C# Ticks To Seconds
In C#, a tick is the smallest unit of time measurement, equal to 100 nanoseconds or 0.1 microseconds. Ticks are used to represent a point in time or a duration of time in the .NET framework. The concept of ticks is crucial when dealing with time-related calculations and comparisons, as it provides a precise and consistent way to handle time intervals.
Converting Ticks to Seconds
To convert ticks to seconds in C#, you can make use of the TimeSpan.Ticks property or the DateTime.Ticks property, both of which provide the necessary functionality for converting ticks to a more human-readable format.
The DateTime.Ticks Property
The DateTime structure in C# provides a property called Ticks, which represents the number of ticks that have elapsed since 12:00:00 midnight on January 1, 0001. This property returns a long value, indicating the number of ticks. To convert ticks to seconds using the DateTime.Ticks property, you can divide the number of ticks by 10,000,000.
Here’s an example of how you can convert ticks to seconds using the DateTime.Ticks property:
“`csharp
long ticks = DateTime.Now.Ticks;
double seconds = ticks / 10_000_000.0;
“`
In the above code, we first retrieve the current number of ticks using DateTime.Now.Ticks. Then, we divide the number of ticks by 10,000,000 to get the equivalent number of seconds.
The TimeSpan.Ticks Property
The TimeSpan structure in C# represents a time interval. It also provides a property called Ticks, which returns the number of ticks in a TimeSpan object. Similar to the DateTime.Ticks property, ticks can be converted to seconds by dividing the number of ticks by 10,000,000.
Here’s an example of converting ticks to seconds using the TimeSpan.Ticks property:
“`csharp
TimeSpan duration = TimeSpan.FromTicks(123456789);
double seconds = duration.Ticks / 10_000_000.0;
“`
In the above code, we create a TimeSpan object with a given number of ticks (123456789). Then, we divide the number of ticks by 10,000,000 to obtain the equivalent number of seconds.
System.Diagnostics.Stopwatch
Another way to convert ticks to seconds is by using the Stopwatch class from the System.Diagnostics namespace. Stopwatch provides high-resolution time measurements with its Elapsed property, which returns the duration measured by the Stopwatch instance.
Here’s an example of using the Stopwatch class to convert ticks to seconds:
“`csharp
Stopwatch stopwatch = Stopwatch.StartNew();
// Perform some calculations or time-consuming operations
stopwatch.Stop();
double seconds = stopwatch.ElapsedTicks / (double)Stopwatch.Frequency;
“`
In the above code, we first start the Stopwatch using Stopwatch.StartNew(). Then, we perform the required calculations or time-consuming operations. Afterward, we stop the Stopwatch using stopwatch.Stop(). Finally, we divide the ElapsedTicks property by Stopwatch.Frequency to obtain the duration in seconds.
Performance Considerations
When performing conversions from ticks to seconds in C#, it’s crucial to consider performance implications, especially when dealing with large datasets or frequent conversions. Division operations can be relatively expensive in terms of computational resources.
If you need to perform frequent tick to second conversions, it’s recommended to store the divisor (10,000,000) as a constant rather than calculating it repeatedly. This can help improve the performance of your application, especially if the conversion is performed in a loop or within critical sections of code.
Summary of Ticks to Seconds Conversion in C#
In summary, ticks are the smallest unit of time measurement in C#, equal to 100 nanoseconds or 0.1 microseconds. To convert ticks to seconds, you can make use of the DateTime.Ticks property, TimeSpan.Ticks property, or the Stopwatch class from the System.Diagnostics namespace. Remember to consider performance implications when performing frequent conversions and use constants for the divisor to optimize performance.
FAQs
Q: Can ticks be negative in C#?
A: Yes, ticks can be negative. In C#, positive ticks represent time intervals after January 1, 0001, while negative ticks represent time intervals before that date.
Q: Can I convert seconds to ticks in C#?
A: Yes, the TimeSpan.FromTicks method can be used to convert seconds to ticks. For example, you can convert 10 seconds to ticks like this: `TimeSpan.TicksPerSecond * 10`.
Q: What is the maximum value of ticks in C#?
A: The maximum value of ticks in C# is `long.MaxValue`, which represents approximately 29,227 years.
Q: Are ticks and milliseconds the same in C#?
A: No, ticks and milliseconds are not the same in C#. Ticks represent a much smaller unit of time measurement, while milliseconds represent 1/1000th of a second. To convert between ticks and milliseconds, you can use the TimeSpan class.
Q: How accurate are ticks in C#?
A: Ticks provide a high level of accuracy in C#. They represent 100 nanoseconds or 0.1 microseconds, allowing for precise time measurements and calculations.
Karik X Only C – Có Chơi Có Chịu (Official Music Video)
Keywords searched by users: c# ticks to seconds
Categories: Top 52 C# Ticks To Seconds
See more here: nhanvietluanvan.com
Images related to the topic c# ticks to seconds
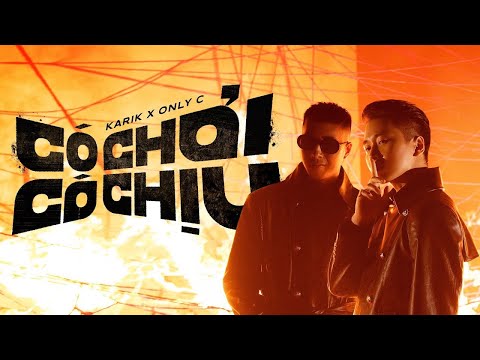
Article link: c# ticks to seconds.
Learn more about the topic c# ticks to seconds.