Insert Datatable To Sql Table C#
Overview of SQL and C#
Firstly, let’s briefly touch on the basics of SQL and C#. SQL (Structured Query Language) is a programming language that is used to manage and manipulate relational databases. It provides a set of commands and syntax for creating, accessing, and modifying database structures, as well as querying and retrieving data.
On the other hand, C# is a popular object-oriented programming language that is commonly used for developing various types of applications including web, desktop, and mobile. It is particularly known for its integration with the .NET framework and its ability to interact with databases.
Overview of Data Tables in C#
In C#, a DataTable is a representation of a table in memory that allows you to store and manipulate data in a tabular format. It consists of a collection of DataColumn and DataRow objects, which define the schema and data of the table respectively. DataTables provide a convenient way to work with structured data and perform various operations such as sorting, filtering, and aggregating.
Methods for creating Data Tables in C#
There are several ways to create a DataTable in C#. Here are a few commonly used methods:
1. Programmatically:
You can create a DataTable programmatically by defining the structure of the table using DataColumn objects and adding them to the Columns collection of the DataTable. You can then populate the table with data by creating DataRow objects and adding them to the Rows collection.
2. Reading from a data source:
You can also populate a DataTable by reading data from a data source such as a CSV file, Excel spreadsheet, or another database table. C# provides various libraries and frameworks to handle different data sources and convert them into DataTable format.
Inserting Data into SQL Tables using C#
To insert data from a DataTable into a SQL table using C#, you need to establish a connection to the SQL server and execute SQL queries to insert the data. Here are the steps involved:
1. Establish a connection:
Before inserting data, you need to establish a connection to the SQL server using the appropriate connection string. C# provides several classes and methods in the System.Data.SqlClient namespace to handle connections, such as the SqlConnection class.
2. Create a SQL query:
Next, you need to create an SQL query that inserts the data from the DataTable into the SQL table. The query should include the table name, column names, and parameter placeholders for the values to be inserted.
3. Execute the query:
Once the connection and query are set up, you can execute the query using a command object, such as the SqlCommand class. You can pass the DataTable values as parameters to the query using the SqlParameter class.
Introduction to SQL Server and the concept of tables
SQL Server is a relational database management system (RDBMS) developed by Microsoft. It provides a robust and scalable platform for storing, retrieving, and managing data. Tables are a fundamental concept in SQL Server, representing structured data in a tabular format. Each table consists of columns (fields) that define the data types and attributes of the data, and rows (records) that store the actual data.
Connecting C# to SQL Server
To connect C# to SQL Server, you need to provide a valid connection string that specifies the server, database, authentication method, and other parameters required to establish the connection. The connection string can be either hardcoded in the application or stored in a configuration file. Here is an example of a connection string:
“`
string connectionString = “Data Source=servername;Initial Catalog=databasename;User ID=username;Password=password”;
“`
Executing SQL queries from C# to insert data into tables
Now that we have established a connection to the SQL server and created a DataTable, it’s time to insert the data into the SQL table. Here is an example of how to execute an SQL query from C# to insert a DataTable into an SQL table:
“`
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
using (SqlBulkCopy bulkCopy = new SqlBulkCopy(connection))
{
bulkCopy.DestinationTableName = “dbo.TableName”;
bulkCopy.WriteToServer(dataTable);
}
}
“`
In the above example, we first open the connection to the SQL server using the SqlConnection class. Then, we create an instance of the SqlBulkCopy class, which provides a fast and efficient way to bulk insert data into SQL tables. We set the DestinationTableName property to the target table name, and then call the WriteToServer method to insert the data from the DataTable into the SQL table.
FAQs
Q: How can I insert a DataTable into a MySQL table using C#?
A: To insert a DataTable into a MySQL table using C#, you can use the MySqlBulkCopy class, which is similar to the SqlBulkCopy class. It allows you to perform bulk inserts into MySQL tables using a DataTable. You need to establish a connection to the MySQL server and execute the bulk copy operation.
Q: Can I insert a List into an SQL Server table using C#?
A: Yes, you can insert a List into an SQL Server table using C#. One way to achieve this is by converting the List to a DataTable and then using the methods mentioned earlier to insert the data into the SQL table. Alternatively, you can loop through the List and execute individual SQL insert statements for each item in the List.
Q: How can I update a DataTable in SQL Server using C#?
A: To update a DataTable in SQL Server using C#, you can use the SqlDataAdapter class, which provides methods to update data in a DataTable and synchronize the changes with the corresponding SQL table. You need to define the necessary SQL update queries and specify the update command for the SqlDataAdapter.
Q: Is it possible to insert data into multiple SQL tables using a single DataTable in C#?
A: Yes, it is possible to insert data into multiple SQL tables using a single DataTable in C#. You need to set up the appropriate SQL queries and use the appropriate techniques to execute multiple insert operations, such as using transactions or batch execution.
Q: What is the benefit of using bulk copy for inserting a DataTable into an SQL table?
A: The benefit of using bulk copy for inserting a DataTable into an SQL table is that it provides a faster and more efficient way to insert large amounts of data compared to individual insert statements. Bulk copy operations minimize the round trips to the database and optimize the data transfer process, resulting in improved performance.
In conclusion, inserting a DataTable into an SQL table using C# is a straightforward process that involves establishing a connection to the SQL server, creating an SQL query, and executing it to insert the data. By using bulk copy operations, you can achieve faster and more efficient data insertion, especially for large datasets.
C# Insert Data Into Sql Table – How To Save C# Data Table Into Sql Server Table
How To Insert Datatable To Sql Table?
Working with data is an integral part of any business or application. Often, we need to deal with datasets stored in memory as DataTables, and eventually, we may need to insert this data into a SQL Server database table. In this article, we will guide you through the process of inserting a DataTable into a SQL table, highlighting the necessary steps and important considerations.
Table of Contents:
1. Introduction to DataTable and SQL Table
2. Establishing a Connection to the SQL Server Database
3. Creating a SQL Table
4. Mapping DataTable Columns to SQL Table Columns
5. Inserting the DataTable into the SQL Table
6. Error Handling and Performance Considerations
7. FAQs
a. Can I insert a DataTable into multiple SQL tables simultaneously?
b. Is it possible to insert a DataTable into SQL tables in a different server?
c. Can I insert a DataTable into a temporary SQL table?
d. Are there any limitations on DataTable size when inserting into a SQL table?
1. Introduction to DataTable and SQL Table:
A DataTable is an in-memory representation of a database table. It holds data in rows and columns, with each column having a corresponding data type. On the other hand, a SQL table is a structured object within a database that stores data in rows and columns, persisted on disk. Both DataTable and SQL table share similar characteristics, making it relatively easier to transfer data between them.
2. Establishing a Connection to the SQL Server Database:
Before working with a SQL server database, establishing a connection is crucial. You need to gather the necessary connection details such as server name, database name, authentication method (Windows or SQL Server), username, and password. Utilizing ADO.NET or Entity Framework, establish a connection to the SQL server database using the provided connection details.
3. Creating a SQL Table:
To insert a DataTable into a SQL table, you must first have a SQL table with corresponding columns. Use SQL Server Management Studio or any SQL script execution tool to create a table. Ensure the column names, data types, and constraints (if any) align with the structure of the DataTable.
4. Mapping DataTable Columns to SQL Table Columns:
The next step involves mapping the columns of the DataTable to the columns of the SQL table. Iterate through the DataColumn collection of the DataTable, retrieve the column names, and match them with the respective column names in the SQL table. This mapping is crucial as it helps establish an appropriate insertion mechanism.
5. Inserting the DataTable into the SQL Table:
After establishing the connection, creating the SQL table, and mapping the columns, it’s time to insert the DataTable into the SQL table. Loop through each DataRow in the DataTable and format the SQL query to insert the data row into the SQL table. Utilize SQL parameters to ensure data integrity and prevent SQL injection attacks.
6. Error Handling and Performance Considerations:
During the data insertion process, it is essential to handle any potential errors that may occur. Implement suitable error handling mechanisms to log or notify about any failures encountered during insertion. Additionally, consider optimizing the performance by utilizing bulk insert operations, such as SqlBulkCopy or Table-Valued Parameters, which significantly improve the efficiency of inserting large DataTables into SQL tables.
7. FAQs:
a. Can I insert a DataTable into multiple SQL tables simultaneously?
Technically, you cannot directly insert a DataTable into multiple SQL tables simultaneously. However, you can execute multiple insert statements within a transaction, ensuring data consistency across tables.
b. Is it possible to insert a DataTable into SQL tables in a different server?
Yes, it is possible to insert a DataTable into SQL tables located on a different server. You need to establish a connection to the target server and specify the fully qualified table names to indicate the target database and schema.
c. Can I insert a DataTable into a temporary SQL table?
Yes, like any other SQL table, you can insert a DataTable into a temporary SQL table. Ensure you create the temporary table with the desired schema beforehand, and use it as the target table for data insertion.
d. Are there any limitations on DataTable size when inserting into a SQL table?
The maximum size of a DataTable is dictated by the available system memory. However, when inserting into a SQL table, the limitations come from SQL Server itself. Ensure you consider the maximum row size, number of columns, and other configurable factors to prevent potential data truncation or performance issues.
In conclusion, inserting a DataTable into a SQL table requires establishing a connection, creating a SQL table, mapping columns, and finally inserting the data using appropriate SQL statements. Implementing error handling mechanisms and optimizing performance significantly contribute to a seamless insertion process. By following the steps outlined in this article, you will be equipped to handle such scenarios efficiently, ensuring data integrity and consistency.
How To Insert A Datatable Into Sql Server Database Table C#?
When working with database applications, it is common to encounter scenarios where data needs to be transferred between different sources. One such scenario involves inserting the data stored in a DataTable object into a SQL Server database table. In this article, we will discuss in depth how to achieve this task using C#.
Before we dive into the implementation details, let’s quickly understand the concept of DataTable and SQL Server database tables.
What is a DataTable?
A DataTable is an in-memory representation of a table, holding rows and columns of data. It belongs to the System.Data namespace and provides various methods and properties to manage and manipulate the data. DataTables offer a convenient way to store and process data retrieved from different sources.
What is SQL Server database table?
A SQL Server database table represents a collection of structured data organized in rows and columns. It is used to store and manage data in a structured manner, offering efficient querying and manipulation capabilities. SQL Server, developed by Microsoft, is one of the most popular relational database management systems used worldwide.
Inserting a DataTable into a SQL Server database table using C#
To insert a DataTable into a SQL Server database table using C#, we will follow these steps:
1. Establish a connection to the SQL Server database:
First, establish a connection to the SQL Server database using a SqlConnection object. Pass the connection string as a parameter to the constructor of the SqlConnection class. The connection string contains information about the database server, database name, and authentication credentials.
2. Create a new SqlCommand object:
Next, create a new SqlCommand object by providing the SQL query to insert data into the table. The query will include the table name and column names where the data will be inserted. The query can also use parameterized queries to prevent SQL injection attacks and provide more flexibility when inserting data.
3. Iterate through the rows of the DataTable:
Now, loop through each row of the DataTable and retrieve the required data using the column names. Assign the retrieved values to SqlParameter objects in the SqlCommand. SqlParameter objects help in preventing data type mismatch issues and any potential data manipulation attacks.
4. Execute the SQL command:
Finally, execute the SQL command using the ExecuteNonQuery method of the SqlCommand object. This method returns the number of rows affected by the SQL command. In the case of an insert operation, it will be 1 for each row inserted into the SQL Server database table.
5. Close the database connection:
Once the data insertion is completed, close the database connection using the Close or Dispose method of the SqlConnection object. Closing the connection is essential to efficiently utilize database resources.
FAQs:
Q1. Can I insert a DataTable with different column names into a SQL Server database table?
A1. Yes, you can insert a DataTable with different column names into a SQL Server database table using column mappings. Create a SqlBulkCopy object and set the ColumnMappings property to map the DataTable column names with the database table column names.
Q2. Is it possible to insert a large DataTable efficiently?
A2. Yes, you can efficiently insert a large DataTable into a SQL Server database table using bulk copy operations. Use the SqlBulkCopy class provided by the System.Data.SqlClient namespace. Set the DestinationTableName property with the target table name and call the WriteToServer method to insert the entire DataTable in a single database operation.
Q3. Can I insert multiple DataTables into different tables within a single database transaction?
A3. Yes, you can insert multiple DataTables into different tables within a single database transaction. Begin a transaction using the BeginTransaction method of the SqlConnection object, and then associate the transaction with each SqlCommand object using the Transaction property. Finally, commit the transaction using the Commit method or roll back using the Rollback method based on the success or failure of the insertion process.
In conclusion, inserting a DataTable into a SQL Server database table using C# is a straightforward process. By following the steps mentioned above and considering the FAQs provided, you can efficiently transfer data from a DataTable object to a SQL Server table. This approach provides flexibility, security, and performance, making it a valuable skill for any C# developer working with databases.
Keywords searched by users: insert datatable to sql table c# C# insert DataTable into SQL table, Insert DataTable to database C#, Vb net insert DataTable into SQL table, C# insert datatable into mysql table, Insert data to sql C#, Update datatable to SQL, Insert List to SQL Server C#, Bulk copy DataTable to SQL Server C#
Categories: Top 75 Insert Datatable To Sql Table C#
See more here: nhanvietluanvan.com
C# Insert Datatable Into Sql Table
Introduction:
In the world of software development, data manipulation is an essential aspect of any application. One common task developers often face is the need to insert a DataTable into a SQL table. This task can be achieved easily using the C# programming language. In this comprehensive guide, we will explore the steps required to insert a DataTable into a SQL table using C# and address some frequently asked questions related to this topic.
Step 1: Create a DataTable in C#:
The first step is to create a DataTable in C#. A DataTable represents a tabular structure to hold data. To create a DataTable, use the following code snippet:
“`csharp
DataTable dataTable = new DataTable(“TableName”);
“`
In the above code snippet, “TableName” represents the name you want to assign to the DataTable. You can define columns and constraints for the DataTable as per your requirements. For instance, to add a column to the DataTable, use the following code snippet:
“`csharp
dataTable.Columns.Add(“ColumnName”, typeof(DataType));
“`
Replace “ColumnName” with the desired column name and “DataType” with the appropriate .NET data type, such as int, string, DateTime, etc. Repeat this step for all the columns you want to add to the DataTable.
Step 2: Populate the DataTable:
Once the DataTable is created, the next step is to populate it with data. There are various ways to accomplish this, depending on the source of data. Here, we will assume the data is available in a CSV file. To read data from a CSV file and populate the DataTable, follow these steps:
1. Import the necessary namespaces to handle file operations:
“`csharp
using System.IO;
using System.Data;
“`
2. Declare a method to read data from the CSV file:
“`csharp
private void ReadDataFromCSVFile(string filePath, DataTable dataTable)
{
var lines = File.ReadAllLines(filePath);
if (lines.Length > 0)
{
var columnHeaders = lines[0].Split(‘,’);
foreach (var header in columnHeaders)
{
dataTable.Columns.Add(header);
}
for (int i = 1; i < lines.Length; i++) { var data = lines[i].Split(','); var row = dataTable.NewRow(); for (int j = 0; j < columnHeaders.Length; j++) { row[j] = data[j]; } dataTable.Rows.Add(row); } } } ``` 3. Call the method to read from the CSV file and populate the DataTable: ```csharp ReadDataFromCSVFile("Path/To/Your/File.csv", dataTable); ``` Replace "Path/To/Your/File.csv" with the actual file path where your CSV file is located. Step 3: Insert the DataTable into a SQL Table: Now that you have a populated DataTable, the final step is to insert it into a SQL table. To accomplish this, follow these steps: 1. Establish a connection to the SQL server: ```csharp using System.Data.SqlClient; ``` ```csharp string connectionString = "Data Source=YourServerName;Initial Catalog=YourDatabaseName;User ID=YourUsername;Password=YourPassword"; SqlConnection connection = new SqlConnection(connectionString); connection.Open(); ``` Replace "YourServerName", "YourDatabaseName", "YourUsername", and "YourPassword" with your SQL server information. 2. Prepare the SQL command to insert the DataTable into the SQL table: ```csharp string sqlQuery = "INSERT INTO TableName (Column1, Column2, Column3) VALUES (@Value1, @Value2, @Value3)"; SqlCommand command = new SqlCommand(sqlQuery, connection); foreach (DataRow row in dataTable.Rows) { command.Parameters.Clear(); command.Parameters.AddWithValue("@Value1", row["Column1"]); command.Parameters.AddWithValue("@Value2", row["Column2"]); command.Parameters.AddWithValue("@Value3", row["Column3"]); command.ExecuteNonQuery(); } ``` Replace "TableName" in the SQL query with the actual table name where you want to insert the data. Also, ensure that the column names specified in the SQL query match the columns present in the DataTable. 3. Close the database connection: ```csharp connection.Close(); ``` FAQs: Q1: Can I insert a DataTable into multiple SQL tables simultaneously? A1: Yes, you can insert a DataTable into multiple SQL tables simultaneously by modifying the SQL query in Step 3. Instead of a single INSERT INTO statement, you can write multiple INSERT INTO statements within a single SQL command. Q2: What if the column names in my DataTable and SQL table differ? A2: In such cases, you need to map the DataTable column names to the corresponding SQL table column names. Modify Step 3 where the SQL command is prepared to use the mapped column names. Q3: How can I handle errors during the insertion process? A3: You can include error handling mechanisms like try-catch blocks around the code in Step 3. Within the catch block, you can log the error details or perform any required error handling tasks. Q4: Can I use a DataTable with a different structure than the target SQL table? A4: Yes, you can use a DataTable with a different structure than the target SQL table. However, you need to handle the mapping of columns and data types appropriately in Step 2. Q5: Are there any performance considerations when inserting large DataTables into SQL tables? A5: When inserting large DataTables, consider using bulk insert operations or optimizing the SQL table for improved performance. Additionally, you may need to batch insert the data instead of inserting it row by row. Conclusion: In this comprehensive guide, we explored the steps required to insert a DataTable into a SQL table using C#. By following these steps, you can effortlessly insert data from a DataTable into a SQL table and perform data manipulation efficiently. Additionally, we addressed some frequently asked questions related to this topic, providing you with a better understanding of this process and potential challenges you may encounter.
Insert Datatable To Database C#
Introduction:
When working with data in C#, there are often situations where we need to insert a DataTable into a database. This article will guide you through the process of inserting a DataTable into a database using C#, covering various aspects, including step-by-step procedures, code examples, and potential challenges.
Step 1: Establish a Database Connection
The first step is to establish a connection with the database. In C#, we can make use of the ADO.NET library for all database-related operations. The SqlConnection class allows us to connect to a SQL Server database. Here’s an example of how to establish a database connection:
“`
string connectionString = “Data Source=YOUR_SERVER_NAME;Initial Catalog=YOUR_DATABASE_NAME;User ID=YOUR_USERNAME;Password=YOUR_PASSWORD;”;
SqlConnection connection = new SqlConnection(connectionString);
connection.Open();
“`
Make sure to replace `YOUR_SERVER_NAME`, `YOUR_DATABASE_NAME`, `YOUR_USERNAME`, and `YOUR_PASSWORD` with the appropriate values for your database.
Step 2: Create a DataTable
Next, we need to create a DataTable object that will store our data. Let’s assume that our DataTable contains three columns: “ID” (integer), “Name” (string), and “Age” (integer). Here’s an example of how to create a DataTable and populate it with sample data:
“`
DataTable dataTable = new DataTable();
dataTable.Columns.Add(“ID”, typeof(int));
dataTable.Columns.Add(“Name”, typeof(string));
dataTable.Columns.Add(“Age”, typeof(int));
// Add some sample data to the DataTable
dataTable.Rows.Add(1, “John Doe”, 25);
dataTable.Rows.Add(2, “Jane Smith”, 30);
“`
Step 3: Bulk Copy the DataTable to the Database
Once we have our DataTable ready, we can use the SqlBulkCopy class to efficiently insert the data into the database. SqlBulkCopy is a high-performance way to transfer large amounts of data from one source to another.
Here’s an example of how to use SqlBulkCopy to insert the DataTable into the database:
“`
using (SqlBulkCopy bulkCopy = new SqlBulkCopy(connection))
{
bulkCopy.DestinationTableName = “YourTableName”;
bulkCopy.WriteToServer(dataTable);
}
“`
Make sure to replace “YourTableName” with the actual name of the table in your database where you want to insert the data.
Step 4: Close the Database Connection
Once we have inserted the DataTable into the database, it is always a good practice to close the database connection to free up resources. Here’s an example of how to close the connection:
“`
connection.Close();
“`
FAQs:
Q1: Can I insert a DataTable into a database without using SqlBulkCopy?
A1: Yes, you can insert a DataTable into a database without using SqlBulkCopy. However, using SqlBulkCopy is the most efficient and recommended approach for large amounts of data.
Q2: Can I insert a DataTable into a database other than SQL Server?
A2: Yes, you can insert a DataTable into other databases like Oracle, MySQL, etc. The process may vary slightly based on the database provider you are using.
Q3: How can I handle errors during the bulk insert operation?
A3: SqlBulkCopy provides an event called “SqlRowsCopied” which can be used to handle errors or exceptions that occur during the bulk insert operation. You can subscribe to this event and handle any exceptions as per your requirements.
Q4: Is there a limit on the number of rows that can be inserted using SqlBulkCopy?
A4: The maximum number of rows that can be inserted using SqlBulkCopy depends on the database and server settings, as well as the available system resources. It is advised to perform bulk inserts in smaller batches to avoid any performance or resource-related issues.
Conclusion:
In this article, we explored how to insert a DataTable into a database using C#. We discussed the necessary steps involved, including establishing a database connection, creating a DataTable, using SqlBulkCopy for efficient insertion, and closing the connection. Additionally, we addressed some frequently asked questions related to this topic. By following these guidelines, you can easily insert a DataTable into a database using C# for efficient and optimized data handling.
Vb Net Insert Datatable Into Sql Table
Introduction:
In modern programming, data manipulation and storage play a vital role in various application development scenarios. Visual Basic .NET (VB.NET) is a versatile programming language that allows developers to efficiently manage data through the use of DataTables. In this article, we will explore how to insert a DataTable into an SQL table using VB.NET, providing you with an in-depth understanding of this process.
Table of Contents:
1. Prerequisite Knowledge
2. Connecting to the SQL Database
3. Creating a DataTable
4. Inserting Data into the DataTable
5. Inserting the DataTable into an SQL Table
6. FAQs
a. How can I handle potential errors during the insertion process?
b. Can I insert a DataTable into multiple SQL tables simultaneously?
c. What are the advantages of using a DataTable for data manipulation?
1. Prerequisite Knowledge:
To make the most of this guide, a basic understanding of VB.NET programming and SQL databases is recommended. Familiarity with ADO.NET and how to establish a connection between VB.NET and an SQL database will be essential for successfully completing the insertion process.
2. Connecting to the SQL Database:
The first step is to establish a connection between your VB.NET application and the SQL database. This can be done using the SqlConnection class provided by the System.Data.SqlClient namespace. By specifying the connection string, you can connect to the SQL server, database, and credentials required for access.
3. Creating a DataTable:
To insert a DataTable into an SQL table, we need to create a corresponding DataTable object in VB.NET. This can be done using the DataTable class from System.Data namespace. You can define the columns and their data types using DataColumn objects, and add them to the DataTable. Additionally, you may specify other properties such as constraints or primary keys.
4. Inserting Data into the DataTable:
Next, populate the DataTable with data. This can be achieved by either manually adding rows to the DataTable using the NewRow method provided by the DataTable object, or retrieving data from external sources like an Excel spreadsheet, CSV files, or fetching data from a database using SQL queries. It is important to ensure that the data being added matches the defined structure of the DataTable.
5. Inserting the DataTable into an SQL Table:
Once the DataTable is populated, we can proceed with inserting the data into an SQL table. The SqlBulkCopy class from System.Data.SqlClient namespace is the most efficient method for bulk insertion of data. By initializing a new instance of SqlBulkCopy, mapping the DataTable columns to the database table columns, and defining the target table in the destination database, you can execute the bulk insert operation using the WriteToServer method.
6. FAQs:
a. How can I handle potential errors during the insertion process?
During the insertion process, it is crucial to anticipate potential errors to ensure data integrity. To handle errors, you can use try-catch blocks to catch exceptions that may occur during the insertion operation. By accessing the SqlBulkCopy class’s SqlRowsCopied event, you can monitor the number of successfully inserted rows and implement error logging or corrective actions if necessary.
b. Can I insert a DataTable into multiple SQL tables simultaneously?
Yes, VB.NET allows the insertion of a DataTable into multiple SQL tables simultaneously. Simply initialize multiple instances of the SqlBulkCopy class, each targeting a different SQL table, and map the DataTable columns to their respective destination table columns.
c. What are the advantages of using a DataTable for data manipulation?
Using a DataTable provides several advantages for data manipulation, especially when dealing with large datasets. It allows for in-memory storage, enabling faster data access and manipulation compared to directly manipulating the SQL database. DataTables also provide a rich set of methods for filtering, sorting, and performing calculations on data, making them highly flexible for various data operations.
Conclusion:
Inserting a DataTable into an SQL table using VB.NET is a powerful feature that simplifies the process of bulk data insertion and manipulation. By establishing a database connection, creating a DataTable, populating it with data, and utilizing the SqlBulkCopy class, developers can efficiently insert large datasets into SQL tables. Remember to handle exceptions and errors gracefully to ensure data integrity throughout the process. With these steps and best practices in mind, you’ll be equipped to leverage VB.NET’s capabilities for seamless data management in your applications.
Images related to the topic insert datatable to sql table c#
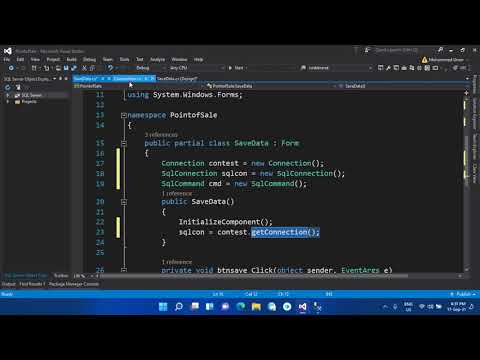
Article link: insert datatable to sql table c#.
Learn more about the topic insert datatable to sql table c#.
- How to insert a data table into SQL Server database table?
- How to INSERT new datatable into a SQL table or UPDATE data if it …
- Bulk Insert In SQL Server From C# – C# Corner
- How to insert a data table into SQL Server database table?
- Insert Data Into The Database In Windows Form Using C#
- How to add Data to DataTable and to SQL Table using C# …
- C# How to insert a data table into SQL Server database table?
- [C#] Hướng dẫn insert nguyên datatable xuống database …
- Bulk Insert In SQL Server From C# – C# Corner
- Insert DataTable into SQL Table in C# – MorganTechSpace
- Insert (Save) DataTable to SQL Server Table using C# and VB …
- How to insert a data table into SQL Server database table?
- Insert dataset or datatable data to sql table using sql bulk copy …