Go String To Int
1. Introduction to String to Int Conversion in Go
Converting a string to an integer is a common operation in many programming languages, including Go. This process, also known as parsing, allows developers to extract numerical values from strings and use them in calculations, comparisons, or other operations.
In Go, the strconv package provides several functions that facilitate string to int conversion. These functions handle different scenarios, such as parsing integers in different number bases, handling signed or unsigned integers, and implementing error handling for invalid inputs.
2. Exploring the strconv package in Go
a. Importing the strconv package:
To use the strconv functions in Go, we need to import the strconv package into our code. This can be done with the following line:
“`go
import “strconv”
“`
b. Overview of strconv.Atoi() function:
The strconv package includes the Atoi() function, which is a shorthand for converting a string to an int in base 10. It returns the converted integer and an error. If the conversion fails, an error is returned. Here is an example usage:
“`go
value, err := strconv.Atoi(“123”)
if err != nil {
fmt.Println(“Conversion error:”, err)
} else {
fmt.Println(“Parsed integer:”, value)
}
“`
c. Understanding the strconv.ParseInt() function:
The strconv package also provides the ParseInt() function, which allows us to convert a string to an int of a specific bit size and base. It returns the converted integer and an error. Here is an example usage:
“`go
value, err := strconv.ParseInt(“123”, 10, 64)
if err != nil {
fmt.Println(“Conversion error:”, err)
} else {
fmt.Println(“Parsed integer:”, value)
}
“`
3. Handling String to Int Conversion Errors
a. Recognizing and handling invalid inputs:
When converting a string to an int, it’s important to handle invalid inputs properly. Invalid inputs can include non-numeric characters, numbers larger than the maximum value that can be represented by the chosen int type, or numbers in the wrong base.
b. Addressing conversion failures:
Both Atoi() and ParseInt() functions return an error when the conversion fails. It is crucial to check for and handle these errors to ensure your program behaves as expected.
c. Implementing error handling with the strconv package:
To handle conversion errors, we can use the error returned by the strconv functions to provide meaningful feedback to the user or take appropriate actions in our code. By checking the error value, we can decide how to handle invalid inputs or conversion failures in a way that suits our application’s needs.
4. Accounting for Different Number Bases
a. Understanding base 10 (decimal) conversion:
By default, both Atoi() and ParseInt() functions assume the input string represents a decimal number. The base parameter in ParseInt() allows us to specify a different number base, such as binary, octal, or hexadecimal.
b. Converting strings with hexadecimal or octal numbers:
To convert a string containing a hexadecimal or octal representation to an integer, we can pass the appropriate base to the ParseInt() function. For example, converting “0xFF” to an integer can be done as follows:
“`go
value, _ := strconv.ParseInt(“FF”, 16, 64)
fmt.Println(“Parsed integer:”, value)
“`
c. Handling different base conversion errors:
When converting strings with different number bases, it’s essential to handle potential conversion errors. If the input string contains characters that are not valid in the chosen base, an error will be returned.
5. Working with Signed and Unsigned Integers
a. Converting signed integers from strings:
By default, the strconv functions assume the input string represents a signed integer. When converting, make sure to use an int type that can accommodate the range of values you expect.
b. Handling errors with signed integers:
When parsing signed integers, it’s crucial to handle errors that may arise due to invalid inputs or values outside the representable range.
c. Converting unsigned integers from strings:
Go also provides functions to convert strings to unsigned integers, such as ParseUint() in the strconv package. These functions allow us to convert strings to integers without a sign.
6. Advanced Techniques for String to Int Conversion
a. Working with non-standard number formats:
While the strconv package provides functions for standard number formats, you may encounter non-standard number formats in certain cases. In such scenarios, custom parsing logic may be required.
b. Parsing integers with custom sizes:
The strconv package supports parsing integers of different sizes, such as int8, int16, or int64. By specifying the desired bit size, we can ensure the converted value fits within the chosen integer type.
c. Utilizing additional functions in the strconv package:
Besides Atoi() and ParseInt(), the strconv package offers various other utility functions, including FormatInt(), FormatUint(), and AppendInt(), which can be helpful in formatting or converting integers to strings.
7. Performance Considerations and Best Practices
a. Benchmarking string to int conversion methods:
When working with large amounts of data or in performance-critical scenarios, it’s vital to consider the efficiency of string to int conversion methods. Benchmarking can help determine the most performant approach for your specific requirements.
b. Optimizing conversion processes:
To optimize string to int conversions, consider using techniques such as reusing buffers, minimizing unnecessary string allocations, or utilizing bitwise operations when applicable.
c. Following best practices for efficient conversion:
To ensure efficient string to int conversion, it’s important to use the appropriate strconv function for the specific scenario, handle errors properly, and utilize Go’s built-in error checks and conversion techniques whenever possible.
8. Comparing Alternative Methods for String to Int Conversion
a. Implementing manual string to int conversion:
While the strconv package provides convenient functions for string to int conversion, alternative methods can be implemented using loops, bitwise operations, or parsing algorithms. These approaches may be necessary in specific cases where the strconv functions do not meet the requirements.
b. Exploring alternative packages and libraries:
In addition to strconv, there are other Go packages and libraries available that offer string to int conversion functionality. Some of these alternatives may provide additional features or better performance in certain scenarios.
c. Evaluating pros and cons of different approaches:
When deciding on the right approach for string to int conversion, it’s important to consider factors such as ease of use, performance, error handling capabilities, and support for different number bases or formats.
9. Examples and Use Cases
a. Converting user input to integers:
String to int conversion is often required when processing user input, such as form fields or command-line arguments. By utilizing the strconv functions and appropriate error handling, developers can ensure accurate conversion and provide meaningful feedback to users.
b. Handling string to int conversion in database operations:
In database operations, converting string representations of numbers to integers may be necessary for data manipulation or querying. Properly converting and handling errors can ensure data integrity and prevent issues such as SQL injection.
c. Parsing numerical values from external files or APIs:
When dealing with external files or APIs that provide data in string format, converting these strings to integers allows for proper data analysis, calculations, or integration with existing systems.
10. Conclusion
String to int conversion is a fundamental operation in any programming language, including Go. By utilizing the strconv package and understanding the various conversion techniques and error handling mechanisms, developers can confidently convert strings to integers and handle different scenarios effectively.
In summary, Go provides a robust set of functions in the strconv package to parse strings to integers, handle errors, and accommodate different number bases or formats. By following best practices, optimizing conversions, and considering alternative approaches when necessary, developers can ensure efficient and accurate string to int conversion in their Go applications.
Golang: Str To Int Conversion | Int To String Conversion In Go
Keywords searched by users: go string to int Convert string to int64 Golang, Convert string to int32 golang, Convert int to int32 golang, Convert interface to int golang, Change string to int golang, Convert any to string golang, Byte to int golang, Convert string to uint Golang
Categories: Top 90 Go String To Int
See more here: nhanvietluanvan.com
Convert String To Int64 Golang
Introduction:
In Golang, converting a string to int64 may seem like a straightforward task, but understanding the intricacies and potential pitfalls is essential. This article aims to provide a comprehensive guide on converting strings to int64 in Golang, covering the various techniques, considerations, and potential challenges that developers may encounter.
Understanding int64:
Before diving into the conversion process, it’s crucial to understand what int64 represents in Golang. Int64 is a signed integer data type that can hold a value between -9223372036854775808 to 9223372036854775807, inclusive. Converting a string to int64 allows for easier manipulation, arithmetic operations, and compatibility with other numerical data types.
Converting string to int64 in Golang:
There are several techniques to convert a string to int64 in Golang. Let’s explore some of the most common methods below:
1. Using the strconv package:
The strconv package in Golang provides functions for converting strings to various numeric types. The strconv.ParseInt() function can be utilized to convert a string to int64. It takes the string to be converted as the first argument, the base (usually 10) as the second argument, and the bit size (64 in this case) as the third argument. The function returns the converted int64 value and an error value that should be checked for any potential conversion issues.
Example code snippet:
“`
import “strconv”
func main() {
str := “1234”
num, err := strconv.ParseInt(str, 10, 64)
if err == nil {
fmt.Println(“Converted to int64:”, num)
}
}
“`
2. Using the strconv.Atoi() function:
Although the strconv.Atoi() function is primarily used to convert a string to int, it can be used to convert strings to int64 as well. However, this function returns an error if the conversion exceeds the int range. To avoid this limitation and ensure successful conversion to int64, it is recommended to use strconv.ParseInt() instead.
Example code snippet:
“`
import “strconv”
func main() {
str := “1234”
num, err := strconv.Atoi(str)
if err == nil {
fmt.Println(“Converted to int64:”, int64(num))
}
}
“`
3. Using the strconv.ParseInt() function with error handling:
To ensure robustness and handle potential errors during string to int64 conversion, it is always advisable to include proper error handling. Upon encountering an error, the ParseInt() function returns 0 as the converted value. Therefore, it is crucial to validate the error before proceeding with the converted value.
Example code snippet:
“`
import “strconv”
func main() {
str := “notANumber”
num, err := strconv.ParseInt(str, 10, 64)
if err != nil {
fmt.Println(“Conversion error:”, err)
} else {
fmt.Println(“Converted to int64:”, num)
}
}
“`
FAQs:
1. What happens if the string cannot be converted to int64?
When a string cannot be converted to int64, the ParseInt() function returns an error, and the converted value is set to 0. It is crucial to handle the error and appropriately handle the failed conversion.
2. Can I convert a string representing a decimal number to int64?
No, the ParseInt() function does not handle decimal numbers. To convert a string representing a decimal number, you can utilize the strconv.ParseFloat() function instead. However, the resulting value will be a float64, not an int64.
3. What happens if the string exceeds the int64 range during conversion?
If the string value exceeds the int64 range, the ParseInt() function wraps the value around within the allowed range. For example, if “9223372036854775808” is converted, the resulting int64 value will be -9223372036854775808. It is crucial to take this behavior into account to avoid unexpected results.
4. Are there any performance considerations when converting strings to int64?
When it comes to performance, using strconv.ParseInt() is generally the most efficient method. However, actual performance can vary based on factors such as the size of input strings and the available system resources. It is advisable to benchmark different conversion techniques against specific use cases to determine the most optimal choice.
Conclusion:
Converting a string to int64 in Golang is an essential skill for developers working with numerical data. By utilizing the strconv package and its various functions, the conversion process can be performed efficiently and handles potential errors effectively. Understanding the limitations, considering edge cases, and implementing proper error handling are crucial for successful and reliable conversions.
With this comprehensive guide, developers can confidently convert strings to int64 in Golang, ensuring accurate data processing and compatibility within their applications.
Convert String To Int32 Golang
Introduction:
Golang, also known as Go, is an open-source programming language developed by Google. It is highly efficient and designed for concurrent, multi-threaded programming. In this article, we will explore how to convert a string to int32 in Golang. We will cover the necessary steps and provide some code examples to help you understand the process. So, let’s dive in!
Converting string to int32 in Golang:
In Golang, converting a string to int32 involves a few steps. First, you need to validate whether the string you want to convert is a valid integer or not. Then, if it is a valid integer, you can use the `strconv` package to convert the string to an `int64`. Finally, you can convert the `int64` to an `int32` if it fits within the int32 range. Let’s look at these steps in detail.
Step 1: Validating the string:
Before converting a string to int32, it is essential to ensure that the string represents a valid integer. Golang provides the `strconv` package, which offers a variety of functions for string conversions. The `Atoi` function, for example, can be used to convert a string to an integer.
“`go
import (
“strconv”
)
func stringToInt32(str string) (int32, error) {
// Validating the string
intValue, err := strconv.Atoi(str)
if err != nil {
return 0, err
}
// String is a valid integer
return int32(intValue), nil
}
“`
Step 2: Converting the string to an int64:
The `strconv.Atoi` function successfully converts a string to an integer. However, since we want to convert the string to an `int32`, which has a smaller range of values compared to `int`, we need to first convert it to `int64`. We can use the `ParseInt` function from the `strconv` package to achieve this.
“`go
func stringToInt32(str string) (int32, error) {
intValue, err := strconv.ParseInt(str, 10, 64)
if err != nil {
return 0, err
}
// Converting int64 to int32
if intValue > math.MaxInt32 || intValue < math.MinInt32 {
return 0, fmt.Errorf("value out of range to convert to int32")
}
return int32(intValue), nil
}
```
Step 3: Converting int64 to int32:
Finally, if the integer value falls within the range of `int32`, we can safely convert it to `int32`. We perform a range check to ensure that the value is within the limits of `math.MaxInt32` and `math.MinInt32` before converting.
Now, let's address some frequently asked questions (FAQs) related to string to int32 conversion in Golang.
FAQs:
Q1. What happens if the string contains non-numeric characters?
A: If the string contains non-numeric characters, the conversion will fail, and an error will be returned.
Q2. Can negative numbers be converted to int32?
A: Yes, negative numbers can be converted to int32 as long as they fall within the range of -2147483648 to 2147483647.
Q3. What if the value is out of the int32 range?
A: If the value is out of the int32 range, an error will be returned, indicating that the value cannot be converted to int32.
Q4. Are there any performance considerations when converting strings to int32?
A: Converting strings to int32 involves some overhead due to string parsing and range checks. However, it is generally efficient enough for most use cases.
Q5. Is there any alternative method to convert strings to int32?
A: Yes, you can use the `strconv.ParseInt` function with the specified base (10 in this case) to directly parse a string as an int64 value. Then, you can check the range and convert it to int32 accordingly.
Conclusion:
Converting a string to int32 in Golang requires validating the string, converting it to an int64, and finally checking the range and converting to int32 if it falls within the acceptable range. We explored the steps involved in this conversion process and provided code examples to assist your understanding. By following these steps, you can effectively convert strings to int32 in your Golang programs.
Images related to the topic go string to int
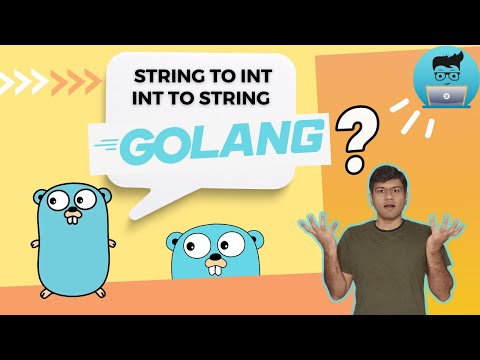
Found 38 images related to go string to int theme

![Go] Chuyển đổi Int/String Slice sang kiểu dữ liệu String trong Golang - Technology Diver Go] Chuyển Đổi Int/String Slice Sang Kiểu Dữ Liệu String Trong Golang - Technology Diver](https://cuongquach.com/wp-content/uploads/2020/06/convert-int-string-slice-to-string.jpg)
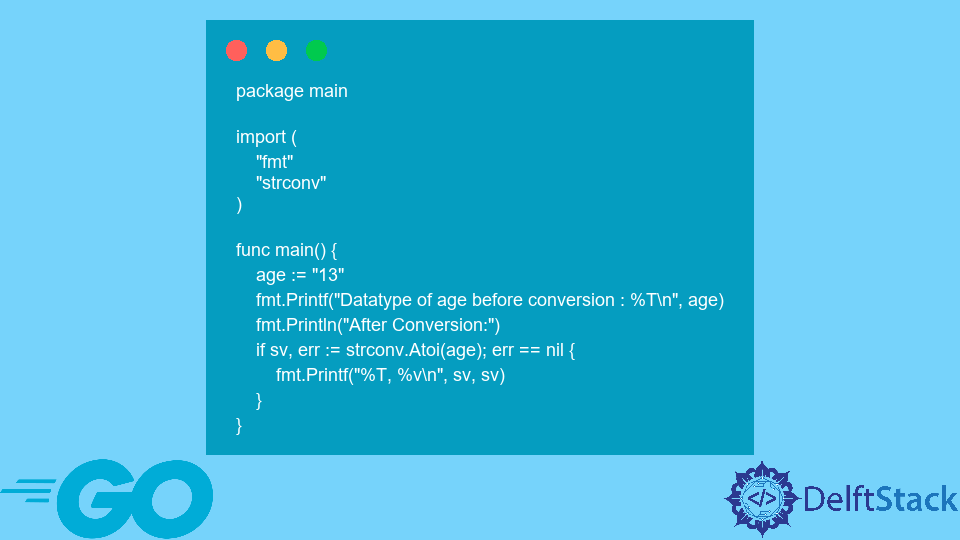
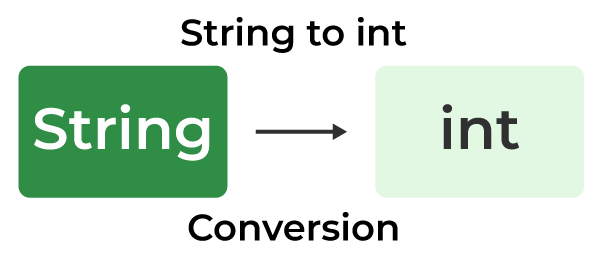
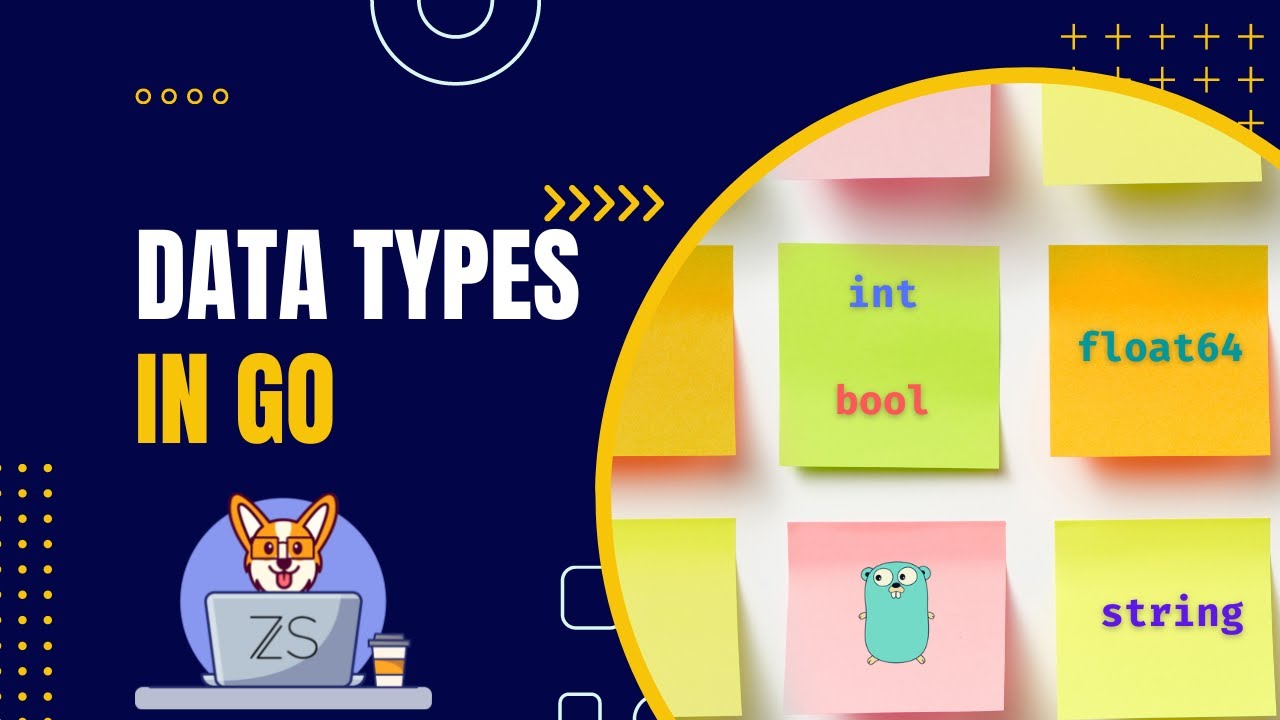
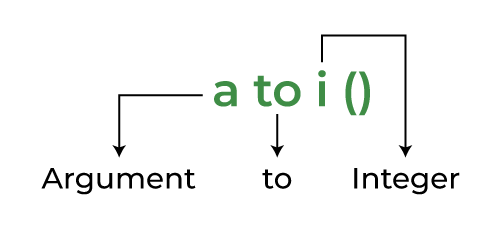
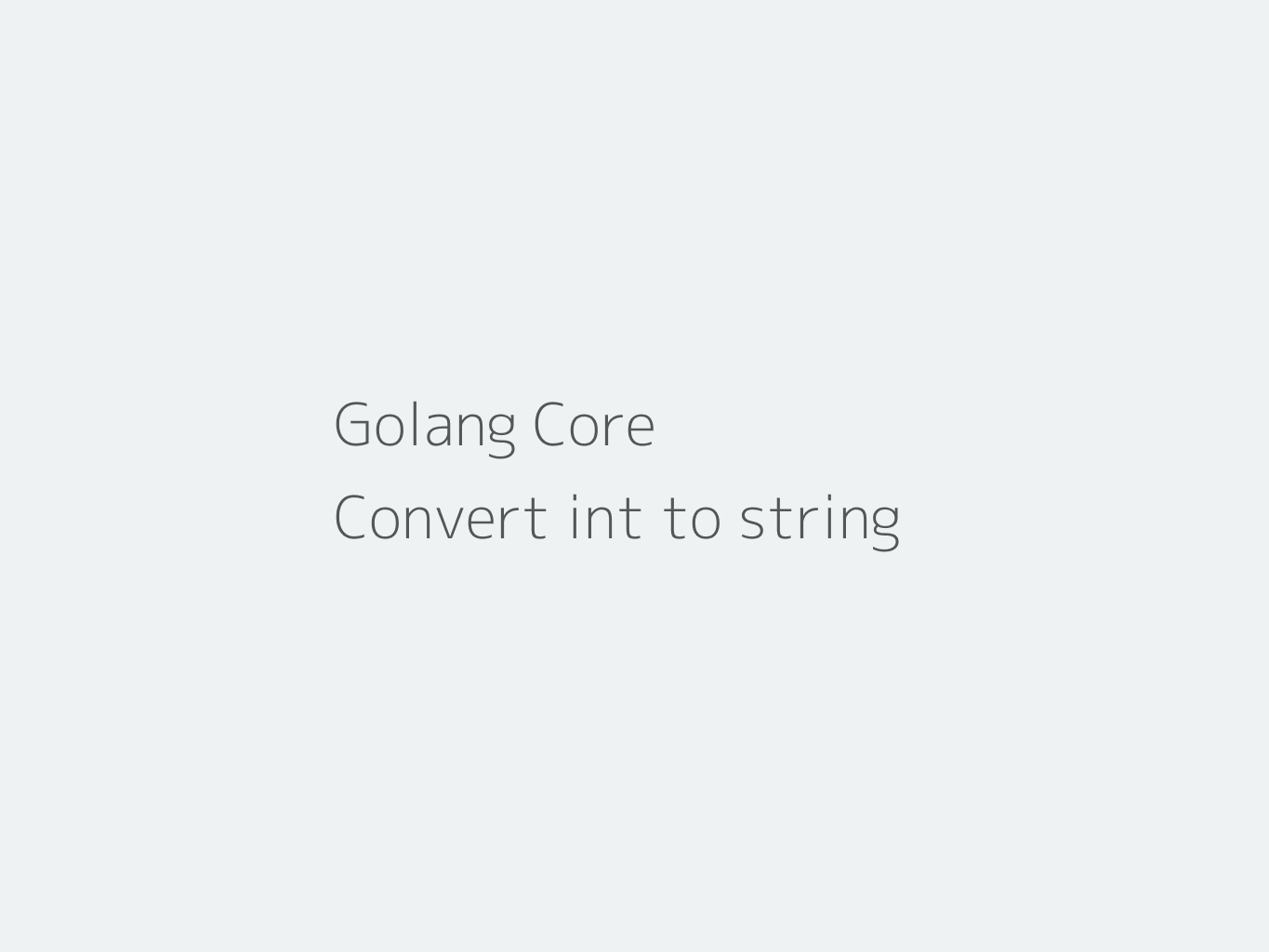
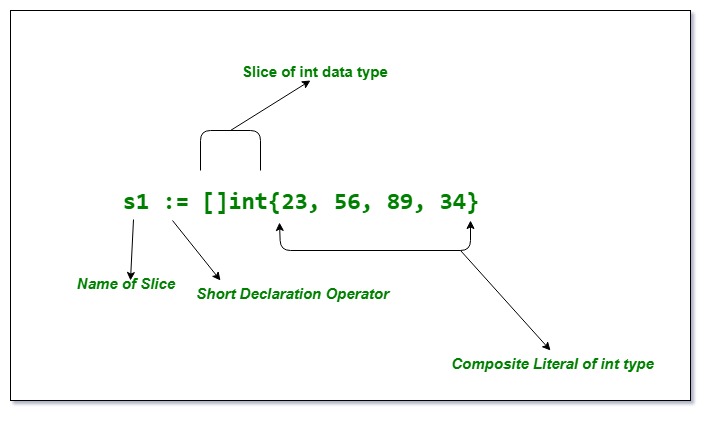

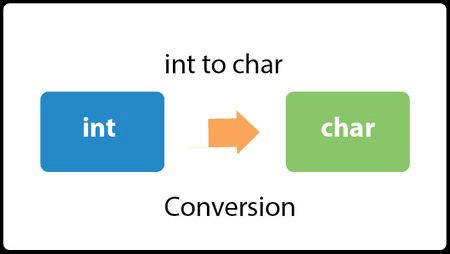
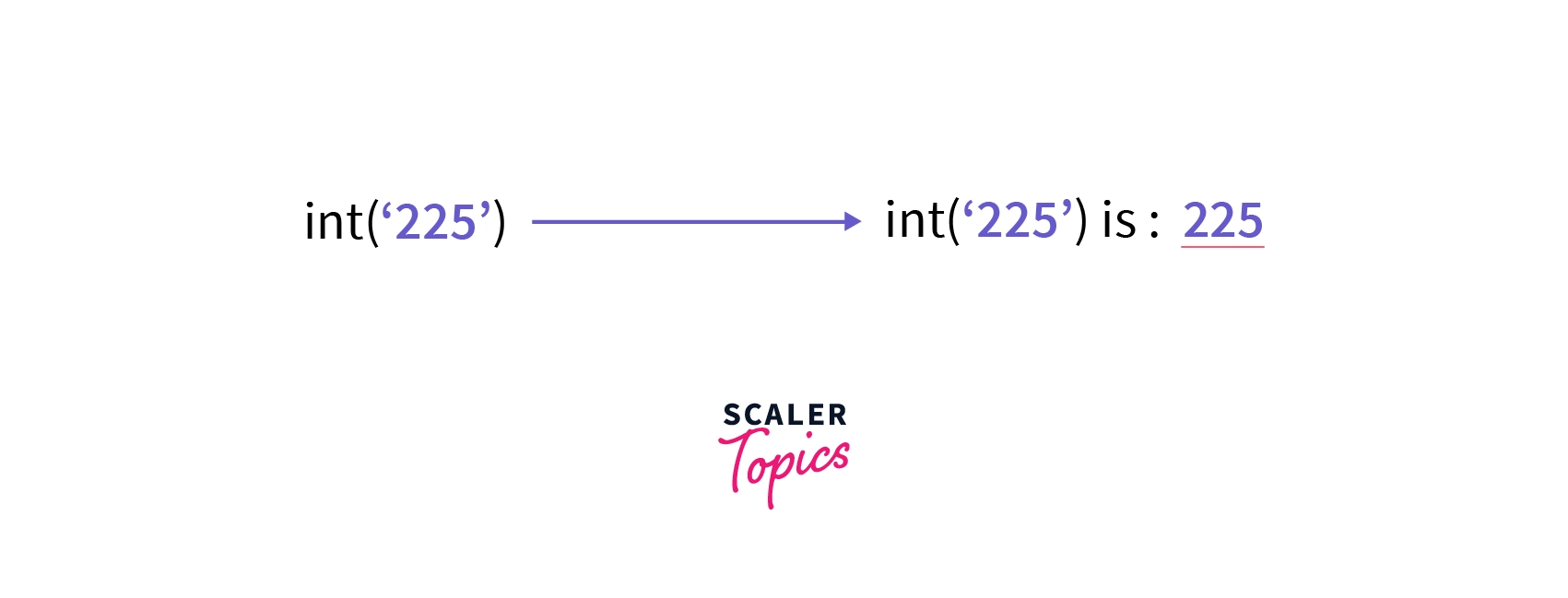
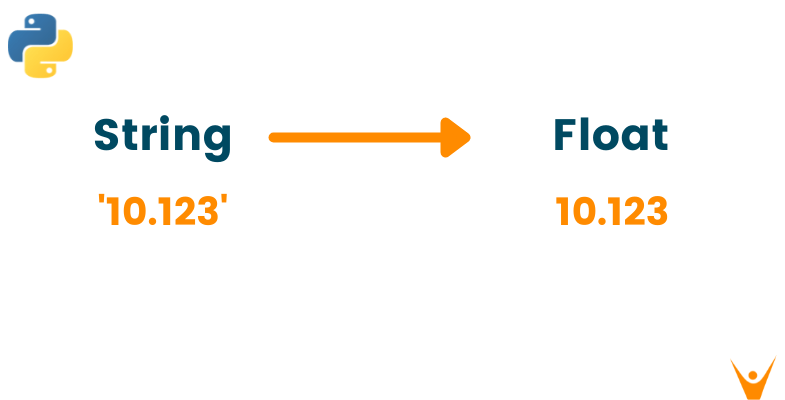

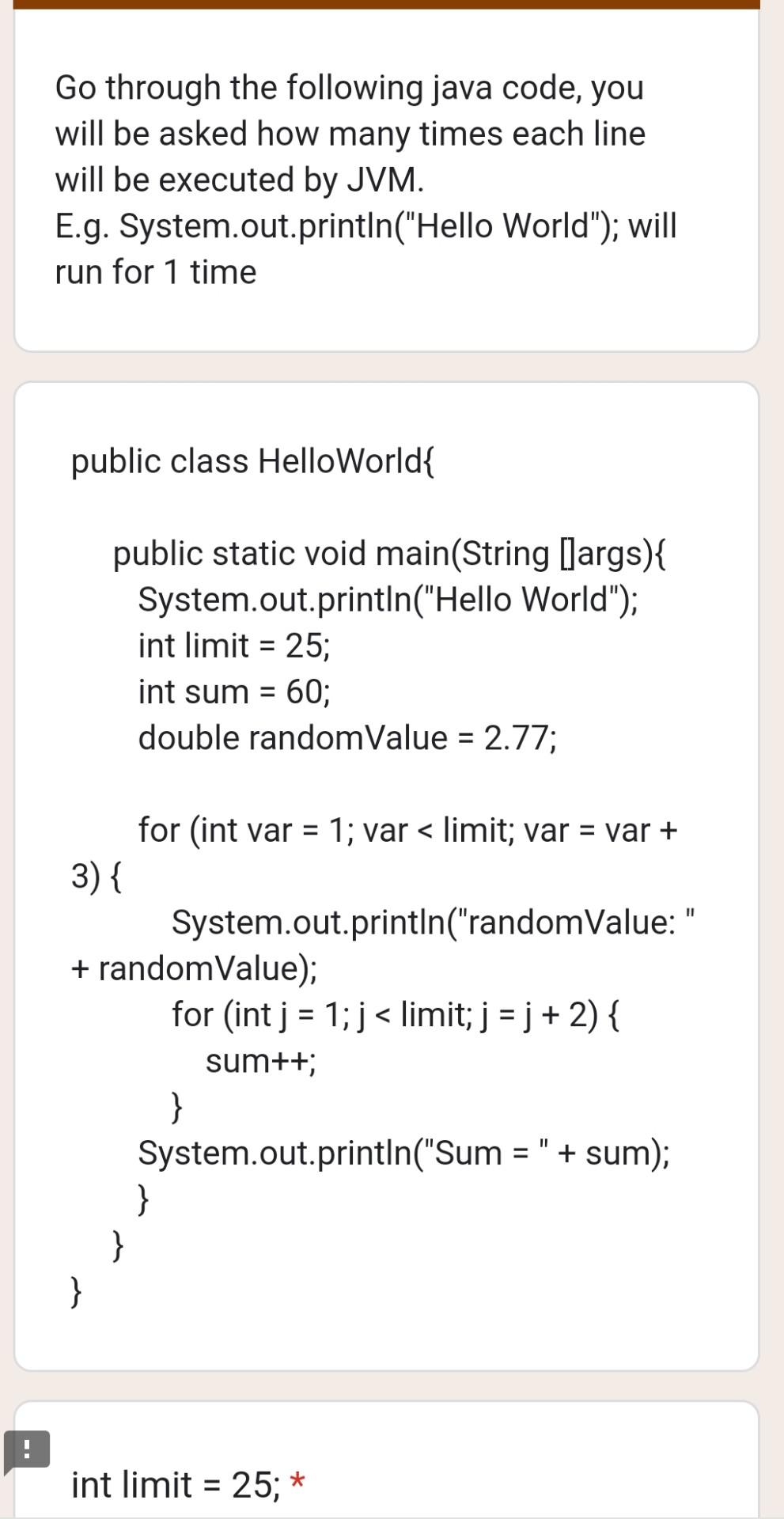
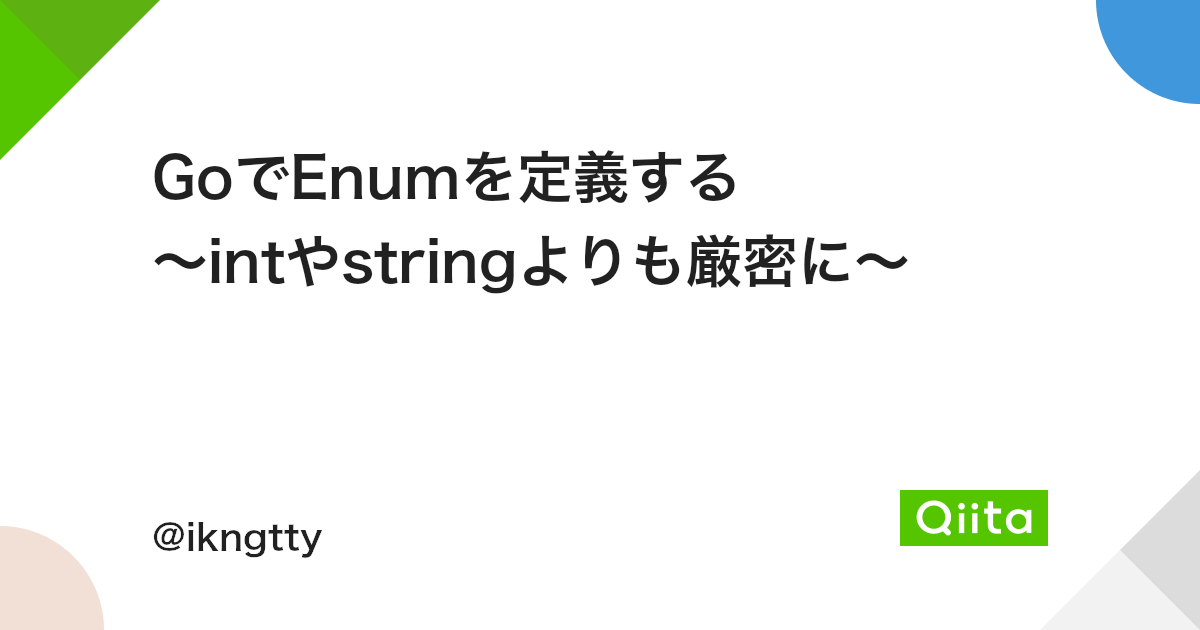
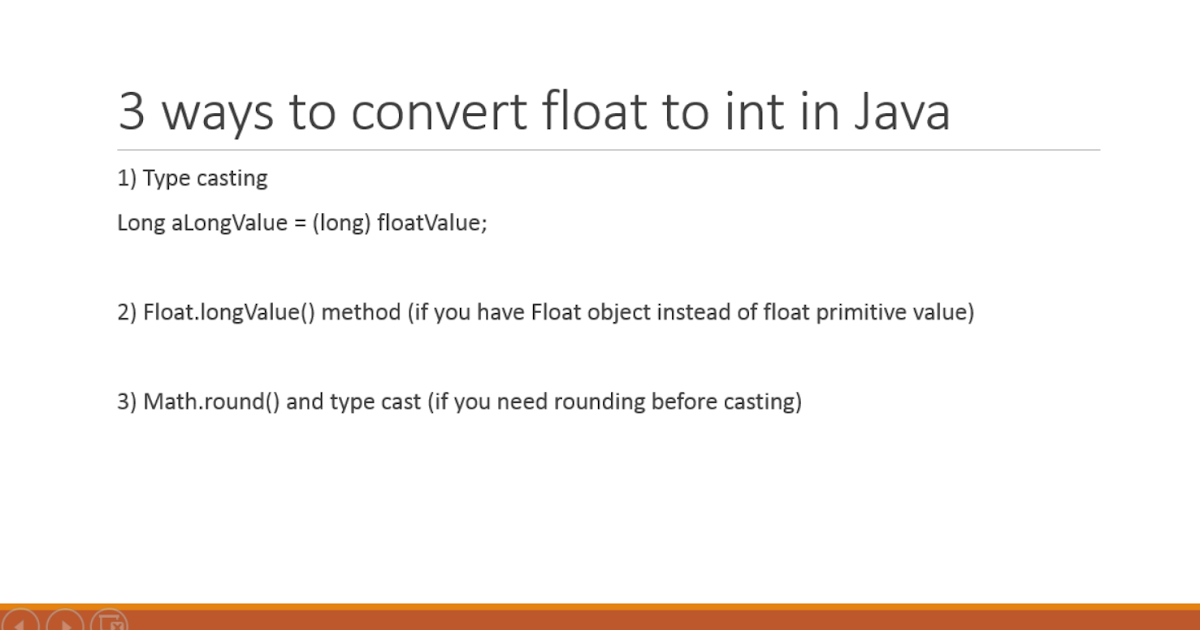
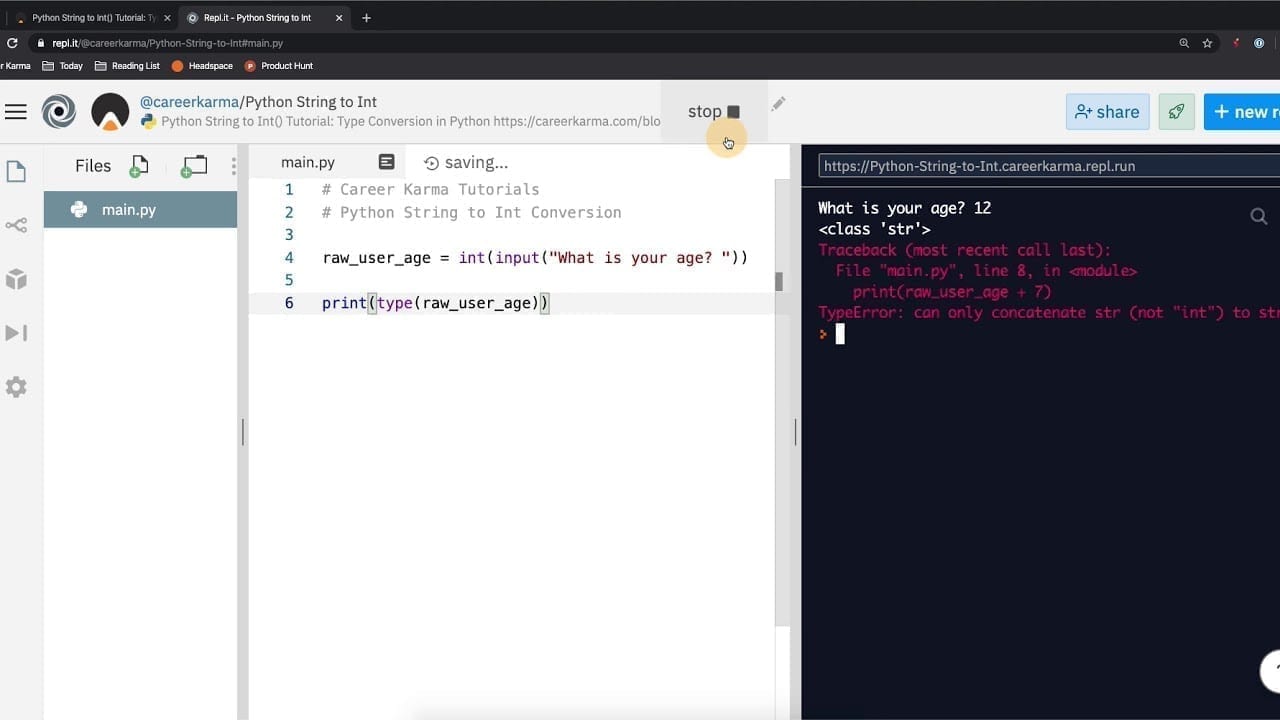
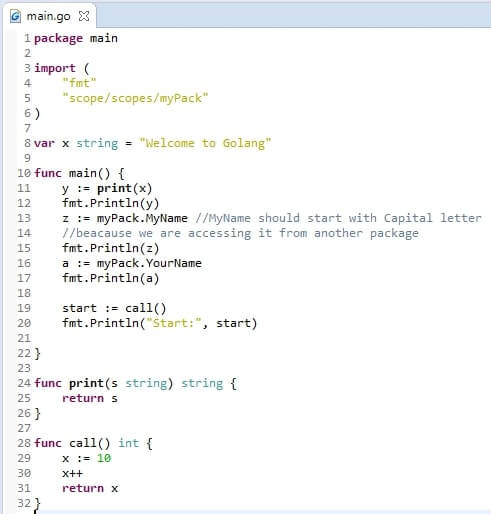
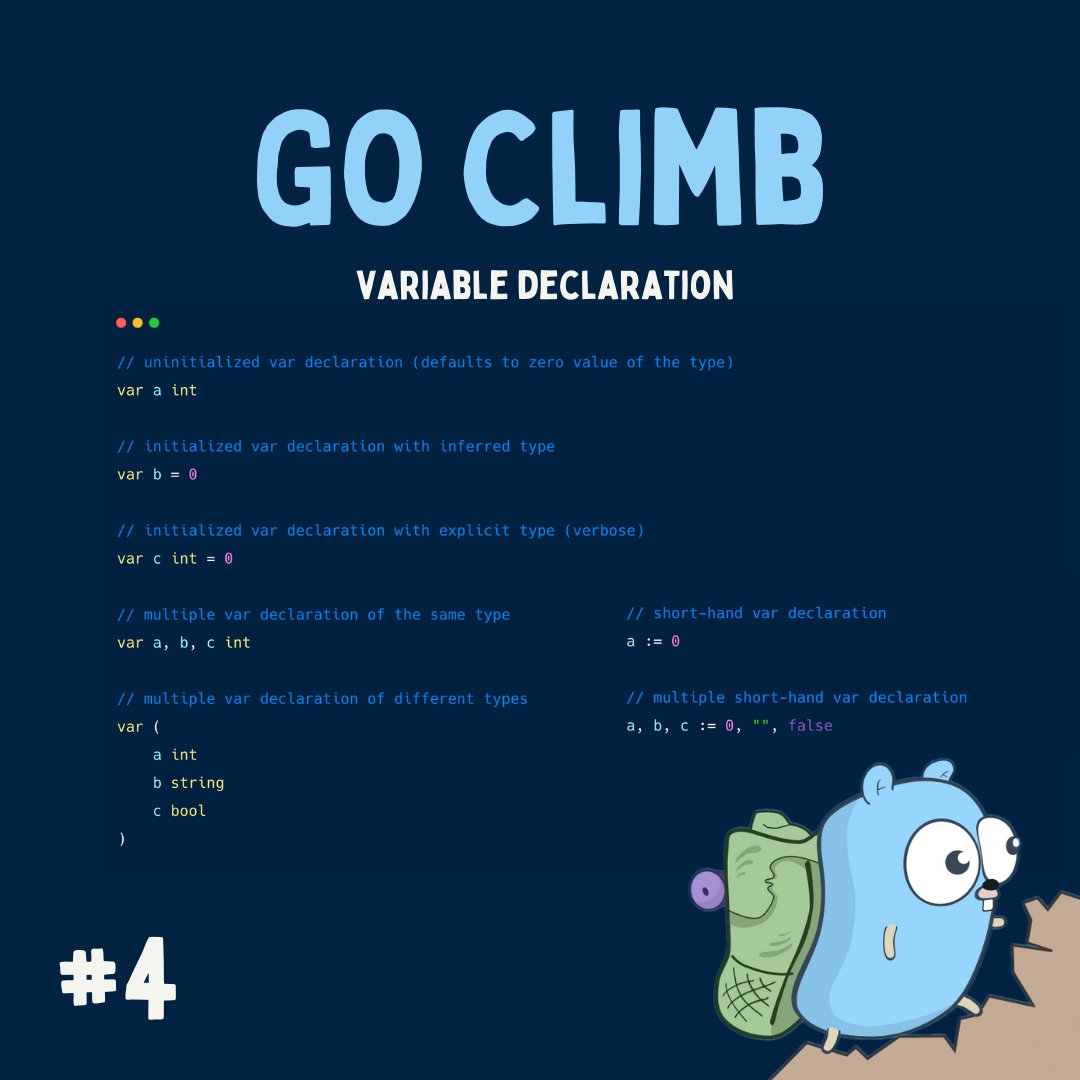
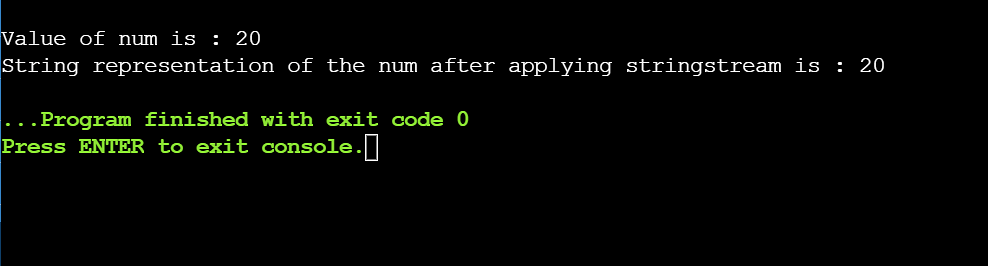
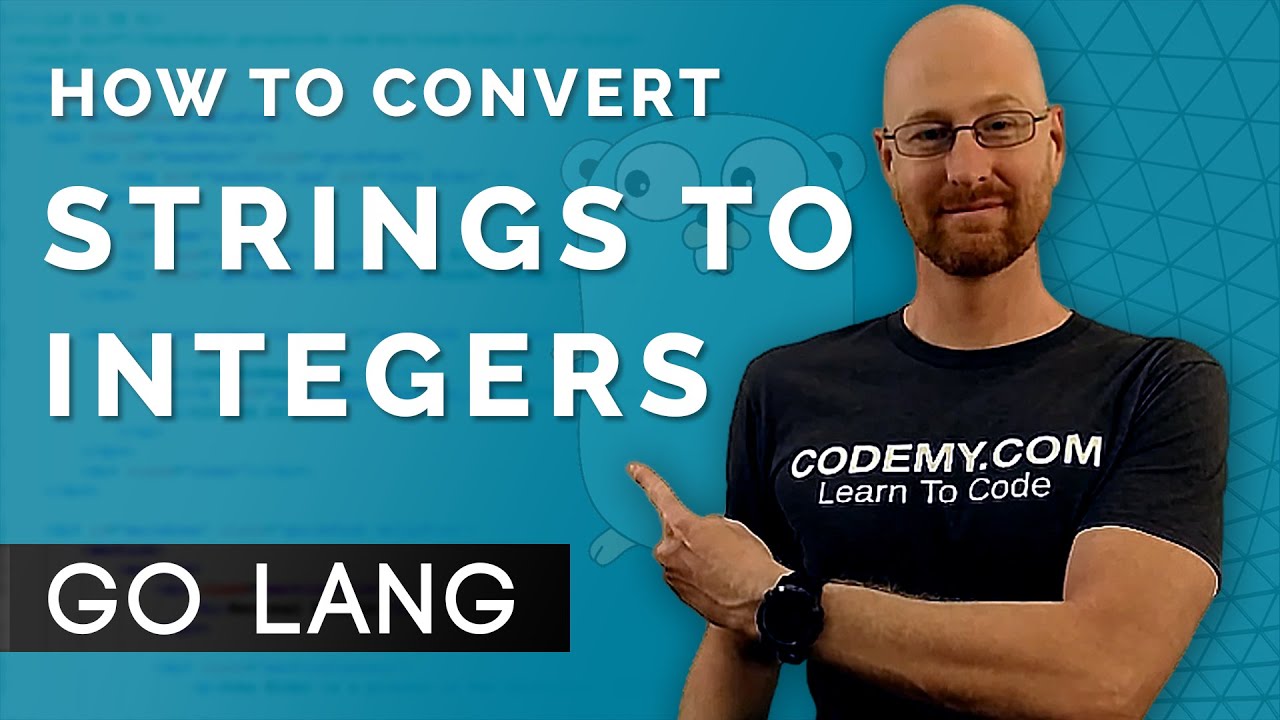
![go - golang - converting [ ]Interface to [ ]strings or joined string - Stack Overflow Go - Golang - Converting [ ]Interface To [ ]Strings Or Joined String - Stack Overflow](https://i.stack.imgur.com/SRZ9V.png)
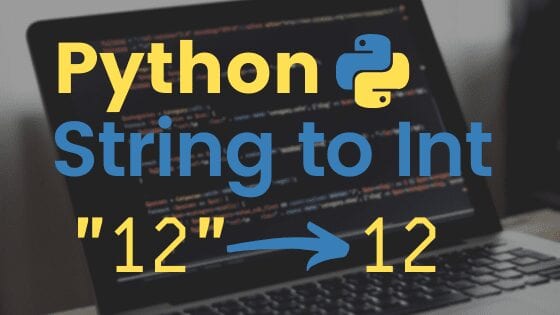

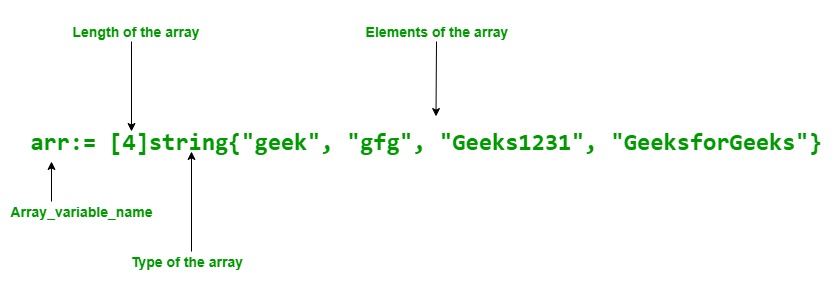

![Go-cheat-sheet - Go-cheat-sheet - Ranges s := []string{"a", "b", "c"} - Studocu Go-Cheat-Sheet - Go-Cheat-Sheet - Ranges S := []String{&Quot;A&Quot;, &Quot;B&Quot;, &Quot;C&Quot;} - Studocu](https://d20ohkaloyme4g.cloudfront.net/img/document_thumbnails/13e282784b2ddfaea0f41da52722e5b2/thumb_1200_1697.png)
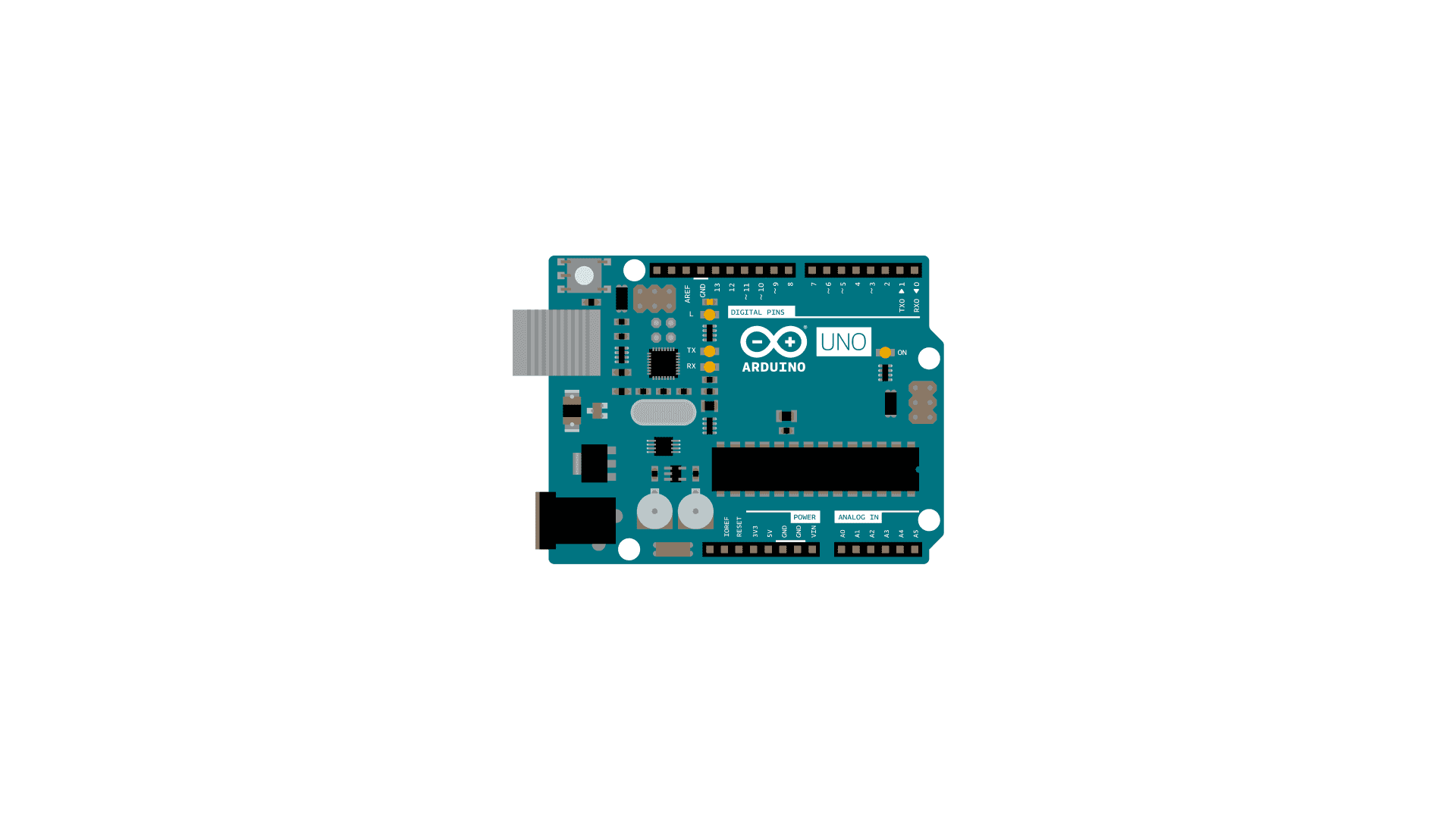

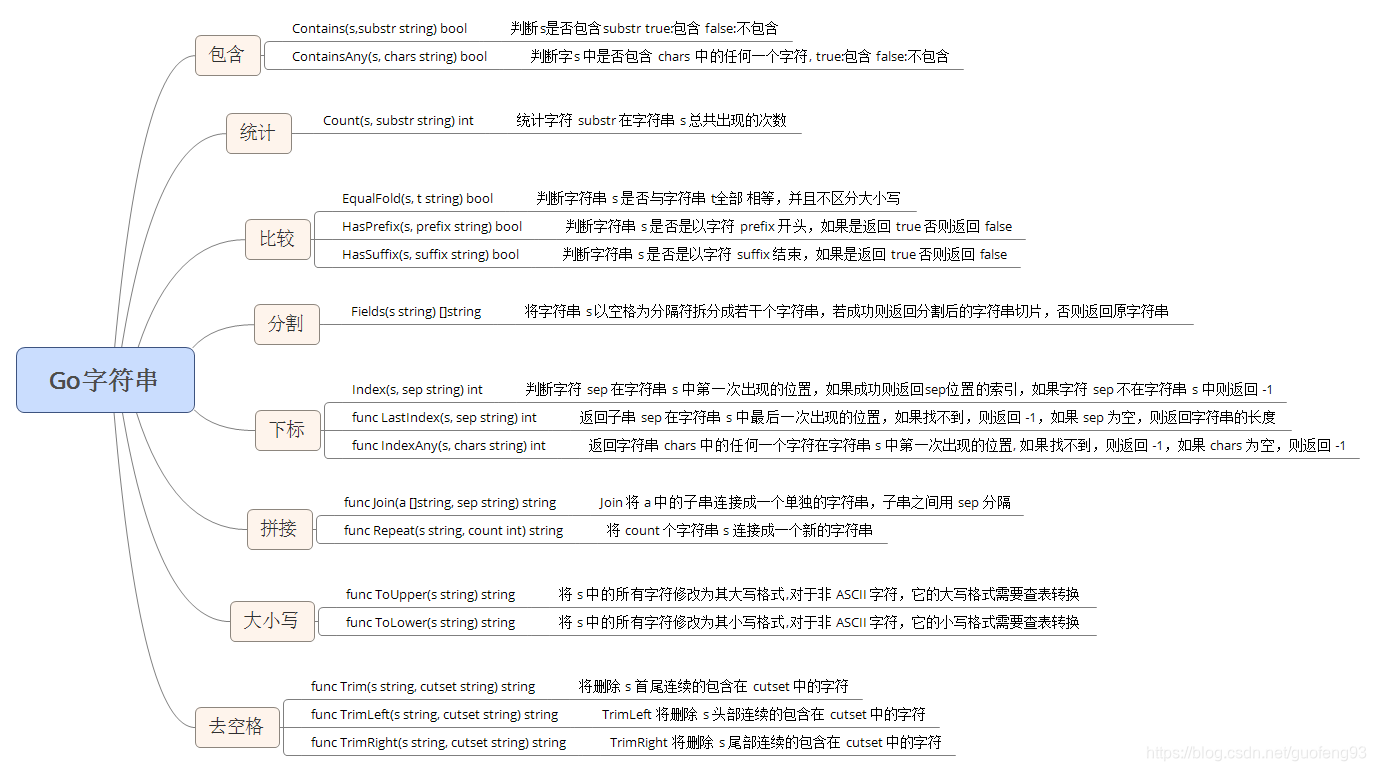
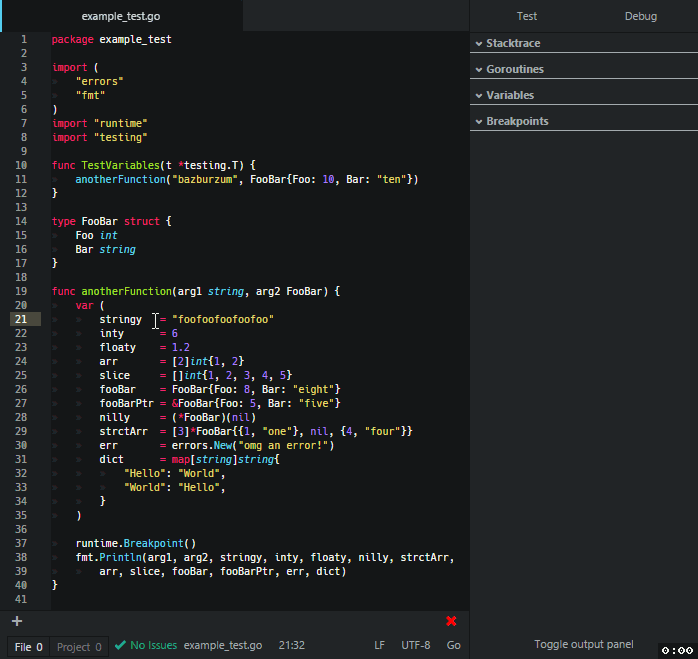
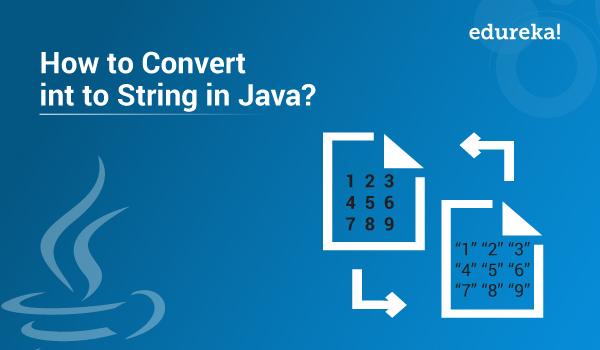

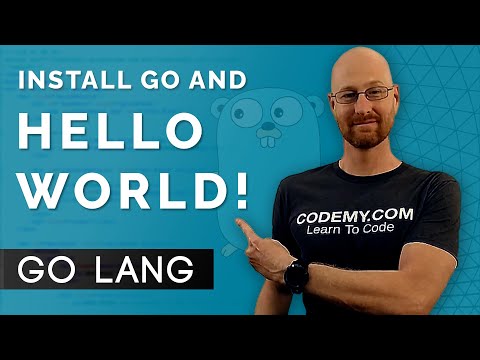
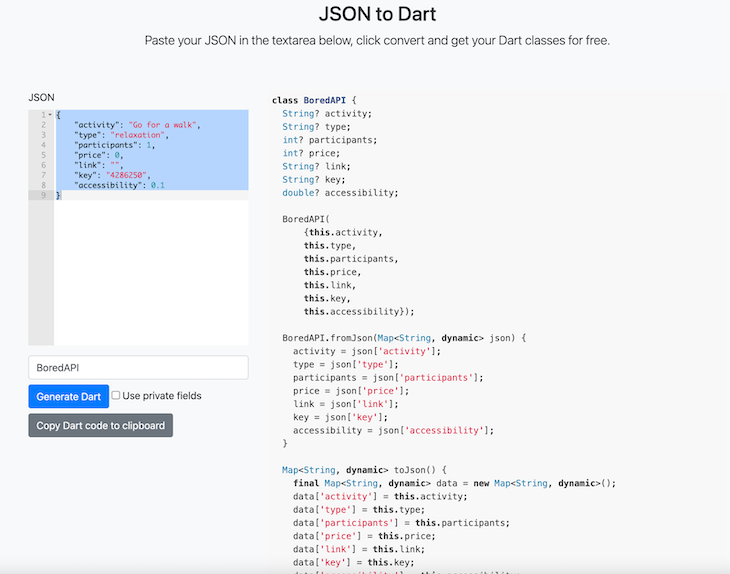
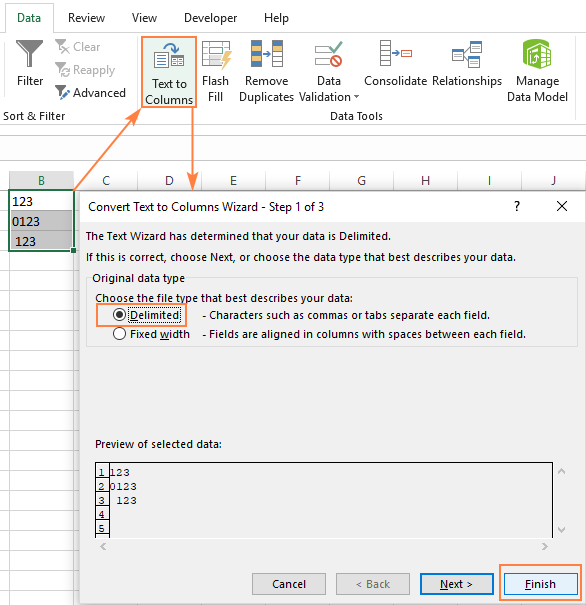
Article link: go string to int.
Learn more about the topic go string to int.
- How to Convert string to integer type in Go? – Eternal Dev
- Convert string to integer type in Go? – Stack Overflow
- How to Convert string to integer type in Go? – Golang Programs
- How to Convert string to integer type in Golang?
- Golang Program to convert string type variables into int
- How to convert String to Integer in Golang? – Tutorial Kart