React Hook Useeffect Has A Missing Dependency
In React, the useEffect hook is a vital tool for managing side effects in functional components. Side effects refer to any actions that happen outside of the component’s render, such as data fetching, subscription setup, or manually changing the DOM.
The useEffect hook allows you to perform these side effects in a declarative manner, ensuring they are properly managed and executed. It takes a function as its first argument, which will be executed after every render. Additionally, it can take a second argument, an array of dependencies, that specify when the effect should be re-executed.
Understanding Missing Dependency Warnings in useEffect
When using the useEffect hook, you may encounter a warning message stating that a dependency is missing from the dependency array. This warning is triggered when the function inside useEffect uses a variable that is not listed as a dependency.
The purpose of listing dependencies is to inform React when the effect should be re-executed. By omitting a dependency, you’re essentially telling React that the effect should not depend on it, potentially leading to unexpected behaviors.
Common Causes of Missing Dependency Warnings
There are a few common causes of missing dependency warnings in useEffect:
1. Forgetting to include a dependency: If a variable is used inside the effect but not listed as a dependency, React will issue a warning. This often occurs when refactoring code and forgetting to update the dependency array accordingly.
2. Incorrectly specifying dependencies: Sometimes, developers mistakenly include unnecessary dependencies or omit required ones. This can happen when they don’t fully understand the effect’s dependencies or mistakenly assume that a certain dependency is not needed.
The Impact of Not Handling Missing Dependency Warnings
Ignoring or not properly addressing missing dependency warnings can lead to subtle bugs and unexpected behavior in your application. Without explicitly defining dependencies, the effect may not re-run when the expected state or props change. This can result in stale data, incorrect UI updates, or even infinite loops.
Additionally, failing to handle these warnings makes your code more difficult to maintain and understand. Future developers or even yourself may have a hard time identifying the intent and potential issues of the effect.
How to Identify Missing Dependencies in useEffect
To identify missing dependencies in useEffect, you need to carefully review the code inside the effect and identify any variables or functions it depends on. Once you have identified them, you should ensure that they are included in the dependency array as well.
To help you catch missing dependencies, React provides a lint rule called `react-hooks/exhaustive-deps`. Enabling this rule will throw a warning whenever a dependency is missing from the array.
Best Practices for Handling Missing Dependency Warnings
To handle missing dependency warnings in useEffect, follow these best practices:
1. Understand the purpose of each dependency: Before adding or removing dependencies, ensure that you understand why they are needed. Documenting the dependencies and their purpose can help you and other developers maintain the codebase.
2. Include all necessary dependencies: By including all the necessary dependencies, you ensure that your effect runs when the expected state or props change. This helps avoid stale data and unexpected behavior.
3. Remove unnecessary dependencies: If a variable or function is not needed in the effect, you can safely remove it from the dependency array. This keeps the code cleaner and helps prevent unnecessary re-renders.
Potential Pitfalls When Dealing with Missing Dependencies
When handling missing dependency warnings, there are a few potential pitfalls to be aware of:
1. Overloading the dependency array: Including unnecessary dependencies or large objects in the array can negatively impact performance. It’s important to strike a balance between providing enough information for React to re-run the effect and preventing unnecessary re-renders.
2. Forgetting to update the dependency array: When refactoring your code or making changes to the effect’s dependencies, it’s easy to forget to update the dependency array accordingly. Always double-check that the dependencies accurately reflect the effect’s requirements.
Workarounds for Missing Dependency Warnings
There may be cases where you intentionally want to omit a dependency from the array. In such situations, React provides workarounds to address the missing dependency warning:
1. Using the useCallback hook: If your effect relies on a function that is created inside the component, you can use useCallback to memoize the function and prevent unnecessary re-creation. This can help avoid adding the function as a dependency.
2. Disabling the lint rule locally: While not recommended, you can disable the `react-hooks/exhaustive-deps` rule for a specific useEffect instance by adding a comment above it with the disable directive.
Benefits of Resolving Missing Dependency Warnings
Resolving missing dependency warnings brings several benefits to your codebase:
1. Improved code reliability: By explicitly listing dependencies, you ensure that the effect runs when the expected state or props change, reducing the likelihood of bugs and unexpected behavior.
2. Enhanced code understanding and maintainability: By addressing the warnings, your code becomes more self-explanatory and easier to maintain. Future developers can better understand the effect’s dependencies and potential issues.
Conclusion
The useEffect hook is a crucial tool for handling side effects in React Hooks. It is important to understand and address missing dependency warnings to ensure the reliability and maintainability of your code. By following best practices and staying vigilant when handling these warnings, you can write cleaner, more robust code in your React applications.
FAQs:
Q: What does the missing dependency warning in useEffect mean?
A: The missing dependency warning in useEffect indicates that a variable or function used inside the effect is not listed as a dependency. This can lead to unexpected behavior and should be addressed to ensure the reliability of your code.
Q: How do I handle missing dependency warnings in useEffect?
A: To handle missing dependency warnings, you should carefully review the code inside the effect, identify any missing dependencies, and add them to the dependency array. You can also leverage the `react-hooks/exhaustive-deps` lint rule to catch missing dependencies.
Q: Can I omit a dependency from the useEffect dependency array?
A: While possible, it is generally not recommended to omit a dependency from the array unless you have a specific reason to do so. Omitting a dependency can lead to stale data, incorrect UI updates, or infinite loops.
Q: What are the best practices for handling missing dependency warnings?
A: The best practices for handling missing dependency warnings include understanding the purpose of each dependency, including all necessary dependencies, and removing unnecessary ones. Also, remember to strike a balance between providing enough information for React to re-run the effect and preventing unnecessary re-renders.
Q: Are there any workarounds for missing dependency warnings?
A: React provides workarounds for missing dependency warnings, such as using the useCallback hook to memoize functions or locally disabling the `react-hooks/exhaustive-deps` rule. However, it is important to use these workarounds judiciously and ensure they do not compromise the reliability of your code.
Fixing Useeffect Has A Missing Dependency Warning In React
Keywords searched by users: react hook useeffect has a missing dependency react-hooks/exhaustive-deps, React hooks must be called in the exact same order in every component render, Either include it or remove the dependency array, useEffect dependency, useEffect without dependency, useEffect not working, useEffect dispatch dependency, React Hook names must start with the word use react-hooks rules of hooks
Categories: Top 93 React Hook Useeffect Has A Missing Dependency
See more here: nhanvietluanvan.com
React-Hooks/Exhaustive-Deps
React is a popular JavaScript library for building user interfaces. It provides a simple and efficient way of creating reusable UI components and managing state within them. One of the key features introduced in React 16.8 was Hooks, which revolutionized how developers write components.
Hooks allow developers to use state and other React features in functional components, whereas before, these features were only available to class components. They provide a more concise and readable way of writing code, making it easier to understand and maintain. One important aspect of using hooks is understanding the concept of exhaustive dependencies.
What are Hooks?
Before diving into exhaustive dependencies, let’s briefly understand what hooks are. Hooks are functions that let you “hook into” React features like state or lifecycle methods within functional components. They aim to provide a simpler alternative to class-based components by allowing you to use state and other React features without writing a class.
There are several built-in hooks in React, such as useState, useEffect, useContext, and more. These hooks allow you to manage state, perform side effects, and access context within a functional component. Instead of writing separate lifecycle methods like componentDidMount or componentDidUpdate, you can use the useEffect hook.
Introducing Exhaustive Dependencies
When using hooks, it’s important to understand how to ensure that the dependencies you pass to them are exhaustive. In simple terms, an exhaustive dependency means that every value that the effect depends on is included in its dependency array. This ensures that the effect is re-run whenever any of the dependencies change.
The dependency array is an optional second parameter to the useEffect hook and is used to specify the values that the effect depends on. If you omit this array, the effect will run after every render. However, if you provide an empty array, the effect will only run once, similar to componentDidMount.
Why are Exhaustive Dependencies Important?
Exhaustive dependencies are important because they ensure that your effects are executed correctly and avoid unintended bugs or side effects. When an effect relies on a value that is not included in the dependency array, it can lead to unexpected behavior.
For example, consider a component that fetches data from an API using an effect. If the effect depends on a prop that updates, but is not listed in the dependency array, it will continue using the previous value of the prop. This can lead to stale or incorrect data being displayed to the user.
By using exhaustive dependencies, you can guarantee that your effects are always up-to-date with the latest values and react accordingly to changes.
How to Use Exhaustive Dependencies?
To use exhaustive dependencies, you need to explicitly list all the values that the effect depends on in the dependency array.
For example, let’s say you have a component that fetches user data based on a dynamic user ID prop. You want the effect to re-run whenever the user ID prop changes. Here’s how you can write the effect with exhaustive dependencies:
“`javascript
useEffect(() => {
// Fetch user data based on the user ID prop
// …
}, [userID]); // userID is the prop this effect depends on
“`
In this example, whenever the userID prop changes, the effect will be re-run with the latest value of the prop. If you forget to include the userID prop in the dependency array, the effect will not re-run when the prop updates.
Frequently Asked Questions (FAQs):
Q1. What happens if I omit the dependency array in useEffect?
A1. If you omit the dependency array, the effect will run after every render. This can lead to performance issues or unnecessary re-rendering, especially if the effect performs heavy computations or updates the UI frequently.
Q2. Can I use multiple values in the dependency array?
A2. Yes, you can include multiple values in the dependency array. The effect will run whenever any of the values in the array change.
Q3. What should I do if my effect depends on a value that doesn’t change?
A3. If your effect depends on a value that doesn’t change, you can provide an empty array ([]), ensuring that the effect only runs once, similar to componentDidMount.
Q4. How can I ensure that all dependencies are included in the array without manually checking each one?
A4. Linting tools like ESLint have plugins (e.g., eslint-plugin-react-hooks) that can automatically warn you if any dependencies are missing in the dependency array. Using these tools can help ensure that your dependencies are exhaustive.
In conclusion, React Hooks have changed the way developers write components in React. With the introduction of exhaustive dependencies, developers can ensure that their effects are executed correctly and react to changes in dependencies. By explicitly listing all the values that an effect depends on, you can avoid unintended bugs and ensure that your components behave as expected. Remember to always include all dependencies in the dependency array to leverage the full power of hooks and create robust React applications.
React Hooks Must Be Called In The Exact Same Order In Every Component Render
React Hooks, introduced in React version 16.8, provide developers with a new way to write reusable and stateful logic without using class components. One of the key rules to keep in mind when working with React Hooks is that they must be called in the exact same order in every component render. This may seem like a simple rule, but it is crucial for maintaining the integrity and predictability of your application. In this article, we will explore why this rule is important, the consequences of breaking it, and provide examples of how to adhere to this rule effectively.
Why is the Order of React Hooks Important?
The order in which React Hooks are called is important because Hooks rely on the order of their calls to associate stateful logic with specific components and maintain correct functionality. When Hooks are called in a different order during different renders of a component, React would not be able to correctly associate the old and new state, leading to unexpected behavior and bugs in your application.
Furthermore, React optimizes the rendering process by associating each Hook with a specific component instance. When the order of Hooks changes between renders, React won’t know how to properly map the stateful logic to the specific component instance. This can result in inconsistencies and potentially cause components to render incorrectly or fail to work altogether.
Consequences of Breaking the Rule
If the order of React Hooks is not maintained consistently in every component render, various problems can arise, including:
1. Loss of State: Hooks are designed to manage component state. If the order is not the same in every render, React would be unable to preserve the state between renders. This means that the component’s state would be lost, and any changes made by the user or other event triggers would not be remembered or reflected in the UI. This can result in a poor user experience and make your application unreliable.
2. Inconsistent Behavior: When the order of Hooks is inconsistent, the behavior of your components becomes unpredictable. For example, you might see state-related issues such as values not updating correctly or components not re-rendering when expected. These inconsistencies can be confusing for developers trying to debug the code and may lead to a significant waste of time and effort.
3. Difficult Debugging: Breaking the rule of calling Hooks in the same order can make it challenging to identify the cause of errors. As the order becomes chaotic, it becomes increasingly difficult to trace the source of a particular bug or issue. Debugging becomes a tedious task that requires careful analysis and trial and error, significantly slowing down the development process.
Adhering to the Rule
To ensure that Hooks are called in the exact same order in every component render, follow these best practices:
1. Consistent Hook Ordering: Make it a habit to maintain a consistent order of Hooks in every render of your component. When adding new Hooks, be mindful of the order in which they are called. Avoid changing the position of existing Hooks. This consistency will prevent any unexpected behavior related to state management.
2. Leverage Linting Tools: Static analysis tools like ESLint can help enforce this rule by providing warnings or errors if Hooks are called in an inconsistent order. Enable linting rules that specifically target Hook ordering, such as the ‘exhaustive-deps’ rule in the eslint-plugin-react-hooks package. Utilizing these tools can help catch issues early in the development process.
3. Code Review: Encourage code reviews within your development team to ensure that the correct ordering of Hooks is followed. Having a fresh pair of eyes on your codebase can help identify potential issues and ensure that the rule is adhered to throughout the project.
4. Regular Testing: Frequent testing is crucial to ensure that your components are rendering as expected and that the order of Hooks is maintained properly. Write comprehensive unit tests that cover all possible scenarios and edge cases. Test the component under different configurations and verify that the state remains consistent despite changing conditions.
FAQs
Q: Can I skip calling a Hook if I don’t need it in a certain render?
A: No, Hooks must be called in the same order on every render. If a Hook is not required for a particular render, it’s essential to call it regardless, but you can simply return a null value to ignore its result.
Q: What happens if I violate the rule of calling Hooks in the same order?
A: Violating this rule can result in unexpected behavior, lost state, and difficulties in debugging. Therefore, it’s crucial to adhere to the rule to ensure the stability and reliability of your React components.
Q: Can I call custom Hooks conditionally?
A: Yes, you can call custom Hooks conditionally as long as the condition doesn’t change between renders. However, you shouldn’t call Hooks inside loops, conditions, or nested functions, as it can lead to inconsistent behavior.
In conclusion, maintaining the same order when calling React Hooks in every component render is crucial for proper state management and consistent functionality. Breaking this rule can lead to a loss of state, unpredictable behavior, and difficulties in debugging. By following best practices, leveraging linting tools, conducting code reviews, and regular testing, you can ensure that your Hooks are called in the correct order, leading to stable and reliable React components.
Images related to the topic react hook useeffect has a missing dependency
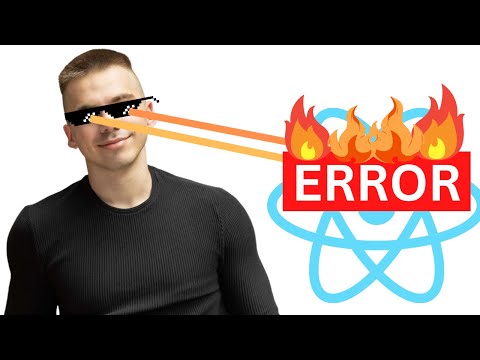
Found 43 images related to react hook useeffect has a missing dependency theme


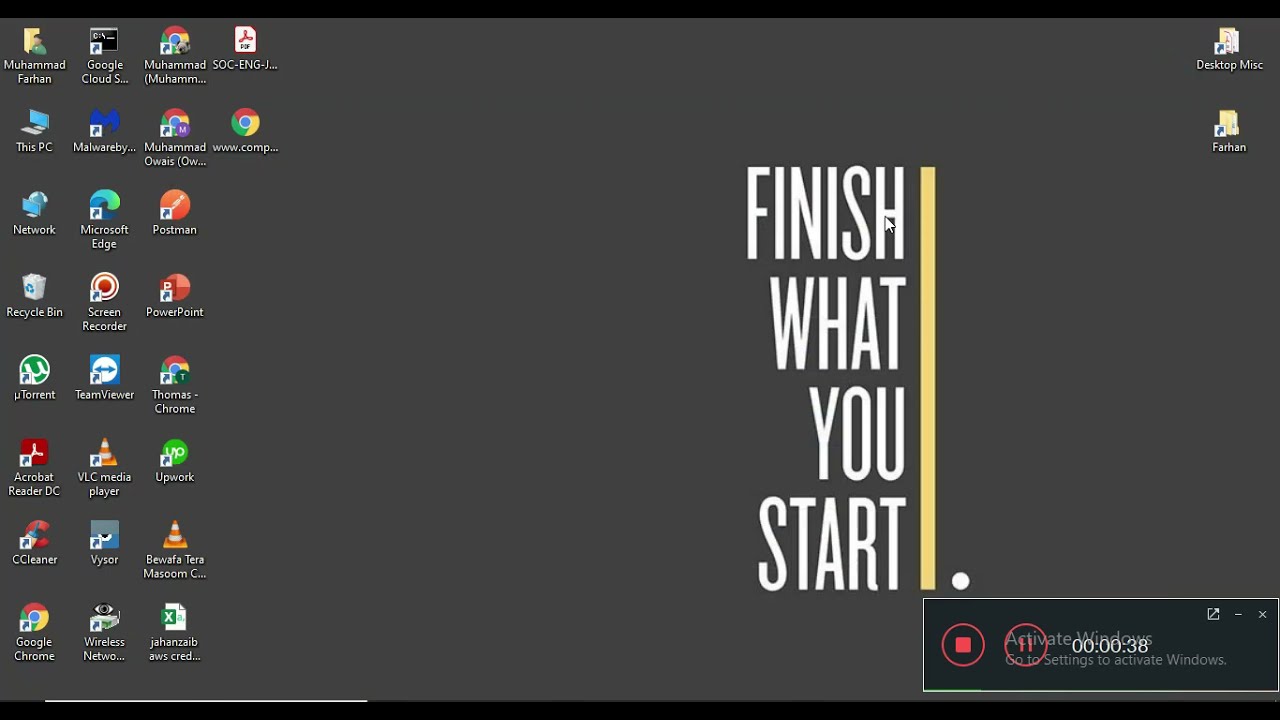
![Solved] React Hook useEffect has a missing dependency: 'Web3Api.account' - Moralis General - Moralis Web3 Forum - Largest Web3 Dev Community 📈 Solved] React Hook Useeffect Has A Missing Dependency: 'Web3Api.Account' - Moralis General - Moralis Web3 Forum - Largest Web3 Dev Community 📈](https://i.ytimg.com/vi/HrlpTJD_CF0/maxresdefault.jpg)

![리액트 에러 해결] React Hook useEffect has a missing dependency 리액트 에러 해결] React Hook Useeffect Has A Missing Dependency](https://blog.kakaocdn.net/dn/LWHIF/btrGGbnHyrp/dJIrC1gTugu8CKXEQHqJQ1/img.png)
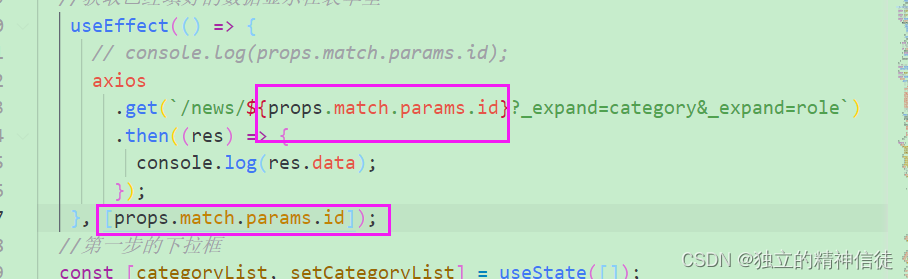
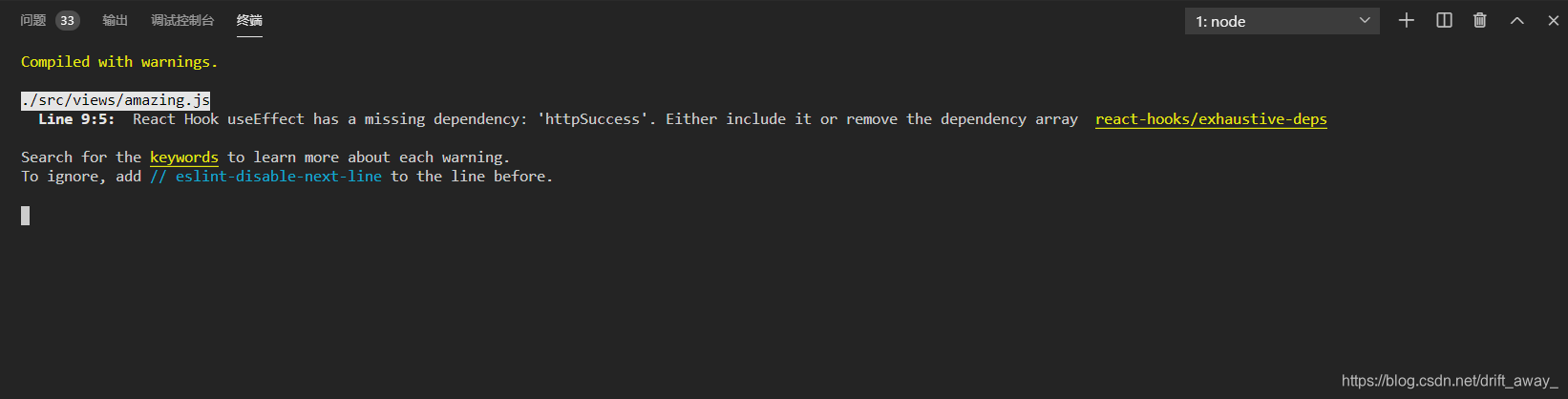
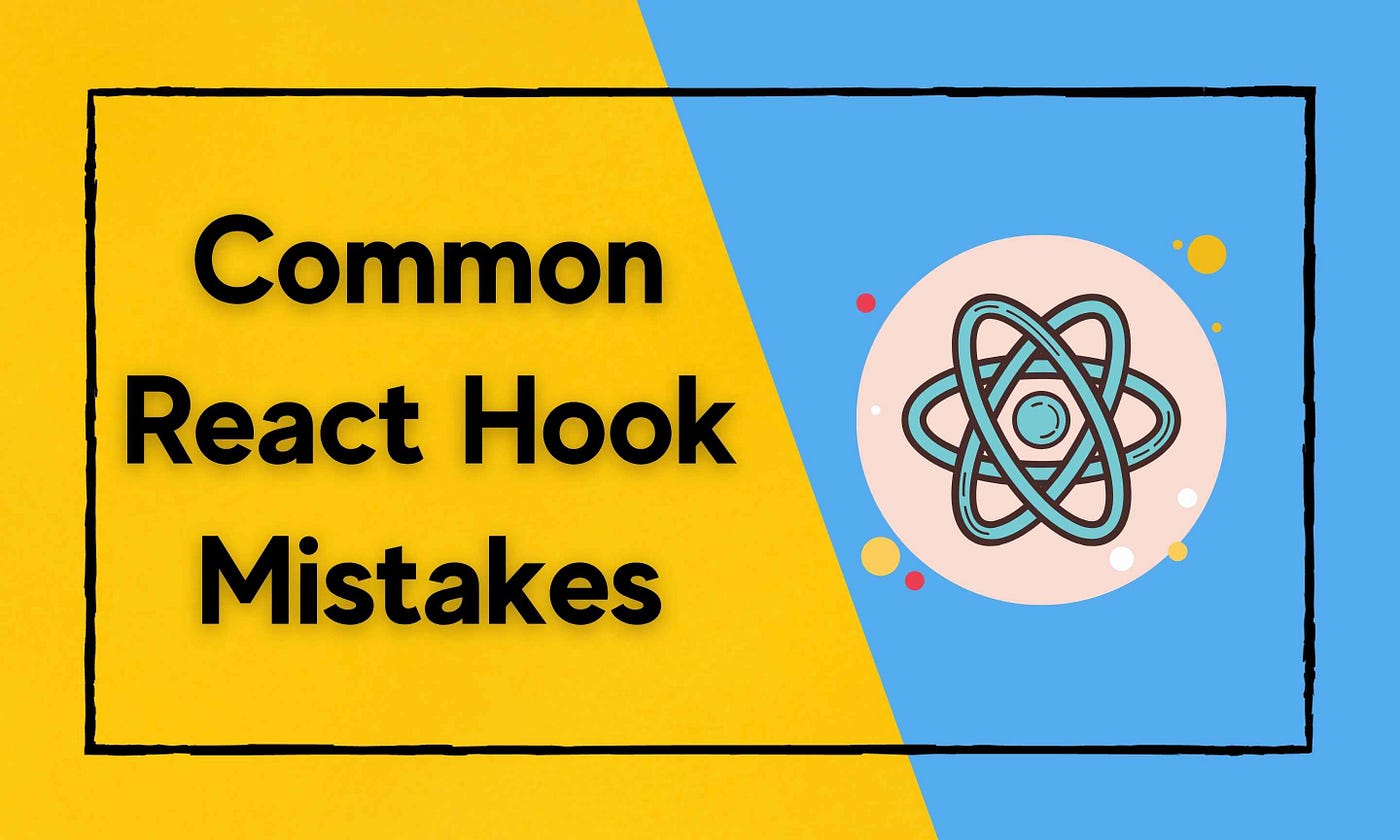
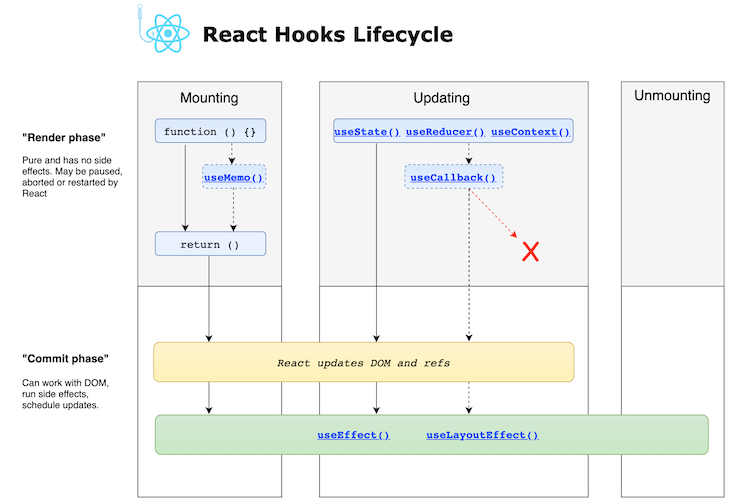

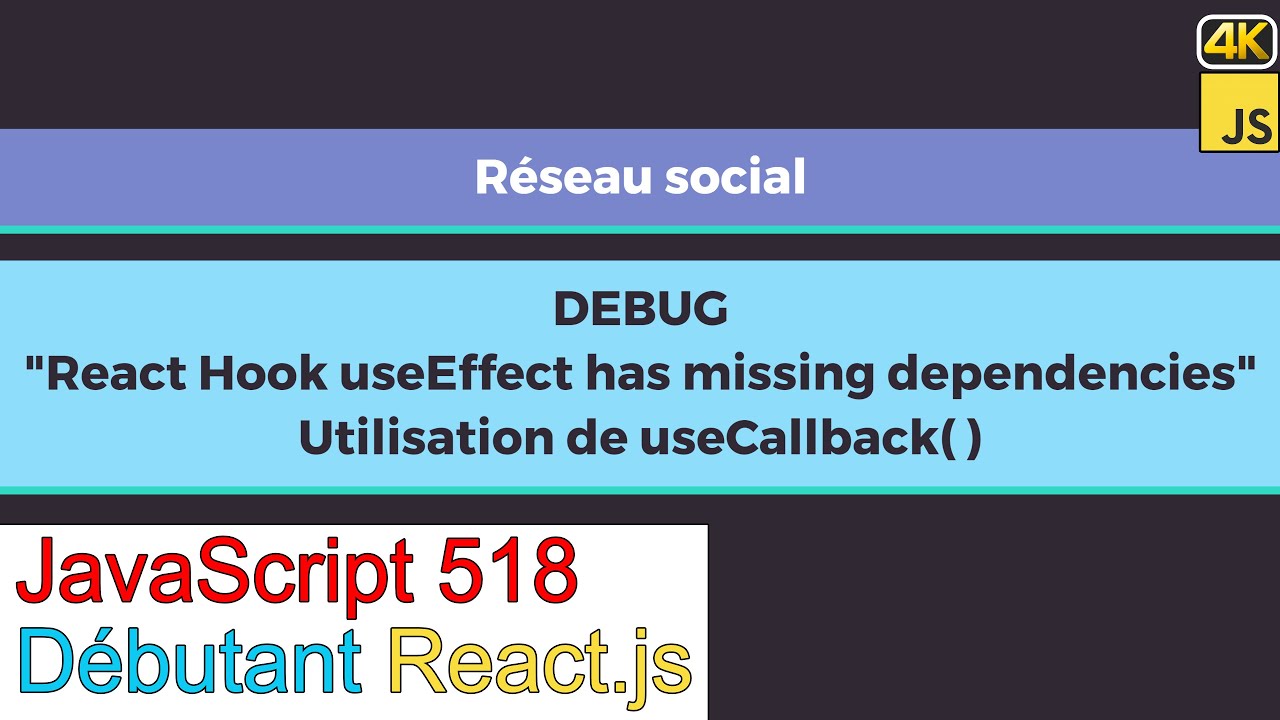

![React 오류] React Hook useEffect has a missing dependency: ' '. Either include it or remove the dependency array react-hooks/exhaustive-deps React 오류] React Hook Useeffect Has A Missing Dependency: ' '. Either Include It Or Remove The Dependency Array React-Hooks/Exhaustive-Deps](https://blog.kakaocdn.net/dn/nMLAz/btq3Mkg9n1l/V2Rdkvt2keMd0wbHKBWjqK/img.png)
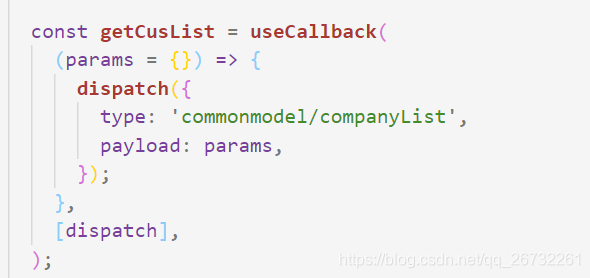


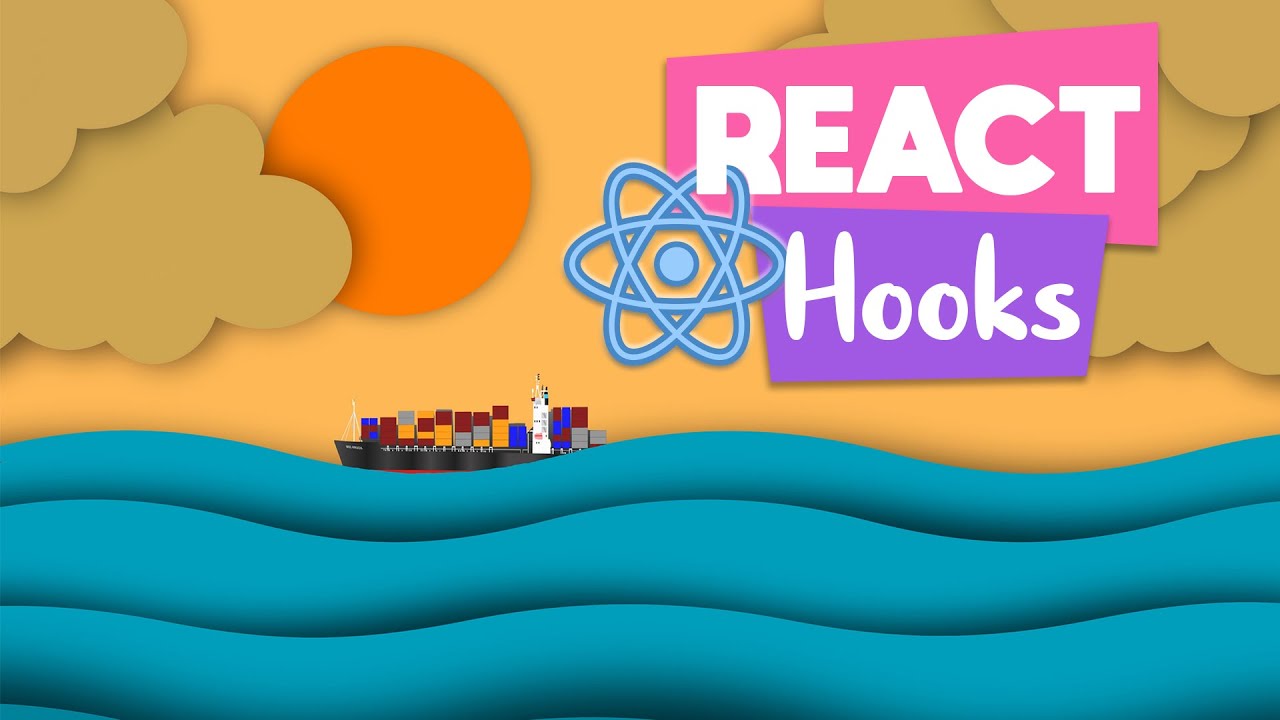
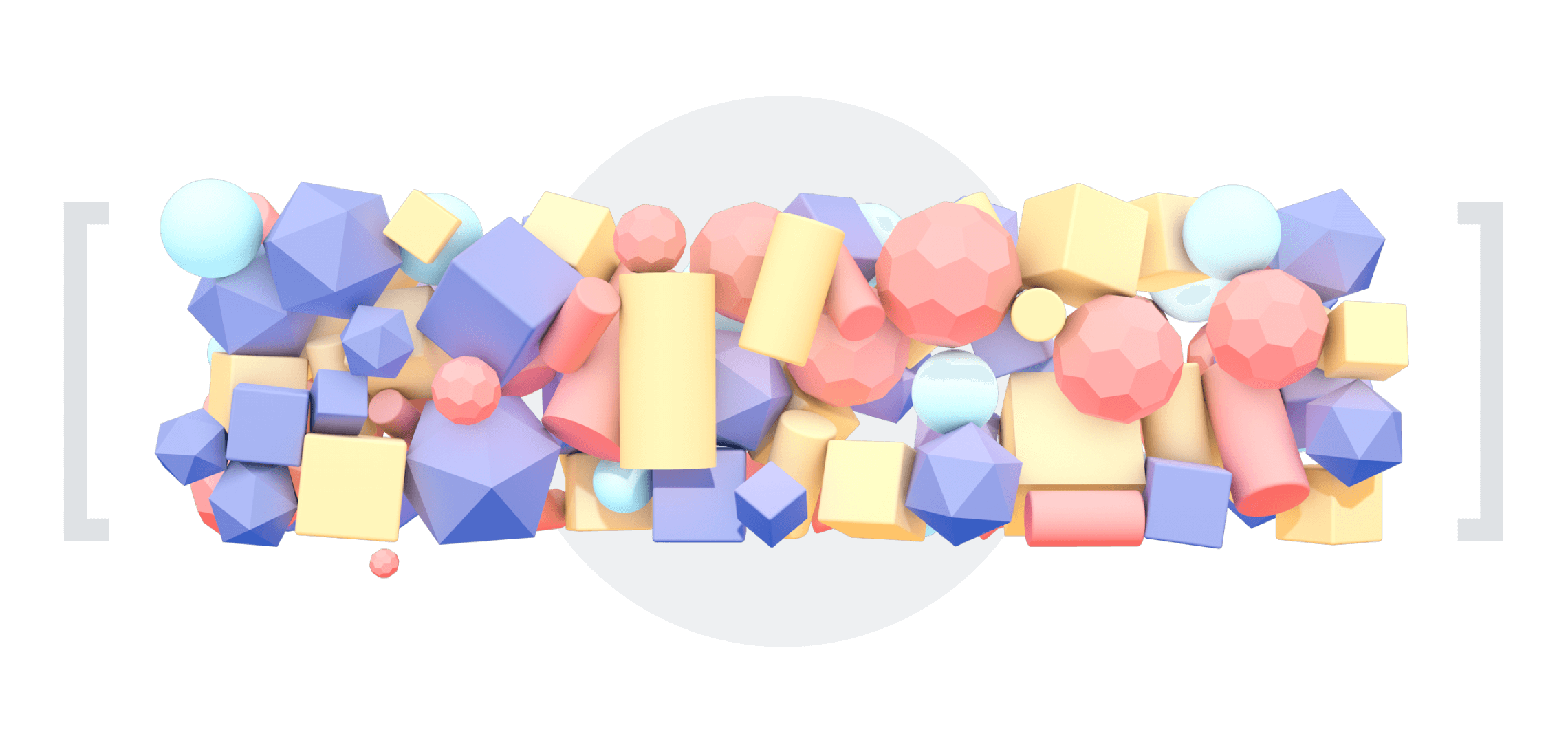
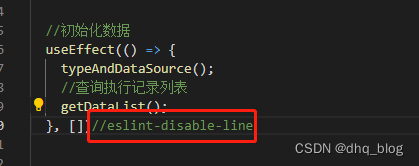
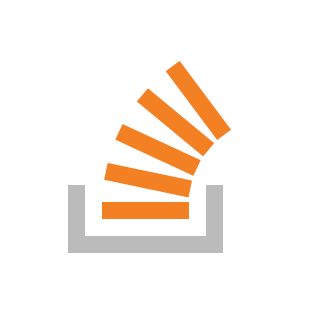

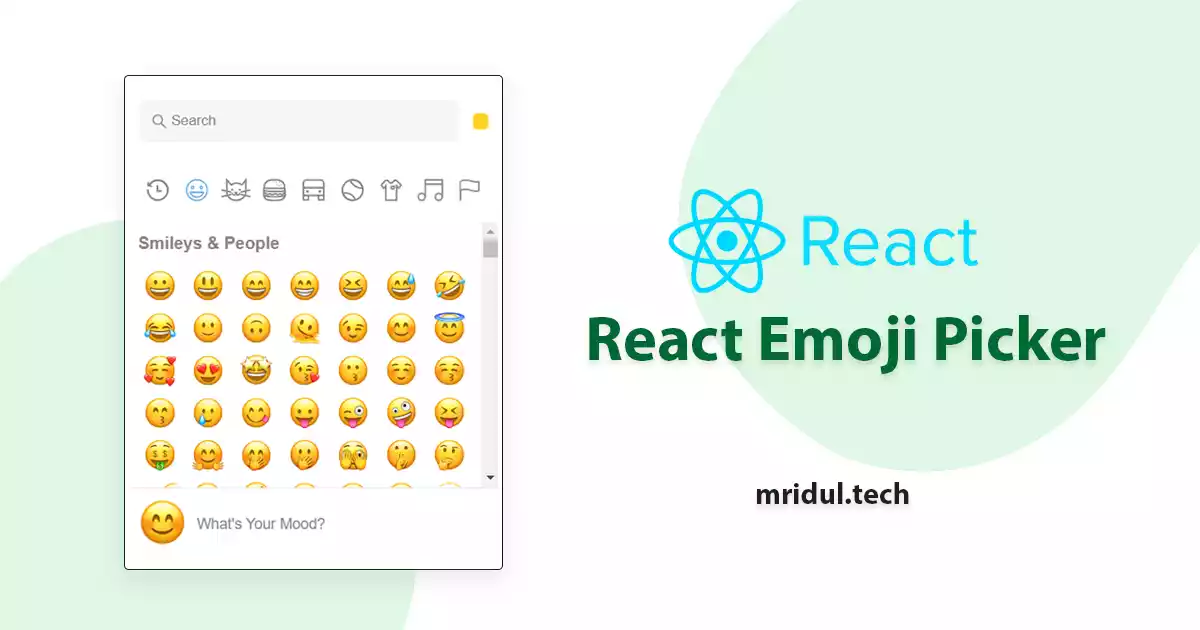

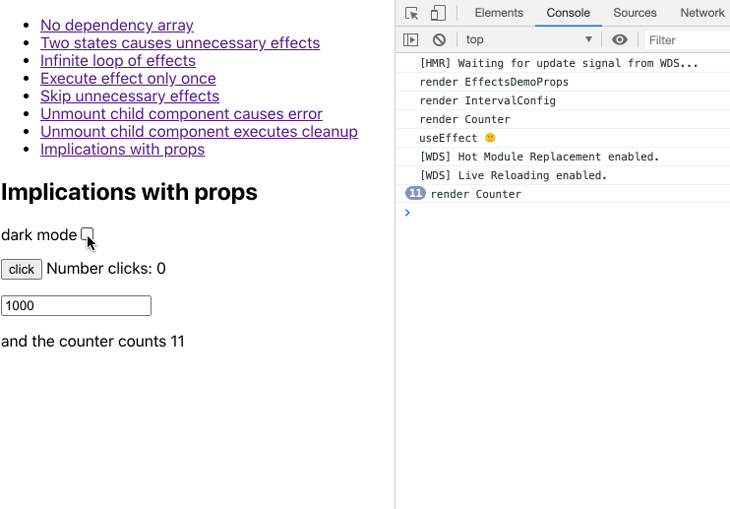

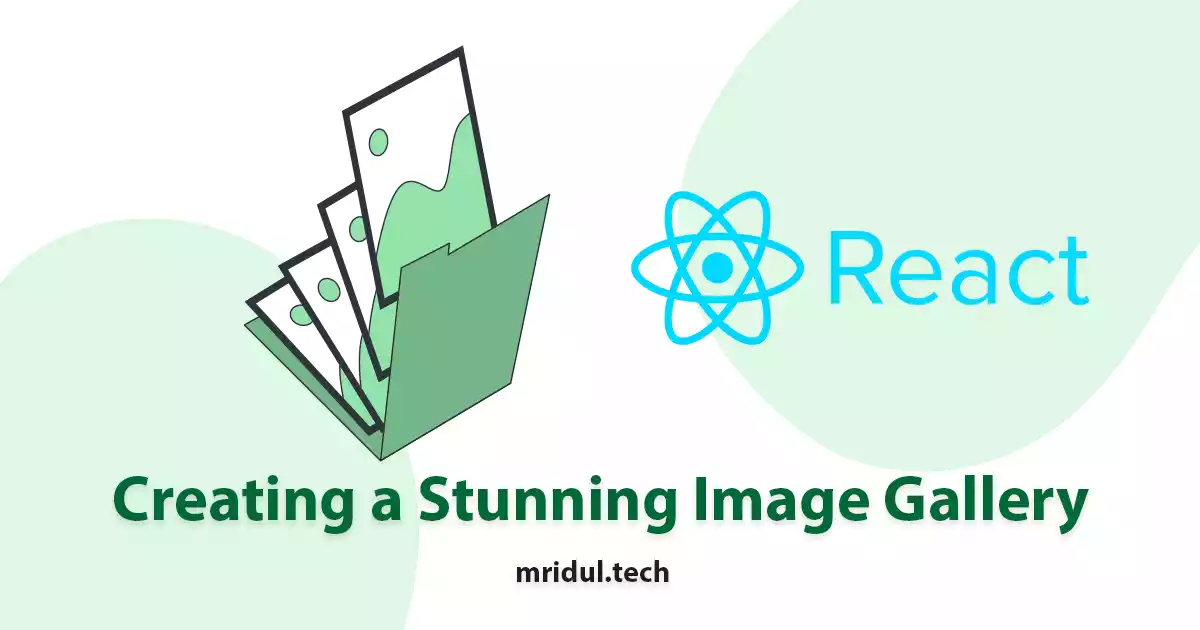

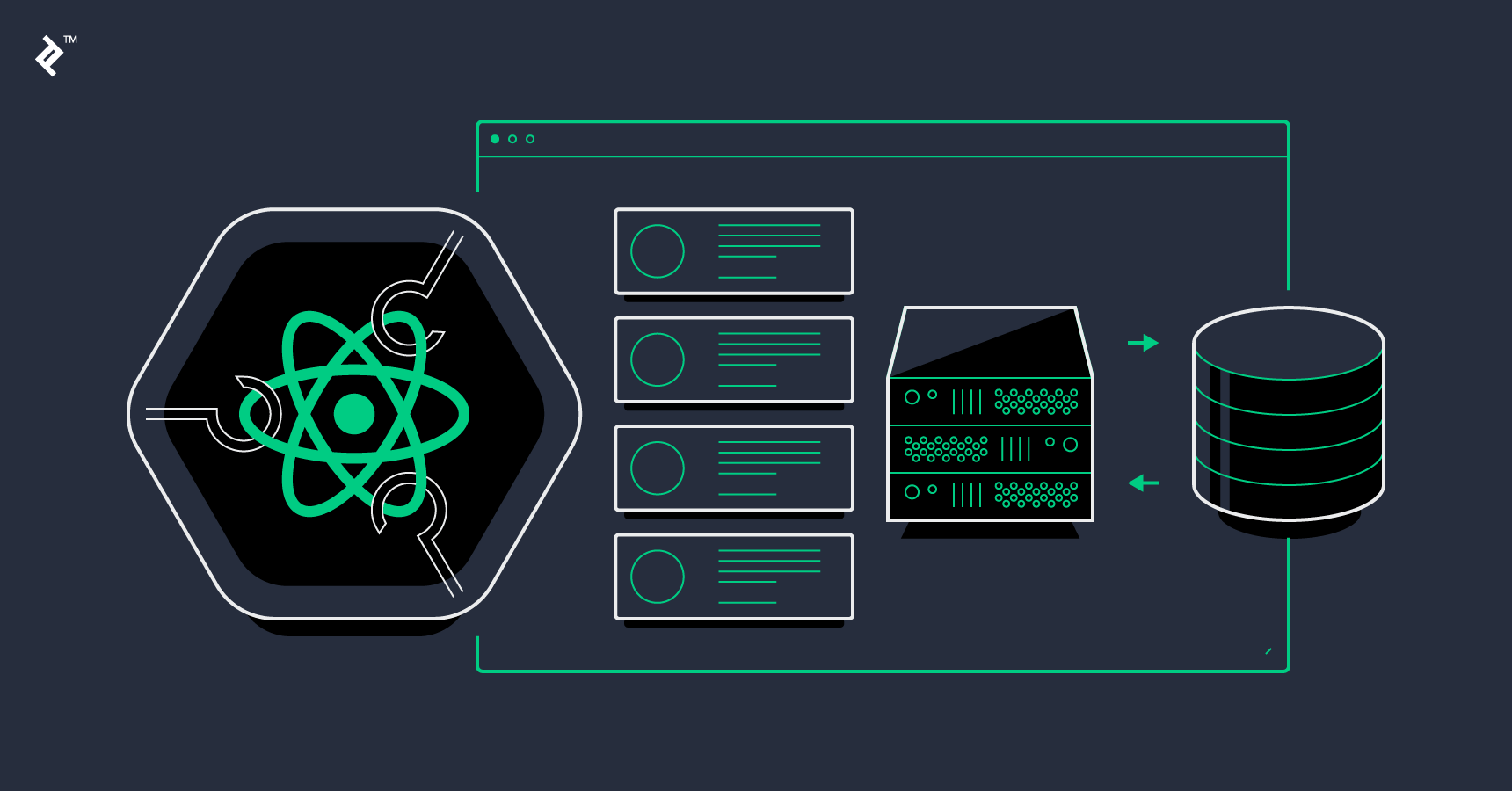
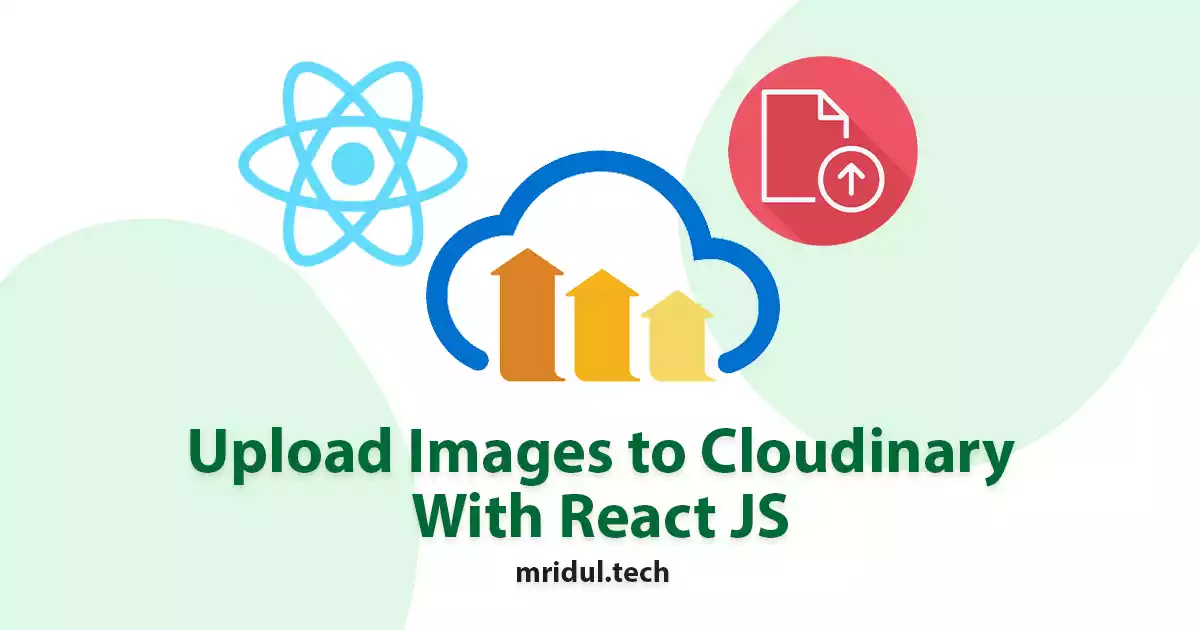

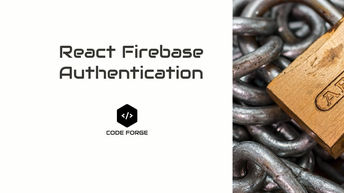



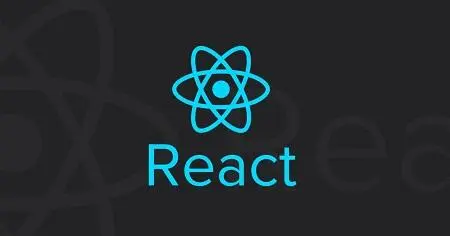



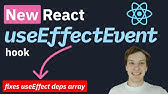

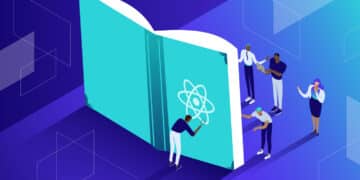

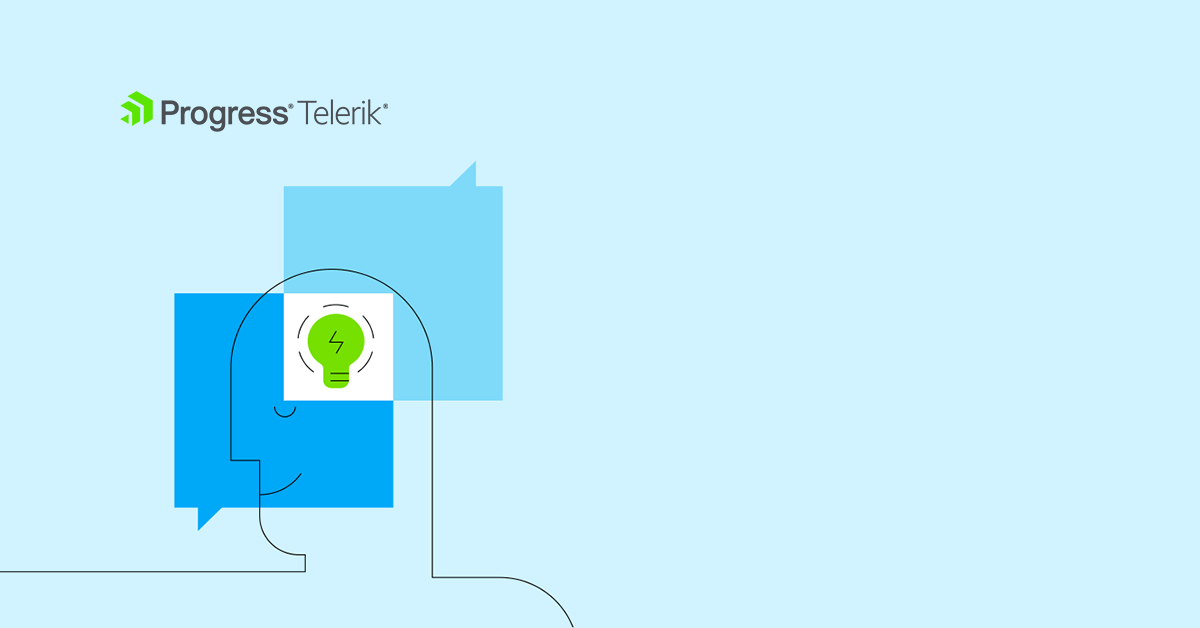
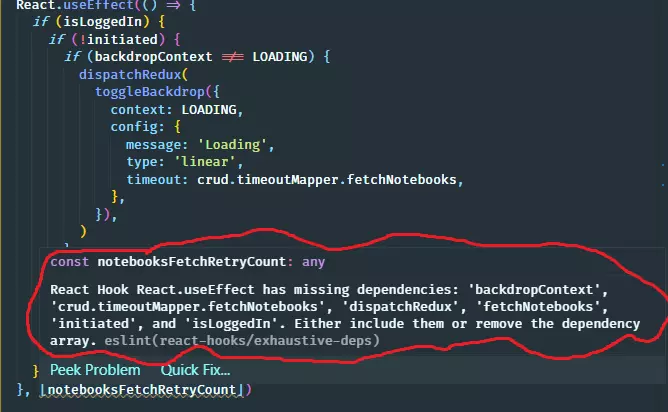
Article link: react hook useeffect has a missing dependency.
Learn more about the topic react hook useeffect has a missing dependency.
- How to fix missing dependency warning when using useEffect …
- How To Fix the “React Hook useEffect Has a Missing … – Kinsta
- React Hook useEffect has a missing dependency error [Fixed]
- How To Fix “react hook useeffect has a missing dependency”?
- Solve – React Hook useEffect has a missing dependency error.
- How to fix – react hook useEffect has missing dependencies?
- How to Fix – React Hook useEffect has a missing dependency
- Fix useEffect missing dependencies warning with useCallback …
- React Hook useEffect Has a Missing Dependency: How to Fix It