Specified Cast Not Valid
In programming languages, a cast is used to explicitly convert a value from one data type to another. A specified cast refers to a situation where the programmer explicitly specifies the type of cast to be performed. This can be done using explicit conversion operators, casting functions, or type casting keywords provided by the programming language.
A specified cast is used when there is a need to convert a value to a specific data type, bypassing any implicit type conversion that may occur by default. It allows the programmer to have more control over the type conversion process, ensuring that the value is treated as the desired data type.
Reasons Why a Specified Cast May Not Be Valid
There are several reasons why a specified cast may not be valid in a program. Here are some common scenarios:
1. Incompatible Data Types: If the data types involved in the cast are not compatible, the specified cast will fail. For example, trying to cast a string to an integer or vice versa would result in an invalid specified cast.
2. Null Values: If the value being cast is null, it can cause a specified cast to fail. Null values cannot be converted to any specific data type as they do not have a defined value.
3. Precision Loss: When casting a value from a data type with higher precision to a data type with lower precision, there may be a loss of precision. If the programmer does not handle this properly, it can result in an invalid specified cast.
4. Invalid Values: If the value being cast is outside the acceptable range for the target data type, the specified cast will fail. For example, trying to cast a negative number to an unsigned integer would result in an invalid specified cast.
Error Messages Associated with Invalid Specified Casts
When a specified cast is not valid, programming languages typically throw an exception with an error message indicating the reason for the failure. Some common error messages associated with invalid specified casts include:
1. “Specified cast is not valid”
2. “InvalidCastException: Specified cast is not valid”
These error messages indicate that the specified cast operation has failed due to one of the reasons mentioned earlier, such as incompatible data types or null values.
Handling Invalid Specified Casts in Programming Languages
When encountering an invalid specified cast, it is essential to handle the exception appropriately to prevent the program from crashing or producing unexpected results. Most programming languages provide mechanisms for exception handling to gracefully handle such errors.
In languages like C# or Java, exception handling can be done using try-catch blocks. The code that involves the specified cast is placed within the try block, and any exceptions that occur are caught and handled in the catch block. This allows the program to continue running smoothly, even if a specified cast fails.
Here is an example of handling an InvalidCastException in C#:
“`csharp
try
{
int value = Convert.ToInt32(“abc”);
}
catch (InvalidCastException e)
{
Console.WriteLine(“Invalid cast: ” + e.Message);
}
“`
Best Practices to Avoid or Resolve Invalid Specified Casts
To avoid or resolve invalid specified casts, it is crucial to follow certain best practices while writing code. Here are some recommendations:
1. Use Appropriate Conversion Functions: Make use of programming language-specific conversion functions or methods to handle type conversions whenever possible. These functions handle any necessary checks and conversions, reducing the chances of an invalid specified cast.
2. Check for Null Values: Before performing a specified cast, check if the value being cast is null. Handling null values appropriately can prevent invalid specified casts.
3. Validate Input Data: Ensure that input data meets the required criteria for the specified cast. Validate the input before attempting the cast, and handle any invalid input scenarios gracefully.
4. Use Type-Safe Techniques: Whenever possible, prefer type-safe techniques and language constructs that minimize the need for explicit casts. This helps to avoid potential pitfalls associated with invalid specified casts.
FAQs
Q: What does the error message “Specified cast is not valid” mean?
A: The error message “Specified cast is not valid” indicates that a specified cast operation has failed due to incompatible data types, null values, or other reasons mentioned earlier.
Q: How can I fix a “Specified cast is not valid” error in SQL?
A: To fix a “Specified cast is not valid” error in SQL, ensure that the data being cast is of a compatible data type or use appropriate conversion functions to handle the cast.
Q: What does the error message “InvalidCastException: Specified cast is not valid” mean?
A: The error message “InvalidCastException: Specified cast is not valid” is similar to the previous error message and indicates a failed specified cast operation.
Q: How can I handle a “Specified cast is not valid” error in C#?
A: In C#, you can handle a “Specified cast is not valid” error using try-catch blocks. Place the code involving the specified cast within the try block and handle the exception in the catch block.
Q: What are some best practices to avoid invalid specified casts?
A: Some best practices to avoid invalid specified casts include using appropriate conversion functions, checking for null values, validating input data, and using type-safe techniques whenever possible.
In conclusion, understanding the concept of a specified cast is crucial for effective programming. By being aware of the reasons behind invalid specified casts and implementing best practices, programmers can handle and prevent such errors effectively. It is important to handle exceptions gracefully and validate input data to avoid unexpected errors and ensure the smooth functioning of programs.
Specified Cast Is Not Valid C#| Windows Form Application| Desktop Application
Keywords searched by users: specified cast not valid Lỗi Specified cast is not valid trong SQL, Specified cast is not valid C#, System InvalidCastException Specified cast is not valid, System invalidcastexception specified cast is not valid sql, Specified cast is not valid unity, Specified cast is not valid SqlManagerUI, Specified cast is not valid xamarin forms, Specified cast is not valid là gì
Categories: Top 12 Specified Cast Not Valid
See more here: nhanvietluanvan.com
Lỗi Specified Cast Is Not Valid Trong Sql
Causes of the “Specified cast is not valid” error:
1. Data type mismatch: One of the most common causes of this error is a data type mismatch between the code and the database column. For example, if you are trying to cast a string value to an integer, but the actual value stored in the database is not a valid integer, this error will occur.
2. Null values: Another possible cause of this error is when you are trying to convert a null value to a specific data type that does not support null values. For instance, if you try to cast a null value to an integer, you will encounter this error.
3. Incorrect syntax: Sometimes, this error can occur due to incorrect syntax in your SQL query. If the query is not written correctly, it can lead to a mismatch between the expected and actual data types.
4. Implicit and explicit conversion: In SQL, there are two types of data type conversions. Implicit conversion happens automatically when the database engine can convert one data type to another without any loss of data. However, explicit conversion requires a CAST or CONVERT function to convert one data type to another explicitly. If you are using explicit conversion and the actual data type is not compatible with the expected data type, you will encounter this error.
Solutions to resolve the “Specified cast is not valid” error:
1. Check data types: To resolve this error, it is important to check the data types of both the code and the database column. Make sure they are compatible and match each other. Use appropriate data types to avoid any mismatch.
2. Handle null values: If you are dealing with null values, ensure that the destination data type supports null values. You can use the ISNULL function to replace null values with a default value or handle them separately in your code.
3. Review SQL syntax: Double-check your SQL syntax to ensure there are no syntax errors. Check for any missing or incorrect syntax that might lead to a data type mismatch.
4. Verify conversion requirements: When performing explicit conversions, ensure that the conversion is valid and supported by the data types involved. Use appropriate CAST or CONVERT functions to perform conversions and avoid any unnecessary errors.
5. Debug your code: Debugging can be an effective way to identify the exact cause of the error. Use breakpoints and step through your code to find out where the error occurs. Examine the values at each step to identify any inconsistencies.
FAQs:
Q: Can this error occur in other programming languages or only in SQL?
A: This error can occur in any programming language that interacts with a SQL database. The root cause of the error is a mismatch between the expected and actual data types, which can happen across different programming languages.
Q: How can I identify the exact data type causing the error?
A: You can use debugging techniques to identify the exact data type causing the error. By examining the values at each step of your code, you can narrow down the problematic data type.
Q: Is there a way to handle this error gracefully?
A: Yes, you can handle this error gracefully by implementing error handling mechanisms in your code. Use try-catch blocks to catch the exception and display a meaningful error message to the user.
Q: Why does this error occur even when the data types seem to match?
A: This error can occur even when the data types seem to match due to various reasons like null values, incorrect syntax, or unsupported conversions. It is important to thoroughly review your code and diagnose the exact cause of the error.
In conclusion, the “Specified cast is not valid” error in SQL occurs when there is a mismatch between the expected and actual data types. By understanding the causes and implementing the suggested solutions, you can effectively resolve this error and ensure the smooth functioning of your SQL database operations. Remember to carefully review your code, handle null values appropriately, and verify your data type conversions to avoid encountering this error in the future.
Specified Cast Is Not Valid C#
Introduction:
C# is a powerful programming language used for developing a wide range of applications, including desktop, web, and mobile. However, like any programming language, it comes with its fair share of errors and exceptions. One common error that developers encounter is the “Specified cast is not valid” exception. In this article, we will delve into this exception, explaining its causes, troubleshooting methods, and frequently asked questions to help you overcome this issue effectively.
Understanding the “Specified cast is not valid” exception:
The “Specified cast is not valid” exception occurs when an attempt is made to convert an object or value from one type to another that is incompatible. This exception is thrown by the .NET framework when an invalid cast operation is encountered.
Causes of the exception:
1. Incompatible data types:
One of the primary causes of this exception is trying to cast one data type to another that is not compatible. For example, trying to cast a string to an integer or an object of one class to another incompatible class.
2. Null values:
Another reason for this exception is when attempting to cast a null reference to a value type. Since value types cannot be null, this exception is raised.
3. Type mismatch or mismatched versions:
Sometimes, this exception can occur due to a mismatch between the expected type and the actual type of the object. For instance, using an older version of a library that expects a different type can result in this exception.
Troubleshooting the exception:
1. Verify data types:
The first step in troubleshooting this exception is to review your code and ensure that the casting being performed is valid. Check the data types of the objects involved in the cast operation and verify their compatibility.
2. Check for null references:
If you are encountering the exception while casting a reference type, verify if the object being cast is null. In such cases, consider implementing null checks to handle the scenario gracefully.
3. Review library versions:
When using third-party libraries or APIs, make sure you are using the correct version. Mismatched versions can lead to type mismatch errors, triggering the “Specified cast is not valid” exception.
4. Use safe casting operators:
Instead of using the traditional casting operators (explicit or implicit), consider using safe casting operators like ‘as’. The ‘as’ operator performs the cast but returns ‘null’ if the cast is not successful, avoiding the exception altogether.
5. Debugging and logging:
Implement good debugging practices, including stepping through your code and logging relevant information. This will help you pinpoint the exact location where the exception occurs and gather useful information for troubleshooting.
Commonly asked questions about the “Specified cast is not valid” exception:
Q1. Can this exception occur even if the cast is valid?
A1. No, the “Specified cast is not valid” exception will only occur if the cast being performed is invalid. Double-check your data types and ensure compatibility to avoid this exception.
Q2. How can I handle the exception gracefully?
A2. You can handle the exception by catching it using a try-catch block. Inside the catch block, you can perform appropriate error handling, such as displaying meaningful error messages to the user or logging the exception for further investigation.
Q3. Why am I encountering this exception when casting between two similar classes?
A3. Even though two classes might seem similar, if they are from different namespaces or assemblies, they are considered distinct types. Ensure that the two classes are identical or derive from the same base class before attempting casting.
Q4. What can I do if a library I am using expects a different type than the one I have?
A4. In such cases, consider using type conversion methods or utility classes provided by the library to convert the types appropriately. If possible, update your application to use the correct version of the library.
Q5. Can the “Specified cast is not valid” exception occur during database operations?
A5. Yes, this exception can occur when working with databases. Ensure that the data types defined in the database table match those being used in your code to avoid casting errors.
Conclusion:
The “Specified cast is not valid” exception can be frustrating for developers, but understanding its causes and implementing appropriate troubleshooting methods can help resolve the issue effectively. Always double-check your casting operations, handle null references, and verify compatibility between types to avoid this exception. Keep in mind the tips mentioned in this article and use debugging techniques to diagnose the problem accurately. By following these best practices, you can overcome this exception and ensure the smooth execution of your C# applications.
System Invalidcastexception Specified Cast Is Not Valid
Understanding the InvalidCastException:
The InvalidCastException is an exception derived from the SystemException class in the .NET Framework. It signals that a cast operation failed during runtime because the target object cannot be converted to the specified type. This error occurs when an implicit or explicit type conversion is attempted between two data types that are not compatible.
Causes of the InvalidCastException:
1. Incompatible data types: The most common cause of the InvalidCastException is an attempt to cast an object to a data type that is not compatible with the target data type. For example, attempting to cast a string to an integer will result in an InvalidCastException if the string does not contain a valid integer value.
2. Incorrect use of APIs: Another cause of this error is when APIs are used incorrectly. If a method or property returns a different data type than expected, attempting to cast it to the expected type will result in an InvalidCastException.
3. Type mismatch in collections: This error can also occur when working with collections, especially if the types of objects stored in the collection are not consistent. For example, if a collection is declared to hold integers, but it contains objects of different types, attempting to access an object and cast it to an integer will throw an InvalidCastException.
Common Scenarios Where the InvalidCastException Occurs:
1. Database operations: When retrieving data from a database using ADO.NET, it is common to encounter the InvalidCastException. This is often caused by incorrect mappings between the database types and the desired data types in the code.
2. Reflection: In certain scenarios where reflection is used, such as dynamically loading assemblies or accessing properties at runtime, the InvalidCastException may occur if the types do not match as expected.
3. Serialization and deserialization: When serializing and deserializing objects, it is critical to ensure that the target and source data types are compatible. Failure to do so can result in an InvalidCastException.
Handling the InvalidCastException:
1. Check data types: The first step in handling this error is to verify that the data types being used are indeed compatible. Double-check if the objects being casted are of the correct type. Using the “as” keyword in C# can be helpful to perform safe conversions, as it returns null if the conversion fails instead of throwing an exception.
2. Use error handling mechanisms: Wrap potential cast operations with try-catch blocks to catch the InvalidCastException and handle it gracefully. This allows the program to proceed with an appropriate fallback mechanism instead of crashing abruptly.
3. Verify APIs and return types: When this error occurs due to API misuse, ensure that the method or property being called returns the expected data type. Reading the documentation and verifying how the API should be used is crucial to avoid InvalidCastException scenarios.
FAQs about the InvalidCastException:
Q: Can this error be avoided altogether?
A: While it is not always possible to avoid the InvalidCastException entirely, adhering to good programming practices, such as strong typing and proper use of APIs, can significantly reduce the occurrence of this error.
Q: How can I know if a cast operation will throw an InvalidCastException?
A: One way to mitigate this error is to use the “is” keyword in C#. This keyword allows you to check if a cast operation will succeed before attempting it. For example, you can use an if statement to verify if an object is of the desired type before casting it.
Q: Are there any tools or techniques to help identify such type casting errors?
A: Yes, there are various code analysis tools available that can detect potential type casting issues at compile-time. These tools can warn you about potential InvalidCastException scenarios, allowing you to address them proactively.
Q: How does the InvalidCastException differ from other type-related exceptions?
A: While the InvalidCastException is specifically thrown when a cast operation fails due to type incompatibility, other type-related exceptions, such as FormatException or ArgumentException, are thrown when type conversion fails due to other reasons, such as incorrect format or invalid argument values.
Conclusion:
The System.InvalidCastException: Specified cast is not valid error is a common hurdle that programmers face while developing software applications. Understanding the causes, being aware of the scenarios where it might occur, and implementing proper error handling techniques can help mitigate this error. By following best practices and using appropriate error handling mechanisms, software developers can effectively manage and resolve the InvalidCastException.
Images related to the topic specified cast not valid
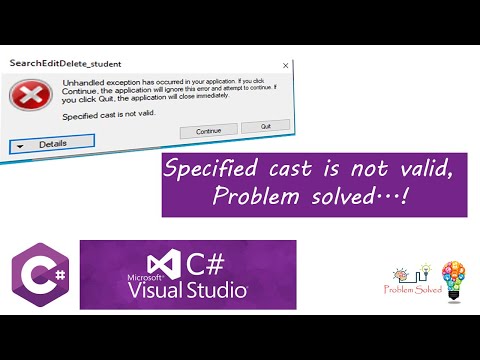
Found 19 images related to specified cast not valid theme


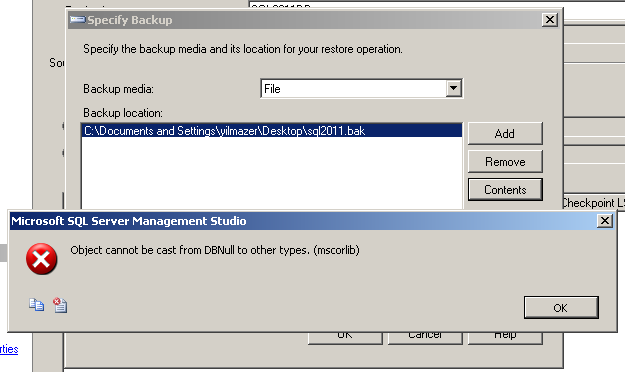
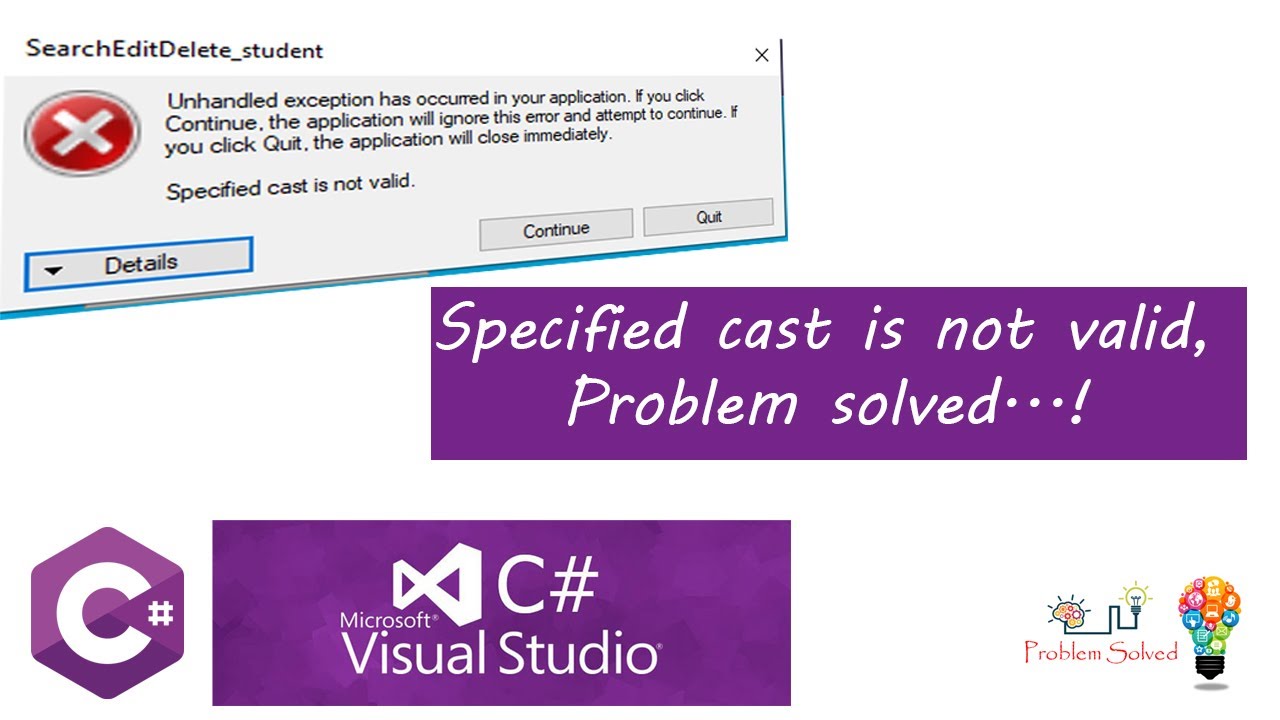






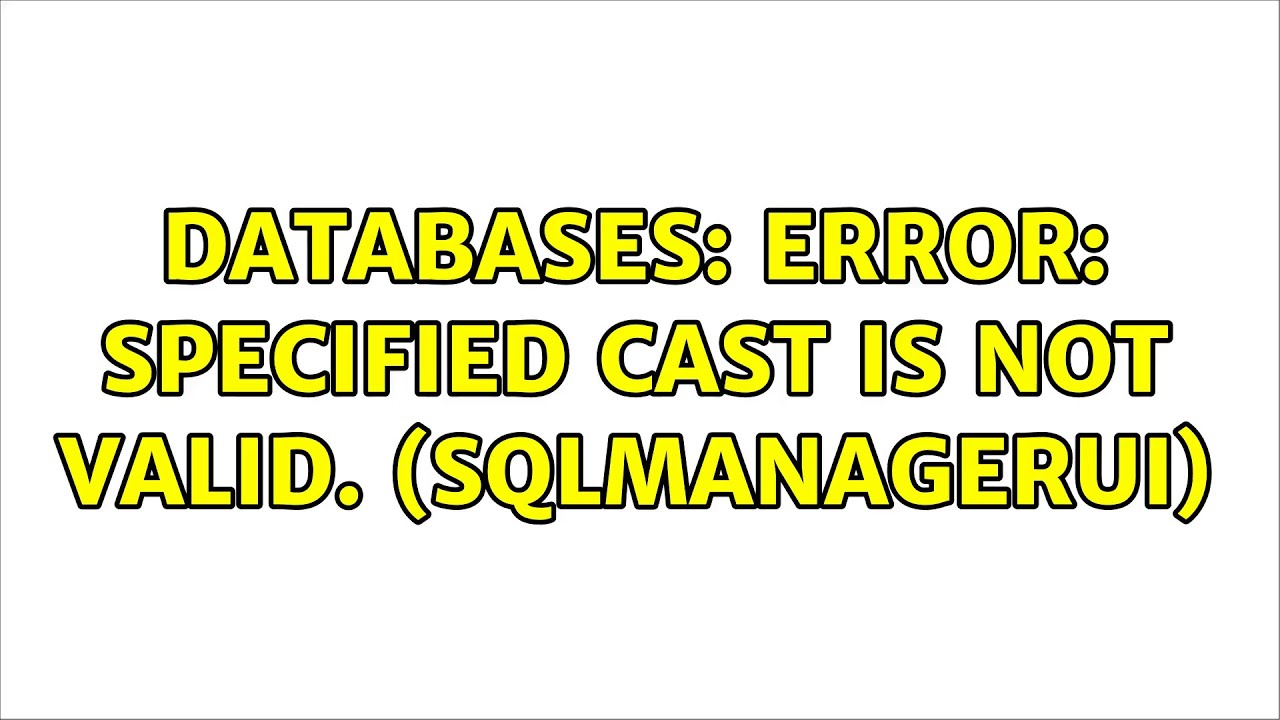



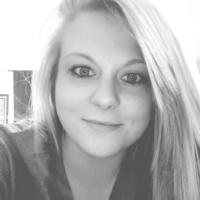




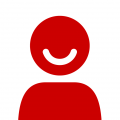
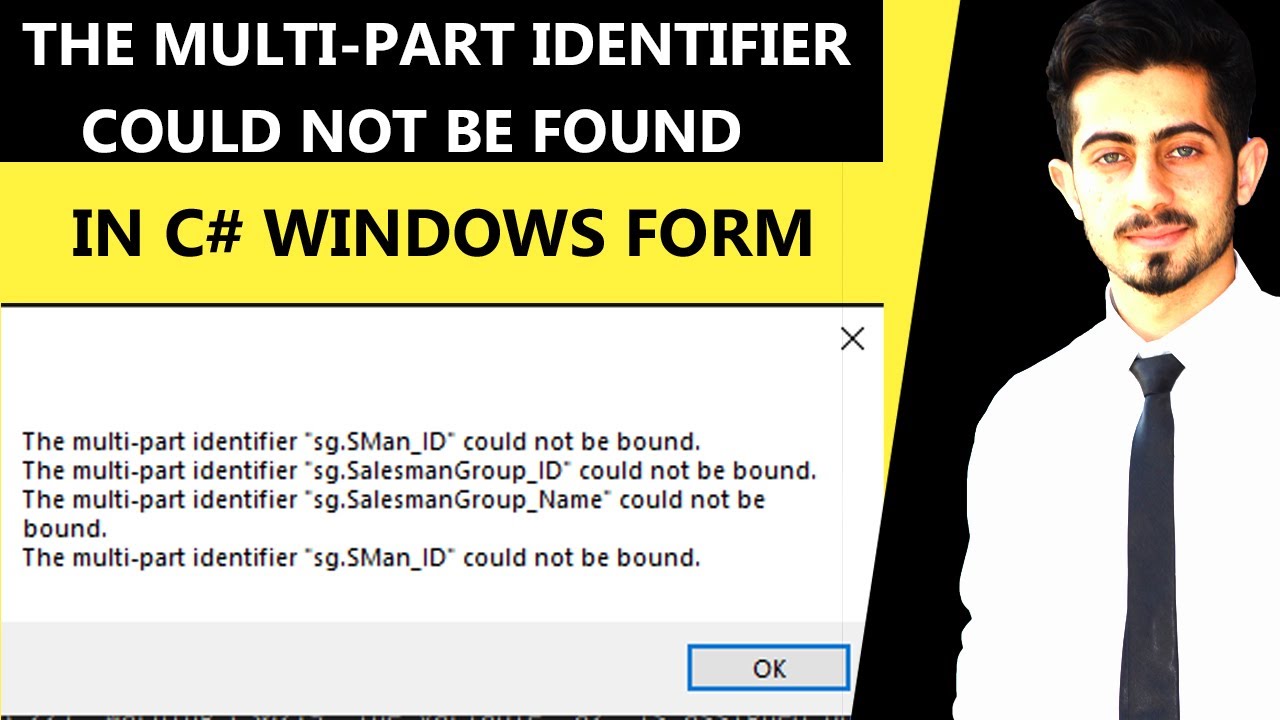
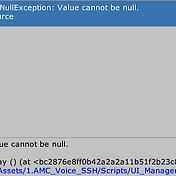

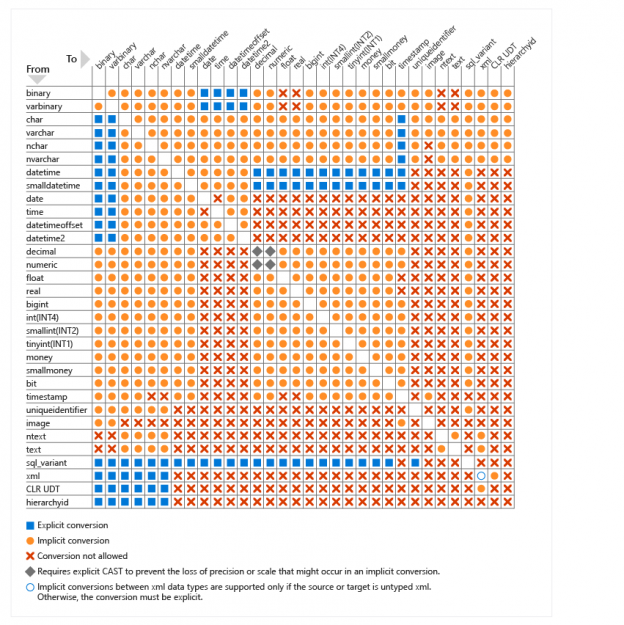
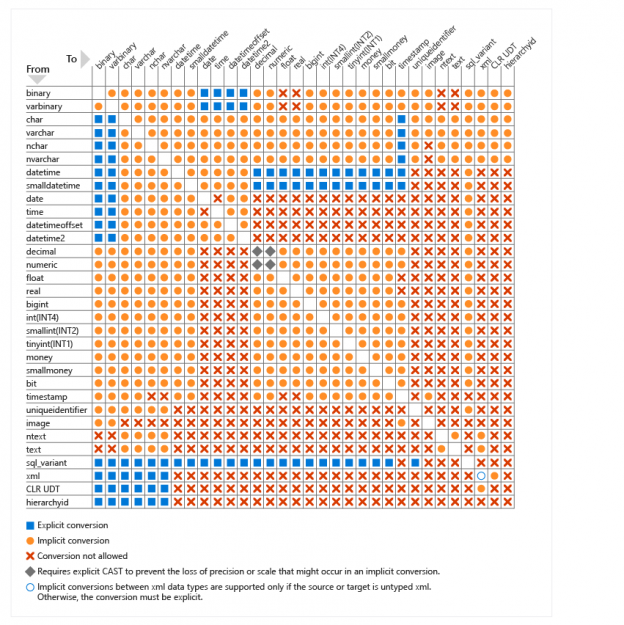
Article link: specified cast not valid.
Learn more about the topic specified cast not valid.
- SQL Server database restore error: specified cast is not valid …
- Specified Cast Is Not Valid – Understanding the Error
- Specified cast is not valid. (SqlManagerUI) – Kodyaz.com
- SQL SERVER – Restore Error: Specified cast is not valid …
- The Specified Cast Is Not Valid. (sqlmanagerui) – SPGeeks
- [Solved] Specified cast is not valid C# – CodeProject
- “specified cast is not valid” when trying to restore SQL Database
- System.InvalidCastException: Specified Cast is not valid …
- Specified cast is not valid. While using For Each – Studio
See more: blog https://nhanvietluanvan.com/luat-hoc