Expression Must Be A Modifiable Lvalue
In the world of programming, expressions play a crucial role in defining and manipulating data. They are the building blocks of code that allow programmers to perform various operations, calculations, and assignments. However, not all expressions are created equal. Some expressions are modifiable, while others are not.
1. Understanding the concept of expression as a modifiable lvalue
To comprehend the concept of a modifiable lvalue expression, we first need to understand the key terms involved. An expression refers to a combination of operands and operators that can be evaluated to produce a value. An lvalue, short for “left value,” represents an expression that refers to an object that occupies some memory location.
A modifiable lvalue expression, therefore, refers to an expression that not only points to a memory location but also allows modification of the value stored in that memory location. In simpler terms, it is an expression that can be used as the left-hand side of an assignment operator (=) to update the value it represents.
2. Exploring the different types of expressions in programming
Expressions in programming can vary greatly in their nature and purpose. They can be arithmetic, logical, relational, or conditional. Arithmetic expressions involve mathematical operations like addition, subtraction, multiplication, and division. Logical expressions deal with boolean values (true or false) and logical operations such as AND, OR, and NOT.
Relational expressions evaluate the relationship between two operands, checking for equality, inequality, or comparison (greater than, less than, etc.). Conditional expressions rely on if-else constructs to select different paths of execution based on certain conditions.
3. The significance of modifiability in an lvalue expression
Modifiability is a fundamental aspect of programming because it enables developers to manipulate and update the values stored in memory locations. Without the ability to modify these values, the flexibility and dynamic nature of programs would be severely limited.
Modifiable lvalue expressions allow for various operations like assignment, incrementing, and decrementing, which are essential for performing computations, updating variables, and implementing control flow in programs.
4. Identifying the characteristics of a modifiable lvalue expression
A modifiable lvalue expression possesses several distinct characteristics:
– It refers to an object or memory location that can hold a value.
– It allows the assignment operator (=) to update the value it represents.
– It can participate in various operations like addition, subtraction, or bitwise operations.
– It can be dereferenced, as in the case of pointers, to access and modify the value pointed to.
In essence, a modifiable lvalue expression provides a means for developers to manipulate data and control program execution efficiently.
5. Differences between modifiable and non-modifiable expressions
While modifiable lvalue expressions readily allow modifications of the values they represent, non-modifiable expressions, or rvalues, do not have this capability. Non-modifiable expressions include literals (e.g., a constant integer or string) and the result of certain operations.
Non-modifiable expressions can be used as operands in various operations, but they cannot be assigned a new value or participate in modification operations. Attempting to modify a non-modifiable expression will result in a compilation error.
6. The role of modifiable lvalues in variable assignments and updates
Modifiable lvalue expressions are crucial for variable assignments and updates in programming. When defining variables, programmers often utilize modifiable lvalues to give a name and memory location to a value that can be modified throughout the program’s execution.
For example, consider the following line of code in C++:
“`cpp
int num = 10;
“`
Here, `num` is a modifiable lvalue that represents an integer. By assigning a value to `num`, we can subsequently update or modify its value as needed during execution.
7. Practical examples of using modifiable lvalue expressions in programming
To comprehend the practical applications of modifiable lvalue expressions, let’s explore a couple of examples:
Example 1: Modifying an Array in C++
“`cpp
char array[] = “Hello”;
array[0] = ‘C’;
“`
In this example, the `array` is a modifiable lvalue that represents a character array. By assigning a new value to a particular index of the array (e.g., `array[0]`), we can modify its contents. Here, the string “Hello” is effectively changed to “Cello.”
Example 2: Incrementing an Integer
“`cpp
int num = 5;
num++;
“`
In this case, `num` is a modifiable lvalue representing an integer. By using the increment operator (++), we can modify its value, incrementing it from 5 to 6.
8. Potential errors and pitfalls when dealing with modifiable lvalues
When working with modifiable lvalue expressions, it is crucial to be aware of potential errors and pitfalls that can arise. Some common errors include:
– Using a non-modifiable expression in a place where a modifiable one is required. For instance, if trying to modify a constant or a non-assignable value.
– Accidentally assigning a value to an expression that is not modifiable, leading to a compilation error.
– Mishandling pointers or arrays, resulting in memory access errors or undefined behavior.
9. Best practices for utilizing modifiable lvalues effectively in your code
To effectively use modifiable lvalue expressions in your code, it is important to adhere to these best practices:
– Ensure that you are using the appropriate types when defining variables and that they are not explicitly marked as const or non-modifiable.
– Properly initialize variables before attempting to modify them.
– Be cautious when working with pointers and arrays, ensuring that they are properly allocated and deallocated, and that memory boundaries are respected.
– Regularly test and debug your code to catch any potential errors or issues related to modifiable lvalues.
FAQs
Q1: What is an lvalue in programming?
An lvalue, or “left value,” refers to an expression that represents an object or memory location. It can appear on the left-hand side of an assignment operator and is typically used to assign or modify values.
Q2: Why is “Expression must be a modifiable lvalue char array c” an error in C++?
The error “Expression must be a modifiable lvalue char array c” occurs when attempting to modify a non-modifiable expression. In C++, a `const` qualifier can be applied to an array, preventing any modifications to its contents.
Q3: Why does the compiler say “I value required as the left operand of the assignment”?
The error “I value required as the left operand of the assignment” occurs when the left-hand side of an assignment operator is not a modifiable lvalue. It typically arises when you attempt to assign a value to an expression that cannot be modified.
Q4: What does “Expression must have integral or unscoped enum type” mean in C++?
The error “Expression must have integral or unscoped enum type” indicates that the expression being used in a particular context must have a type that is either integral (e.g., int, char) or an unscoped enumeration. Any other types will result in a compilation error.
Q5: How do you declare an array in C++ as a modifiable lvalue?
To declare an array in C++ as a modifiable lvalue, you can use the following syntax:
“`cpp
int array[10];
“`
Here, `array` is a modifiable lvalue representing an integer array with a size of 10. You can subsequently modify the contents of this array as needed.
Expression must be a modifiable lvalue char array c, Expression must be a modifiable lvalue pointer, What is lvalue, I value required as the left operand of assignment, Expression must have integral or unscoped enum type, New array C++, Array type is not assignable, Declare array C++expression must be a modifiable lvalue
Expression Must Be A Modifiable Lvalue In C Language
What Is A Modifiable Lvalue In C++?
In C++, an lvalue is essentially an expression that refers to a memory location and can be assigned a value. It is a term borrowed from the C programming language, where it stands for “left value” as it is usually found on the left side of an assignment operator.
An lvalue can come in two different types: modifiable and non-modifiable. In this article, we will focus specifically on modifiable lvalues and their significance in C++.
Modifiable lvalues are expressions that can be both read from and assigned a new value. This means that variables declared as modifiable lvalues can be modified throughout the program execution. In C++, almost all variables fall into this category by default.
For example, consider the following code snippet:
“`
int x = 5; // x is a modifiable lvalue
x = 10; // x can be assigned a new value
“`
In this case, the variable `x` is declared as an `int` and assigned an initial value of 5. However, being a modifiable lvalue, it can be later assigned a new value, as shown in the second line where `x` is assigned the value of 10.
It is important to note that not all lvalues are modifiable. Some lvalues are deemed non-modifiable, meaning they cannot be changed once assigned a value. For instance, constants, literals, and expressions that are not proper lvalues, such as the result of an arithmetic expression or a function call, are considered non-modifiable lvalues.
Here is an example that illustrates the difference between modifiable and non-modifiable lvalues:
“`
const int y = 2; // y is a non-modifiable lvalue
int z = 3; // z is a modifiable lvalue
y = 5; // Error! y is a non-modifiable lvalue
z = 7; // z can be assigned a new value
“`
In this code snippet, `y` is declared as a constant with the value of 2. As a non-modifiable lvalue, attempting to assign a new value to `y` would result in a compilation error. On the other hand, the variable `z` is a modifiable lvalue and can be assigned a new value without any issues.
Frequently Asked Questions (FAQs)
Q: Can I modify an lvalue directly within an expression?
A: Yes, you can modify an lvalue directly within an expression using compound assignment operators. For example, `x += 5;` would increment the value of `x` by 5.
Q: What happens if I try to modify a non-modifiable lvalue?
A: Modifying a non-modifiable lvalue results in a compilation error. The compiler ensures that non-modifiable lvalues maintain their assigned values throughout the program execution.
Q: Are all variables in C++ modifiable lvalues by default?
A: Yes, almost all variables in C++ are modifiable lvalues by default. However, if you declare a variable as `const`, it becomes a non-modifiable lvalue.
Q: Can member variables or objects of a class be modifiable lvalues?
A: Yes, member variables or objects of a class can be modifiable lvalues if they are not declared as `const`. However, if a member variable is declared as `const`, it becomes a non-modifiable lvalue.
Q: Are modifiable lvalues necessary in C++ programming?
A: Modifiable lvalues are crucial in C++ programming as they allow for the modification of variables throughout the program execution. They play an essential role in supporting various operations and calculations.
In conclusion, modifiable lvalues in C++ are expressions that can be both read from and assigned a new value. They are an integral part of C++ programming as they enable variable modification during program execution. Understanding the concept of modifiable lvalues helps in writing more flexible and dynamic code.
What Is L Value Error In C++?
C++ is a powerful programming language that offers a variety of features and capabilities to developers. However, like any other programming language, it also comes with its fair share of errors and challenges. One such error that programmers often encounter is L value error. In this article, we will explore what L value error is in C++ and how to handle it effectively.
To understand L value error, we must first understand the concept of L values and R values in C++. In simple terms, an L value refers to a value that can be assigned to, while an R value refers to a value that can be read from but cannot be assigned to. For example, variables and objects are L values, while literals or constants are R values.
Now, let’s dive into the L value error. In C++, an L value error occurs when we try to assign or modify the value of an R value, which is not allowed. This often happens when we mistakenly attempt to modify a constant or try to assign a value to a temporary object. The compiler considers this as a violation of the language rules and throws an L value error.
For instance, consider the following code snippet:
“`cpp
int sum = 10 + 20;
sum = sum * 2;
“`
In this code, the first line assigns the sum of 10 and 20 (an R value) to the variable `sum` (an L value). However, the second line attempts to modify the R value `sum * 2`, which is not permissible. As a result, the compiler would catch this error and display an L value error message.
Here are a few common scenarios where L value errors can arise:
1. Modifying a constant:
“`cpp
const int MAX_VALUE = 100;
MAX_VALUE = 200; // L value error
“`
In this case, `MAX_VALUE` is a constant with a fixed value of 100. Attempting to modify its value would result in an L value error.
2. Assigning a value to a temporary object:
“`cpp
int GetNumber() {
return 42;
}
GetNumber() = 50; // L value error
“`
The function `GetNumber()` returns a temporary object (R value) with the value of 42. Trying to assign a new value to this temporary object would be considered an L value error.
3. Modifying a literal:
“`cpp
“Hello, World!” = “Goodbye, World!”; // L value error
“`
Literals are R values and are typically used for read-only purposes. Therefore, trying to modify a literal would result in an L value error.
Handling L value errors in C++ requires careful consideration and understanding of the underlying concepts. Here are a few approaches to deal with L value errors effectively:
1. Review your code for improper assignments:
Double-check your code and ensure that you’re not attempting to modify or assign values to R values such as literals or constants. If you come across such instances, refactor your code to adhere to the language rules.
2. Validate function return types:
When working with functions that return values, ensure that the return type is appropriately defined as an L value if you intend to modify it. By specifying the correct return type, you can avoid L value errors that may occur due to invalid assignments.
3. Understand the scope of variables:
Variables declared within a limited scope, such as those created inside a function, are often temporary and, therefore, R values. Avoid trying to assign values to these temporary objects to prevent L value errors.
FAQs:
1. What is the difference between an L value and an R value in C++?
In C++, an L value refers to a value that can be assigned to. It represents an object or a memory location. On the other hand, an R value refers to a value that cannot be assigned to and is typically used for read-only purposes like literals or constants.
2. Can L value errors be ignored?
L value errors should not be ignored as they indicate a violation of the language rules in C++. Ignoring these errors could lead to unexpected behavior in the program or even compiler crashes. It is essential to review and fix any L value errors encountered during the development process.
3. Are L value errors specific to C++?
No, L value errors are not specific to C++. Similar concepts of L values and R values exist in other programming languages as well. However, the specific error messages and handling may vary between different languages.
4. Can I modify an R value in C++?
No, R values are read-only in C++. Modifying an R value would result in an L value error. The purpose of R values is to represent values that can only be read from, such as constants or literals.
Conclusion:
L value errors in C++ occur when an attempt is made to modify an R value, which violates the language rules. Being aware of the distinction between L values and R values is crucial in understanding and resolving L value errors effectively. By double-checking assignments, validating function return types, and understanding variable scopes, programmers can prevent L value errors and ensure the smooth execution of their code. So, always keep L value errors in mind while developing C++ programs and handle them appropriately for robust and error-free code.
Keywords searched by users: expression must be a modifiable lvalue Expression must be a modifiable lvalue char array c, Expression must be a modifiable lvalue pointer, What is lvalue, I value required as left operand of assignment, Expression must have integral or unscoped enum type, New array C++, Array type is not assignable, Declare array C++
Categories: Top 70 Expression Must Be A Modifiable Lvalue
See more here: nhanvietluanvan.com
Expression Must Be A Modifiable Lvalue Char Array C
In the world of programming, understanding the syntax and requirements of different expressions is crucial. One such requirement that often arises in C programming is the need for an expression to be a modifiable lvalue char array. This article will delve into the concept of a modifiable lvalue char array expression, its significance, and how it impacts C programming. We will also address some frequently asked questions to help clarify any doubts or misconceptions surrounding this topic.
To begin with, let’s break down the different components of this requirement. An expression refers to a combination of values, variables, operators, and function calls that can be evaluated to produce a result. In C, an expression is often used to assign values to variables, perform calculations, or make decisions based on conditions.
A modifiable lvalue refers to an expression that can be assigned a value and can also appear on the left-hand side of an assignment statement. It can be thought of as a location in memory that can store data. In comparison, a non-lvalue expression refers to a value or a temporary result that cannot be assigned a new value. For instance, the constant value ‘5’ is a non-lvalue expression as it cannot be modified.
Now, let’s focus on the term ‘char array.’ In C, a char array is a sequence of characters stored in contiguous memory locations, terminated by a null character (‘\0’). It can be considered as a string of characters, such as “hello world.” Each character in the array occupies one byte of memory. To declare a char array in C, we use the syntax: “char array_name[size];”. For example, “char name[20];” declares a char array named ‘name’ that can store up to 20 characters.
Putting these pieces together, a modifiable lvalue char array expression is an expression that represents a char array and can be modified or assigned a new value. This expression can be used to access individual characters of the array or modify the entire array. It plays a fundamental role in various C programming tasks, such as manipulating text, reading user input, or processing file contents.
Now, let’s consider some examples to illustrate the significance of a modifiable lvalue char array expression. Imagine a scenario where we want to read a user’s name from the standard input and store it in a character array. We would require a modifiable lvalue char array expression to perform this task. Here’s an example:
“`c
char name[50];
scanf(“%s”, name);
“`
In the above code snippet, ‘name’ is a modifiable lvalue char array expression that allows us to store the user’s input. Similarly, if we wish to update or modify the name later in the program, we can simply assign a new value to it using a modifiable lvalue char array expression:
“`c
char name[50];
strcpy(name, “John Doe”);
“`
Here, we assign the value “John Doe” to the char array ‘name’ using the strcpy() function.
Now that we have discussed the concept and significance of a modifiable lvalue char array expression, let’s address some common questions:
Q: Can a modifiable lvalue char array expression be of any size?
A: Yes, a modifiable lvalue char array expression can be of any size as long as it is within the limits defined by the maximum memory available.
Q: Is it possible to modify individual characters within a char array using a modifiable lvalue char array expression?
A: Yes, individual characters within a char array can be modified using a modifiable lvalue char array expression. For example, ‘name[0] = ‘H’;’ would modify the first character of the ‘name’ array to ‘H’.
Q: How do I determine the length of a modifiable lvalue char array expression?
A: In C, the length of a char array can be determined using the strlen() function, which counts the number of characters in a string until it reaches the null character (‘\0’).
Q: Can a modifiable lvalue char array expression be passed as an argument to a function?
A: Yes, a modifiable lvalue char array expression can be passed as an argument to a function. However, it is essential to ensure that the function parameters are appropriately defined to handle char arrays.
In conclusion, mastering the concept of a modifiable lvalue char array expression is essential for any C programmer. It enables the manipulation of strings, reading and storing user input, and various other essential tasks. By understanding the role and significance of this requirement, programmers can unlock the full potential of C programming and create robust and efficient code.
Expression Must Be A Modifiable Lvalue Pointer
In the world of programming, pointers play a vital role in manipulating and accessing data in computer memory. Pointers allow programmers to work with memory addresses directly, enabling them to efficiently manage resources and create complex data structures. However, when working with pointers, it is important to understand the concept of modifiable lvalue pointers and the restrictions associated with them.
In simple terms, a modifiable lvalue pointer refers to a pointer that can be modified and assigned a different memory address. The term “lvalue” stands for an expression that represents a memory location that can be assigned a value. An expression that is an lvalue pointer means that it can be modified, allowing the programmer to change the memory location it points to.
When using pointers, it is essential to consider whether an expression represents a modifiable lvalue pointer. In C and C++ programming languages, several scenarios require modifiable lvalue pointers to achieve certain operations. One such scenario is when dynamically allocating memory using dynamic memory allocation functions like `malloc()` or `new`.
Consider the following example:
“`
int* a = malloc(sizeof(int));
“`
In this example, `malloc()` is used to allocate memory for an integer. The return value of `malloc()` is assigned to the pointer `a`. Here, `a` is a modifiable lvalue pointer, as it can be modified to point to a different memory location.
However, it is important to note that not all pointers are modifiable lvalue pointers. Some pointers, such as constants or pointers to read-only data, cannot be modified. For instance, consider the following example:
“`
const int* b = someData;
“`
Here, `b` is a pointer to a constant integer. The `const` keyword indicates that the data pointed to by `b` cannot be modified. Consequently, `b` is not a modifiable lvalue pointer.
Restrictions of modifiable lvalue pointers
Modifiable lvalue pointers come with certain restrictions that programmers must adhere to. One primary restriction is that a modifiable lvalue pointer must be assigned a valid memory address. Attempting to assign an invalid memory address can lead to runtime errors, crashes, and other unexpected behaviors.
For instance, consider the following code snippet:
“`
int* c = 0;
“`
Here, `c` is assigned the null pointer, which is an invalid memory address. Assigning an invalid memory address to a modifiable lvalue pointer is a dangerous practice and can result in crashes or segmentation faults.
In addition to this restriction, modifiable lvalue pointers cannot be used to directly modify values that are not within the scope of the pointer itself. For instance, consider the following code snippet:
“`
int* d;
*d = 5;
“`
In this example, `d` is a modifiable lvalue pointer but does not point to a valid memory location. Dereferencing the pointer using the `*` operator to modify the value leads to undefined behavior and can cause segmentation faults.
FAQs:
Q: Can an expression be a non-modifiable lvalue pointer?
A: Yes, an expression can be a non-modifiable lvalue pointer. Pointers to constant data or read-only variables are examples of non-modifiable lvalue pointers.
Q: How can I ensure that a modifiable lvalue pointer points to a valid memory location?
A: Before using a modifiable lvalue pointer, ensure that it is assigned a valid memory address. This can be achieved through dynamic memory allocation techniques like `malloc()` or by assigning the address of a valid variable.
Q: What are the consequences of using an invalid memory address with a modifiable lvalue pointer?
A: Assigning an invalid memory address to a modifiable lvalue pointer can lead to runtime errors, crashes, and undefined behavior. It is crucial to ensure that modifiable lvalue pointers always point to valid memory locations.
Q: Can a modifiable lvalue pointer be modified to point to a different memory location?
A: Yes, a modifiable lvalue pointer can be modified to point to a different memory location. This flexibility allows programmers to manage and manipulate memory efficiently.
In conclusion, understanding the concept of modifiable lvalue pointers is crucial for efficient memory management in programming. Modifiable lvalue pointers provide programmers with the flexibility to change the memory location it points to, enabling them to create dynamic data structures and allocate memory efficiently. It is essential to adhere to the restrictions associated with modifiable lvalue pointers to prevent runtime errors and unexpected behaviors.
What Is Lvalue
In computer programming, an lvalue (locally known as “left value”) represents an entity that can be assigned a value. It is an expression that refers to a specific memory location or an object that resides in the memory. The term “lvalue” stands for “left value” as it can appear on the left side of an assignment operator (=) in an expression.
In simple terms, an lvalue is anything that has an address, allowing you to assign a value to it. It is an expression that is both a value and a location in memory. An lvalue can be a variable, an array element, or even a dereferenced pointer.
To understand lvalues further, let’s delve into a few examples:
1. Variables:
“`
int x = 5;
x = 10;
“`
In this example, “x” is an lvalue as it refers to a specific memory location. It can be assigned a new value, such as 10.
2. Array Elements:
“`
int arr[5] = {1, 2, 3, 4, 5};
arr[3] = 8;
“`
Here, “arr[3]” is an lvalue as it represents the third element of the array “arr”. It can be assigned a new value, which is 8 in this case.
3. Pointers:
“`
int y = 10;
int* ptr = &y;
*ptr = 20;
“`
In this example, “*ptr” is an lvalue as it represents the value pointed to by the pointer “ptr.” By assigning a new value to “*ptr,” which is 20, we change the value of “y” indirectly.
Understanding the concept of lvalues is essential for various programming tasks. It helps in distinguishing the left side of an assignment from the right side, which consists of rvalues (non-lvalues).
Frequently Asked Questions (FAQs):
Q1: What is the difference between lvalue and rvalue?
A1: An lvalue represents an entity with an addressable memory location, while an rvalue represents a temporary or constant value. An lvalue can appear on the left side of an assignment, while an rvalue can only appear on the right side.
Q2: Can an lvalue be an expression?
A2: Yes, an lvalue can be an expression as long as it refers to a memory address. For example:
“`
int x = 2;
int y = x + 3;
“`
In this case, the expression “x + 3” is an lvalue because it can be assigned a value.
Q3: Are constants lvalues or rvalues?
A3: Constants are generally treated as rvalues. However, there are exceptions in certain cases, such as when the constant is assigned to an lvalue reference. In such cases, the constant can be treated as an lvalue.
Q4: Why is knowing lvalues important?
A4: Understanding lvalues is crucial for various programming tasks, such as assigning values, passing arguments to functions, and using operators like the dereference operator (*) or the address-of operator (&). It helps in clear differentiation between values that can be modified and those that are read-only.
Q5: Can an lvalue reference bind to an rvalue?
A5: No, an lvalue reference cannot directly bind to an rvalue. However, in C++11 and later versions, an lvalue reference can bind to an rvalue if it is explicitly converted into an rvalue reference using std::move().
Q6: Can function calls be lvalues?
A6: Function calls can be lvalues if they return a reference to an object. For example:
“`
int& foo() {
static int x = 5;
return x;
}
foo() = 10;
“`
In this case, the function call “foo()” is an lvalue because it returns a reference to the static variable “x.” Consequently, we can assign a new value (10) to “foo()”.
In conclusion, an lvalue in English refers to an expression that can be assigned a value. It represents a memory location or an object in memory. Understanding lvalues is crucial for programming tasks that involve assigning values, passing arguments, and using operators. By differentiating between lvalues and rvalues, programmers can efficiently manipulate and modify data within their programs.
Images related to the topic expression must be a modifiable lvalue
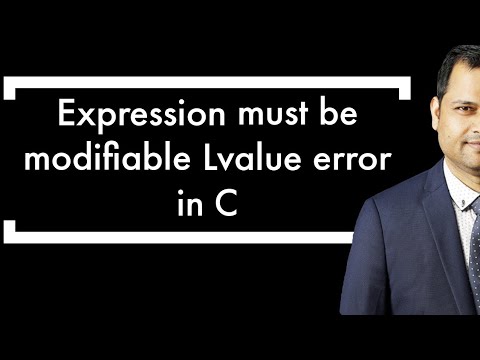
Found 23 images related to expression must be a modifiable lvalue theme
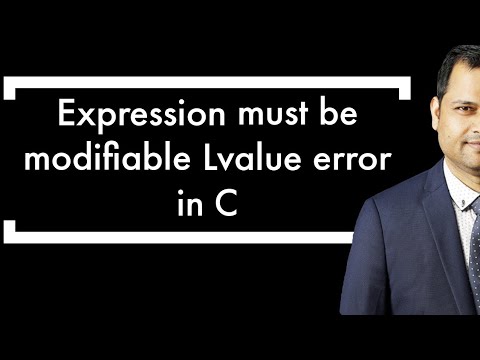




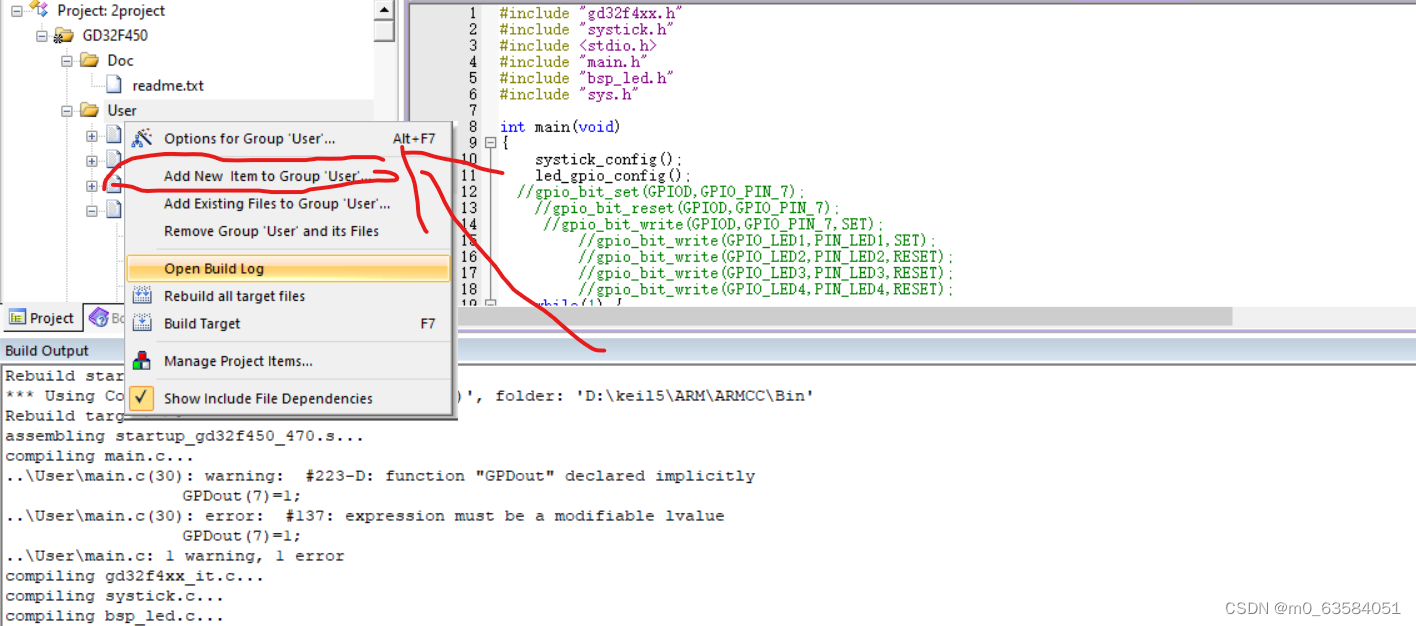
Article link: expression must be a modifiable lvalue.
Learn more about the topic expression must be a modifiable lvalue.
- Expression must be a modifiable lvalue – c++ – Stack Overflow
- Expression Must Be a Modifiable Lvalue: Error Solved
- Expression must be a modifiable lvalue – IAR Systems
- Lvalues and rvalues – IBM
- Else without IF and L-Value Required Error in C – GeeksforGeeks
- Lvalue Required as Left Operand of Assignment: Effective Solutions
- How to Create, Access, and Modify the arrays in C – SillyCodes
- C++ Error: Expression Must Be A Modifiable Lvalue – Linux Hint
- Expression must be a modifiable lvalue – Microsoft Q&A
- What does ‘Expression must be a modifiable lvalue’ mean?
- Compiler Error 137 – Cookbook – Mbed
- c++ expression must be a modifiable lvalue [SOLVED]
- CCS/TM4C123GH6PM: error #138: expression must be a …
See more: nhanvietluanvan.com/luat-hoc