C# Create A Directory
Introduction:
In C#, creating directories is an essential part of many applications. Whether you need to organize files, store user-specific data, or manage various resources, understanding how to create directories is crucial. In this article, we will delve into the intricacies of creating directories in C#, including the Directory class, DirectoryInfo class, handling exceptions, permissions, and important considerations. So, let’s get started by understanding what a directory is in C#.
What is a directory in C#?
In the context of C#, a directory is synonymous with a folder, which is a location for storing files and other directories. Directories provide a way to organize and manage data efficiently. When you create a directory, you can specify its location, name, and optionally, set permissions.
How to create a directory in C# using the Directory class?
The Directory class in C# provides methods for creating, moving, and enumerating directories. To create a directory using the Directory class, follow these steps:
1. Import the System.IO namespace at the beginning of your code:
“`csharp
using System.IO;
“`
2. Use the CreateDirectory method by passing the desired directory path as an argument:
“`csharp
string path = @”C:\myDirectory”;
Directory.CreateDirectory(path);
“`
This code snippet creates a directory named “myDirectory” in the C: drive.
How to create a directory in C# using the DirectoryInfo class?
The DirectoryInfo class in C# provides an alternative approach for creating directories. It offers additional methods and properties for directory manipulation. Here’s how you can create a directory using the DirectoryInfo class:
1. Import the System.IO namespace at the beginning of your code:
“`csharp
using System.IO;
“`
2. Instantiate the DirectoryInfo class by specifying the desired directory path:
“`csharp
string path = @”C:\myDirectory”;
DirectoryInfo directoryInfo = new DirectoryInfo(path);
“`
3. Use the Create method to create the directory:
“`csharp
directoryInfo.Create();
“`
This code snippet will create a directory named “myDirectory” in the C: drive, similar to using the Directory class.
How to create a directory in C# with specific permissions?
Sometimes, you may need to create directories with specific permissions to restrict or allow certain actions. To create a directory with specific permissions, you can utilize the DirectorySecurity and FileSystemAccessRule classes from the System.Security.AccessControl namespace. Here’s an example of how you can achieve this:
1. Import the required namespaces at the beginning of your code:
“`csharp
using System.IO;
using System.Security.AccessControl;
“`
2. Specify the desired directory path and permissions:
“`csharp
string path = @”C:\myDirectory”;
DirectorySecurity directorySecurity = new DirectorySecurity();
// Example: Grant “Full Control” access to the Users group
directorySecurity.AddAccessRule(new FileSystemAccessRule(“Users”, FileSystemRights.FullControl, InheritanceFlags.ContainerInherit | InheritanceFlags.ObjectInherit, PropagationFlags.None, AccessControlType.Allow));
“`
3. Use the CreateDirectory method while passing the directory path and permissions:
“`csharp
Directory.CreateDirectory(path, directorySecurity);
“`
By providing specific permissions, you can control who can access, modify, or delete the directory.
How to handle exceptions when creating a directory in C#?
When creating directories in C#, it’s essential to handle potential exceptions to avoid unexpected errors. Common exceptions that may occur include UnauthorizedAccessException, IOException, and SecurityException. To handle these exceptions, consider using a try-catch block:
“`csharp
try
{
string path = @”C:\myDirectory”;
Directory.CreateDirectory(path);
}
catch (UnauthorizedAccessException)
{
// Handle unauthorized access exception
}
catch (IOException)
{
// Handle input-output exception
}
catch (SecurityException)
{
// Handle security exception
}
“`
By implementing appropriate exception handling, you can gracefully handle any unexpected events during the directory creation process.
Important considerations when creating a directory in C#:
When creating directories in C#, there are a few key considerations to keep in mind:
1. Permission: Ensure the user executing the code has the necessary permissions to create directories at the specified location.
2. Path: Double-check the path you provide to create the directory, ensuring that it is valid and properly formatted.
3. Exception handling: Implement proper exception handling to gracefully handle potential errors during the creation process.
4. Avoid duplication: Before creating a directory, check if a directory with the same name already exists to prevent unintentional duplication.
FAQs:
Q: Can I create nested directories using the Directory or DirectoryInfo classes?
A: Yes, both classes support creating nested directories by specifying the full path, including any necessary subdirectories.
Q: Is it possible to create a directory with a custom name?
A: Absolutely! You can name your directory as desired by providing the chosen name as an argument while using the appropriate method.
Q: Are there any limitations on directory names or paths?
A: Yes, directory names and paths are subject to specific naming conventions and limitations imposed by the underlying file system. Ensure your names and paths comply with these restrictions to avoid any issues.
Conclusion:
In this comprehensive guide, we explored how to create directories in C# using the Directory and DirectoryInfo classes. We also covered specific permissions, exception handling, and important considerations for a successful directory creation process. By leveraging these techniques, you can efficiently manage your directories within C# applications, allowing for better organization and data management. Remember to implement proper permissions, validate paths, and handle exceptions to ensure smooth execution. Happy coding!
Karik X Only C – Có Chơi Có Chịu (Official Music Video)
Keywords searched by users: c# create a directory
Categories: Top 70 C# Create A Directory
See more here: nhanvietluanvan.com
Images related to the topic c# create a directory
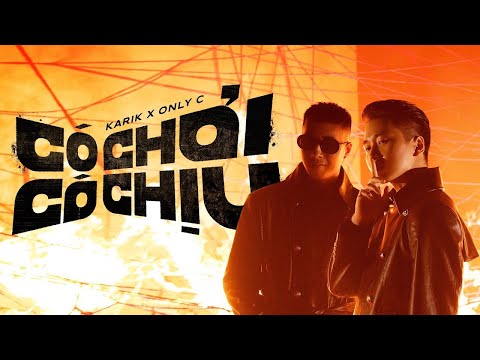
Article link: c# create a directory.
Learn more about the topic c# create a directory.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: nhanvietluanvan.com/luat-hoc