Module ‘Datetime’ Has No Attribute ‘Strptime’
The ‘AttributeError: module ‘datetime’ has no attribute ‘strptime” error occurs when attempting to use the ‘strptime’ function from the ‘datetime’ module in Python. This error indicates that the ‘strptime’ function is not recognized within the ‘datetime’ module, leading to a failure in executing any code that relies on this function.
Explanation of the ‘strptime’ Function in the ‘datetime’ Module
The ‘strptime’ function in the ‘datetime’ module is used to convert a string representation of a date and time into a datetime object. It takes two parameters – the string to be converted and a format string that specifies the expected format of the input string.
Reasons for the Attribution Error ‘module ‘datetime’ has no attribute ‘strptime”
1. Incorrect Import Statement for the ‘datetime’ Module: One possible reason for this error is importing the ‘datetime’ module incorrectly. It is important to ensure that the module is imported properly before using any of its functions.
2. Incorrect Usage of the ‘strptime’ Function: Another reason for the error could be a mistake in how the ‘strptime’ function is being used. It is important to pass the correct parameters to the function and ensure that the input string matches the specified format.
3. Python Version Incompatibility: The ‘strptime’ function is available in certain versions of Python. If you are using an older version of Python, it is possible that the function is not supported, resulting in the attribute error.
Solutions for Fixing the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime”
1. Importing the ‘datetime’ Module Correctly: To fix this error, ensure that the ‘datetime’ module is imported correctly at the beginning of your code. The correct import statement for the ‘datetime’ module is:
“`
import datetime
“`
2. Using the ‘strptime’ Function Correctly: Double-check the usage of the ‘strptime’ function and ensure that the parameters are passed correctly. Make sure that the input string matches the specified format string.
3. Checking for Python Version Compatibility: Verify the version of Python you are using. If you are using an older version that does not support the ‘strptime’ function, consider upgrading to a newer version that does.
Useful Tips and Considerations when Dealing with the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime”
1. Verifying the Installed Python Version: To check the version of Python installed on your system, open a terminal or command prompt and enter the following command:
“`
python –version
“`
2. Checking the Available ‘datetime’ Module Functions: Use the dir() function to see a list of available functions in the ‘datetime’ module. This can help identify any potential issues with the ‘strptime’ function not being recognized.
3. Utilizing Alternative Time Parsing Methods: If the ‘strptime’ function is not available or not suitable for your specific use case, consider using alternative methods for parsing dates and times in Python, such as regular expressions or third-party libraries like dateutil.
4. Seeking Help from the Python Community: If you are still unable to resolve the attribute error, consider seeking help from the Python community. Online forums and communities such as Stack Overflow can provide valuable insights and solutions to specific coding problems.
Conclusion of the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime” Error Explanation
In conclusion, the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime” error occurs when attempting to use the ‘strptime’ function in the ‘datetime’ module. This error can be fixed by ensuring the correct import statement for the module, using the function correctly, and checking for Python version compatibility. Additionally, there are useful tips and alternative methods available for dealing with this error. If difficulties persist, seeking help from the Python community can provide further assistance.
FAQs
Q: What is the purpose of the ‘strptime’ function in the ‘datetime’ module?
A: The ‘strptime’ function is used to convert a string representation of a date and time into a datetime object in Python.
Q: How can I fix the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime” error?
A: To fix this error, make sure the ‘datetime’ module is imported correctly, use the ‘strptime’ function with the correct parameters, and ensure compatibility with the Python version you are using.
Q: Are there alternative methods for parsing dates and times in Python?
A: Yes, there are alternative methods such as using regular expressions or third-party libraries like dateutil that can be used for parsing dates and times in Python.
Q: Where can I seek help if I am unable to fix the ‘AttributeError: module ‘datetime’ has no attribute ‘strptime” error?
A: If you are still facing difficulties in resolving the error, you can seek help from the Python community, online forums, or platforms like Stack Overflow for assistance and solutions.
Q: How can I check the version of Python installed on my system?
A: To check the version of Python installed on your system, open a terminal or command prompt and enter the command “python –version”. This will display the installed Python version.
Datetime Module Has No Attribute Strptime
Keywords searched by users: module ‘datetime’ has no attribute ‘strptime’ Strptime Python, Python print datetime, Strptime() argument 1 must be str, not datetime datetime, Python datetime to timestamp, Python strptime stackoverflow, Convert string to datetime Python, Python datetime add days, Must be str not datetime date
Categories: Top 30 Module ‘Datetime’ Has No Attribute ‘Strptime’
See more here: nhanvietluanvan.com
Strptime Python
Introduction:
Working with date and time is a fundamental aspect of many programming tasks. Python, being renowned for its ease of use and extensive support for various libraries, offers powerful tools to handle date and time operations. One such tool is the strptime function, which is part of the datetime module. In this article, we will delve deeper into how to utilize the strptime function in Python, its syntax, capabilities, and provide some common FAQs to enhance your understanding.
Understanding the strptime Function:
The strptime function in Python is used to convert a string representation of a date and/or time into a Python datetime object. The function name, “strptime,” stands for “string parse time,” indicating its purpose to parse date and time strings.
Syntax:
The general syntax for the strptime function is as follows:
“`
datetime.datetime.strptime(date_string, format)
“`
Where:
– `date_string`: The string representing the date and/or time that you want to convert
– `format`: A formatting string that specifies the expected format of the `date_string`
The `format` string follows a specific format code syntax that determines how the strptime function interprets the given `date_string`. Different format codes can be combined to build a format string that matches the input date format precisely.
Let’s explore a few examples to gain a better understanding of how the strptime function works.
Example 1: Converting a String to a Datetime Object
“`python
from datetime import datetime
date_string = “2022-03-15”
format = “%Y-%m-%d”
parsed_date = datetime.strptime(date_string, format)
print(parsed_date)
“`
Output:
“`
2022-03-15 00:00:00
“`
In this example, we create a string `date_string` representing the date “March 15th, 2022.” We use the `%Y-%m-%d` format code to match the string’s format. The strptime function then converts the string to a datetime object, and the output represents the parsed date and time.
Example 2: Parsing Dates with Time
“`python
from datetime import datetime
date_string = “2022-03-15 09:30:45”
format = “%Y-%m-%d %H:%M:%S”
parsed_date = datetime.strptime(date_string, format)
print(parsed_date)
“`
Output:
“`
2022-03-15 09:30:45
“`
In this example, we extend the previous example by including the time information in the `date_string`. By extending the `format` string to include `%H:%M:%S` format codes, we ensure that the strptime function parses both the date and time correctly.
Common Format Codes:
The `format` string can include numerous format codes to match specific parts of the `date_string`. Here are some frequently used format codes:
– `%Y`: Year with a century as a decimal number (e.g., “2022”)
– `%m`: Month as a zero-padded decimal number (e.g., “01” for January)
– `%d`: Day of the month as a zero-padded decimal number (e.g., “01” for the 1st day)
– `%H`: Hour (24-hour clock) as a zero-padded decimal number (e.g., “08” for 8 AM)
– `%M`: Minute as a zero-padded decimal number (e.g., “05” for 5 minutes)
– `%S`: Second as a zero-padded decimal number (e.g., “10” for 10 seconds)
These are just a few examples, and the datetime module documentation provides an extensive list of all available format codes.
FAQs (Frequently Asked Questions):
Q1. Can the strptime function handle different localized date formats?
A1. Yes, the strptime function can handle different localized date formats. You can specify the appropriate format using the corresponding format codes for the desired locale.
Q2. What happens if the format does not match the given date_string?
A2. If the `date_string` does not match the specified `format`, a ValueError will be raised. Therefore, it’s crucial to ensure the `date_string` follows the specified format.
Q3. How can I extract specific date and time components from a parsed datetime object?
A3. Once you have a parsed datetime object, you can use various methods provided by the datetime module to extract specific date and time components. For example, you can use `parsed_date.year` to get the year, `parsed_date.month` for the month, and so on.
Q4. Is the strptime function case-sensitive when interpreting the `date_string` format?
A4. Yes, the format codes provided in the `format` string are case-sensitive. For example, `%M` represents minutes, while `%m` represents months.
Q5. Can I use the strptime function to parse time intervals or durations?
A5. No, the strptime function is only useful for parsing specific date and time representations. To handle durations or intervals, you may need to use other functions or utilize appropriate date and time libraries.
Conclusion:
The strptime function in Python’s datetime module provides a powerful tool to convert string representations of dates and times to usable datetime objects. By understanding the syntax and using appropriate format codes, you can parse date and time strings accurately. This functionality proves invaluable when dealing with various data sources that provide time information in string format. However, it’s crucial to ensure the `date_string` matches the specified `format` to avoid any potential errors. Remember to consult the official Python documentation for a complete list of format codes and their usage.
Python Print Datetime
The datetime module in Python provides classes for manipulating dates and times. It offers a convenient way to work with datetime-related operations, including formatting and parsing dates, calculations, and comparisons. To access the datetime module, we need to import it into our Python script using the following statement:
“`python
import datetime
“`
Once the module is imported, we can utilize its various classes and methods. To display the current date and time in English, we can use the `datetime` class, which offers several attributes and methods to extract specific components of the date and time.
To obtain the current date and time, we first create an instance of the `datetime` class. We can achieve this by calling the `now()` method of the class as shown below:
“`python
current_datetime = datetime.datetime.now()
“`
Now that we have the current date and time, we can access the individual components, such as the year, month, day, hour, minute, and second. These components can be obtained using the respective attributes of the `datetime` object.
“`python
year = current_datetime.year
month = current_datetime.month
day = current_datetime.day
hour = current_datetime.hour
minute = current_datetime.minute
second = current_datetime.second
“`
With the individual components in hand, we can format them in the desired way. The most common formatting requirement is to display the date and time in a specific pattern. To achieve this, we use the `strftime()` method available within the `datetime` class. This method takes a formatting string as an argument and returns a formatted string representing the datetime object.
For example, to print the current date and time in the format `Month Day, Year Hour:Minute AM/PM`, we can use the following code:
“`python
formatted_datetime = current_datetime.strftime(“%B %d, %Y %I:%M %p”)
print(formatted_datetime)
“`
The `%B` represents the full month name, `%d` represents the day of the month (padded with zeros if necessary), `%Y` represents the four-digit year, `%I` represents the hour (12-hour clock), `%M` represents the minute, and `%p` represents the AM/PM indicator.
Running the above code will output something like `February 05, 2022 08:30 AM`, representing the current date and time in the desired English format.
Now that we have covered the basics of printing the datetime in English, let’s address some frequently asked questions related to this topic.
#### FAQs
1. **How can I print the current date without the time?**
To print only the current date without the time, you can use the `strftime` method with the formatting string `%Y-%m-%d`. Example: `current_datetime.strftime(“%Y-%m-%d”)`.
2. **What if I want to display the time in the 24-hour format?**
If you prefer to print the time in the 24-hour format, you need to modify the formatting string accordingly. Instead of `%I` for the hour, you should use `%H`. Example: `current_datetime.strftime(“%Y-%m-%d %H:%M:%S”)`.
3. **Can I print the current year or month separately?**
Yes, you can print the current year or month separately using the respective attributes of the `datetime` object. Example: `year = current_datetime.year` or `month = current_datetime.month`.
4. **Is it possible to display the day of the week as well?**
Absolutely. You can utilize the `%A` directive in the formatting string to obtain the full name of the day of the week. Example: `current_datetime.strftime(“%A”)`.
5. **Can I customize the formatting further?**
Certainly! Python’s datetime module provides a variety of directives that you can include in the formatting string. Feel free to refer to the official Python documentation to explore all the available options.
Printing the datetime in English using Python is a simple yet essential task that can enhance the readability and usability of your programs. By leveraging the datetime module and its various functionalities, you can easily format and display dates and times in numerous desired formats. By understanding the different formatting options and the associated directives, you can customize the output according to your specific requirements.
Images related to the topic module ‘datetime’ has no attribute ‘strptime’
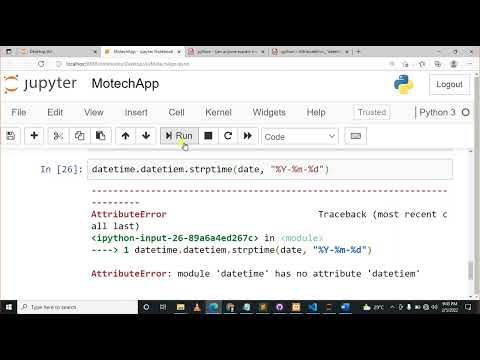
Found 31 images related to module ‘datetime’ has no attribute ‘strptime’ theme
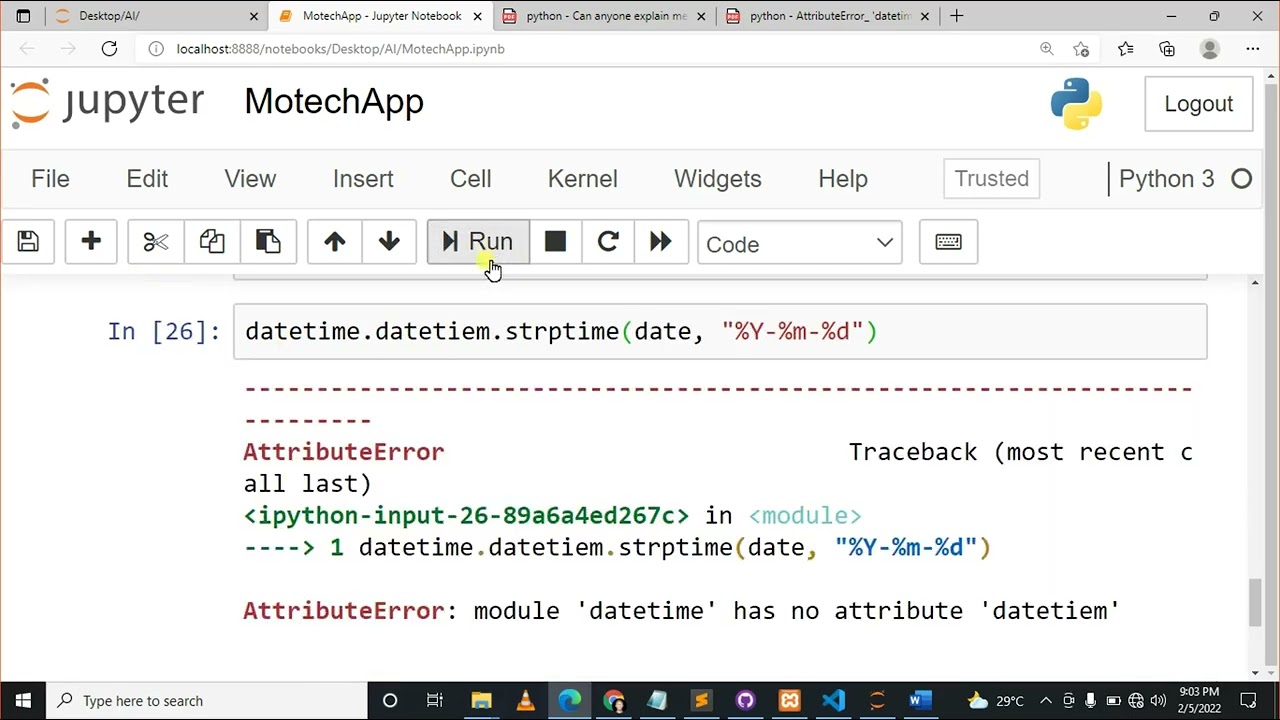
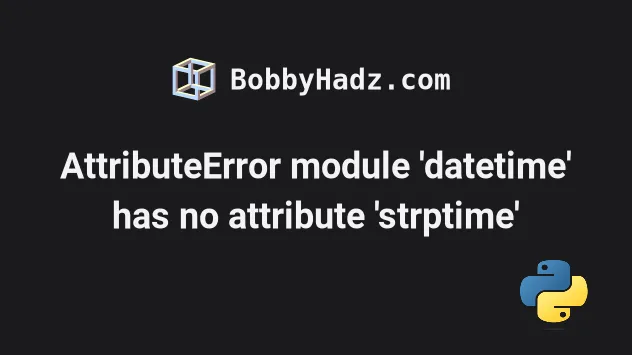
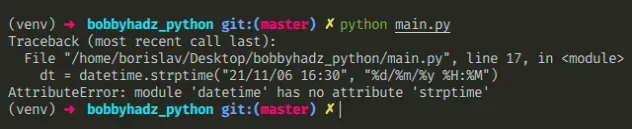
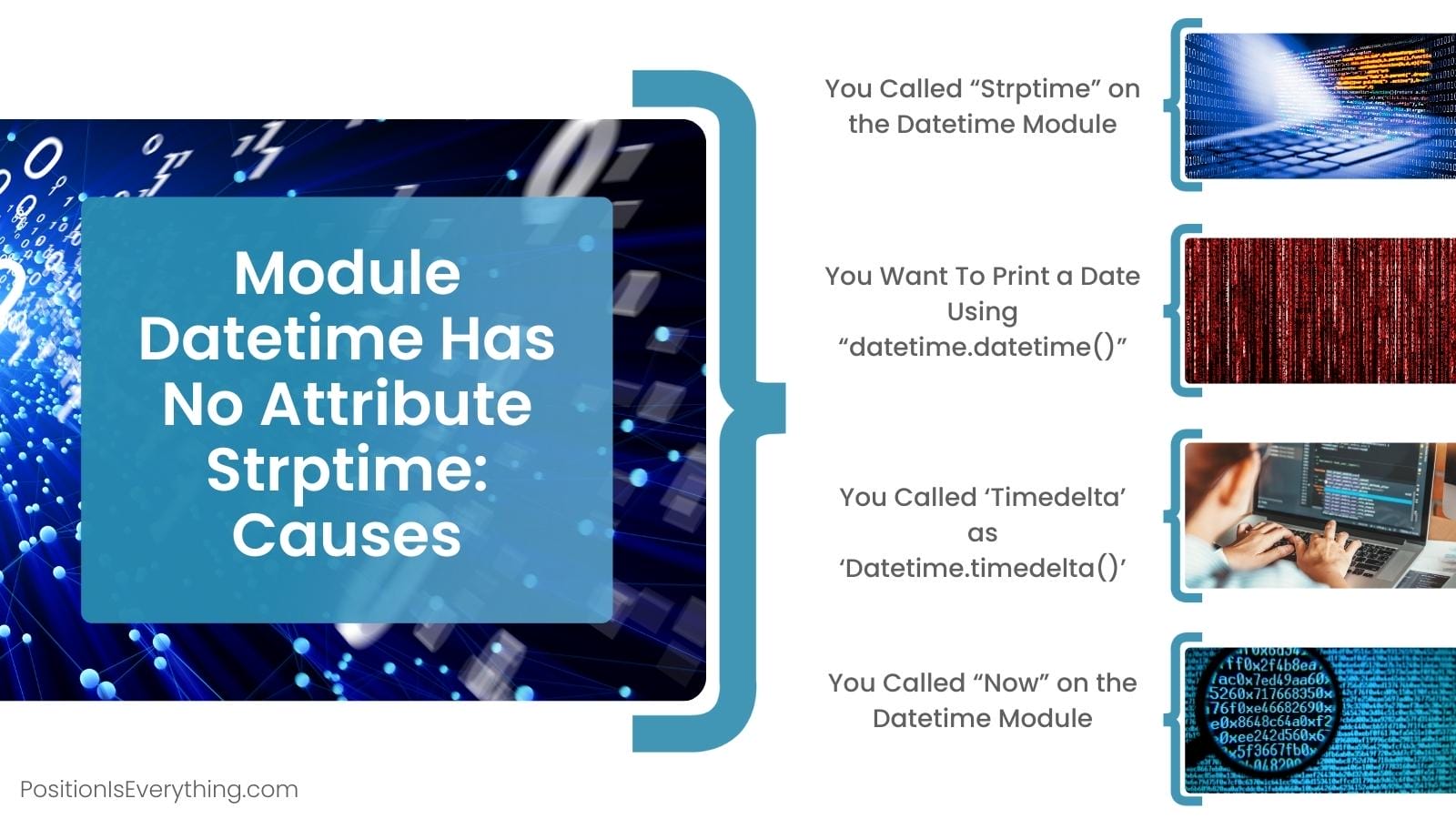



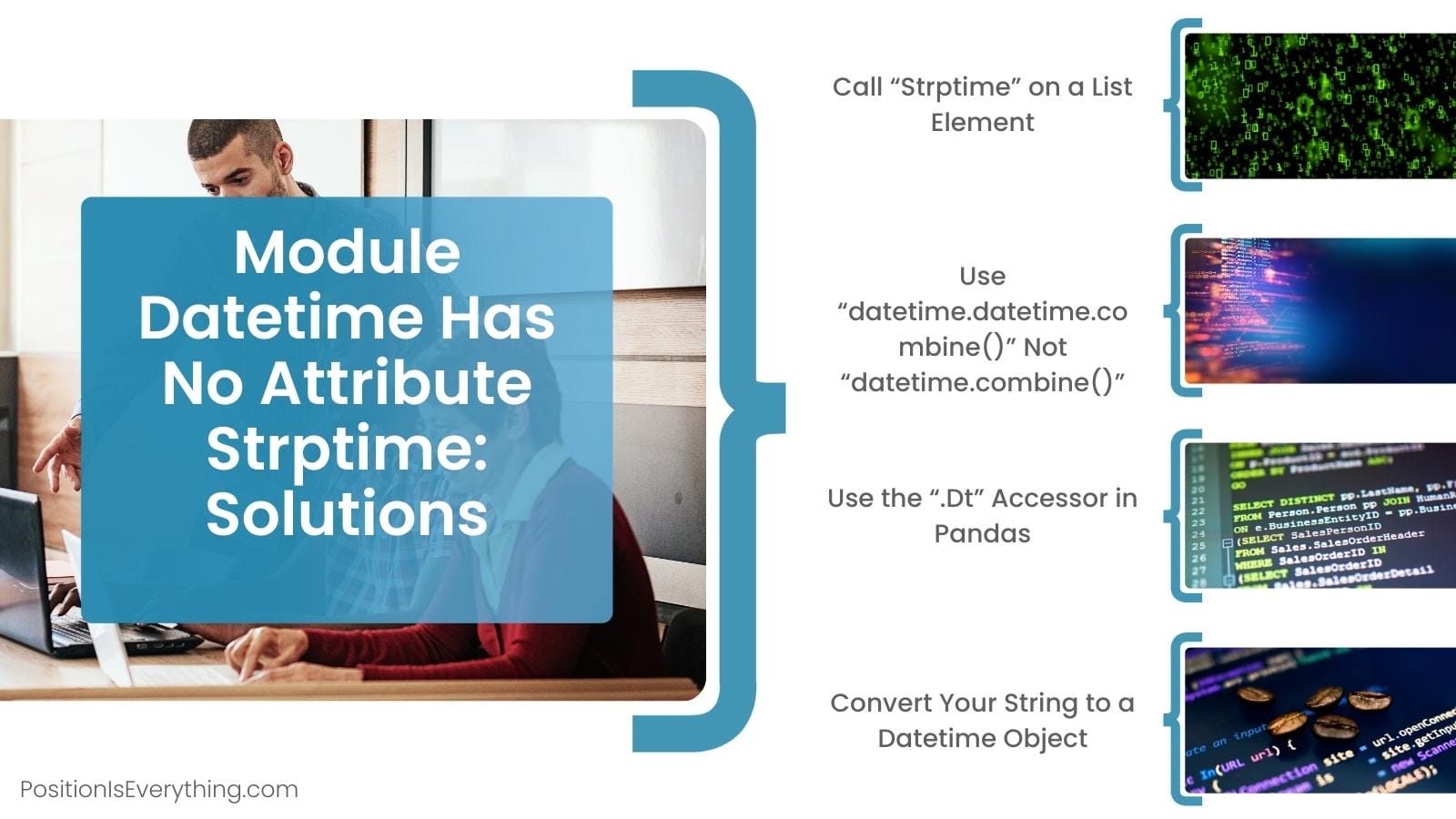
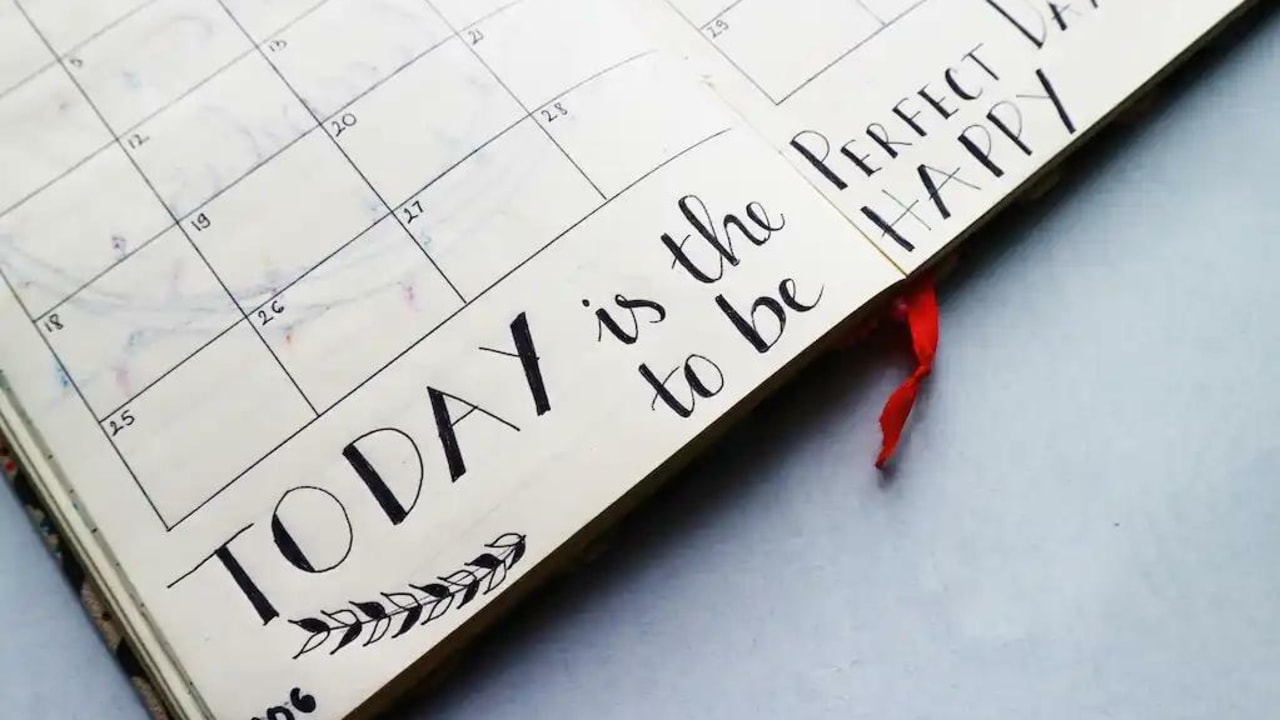

![Attributeerror: series object has no attribute strftime [SOLVED] Attributeerror: Series Object Has No Attribute Strftime [Solved]](https://itsourcecode.com/wp-content/uploads/2023/03/Attributeerror-series-object-has-no-attribute-strftime.png)


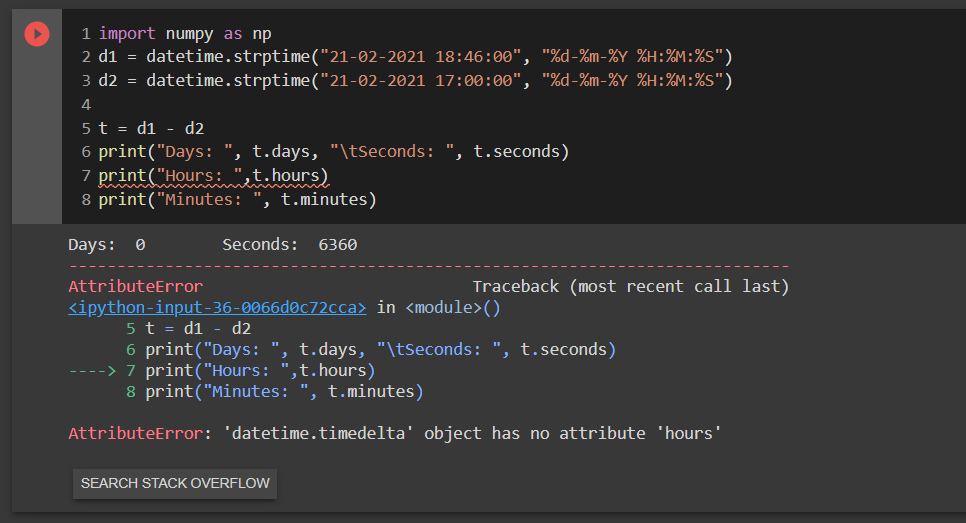

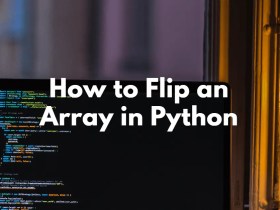
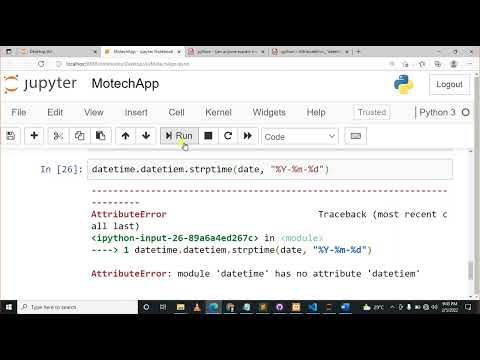
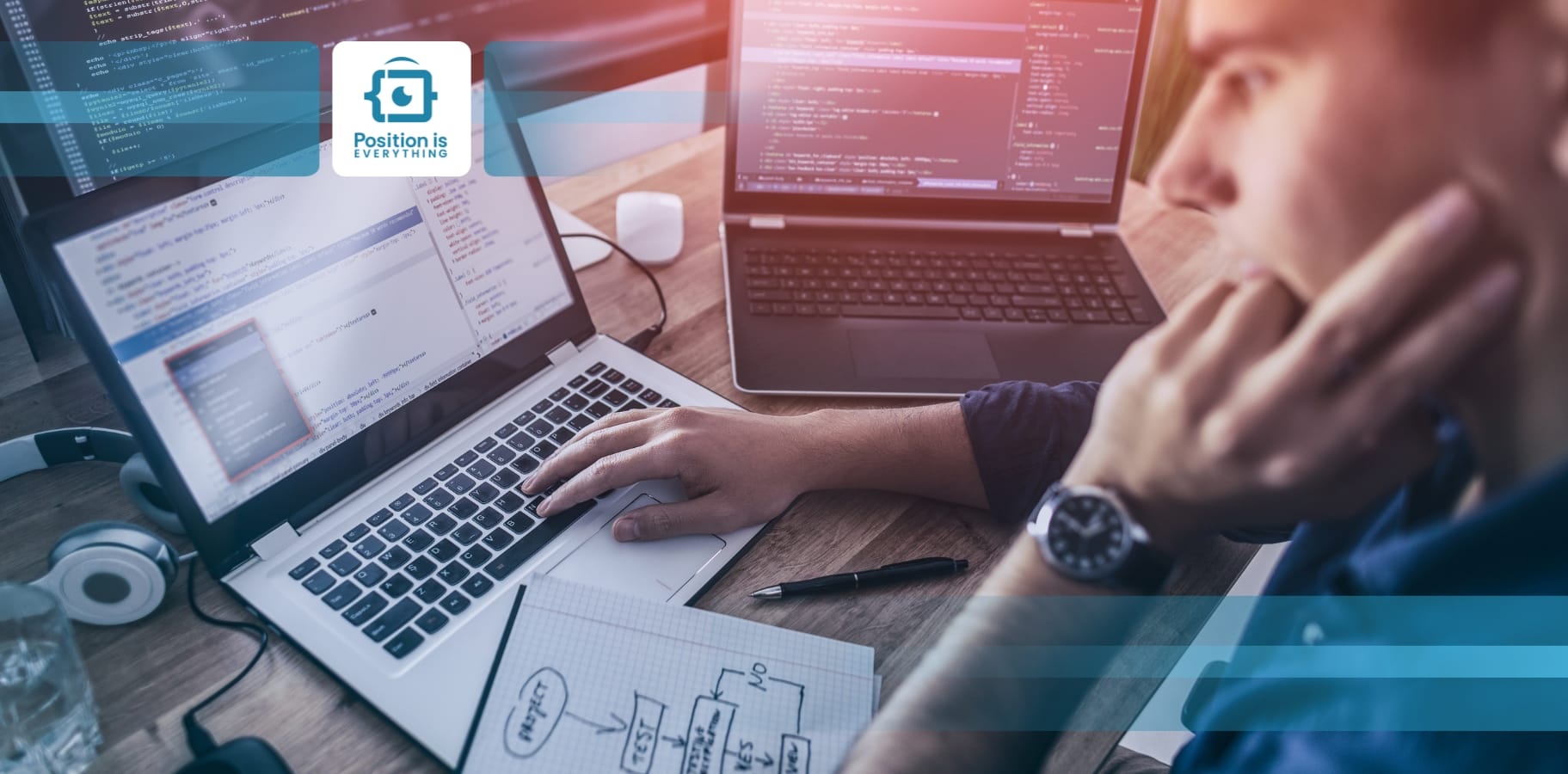
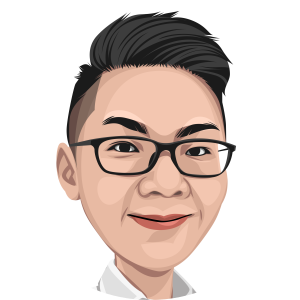
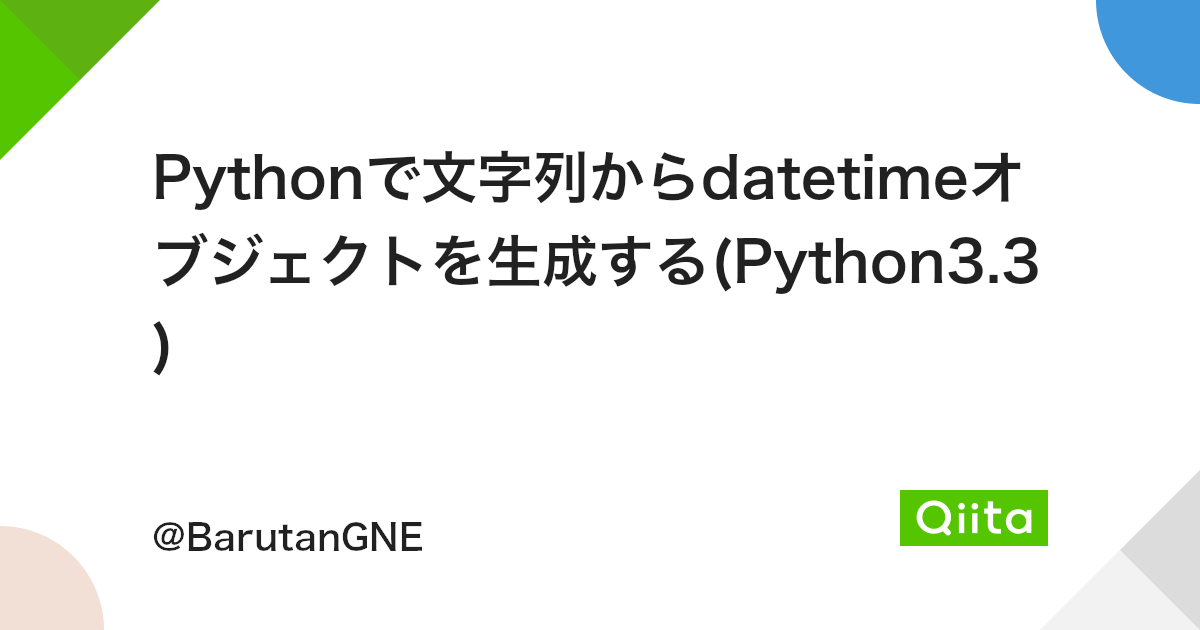

![General] 天數相差· Issue #304 · coding-coworking-club/python-2020-spring · GitHub General] 天數相差· Issue #304 · Coding-Coworking-Club/Python-2020-Spring · Github](https://user-images.githubusercontent.com/36917812/79625528-4a323480-815c-11ea-85c8-a760f386b1f1.png)
Article link: module ‘datetime’ has no attribute ‘strptime’.
Learn more about the topic module ‘datetime’ has no attribute ‘strptime’.
- AttributeError: ‘datetime’ module has no attribute ‘strptime’
- AttributeError module ‘datetime’ has no attribute ‘strptime’
- module datetime has no attribute strptime ( Solved )
- module ‘datetime’ has no attribute ‘strptime’ – sebhastian
- Fix “AttributeError: module ‘DateTime’ has no attribute ‘strptime …
- module ‘datetime’ has no attribute ‘strptime’ – Statistics Globe
- Module Datetime Has No Attribute Strptime: 8 Solutions for You!
- How to Fix AttributeError: module ‘datetime’ has no attribute …
- ‘module’ object has no attribute ‘strptime’ then how to resolve it?