Lvalue Required As Left Operand Of Assignment
In the world of programming, errors and bugs are an inevitable part of the process. One such error that programmers often encounter is the “lvalue required as left operand of assignment” error. This error occurs when an lvalue, which stands for “left-value,” is not present on the left side of an assignment operation. In this article, we will delve into the concept of an lvalue, discuss the causes of this error, and provide solutions to fix it.
What is an lvalue in programming?
Before we dive into the error itself, it is crucial to understand what an lvalue is in programming. An lvalue refers to a value that has an address and can be assigned a new value. In simple terms, an lvalue can be thought of as a variable or an object that can appear on the left side of an assignment operation. It is important to note that an lvalue can only be modified by an assignment operator, such as the “=” operator.
Types of values in programming
In programming, there are two primary types of values: lvalues and rvalues. We have already discussed what an lvalue is, so let’s explore the concept of an rvalue. An rvalue refers to a value that does not have an address and cannot be assigned a new value. It is typically a constant or a literal value. Unlike an lvalue, an rvalue cannot appear on the left side of an assignment operation.
Understanding assignment operations
In programming languages such as C and C++, assignment operations are a fundamental concept. They involve assigning a new value to a variable or an object using the “=” operator. For example, consider the following line of code in C:
int x = 5;
In this case, the lvalue “x” on the left side of the assignment operation is assigned the rvalue “5” on the right side.
The lvalue required as left operand of assignment error
Now that we have a basic understanding of lvalues, rvalues, and assignment operations, let’s explore the “lvalue required as left operand of assignment” error. This error occurs when an rvalue is mistakenly used on the left side of an assignment operation instead of an lvalue.
Common causes of the error
There are several common causes for the “lvalue required as left operand of assignment” error. This error typically occurs when:
1. An rvalue is mistakenly used on the left side of an assignment operation.
2. A constant or literal value is used instead of a variable or an object.
3. A function or expression is used instead of a variable or an object.
How to fix the lvalue required as left operand of assignment error
Fixing the “lvalue required as left operand of assignment” error involves identifying and correcting the underlying cause. Here are some solutions to resolve this error:
1. Check the assignment operation: Ensure that you are using the correct assignment operator (“=”) and that the lvalue is present on the left side of the assignment.
2. Verify the use of variables or objects: Double-check that you are using variables or objects on the left side of the assignment, rather than constants or literals.
3. Examine functions and expressions: If you are using functions or expressions on the left side of the assignment, confirm that you are assigning the result of the operation to a variable or an object.
Avoiding the error through proper coding practices
To avoid encountering the “lvalue required as left operand of assignment” error, it is essential to follow proper coding practices. Some tips to prevent this error include:
1. Be mindful of assignment operations: Always ensure that you are using the correct syntax for assignment operations and that lvalues are present on the left side.
2. Use meaningful variable names: Assign meaningful names to your variables and objects, making it easier to identify and differentiate lvalues from rvalues.
Examples of the lvalue required as left operand of assignment error
Let’s demonstrate a few examples of the “lvalue required as left operand of assignment” error to further clarify its occurrence:
Example 1:
5 = x; // Error: rvalue (5) used on the left side of the assignment
Example 2:
int add(int a, int b) {
return a + b;
}
add(2, 3) = x; // Error: the result of the function call used on the left side of the assignment
Conclusion
The “lvalue required as left operand of assignment” error is a common issue faced by programmers when an rvalue is mistakenly used on the left side of an assignment operation. Understanding the concept of lvalues, rvalues, assignment operations, and following proper coding practices are key to avoiding and fixing this error. By being mindful of these concepts and implementing the suggested solutions, programmers can minimize the occurrence of this error and write robust code.
FAQs
1. What is “Lvalue required as left operand of assignment là gì”?
“Lvalue required as left operand of assignment là gì” is a phrase in Vietnamese that translates to “What is ‘lvalue required as left operand of assignment’ in English?” This error occurs when an lvalue is missing on the left side of an assignment operation.
2. What is “Lvalue required as left operand of assignment in C”?
“Lvalue required as left operand of assignment in C” refers to the occurrence of this error specifically in the C programming language. It indicates that an lvalue is absent on the left side of an assignment operation.
3. Can this error occur in Arduino programming?
Yes, this error can occur in Arduino programming as well, as it is based on the C++ programming language. The same principles and solutions apply to fix this error in Arduino.
4. What does “Error lvalue required” mean?
The phrase “Error lvalue required” is a concise expression that suggests that an lvalue is missing on the left side of an assignment operation. It implies that an rvalue or an incorrect expression is being used in place of an lvalue.
5. What is meant by “Lvalue required as unary ‘&’ operand”?
“Lvalue required as unary ‘&’ operand” refers to an error that occurs when the “&” operator, which is commonly used to retrieve the memory address of an lvalue, is applied to an rvalue that does not have an address.
6. What does “Lvalue required as increment operand” signify?
“Lvalue required as increment operand” signifies an error that takes place when the increment (++) operator is used on an rvalue instead of an lvalue. The increment operator is designed to modify the value of an lvalue, not an rvalue.
7. What is the difference between lvalue and rvalue in C++?
In C++, an lvalue is a value that has an address, can be assigned a new value, and can appear on the left side of an assignment operation. On the other hand, an rvalue is a value that does not have an address, cannot be assigned a new value, and cannot appear on the left side of an assignment operation.
8. What is the significance of lvalue required as left operand of assignment?
The significance of the “lvalue required as left operand of assignment” error lies in its indication of incorrect usage of variables or objects on the left side of an assignment operation. By identifying and fixing this error, programmers can ensure that their code functions as intended.
Lvalue Required As Left Operand Of Assignment
What Does Lvalue Required As Left Operand Of Assignment Mean In C++?
In C++, the error message “lvalue required as left operand of assignment” is one that is encountered by many programmers, especially when they are new to the language. This error message is often confusing and may leave programmers wondering what exactly went wrong. In this article, we will delve into the meaning of this error message and explain the concept of lvalues in C++.
Understanding lvalues and rvalues:
Before explaining the error message, it is important to grasp the concept of lvalues and rvalues in C++. In simple terms, an lvalue refers to an object that can appear on the left side of an assignment expression, whereas an rvalue is an object that can only appear on the right side of an assignment expression.
Lvalues are typically variables or memory locations that have a specific name and storage location. They are objects that can be modified and have a definite place in memory. On the other hand, rvalues are temporary objects or values that cannot be modified directly. They only have a temporary existence during an expression evaluation.
For example, consider the following code snippet:
int x = 5;
int y = x + 3;
In this example, ‘x’ is an lvalue because it has a specific memory location and can be modified. It can be used on the left side of an assignment expression, such as when assigning a value to ‘y’. On the other hand, ‘3’ is an rvalue because it is a temporary value used in the expression evaluation.
The ‘lvalue required as left operand of assignment’ error:
Now that we understand the difference between lvalues and rvalues, let’s explore why this error message occurs. This error occurs when an rvalue is used as the left operand of an assignment operator (=), which is strictly reserved for lvalues.
Consider the following code snippet that triggers the error:
5 = x;
In this example, the constant value ‘5’ is an rvalue, and it is being used as the left operand of the assignment operator. Since an rvalue cannot be modified directly, this statement is invalid, and the compiler throws the error message ‘lvalue required as left operand of assignment’.
How to fix the error:
To fix the ‘lvalue required as left operand of assignment’ error, you need to ensure that the left operand of the assignment operator is an lvalue. In other words, you need to use a variable or an object that can be modified.
For example, consider the revised code snippet:
int x = 5;
int y = 6;
x = y;
In this updated code, ‘x’ is an lvalue, ‘y’ is an lvalue, and the assignment is valid. ‘x’ receives the value of ‘y’, which is a valid operation.
FAQs about ‘lvalue required as left operand of assignment’:
Q: Can I use an rvalue as the left operand of an assignment operator?
A: No, an rvalue cannot be used as the left operand of an assignment operator. The left operand should always be an lvalue.
Q: Why is this restriction imposed in C++?
A: The restriction where the left operand of an assignment operator should be an lvalue is imposed to prevent accidental assignment to temporary values or values that cannot be modified directly.
Q: What are some common examples of rvalues?
A: Constants, literals, and temporary objects are some common examples of rvalues.
Q: Can I assign an rvalue to an lvalue reference?
A: Yes, C++ allows you to bind an rvalue to an lvalue reference, but this is a different concept than using an rvalue as the left operand of an assignment operator.
Q: Can I assign an lvalue to an rvalue reference?
A: No, C++ does not allow you to assign an lvalue to an rvalue reference. An rvalue reference is designed to bind to temporary objects only.
In conclusion, understanding the concept of lvalues and rvalues is crucial to comprehend the error message ‘lvalue required as left operand of assignment’ in C++. By ensuring that the left operand of an assignment operator is always an lvalue, you can avoid this error and write clean and error-free code.
What Does Lvalue Required Mean In C++?
In C++, an lvalue is a term that refers to an object that occupies some identifiable location in memory. It is typically an expression to which we can assign a value. The term “lvalue” stands for “left value,” indicating that lvalues can generally appear on the left side of an assignment expression.
The phrase “lvalue required” appears as an error message in C++ when a particular syntax or semantic rule requires an lvalue, but an rvalue (temporary value that does not have a persistent memory location) is provided instead. This error message is designed to help programmers identify potential issues in their code and ensure that correct and safe assignments are made.
To understand this concept more clearly, let’s examine some common scenarios where the “lvalue required” error can occur:
1. Assignment to an rvalue:
Error: lvalue required as left operand of assignment
“`
int x = 5;
10 = x;
“`
Here, the second line fails to compile because the constant value “10” is an rvalue and cannot be assigned a value. The error message suggests that an lvalue is needed on the left side of the assignment operator.
2. Modification of a constant:
Error: assignment of read-only location
“`
const int y = 7;
y = 8;
“`
In this case, the constant variable “y” is read-only and cannot be modified. The compiler generates the “lvalue required” error to indicate that it is not possible to assign a new value to this constant.
3. Invalid assignment in a conditional statement:
Error: lvalue required as left operand of comma operator
“`
int a = 10;
int b = 5;
(a + b) = 15;
“`
Here, the expression `(a + b)` evaluates to an rvalue and cannot serve as the left operand of the assignment operator. The error message indicates that an lvalue is required in this context.
4. Incorrect usage of the address-of operator:
Error: lvalue required as unary ‘&’ operand
“`
int z = 42;
&42;
“`
In this case, the address-of operator is attempting to take the address of the constant value “42,” which is an rvalue. The error message alerts the programmer that the operand must be an lvalue for the address-of operator to work correctly.
FAQs:
Q: Can you provide examples of valid lvalues?
A: Certainly! Variables created with proper declarations are lvalues. For example:
“`
int x;
int& ref = x;
ref = 10; // x is an lvalue
“`
Q: Is it possible to convert an rvalue to an lvalue?
A: No, you cannot directly convert an rvalue to an lvalue. However, in some cases, you can create a reference to an rvalue and operate on it. This is possible using rvalue references in C++11 and later versions.
Q: How can I fix the “lvalue required” error?
A: To resolve the error, you need to ensure that you are providing an lvalue where it is expected. Verify that constants are not being modified, proper variables are used in assignments, and the address-of operator is correctly applied to lvalues.
Q: Are all variables lvalues?
A: No, not all variables are lvalues. Some are rvalues, such as the result of an arithmetic operation or a literal value. Only variables that represent memory locations (lvalues) can be assigned new values.
Q: Are there any instances where an rvalue is required?
A: Yes, there are cases where an rvalue is expected. For instance, when passing arguments to a move constructor or move assignment operator, rvalues are required to efficiently transfer ownership of resources.
In conclusion, the “lvalue required” error in C++ arises when an lvalue is expected, but an rvalue is provided instead. Understanding the difference between lvalues (objects with persistent memory locations) and rvalues (temporary values) is crucial to identify and resolve such errors. By keeping in mind the scenarios discussed above and referring to the FAQs section, programmers can gain a better understanding of this concept and write more robust and error-free C++ code.
Keywords searched by users: lvalue required as left operand of assignment Lvalue required as left operand of assignment là gì, Lvalue required as left operand of assignment in C, Lvalue required as left operand of assignment arduino, Error lvalue required, Lvalue required as unary ‘&’ operand, Lvalue required as increment operand, Lvalue and rvalue C++, What is lvalue
Categories: Top 68 Lvalue Required As Left Operand Of Assignment
See more here: nhanvietluanvan.com
Lvalue Required As Left Operand Of Assignment Là Gì
Introduction
In the world of programming, encountering errors is a common occurrence, and one such error that developers often come across is the “lvalue required as left operand of assignment” error. This error message might appear cryptic to beginners, but it is essentially a protective mechanism of the programming language to ensure that certain expectations are met. In this article, we will delve into the meaning of this error message in the context of programming, its causes, and provide insights on how to resolve it. Additionally, a FAQs section will address some common queries related to this topic.
Understanding the Error Message
The error message “lvalue required as left operand of assignment” prompts when you attempt to assign a value to an expression that does not satisfy the criteria of being an lvalue. In simple terms, an lvalue is an expression that can be assigned a value. It refers to something that resides in the memory and can be manipulated by the assignment operator (=).
The concept of an lvalue is derived from the assignment operator itself. When we use the assignment operator, the left operand, often called the lvalue, receives the value of the right operand. For example, in the statement “x = 5,” x is an lvalue. On the other hand, the value being assigned, which is 5 in this case, is termed the rvalue.
Causes of the Error
The error message “lvalue required as left operand of assignment” occurs when you attempt to assign a value to an rvalue, thereby violating the rule that specifies an lvalue should be used as the left operand of the assignment operator. This error typically arises due to one of the following scenarios:
1. Trying to assign a value to a constant or literal: Constants and literals are not lvalues, as they cannot be modified. Assigning a value to them will result in the aforementioned error.
2. Incorrect use of operators: Certain operators, such as the ternary conditional operator, ?:, can lead to this error. It is important to understand the specific requirements of each operator and ensure that they are being used correctly.
3. Missing reference or pointer: When working with references or pointers, forgetting to dereference or reference them properly can cause this error.
4. Incorrect declaration of variables: Declaring a variable with an incorrect data type or failing to initialize it can also lead to this error. It is important to ensure that variables are properly declared and initialized before attempting to use them.
Resolving the Error
To resolve the “lvalue required as left operand of assignment” error, it is crucial to identify the specific cause of the error. Here are some steps you can take to rectify this error:
1. Ensure variables are properly declared and initialized: Check the declaration and initialization of variables to confirm that they are correct. Make sure the data type matches the intended usage and that the variables are initialized before being used.
2. Verify correct usage of operators: Go through the code and examine the usage of operators, especially when using ternary conditional operators or composite assignment operators. Confirm that the operators are being used appropriately and that they are expecting lvalues rather than rvalues.
3. Check for missing references or pointers: If the error is related to references or pointers, double-check that they have been correctly referenced or dereferenced in the code. Ensure that the proper syntax for referencing or dereferencing has been used.
4. Review any constants or literals: If you are attempting to assign a value to a constant or literal, be aware that they cannot be modified. Consider using variables instead, whenever applicable.
FAQs
Q1. Can an lvalue be an rvalue?
No, an lvalue cannot be an rvalue. An rvalue is an expression that yields a temporary value, while an lvalue represents something that resides in the memory and allows modification.
Q2. Why does assigning to an rvalue cause an error?
Assigning to an rvalue causes an error because the assignment operator expects an lvalue, which represents a memory location that can be assigned a value. Since rvalues do not have a memory location, they cannot receive a value.
Q3. How can I differentiate between lvalues and rvalues in my code?
Lvalues often include variables, objects, or expressions that can be modified, such as function return values. On the other hand, rvalues typically consist of literals, constants, or temporary results of expressions.
Q4. Are there any exceptions when it comes to assigning to an rvalue?
No, assigning to an rvalue is not permitted in most programming languages. However, some languages might provide specific mechanisms or syntax to achieve similar effects.
Conclusion
The error message “lvalue required as left operand of assignment” is encountered when attempting to assign a value to an expression that does not qualify as an lvalue. By understanding the concept of lvalues and their role in the assignment process, developers can identify the specific causes of this error and rectify them accordingly. Remember to double-check variable declarations, operator usage, and references or pointers to resolve this error effectively. By following these guidelines, you can navigate through this error and enhance your programming skills.
Lvalue Required As Left Operand Of Assignment In C
In the programming language C, there is a strict rule that states an “lvalue” is required as the left operand of an assignment. This rule can sometimes be confusing for beginners, but understanding it is crucial for writing correct and efficient C programs. In this article, we will delve into the concept of lvalues, discuss their importance, and explore some frequently asked questions related to this topic.
Understanding Lvalues and Rvalues
To grasp the concept of lvalues, it is essential to differentiate them from “rvalues.” An lvalue refers to an expression that identifies an object or a function capable of returning an object. On the other hand, an rvalue denotes any expression that can only produce a value but not be assigned to directly. In simple terms, lvalues are expressions that can appear on the left side of an assignment operator, while rvalues can only appear on the right side.
The Importance of Lvalues
The requirement for an lvalue as the left operand of an assignment is vital for maintaining the well-defined behavior of C programs. By ensuring that assignment operations work only on lvalues, C prevents accidental assignments to constants, literals, or expressions that should not be modified. This rule helps in preventing errors and promotes cleaner and more reliable code.
Scenarios Where Lvalues Are Required
In C, lvalues are needed in various situations, including:
1. Assignments: As mentioned before, lvalues must be used as the left operand in assignment statements. For instance:
“`c
int var = 10; // Valid assignment
10 = var; // Error: Lvalue required
“`
2. Increment/Decrement Operators: The increment and decrement operators (++, –) operate on lvalues. For example:
“`c
int count = 5;
count++; // Valid: incrementing an lvalue
10++; // Error: Lvalue required
“`
3. Address-of Operator (&): The address-of operator requires an lvalue as its operand, as it needs to determine the address of a particular object. For instance:
“`c
int var = 10;
int* ptr = &var; // Valid: Taking the address of an lvalue
int* ptr = &10; // Error: Lvalue required
“`
FAQs
1. Q: Why does C require lvalues as the left operand of an assignment?
A: Requiring an lvalue as the left operand ensures that assignment operations are applied only to mutable entities. This prevents accidental modifications of constants or literals, promoting program correctness and robustness.
2. Q: What happens if I use an rvalue as the left operand of an assignment?
A: If you attempt to use an rvalue as the left operand of an assignment, the compiler will generate an error, indicating that an lvalue is required. This prevents accidental incorrect assignments, ensuring the stability of your code.
3. Q: Can I assign an rvalue to an lvalue?
A: While direct assignment of rvalues to lvalues is not allowed, you can indirectly assign them by using pointers. By assigning the address of an rvalue to a pointer variable, you can indirectly modify the value it represents.
4. Q: How can I identify if an expression is an lvalue or an rvalue?
A: In general, the rule of thumb is that if an expression refers to a mutable entity (such as a variable or an object), it is an lvalue. If it only produces a value but cannot be assigned to, it is an rvalue.
5. Q: Are there any exceptions to the lvalue requirement rule?
A: Yes, there are a few exceptions where an rvalue can be used as the left operand. One such case is when using the comma operator (,) to separate expressions. However, these exceptions are relatively rare and should not be relied upon for general assignments.
In conclusion, the lvalue requirement as the left operand of an assignment in C is a crucial rule that ensures program correctness and stability. By understanding the concept of lvalues and differentiating them from rvalues, developers can write cleaner and more reliable code. Remember to always use lvalues when necessary and refer to the provided FAQs section for additional clarification. Happy coding!
Images related to the topic lvalue required as left operand of assignment
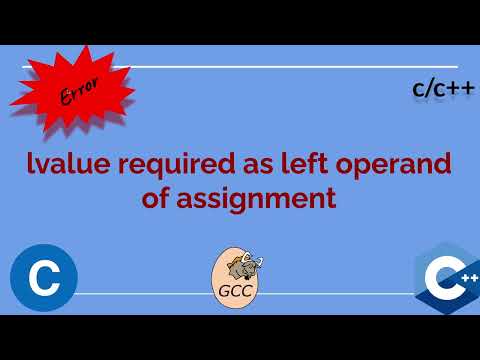
Found 14 images related to lvalue required as left operand of assignment theme
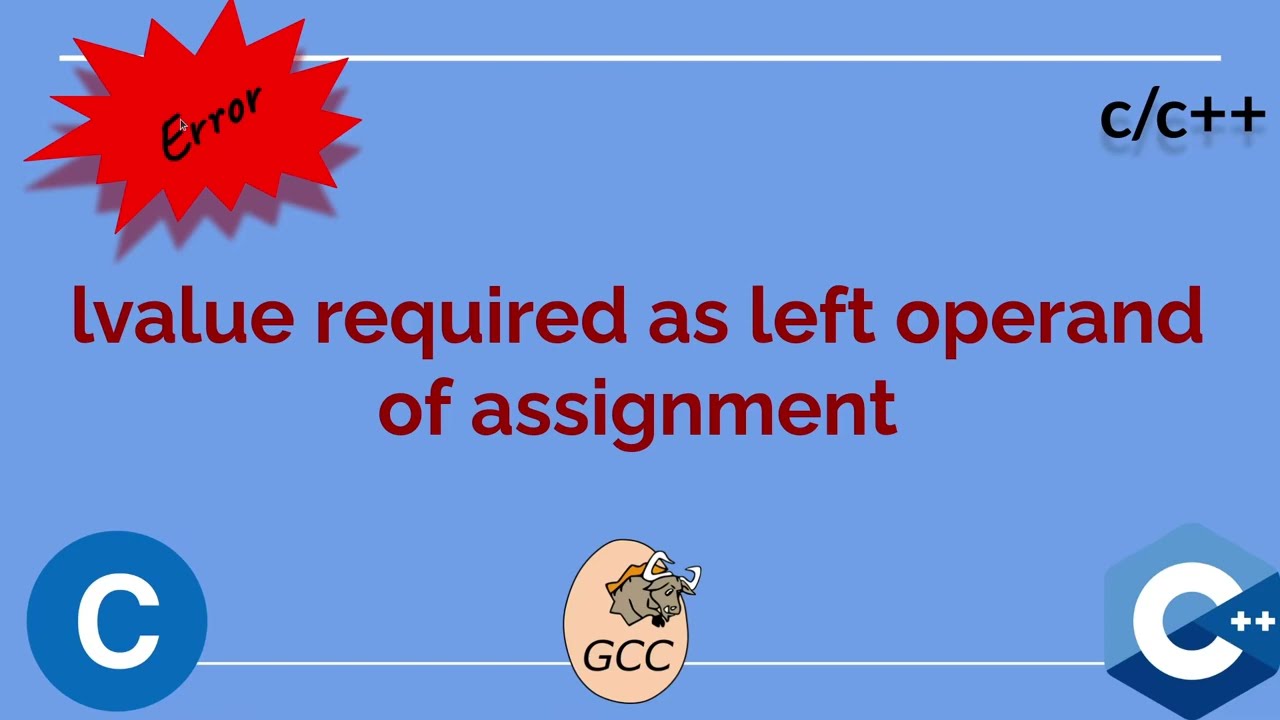

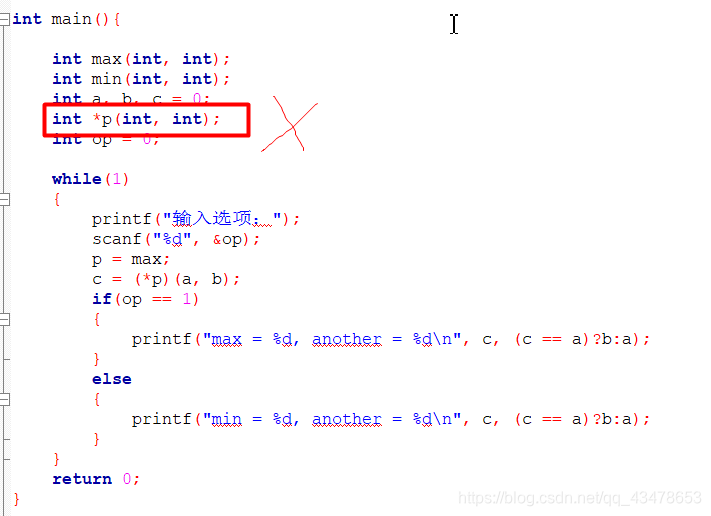
![FIX] C++ | How to fix: Turbo C | Lvalue required error | String/Char array[] assignment error - YouTube Fix] C++ | How To Fix: Turbo C | Lvalue Required Error | String/Char Array[] Assignment Error - Youtube](https://i.ytimg.com/vi/63azFnAiolw/mqdefault.jpg)
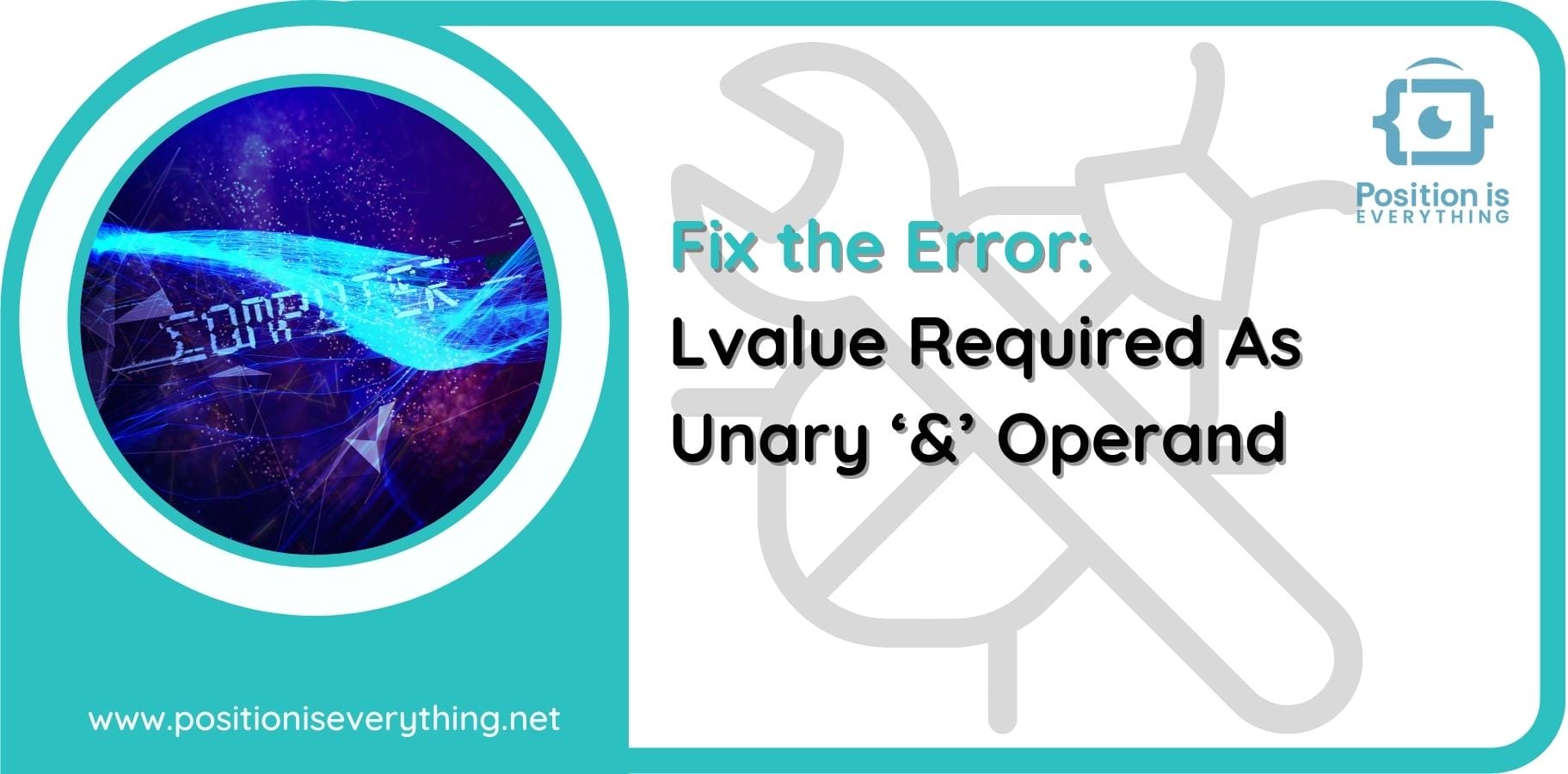
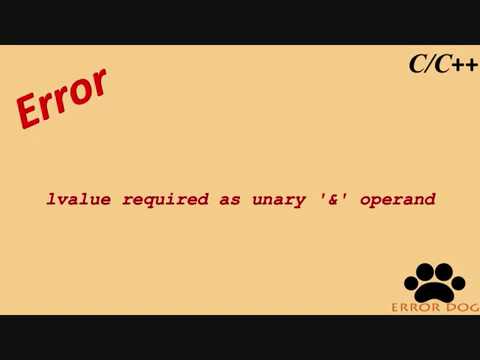

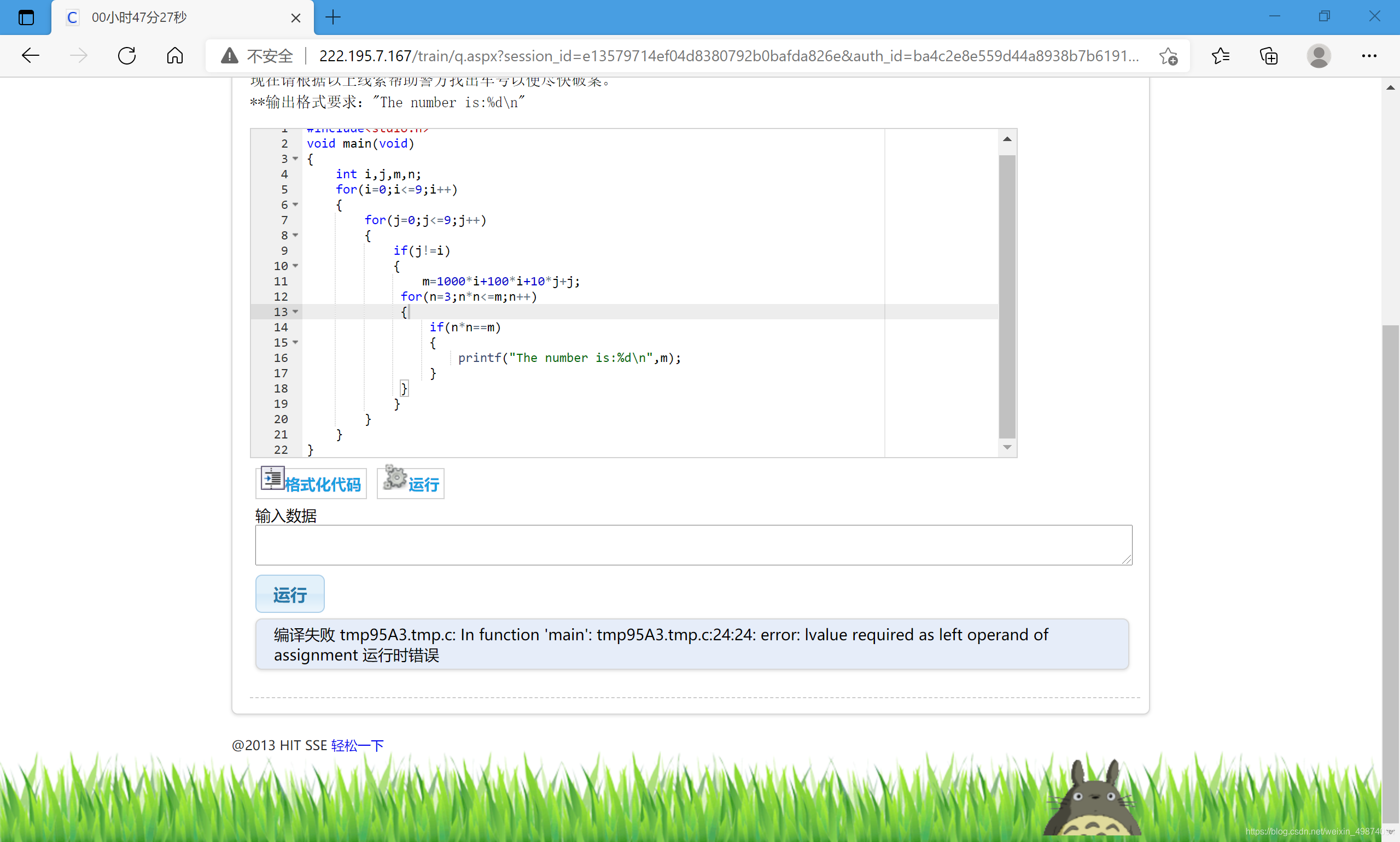
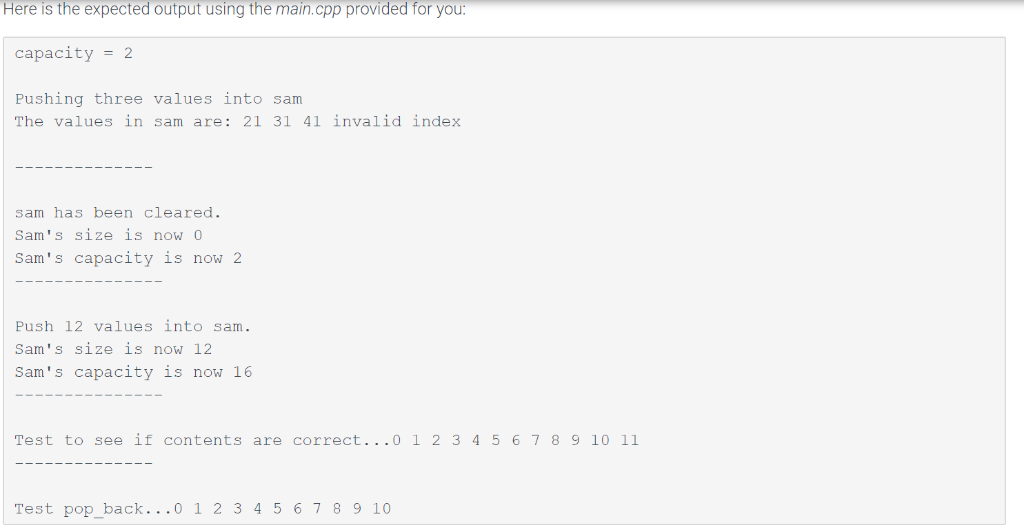
![C语言--[Error] lvalue required as left operand of assignment --的一个解决方案。_字母的小草的博客-CSDN博客 C语言--[Error] Lvalue Required As Left Operand Of Assignment --的一个解决方案。_字母的小草的博客-Csdn博客](https://img-blog.csdnimg.cn/5119f0ec6f024a2c8d6b832a81c96920.png)
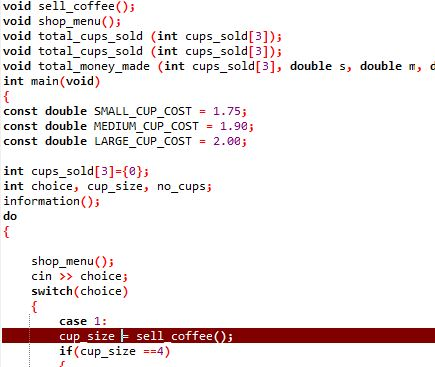
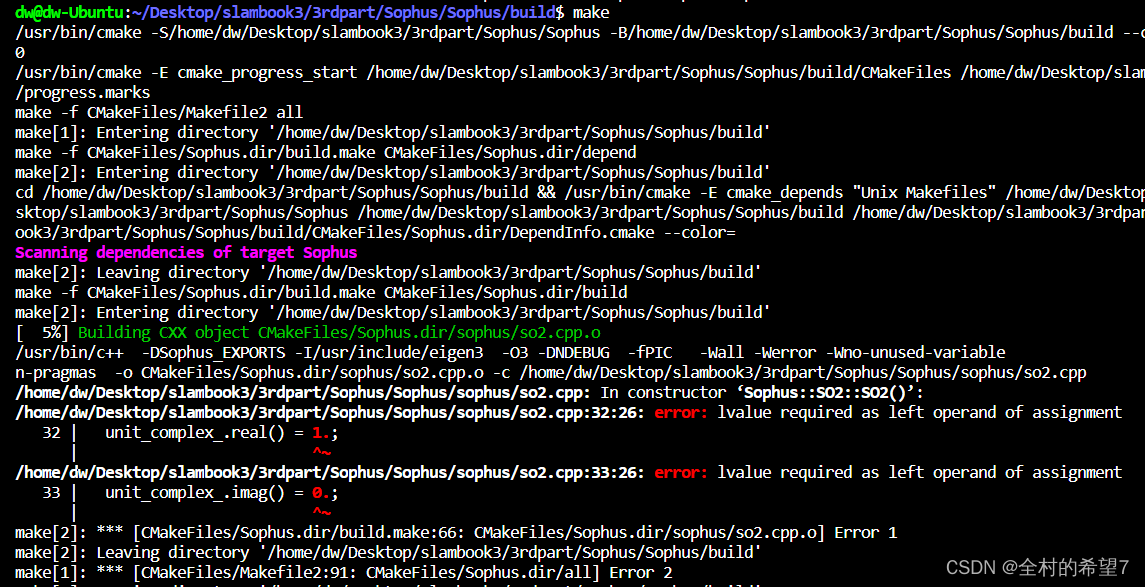
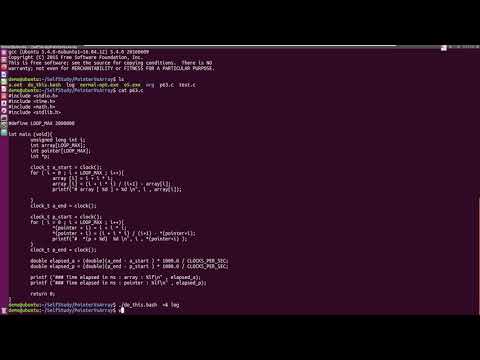

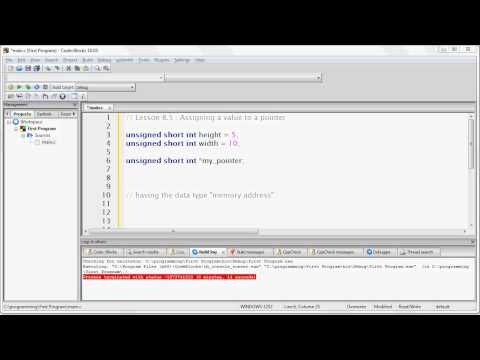
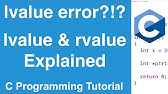


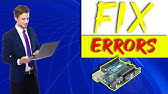
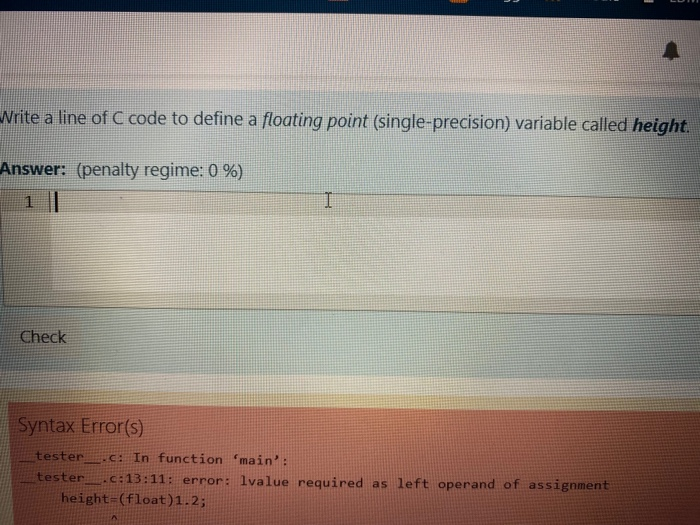
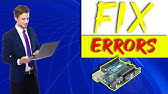


Article link: lvalue required as left operand of assignment.
Learn more about the topic lvalue required as left operand of assignment.
- Assignment operators – IBM
- lvalue required as left operand of assignment error when …
- C++ ERROR: lvalue Required as Left Operand of Assignment
- lvalue required as left operand of assignment – C Việt
- lvalue required as left operand of assignment – Stack Overflow
- Else without IF and L-Value Required Error in C – GeeksforGeeks
- Lvalue Required as Left Operand of Assignment: Effective Solutions
- Solved What is an Ivalue? point Something that names a box. – Chegg
- Solve error: lvalue required as left operand of assignment
- [Solved] lvalue required as left operand of assignment
- lvalue required as left operand of assignment – Arduino Forum
- lvalue required as left operand of assignment dịch
- How to solve the error, ‘lvalue required as left operand … – Quora
See more: nhanvietluanvan.com/luat-hoc