Jsx Expressions Must Have One Parent Element
In the world of React.js, JSX expressions play a vital role in creating dynamic and interactive user interfaces. JSX, which stands for JavaScript XML, allows developers to write HTML-like syntax within JavaScript code. This seamless integration of HTML and JavaScript makes it easier to build and manage web applications.
However, one important rule that developers must follow when working with JSX expressions is that every expression must have one parent element. This means that all JSX expressions should be wrapped within a single container element. In this article, we will explore the reasons behind this rule, the consequences of not following it, and some techniques to work around it.
Definition of JSX Expressions
JSX expressions are a combination of HTML-like tags and JavaScript code. They allow developers to write declarative views and components in a more concise and expressive manner. Here’s an example of a JSX expression:
“`jsx
const element =
;
“`
In this example, the JSX expression creates a `div` element with the text “Hello, World!”.
Nested Elements in JSX
One of the powerful features of JSX is the ability to nest elements within each other. This allows developers to create complex structures and hierarchies. For example:
“`jsx
const element = (
Title
Content
);
“`
In this example, the `div` element contains an `h1` element and a `p` element.
The Need for a Single Parent Element
The rule that JSX expressions must have one parent element is in place to ensure consistency and clarity in the code. The parent element acts as a container for all the nested elements. Without a single parent element, it would be difficult to determine the structure and relationships between the elements.
Syntax Error Without Single Parent Element
If you try to create a JSX expression without a single parent element, you will encounter a syntax error. For example:
“`jsx
const element = (
Title 1
Title 2
);
“`
This code will produce a syntax error because the JSX expression contains two sibling elements that are not wrapped in a parent element.
Wrapping Multiple Elements with a `div` Element
The most straightforward solution to the “single parent element” rule is to wrap multiple elements with a `div` element. For example:
“`jsx
const element = (
Title 1
Title 2
);
“`
By wrapping the `h1` and `h2` elements within a `div`, we adhere to the rule and the code becomes syntactically correct.
Alternative to a `div` Element
In some cases, using a `div` element as a wrapper may not be desirable, especially if the extra `div` affects the styling or layout of the components. In such scenarios, React provides an alternative called Fragments.
Using Fragments to Wrap Multiple Elements
Fragments are a lightweight syntax for declaring a grouping of elements without introducing an extra DOM node. Instead of using a `div` as a wrapper, we can use a Fragment. Here’s an example:
“`jsx
import React, { Fragment } from ‘react’;
const element = (
Title 1
Title 2
);
“`
Using Arrays to Avoid a Wrapper Element
Another technique to avoid wrapping elements with a wrapper element is to use an array. We can wrap the multiple elements within square brackets `[]` to create an array. For example:
“`jsx
const element = [
Title 1
,
Title 2
];
“`
By assigning a unique `key` prop to each element, we ensure proper rendering and performance.
The Key Prop and Lists of Elements
When working with lists of elements, it’s important to assign a unique `key` prop to each child element. The `key` prop helps React identify which elements have changed, added, or removed. This improves rendering performance and avoids duplication or misalignment of data.
Common Mistakes and Pitfalls
While working with JSX expressions, there are a few common mistakes and pitfalls to avoid:
1. JSX expressions must have one parent element: It’s important to always wrap multiple elements with a single parent element or use alternatives like Fragments or arrays.
2. Each child in a list should have a unique `key` prop: Assigning a `key` prop to each element in a list helps React efficiently update the DOM.
3. Adjacent JSX elements must be wrapped in an enclosing tag: JSX expressions cannot have sibling elements without a parent element.
4. JSX expressions are wrapped in: JSX expressions are wrapped in parentheses `()`. Make sure not to forget these parentheses when working with JSX.
5. “Failed to find fragment for React root view” (React Native): When working with React Native, make sure to import the `Fragment` component correctly and use it instead of a `div` element.
FAQs
Q: Can I have multiple parent elements in a JSX expression?
A: No, JSX expressions must have one parent element. Multiple parent elements can make it difficult to understand the structure and relationships between the elements.
Q: What is the purpose of the `key` prop in JSX?
A: The `key` prop helps React identify which elements have changed, added, or removed. It improves rendering performance and avoids duplication or misalignment of data.
Q: What should I do if I want to avoid using a `div` as a wrapper element?
A: React provides alternatives like Fragments and arrays to avoid using a `div` as a wrapper element. Fragments allow you to group elements without introducing an extra DOM node, while arrays provide a way to wrap multiple elements using square brackets `[]`.
In conclusion, adhering to the rule that JSX expressions must have one parent element is essential for writing clean and maintainable code. Wrapping multiple elements with a `div` element, using Fragments, or using arrays are effective techniques to comply with this rule. By understanding and following this rule, developers can create more structured and readable code in their React applications.
✅ Solved Ts3657: (Js) Jsx Expressions Must Have One Parent Element.Ts (2657)
Why Jsx Must Have One Parent Element?
JSX, or JavaScript XML, is an essential part of the React framework and plays a crucial role in enabling developers to write component-based, reusable UI code. One of the fundamental rules when working with JSX is that it must have one parent element. This rule is often a cause of confusion for newcomers to React, but it serves a crucial purpose in ensuring the efficient rendering of components and maintaining a clean code structure. In this article, we will delve deeper into why JSX must have one parent element, exploring the reasons behind this rule and addressing common questions or misconceptions.
Understanding JSX and its Purpose
To understand why JSX requires a single parent element, it is crucial to have a solid grasp on what JSX is and how it works. JSX is a syntax extension for JavaScript that allows the rendering of HTML-like elements and components within JavaScript code. It provides a way to write declarative, intuitive, and efficient UI code by combining JavaScript logic and HTML-like syntax.
As JSX elements closely resemble HTML, they can be nested within each other to form complex component structures. However, the JSX specification mandates that all JSX expressions must be wrapped within a single parent element. Consequently, a JSX expression with multiple top-level elements or components will throw an error during compilation.
Maintaining a Clean DOM Structure
One of the primary reasons why JSX enforces the one-parent rule is to ensure a clean and organized DOM structure. By having a single parent element, JSX components can be easily encapsulated and inserted into the DOM, improving code readability and maintainability. A single parent element allows for better separation of concerns, making it easier to reason about and debug the application’s hierarchical structure.
Efficient DOM Manipulation and Rendering
Another significant advantage of having one parent element in JSX is that it enables efficient DOM manipulation and rendering. When a JSX component with multiple top-level elements is rendered, React needs to create a wrapper container under the hood. This extra layer results in additional DOM nodes, which can impact performance, especially for large-scale applications.
By having one parent element, React can efficiently manipulate and update only the necessary parts of the DOM when a component’s state or props change. It allows for optimized rendering and minimizes unnecessary re-renders, ensuring a smooth and performant user experience.
Common Questions and FAQs about JSX and its One Parent Rule:
Q: Can I bypass the one-parent rule in JSX?
A: While it is technically possible to bypass the one-parent rule, doing so would go against the recommended best practices and could lead to code that is harder to read and maintain. It is always advisable to follow the one-parent rule for cleaner and more efficient code.
Q: Can I use fragments to overcome the one-parent rule?
A: Yes, React provides a feature called fragments that allow you to group a list of children elements without adding extra nodes to the DOM. Fragments provide a concise way to write JSX components with multiple top-level elements while still adhering to the one-parent rule.
Q: Why doesn’t React automatically wrap multiple top-level elements for us?
A: React’s decision not to automatically wrap multiple top-level elements is rooted in its philosophy of explicitness and developer control. By enforcing a single parent element, React encourages developers to be deliberate about their component structure, leading to cleaner and more manageable code.
Q: Can I use arrays as a workaround to have multiple top-level elements?
A: While you can use arrays to render multiple elements in a given JSX expression, you still need to wrap them in a parent element. React requires a single parent element, be it a div, a fragment, or any other valid JSX container.
In conclusion, the one-parent rule in JSX is an essential rule that must be followed when working with React. It helps maintain a clean DOM structure, enables efficient rendering, and facilitates better code organization. While it may seem restrictive at first, understanding and adhering to this rule will lead to more readable, performant, and maintainable code.
How Are Expressions Specified In Jsx?
JSX, which stands for JavaScript XML, is an extension of JavaScript that allows us to write HTML-like code in our JavaScript files. It is commonly used with React, a popular JavaScript library for building user interfaces. JSX allows us to write code that looks similar to HTML but is actually JavaScript. One of the key features of JSX is the ability to specify expressions within the code. In this article, we will explore how expressions are specified in JSX and understand their role in rendering dynamic content.
Expressions in JSX are enclosed within curly braces ({}) and can be used anywhere within the JSX code. These expressions are evaluated at runtime and their results are incorporated into the rendered output.
For example, consider the following JSX code snippet:
“`
const name = ‘John Doe’;
const element =
Hello, {name}
;
“`
In this example, the value of the `name` variable is inserted into the JSX code using curly braces. During runtime, the value of `name` will be evaluated and displayed within the `
` tag, resulting in the rendered output: “Hello, John Doe”.
Expressions can include variables, JavaScript operators, function calls, and even other JSX elements or components. This flexibility allows us to dynamically render content based on the current state of our application or the values of variables.
Let’s explore a few more examples to understand the different ways expressions can be specified in JSX:
1. Variables and Operators:
“`
const num1 = 5;
const num2 = 10;
const element =
The sum of {num1} and {num2} is {num1 + num2}.
;
“`
In this example, we’re performing a simple addition using the `+` operator within the JSX code. The expression `{num1 + num2}` will be evaluated to 15, and the result will be displayed within the `
` tag.
2. Function Calls:
“`
function sayHello() {
return ‘Hello, world!’;
}
const element =
;
“`
Here, the JSX element `
3. JSX elements and components:
“`
const ListItem = (props) => {
return
;
}
const listItems = [‘Apple’, ‘Banana’, ‘Orange’];
const element = (
-
{listItems.map((item) => (
))}
);
“`
In this example, we have a custom JSX component called `ListItem` that renders a list item with the value provided via the `text` prop. We use the `map()` method to iterate over the `listItems` array and dynamically create multiple instances of the `ListItem` component. The list items are then rendered within the `
- ` tag.
FAQs:
Q: Can JSX expressions be used outside of JSX code?
A: Yes, JSX expressions can be used anywhere within JavaScript code. They are not limited to JSX code and can be used in regular JavaScript statements or assignments.
Q: Can I use conditional statements in JSX expressions?
A: Yes, you can use conditional statements such as `if-else` or ternary expressions within JSX expressions. For example:
“`
const isLoggedIn = true;
const element =
{isLoggedIn ? ‘Welcome, user!’ : ‘Please log in.’}
;
“`
This will display either “Welcome, user!” or “Please log in.” based on the value of the `isLoggedIn` variable.
Q: Can I use expressions in JSX attributes?
A: Yes, you can use expressions within JSX attributes. For example:
“`
const src = ‘path/to/image.jpg’;
const element = ;
“`
Here, the `src` variable is used within the `src` attribute of the `` tag. The value of the `src` variable will be evaluated and assigned to the `src` attribute.
In conclusion, JSX provides a powerful way to specify expressions within JavaScript code, allowing us to dynamically render content in our React applications. By using expressions, variables, operators, function calls, and even other JSX elements or components, we can create dynamic and interactive user interfaces. Understanding how to specify expressions in JSX is essential for harnessing the full potential of React and delivering dynamic web experiences.
Keywords searched by users: jsx expressions must have one parent element JSX expressions must have one parent element, Each child in a list should have a unique key” prop, Adjacent JSX elements must be wrapped in an enclosing tag, Jsx expressions are wrapped in, Failed to find fragment for react root view react-native
Categories: Top 93 Jsx Expressions Must Have One Parent Element
See more here: nhanvietluanvan.com
Jsx Expressions Must Have One Parent Element
JSX (JavaScript XML) is an extension to the JavaScript language that allows developers to write HTML-like code within their JavaScript files. It is commonly used with React, a popular JavaScript library for building user interfaces. JSX offers a more concise and expressive way to create components, but it comes with a few restrictions. One such restriction is that JSX expressions must have one parent element. In this article, we will delve into why this requirement exists, how it impacts developers, and provide solutions to common issues encountered.
Understanding JSX
Before we explore the necessity of a single parent element in JSX expressions, let’s quickly review what JSX is. JSX is a syntactic sugar for React.createElement(), a method used to create React elements. It allows developers to write familiar HTML-like tags and structures in their JavaScript code, making it easier to visualize and create the desired user interface.
Consider the following example:
“`jsx
const element =
Hello, World!
;
“`
In this example, we assign a JSX expression to a variable called “element.” The expression looks like HTML, but it is actually JavaScript. Under the hood, React translates this JSX into a JavaScript function call to create a React element. JSX allows embedding JavaScript variables or expressions within curly braces, enabling dynamic content and rendering.
Why Must JSX Expressions Have One Parent Element?
One of the fundamental rules of JSX is that it requires a single parent element. In other words, when rendering JSX code, you must wrap all elements within a single container. This constraint may seem restrictive at first, but it serves an essential purpose.
JSX expressions are ultimately transformed into JavaScript function calls, and those functions expect a single element as an argument. By enforcing a single parent element, React is able to efficiently render and update the DOM. Instead of having to manage multiple root elements, React can treat the parent element as a wrapper, simplifying the rendering process.
Consider the following invalid JSX code:
“`jsx
// Invalid JSX code
const element = (
Hello, World!
Welcome to the world of JSX!
);
“`
In this example, we have two sibling elements: an h1 tag and a p tag. React would not know how to handle this code since it expects a single parent element. To address this, developers need to wrap the two elements in a parent container, as shown below:
“`jsx
// Valid JSX code
const element = (
Hello, World!
Welcome to the world of JSX!
);
“`
Now, we have a single parent element, div, wrapping the h1 and p tags. React can handle this structure when rendering or updating the DOM.
Common Issues Faced by Developers
Working with JSX can sometimes be a bit challenging for developers, particularly when it comes to adhering to the requirement of a single parent element. Let’s address some frequently asked questions and shed light on potential solutions:
Q: Can I use an array as the parent element?
A: No, JSX expressions cannot have arrays as the parent element. However, you can use the React.Fragment component, shorthand syntax <>…>, or wrap the array elements in a div.
Q: What if I don’t want to introduce an extra div just for the sake of satisfying the requirement?
A: You don’t have to. As mentioned earlier, you can use React.Fragment or the shorthand syntax <>…> as the parent element. Both options allow you to wrap multiple elements without introducing an additional div.
Q: Can I use a custom component as the parent element?
A: Yes, you can use a custom component as the parent element, as long as it returns a single element. For example:
“`jsx
const Wrapper = ({ children }) =>
;
const element = (
Hello, World!
Welcome to the world of JSX!
);
“`
Here, the Wrapper component acts as the parent element and contains the desired child elements.
In conclusion, JSX expressions in React must have one parent element. This requirement is necessary to ensure efficient rendering and updating of the DOM. By forcing developers to wrap multiple elements in a single container, React can simplify the rendering process. Although it may introduce some initial challenges, understanding and embracing this requirement will help you leverage the power and flexibility of JSX effectively. So next time you build a React component, remember to follow this rule for a smooth coding experience.
FAQs:
1. Q: Can I use an array as the parent element?
A: No, JSX expressions cannot have arrays as the parent element. However, you can use the React.Fragment component, shorthand syntax <>…>, or wrap the array elements in a div.
2. Q: What if I don’t want to introduce an extra div just for the sake of satisfying the requirement?
A: You don’t have to. As mentioned earlier, you can use React.Fragment or the shorthand syntax <>…> as the parent element. Both options allow you to wrap multiple elements without introducing an additional div.
3. Q: Can I use a custom component as the parent element?
A: Yes, you can use a custom component as the parent element, as long as it returns a single element. For example:
“`jsx
const Wrapper = ({ children }) =>
;
const element = (
Hello, World!
Welcome to the world of JSX!
);
“`
Here, the Wrapper component acts as the parent element and contains the desired child elements.
Each Child In A List Should Have A Unique Key” Prop
Why is a unique key necessary for each child element in a list?
When rendering a list of elements in React, it is essential to assign a unique “key” prop to each child component. This key serves as an identifier, allowing React to efficiently update and reorder elements when necessary. Without a unique key, React has to resort to more time-consuming methods to update the DOM tree, resulting in decreased performance and potential issues with component state.
React uses reconciliation, a process that compares the previous and new versions of a component tree, to determine the most efficient way to update the DOM. During reconciliation, React analyzes the keys assigned to each element in the list and identifies changes, additions, or removals. Incorporating a unique key for each child enables React to quickly and accurately identify these modifications, thereby minimizing unnecessary re-rendering and improving overall performance.
Benefits of using unique keys
1. Performance optimization: By providing a unique key to each child, React can effortlessly identify and update only the elements that have changed, rather than re-rendering the entire list. This selective rendering significantly improves performance, especially when dealing with large lists or frequently updated UIs.
2. Prevents incorrect component reuse: Keys help React differentiate between components, particularly when elements are dynamically added or removed. Without unique keys, React may mistakenly reuse components, causing unintended side effects like retaining incorrect component state or failing to handle certain lifecycle methods correctly.
3. Supports stable component state: React relies on stable keys to maintain component state correctly. When keys are consistent across re-renders, React knows which components to update and preserve their state. Without unique keys, React may reset component state or trigger unexpected behaviors, leading to a poor user experience.
Best practices for using unique keys
1. Choose keys that are stable and unique: Keys should be assigned based on a property of the child element that is guaranteed to be unique. Using an index as a key is discouraged, as it may lead to incorrect reconciliation. Instead, opt for a unique identifier associated with the data being rendered, such as an ID or a combination of properties that uniquely identify the element.
2. Avoid using random or non-deterministic keys: Keys should not be randomly generated or use values that could change between re-renders, such as timestamps. Non-deterministic keys can cause unnecessary re-rendering, defeating the purpose of key optimization.
3. Do not rely on sibling keys for uniqueness: Each key must be unique among sibling elements, but it does not need to be globally unique within the entire application. React only requires keys to be unique within a particular context, such as a list or a set of siblings.
4. Refrain from using array indices as keys: While tempting, using array indices as keys should generally be avoided. If the order of elements changes, it can create inconsistencies in the React reconciliation process. Only consider employing array indexes as keys if the list is static and its items will not be reordered or updated.
5. Update keys when the order changes: If the order of child elements in a list can be modified, it is essential to update the corresponding keys accordingly. React depends on keys to identify and reconcile changes, so modifying the order without updating the keys can lead to incorrect rendering or loss of component state.
FAQs:
Q1. What happens if I don’t provide a key prop for child elements in a list?
Without a key prop, React may issue a warning in the console, reminding you to add unique keys to each child. While the application may still work, it will not benefit from the performance optimizations that keys provide. Additionally, omitting keys can result in incorrect rendering and component reuse issues, compromising the stability of the UI.
Q2. Can I use the same key for different elements in separate lists?
Yes, keys only need to be unique within the context of their own list or siblings. You can reuse the same key value for different elements in separate lists or within different branches of the component tree.
Q3. Does the choice of key affect performance?
In terms of performance, the choice of key does not directly matter as long as it is stable and unique. However, poorly chosen keys, such as random or non-deterministic values, can lead to unnecessary re-renders and impact overall performance. Therefore, it is important to adhere to best practices and select keys that remain consistent throughout the lifecycle of the corresponding elements.
Q4. What if I have multiple dynamically rendered lists on a page?
In scenarios where multiple dynamically rendered lists appear on the same page, it is crucial to ensure that keys are unique within the scope of each list. This way, React can distinguish between the different lists and perform the necessary optimizations independently.
In conclusion, providing a unique “key” prop for each child element in a list is essential in React development. The key prop enables React to efficiently reconcile changes, optimize performance, and maintain stable component state. By adhering to best practices and understanding the importance of unique keys, developers can ensure optimal rendering and enhance overall application performance.
Adjacent Jsx Elements Must Be Wrapped In An Enclosing Tag
When developing applications using React, developers often come across a peculiar requirement – “Adjacent JSX elements must be wrapped in an enclosing tag.” This rule is designed to ensure that JSX code is compiled correctly and rendered accurately by React.
JSX (JavaScript XML) is a syntax extension for JavaScript that allows the inclusion of HTML-like code within JavaScript. It is widely used with React to define the structure and components of a user interface. JSX code is not directly understood by the browser; it needs to be transformed into JavaScript before it can be executed.
React uses a process called “reconciliation” to efficiently update the rendered components in the DOM (Document Object Model) when changes occur. In order to perform this process accurately, React requires JSX elements to be grouped within a single enclosing tag.
Why do adjacent JSX elements need to be wrapped?
The need to wrap adjacent JSX elements in an enclosing tag arises from the way React processes and renders those elements. When JSX elements are transpiled into JavaScript, they are essentially treated as expressions, which are then transformed into React.createElement() calls.
In JavaScript, adjacent JSX elements implicitly create an array. However, when React is rendering components, it expects a single element, not an array. Wrapping adjacent JSX elements within an enclosing tag allows React to correctly treat them as a single element.
Consider the following example:
“`jsx
// Incorrect code
const MyComponent = () => {
return (
Hello
World
);
};
“`
In this case, React would throw a syntax error because the two JSX elements `
` and `
` are not enclosed within a single element. To fix this, we must wrap them within a parent element:
“`jsx
// Correct code
const MyComponent = () => {
return (
Hello
World
);
};
“`
By enclosing the JSX elements within a `
What can be used as an enclosing tag?
In React, any valid HTML element can be used as an enclosing tag. Developers often opt for a `
One such alternative is to use a special React Fragment, denoted by `<>` or `
The previous example can be rewritten using a fragment:
“`jsx
const MyComponent = () => {
return (
<>
Hello
World
>
);
};
“`
Using fragments in this manner allows the components to compile and render correctly without adding an extra node to the DOM.
Is there any other way to handle adjacent JSX elements without an enclosing tag?
While wrapping adjacent JSX elements is the recommended way to satisfy the React rule, there are alternative approaches. One such approach is to use an array to render multiple JSX elements without enclosing tags.
The following example demonstrates this approach:
“`jsx
const MyComponent = () => {
return [
Hello
,
World
];
};
“`
By assigning each element a unique `key` prop, React understands that these elements belong together. Although this method can work, it is generally recommended to use an enclosing tag or a fragment for better code readability and maintainability.
FAQs about wrapping adjacent JSX elements:
Q. Can I use any HTML element as an enclosing tag?
A. Yes, you can use any valid HTML element as an enclosing tag.
Q. Is it possible to use multiple enclosing tags for adjacent JSX elements?
A. No, adjacent JSX elements must be wrapped in a single enclosing tag or a fragment. Multiple enclosing tags are not allowed.
Q. Why does React require this rule?
A. React needs to process and render JSX elements in a predictable and efficient manner. By requiring adjacent JSX elements to be wrapped, React can ensure that components are rendered accurately.
Q. Can I use an HTML comment as an enclosing tag?
A. No, HTML comments are not valid enclosing tags. They are not considered as real elements in the DOM and will not satisfy the React rule.
Q. What should I do if I need to conditionally render adjacent JSX elements?
A. In such cases, conditional rendering should be performed within the enclosing tag or the fragment containing the adjacent JSX elements. This allows React to handle the conditionally rendered elements correctly.
In conclusion, the React rule of wrapping adjacent JSX elements in an enclosing tag or a fragment is crucial for correct rendering and efficient reconciliation. It ensures that JSX code is transformed into JavaScript properly and that components are rendered accurately. By following this rule, developers can build robust and predictable React applications.
Images related to the topic jsx expressions must have one parent element
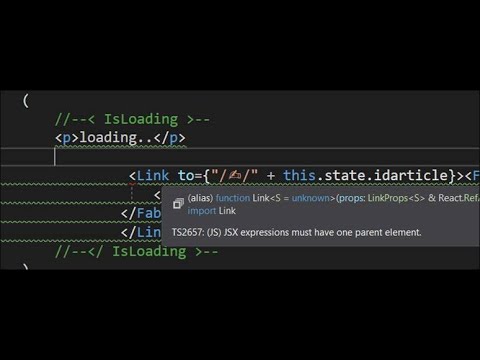
Found 25 images related to jsx expressions must have one parent element theme
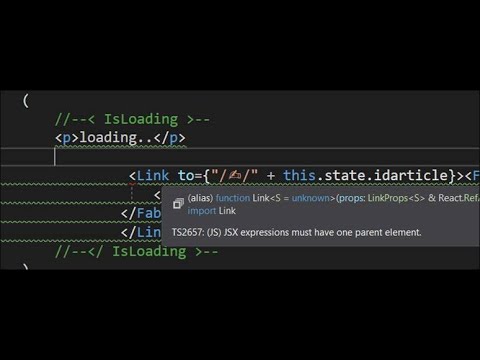
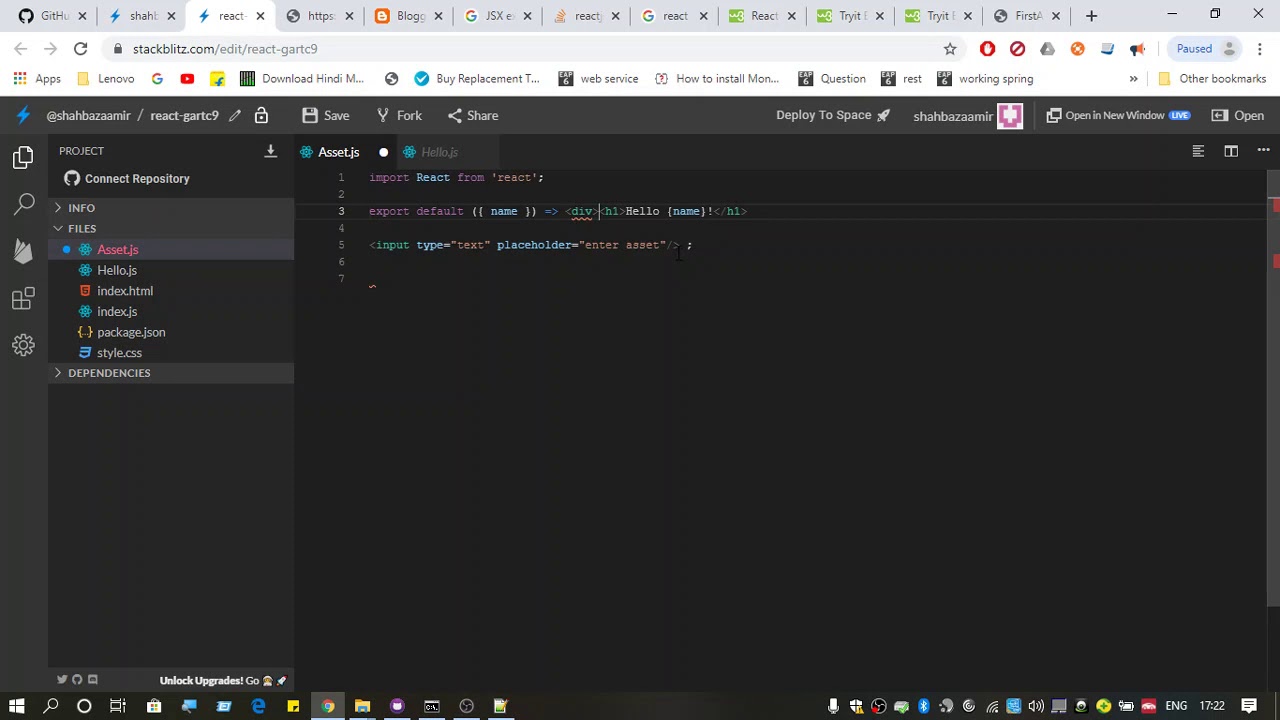

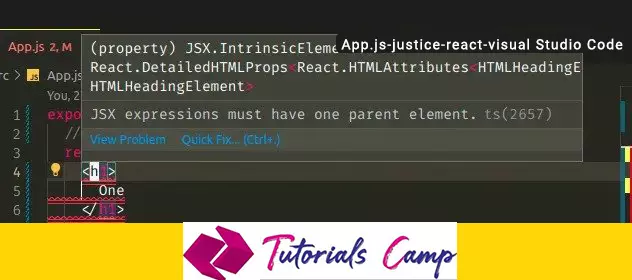


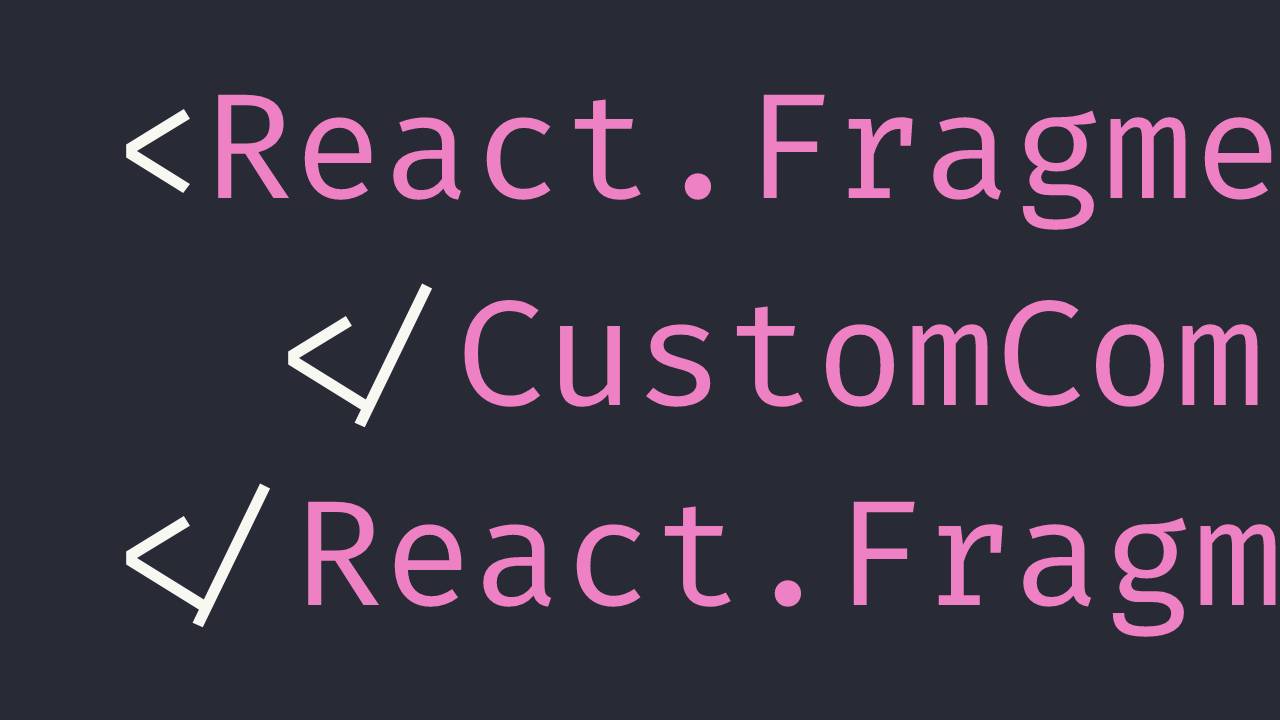
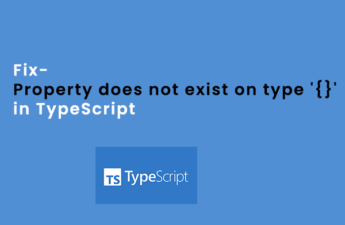

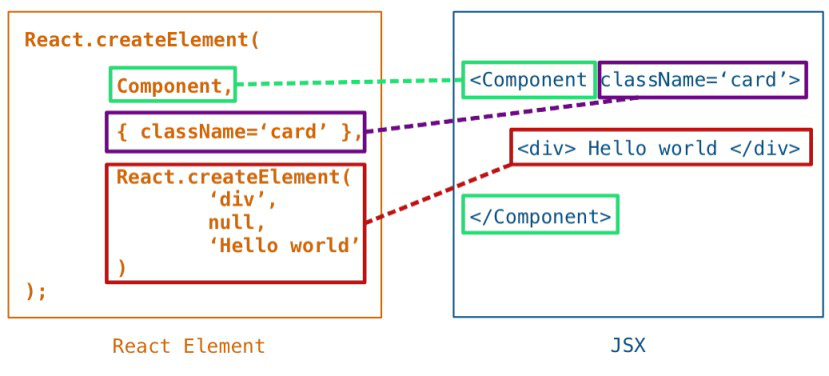
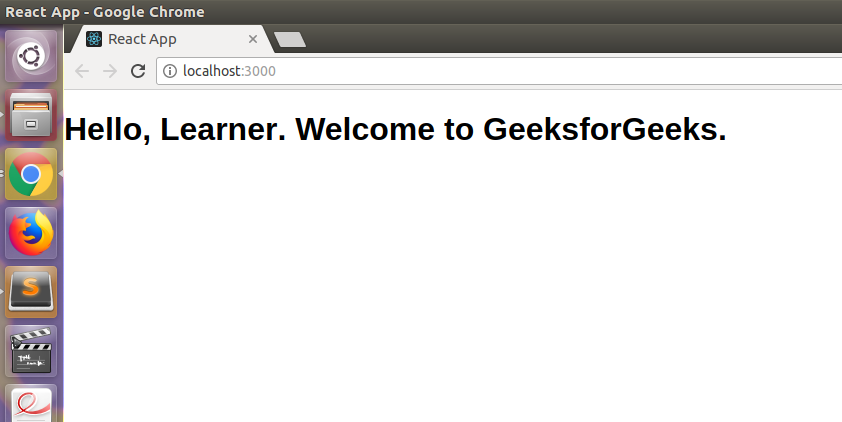

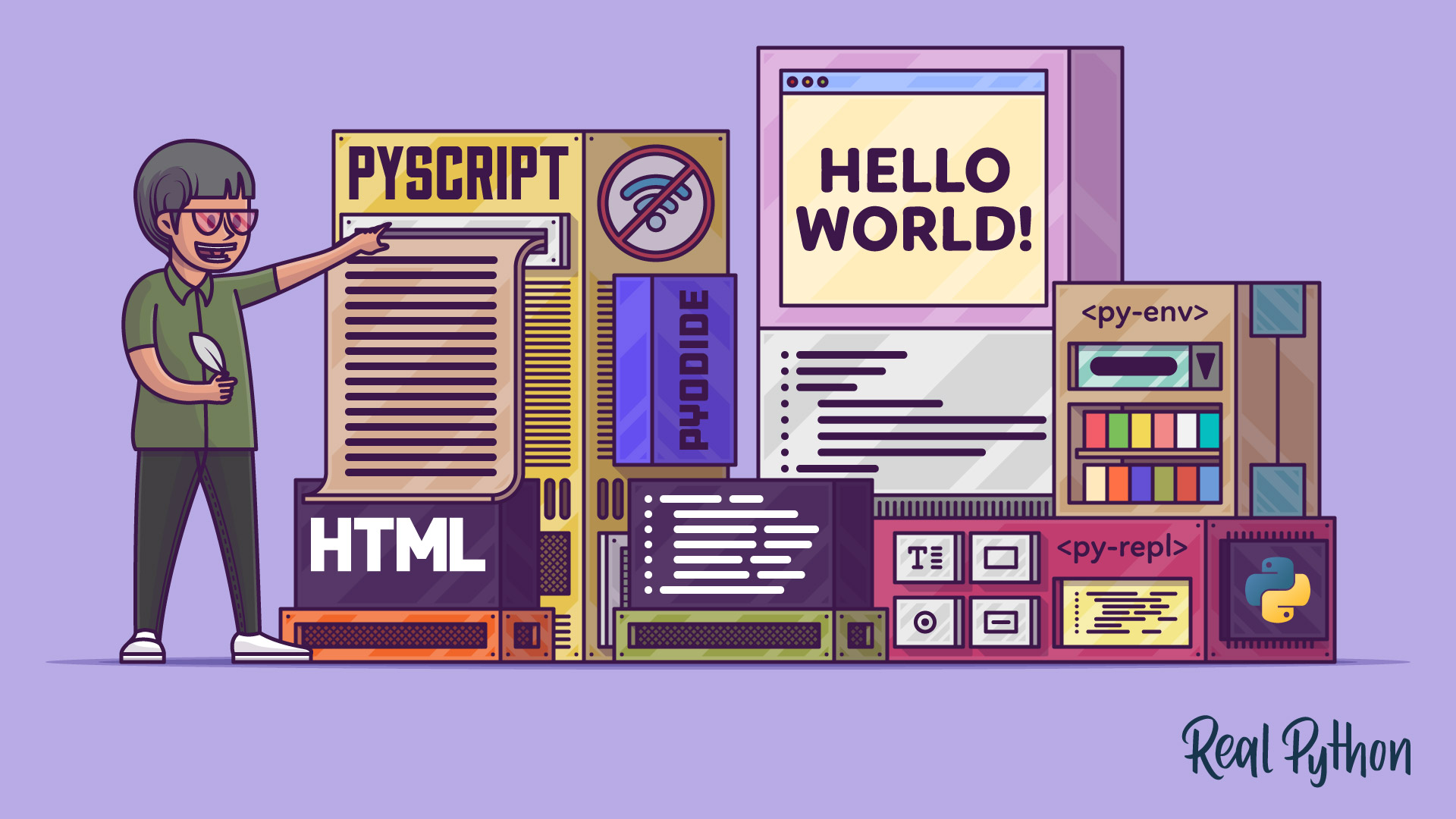
Article link: jsx expressions must have one parent element.
Learn more about the topic jsx expressions must have one parent element.
- JSX expressions must have one parent element in React
- How to Fix “JSX expressions must have one parent element …
- Why do I get the error “expressions must have one parent …
- Why must JSX expressions have only ONE parent element?
- How to Fix “JSX expressions must have one parent element …
- JSX in React – Explained with Examples – freeCodeCamp
- Why does react component return multiple elements? – Stack Overflow
- How to return multiple elements in JSX – Flavio Copes
- JSX Expressions Must Have Only One Parent Element – Why?
- JSX expressions must have one parent element in React
- React – JSX expressions must have one parent element error
See more: blog https://nhanvietluanvan.com/luat-hoc