Check If Element Exists Cypress
Cypress is a powerful testing framework that allows developers to automate tests for web applications. One common task in Cypress is checking if an element exists on a webpage. In this article, we will explore various methods to accomplish this task and provide useful tips and best practices for efficient element existence checks in Cypress.
Using the `get` command to check if an element exists in Cypress:
The `get` command in Cypress is the primary method to locate and interact with elements on a webpage. It allows you to select elements using CSS selectors. We can use this command to check if an element exists on a webpage by asserting that the length of the returned elements is greater than zero.
“`javascript
cy.get(‘element-selector’).then(($elements) => {
if ($elements.length > 0) {
// Element exists
} else {
// Element does not exist
}
});
“`
By utilizing the `get` command, you can easily check the existence of an element on a webpage.
Using the `should` command to assert element existence in Cypress:
In Cypress, the `should` command plays a crucial role in performing assertions on various elements. To check the existence of an element, we can leverage the `should` command, along with the `exist` keyword.
“`javascript
cy.get(‘element-selector’).should(‘exist’);
“`
This statement will assert that the element exists on the webpage. If it doesn’t, Cypress will automatically handle the assertion failure and log an error.
Using querying commands to check if an element exists in Cypress:
Cypress provides additional querying commands, such as `find`, `contains`, and `filter`, that can be used to enhance element existence checks. These commands allow you to perform more specific searches on a selected element.
“`javascript
cy.get(‘parent-element-selector’).find(‘child-element-selector’).should(‘have.length’, 1);
“`
By using `find` and specifying the parent and child element selectors, you can precisely search for an element’s existence within a specific context.
Checking for the visibility of an element in Cypress:
It is important to differentiate between element existence and visibility checks. While an element may exist in the DOM, it might not be visible on the webpage. Cypress provides the `be.visible` keyword that can be used with the `should` command to assert the visibility of an element.
“`javascript
cy.get(‘element-selector’).should(‘be.visible’);
“`
This statement will verify that the element is both present in the DOM and visible on the webpage.
Verifying the absence of an element in Cypress:
In certain cases, you may want to assert the non-existence of an element. Cypress allows us to use the `should` command with the `not.exist` keyword to verify that an element is not present on a webpage.
“`javascript
cy.get(‘element-selector’).should(‘not.exist’);
“`
By using this statement, the test will pass only if the specified element is not found on the webpage.
Handling dynamic elements in Cypress:
One common challenge in Cypress is dealing with dynamic elements that may appear or disappear based on certain conditions or user interactions. To handle such elements, Cypress provides the `within` and `parent` commands, which allow you to narrow down the scope of your element search.
“`javascript
cy.get(‘parent-element-selector’).within(() => {
cy.get(‘dynamic-element-selector’).should(‘exist’);
});
“`
By using `within` or `parent` command within a specific context, you can effectively check for the existence of dynamic elements.
Best practices for efficient element existence checks in Cypress:
To improve the efficiency and maintainability of your code, consider the following best practices for element existence checks in Cypress:
1. Utilize custom commands and aliases for reusable element checks.
2. Use test data fixtures to improve the identification of elements.
3. Optimize your test scripts to avoid unnecessary checks and improve overall performance.
Extending Cypress with custom plugins for element existence checks:
Cypress provides a powerful plugin system that allows developers to extend its functionality. You can create custom plugins to streamline element existence checks and share them across your Cypress projects.
Creating custom plugins in Cypress is beyond the scope of this article, but it’s worth exploring to boost your testing capabilities.
Common pitfalls and troubleshooting tips for element existence checks:
Even with the best practices in place, there are common pitfalls and issues that can occur when checking element existence. Some possible challenges include intermittent failures, flaky tests, or issues related to the configuration of Cypress itself.
To troubleshoot such problems, make sure to thoroughly analyze error logs, review your test code, and consider adjusting Cypress configuration and options to improve element checking reliability.
FAQs:
1. What is the difference between element existence and visibility checks in Cypress?
Element existence checks ensure that an element is present in the DOM, while visibility checks ensure that the element is both present and visible on the webpage.
2. How can I handle dynamically generated elements in Cypress?
You can use the `within` or `parent` command to narrow down the scope of your element search and effectively handle dynamically generated elements.
3. Can I use custom plugins in Cypress to enhance element existence checks?
Yes, you can create custom plugins to extend the functionality of Cypress and streamline your element existence checks.
4. How can I troubleshoot issues related to element existence checks?
Thoroughly analyze error logs, review your test code, and consider adjusting Cypress configuration and options to improve reliability. Don’t hesitate to seek help from the Cypress community or refer to their documentation.
To conclude, checking if an element exists in Cypress is a crucial task in test automation. This article has explored various methods and techniques for accomplishing this task, along with best practices and troubleshooting tips. By following these guidelines, you can ensure the effectiveness and efficiency of your element existence checks in Cypress.
Test Automation With Cypress #5 Conditional Testing
How To Check Element Value In Cypress?
Cypress is a powerful end-to-end testing framework that simplifies the process of writing and running tests for web applications. When testing web elements, it is crucial to be able to check their values accurately. In this article, we will delve into various techniques to effectively check element values in Cypress, covering common scenarios and providing step-by-step instructions. So, let’s get started!
## Checking Element Value using `should` Command
One of the simplest and most commonly used methods to check an element’s value in Cypress is through the `should` command. This command allows us to assert on various properties and values of an element. Here’s how it works:
“`javascript
cy.get(‘#elementId’).should(‘have.value’, ‘expectedValue’);
“`
In the above code snippet, we use the `get` command to select the element with the specified ID, and then we chain the `should` command to perform the assertion. In this case, we are checking if the element’s value matches the expected value.
Additionally, you can also utilize the `invoke` command in combination with `should` to perform more complex assertions on elements or check specific properties:
“`javascript
cy.get(‘.elementClass’).invoke(‘attr’, ‘propertyToCheck’).should(‘equal’, ‘expectedValue’);
“`
Here, we use the `invoke` command to extract a property value from the element, and then we assert on that value using the `should` command.
## Checking Element Value by Comparing Text Content
In some cases, the value of an element may not be accessible directly through its attributes. Instead, it may be embedded within the element’s text content. To check such values, we can leverage the `contains` command:
“`javascript
cy.contains(‘.elementClass’, ‘expectedTextContent’).should(‘exist’);
“`
In this example, we select the element with the specified class name and check if it contains the expected text content. The `should(‘exist’)` assertion ensures that the element is present on the page.
## Checking Dynamic Element Values
Web applications frequently include dynamic elements that change their values dynamically based on user interactions or external events. To test such elements, we need to capture and check their values at runtime. Cypress provides various techniques to accomplish this.
### Using Cypress Variables
Cypress allows us to assign the values of elements to variables, enabling us to check them dynamically:
“`javascript
let elementValue;
cy.get(‘#elementId’)
.invoke(‘val’)
.then(value => {
elementValue = value;
// Perform any necessary assertions on elementValue
});
“`
In this example, we use the `invoke(‘val’)` command to extract the value of the element and store it in the variable `elementValue`. This allows us to assert on the value and perform further actions as needed.
### Triggering Events and Checking Changes
If an element’s value changes dynamically after some event or action, we can simulate that event using Cypress and then check for the updated value. For example, let’s say we have an input field that updates its value on a button click:
“`javascript
cy.get(‘#inputField’).type(‘new value’);
cy.get(‘#submitButton’).click();
cy.get(‘#inputField’).should(‘have.value’, ‘expectedUpdatedValue’);
“`
In this scenario, we first type a new value into the input field and then click the submit button. Finally, we use the `should` command to verify if the input field’s value has been updated as expected.
## FAQs
**Q: What happens if an element’s value does not match the expected value in Cypress?**
A: If the assertion fails, Cypress will throw an error and your test will fail. You can customize error messages and handle assertions using various Cypress commands and APIs.
**Q: Can I check multiple properties or values of an element in a single test?**
A: Absolutely! Cypress allows you to chain multiple `should` commands together to check various properties or values of an element within a single test.
**Q: Can I check element values in an asynchronous manner using Cypress?**
A: Yes, you can handle asynchronous actions using Cypress’s powerful command queue and usage of promises, async/await, or `.then()` syntax.
**Q: Is it possible to check element values within nested iframes or shadow DOM using Cypress?**
A: Yes, Cypress provides robust support for working with iframes and shadow DOM. You can switch contexts using the `within()`, `iframe()`, or `shadow()` commands to access and check element values within these contexts.
## Conclusion
Checking element values is a fundamental aspect of web application testing, and Cypress offers a comprehensive range of techniques to accomplish this task efficiently. By using the `should` command, comparing text contents, and employing Cypress variables and event simulations, you can accurately check element values in your Cypress tests. Remember to handle both static and dynamic scenarios and make use of the Cypress commands and APIs to customize your assertions and error messages.
How To Check Hidden Element In Cypress?
Cypress is an end-to-end testing framework that allows developers to write reliable and robust tests for their web applications. One common challenge that arises when testing web applications is dealing with hidden elements. Hidden elements refer to elements that are not directly visible on the user interface, potentially obstructing test execution and verification. In this article, we will explore various techniques and approaches to checking hidden elements in Cypress.
Why Check Hidden Elements?
Hidden elements often play a crucial role in web applications, especially for displaying notifications, pop-ups, or asynchronous content loading. Verifying the behavior and correctness of such elements is vital to ensure the overall functionality and user experience of the application. Properly addressing hidden elements in your Cypress tests will help you build more reliable and accurate test suites.
Method 1: Using Cypress .should()
Cypress provides a powerful assertion method called `.should()` that allows us to make assertions on element visibility. By default, `.should()` waits up to 4 seconds for an element to meet the assertion criteria.
To check if an element is hidden, we can use the `.should(‘not.be.visible’)` command. For example:
“`javascript
cy.get(‘.my-element’).should(‘not.be.visible’);
“`
The above command will fail if the element with the class `my-element` is visible.
Method 2: Using CSS Properties
We can also check the visibility status of an element by inspecting its CSS properties using `.get()` and the `.invoke()` command. Here’s how it can be done:
“`javascript
cy.get(‘.my-element’)
.invoke(‘css’, ‘visibility’)
.then((visibility) => {
expect(!!visibility && visibility !== ‘hidden’)
.to.be.false;
});
“`
The above code will pass if the element with the class `my-element` is hidden.
Method 3: Observing Element Attributes
Some web applications utilize specific attributes to control the visibility of elements. You can check the presence or absence of such attributes to determine the visibility status. For instance:
“`javascript
cy.get(‘.my-element’)
.should(‘have.attr’, ‘aria-hidden’, ‘true’);
“`
The above code checks if the element with the class `my-element` has the attribute `aria-hidden` set to `true`.
FAQs:
Q1: How can I check if an element is hidden due to CSS `display: none;` property?
A1: You can use the Cypress `.should()` method with `.have.css` assertion. Here’s an example:
“`javascript
cy.get(‘.my-element’)
.should(‘have.css’, ‘display’, ‘none’);
“`
Q2: What if an element is hidden but still occupies space on the page?
A2: In such cases, you can use a combination of approaches. Firstly, check if the element has a CSS property `visibility` set to `hidden`. If not, you can check if the element has a size of 0px width and height.
“`javascript
cy.get(‘.my-element’)
.invoke(‘css’, ‘visibility’)
.then((visibility) => {
if (!(!!visibility && visibility !== ‘hidden’)) {
cy.get(‘.my-element’)
.should(‘have.css’, ‘width’, ‘0px’)
.and(‘have.css’, ‘height’, ‘0px’);
}
});
“`
Q3: Are there any other ways Cypress can check hidden elements?
A3: Yes, Cypress provides various powerful commands to assert on an element’s attributes, classes, and styles. You can explore Cypress documentation for additional techniques tailored to your specific use cases.
Q4: How can I handle hidden elements that become visible after an action (e.g., click or hover)?
A4: You can use Cypress `.trigger()` command to simulate actions like click or hover and then assert on the visibility status.
“`javascript
cy.get(‘.my-button’).click();
cy.get(‘.my-element’).should(‘be.visible’);
“`
In conclusion, checking hidden elements in Cypress is vital for ensuring the integrity and completeness of your tests. By employing the techniques discussed in this article, you can effectively verify the visibility status of elements and fine-tune your test suite accordingly. Remember to leverage Cypress’s rich command set and consult the documentation to explore more advanced techniques for testing hidden elements in Cypress. Happy testing!
Keywords searched by users: check if element exists cypress Check element exist Cypress, Cypress check element visible, Cypress Chainable, If else Cypress, beforeEach cypress, Timed out retrying after 4000ms cypress, Force: true Cypress, Cypress count elements
Categories: Top 26 Check If Element Exists Cypress
See more here: nhanvietluanvan.com
Check Element Exist Cypress
Introduction
Web application testing is an integral part of the software development lifecycle. Testing frameworks and tools help ensure the quality and reliability of web applications. Cypress, a modern JavaScript-based testing framework, offers a vast array of features to simplify the testing process. One crucial feature is the ability to check whether an element exists on a web page. In this article, we will delve into how to check if an element exists using Cypress, explore different approaches, and answer frequently asked questions.
What is Cypress?
Cypress is a JavaScript-based end-to-end testing framework that enables developers to write tests for web applications. Unlike traditional testing frameworks, Cypress does not rely on Selenium or WebDriver. It operates directly in the browser, allowing for faster and more reliable testing. Cypress offers a wide range of built-in functionalities, including the ability to check if an element exists on a web page.
Checking Element Existence in Cypress
Cypress provides various methods to verify the presence and absence of elements on a web page. Let’s explore the most commonly used ones:
1. cy.get(): This method allows us to locate an element based on its selector. If the element is found, Cypress considers it to exist; otherwise, an error is thrown, halting the test. For example, to check if a button with the class “submit-btn” exists, we would use cy.get(‘.submit-btn’).
2. cy.contains(): If you know the text content of an element, you can use cy.contains() to check its existence. This method takes a selector and the expected text as parameters. It will return the element if found, else throw an error. For instance, cy.contains(‘button’, ‘Submit’) will search for a button element with the text “Submit”.
3. cy.get() with should(): Cypress allows us to chain assertions onto cy.get() calls using the should() method. We can use this feature to check if an element exists by asserting its length to be greater than zero. For instance, cy.get(‘.submit-btn’).should(‘have.length.gt’, 0) ensures that at least one element with the class “submit-btn” exists.
4. cy.get() with find(): The find() method can be used to find child elements within a parent element. By leveraging this method, we can check for the existence of a specific element within another element. For example, cy.get(‘.parent’).find(‘.child’) will search for an element with the class “child” inside an element with the class “parent”.
Frequently Asked Questions
Q1. What happens if the element does not exist?
If the element does not exist and we use the cy.get() method, Cypress will throw an error indicating that it cannot find the element. This error will cause the test to fail. However, if we use cy.get() with should(), the assertion will automatically fail, producing an error message but allowing the test to continue.
Q2. Can we check if an element is not present?
Yes, we can verify if an element is not present by using Cypress’s negation selector – :not. For instance, cy.get(‘.submit-btn:not(.disabled)’) checks whether an element with the class “submit-btn” and without the class “disabled” exists.
Q3. How can we handle asynchronous loading of elements?
Cypress automatically waits for elements to appear on the page. However, in some cases, elements may be loaded dynamically, causing tests to fail if we do not handle the timing properly. Cypress provides utilities like cy.wait() and cy.intercept() that allow us to handle asynchronous loading effectively.
Q4. Are there any additional options to check element existence?
Cypress provides many other methods, such as cy.should(), cy.filter(), and cy.contains(), that can be used to verify element existence based on various conditions. Exploring the Cypress documentation can provide further insight into these options.
Conclusion
Checking element existence is an essential aspect of web application testing. Thanks to Cypress’s powerful features, verifying the presence or absence of elements on web pages is simplified. Through methods like cy.get(), cy.contains(), and chaining assertions, developers can effortlessly ensure that their web applications are functioning as expected. By employing Cypress’s extensive functionalities, testing becomes more efficient and reliable, improving the overall software development process.
Frequently Asked Questions
Q1. What happens if the element does not exist?
Q2. Can we check if an element is not present?
Q3. How can we handle asynchronous loading of elements?
Q4. Are there any additional options to check element existence?
Cypress Check Element Visible
Cypress has revolutionized web testing by providing developers with a robust and versatile framework. One of its most useful features is the ability to check if an element is visible on the page. In this article, we will explore the Cypress check element visible command, its syntax, and how it can be effectively used in web testing.
Understanding Cypress Check Element Visible
Cypress check element visible is a command that allows developers to verify if an element is currently visible on a web page. This is particularly useful when testing the visibility of certain UI components, such as buttons, images, or text. By ensuring that the desired elements are visible, developers can ensure that the web application behaves as expected.
Syntax and Usage
The Cypress check element visible command follows a simple syntax:
cy.get(‘element-selector’).should(‘be.visible’);
Here, ‘element-selector’ can be replaced with any valid CSS selector or Cypress selector that targets the desired element. The should(‘be.visible’) assertion ensures that the element is visible on the page.
It is worth noting that the check element visible command only checks the visibility of an element at a single point in time. If the element becomes hidden or changes its visibility during the test execution, the test will continue running without failing. To perform continuous visibility checks, developers can use Cypress commands like cy.waitUntil() or loop through the assertion until the desired condition is met.
Using Check Element Visible in Web Testing
The Cypress check element visible command can be used in a variety of scenarios during web testing to ensure the visibility of UI elements. Below, we will discuss a few common use cases.
1. Verifying button visibility: Buttons play a crucial role in user interactions. By asserting the visibility of buttons using the check element visible command, developers can guarantee that users can easily identify and interact with them.
2. Checking image visibility: Images can significantly impact the user experience of a web application. By verifying the visibility of images, developers can ensure that the intended visual content is correctly displayed.
3. Ensuring text visibility: The visibility of text elements, such as headers, paragraphs, or labels, is essential for delivering clear and understandable content. The check element visible command allows developers to validate the visibility of such elements.
4. Testing dynamic UI components: Many web applications render UI components dynamically based on user interactions or backend data. With check element visible, developers can ensure that dynamically generated components appear as expected during the test execution.
Frequently Asked Questions (FAQs)
Q1. What is the difference between Cypress check element visible and check element existence?
While Cypress check element visible verifies the visibility of an element on the page, the check element existence command is used to check if an element exists in the DOM, regardless of its visibility. If an element is present but hidden, the check element existence command will still pass.
Q2. Can check element visible be used to validate the visibility of multiple elements?
Yes, the check element visible command can be used to validate the visibility of multiple elements. Developers can use Cypress’s .each() command to iterate through a collection of elements and apply the check element visible command to each element individually.
Q3. What happens if the check element visible command fails during a test?
If the check element visible command fails, the test will fail, and Cypress will display an error message indicating that the element is not visible. This helps developers identify and troubleshoot issues quickly.
Q4. Can check element visible be used to check the visibility of elements within iframes?
Yes, the check element visible command can be used to verify the visibility of elements within iframes. Developers need to select the iframe element first using the .iframe() command and then apply the check element visible command to the desired element within the iframe.
Conclusion
Cypress check element visible is a powerful tool for web testing. By ensuring the visibility of UI elements, developers can validate the behavior and user experience of web applications. Understanding the syntax and usage of this command allows developers to effectively leverage it in their test suites. With its support for CSS selectors, dynamic component testing, and compatibility with iframes, Cypress enables developers to conduct thorough and reliable web testing.
Cypress Chainable
The concept of chainable commands in Cypress is similar to the method chaining pattern in object-oriented programming. It allows developers to perform a series of actions on elements of a web page in a single statement, without the need for additional nesting or complex logic. This leads to more readable and maintainable code, as well as improved test execution speed.
To better understand the concept of Cypress chainable, let’s consider an example. Assume we have a web page that contains a form with two input fields, “username” and “password”, and a submit button. With Cypress, we can write a test to fill in the username and password fields, and click the submit button, all in a single chainable command.
“`javascript
cy.get(‘input[name=”username”]’).type(“john_doe”)
.get(‘input[name=”password”]’).type(“password123″)
.get(‘button[type=”submit”]’).click();
“`
In the above code, we use the `cy.get()` command to locate the required elements on the web page. We then use the `.type()` command to enter the desired values in the input fields, and finally, we use the `.click()` command to simulate a click on the submit button. Note that each command is chained using the dot operator, making the code more readable and expressive.
The power of Cypress chainable lies in its ability to execute commands in sync with the browser’s rendering. Unlike other test automation tools, Cypress automatically waits for each command to finish before moving on to the next one. This ensures that the tests are not affected by any asynchronous behavior, such as network requests or animations.
Another advantage of Cypress chainable is its built-in retry mechanism. If a command fails, Cypress automatically retries it up to four times before throwing an error. This ensures that flaky tests, which may fail intermittently due to timing issues, can still pass reliably. Additionally, Cypress provides a comprehensive set of assertions and expectations to validate the state and behavior of elements on the web page. These assertions can be easily chained with other commands to perform complex validations.
Now that we have explored the concept and benefits of Cypress chainable, let’s address some frequently asked questions about this feature:
Q: Can I chain commands from different selectors or elements?
A: Yes, Cypress allows you to chain commands from different elements. You can use the `.find()` command to locate child elements within a parent element and chain commands accordingly.
Q: Is there a limit to the number of commands that can be chained?
A: No, there is no specific limit to the number of commands that can be chained together. However, it is important to maintain readability and avoid excessive chaining for the sake of simplicity and maintainability.
Q: Can I chain custom commands created using Cypress?
A: Yes, you can chain custom commands created using the `Cypress.Commands.add()` method. Custom commands can be defined in support files and easily invoked and chained in tests.
Q: What happens if a command in the chain fails?
A: If a command fails, Cypress automatically retries it up to four times before throwing an error. This retry mechanism helps in dealing with flaky tests and improves the overall reliability of the test suite.
Q: Can I chain commands with conditional statements or loops?
A: Cypress chainable is not designed to be used with complex conditional statements or loops. It is primarily meant to chain commands in a linear and streamlined manner. However, Cypress does provide other APIs, such as `.then()` and `.each()`, that can be used to perform conditional and iterative operations.
In conclusion, Cypress chainable is a powerful feature that makes writing end-to-end tests for web applications a breeze. It simplifies the test code by allowing developers to chain multiple commands together, leading to more readable and maintainable code. With its synchronization and retry mechanisms, Cypress ensures that tests run reliably and consistently. Use Cypress chainable to supercharge your test automation efforts and deliver high-quality web applications.
Images related to the topic check if element exists cypress
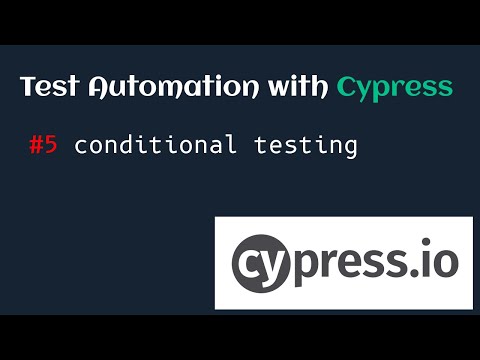
Found 27 images related to check if element exists cypress theme

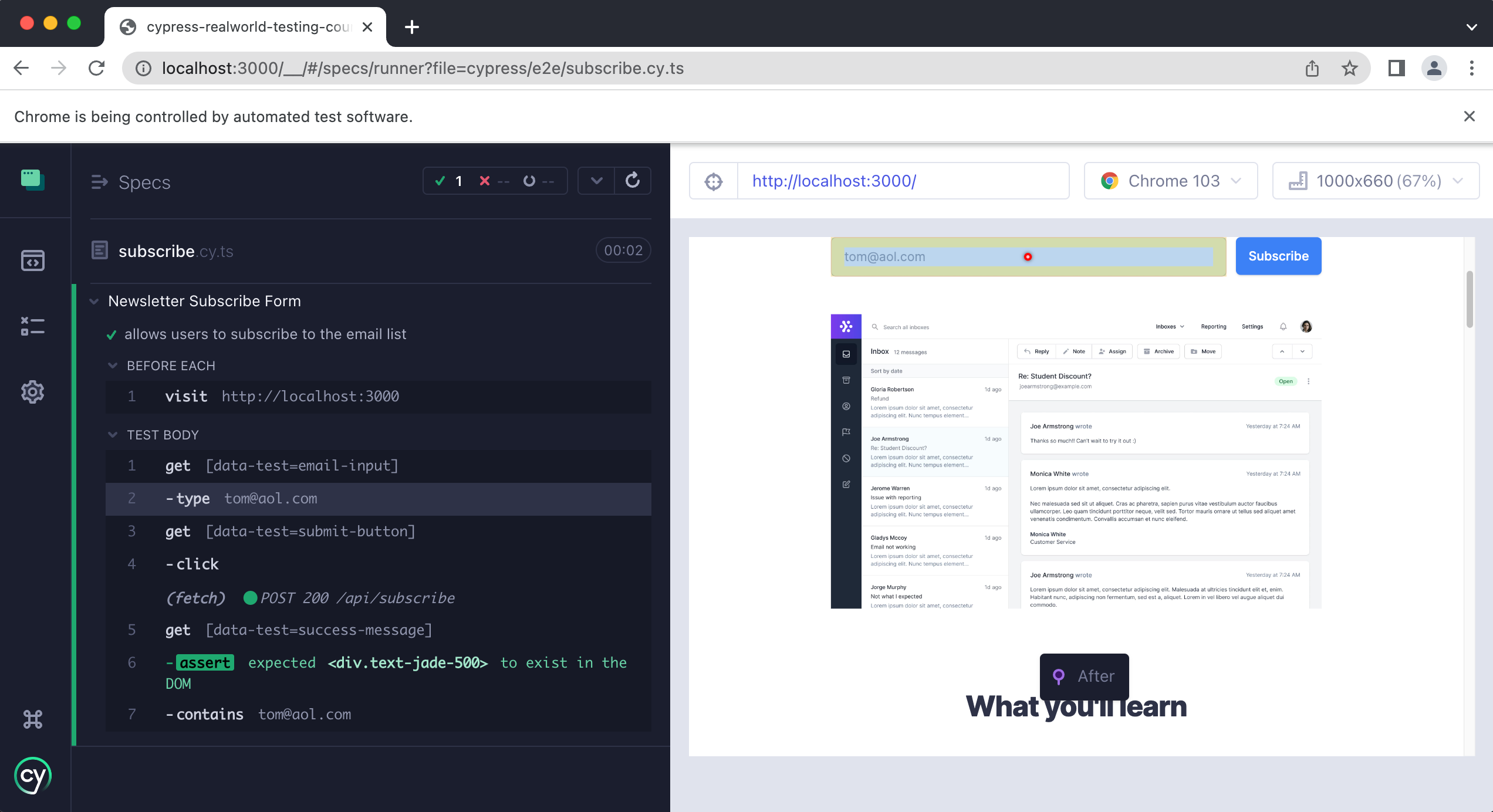
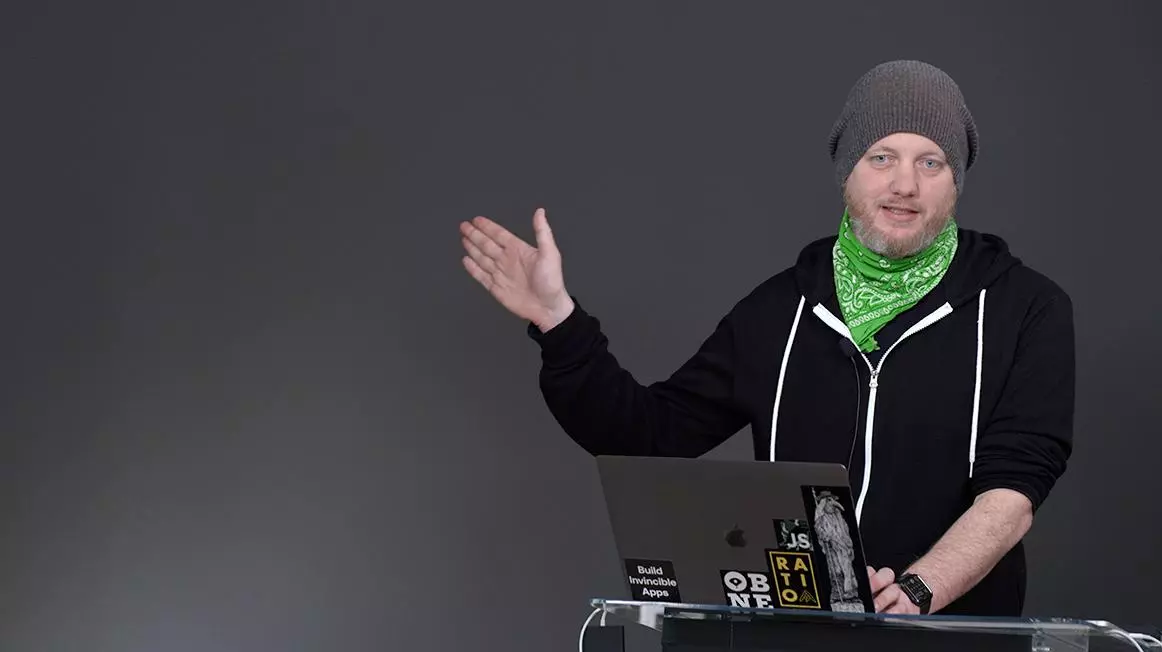

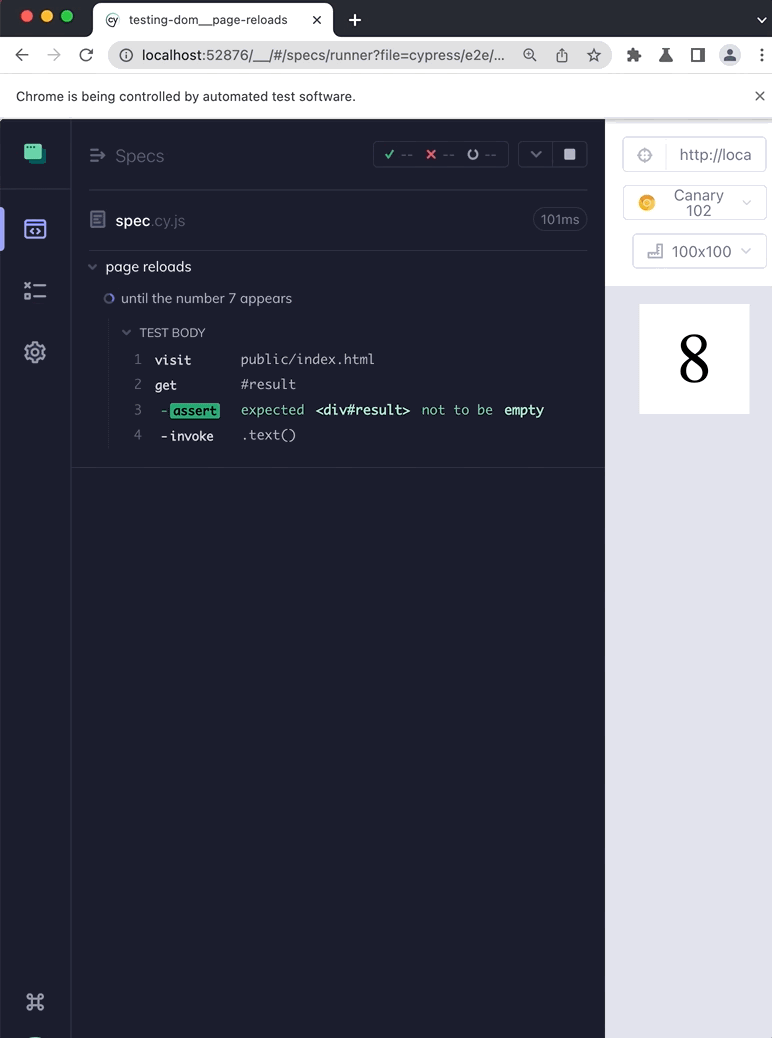

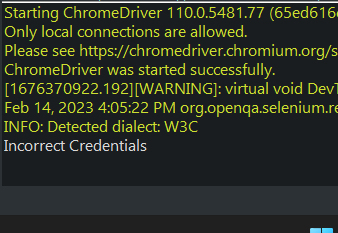

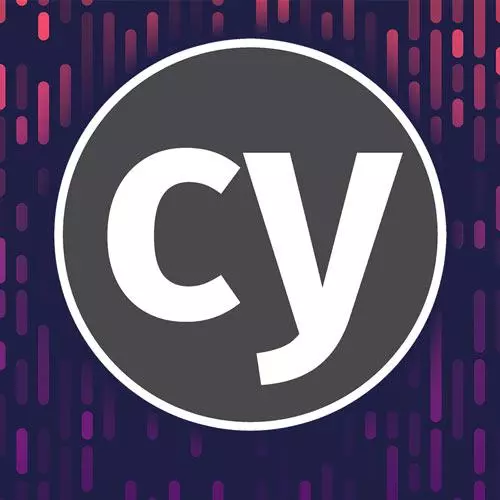
Article link: check if element exists cypress.
Learn more about the topic check if element exists cypress.
- How to check if an Element exists using Cypress?
- How to check if element exists using Cypress.io – Stack Overflow
- How to check if an element exists or not using Cypress.io
- Cypress basics: check if element exists – Filip Hric
- Conditional Testing | Cypress Documentation
- How to Check if Element Exists Without Failing in Cypress
- How to check for Attribute Values in Cypress? – BrowserStack
- Cypress – Hidden Elements – Tutorialspoint
- How to Get Text from List of Elements in Cypress | BrowserStack
- How to run your first Visual Test with Cypress | BrowserStack
- Conditional testing | Cypress examples (v12.14.0)
- cypress check if element exists without failing – You.com
- How to Check if Element Exists Without Failing in Cypress
- cy.exist function to allow existence checking conditional testing
See more: nhanvietluanvan.com/luat-hoc