Django Get Or Create
Introduction
When working with Django, you will often come across scenarios where you need to fetch data from a database or create a new object if it doesn’t exist. Fortunately, Django provides a convenient method for handling such situations through its “get_or_create” function. In this article, we will explore what Django get or create is, its benefits, how to use it, its syntax and parameters, exception handling, common mistakes, best practices, and several examples to demonstrate its versatility.
What is Django Get or Create?
Django Get or Create is a method offered by the Django ORM (Object-Relational Mapping) that allows you to retrieve an object from the database based on specified parameters or create a new object if none match the given criteria. It provides a concise and efficient way to handle the common “get or create” pattern with a single database query.
Benefits of Using Django Get or Create
1. Simplicity and Conciseness: Django Get or Create simplifies the code required to fetch or create objects. It eliminates the need for boilerplate code and reduces the number of queries sent to the database, resulting in optimized performance.
2. Atomicity: The get_or_create method is executed as an atomic operation, ensuring that multiple simultaneous requests do not interfere with each other. This means that there will be no race conditions when creating or retrieving objects.
3. Efficiency: By combining the operations of checking if an object exists and creating it if necessary into a single query, Django Get or Create reduces the number of database calls required. This not only enhances performance but also improves the scalability of your application.
How to Use Django Get or Create?
To use Django Get or Create, follow these simple steps:
1. Import get_or_create from Django:
“`python
from django.db.models import get_or_create
“`
2. Specify the model and any necessary parameters:
“`python
obj, created = ModelName.objects.get_or_create(parameter1=value1, parameter2=value2, …)
“`
Here, `ModelName` refers to the model you want to query, and `parameter` and `value` represent the attributes and values you are searching for.
3. Analyze the output:
– `obj` represents the retrieved or created object.
– `created` is a boolean value indicating if the object was newly created (True) or already existed in the database (False).
Syntax and Parameters of Django Get or Create
The syntax of the get_or_create method in Django is as follows:
“`python
ModelName.objects.get_or_create(defaults=None, **kwargs)
“`
The `defaults` parameter is optional and is used to specify default values while creating a new object. It takes a dictionary as input, where the keys represent the field names, and the values denote the default values.
The `**kwargs` parameter is mandatory and accepts keyword arguments. It allows you to pass the attributes and their corresponding values that will be used to filter the objects. Multiple keyword arguments can be provided to narrow down the search criteria.
Handling Exceptions in Django Get or Create
Django Get or Create raises two exceptions:
1. `MultipleObjectsReturned`: This exception is thrown when multiple objects are found matching the provided search criteria. To avoid this, ensure that your keyword arguments are specific enough to produce a unique result.
2. `DoesNotExist`: This exception is thrown when no objects are found matching the provided search criteria. You can catch this exception to handle such cases gracefully in your code.
Creating a New Object if It Doesn’t Exist
One of the prominent use cases of Django Get or Create is creating a new object if it doesn’t exist in the database. By using the `defaults` parameter, you can define default values for the object’s attributes during the creation process. If an object with the same attributes already exists, Django will retrieve it instead.
“`python
obj, created = ModelName.objects.get_or_create(defaults={‘attribute1’: value1, ‘attribute2’: value2, …}, **kwargs)
“`
Updating an Existing Object
If an object already exists in the database but needs to be updated with new values, you can modify its attributes using the `update()` method. This method updates the object’s fields and returns the number of objects updated.
“`python
obj, created = ModelName.objects.get_or_create(**kwargs)
if not created:
obj.attribute1 = new_value1
obj.attribute2 = new_value2
obj.save()
“`
Common Mistakes and Best Practices
1. Incorrect Keyword Arguments: Ensure that the keyword arguments provided match the attributes of the model. Mismatched or misspelled attributes can lead to unexpected behavior.
2. Handling Exceptions: Always handle the `MultipleObjectsReturned` and `DoesNotExist` exceptions appropriately in your code to avoid crashes or incorrect functionality.
3. Efficiency and Scalability: Use Django Get or Create judiciously to keep the number of database queries to a minimum. This helps maintain a scalable and efficient application.
Examples of Django Get or Create in Action
Let’s explore some practical examples to better understand the versatility of Django Get or Create.
1. DRF Get or Create:
“`python
from rest_framework import serializers
class ExampleSerializer(serializers.ModelSerializer):
def create(self, validated_data):
obj, created = ModelName.objects.get_or_create(**validated_data)
return obj
“`
2. Django Update or Create:
“`python
obj, created = ModelName.objects.update_or_create(defaults={‘attribute1’: value1, ‘attribute2’: value2, …}, **kwargs)
“`
3. Django Get or None:
“`python
try:
obj = ModelName.objects.get(**kwargs)
except ModelName.DoesNotExist:
obj = None
“`
4. Import get_or_create Django:
“`python
from django.db.models import get_or_create
“`
5. Django Create:
“`python
obj = ModelName.objects.create(attribute1=value1, attribute2=value2, …)
“`
6. DoesNotExist Django:
“`python
try:
obj = ModelName.objects.get(**kwargs)
except ModelName.DoesNotExist:
# Handle DoesNotExist exception
“`
7. Django-filter Example:
“`python
class ExampleFilter(django_filters.FilterSet):
class Meta:
model = ModelName
fields = {‘attribute1’: [‘exact’], ‘attribute2’: [‘contains’]}
“`
8. Annotate Django Get or Create:
“`python
from django.db.models import Count
obj, created = ModelName.objects.get_or_create(**kwargs).annotate(attribute_count=Count(‘related_attribute’))
“`
FAQs
Q1. Can Django Get or Create be used with related models?
A1. Yes, Django Get or Create can be used with related models by specifying the related attributes in the keyword arguments.
Q2. Does get_or_create support complex queries?
A2. Yes, get_or_create supports complex queries with multiple keyword arguments, allowing you to narrow down the search criteria.
Q3. How does get_or_create handle race conditions?
A3. Django’s get_or_create method is executed as an atomic operation, ensuring that concurrent requests do not interfere with each other.
Q4. Can I use get_or_create without specifying any keyword arguments?
A4. No, specifying keyword arguments is mandatory in order to filter or create objects.
Q5. Does get_or_create increase the database’s write load?
A5. While get_or_create does perform writes, it reduces the number of queries sent to the database, resulting in an optimized write load.
Conclusion
Django Get or Create is a powerful method that simplifies the retrieval and creation of objects in a Django application. Its concise syntax, atomicity, and efficiency make it an essential tool for working with databases. By understanding its usage, syntax, exception handling, and best practices, you can maximize the benefits provided by Django Get or Create and optimize your database operations effectively.
Correct Way To Use Get_Or_Create? | Get Or Create Django | Django Tips#6
Keywords searched by users: django get or create Drf get or create, Django update or create, Django get or none, Import get_or_create django, Django create, DoesNotExist django, django-filter example, Annotate Django
Categories: Top 68 Django Get Or Create
See more here: nhanvietluanvan.com
Drf Get Or Create
DRF Get Operation:
The get operation in DRF provides a mechanism to retrieve resources from the server. It allows users to query for specific data or retrieve individual resources based on certain criteria. The GET operation is often used to fetch data from the server to populate web pages, mobile apps, or other types of clients.
To implement a GET operation in DRF, you need to define a serializer class, which converts complex data types into Python native data types that can then be easily rendered into JSON, XML, or other formats. The serializer also handles deserialization, allowing parsed data to be converted back into complex types.
DRF’s get functionality is built upon the Django’s ORM (Object Relational Mapper), which abstracts the database layer and provides a simplified interface for interacting with the database. This powerful combination allows developers to write flexible and efficient queries to retrieve the desired data.
DRF Create Operation:
The create operation in DRF is used to create or insert new resources into the server. This operation is vital when you want to enable users to submit data and store it persistently. Like the get operation, the create operation also relies on serializer classes for data manipulation.
To create a resource, you need to define a view class that extends DRF’s `APIView` or one of its subclasses, such as `CreateAPIView`. This class provides the necessary methods to handle incoming requests and perform the create operation.
When a client sends a POST request to create a resource, DRF automatically applies data validation based on the serializer’s definition. This validation ensures that the submitted data adheres to the defined rules, such as required fields, data types, or custom validations. If the data fails validation, appropriate error messages are returned to the client.
Benefits of DRF Get and Create:
1. Rapid Development: DRF simplifies the process of building APIs by providing pre-built components, such as serializers and views, which handle most common use cases. This accelerates development by eliminating the need to write boilerplate code.
2. Flexibility: DRF allows developers to customize and extend its functionality to fit their specific requirements. This flexibility is crucial when dealing with complex data structures or unique business logic.
3. Authentication and Authorization: DRF includes robust authentication and authorization mechanisms, making it straightforward to secure your API endpoints. It supports various authentication methods, such as basic authentication, token-based authentication, or OAuth.
4. Serialization and Deserialization: DRF’s serializers handle the conversion between complex data types and simpler native types, facilitating data handling and making your code more readable and maintainable. The deserialization process enables you to receive and process data from clients seamlessly.
5. Database Interaction: By leveraging Django’s powerful ORM capabilities, DRF abstracts the complexities of database interaction. This allows developers to focus on writing business logic without worrying about low-level database operations.
Possible Use Cases:
1. Mobile Apps: DRF’s get and create functionality is perfect for building APIs that mobile apps can consume. Mobile apps can retrieve data from the server efficiently using GET operations and submit user-created data via POST requests.
2. Single-Page Applications (SPAs): SPAs often require frequent updates of data without refreshing the entire web page. DRF’s get operation provides a straightforward and efficient way to fetch data from the server and update the UI in real-time.
3. Internet of Things (IoT): When building applications for IoT devices that require data retrieval and storage, DRF’s get and create operations can be invaluable. You can fetch sensor data, settings, or issue commands using DRF’s GET operations and store new states or configurations using the create operation.
FAQs:
Q1: Can I use DRF with frameworks other than Django?
DRF is specifically built as an extension of Django and is tightly integrated with its ecosystem. While you may find some alternative frameworks for building APIs, DRF’s extensive features and seamless integration with Django make it the ideal choice for Django-based projects.
Q2: Can I combine both get and create operations in a single API endpoint?
Yes, it is possible to have an API endpoint that supports both GET and POST requests. DRF provides view classes, such as `ListCreateAPIView`, that combine both get and create operations, allowing you to handle multiple HTTP methods within a single API endpoint.
Q3: What is the difference between GET and POST requests?
GET requests are used to retrieve resources from the server, while POST requests are used to send data to the server for creating new resources. GET requests are generally read-only, and their execution should not have any side effects, while POST requests can have side effects like data creation or modification.
Q4: How can I handle authentication and authorization in DRF?
DRF provides multiple authentication and authorization options out of the box. You can configure your API views to require authentication using authentication classes like TokenAuthentication or SessionAuthentication, and control access using permission classes like IsAuthenticated or Django’s built-in permissions.
In conclusion, DRF’s get and create operations are essential components for building robust and scalable web APIs. Their flexible nature, seamless integration with Django, and extensive features make DRF the go-to choice for developers looking to implement powerful APIs efficiently. Whether you are building mobile apps, SPAs, or IoT applications, DRF’s get and create operations provide the necessary tools to handle data retrieval and creation smoothly.
Django Update Or Create
Django, a high-level Python web framework, offers a wide range of powerful tools and functionalities to simplify the process of building web applications. One such feature is the update or create operation, which allows developers to efficiently update existing records or create new ones if they don’t already exist. In this article, we will delve into the details of Django’s update or create operation, its benefits, and provide some helpful tips and examples. So, let’s get started!
Understanding Django’s Update or Create Operation:
Django’s update or create operation combines two essential operations – update and create – into a single efficient database query. This operation is particularly useful when working with models that have unique constraints, such as unique fields or unique together constraints. It helps developers avoid unnecessary queries and simplifies code maintenance.
When using the update or create operation, Django attempts to update an existing record that matches the provided query parameters. If the record does not exist, Django creates a new record using the provided data. This operation eliminates the need for additional queries to check for existing records before performing an update or create action.
Benefits of Using Django’s Update or Create Operation:
1. Increased Efficiency: By combining the update and create operations, Django reduces the number of database queries required, leading to improved performance and reduced response times.
2. Simplified Code: The update or create operation simplifies code and reduces the chances of errors. Developers can focus on writing clean and concise code without worrying about handling update and create operations separately.
3. Maintain Data Integrity: Django’s unique constraint handling ensures that duplicate records are not created when using the update or create operation. This prevents data redundancy and maintains data integrity within the database.
4. Easy Migration: When migrating data from one database to another or during version updates, the update or create operation smoothly handles the process without causing any issues related to duplicate records or failed updates.
5. Enhanced Readability: The update or create operation is highly readable, making it easier for developers to understand and maintain the codebase.
How to Implement Django’s Update or Create Operation:
To leverage Django’s update or create operation, you need to follow these simple steps:
1. Define a unique constraint on the relevant fields in your model. For example, in Django’s models.py file, you can add `unique=True` to a field definition to specify a unique constraint.
2. Prepare the data you wish to update or create. Organize the data in a dictionary format to match the fields of your model.
3. Use the `update_or_create()` method, available in Django’s Django QuerySet API, to perform the update or create operation. This method accepts two parameters – `defaults` and `**kwargs`. `Defaults` is a dictionary containing the data to update, while `**kwargs` defines the query parameters to match the existing record.
4. Handle the returned values or exceptions as per your application’s requirements. The `update_or_create()` method returns a tuple consisting of the updated object and a boolean value indicating whether a new object was created or not.
Example Code:
Let’s demonstrate the usage of Django’s update or create operation with an example:
“`python
from myapp.models import Article
data = {
“title”: “Django Update or Create”,
“content”: “Efficiency and simplicity combined”,
}
article, created = Article.objects.update_or_create(
title=”Django Update or Create”,
defaults=data
)
if created:
print(“A new article has been created!”)
else:
print(“The article has been updated!”)
“`
In this example, we update or create an article with a title “Django Update or Create”. If the article already exists, it will be updated with the provided data. Otherwise, a new article will be created using the same data.
FAQs:
Q1. Can I use the update_or_create() method with multiple fields to define the uniqueness constraint?
A1. Yes, you can define a unique together constraint in your model’s Meta class. By using the update_or_create() method with multiple field values, Django ensures that the combination of those fields remains unique.
Q2. What happens if an exception occurs during the update or create operation?
A2. The update_or_create() method raises an exception if any database error occurs during the operation. You can wrap the method call in a try-except block to handle and log the exception accordingly.
Q3. Is there any additional overhead in terms of performance when using update_or_create() over separate update and create operations?
A3. No, Django’s update_or_create() operation is designed to optimize performance by reducing the number of database queries. It is a more efficient and recommended approach compared to handling updates and creates separately.
Q4. Does the update_or_create() method work with related models?
A4. Yes, the update_or_create() method can be used with related models by specifying the related field values in the query parameters. Django takes care of handling the update or create operation for related models seamlessly.
Conclusion:
Django’s update or create operation simplifies the process of updating existing records or creating new ones, while ensuring data integrity and optimizing performance. By leveraging this powerful feature, developers can write cleaner and more efficient code, resulting in faster web application development. The update or create operation is a testament to Django’s commitment to providing developers with tools that streamline development and simplify complex database operations.
Django Get Or None
When working with Django, one of the most powerful features is its ORM (Object-Relational Mapping) system, which allows developers to interact with the database using Python objects. This greatly simplifies the process of querying the database and manipulating data. In this article, we will explore the `get()` and `none()` methods in Django and discuss how they can be utilized to streamline our queries and handle various scenarios.
Understanding the `get()` Method:
The `get()` method provided by Django’s ORM allows us to retrieve a single object from the database based on certain criteria. The syntax of the `get()` method is as follows:
“`python
ModelName.objects.get([field_name=value])
“`
Here, `ModelName` refers to the Django model we want to query, and `[field_name=value]` represents the condition to filter the objects. For instance, if we have a model called `Person` with a field called `name`, we can retrieve a person object with the name “John” as follows:
“`python
person = Person.objects.get(name=”John”)
“`
If no object matching the specified condition is found, the `get()` method will raise a `ModelName.DoesNotExist` exception. Therefore, it is essential to handle this exception appropriately, especially when dealing with user input or conditional querying.
Introducing the `none()` Method:
Unlike the `get()` method, which retrieves a single object, the `none()` method returns an empty queryset. This can be useful when we want to write complex queries with conditional logic. For instance, consider a scenario where we want to retrieve all people whose age is greater than 30. However, if the age field is not provided or has an invalid value, we want to retrieve all objects. We can achieve this using the `none()` method, as shown below:
“`python
age = request.GET.get(‘age’)
if age and age.isnumeric():
queryset = Person.objects.filter(age__gt=int(age))
else:
queryset = Person.objects.none()
“`
In the above code, we first check if the `age` parameter is provided and is a valid numeric value. If it meets the criteria, we filter the `Person` objects using the `gt` (greater than) lookup. Otherwise, we set the queryset to `none()` which will return no objects.
FAQs:
Q1. Can the `get()` method be used with multiple conditions?
Yes, the `get()` method can be used with multiple conditions by combining them with the `__` (double underscore) notation. For example:
“`python
person = Person.objects.get(name=”John”, age=30)
“`
In the above code, we retrieve a `Person` object with the name “John” and age 30.
Q2. What happens if multiple objects match the `get()` condition?
If multiple objects match the `get()` condition, a `ModelName.MultipleObjectsReturned` exception is raised. To handle such scenarios, it is recommended to use the `filter()` method instead.
Q3. How can the `none()` method be useful in practice?
The `none()` method is particularly useful when constructing complex queries with conditional logic. It allows us to seamlessly write conditional filters and return an empty queryset when certain conditions are not met. This helps in ensuring consistent behavior even when dealing with dynamic inputs.
Q4. When should I use `get()` instead of `filter()`?
The `get()` method is useful when we expect a single object to match the specified condition. It can simplify the code by directly retrieving the object or raising an exception if no object is found. On the other hand, the `filter()` method returns a queryset containing all objects that match the condition, even if there is a single object. Therefore, it is recommended to use `get()` only when we are confident that a single object will fulfill the query condition.
Q5. Can we combine `get()` and `none()` methods in a single query?
No, the `get()` and `none()` methods cannot be combined in a single query. Each method serves a distinct purpose, and their usage depends on the specific requirements of the query.
In conclusion, the `get()` and `none()` methods provided by Django’s ORM are powerful tools for querying the database and handling various scenarios. While the `get()` method retrieves a single object based on a specified condition, the `none()` method returns an empty queryset. By utilizing these methods appropriately, developers can ensure efficient and flexible querying in Django applications.
Images related to the topic django get or create
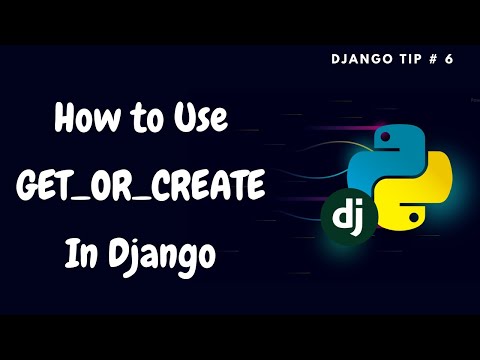
Found 31 images related to django get or create theme



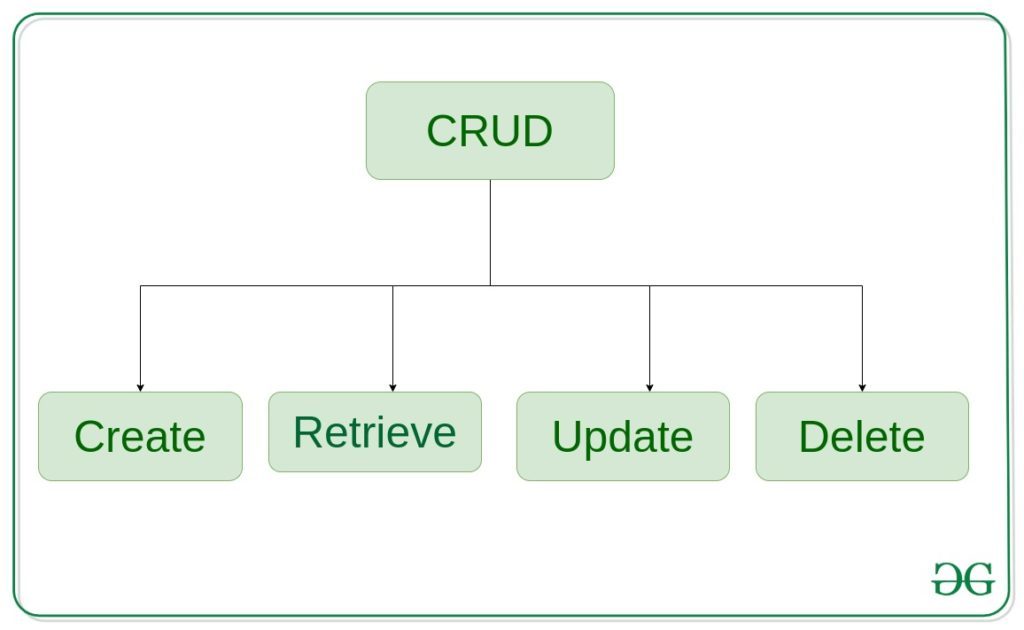


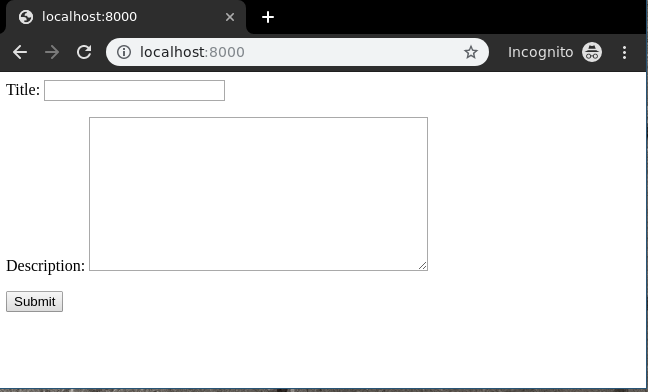
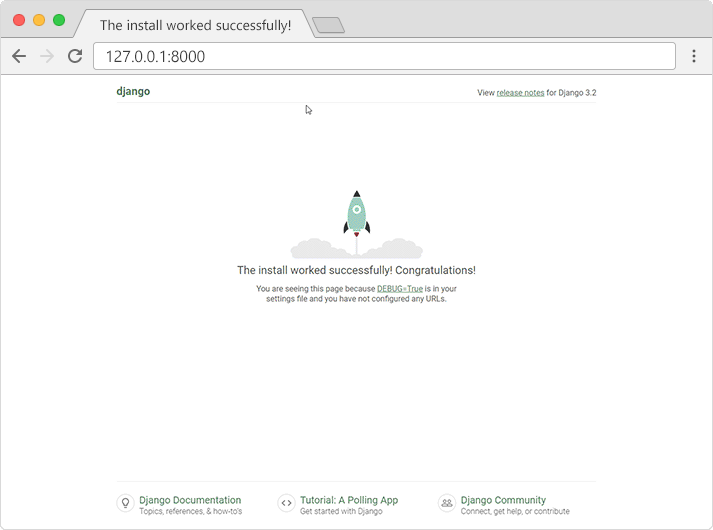
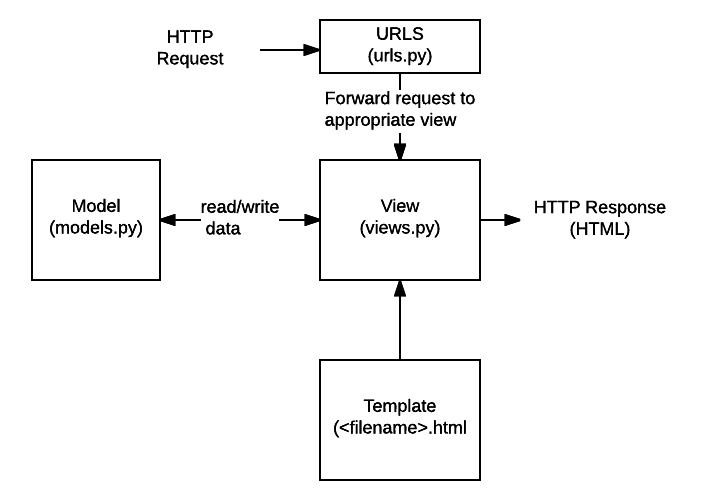
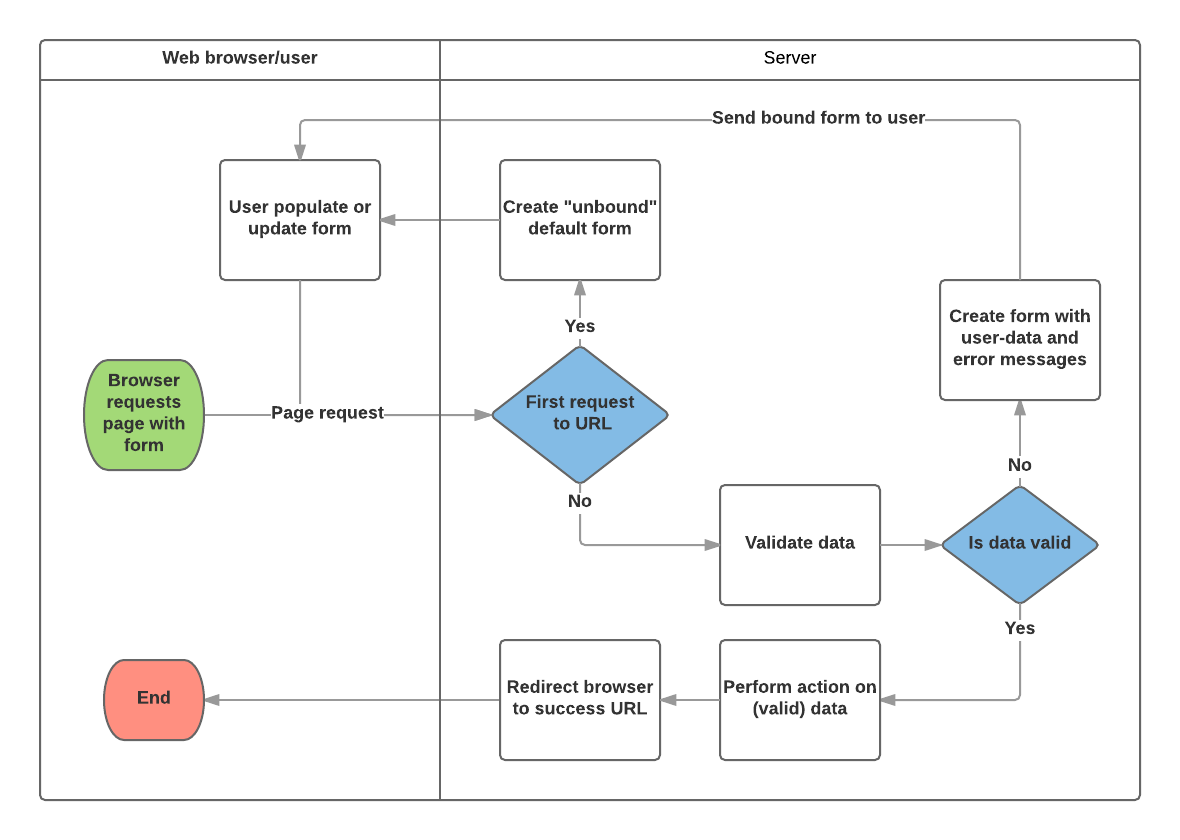
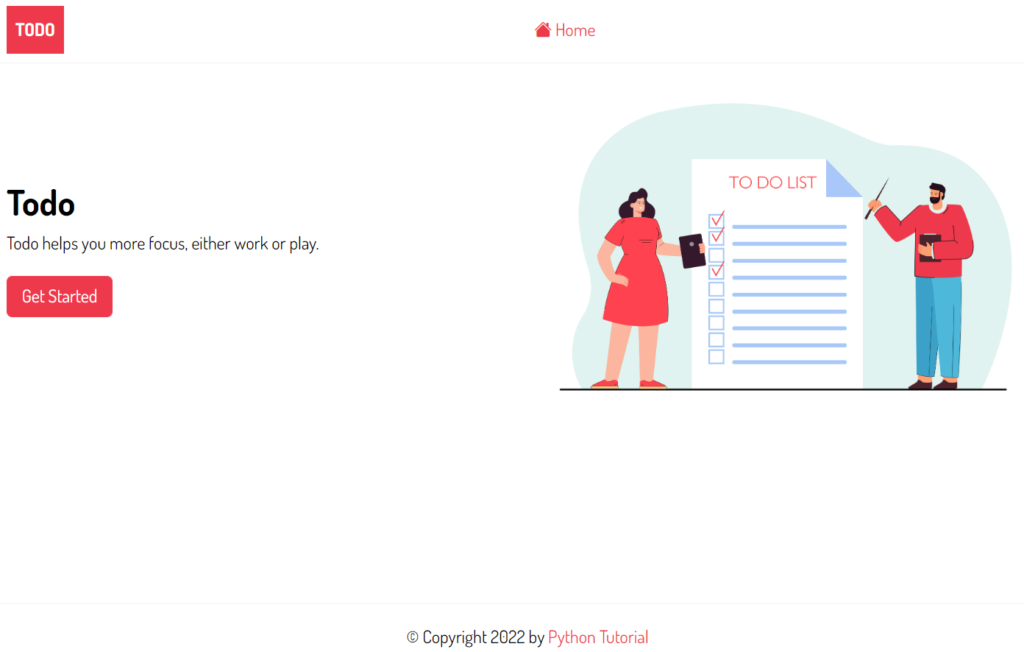
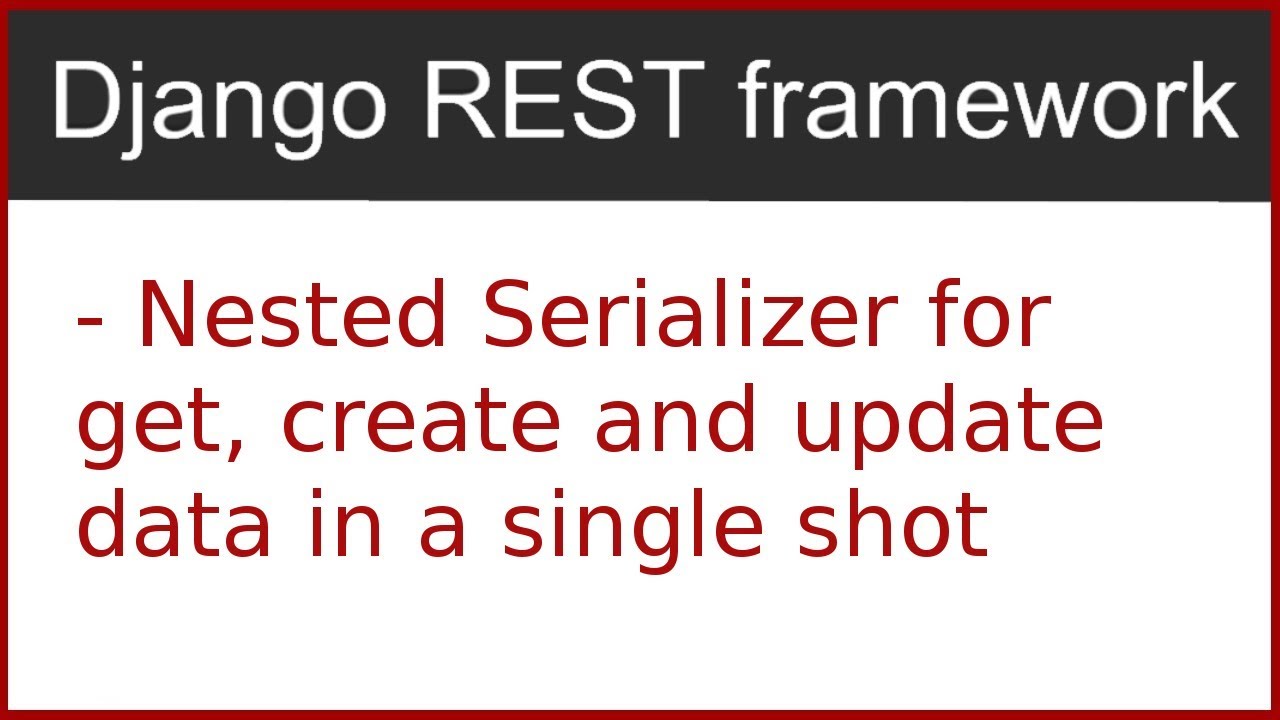
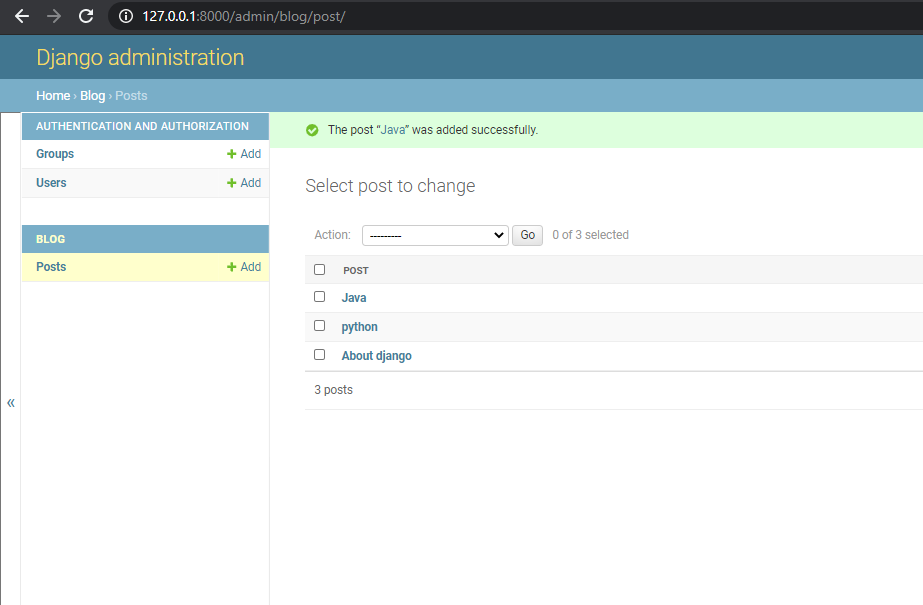
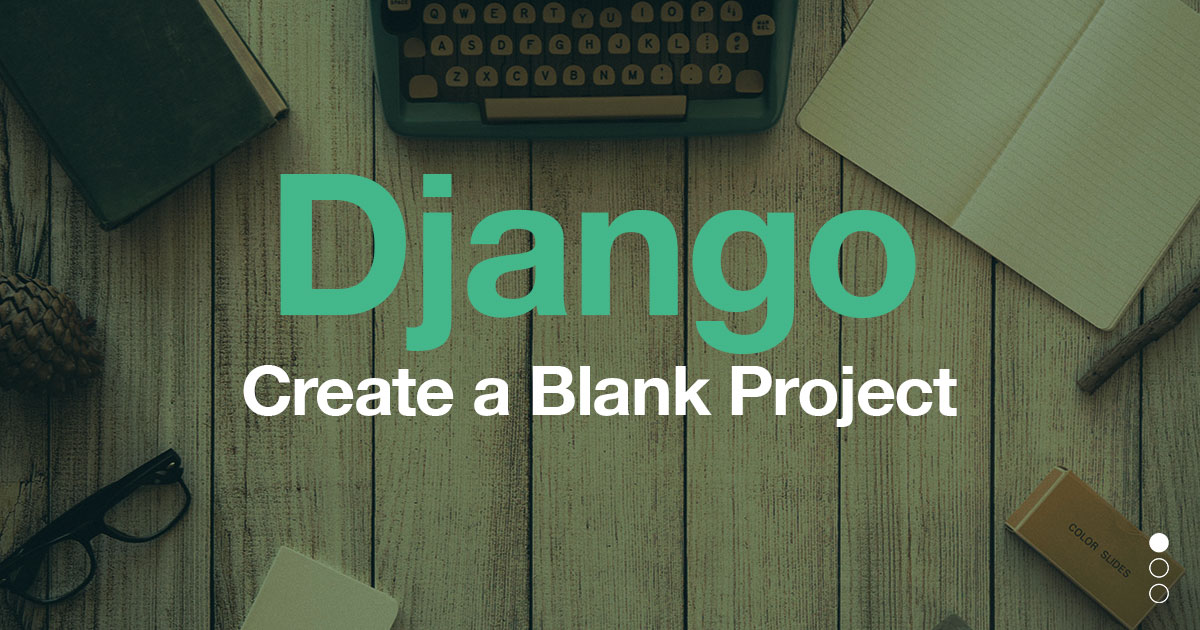

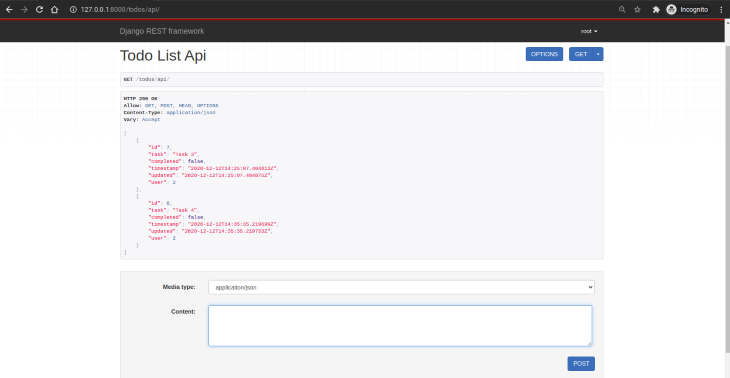
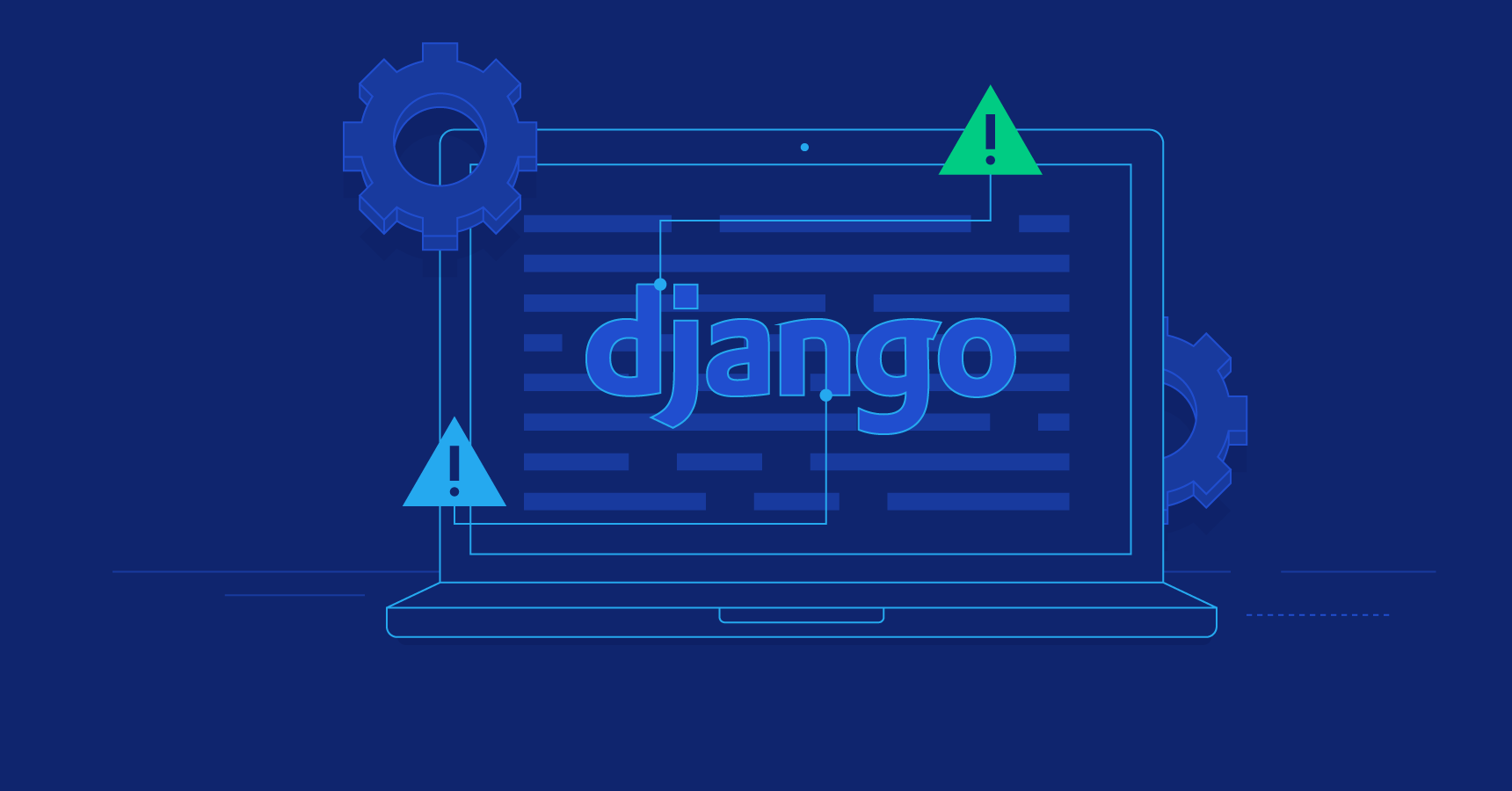

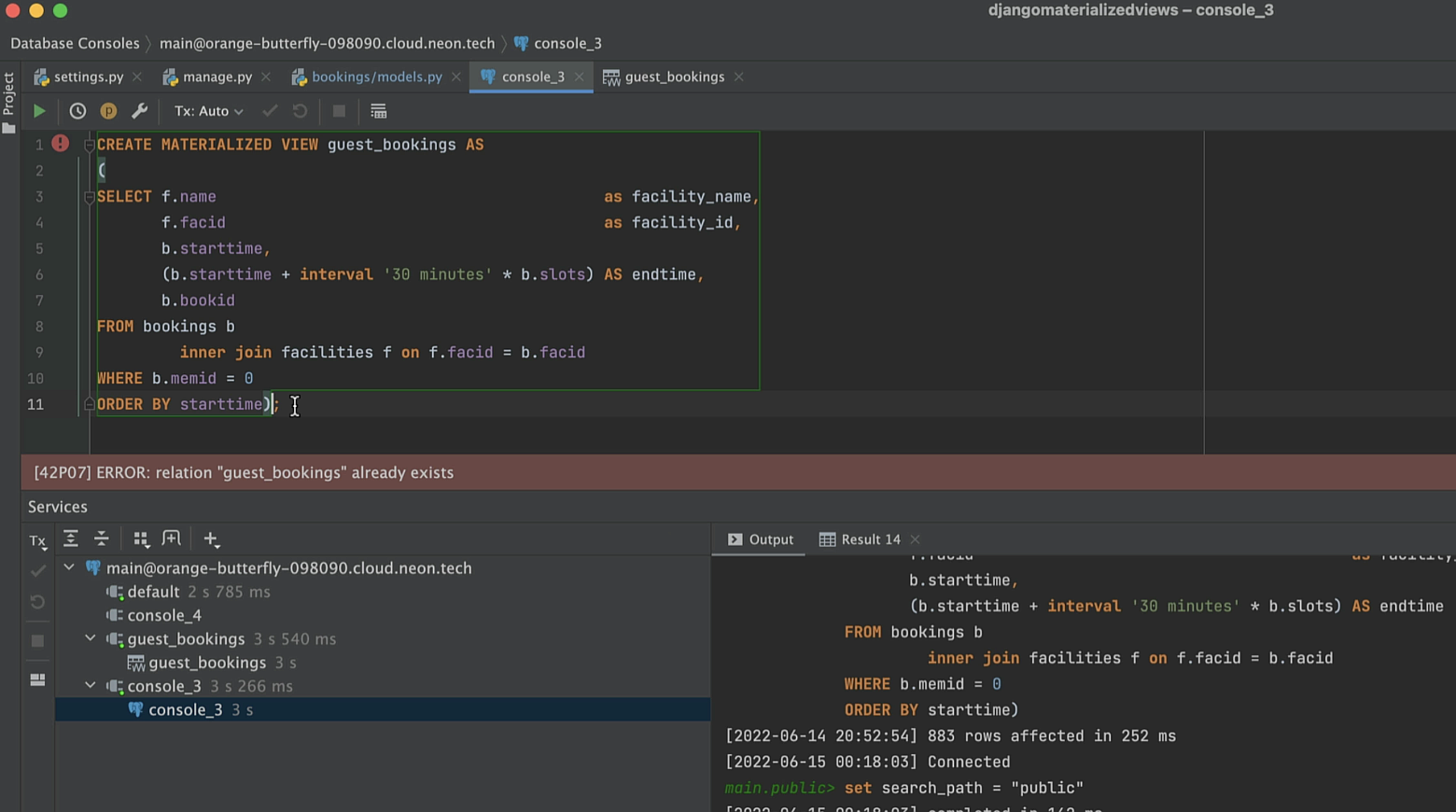
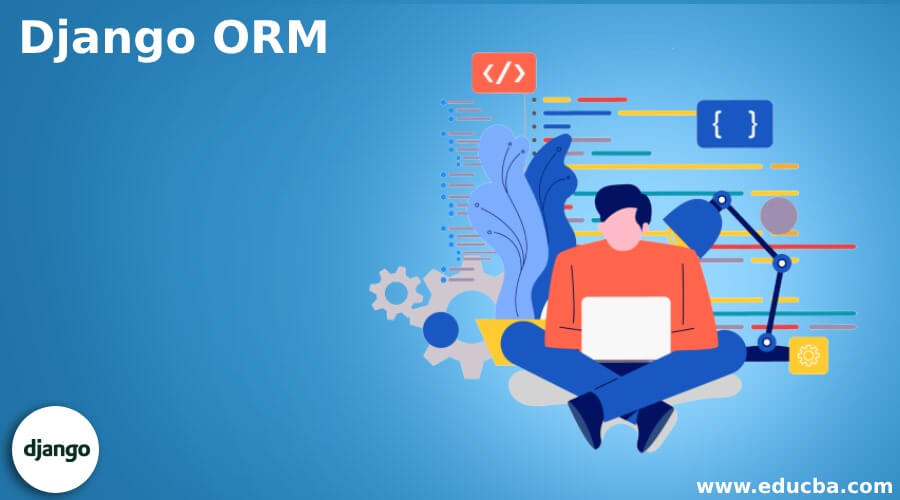
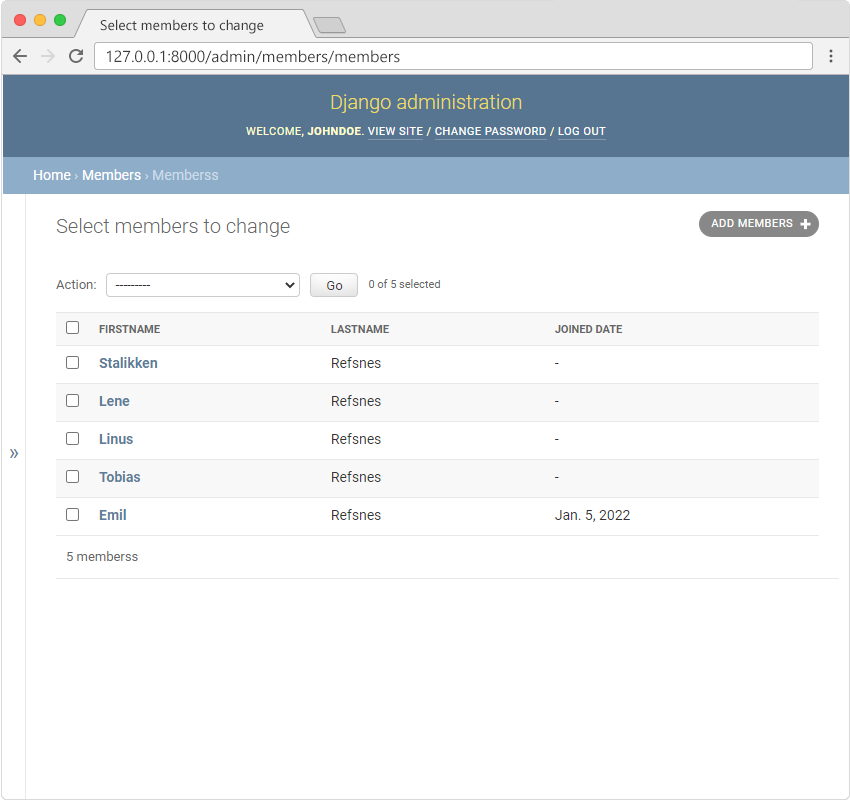


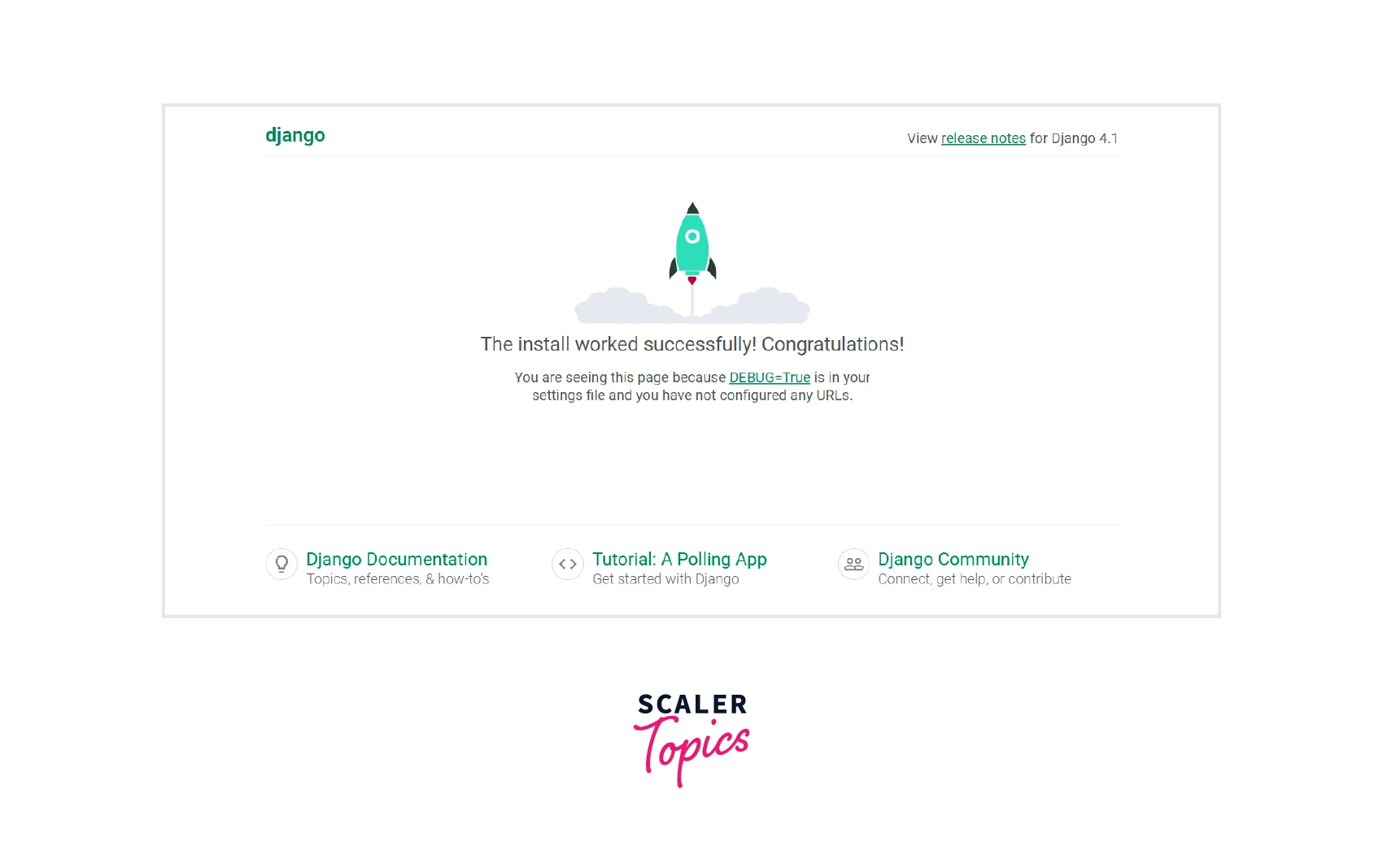
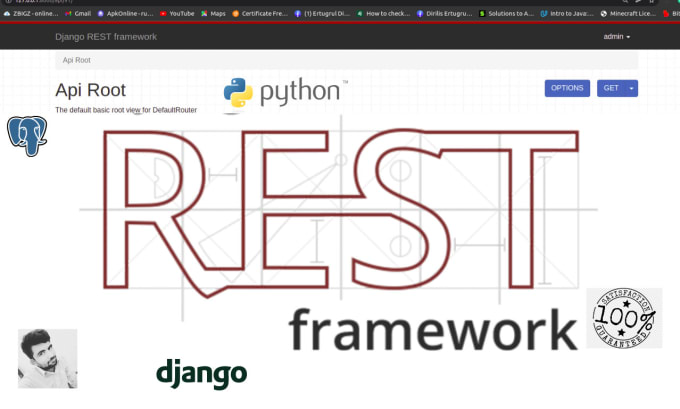
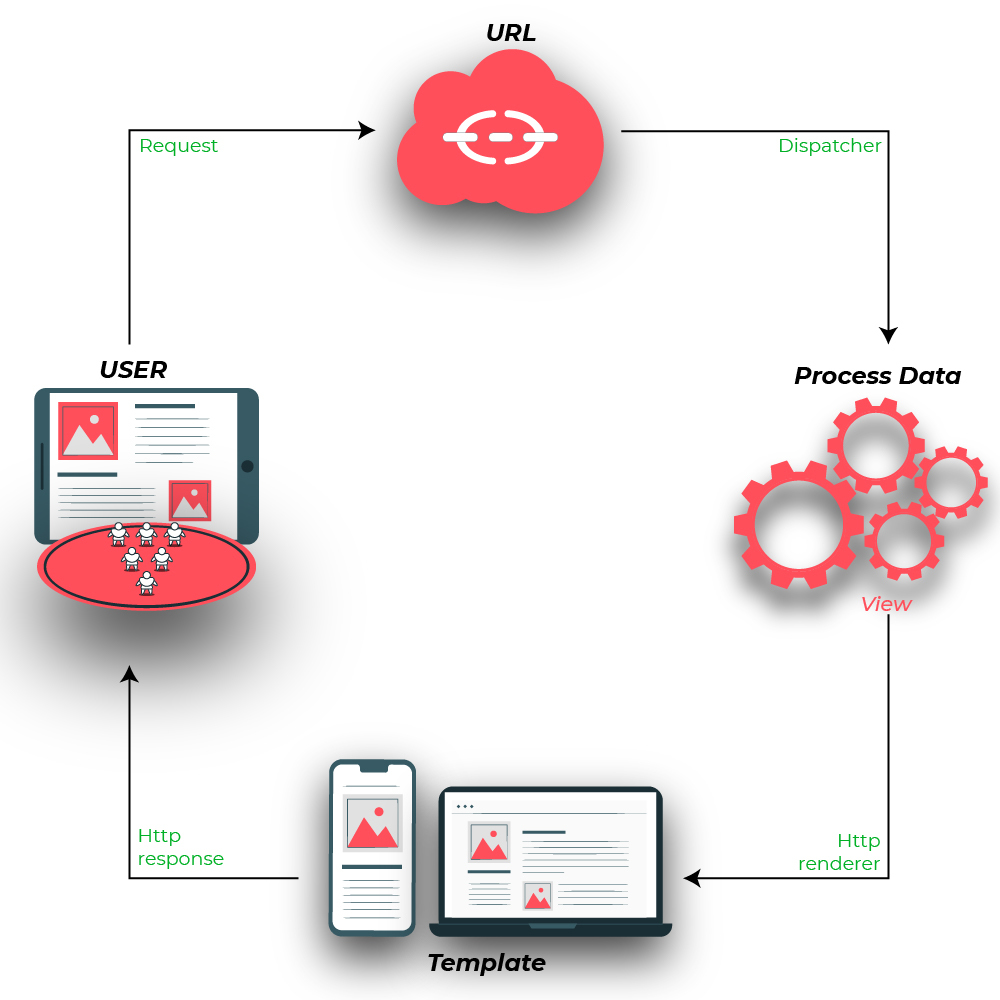


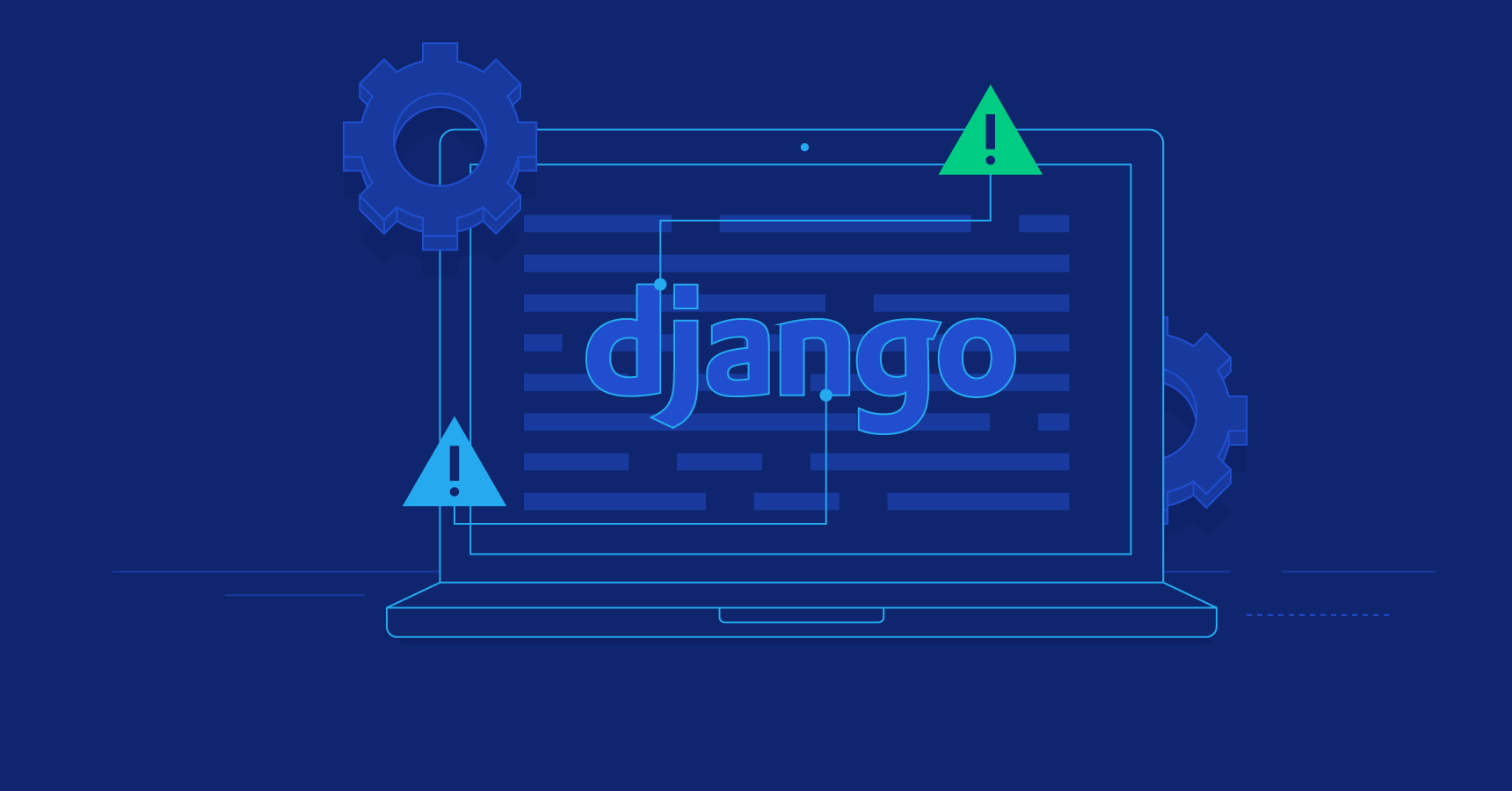

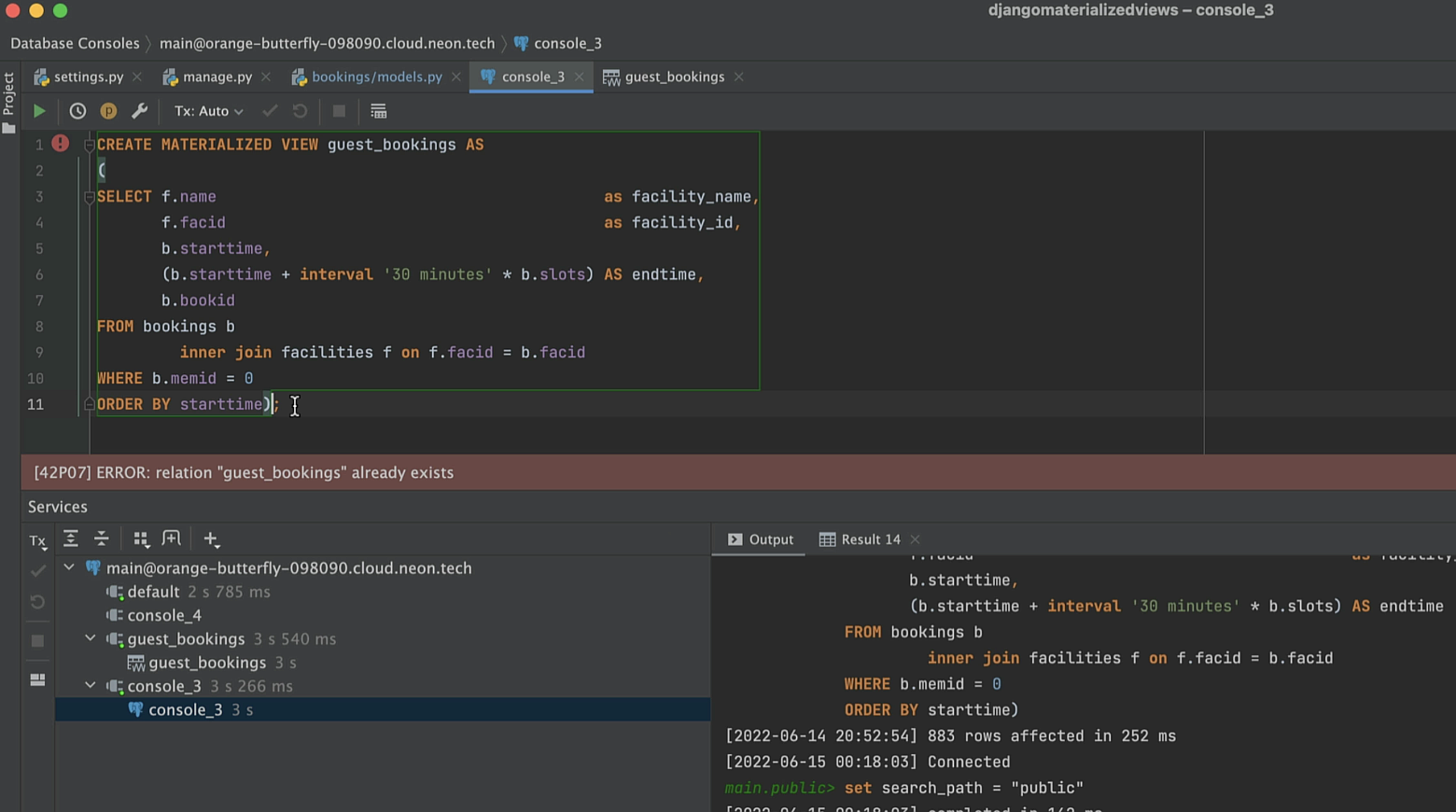
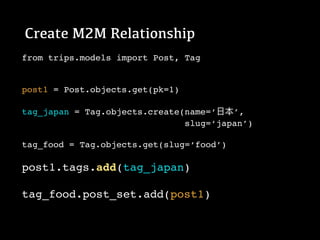

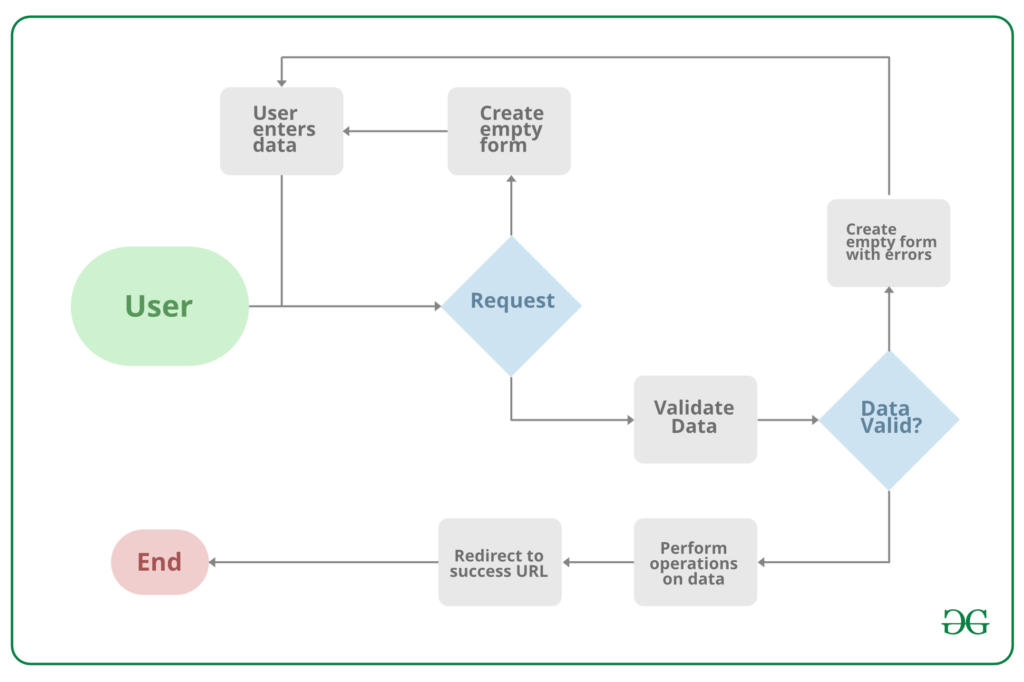

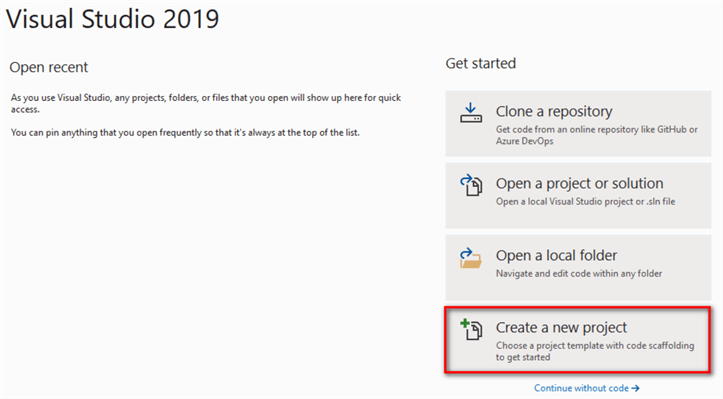
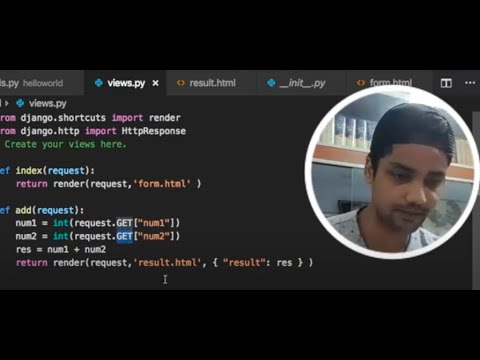

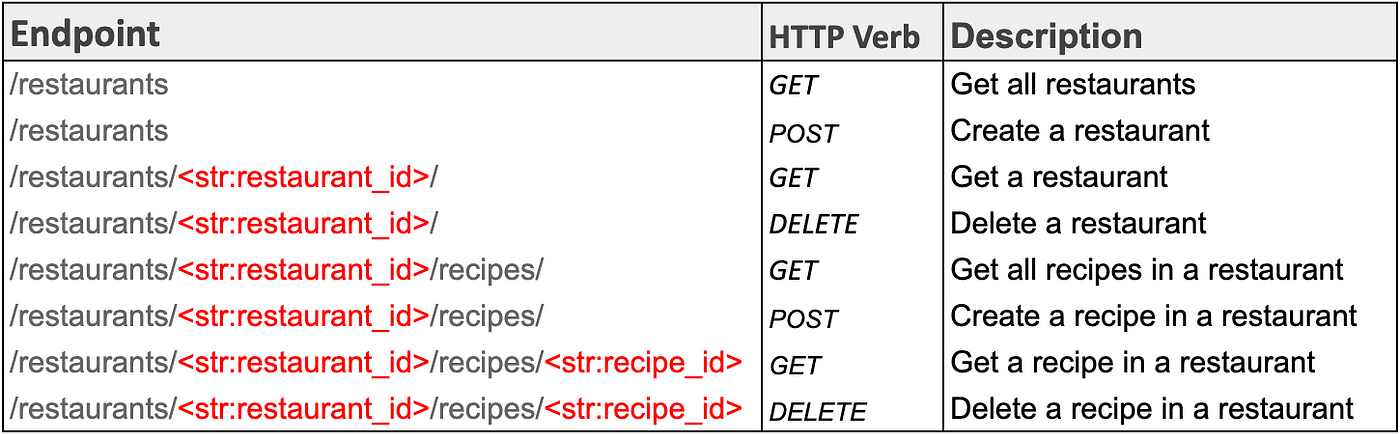
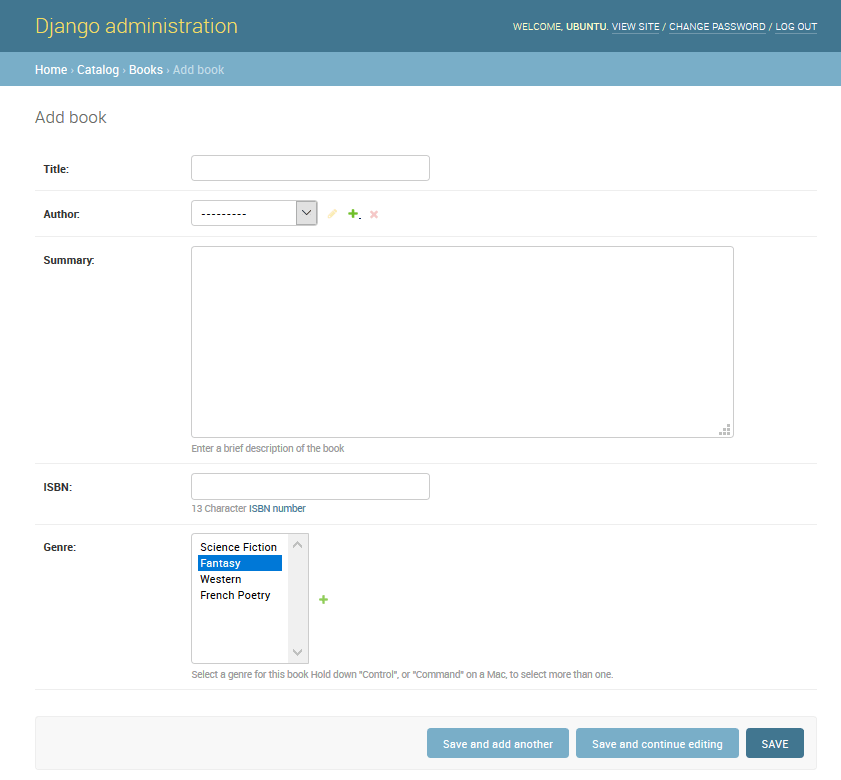

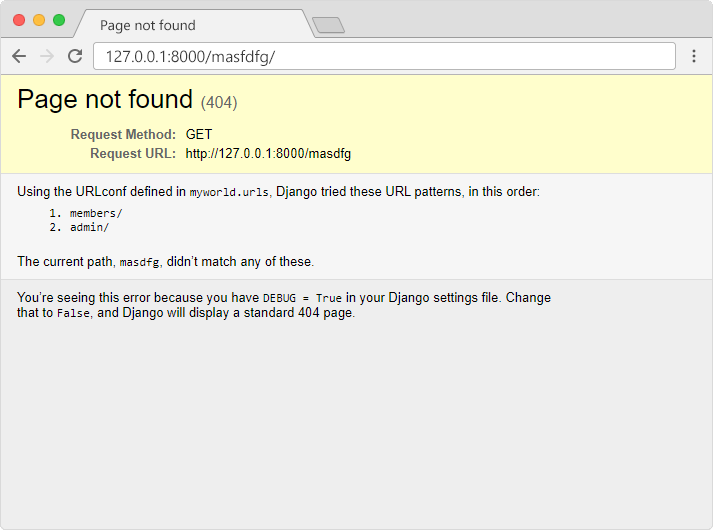

![Top Django Courses Online - Updated [July 2023] | Udemy Top Django Courses Online - Updated [July 2023] | Udemy](https://img-c.udemycdn.com/course/240x135/2045310_f8a2_6.jpg)
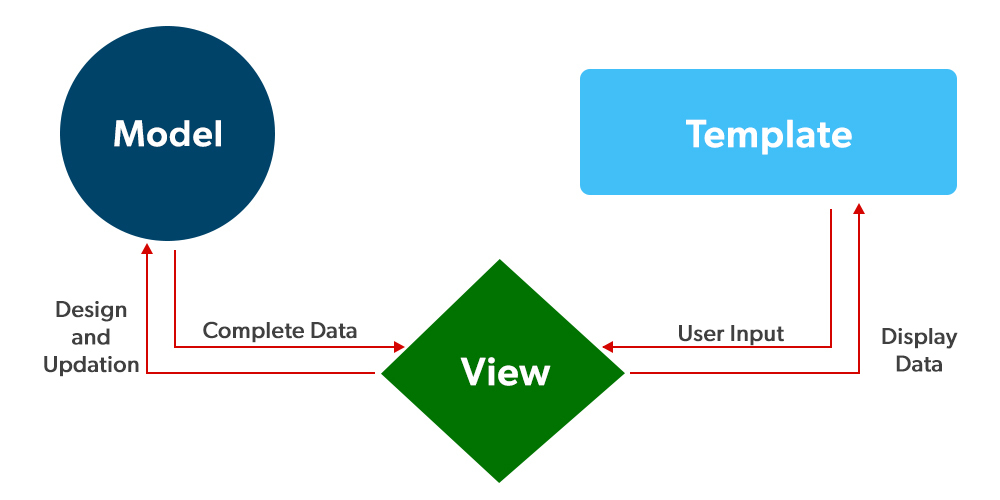
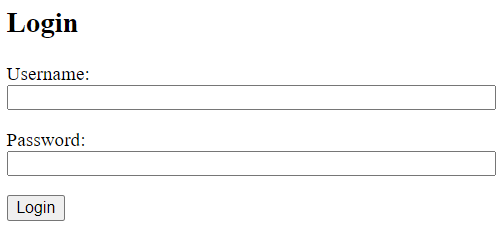
Article link: django get or create.
Learn more about the topic django get or create.
- QuerySet API reference | Django documentation
- Django get_or_create – QueWorx
- How to use “get_or_create()” in Django? – Stack Overflow
- Django get_or_create – Complete guide
- How to use get_or_create in Django? – The TLDR Tech
- Everything to Know About get_or_create() and … – Nsikak Imoh
- Django Tips #6 get_or_create – Simple is Better Than Complex
- Create a Django Get_Or_Create Field – eduCBA
See more: blog https://nhanvietluanvan.com/luat-hoc