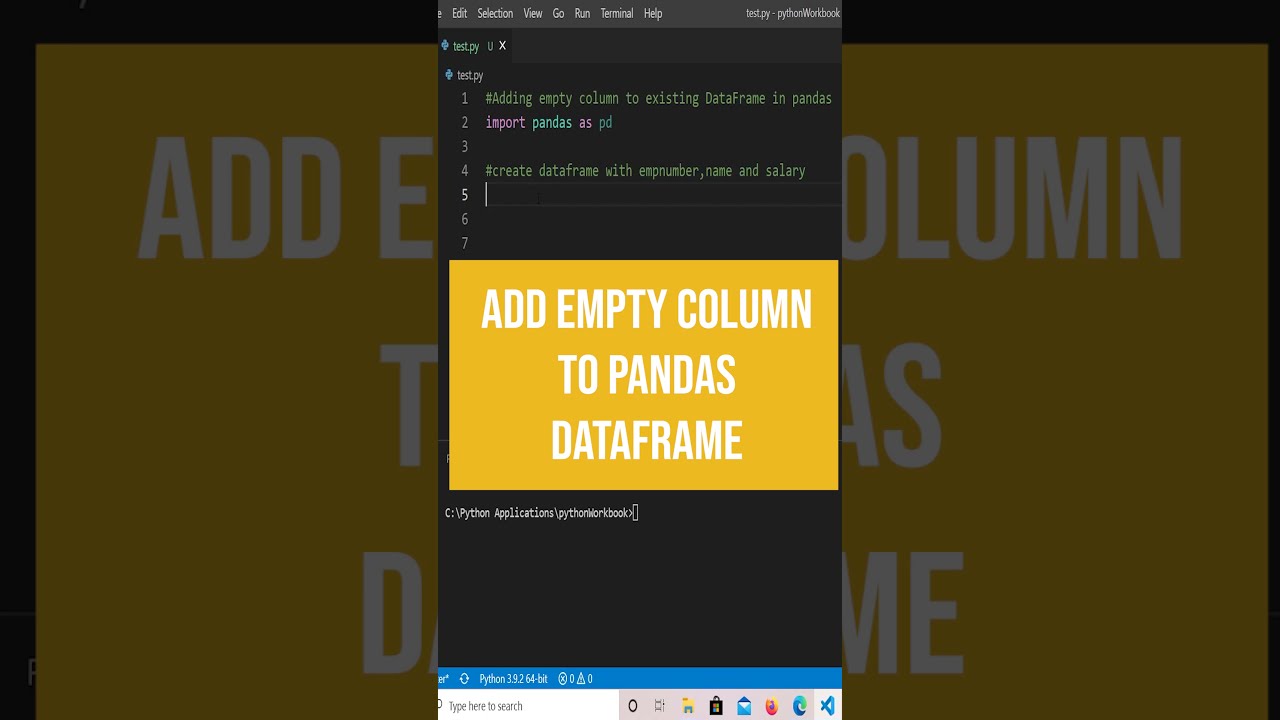
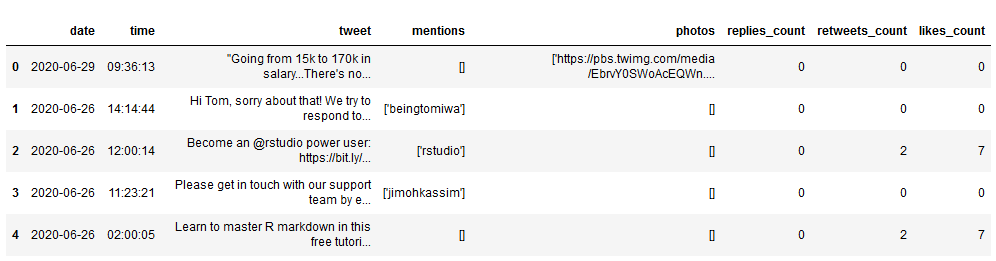
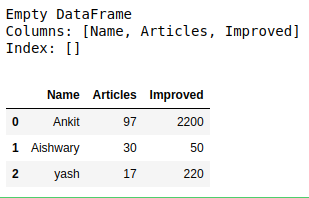



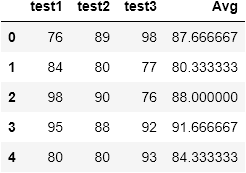
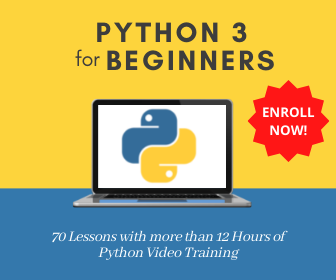


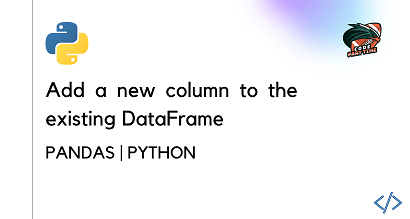
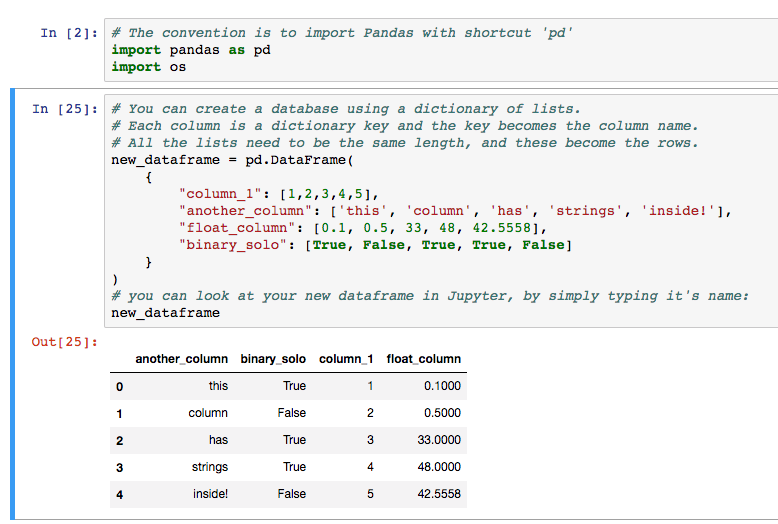

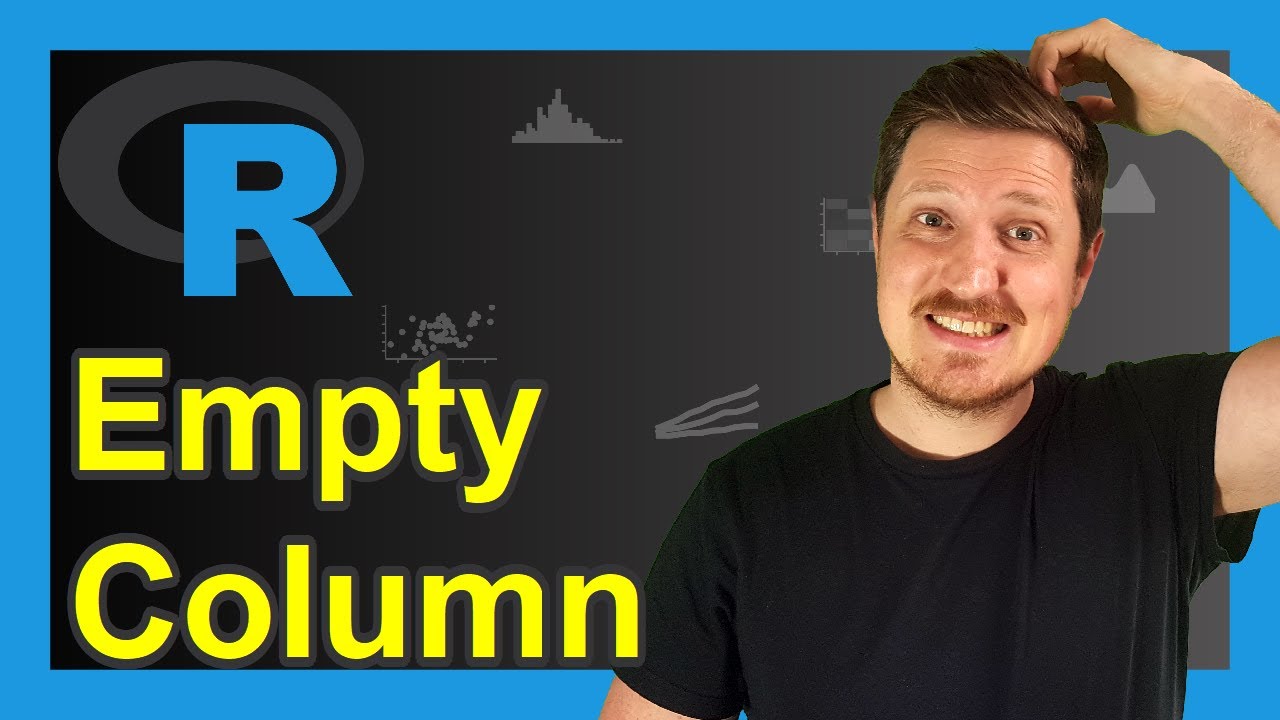
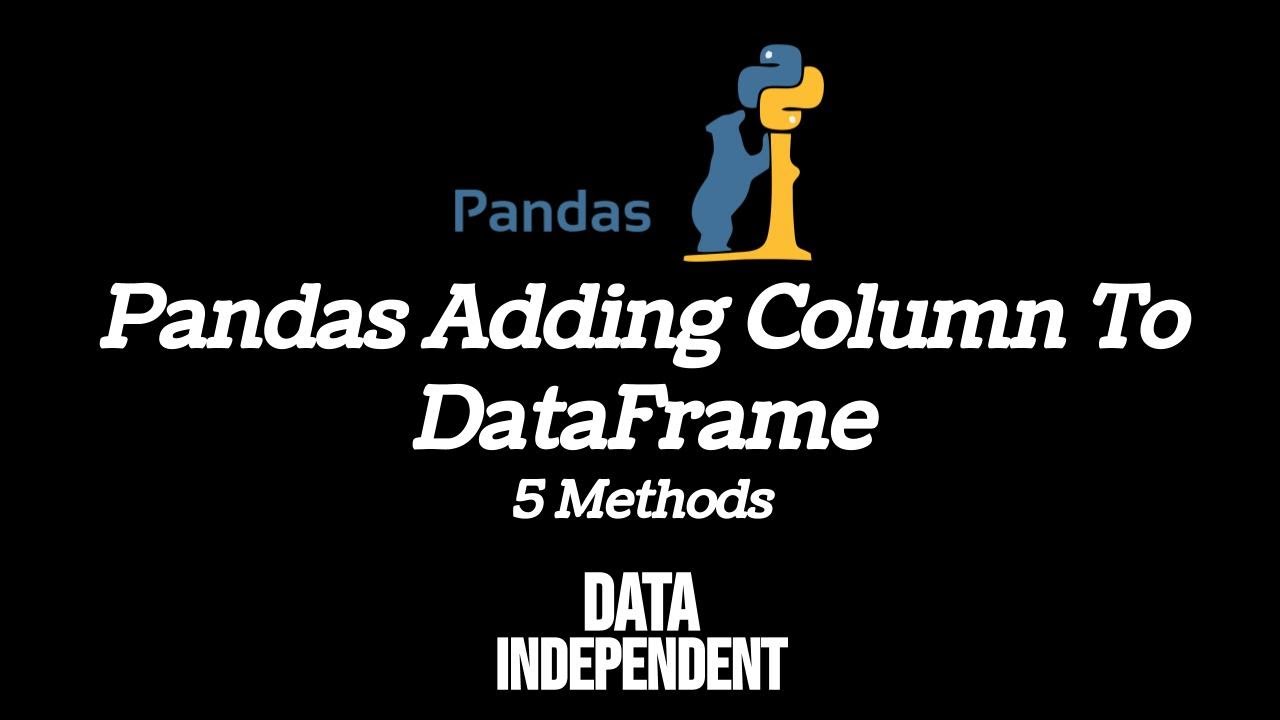
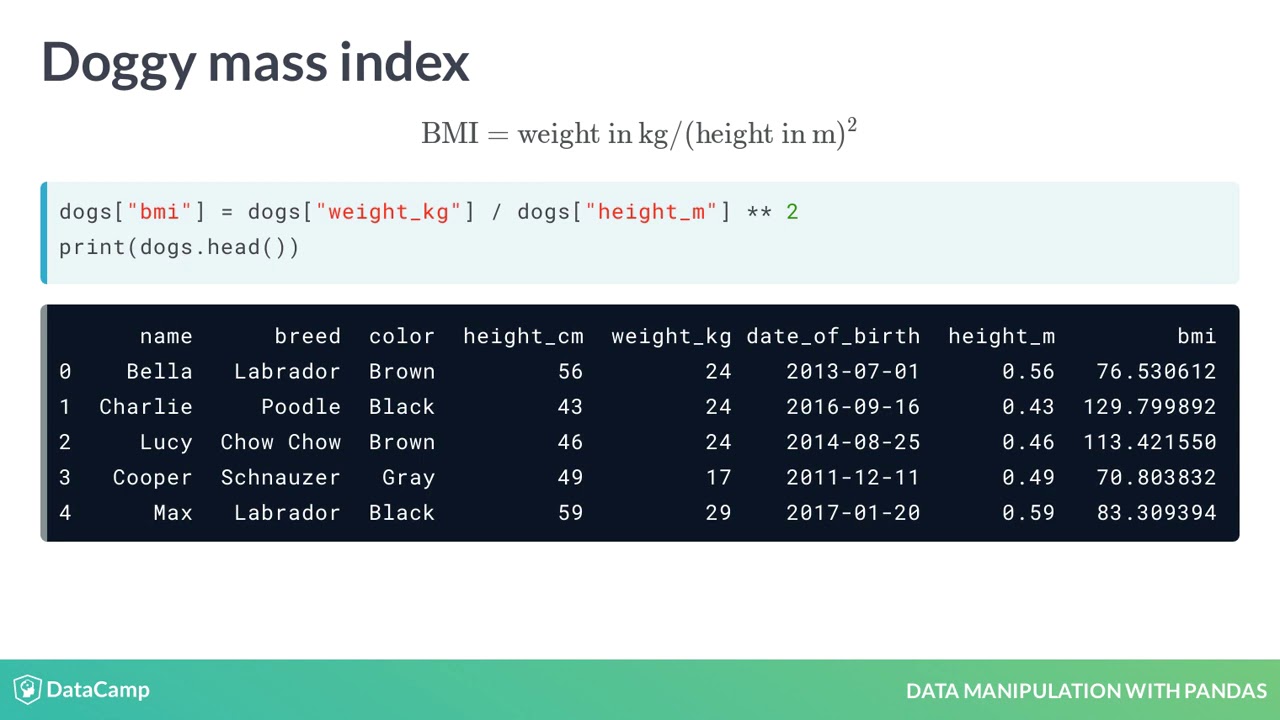
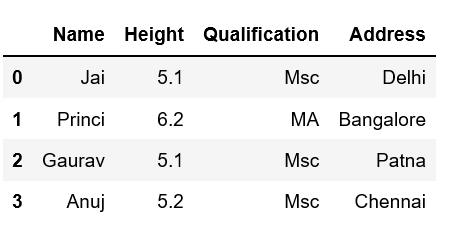

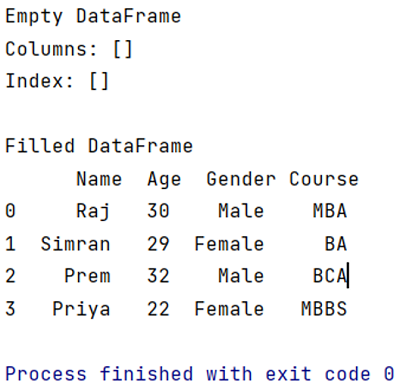
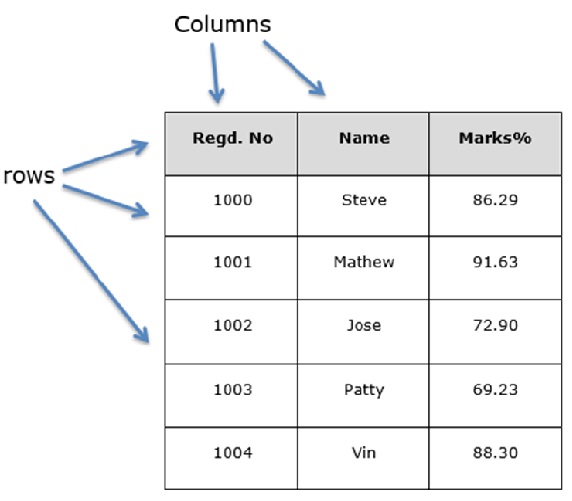
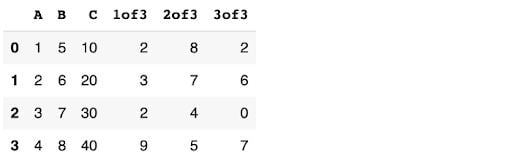
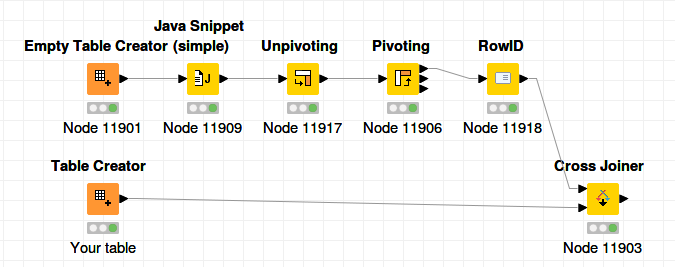


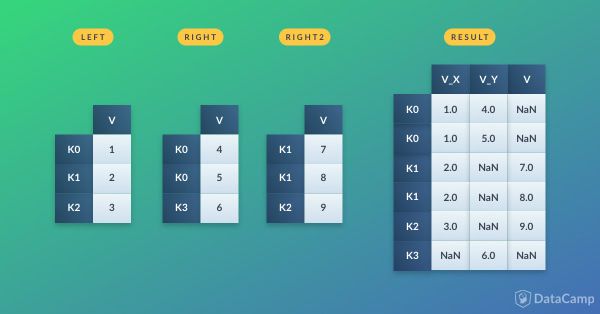

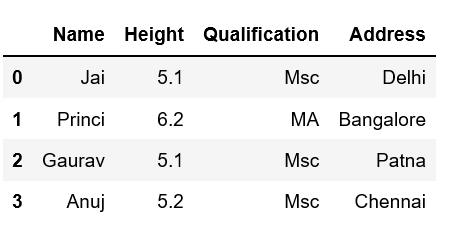

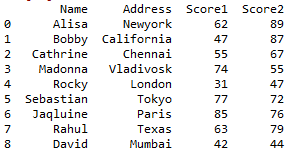

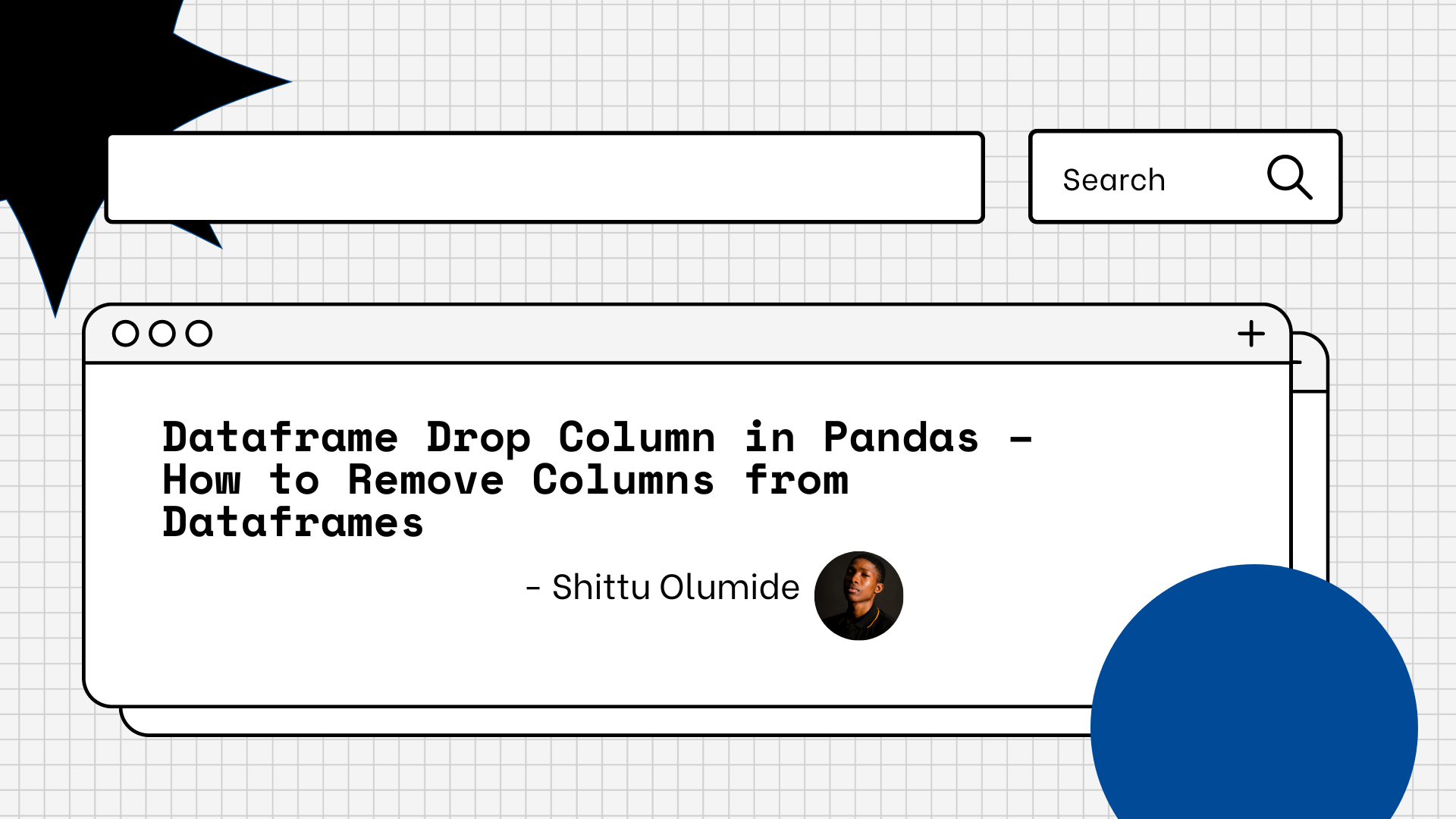

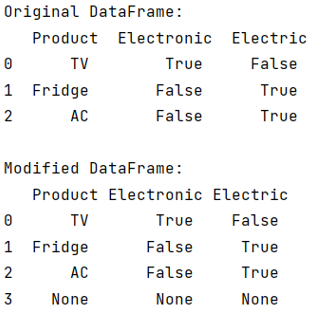
Article link: add empty column to dataframe pandas.
Learn more about the topic add empty column to dataframe pandas.
- Pandas – Add an Empty Column to a DataFrame
- How to add Empty Column to Dataframe in Pandas?
- How to add an empty column to a dataframe? – Stack Overflow
- How To Add An Empty Column To Pandas Dataframe
- Pandas Add Column to DataFrame – Spark By {Examples}
- How to Fill In Missing Data Using Python pandas – MakeUseOf
- 4 ways to add empty column to pandas DataFrame
- How to create an empty column in a Pandas dataframe in …
- How To Add a New Empty Column in Pandas
- Add Empty Column to dataframe in Pandas : 3 Methods
- How To Add Empty Column In DataFrame In Python
- Add Empty Column to pandas DataFrame in Python
See more: blog https://nhanvietluanvan.com/luat-hoc