Runtimeerror: Dictionary Changed Size During Iteration
Introduction:
When working with dictionaries in Python, it is essential to understand the concept of iteration and the potential issues that can arise when the size of a dictionary is modified during the iteration process. One common error that can occur is the “RuntimeError: dictionary changed size during iteration”. In this article, we will explore the causes of this error, along with possible solutions and best practices for working with dictionaries.
Causes of the “RuntimeError: dictionary changed size during iteration”:
1. Adding or Removing Elements in a Dictionary During Iteration:
One of the primary causes of the “RuntimeError: dictionary changed size during iteration” error is modifying the size of the dictionary while iterating over it. If a new element is added to the dictionary or an element is removed during the iteration, it can lead to an inconsistent state and cause the error to occur.
2. Using List Comprehension or Generator Expressions During Iteration:
List comprehension and generator expressions provide a concise way to iterate over a dictionary. However, if these expressions are used to create a new dictionary or modify the existing one during iteration, the size of the dictionary can change, resulting in the runtime error.
Handling the “RuntimeError: dictionary changed size during iteration”:
1. Use a Copy of the Dictionary for Iteration:
To avoid modifying the dictionary while iterating over it, create a copy of the dictionary and iterate over the copy instead. This way, any changes made to the original dictionary won’t affect the iteration process.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
# Creating a copy of the dictionary
copy_dict = my_dict.copy()
# Iterating over the copy
for key, value in copy_dict.items():
# Perform desired operations
print(key, value)
“`
2. Utilize the items() Method for Iteration:
The items() method of a dictionary returns a view object containing key-value pairs. Iterating over this view object ensures that any modifications made to the dictionary won’t interfere with the iteration process.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
# Iterating over the items view object
for key, value in my_dict.items():
# Perform desired operations
print(key, value)
“`
3. Apply a Try-Except Block to Handle the Error:
If modifying the dictionary during iteration is unavoidable, you can handle the “RuntimeError: dictionary changed size during iteration” error by using a try-except block. This allows you to catch the error and implement appropriate error handling procedures.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
# Modifying the dictionary during iteration
for key, value in my_dict.items():
del my_dict[key]
try:
# Perform desired operations
print(key, value)
except RuntimeError as e:
print(“Error:”, str(e))
“`
Common Mistakes and Pitfalls to Avoid:
1. Not understanding the implications of changing dictionary size during iteration: Modifying a dictionary while iterating can result in unpredictable behavior and errors. It is crucial to be aware of the consequences and take appropriate precautions.
2. Neglecting to use appropriate methods for iteration: Using the wrong methods, such as keys() or values(), instead of items() for iteration, can lead to the “RuntimeError: dictionary changed size during iteration”. Make sure to use the correct method to iterate over the dictionary.
3. Overlooking the importance of handling the error: Ignoring or not properly handling the “RuntimeError: dictionary changed size during iteration” error can lead to program crashes or incorrect results. It’s essential to implement error handling mechanisms to prevent and manage this error effectively.
Best Practices for Working with Dictionaries:
1. Think ahead and consider potential changes to the dictionary: Before iterating over a dictionary, evaluate if there is a possibility of modifying the dictionary size. If so, implement appropriate precautionary measures to avoid the runtime error.
2. Plan the iteration process accordingly: Design your code and logic in a way that accommodates any necessary modifications to the dictionary during iteration. This can include utilizing the recommended methods or using a separate copy of the dictionary.
3. Test and iterate in controlled environments: To minimize the risk of errors, test your code and iterate over dictionaries in controlled environments. This allows you to identify and resolve any issues before deploying the code in production.
Other Related Errors and Their Solutions:
1. Delete key in dictionary Python while iterating:
To delete a key from a dictionary while iterating, make a copy of the dictionary and then iterate over the copy. In the iteration process, use the del keyword to delete the desired key from the original dictionary.
2. Python delete dictionary item while iterating:
Similar to deleting a key, make a copy of the dictionary and iterate over the copy. Inside the iteration loop, use the del keyword to delete the desired item from the original dictionary.
3. Change value in dictionary Python loop:
To change the value of a dictionary item during iteration, access the key-value pair using the key. Assign the new value to the corresponding key to update the dictionary item.
4. Update keys in dictionary Python:
To update the keys in a dictionary during iteration, make a copy of the dictionary and iterate over it. Use the del keyword to delete the respective key-value pair and add a new key-value pair to the dictionary.
5. How to check a key in dictionary Python:
To check if a key exists in a dictionary, use the in keyword followed by the name of the dictionary and the key you want to check. This expression will return True if the key is present and False otherwise.
6. Delete multiple keys in dictionary Python:
To delete multiple keys from a dictionary while iterating, make a copy of the dictionary and use the del keyword to delete the desired keys in the iteration loop.
7. Remove element in dict Python:
To remove an element from a dictionary in Python, you can use the pop() method. This method takes the key of the item to be removed as an argument and removes the item from the dictionary.
Wrap-Up:
Understanding dictionary iteration and potential errors like the “RuntimeError: dictionary changed size during iteration” is crucial for writing robust Python code. By following best practices, utilizing appropriate methods for iteration, and implementing error handling solutions, you can avoid these errors and ensure the smooth execution of your programs. Remember to plan ahead, test thoroughly, and continuously improve your understanding of dictionaries in Python.
Python : How To Avoid \”Runtimeerror: Dictionary Changed Size During Iteration\” Error?
Keywords searched by users: runtimeerror: dictionary changed size during iteration Delete key in dictionary Python while iterating, Python delete dictionary item while iterating, Change value in dictionary Python loop, Update keys in dictionary Python, How to check a key in dictionary Python, Delete multiple keys in dictionary Python, Remove element in dict Python, Python dict pop
Categories: Top 21 Runtimeerror: Dictionary Changed Size During Iteration
See more here: nhanvietluanvan.com
Delete Key In Dictionary Python While Iterating
The delete key in dictionary Python plays a critical role when it comes to modifying and removing key-value pairs while iterating through dictionaries. Understanding how to properly use this key is essential for efficient programming and data manipulation. In this article, we will delve deep into the usage of the delete key in Python dictionaries, covering various scenarios and providing helpful insights.
Basic Concept of a Dictionary in Python
Before diving into the delete key, let’s briefly revisit the basic concept of a dictionary in Python. Dictionaries are mutable data structures that allow us to store key-value pairs. The keys are unique and act as labels to access the corresponding values. This makes dictionaries a powerful tool, especially when dealing with large datasets or complex data structures.
The Delete Key: del Statement
In Python, the del statement is responsible for removing elements from a dictionary, including key-value pairs. When removing a key-value pair, the statement utilizes the delete key. The general syntax to delete a key-value pair is as follows:
“`python
del dict_name[key]
“`
In the above syntax, `dict_name` refers to the name of the dictionary, and `key` represents the specific key that needs to be deleted. Let’s explore various scenarios where the delete key can be employed.
Deleting a Key-Value Pair
To delete a specific key-value pair in a dictionary, we need to know the key that corresponds to the pair we want to remove. Consider the following example:
“`python
fruits = {
“apple”: 2,
“banana”: 3,
“mango”: 1
}
del fruits[“banana”]
print(fruits)
“`
In the above code snippet, the dictionary `fruits` contains three key-value pairs. By using the del statement and specifying the key `”banana”`, we delete the corresponding pair. After executing the code and printing the resulting dictionary, we observe that the key-value pair “banana: 3” no longer exists:
“`
{‘apple’: 2, ‘mango’: 1}
“`
Deleting a Key-Value Pair Using a Variable
The delete key can also be accessed using a variable. This scenario is especially useful when we iterate through dictionaries and want to delete a particular key-value pair based on certain conditions. Let’s consider an example:
“`python
contacts = {
“Alice”: 1234,
“Bob”: 5678,
“Charlie”: 9101
}
key_to_delete = “Bob”
del contacts[key_to_delete]
print(contacts)
“`
In the above code snippet, we want to delete the key “Bob” and its corresponding value from the dictionary `contacts`. Instead of directly using the value, we assign the key to the variable `key_to_delete`. This approach provides flexibility and allows us to delete any key-value pair based on the condition specified within the variable.
Deleting a Key-Value Pair in a Loop
When iterating through a dictionary, deleting key-value pairs can be slightly trickier. It is important to note that we should not modify the dictionary size while iterating, as this can lead to unexpected results or errors. To overcome this issue, we use a copy of the keys or values while operating on the original dictionary.
To illustrate this concept, let’s consider an example where we remove key-value pairs that satisfy a certain condition:
“`python
scores = {
“Alice”: 85,
“Bob”: 90,
“Charlie”: 75,
“David”: 95
}
for name, score in scores.copy().items():
if score < 80:
del scores[name]
print(scores)
```
In the above code snippet, we iterate through the copy of the dictionary's items using the `.items()` method. This allows us to access both the key and the value within the loop. If a condition is met, such as a score being less than 80, we use the delete key to remove the corresponding key-value pair from the original dictionary. The resulting dictionary, `scores`, contains only the key-value pairs that satisfy the given condition:
```
{'Alice': 85, 'Bob': 90, 'David': 95}
```
FAQs about the Delete Key in Dictionary Python
Q1. Can I use the delete key to remove multiple key-value pairs at once?
A1. Yes, you can use the delete key multiple times to remove multiple key-value pairs. Simply specify each key individually, or use loops to delete many elements efficiently.
Q2. What happens if I try to delete a key that does not exist in the dictionary?
A2. If you try to delete a non-existent key, a KeyError will be raised. To avoid this, you can either check if the key exists before attempting deletion or use the `.pop()` method, which allows you to provide a default value to return if the key does not exist.
Q3. Are dictionaries the only Python data structure that uses the delete key?
A3. No, the delete key is not exclusive to dictionaries. It can be used to modify or remove elements in other mutable data structures as well, such as lists and sets.
Q4. Can I delete just the value associated with a key without deleting the key itself from the dictionary?
A4. No, when using the delete key, both the key and its corresponding value are removed as a pair. If you only want to modify the value, you can directly assign a new value to the key.
Conclusion
Understanding the usage of the delete key in Python dictionaries is crucial for efficient and accurate data manipulation. By leveraging the del statement and corresponding key, we can easily remove key-value pairs from dictionaries. Whether deleting a single pair, using variables for greater flexibility, or removing pairs in loops, the delete key proves to be a valuable tool. Armed with this knowledge, you can confidently manipulate dictionaries with ease and precision in your Python programs.
Python Delete Dictionary Item While Iterating
Python dictionaries store data as key-value pairs, where each key is unique within the dictionary. This allows for efficient retrieval of values based on their associated keys. However, when we need to remove items from a dictionary while iterating over it, we can encounter a RuntimeError: “dictionary changed size during iteration” error. This error occurs because the dictionary gets modified during iteration, leading to inconsistencies and undefined behavior.
To overcome this issue, we can employ different methods and techniques. Let’s explore some of the popular approaches below:
1. Creating a Copy or Snapshot:
One way to delete dictionary items while iterating is by taking a copy or snapshot of the dictionary before iterating. This can be achieved using the `copy()` or the `dict()` function. By iterating over the copy instead of the original dictionary, we can safely modify the dictionary without encountering any errors. Here’s an example:
“`python
my_dict = {1: “Apple”, 2: “Banana”, 3: “Cherry”}
for key in my_dict.copy():
if key % 2 == 0:
del my_dict[key]
“`
In this example, a copy of `my_dict` is created using the `copy()` method, and the original dictionary is modified based on a condition. By iterating over the copy, we avoid the RuntimeError mentioned earlier.
2. Using a Flag to Mark Items for Deletion:
Another way to delete dictionary items while iterating is by marking items for deletion using a flag variable. After the iteration is complete, we can iterate over the dictionary again to remove the marked items. Here’s an example:
“`python
my_dict = {1: “Apple”, 2: “Banana”, 3: “Cherry”}
to_delete = []
for key in my_dict:
if key % 2 == 0:
to_delete.append(key)
for key in to_delete:
del my_dict[key]
“`
In this example, we use a list called `to_delete` to store the keys that need to be removed. During the first iteration, we check the condition and append the keys to the list. In the second iteration, we delete the marked keys from the dictionary.
3. Generating a New Dictionary:
One more approach is to generate a new dictionary consisting only of the desired items, instead of modifying the original dictionary. This can be done using dictionary comprehension or using the `filter()` function. Here’s an example using dictionary comprehension:
“`python
my_dict = {1: “Apple”, 2: “Banana”, 3: “Cherry”}
new_dict = {key: value for key, value in my_dict.items() if key % 2 != 0}
“`
In this example, a new dictionary `new_dict` is created based on a condition using dictionary comprehension. Only the items with odd keys are included, effectively deleting the items with even keys.
Frequently Asked Questions (FAQs):
Q1. Why do I get a “dictionary changed size during iteration” error?
A1. This error occurs when you modify the size of a dictionary while iterating over it. It is raised to prevent inconsistencies and undefined behavior. To resolve this error, you can use approaches like creating a copy, marking items for deletion, or generating a new dictionary.
Q2. Can I use the ‘del’ keyword directly within a loop to delete dictionary items?
A2. No, it is not recommended. If you try to directly delete an item using `del` within a loop, you will encounter the “dictionary changed size during iteration” error. It is best to use one of the aforementioned approaches to delete items from a dictionary while iterating.
Q3. Are there any performance implications when deleting dictionary items while iterating?
A3. Yes, there can be performance implications when deleting items while iterating over large dictionaries. Creating a copy or generating a new dictionary consumes additional memory, while marking items for deletion requires extra iterations. Consider the size and complexity of your dictionary, as well as the frequency of the operation, to choose the most efficient approach.
Q4. Can I delete items from a dictionary while iterating using the ‘pop()’ method?
A4. Yes, you can use the `pop()` method to remove items from a dictionary while iterating. However, it is essential to track the keys iterated and not change the dictionary’s size during iteration to avoid errors.
In conclusion, deleting dictionary items while iterating in Python requires caution and appropriate techniques to avoid runtime errors. This article explored various approaches, including creating a copy, marking items for deletion, and generating a new dictionary. By understanding these methods, you can safely and efficiently delete dictionary items based on specific conditions, enhancing your Python programming skills.
Change Value In Dictionary Python Loop
In Python, dictionaries are a versatile data structure that allows us to store key-value pairs. They are widely used in programming tasks as they provide a convenient way to access, modify, and manage data. One common operation that we may need to perform is changing the value of a specific key within a dictionary. In this article, we will explore various techniques to accomplish this task using loops in Python. We will also provide answers to some frequently asked questions related to this topic.
Before diving into the details, let’s quickly go through the basic syntax of a Python dictionary. Dictionaries are defined using curly braces {} and consist of key-value pairs, where each key is separated from its corresponding value by a colon. Here’s an example:
“`python
my_dict = {‘key1’: value1, ‘key2’: value2, ‘key3’: value3}
“`
To change the value associated with a specific key in a dictionary, we first need to access that key. This can be achieved using bracket notation combined with the key name. Once we have accessed the key, we can assign a new value to it. Let’s take a look at a simple example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict[‘age’] = 30
print(my_dict)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
“`
In the example above, we accessed the key ‘age’ using bracket notation and assigned a new value of 30 to it. The updated dictionary was then printed, showing the modified value of ‘age’.
Now let’s move on to the main topic of this article – changing the values in a dictionary using loops. There are multiple scenarios where we might need to iterate through a dictionary and update its values based on certain conditions. We will discuss two common approaches to achieve this.
1. Using a for loop:
One way to change values in a dictionary is by iterating through its keys using a for loop and updating the values accordingly. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key in my_dict:
if key == ‘age’:
my_dict[key] = 30
print(my_dict)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
“`
In the above example, we looped through every key in the dictionary using a for loop. When we encountered the key ‘age’, we assigned a new value of 30 to it.
2. Using dictionary comprehension:
Another efficient way to change values in a dictionary is by using dictionary comprehension. Dictionary comprehension provides a concise way to create dictionaries based on some condition. Here’s an example that demonstrates this approach:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict = {key: 30 if key == ‘age’ else value
for key, value in my_dict.items()}
print(my_dict)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
“`
In this example, we used dictionary comprehension to iterate through the items in our dictionary. If the key is ‘age’, the value is updated to 30; otherwise, it remains the same.
Frequently Asked Questions (FAQs):
Q: Can I change multiple values in a dictionary at once?
A: Yes, you can change multiple values using any of the aforementioned methods. Simply add conditions based on different keys to modify the desired values.
Q: How can I change the values of multiple keys based on certain conditions?
A: You can use if statements or any other conditional statements within the loop to modify the values based on specific conditions. This allows you to update several values simultaneously.
Q: Is it possible to change dictionary values without using loops?
A: Yes, it is possible to change dictionary values without using loops. You can directly access and modify the values using the key. However, loops are useful when you need to iterate through a dictionary and update multiple values based on certain conditions.
Q: Can I change the keys of a dictionary using the same techniques?
A: No, the discussed techniques are focused on changing values, not keys. Keys in a dictionary are immutable, meaning they cannot be changed. If you need to change a key, you will have to create a new key-value pair and remove the old one.
Conclusion:
Changing values in a dictionary using loops is a common operation in Python programming. We explored two approaches – using a for loop and dictionary comprehension – to modify values based on certain conditions. By understanding these techniques, you can efficiently update values in a dictionary according to your requirements.
Images related to the topic runtimeerror: dictionary changed size during iteration
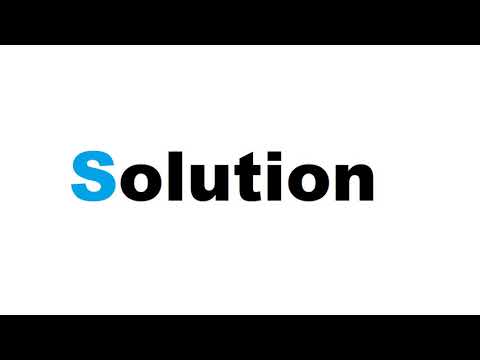
Found 25 images related to runtimeerror: dictionary changed size during iteration theme
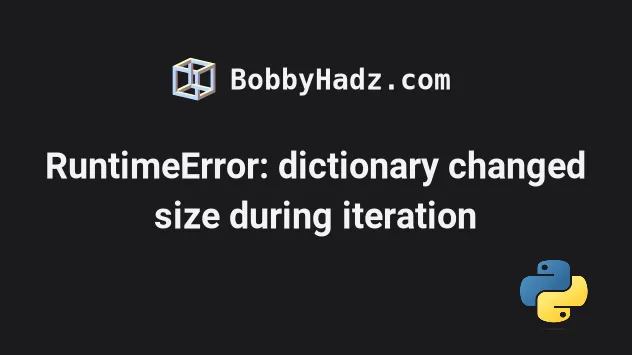

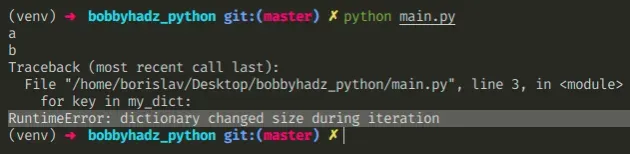
![SOLVED] Runtimeerror: dictionary changed size during iteration Solved] Runtimeerror: Dictionary Changed Size During Iteration](https://itsourcecode.com/wp-content/uploads/2023/05/Runtimeerror-dictionary-changed-size-during-iteration.png)

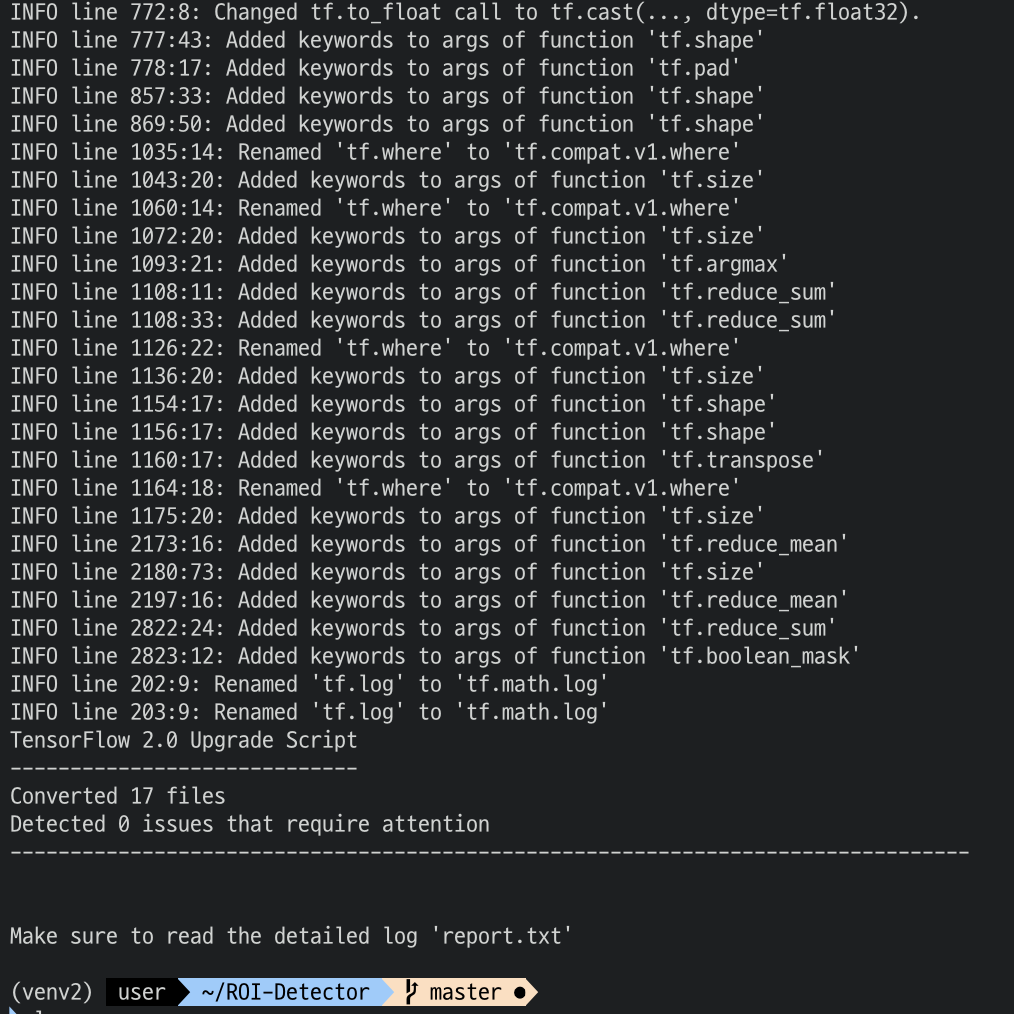
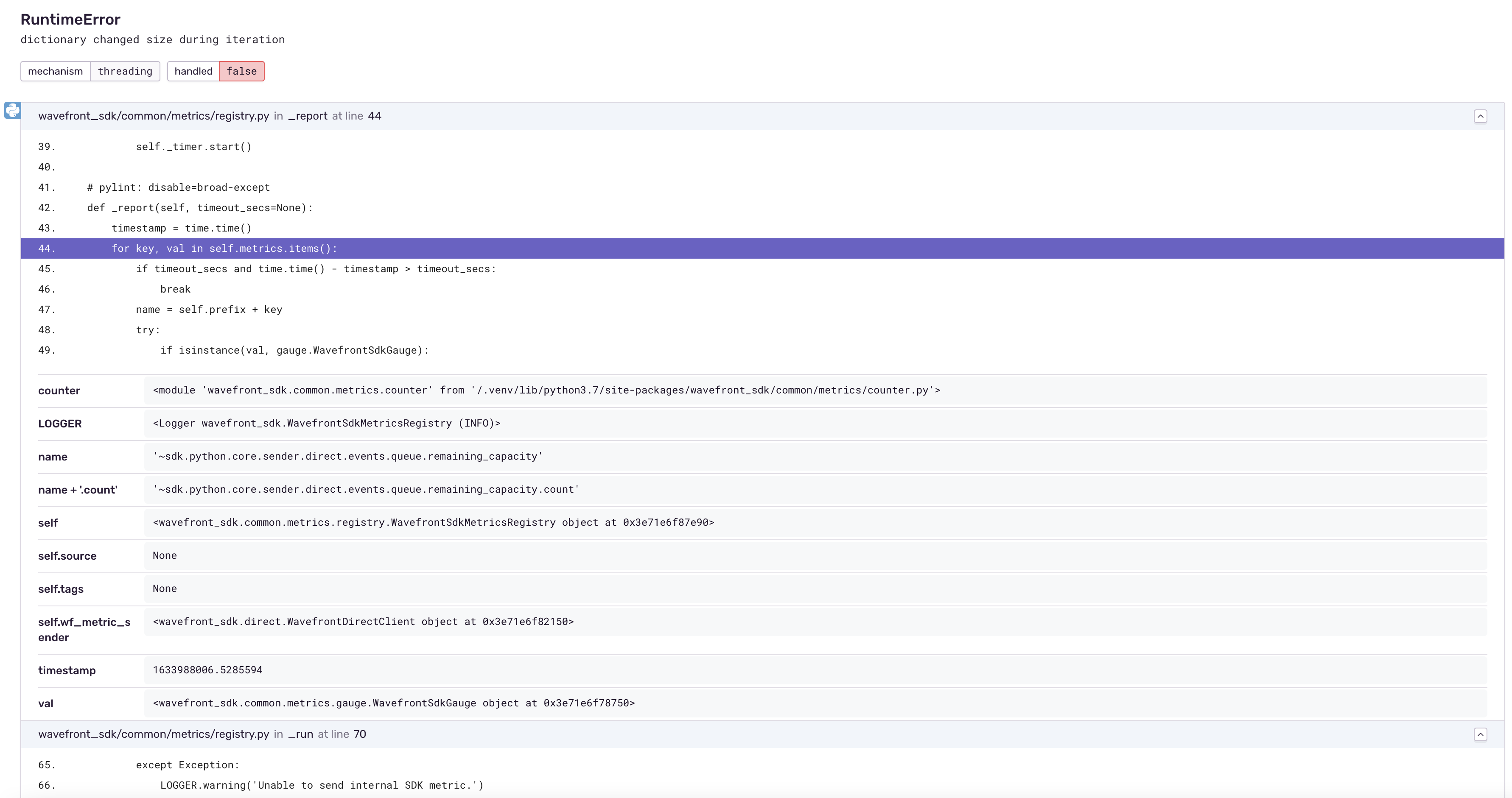

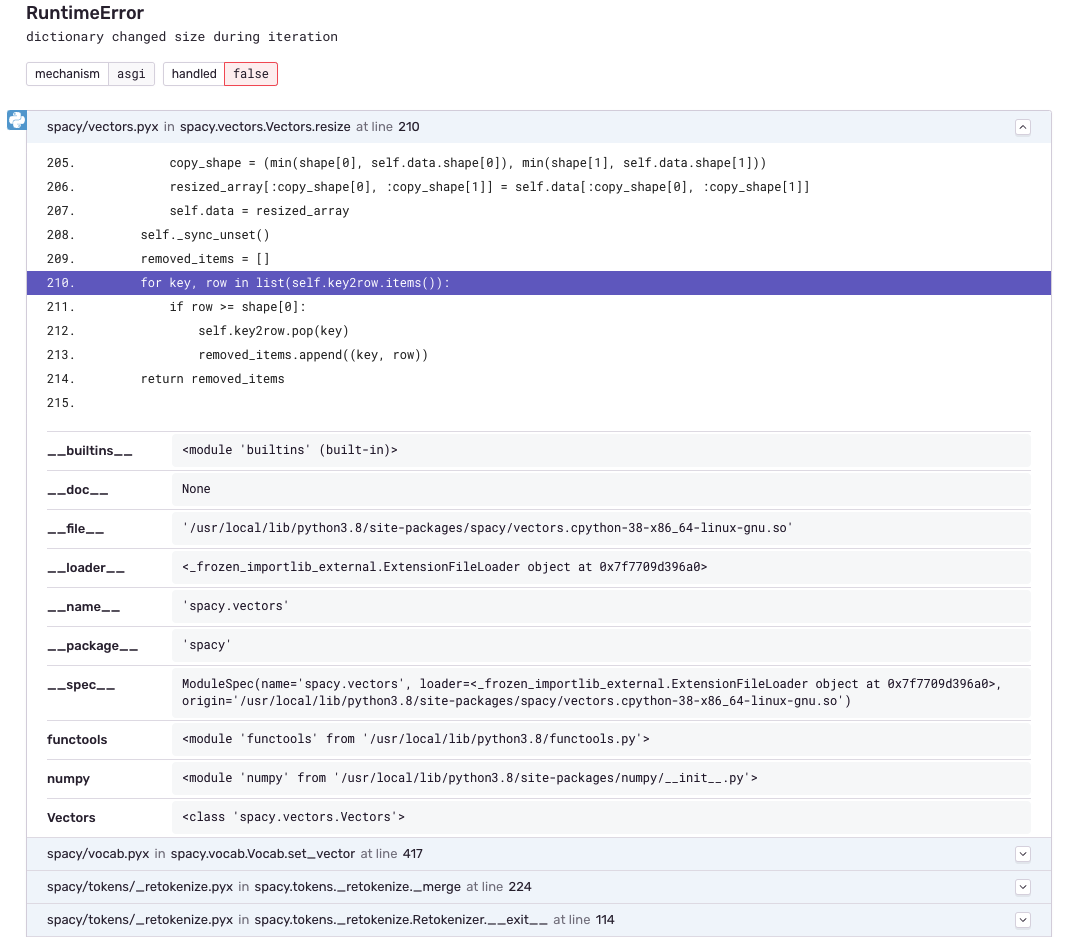

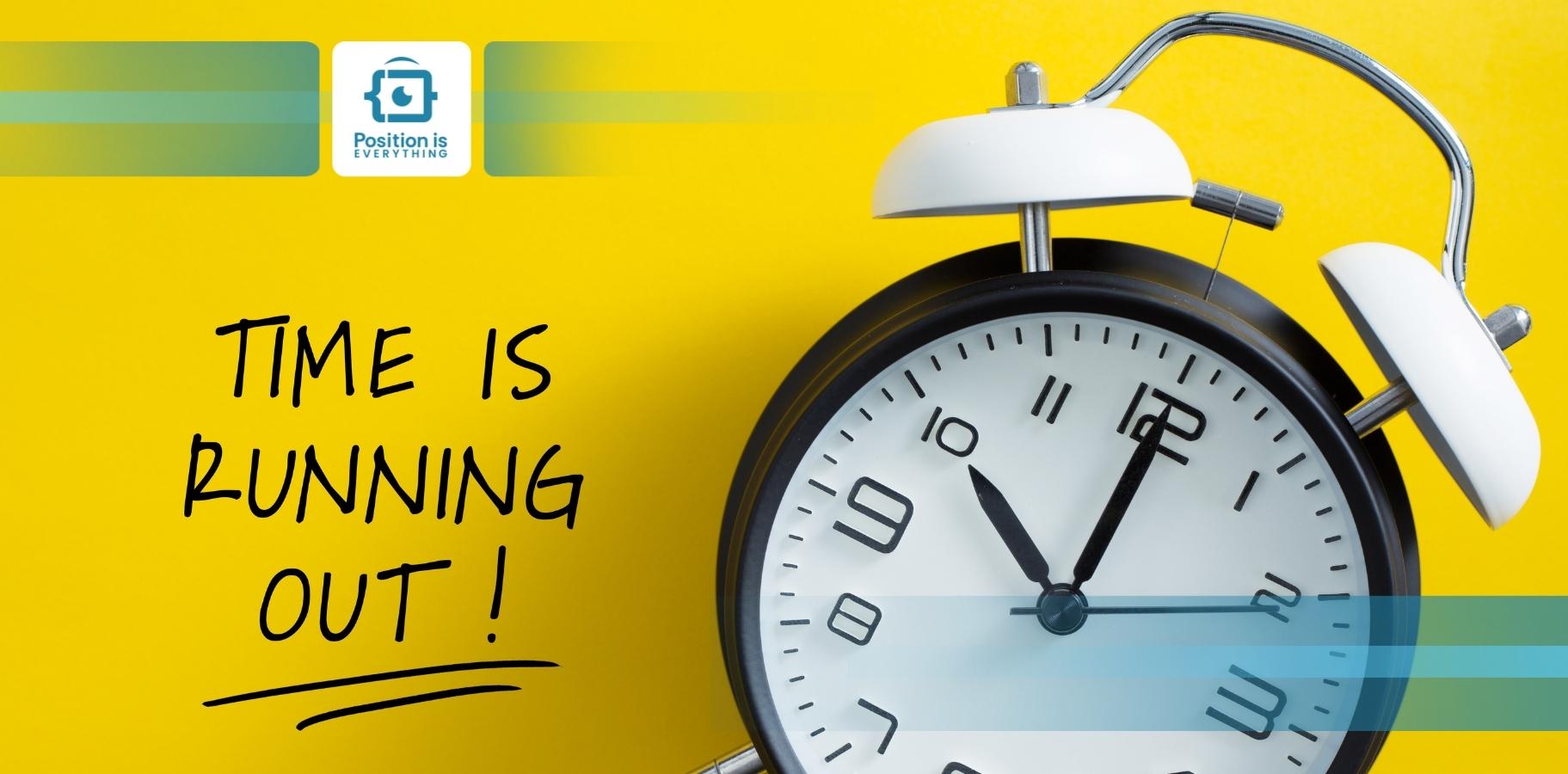
![Python] dict 에서 RuntimeError: dictionary changed size during iteration 해결하는 방법 Python] Dict 에서 Runtimeerror: Dictionary Changed Size During Iteration 해결하는 방법](https://blog.kakaocdn.net/dn/bDCxaJ/btqDlOBKUX1/eutySgtO3WFJRptKtKYnJ0/img.png)

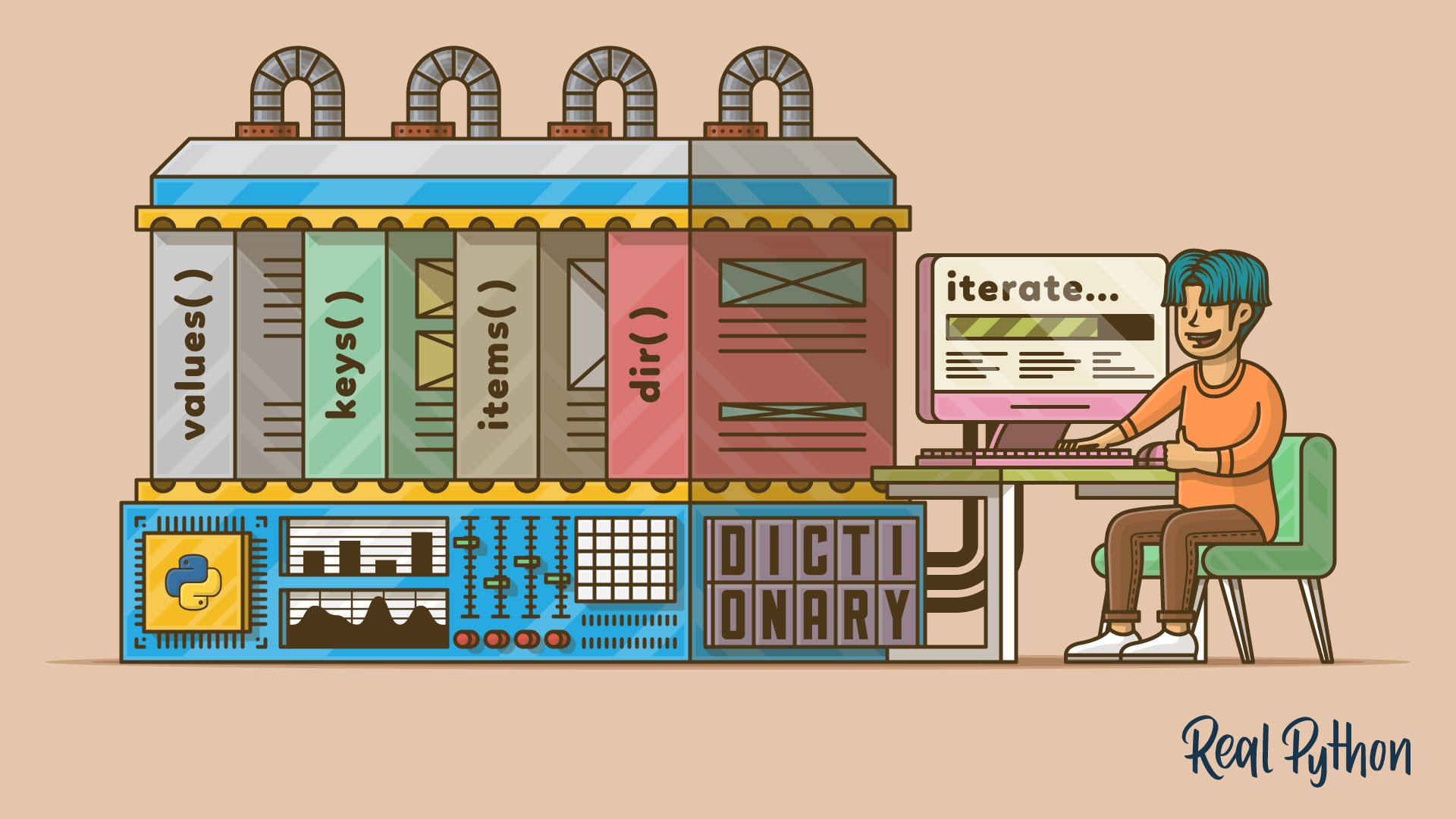
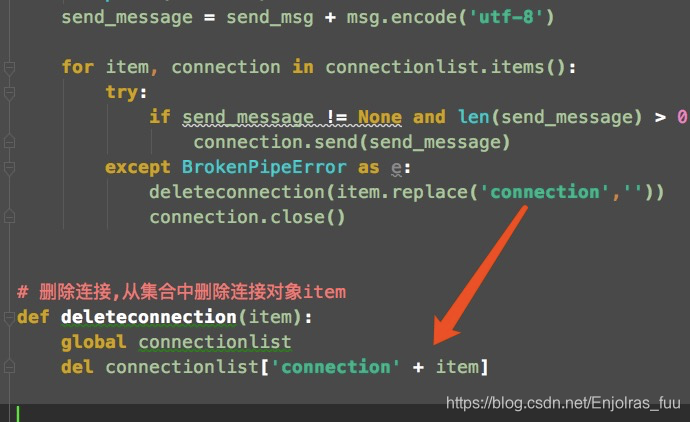
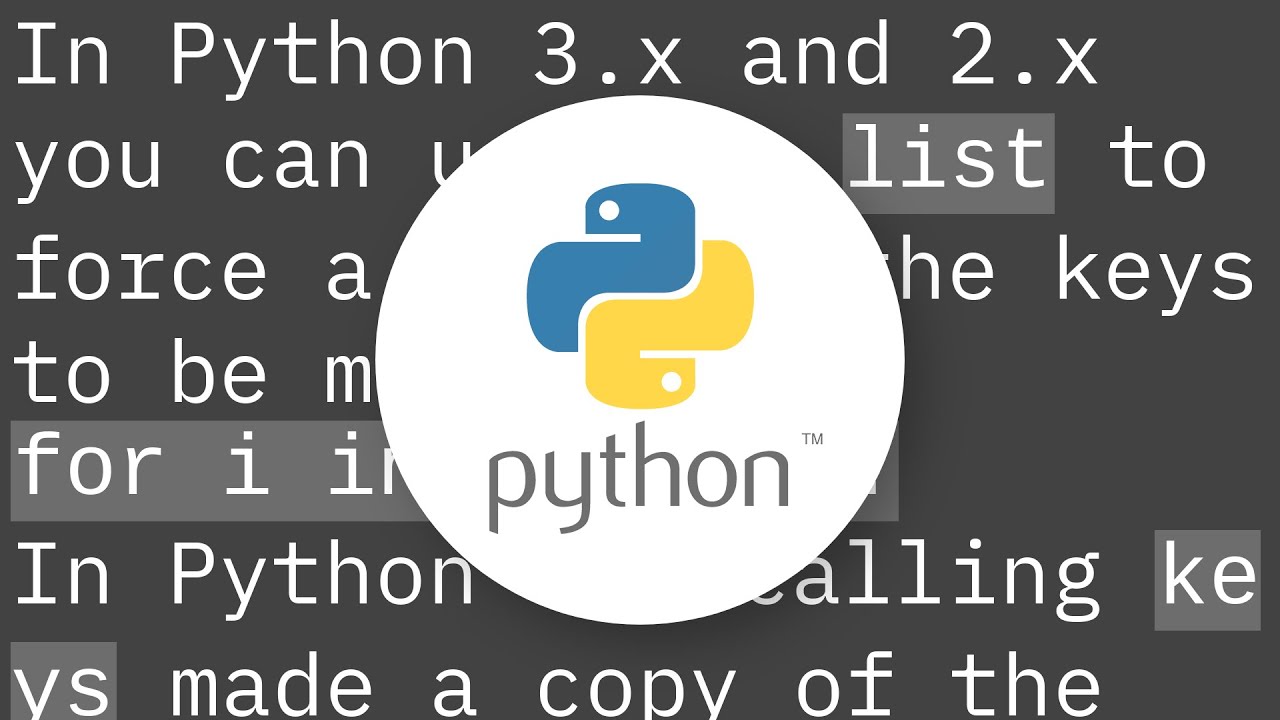

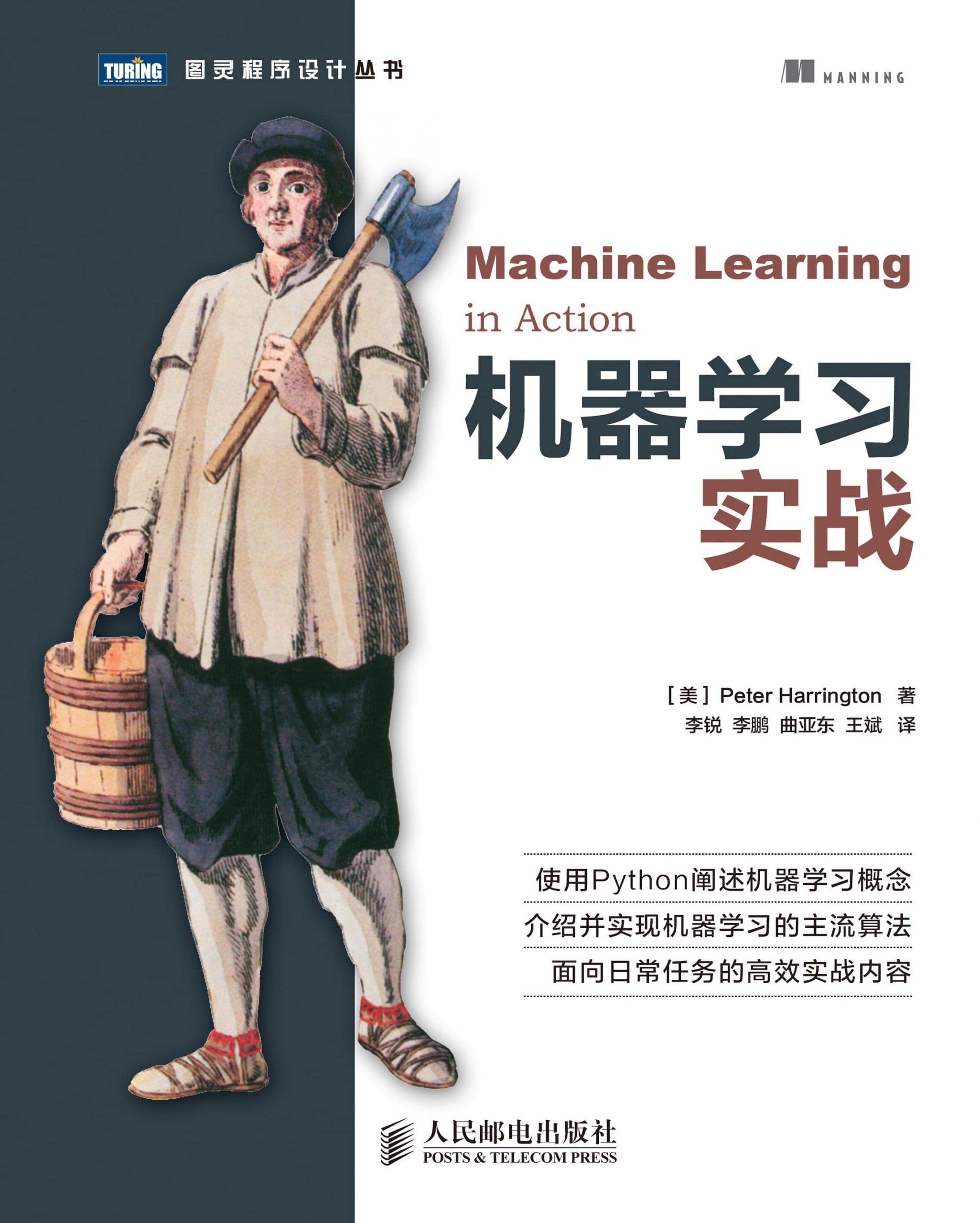
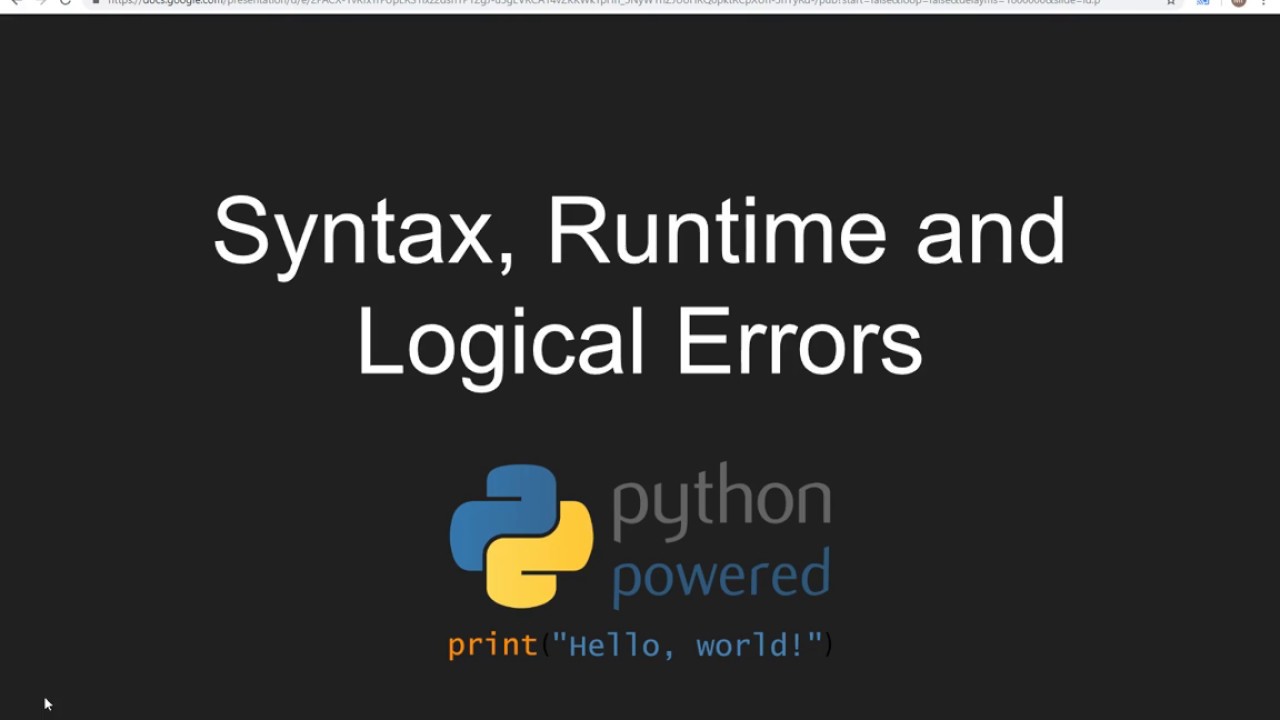
![Python] dictionary changed size during iteration Python] Dictionary Changed Size During Iteration](https://blog.kakaocdn.net/dn/Zlv3Q/btrikMdHyb4/iB0ZrhMlqQCwWzNRQiFBv0/img.jpg)


![Python] dict 에서 RuntimeError: dictionary changed size during iteration 해결하는 방법 Python] Dict 에서 Runtimeerror: Dictionary Changed Size During Iteration 해결하는 방법](https://blog.kakaocdn.net/dn/WmrKM/btqDj28RBTg/jZKK9VC9qOPvmtzzR7T0d1/img.png)

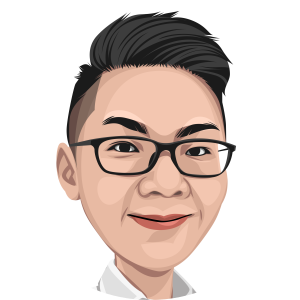



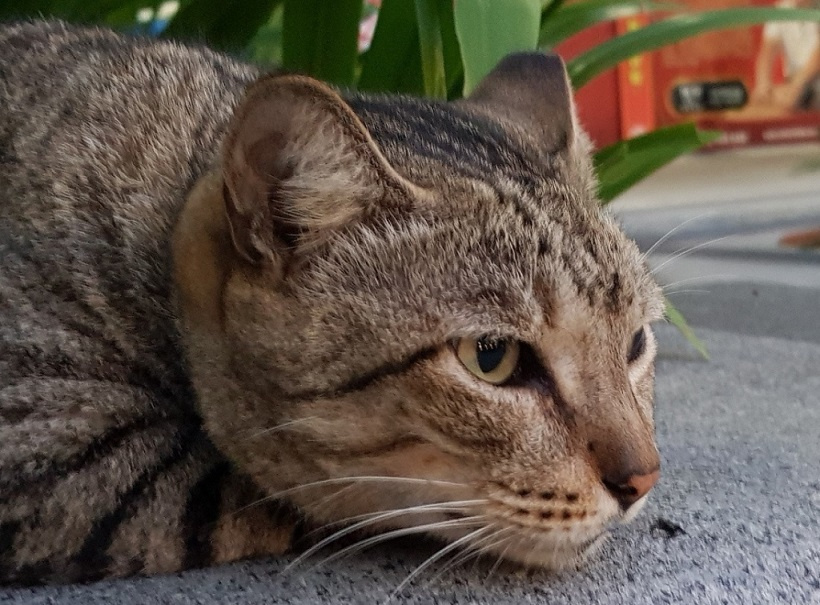
![CLI] RuntimeError: OrderedDict mutated during iteration Cli] Runtimeerror: Ordereddict Mutated During Iteration](https://user-images.githubusercontent.com/8812459/121072347-f3375680-c785-11eb-8449-ff4bfdd72fad.png)

![SOLVED] Runtimeerror: cuda out of memory. Solved] Runtimeerror: Cuda Out Of Memory.](https://itsourcecode.com/wp-content/uploads/2023/05/Runtimeerror-cuda-out-of-memory.png)
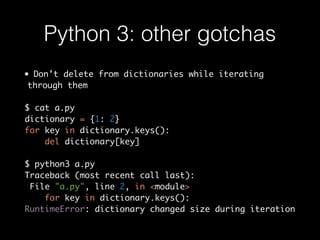
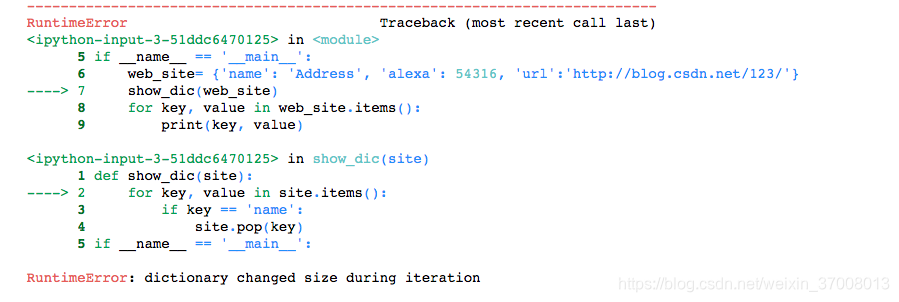

Article link: runtimeerror: dictionary changed size during iteration.
Learn more about the topic runtimeerror: dictionary changed size during iteration.
- dictionary changed size during iteration” error? – Stack Overflow
- RuntimeError: dictionary changed size during iteration
- Dictionary Changed Size During Iteration – Python Clear
- How to fix RuntimeError: dictionary changed size during iteration
- Runtimeerror: Dictionary Changed Size During Iteration
- Fix Error – Dictionary Changed Size During Iteration – Delft Stack
- Getting RuntimeError: dictionary changed size during iteration …
- [SOLVED] Runtimeerror: dictionary changed size during iteration
- 3 Ways to Fix RuntimeError: dictionary changed size during …
See more: blog https://nhanvietluanvan.com/luat-hoc