Typeerror: Descriptors Cannot Not Be Created Directly.
TypeError is a common error in Python that occurs when an operation is performed on an object of an inappropriate type. It indicates that the type of the object does not support the specific operation being performed. This error can occur in various scenarios and can be caused by a range of reasons.
Creating Descriptors in Python
In Python, descriptors are a powerful feature that allows developers to define how attributes of a class should be accessed, assigned, or deleted. Descriptors provide a way to customize the behavior of attribute access and provide a mechanism for implementing Object-oriented programming concepts like encapsulation and abstraction.
Descriptors are defined as classes that implement certain methods like `__get__`, `__set__`, and `__delete__`. These methods define how the descriptor interacts with the attribute it is attached to. Descriptors can be used to define both instance-level and class-level attributes.
Why Descriptors Cannot Be Created Directly
Despite the flexibility and usefulness of descriptors, they cannot be created directly. This means that developers cannot create a descriptor object and directly assign it to an attribute of a class. Instead, descriptors need to be used in conjunction with another feature in Python called property decorators.
The reason descriptors cannot be created directly is that they require a class definition to be able to function properly. Descriptors operate at the class level and need to be defined within a class. They rely on the descriptor protocol, which defines how the attribute access and modification should be handled by the descriptor.
Alternatives to Creating Descriptors Directly
Since descriptors cannot be created directly, developers can use an alternative approach to achieve similar functionality. One popular alternative is to use property decorators.
Property decorators provide a more concise and readable syntax for defining descriptors. They allow a developer to define a getter, setter, and deleter for a class attribute using a simple and intuitive syntax.
Using Property Decorators as Descriptors
To use property decorators as descriptors, developers can define a method within a class and decorate it with the `@property` decorator. This method will act as a getter for the attribute.
Additionally, developers can define another method and decorate it with `@
Lastly, developers can define a method and decorate it with `@
Implementing Descriptors using the Descriptor Protocol
Implementing descriptors using the descriptor protocol involves defining a class that implements the `__get__`, `__set__`, and `__delete__` methods. These methods define how the descriptor interacts with the attribute it is attached to.
The `__get__` method is called when the attribute value is accessed. It takes two parameters – `self` and `instance`, where `self` is the descriptor object itself and `instance` is the instance of the object that the attribute belongs to.
The `__set__` method is called when the attribute value is assigned a new value. It takes three parameters – `self`, `instance`, and `value`, where `self` is the descriptor object, `instance` is the instance of the object, and `value` is the new value to be assigned.
The `__delete__` method is called when the attribute is deleted. It takes two parameters – `self` and `instance`, where `self` is the descriptor object and `instance` is the instance of the object.
Common Errors and Troubleshooting Descriptors
When working with descriptors, developers may encounter several common errors. One such error is the “TypeError: descriptors cannot not be created directly.” This error occurs when a descriptor is created directly and assigned to an attribute without using property decorators.
To resolve this error, developers should use property decorators to define descriptors. By using property decorators, developers can define a more concise and readable syntax for defining descriptors.
FAQs
Q: Can descriptors be used with both instance-level and class-level attributes?
A: Yes, descriptors can be used with both instance-level and class-level attributes. Instance-level descriptors are defined within a class and are attached to specific instances of the class. Class-level descriptors are defined within a class and are shared among all instances of the class.
Q: Are property decorators the only way to create descriptors in Python?
A: No, property decorators are not the only way to create descriptors in Python. Developers can also implement descriptors using the descriptor protocol by defining a class that implements the `__get__`, `__set__`, and `__delete__` methods.
Q: How can I downgrade the protobuf package to 3.20.x or lower?
A: To downgrade the protobuf package to a specific version, you can use the pip package manager. Run the command `pip install protobuf==3.20.x` to install the desired version.
Q: What is the difference between Protobuf Python and Protobuf cpp?
A: Protobuf Python is a Python implementation of Protocol Buffers, a language-agnostic data serialization format. Protobuf cpp, on the other hand, is a C++ implementation of Protocol Buffers. Both implementations provide similar functionality but are optimized for their respective languages.
Q: How can I troubleshoot the error “Cannot import name ‘builder’ from ‘google.protobuf.internal'”?
A: This error is usually caused by a mismatch between the installed versions of the protobuf package and the TensorFlow package. To resolve this issue, you can try downgrading the protobuf package to a lower version using the steps mentioned earlier. Additionally, ensure that you have the latest versions of both the protobuf and TensorFlow packages installed.
Q: Where can I find the Protobuf documentation?
A: The Protobuf documentation can be found on the official Protocol Buffers website. The documentation provides detailed information on how to define and use Protocol Buffers, as well as examples and guidelines for different programming languages.
Error In Tensorflow
Keywords searched by users: typeerror: descriptors cannot not be created directly. downgrade the protobuf package to 3.20.x or lower., set protocol_buffers_python_implementation=python, Protobuf, Protobuf Python, TensorFlow, Protobuf cpp, Cannot import name ‘builder’ from ‘google protobuf internal, Protobuf documentation
Categories: Top 47 Typeerror: Descriptors Cannot Not Be Created Directly.
See more here: nhanvietluanvan.com
Downgrade The Protobuf Package To 3.20.X Or Lower.
Introduction:
Protobuf (Protocol Buffers) is a widely-used data serialization format created by Google, enabling efficient and language-independent communication between different systems. However, occasionally, it may become necessary to downgrade the Protobuf package to a specific version, such as 3.20.x or lower. This article will provide a detailed guide on how to perform this downgrade, covering its importance and potential challenges.
Understanding the Considerations:
1. Why would one need to downgrade the Protobuf package?
There could be various reasons behind the need to downgrade Protobuf, such as:
– Compatibility issues: The codebase may include dependencies or systems that are only compatible with older Protobuf versions.
– Breaking changes: A recent Protobuf update may have introduced breaking changes, requiring a regression to a previous version.
– Specific feature requirements: Certain projects may demand a feature available only in older Protobuf packages.
2. The implications of downgrading Protobuf:
It is important to consider the implications before initiating a downgrade:
– Compatibility: Ensure the downgraded Protobuf version is compatible with the entire system, including dependencies, frameworks, and other packages.
– Security and Bug Fixes: Downgrading may potentially result in the loss of critical security patches or bug fixes. Evaluate the impact and weigh it against specific requirements.
Step-by-Step Guide to Downgrading Protobuf:
Step 1: Assess the existing Protobuf version
– Identify the current Protobuf package version used in the system. Determine whether it exceeds the desired version range (3.20.x or lower).
Step 2: Study the release notes
– Review the release notes of the desired Protobuf version to understand any potential issues or changes that could impact the system.
– Pay particular attention to backward-incompatible changes that may require modifications in the codebase or configuration.
Step 3: Backup your codebase and dependencies
– Before proceeding with any changes, ensure you have a backup of the entire codebase.
– Ideally, use a version control system like Git to create a branch or tag that preserves the current state.
Step 4: Update package dependencies
– Review the dependencies of the system and determine if any updates or modifications are required to accommodate the downgraded Protobuf version.
– Identify any dependencies with explicit Protobuf version constraints. Adjust them accordingly.
Step 5: Update the protobuf package
– Utilize the package management system specific to your programming language to downgrade the Protobuf package to the desired version (3.20.x or lower).
Step 6: Validate the downgrade
– Run your entire test suite to ensure the system functions correctly with the downgraded Protobuf version.
– Pay close attention to any issues or errors encountered during the validation process.
Step 7: Fix compatibility issues (if any)
– If any compatibility issues arise during validation, carefully analyze and address them in the codebase.
– Utilize Protobuf documentation and online resources to resolve any compatibility-related problems.
FAQs:
Q1: Can I downgrade Protobuf in all programming languages?
A: Yes, Protobuf can be downgraded in most programming languages, such as Python, Java, C++, and more, as long as the desired version is available for the specific language.
Q2: What if my system has dependencies that require a newer Protobuf version?
A: Downgrading Protobuf may require adjusting or finding alternative dependencies that are compatible with the desired Protobuf version.
Q3: Will downgrading Protobuf impact performance?
A: The impact on performance is generally minimal, but it is recommended to run thorough tests to ensure compatibility and performance remain satisfactory.
Q4: How can I update dependencies with explicit Protobuf version constraints?
A: Update your dependency configuration file (e.g., package.json, requirements.txt) to specify the desired Protobuf version.
Conclusion:
Downgrading Protobuf to version 3.20.x or lower can be essential in certain cases. By following the step-by-step guide provided here, carefully considering compatibility and safety, and addressing any encountered issues, the downgrade process can be performed effectively. Remember, it is important to weigh the benefits of downgrading against potential security and bug-fix implications.
Set Protocol_Buffers_Python_Implementation=Python
Protocol Buffers, also known as Protobuf, is a language-agnostic data serialization format developed by Google. It allows you to define the structure of your data using a simple language and then generate code in various languages to read and write that data. In this article, we will explore the Python implementation of Protocol Buffers and discuss its usage in detail.
Protocol Buffers Python Implementation
The official implementation of Protocol Buffers for Python is called “protobuf-python” or simply “protobuf”. It is an open-source project developed by Google and is widely used in both small-scale and large-scale applications. The protobuf-python library provides a powerful and efficient way to serialize structured data, making it perfect for working with complex data structures.
Installation
To use the protobuf-python library, you need to install it first. It can be installed using pip, the Python package manager, by running the following command:
“`
pip install protobuf
“`
Alternatively, you can also install it from the source code available on the official GitHub repository.
Defining Message Types
The first step in using Protocol Buffers in Python is to define the message types using a .proto file. A .proto file is essentially a schema that describes the structure of the message. It defines the fields and their types, along with any optional or repeated fields. Here’s an example of a simple .proto file:
“`
syntax = “proto3”;
message Person {
string name = 1;
int32 age = 2;
repeated string address = 3;
}
“`
In this example, we define a message type called “Person” with three fields: “name” (of type string), “age” (of type int32), and “address” (which can be repeated). The numbers (1, 2, 3) are field numbers and are used to identify the fields in the serialized data.
Generating Python Code
Once we have defined the message types in a .proto file, we need to generate the corresponding Python code. The protobuf-python library provides a command-line tool called “protoc” that can be used to generate the code. Assuming you have installed protobuf-python and protoc, you can generate the Python code using the following command:
“`
protoc –python_out=. myproto.proto
“`
This command will generate a Python file called “myproto_pb2.py” that contains the generated code for the message types defined in “myproto.proto”. You can then import this file in your Python program and use the generated classes to work with Protocol Buffers.
Serializing and Deserializing Data
Once we have the generated code, we can start serializing and deserializing data using Protocol Buffers. The generated classes provide convenient methods to set and get the values of the fields. Here’s an example of serializing and deserializing a “Person” message:
“`python
import myproto_pb2
person = myproto_pb2.Person()
person.name = “John Doe”
person.age = 30
person.address.extend([“123 Main St”, “456 Elm St”])
# Serialize the message to bytes
data = person.SerializeToString()
# Deserialize the bytes back to a message
new_person = myproto_pb2.Person()
new_person.ParseFromString(data)
print(new_person.name) # Output: John Doe
print(new_person.age) # Output: 30
print(new_person.address) # Output: [‘123 Main St’, ‘456 Elm St’]
“`
In this example, we create a new instance of the “Person” class, set its fields with some values, and then serialize it to bytes using the “SerializeToString()” method. We can then deserialize the bytes back to a message using the “ParseFromString()” method of the generated class.
Advanced Features
The protobuf-python library provides many advanced features to make working with Protocol Buffers even more powerful. Some of these features include:
– Nested message types: You can define nested message types within a .proto file, allowing you to create complex data structures.
– Enumerations: You can define enums in a .proto file, allowing you to have predefined options for fields.
– Oneof: It allows specifying that only one field in a group can be set at a time.
– Extension fields: It allows adding extra fields to a message without modifying its .proto file.
FAQs (Frequently Asked Questions)
Q: Is Protocol Buffers a replacement for JSON or other serialization formats?
A: Protocol Buffers can be a more efficient and compact alternative to serialization formats like JSON, especially when dealing with large and complex data structures. However, the choice between Protocol Buffers and other formats depends on the specific requirements and constraints of your application.
Q: Can I use Protocol Buffers with existing data structures or databases?
A: Yes, you can map existing data structures to Protocol Buffers message types, allowing you to easily serialize and deserialize them. However, you may need to write custom code to handle the mapping between the two formats.
Q: Are there any limitations to using Protocol Buffers in Python?
A: While Protocol Buffers is a powerful tool, it has a few limitations. For example, Protocol Buffers does not support dynamically adding or removing fields from a message. Once a message type is defined, its fields cannot be modified. Additionally, Protocol Buffers does not handle versioning and schema evolution automatically, so you need to carefully manage changes to your message types.
Q: Is the protobuf-python library actively maintained?
A: Yes, the protobuf-python library is actively maintained by Google and the open-source community. It receives regular updates and bug fixes, ensuring its continued compatibility and performance improvements.
Conclusion
Protocol Buffers provide a flexible and efficient way to serialize and deserialize structured data. The protobuf-python library offers a Python implementation of Protocol Buffers, allowing developers to work with complex data structures easily. By defining message types, generating Python code, and utilizing the provided methods, developers can serialize and deserialize data efficiently. With its advanced features and wide adoption, the protobuf-python implementation is a valuable tool for building robust applications.
Protobuf
Introduction:
In the world of software development, efficient data serialization is a crucial aspect to achieve optimal performance in communication between different systems. Protobuf, short for Protocol Buffers, is a widely-used data serialization format developed by Google. It offers a compact binary representation for structured data, making it ideal for data storage, communication protocols, and more. In this article, we will delve into the depths of Protobuf, exploring its features, advantages, and how it compares to other serialization formats.
Overview of Protobuf:
Protobuf is a language-agnostic serialization format, meaning it can be used with various programming languages such as C++, Java, Python, and more. It uses a well-defined schema to specify the structure of the data, which is then compiled into code that can be used to serialize or deserialize the data.
Key Features of Protobuf:
1. Efficient Data Size: Since Protobuf uses a binary format, it optimizes the size of the serialized data. It achieves this by using numerical tags to identify fields, allowing for efficient encoding and decoding of structured data. Compared to other serialization formats like XML or JSON, Protobuf typically produces significantly smaller payload sizes.
2. Language and Platform Independence: Protobuf’s language neutrality is one of its most advantageous features. It enables seamless interoperability between systems developed in different programming languages. By using the generated code, developers can easily work with Protobuf data structures without worrying about language-specific implementations.
3. Backward and Forward Compatibility: Data evolution is an important consideration for any serialization format. Protobuf, by design, provides backward and forward compatibility. It allows the addition or removal of fields in the evolving schema, making it easy to handle changes in the data structure without breaking the compatibility between different versions.
4. Extensibility: Protobuf allows for the extension of data structures through optional fields. These optional fields can be added or removed as needed without affecting the backward compatibility of existing data. This feature makes Protobuf an excellent choice for long-term data storage and evolution.
Advantages of Protobuf:
1. Performance: Protobuf’s efficiency lies not only in its smaller data size but also in its speed. The binary format allows for rapid serialization and deserialization, reducing the processing time for data transfer. This makes it highly suitable for applications where performance matters, such as real-time systems, distributed computing, and inter-process communication.
2. Language Support: With support for multiple programming languages, Protobuf offers developers the flexibility to choose their preferred language while still benefiting from the same serialized data format. This ensures smooth communication between systems regardless of the language used.
3. Code Generation: Protobuf provides automatic code generation from its schema definition. The generated code includes strongly typed classes or structs specific to each programming language. This eliminates the need for manual parsing and serialization code, reducing the chance of human error and saving development time.
Frequently Asked Questions (FAQs):
Q1. Is Protobuf only suitable for large-scale systems?
A1. While Protobuf excels in large-scale systems with high-performance requirements, it can also be used effectively in smaller projects. Its advantages, such as efficient serialization and language independence, make it a good choice for any system that requires fast and reliable communication.
Q2. How does Protobuf compare to other serialization formats like XML or JSON?
A2. Protobuf offers superior performance and smaller data sizes compared to XML or JSON. Protobuf’s binary format reduces overhead and is faster to parse and serialize, resulting in better efficiency and reduced network usage.
Q3. Can I use Protobuf with existing data structures in my application?
A3. Yes, you can use Protobuf with existing data structures. By defining Protobuf messages that align with your current data structures, you can seamlessly transfer data between systems using Protobuf serialization.
Q4. Does Protobuf support schema evolution?
A4. Yes, Protobuf supports schema evolution. You can add or remove fields in the evolving schema, making it easy to handle data structure changes over time. This allows for backward compatibility, ensuring smooth integration between different versions of the data.
Q5. Are there any security concerns with Protobuf data?
A5. Protobuf itself does not provide encryption or security measures. However, since Protobuf is language-agnostic, it can be used in conjunction with secure communication protocols to ensure the confidentiality and integrity of the data being transferred.
Conclusion:
Protobuf offers a powerful solution for efficient data serialization and communication between systems. Its compact size, multi-language support, and flexibility make it highly adaptable to a wide range of use cases. By leveraging its performance benefits and schema evolution capabilities, developers can create robust and future-proof systems. Protobuf is undoubtedly a strong contender in the world of data serialization, empowering developers to build efficient, interoperable, and scalable applications.
Images related to the topic typeerror: descriptors cannot not be created directly.
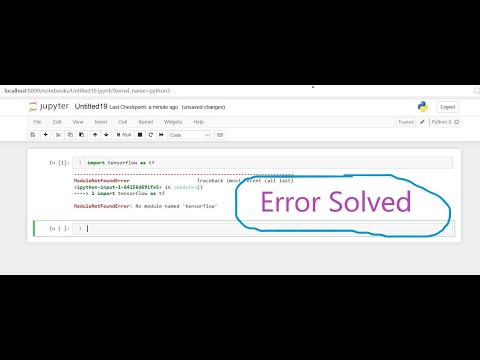
Found 35 images related to typeerror: descriptors cannot not be created directly. theme


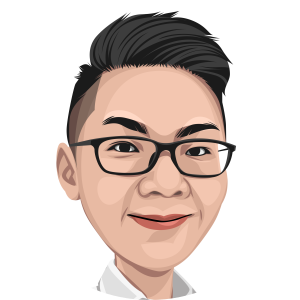
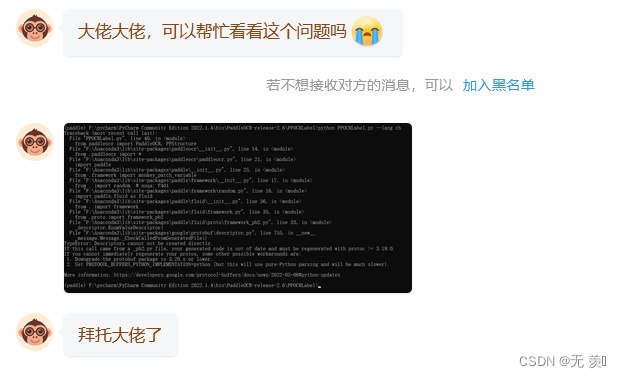

Article link: typeerror: descriptors cannot not be created directly..
Learn more about the topic typeerror: descriptors cannot not be created directly..
- python – TypeError: Descriptors cannot not be created directly
- TypeError: Descriptors cannot not be created directly [Fixed]
- Python TypeError: Descriptors cannot not be created directly
- Typeerror: descriptors cannot not be created directly ( Solved )
- How to Fix TypeError: descriptors cannot not be created directly
- TypeError: Descriptors cannot not be created directly – Streamlit
- Troubleshoot descriptors cannot not be created directly error
- TypeError: Descriptors cannot not be created directly – velog
See more: nhanvietluanvan.com/luat-hoc